Sensitive PII Information Detection in Email Sends using Azure AI, .NET and Twilio SendGrid
Time to read: 3 minutes
Sensitive PII Information Detection in Email Sends using Azure AI, .NET and Twilio SendGrid
Introduction
Accidentally sharing sensitive data in automated or manual emails can lead to data leaks. In this post, you’ll learn how to implement PII detection using Azure AI and .NET, then automatically mask confidential information before sending emails via Twilio SendGrid.
Prerequisites
In order to be successful in this tutorial, you will need the following:
- Free Azure account
- A free Twilio SendGrid account
- Visual Studio free Community Edition or Visual Studio Code
Building the app
The expected flow of the application is: when an email send is triggered, the app passes it to a PII detection service to identify and mask any sensitive data. The sanitized email is then sent to SendGrid to complete the sending process.
Setup Azure Text Analytics Resource
Log in to the Azure Portal then click on Create a resource as shown below:
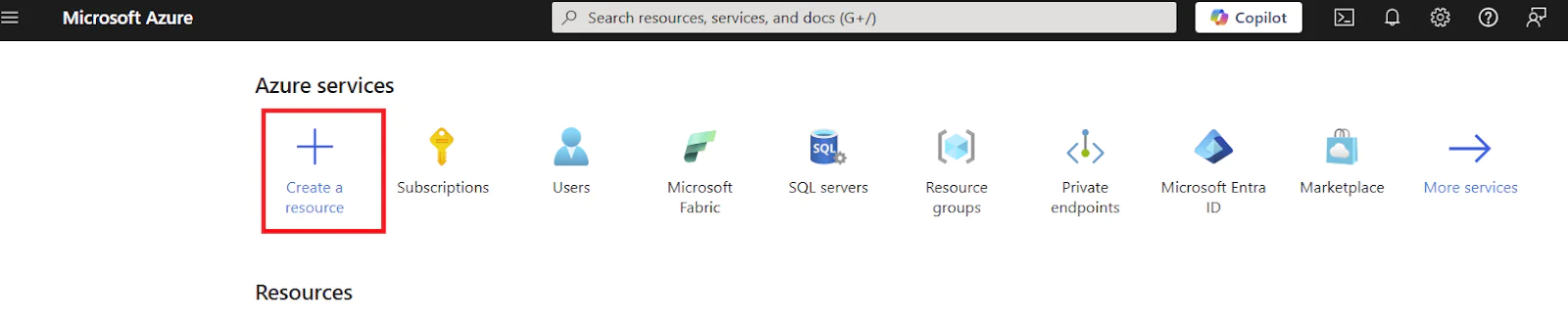
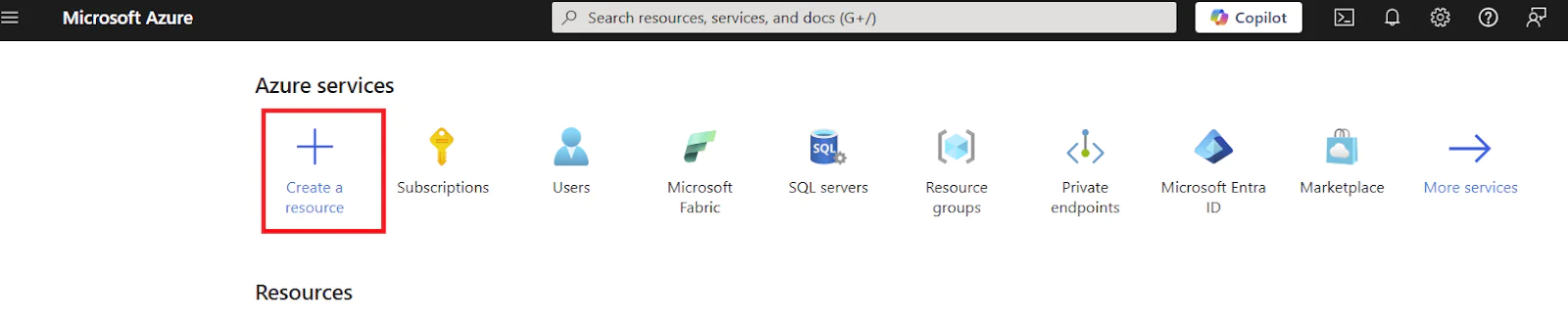
Search for “language” then click on Create for a Language service.
