How to Send a WhatsApp Message in 30 Seconds with JavaScript
Time to read:
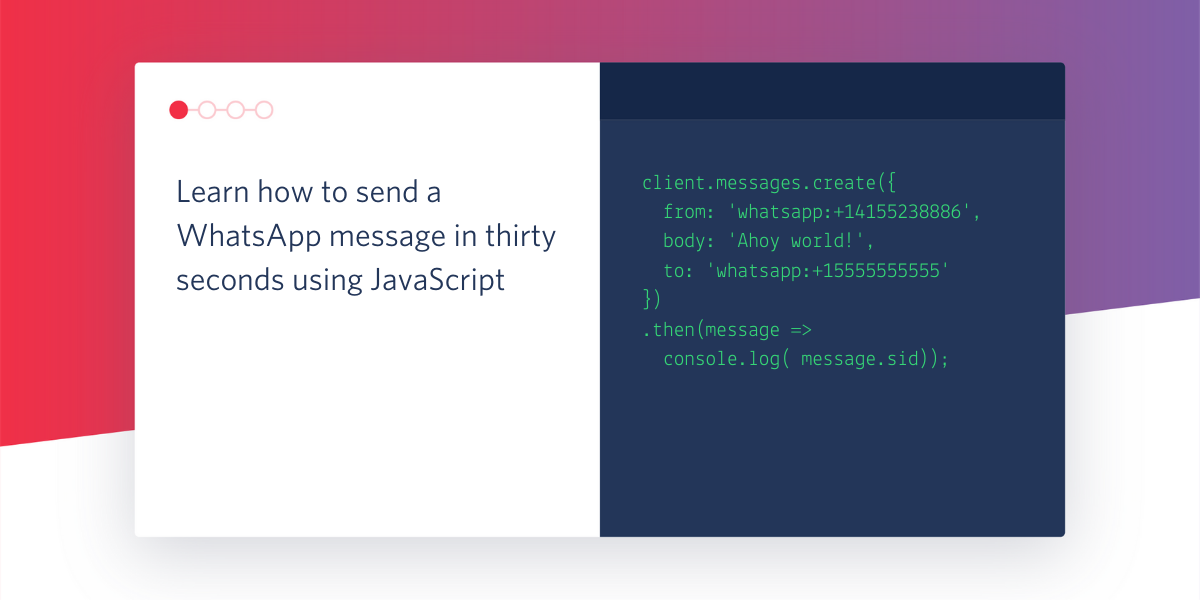
Just like Memphis Raines figured out how to steal fifty cars in one night, you’re going to learn how to send one WhatsApp message in thirty seconds (using JavaScript!).
Buckle up, cause here we go.
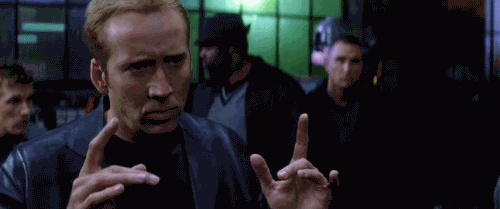
Prerequisites
- A free Twilio account (if you sign up with this link, we'll both get $10 in Twilio credit when you upgrade)
- Node.js and npm
Get your development environment setup
Create a new directory on your computer, change into it, and initialize a new Node.js project:
Install the Twilio Node Helper Library and the dotenv package:
Create two new files inside your new whatsapp directory:
Open the .env file and add two environment variables to store your Twilio Account SID and Twilio Auth Token. Leave the values for each variable blank for the moment, you’ll collect these credentials shortly.
Activate the Twilio Sandbox for WhatsApp
Head to the WhatsApp section of your Twilio Console to activate the Twilio Sandbox for WhatsApp. The sandbox lets you test out Twilio’s WhatsApp API using a shared, universal number without having to wait for your Twilio number to be approved by WhatsApp.
