Mass texting
Build a mass texting service to make bulk text messaging easier. Twilio’s reliable Programmable Messaging API can scale to handle your largest messaging use cases, globally.
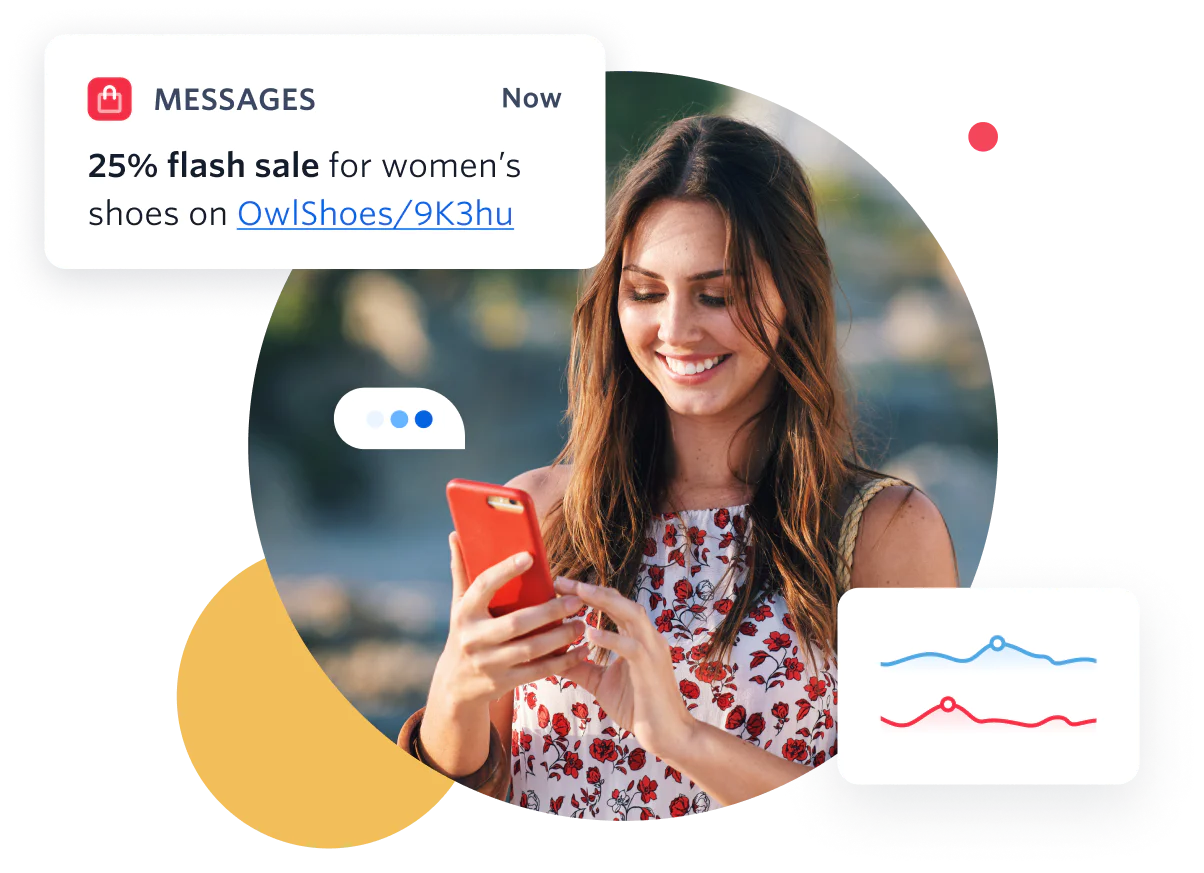
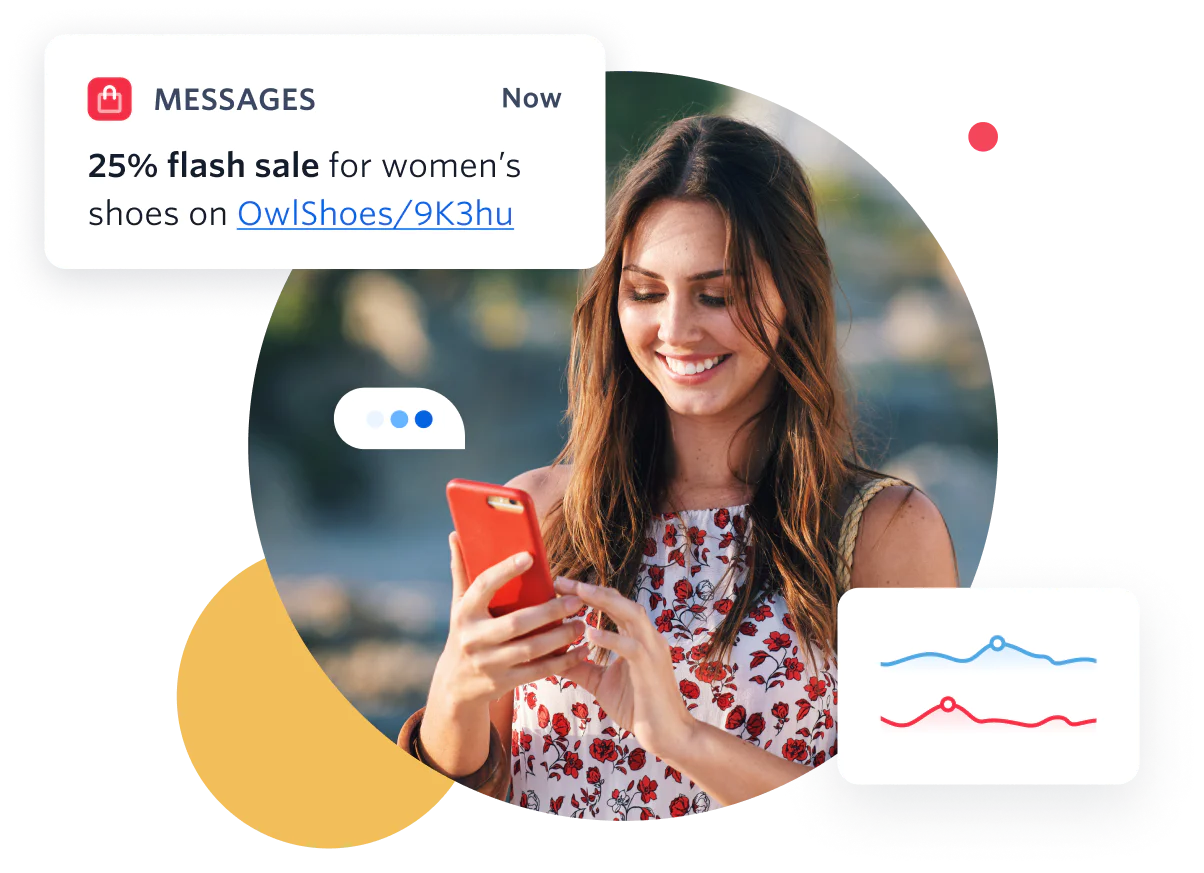
Build a mass texting service to make bulk text messaging easier. Twilio’s reliable Programmable Messaging API can scale to handle your largest messaging use cases, globally.
Bulk SMS or MMS, mass notifications, mass texting—it’s all a way of saying you’re sending a lot of messages to your audience at once. The occasion might be a promotional message for Black Friday, an alert about a regional weather event, or a school cancellation notice to parents.
Twilio APIs are easy to integrate with your current tech stack, including your Customer Data Platform (CDP) and CRM. Build and deploy your bulk texting use cases quickly and at scale.
Step 1
Set up your Twilio account
Sign up for a free Twilio account, then select from our inventory of business phone numbers with SMS capabilities to engage your audience or customers.
Step 2
Create and configure a messaging service
Utilize the default messaging service that’s automatically generated for your account, or create your own through the Twilio Console or using the REST API.
Step 3
Develop your mass texting solution
Build a bulk texting application in your choice of language including Python, Golang, PHP, Java, Ruby, C# / .NET, and Node.js, or use Twilio Notify to send messages at scale.
Step 4
Test your application
Test your queuing and sending capabilities are working correctly to ensure accurate and timely delivery of your bulk messages.
Step 5
Deploy with confidence
Now your solution is ready to roll. Launch your bulk texting communications and watch your messaging program thrive.
Step 6
Leverage data
Measure and optimize delivery with Twilio’s out-of-the-box reporting from Messaging Insights or customize reporting with data from CSV downloads, webhooks, and Event Streams.
The Twilio Customer Engagement platform has text messaging solutions for maximum deliverability at scale—we send and receive 167B+ messages per year.
Sign up for a Twilio account to start sending text messages at scale. Use our quickstart guides and up-to-date docs to get set up using your preferred programming language.
Programmable Messaging Quickstart
Get a step-by-step guide for setting up your Twilio account, and sending and receiving your first messages.
Sending bulk SMS with Twilio and Node.js
Learn how to scale from a small use case to a mass texting use case as your subscriber list grows.
Understanding rate limits and message queues
Learn about the wireless carrier network limits for message delivery and how Twilio handles your messages requests to navigate limitations.
You can use Twilio messaging for your bulk texting solution even if you don’t have coding experience to build it yourself. Our trusted partners can help you bring your texting solution to life.