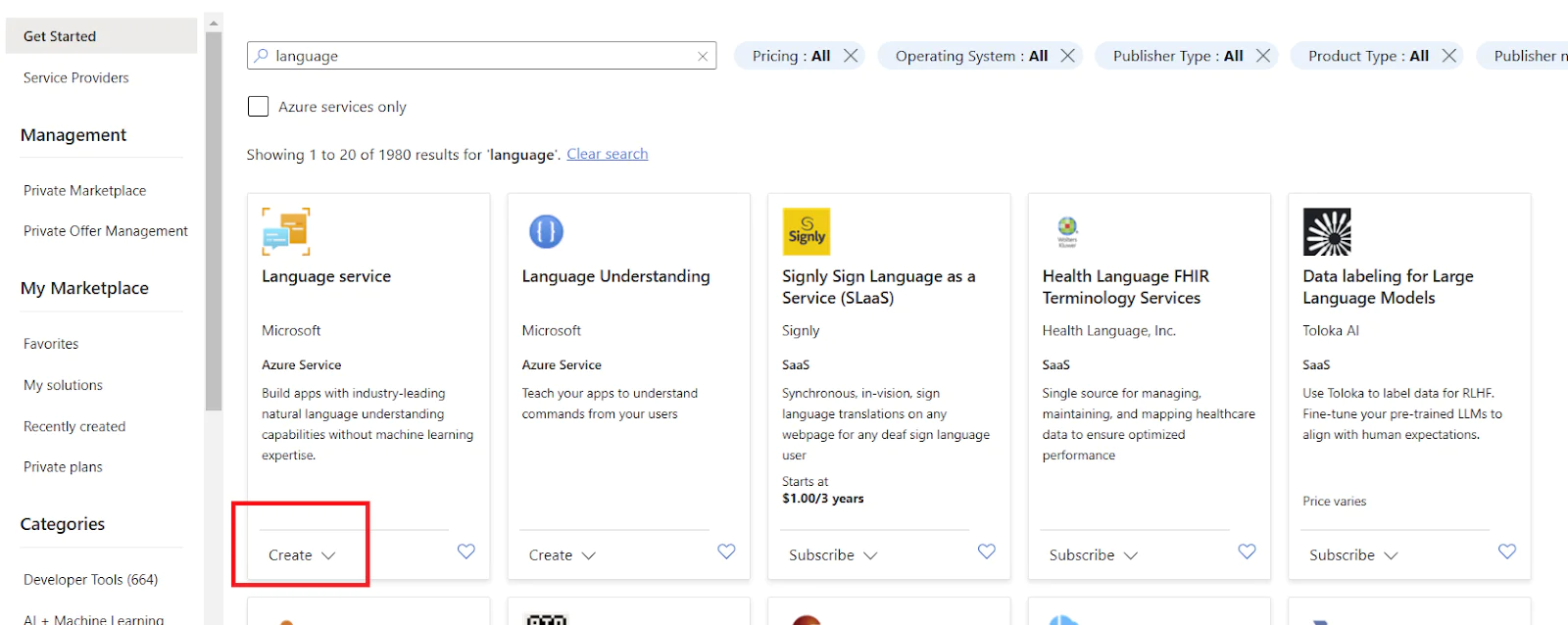
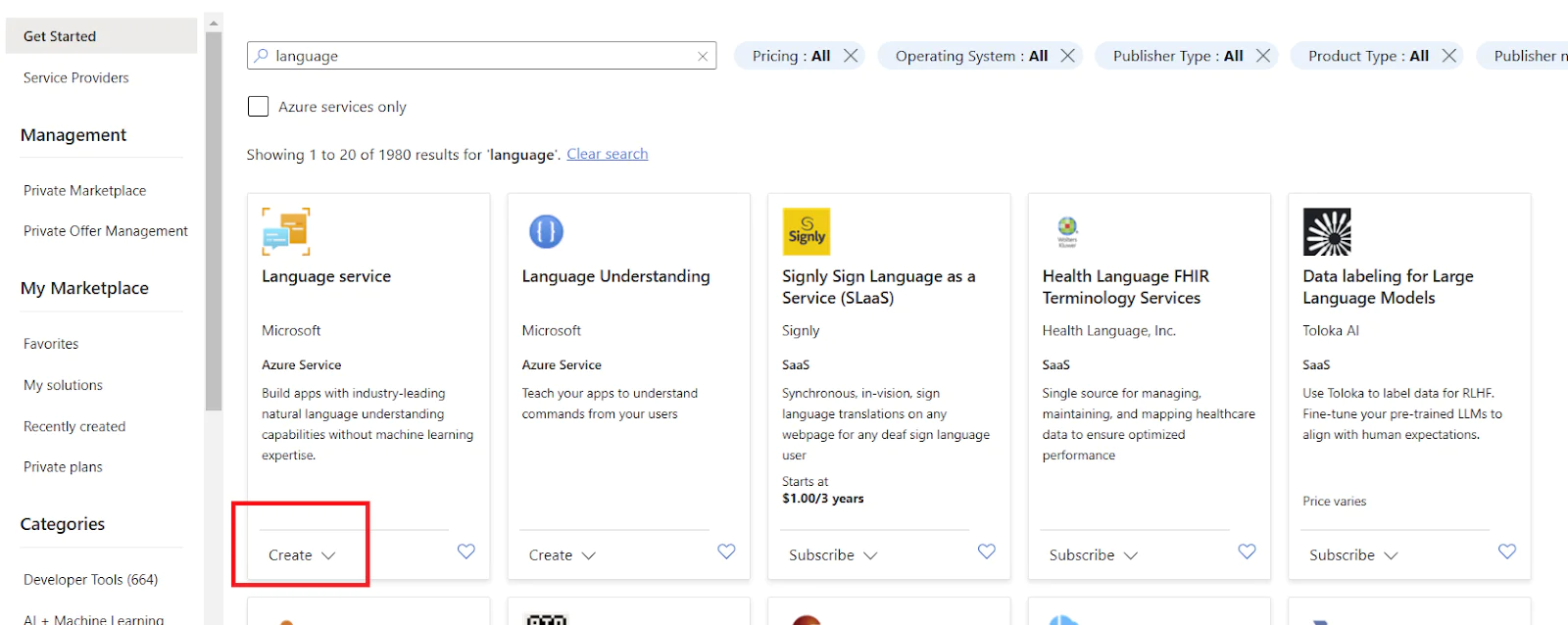
Then select Custom text classification, Custom named entity recognition, Custom sentiment analysis & Custom Text Analytics for health. Finally, click Continue to create your resource as shown below.