After checking the box to activate the sandbox, you’ll be asked to send a specific code message from your WhatsApp number to the provided universal WhatsApp number. After completing this connection, you’ll see this success screen:
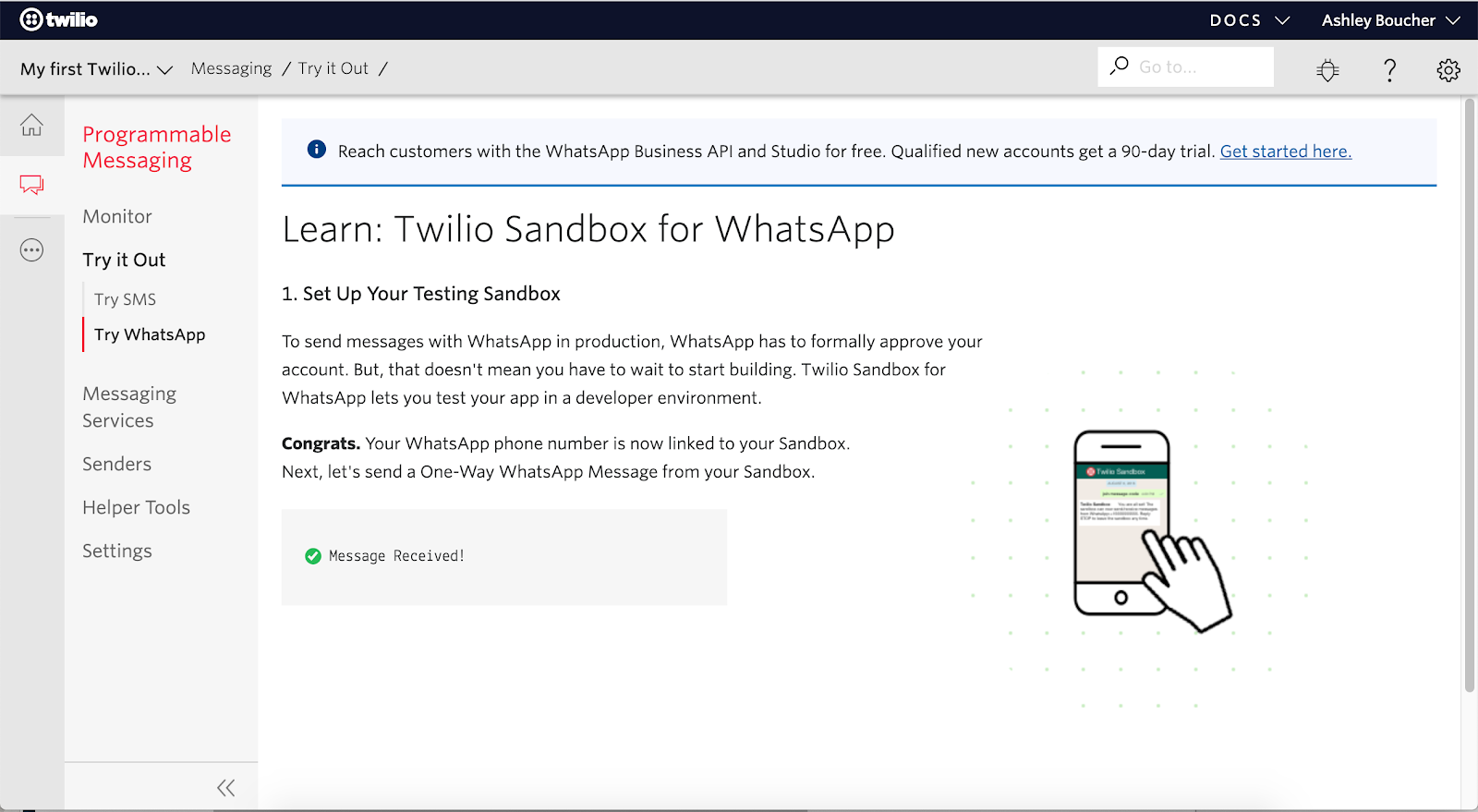
Write some code
Head to your main dashboard within the Twilio Console and find your Account SID and Auth Token. Copy and paste these values into the .env file you created earlier as the values for the variables TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
respectively.
Open up the index.js file you created earlier in your favorite text editor. Copy and paste the following code into this file:
The code above loads the Twilio library. Then it creates and sends a new message from the shared WhatsApp Sandbox number to your personal WhatsApp number.
Save your file.
Back in your command line, enter the following command to run your file and test out your work:
When the message has been sent, a success message will be logged to your command line, and you’ll receive a WhatsApp message that says “Ahoy world!”
