Get SMS Notifications When Your Friends Send You Stellar
Time to read: 6 minutes
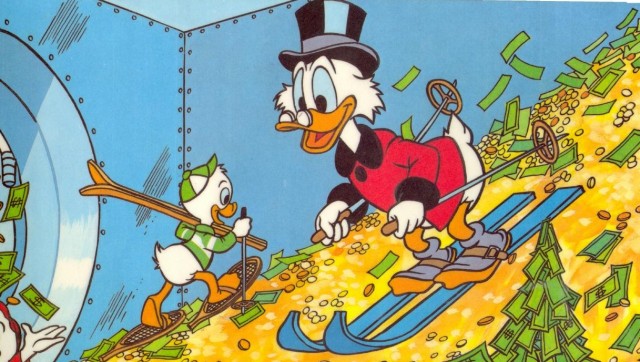
Yesterday the fine folks at Stripe brought to the world of digital currency yet another player with the announcement of the Stellar Organization, stewards of a new decentralized protocol for sending and receiving money in any pairs of currency and a new companion digital currency called the stellar (STR). This new protocol and currency, while having its own market determined value, is intended to provide a simpler path for converting between other currencies.
Never one to back away from a bandwagon, I immediately signed up and started playing with stellar, having a ton of fun exchanging the currency with friends and bugging my twitter network to send me stellars. As my friends and I continued, I thought, “Wouldn’t it be great to get an SMS message every time I received some stellar? If only there was an API for stellar that I could tap into”.
If only?!? Of course stellar has an API! There are APIs for everything: thermostats, coffee machines, lightbulbs, even cars. Why wouldn’t currency have an API too? In this post I’ll show you how to take the Stellar API, mix in a pinch of Node.js and a dash of Twilio SMS and create a simple stellar notification system. If you want to skip ahead, you can view the final code for our app here.
Lets get started!
Your Tools
Before you dive into the code, let’s make sure you have everything you need:
- A Stellar Account – this lets you send and receive STR. Good news, they give you 5000 STR when you register.
- Twilio SMS – sign up for a free Twilio account here.
- Node.js – a platform built on Chrome’s JavaScript runtime for easily building fast, scalable network applications.
Interaction With The Stellar API
You can interact with the Stellar API in 3 different ways: RPC, Command Line and Websockets. For this app we’ll be using Websockets but you can read about each method in the API documentation. Specifically, we’ll be using the node ws module. Let’s install it using npm:
Now let’s create a new file called app.js and initiate a connection to the Stellar API over Websockets:
While we’re here, let’s also add a variable containing our Stellar Address. You can find this in your Stellar dashboard. Just click receive money and then Show Your Stellar Address. We’ll be using this variable a bit later:
Now that we have that in place, we need to add a handler that will let us know that our connection is opened:
Let’s run our app and make sure we’re connecting successfully.
We should see the “ws connected” sent to the console. We’re now ready to subscribe to updates from the Stellar API. To do that we’ll be using the subscribe endpoint. We’re going to add some new code within the callback for when our Websocket is opened:
We first build out an object with the data we want to send the Stellar API. There are two pieces of information we’re sending, command and accounts. Since we want to subscribe to transactions we’re sending the command “subscribe”. Accounts lets us pass all the accounts we want to subscribe to in an array. In this case we just want to subscribe to our own updates so we’ll be using the stellarAddress variable we created earlier. We use JSON.stringify() to turn this object into a string as we send it to the Stellar API. This code will trigger once when our Websocket opens. After it runs, we’ll receive notifications for the length of our connection.
Before we run this code, we need to setup a way to receive the data we get back from the Stellar API. We’re going to add a new handler for the message event. This will get called whenever Stellar sends us a message indicating there has been a transaction on our account. Because all responses from the Stellar API are JSON, we’re going to parse the data and log it to our console:
Let’s try running our app again:
Now if someone sends you stellar you will see some information about that transaction logged into your console. You may notice that if you send someone stellar it will also get logged. Let’s add a basic conditional to only show transactions you receive:
We’re checking the JSON we receive from Stellar for 3 things:
- That the engine_result_code is 0 (aka success)
- That the message type is “transaction”
- That the transaction destination is your stellarAddress.
Now if you rerun the app you should only see the output when you’re on the receiving end of a payment.
