What’s In The Box!? Tracking What’s In Your Moving Boxes with Twilio SMS and Clarify.io
Time to read: 3 minutes
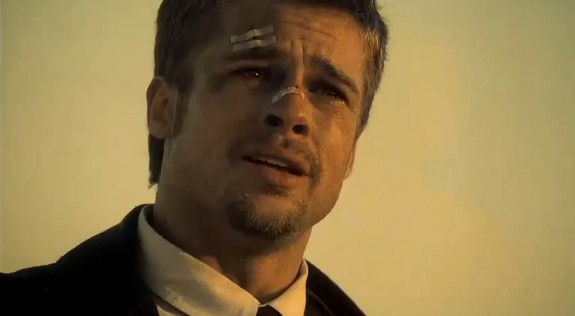
Nothing fragments your focus like moving. Even a simple task like boxing up picture frames can induce a nightmarish sense of dread. Once you finishing packing the picture frame box you’ll put it down atop the sea of other indistinguishable brown boxes, and realize you have absolutely no idea which box the frames are in. You have no idea which box anything is in. The anxiety is palpable, and also avoidable.
Graham Christensen, a developer at Clarify.io, put an app together using Twilio’s API and Clarify.io to help make his move from house to new house smoother.
Here’s how Graham conquered the “too many boxes” trap. As he packed he labeled his boxes 1, 2, 3, etc and took a voice recording of what was in the boxes. He then uploaded all the audio data to Clarify.io, an API for audio and video that allows you to programmatically search for media and add actions.
Using Clarify.io in conjunction with Twilio, Graham easily found his stuff by texting a Twilio number. If he was looking for his silverware, he’d text the number “silverware” and immediately receive a text back, telling him what box it was in.
Graham said he knew he’d lose track of what he packed in which box, hence the app. “I knew that there would be two things that would not be misplaced no matter what: my phone and the dog. Since the dog didn’t volunteer to help, I opted to use the phone,” Graham said.
Graham outlined the flow of the app and included code samples below so you can get building your own MoveApp:
My flow was relatively simple:
- First, I label all my boxes 1, 2, 3…
- Then, I call my Twilio number
- It prompts me for the box number which I type in and press #
- Then as I packed things, I simply said what it is.
Building MoveApp
This looks like a lot of moving parts, but there are only four scripts that matter. You can follow along from my Github repository but here are the details.
The first script –
start.php – receives the incoming call and prompts the user for a box number:
The second script – gather.php – receives the box number, starts the recording, and sets the action callback. The action callback is the most important part. This will make sure that once the recording is done on the Twilio side, the recording URL will be sent to the next script.
Note: By default, this will allow a recording of up to 3600 seconds (60 minutes) and only stop when you hang up or hit the # sign. Don’t forget to speak clearly!
The third script – save.php – catches the recording URL and the box number and submits it to Clarify for processing.
Note: Clarify will take roughly one minute of processing for every minute of audio. Since this only happens once, it’s not an issue.
The final script – search.php – receives the incoming text message, submits the search to Clarify, processes the results and responds with a simple text:
A Few Parting Words From Graham
I’m happy to say that I used this for my move last month. While there were still a lot of complexities with moving, the application worked flawlessly, and it turns out that I didn’t need to be that organized. After the move, finding everything was faster and easier than I could have imagined.
You can get and deploy all the code from my Github repository or just click the “Deploy to Heroku” button on that page, fill in your Clarify credentials, and then set your Twilio voice and SMS urls to point at the app. It will be the easiest part of your move.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.