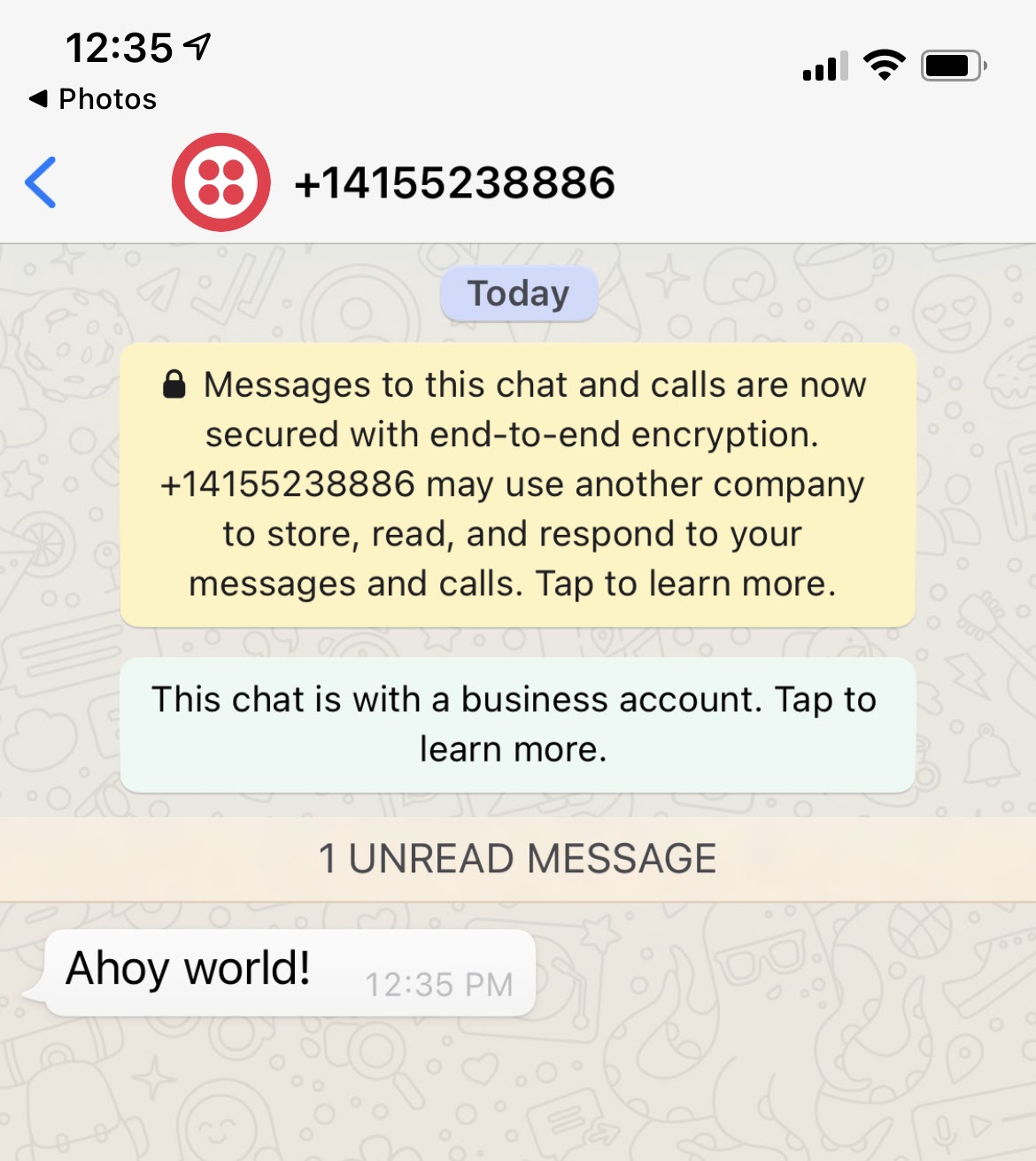
Next steps
Expand your new found JavaScript and WhatsApp skills by trying out another tutorial, like using location data in WhatsApp to find nearby healthy restaurants. Have fun, and let me know on Twitter what projects you’re building!
Ashley is a JavaScript Editor for the Twilio blog. To work with her and bring your technical stories to Twilio, find her at @ahl389 on Twitter. If you can’t find her there, she’s probably on a patio somewhere having a cup of coffee (or glass of wine, depending on the time).
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.