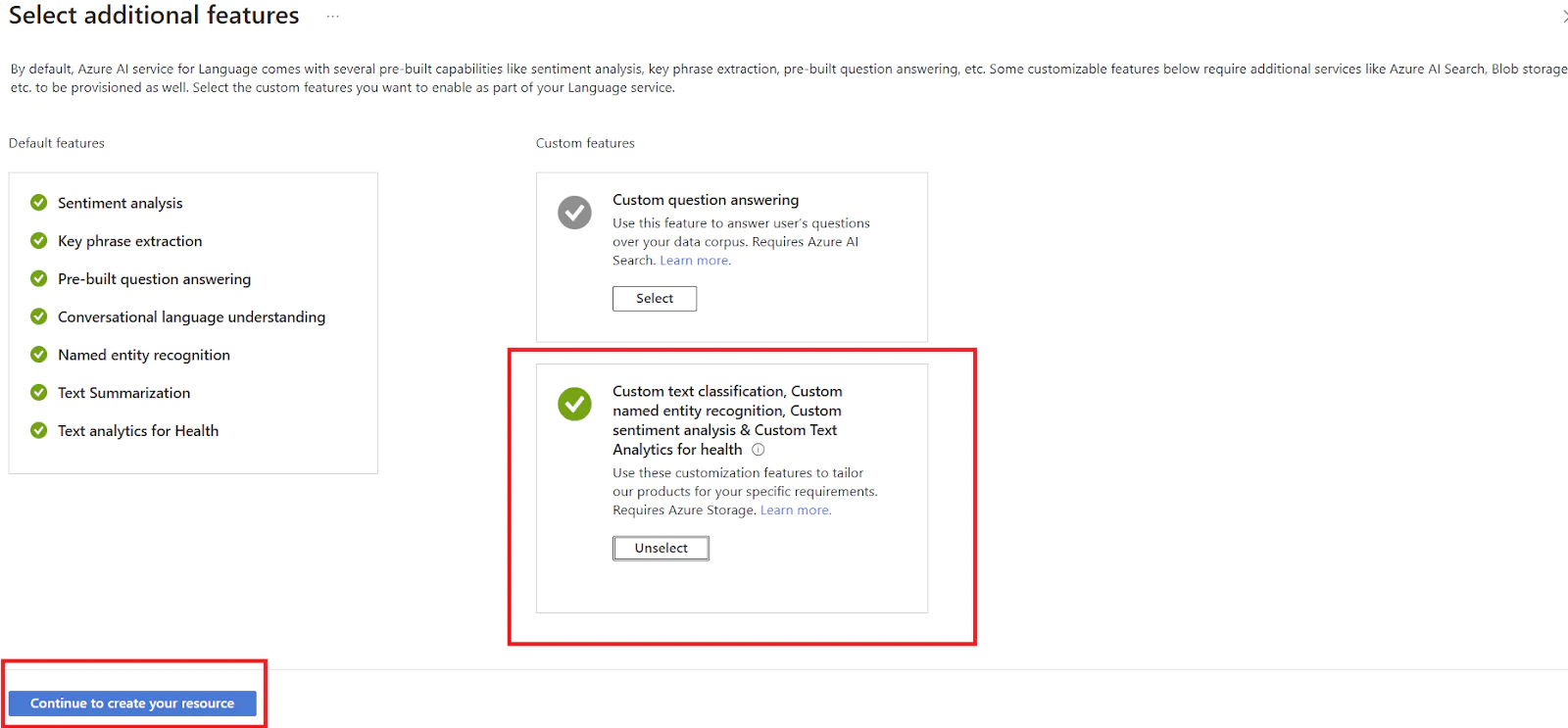
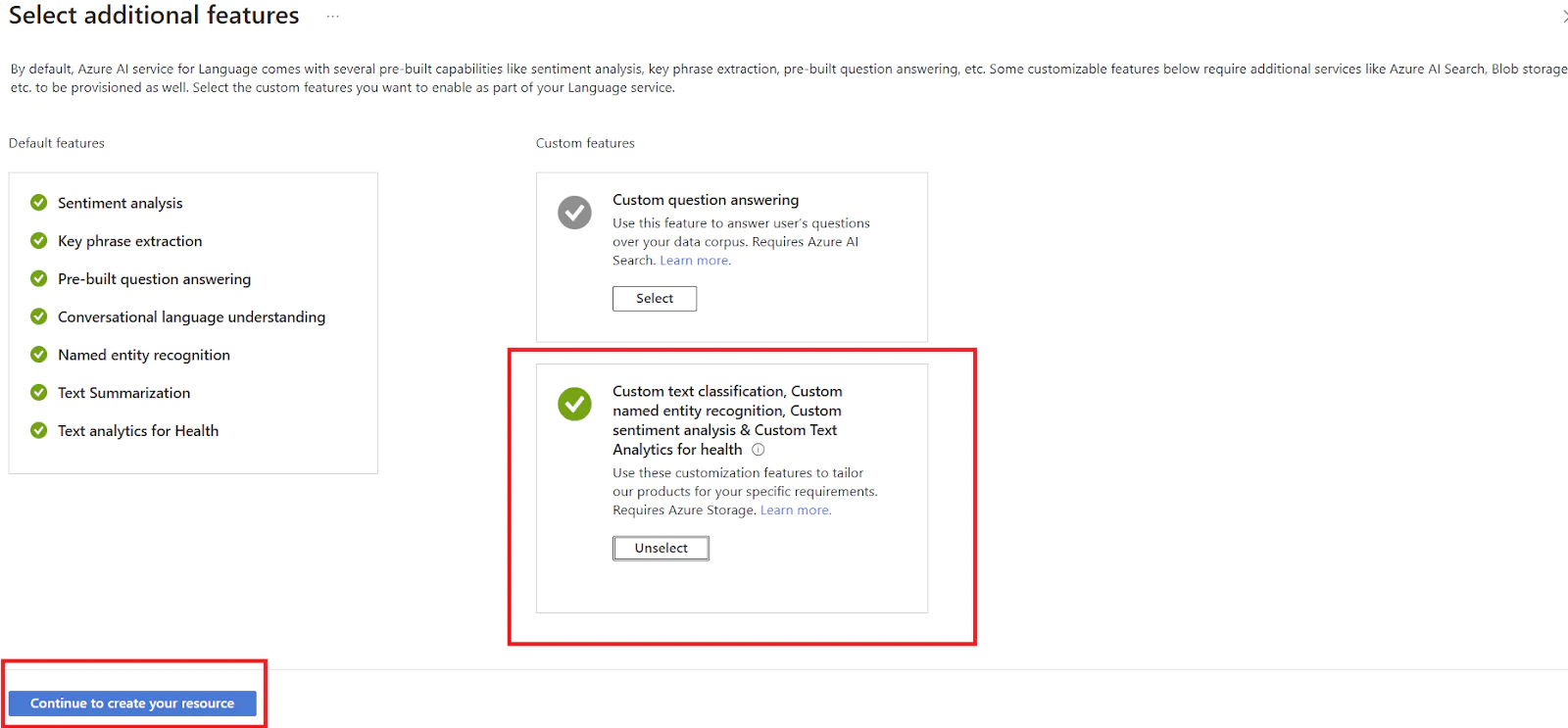
Select your Azure subscription and region you want to deploy your service. Add a name for the resource, then choose the free pricing plan, and the storage of type Standard ZRS as shown below.
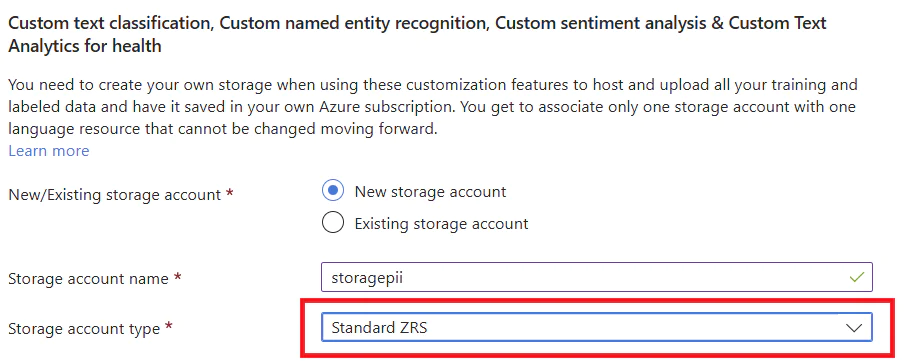
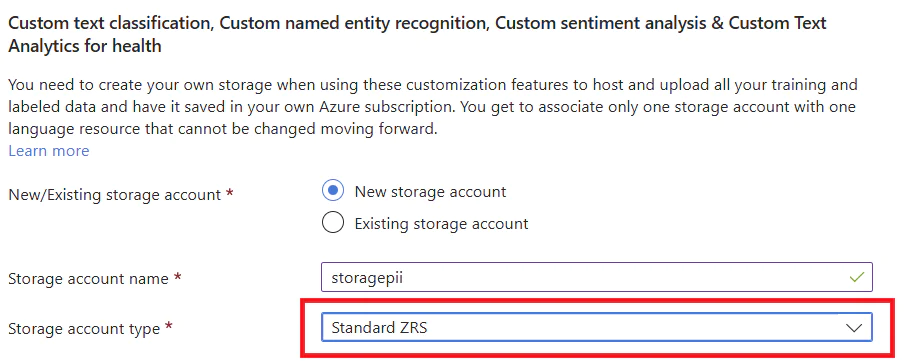
Then review the AI policies and check the acceptance checkbox. Click on Review + Create. Wait for the deployment to finish, which may take a few seconds. When the deployment is complete, click on Go to resource group then select the created language service. Click on the left navigation’s Resource Management > Keys and Endpoint and observe the keys and endpoint as you will need them later in the application.