2024 guide to scaling your SMS messaging solution
Read up on everything you’ll need to consider for your mass texting use case to set yourself up for success.
Send SMS notifications from Google Sheets
Learn how to create a custom menu in Google Sheets to send SMS notifications to your contacts.
Twilio’s reliable communications APIs delivered a 3% increase in message delivery versus other providers and 132% ROI for customers(1).
Twilio is loved by 10+ million developers and is ready to handle your biggest workloads.
In 2023, over 830 million messages were sent and received by Twilio on Cyber Monday and we sent over 4 billion messages during Cyber Week—with zero down time in core messaging services.
Twilio reaches over 180 countries to connect you with customers around the world. The Super Network has over 4,800 global carrier connections and monitors routes for latency and outages to optimize delivery rates.
No-shenanigans pricing means you only pay for what you use. You can scale up or down as needed, and you’ll unlock pricing discounts as your usage increases.
We’re here to help you meet carrier requirements for sending text message traffic. From our vetted phone number inventory of more than 200 number types to our simplified registration process, you can join the trusted messaging ecosystem and keep your communications compliant.
Related use cases
FAQs
Businesses can use mass texting for use cases like: sending security notifications, emergency alerts, reminders, feedback requests, or special marketing offers to a large audience at once.
Mass texting simplifies the work of sending individual messages to separate recipients.
Bulk messages can be targeted and personalized to the audience. We recommend segmenting your recipients and sending tailored messages to increase the effectiveness of campaigns such as for marketing and promotions.
At Twilio, the cost of a mass texting solution depends on how many messages you send. We use pay-as-you-go pricing with volume discounts available. So the more you send, the more you save.
View Twilio’s SMS pricing for full cost details. There may be additional carrier fees for some markets, countries, and carriers.
Group texting refers to an individual sending a message to a group of selected recipients. Some smartphone models now allow users to send a single message to up to 30 recipients, while others max out at 10.
Mass texting, on the other hand, refers to messages sent with a text messaging API or SMS service that delivers texts to a list of recipients. The list can include as many people as a group message or up to millions of recipients.
You can send mass text messages to existing customers if they have opted in to receive those specific kinds of text messages from you.
If you do not have explicit consent from existing customers, you’ll need to get it before you can send them any text messages. You can ask for their permission through an email, over the phone, or at a point of sale with options for the types of messages they can choose to receive from you.
Once you have a customer’s opt-in, you can add them to your mass texting list and start communicating with them via text.
In general, mass texting is legal when you have explicit opt-in consent from recipients, you send messages from a verified number, you respect opt-out requests and you adhere to legal and regulatory requirements.
Due to the rise in spam messages and smishing scams in the past decade, there is additional scrutiny for mass texting and you need to take extra care to stay in compliance.
Review our Guide to US SMS Compliance for more information and be sure to convene with your legal counsel for understanding and complying with any industry or regional regulations.
A mass text is sent with an SMS API or an SMS service. Through your phone, you can send group messages to 10-30 recipients.
Learn more about Twilio’s SMS API for sending mass texts.
You can choose the number you’ll send your text messages from. The most common number types for mass texting are: short codes, toll-free numbers, and A2P 10DLC long codes, and alphanumeric numbers.
Twilio has a massive inventory of phone numbers to choose from for your mass texting use cases.
You can only send mass text messages to customers who have given opt-in permission. And more specifically, you can only send them the types of messages they’ve opted in to receive (such as shipping notifications). If you want to send recipients a marketing message, you need to get explicit opt-in for those types of messages as well.
If you don’t respect recipients’ opt-in preferences, you could be flagged as a non-compliant sender and carriers may not pass through your messaging traffic.
1. Forrester Consulting’s Total Economic ImpactTM (TEI) of Twilio Messaging report
2. Gartner® Critical Capabilities for Communications Platform as a Service, Lisa Unden-Farboud, Ajit Patankar, Brian Doherty, Daniel O’Connell, 18 September 2023. GARTNER is a registered trademark and service mark of Gartner, Inc. and/or its affiliates in the U.S. and internationally and is used herein with permission. All rights reserved. This graphic was published by Gartner, Inc. as part of a larger research document and should be evaluated in the context of the entire document.
The Gartner document is available upon request from Twilio. Gartner does not endorse any vendor, product or service depicted in its research publications and does not advise technology users to select only those vendors with the highest ratings or other designation.
Gartner research publications consist of the opinions of Gartner’s Research & Advisory organization and should not be construed as statements of fact. Gartner disclaims all warranties, expressed or implied, with respect to this research, including any warranties of merchantability or fitness for a particular purpose.