Finally, for the sake of completeness let’s add some basic error handling when we send our data to the Stellar API:
A Wild SMS Message Appears!
We’re now able to programmatically determine when our friends are sending us stellar, but we probably don’t want to sit in front our terminal watching console output all day. Fortunately, we can use Twilio to send an SMS message when it happens. To do this we’ll be using the twilio node library. First, we’ll need to install the library using npm:
Next let’s initialize it at the top of our app.js file:
We’ll be using our Twilio accountSid and authToken to create an authenticated client for the Twilio REST API. You can find these both in your account dashboard.
Now that we have our tools in place, we can update our code to send an SMS message instead of outputting to console:
We’ll use the sendMessage function to send our SMS message. In the function, we pass the number we want to send to, the number we want send from and the body of our message. We’re using the msg.transaction.Amount to dynamically show how much stellar we’ve been sent. Since an amount of stellar is always represented in microstellars in the Stellar API, we have to divide by 1,000,000 to convert to STR. Next, we provide a callback for when the message has sent. In this case we’re just going to output the body, so we know the message sent successfully.
Let’s run our app:
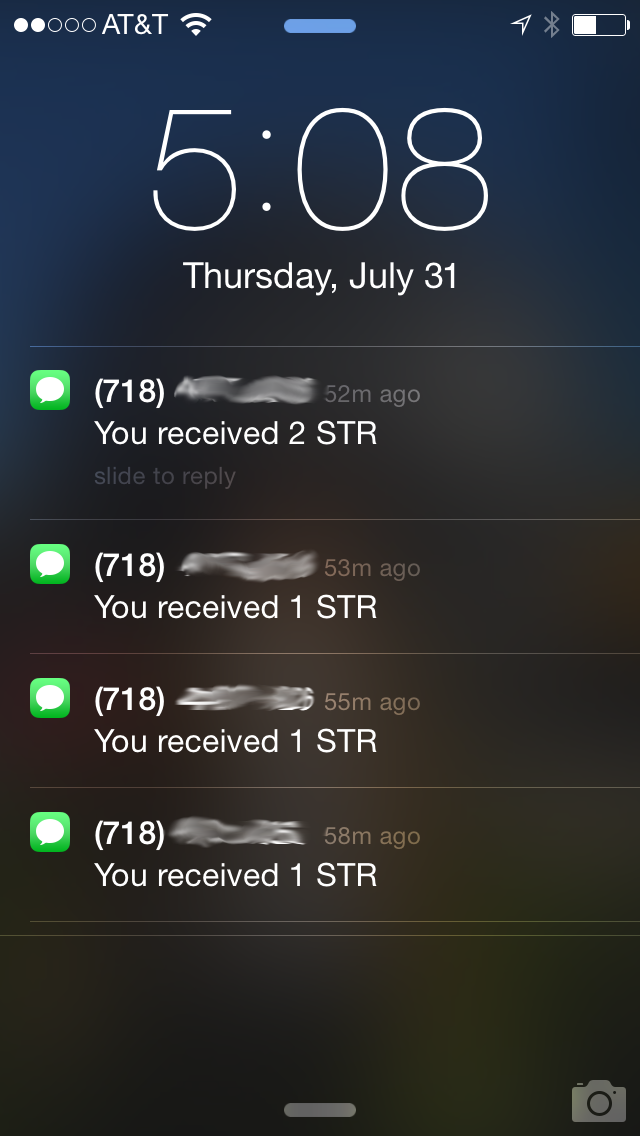
Look at that stellar roll in! Let’s do one last thing to help ensure your phone keeps buzzing as your friends share their stellar wealth with you. We’re going to keep this code running continuously using forever. First, let’s install forever:
You’ll notice we’re using the -g option to install this globally. This is required since we’ll be running forever from the command line.
Now that we’ve installed forever we can start our app:
Our app is now running continuously in the background and we don’t have to worry about missing any SMS messages as more and more stellar comes in.
Found that easy? Why don’t you try building another mashup with Twilio and the Stellar API? Maybe something to access your account info with a phone call or send stellar via SMS.
Tweet me a screenshot of your stellar SMS notifications + your Stellar username and I just may send you some stellar to make your phone buzz even more! Have questions? Just leave a comment below or drop me an e-mail.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.