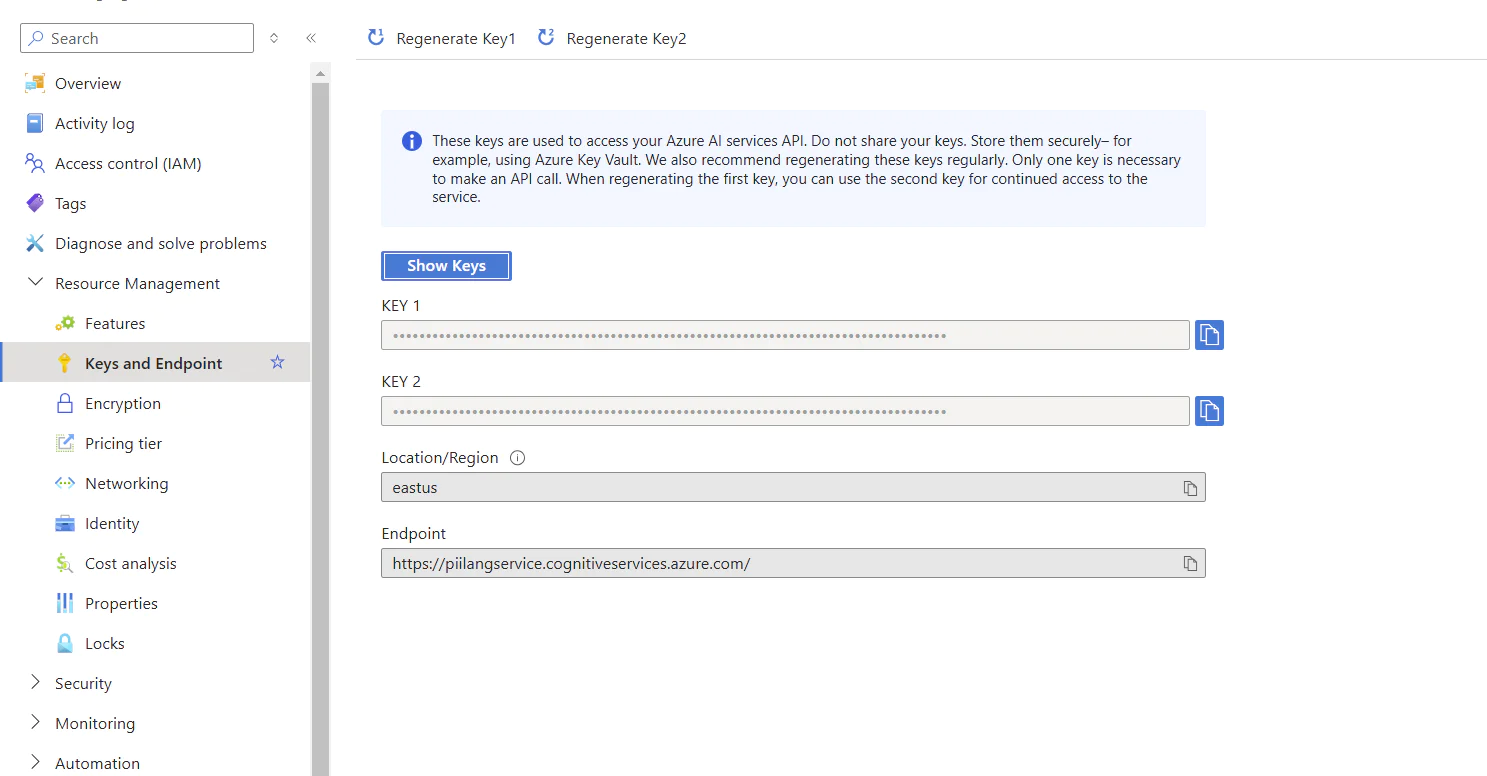
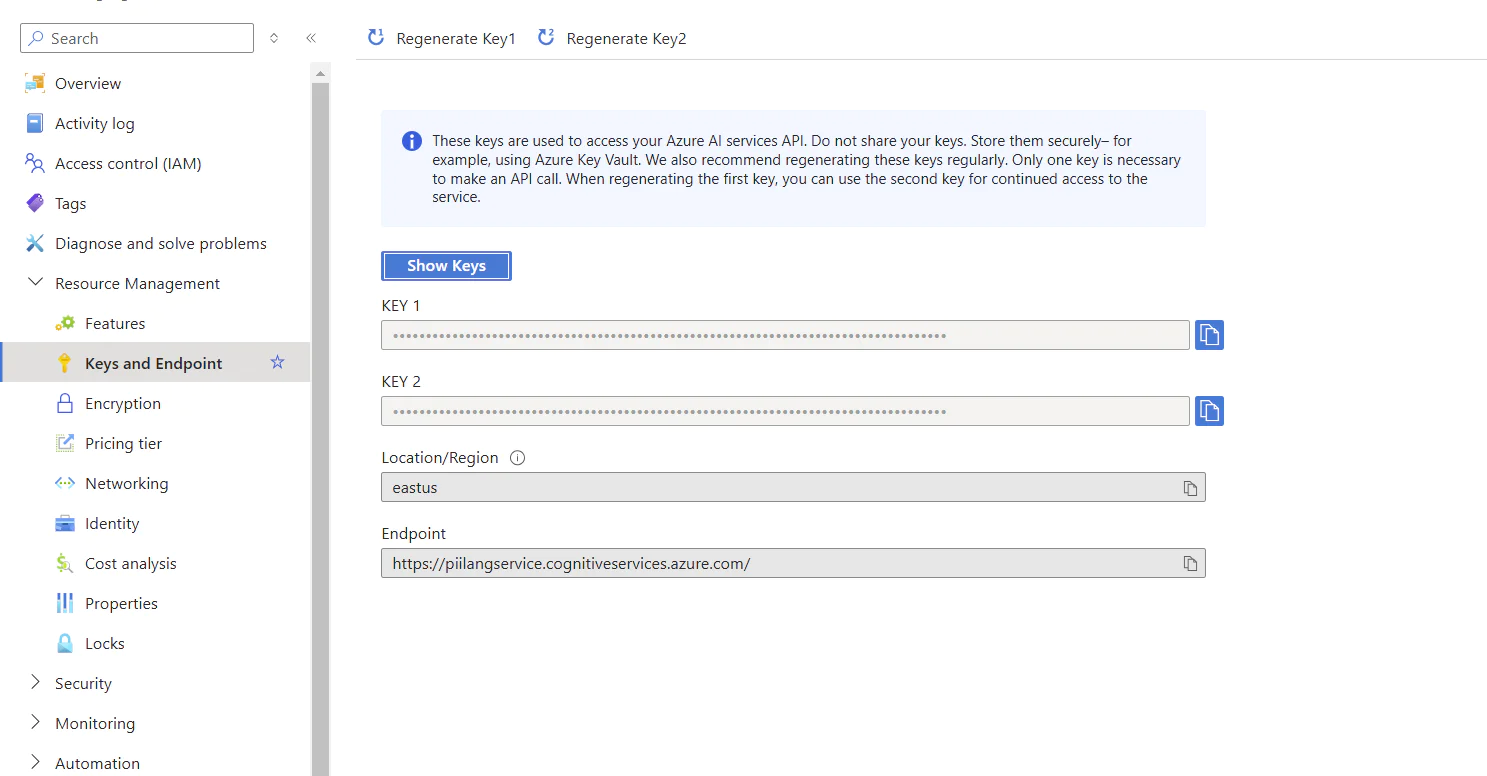
Create the .NET application
Open Visual Studio Code and select a folder to save your application. Run the following commands in the terminal to create a .NET console application.
Install Required NuGet Packages
Next, run the following commands in the terminal to install SendGrid and Azure.AI.TextAnalysis.
Setup the application configuration keys
You’ll need to create a new SendGrid API Key with restricted access. On the Restricted Access tab, be sure your API Key is set to allow Email Sending access. Give your API key a name, and click Create + View. Keep this key somewhere safe as you will need it in the subsequent steps, and SendGrid will not show it again.
In the app, create a new file called AppConfig.cs and add the language service’s endpoint and key, the SendGrid API key and sender email and name in the code below.
Create the PII detector service
Create a new file called PiiDetector.cs. Then add the following code to use Azure.AI.TextAnalysis to detect and redact sensitive data before sending the text to the email send service.
Create the email service
Create a new file called EmailService.cs and add the following code.
Configure the console
Open program.cs and replace it with the following code. This code will enable the application flow of entering the email data, then trigger PII detection, and, finally, send the email.
Running the app
To run the console application you can write the following command in the terminal in VS Code
It will ask you in the console to enter the email text, recipient’s email and name. Make sure to enter example (fake!) sensitive data in the email text to be able to test the redaction of the sensitive data as shown below.
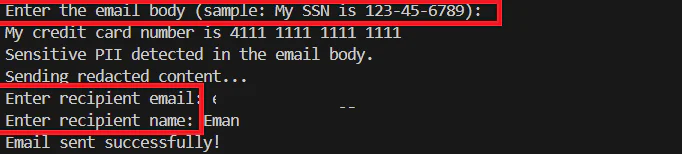
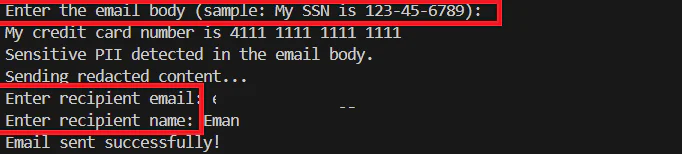
Then check the recipient’s email. You will see the email text sent with redacted data if any PII information was detected.
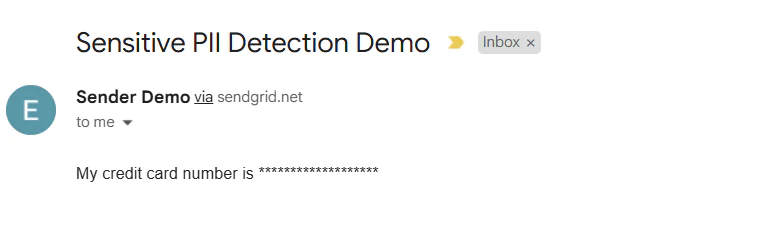
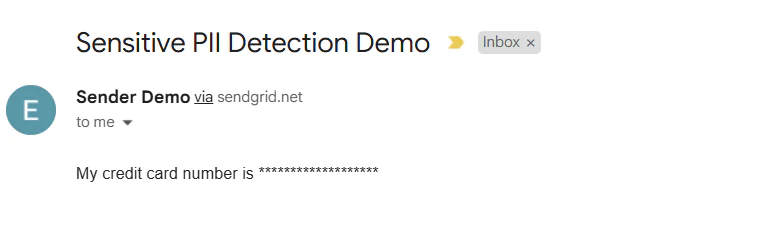
Extending your Azure Application
By proactively detecting and handling PII, you significantly reduce the risk of accidentally leaking sensitive information, maintaining compliance and user trust. You can extend this application to be used for messages, files and any shared resources to detect any data leaks. You can also extend the applications to APIs or automated job runs.
Eman Hassan, a dedicated software engineer and a technical author, prioritizes innovation, cutting-edge technologies, leadership, and mentorship. She finds joy in both learning and sharing expertise with the community. Explore more courses, code labs and tutorials here https://app.pluralsight.com/profile/author/eman-m
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.