How to Send Messages Between WhatsApp and Slack Using Twilio and CakePHP
How to Send Messages Between WhatsApp and Slack Using Twilio and CakePHP
Efficient communication across multiple platforms is crucial for businesses and teams, as it enhances workflow and ensures that important information is shared seamlessly.
In this tutorial, you will learn how to send messages from WhatsApp to a Slack channel using the Twilio WhatsApp API.
Requirements
To proceed with this tutorial, ensure that you have the following:
- PHP 8. 2 or higher with the Curl, DOM, Intl, JSON, LibXML, MBString, OpenSSL, PCRE, Phar, SimpleXML, Tokenizer, XML, and XMLWriter extensions
- Composer installed globally
- A Twilio account (free or paid). Create a free account if you don't already have one.
- A Slack account with an active channel
- Ngrok installed on your computer with an active account
Create a Slack app
Let's set up a Slack API app that will allow the CakePHP application to interact with Slack channels. To do so, log in to your Slack API dashboard and click on the Create an App button, as shown in the screenshot below.
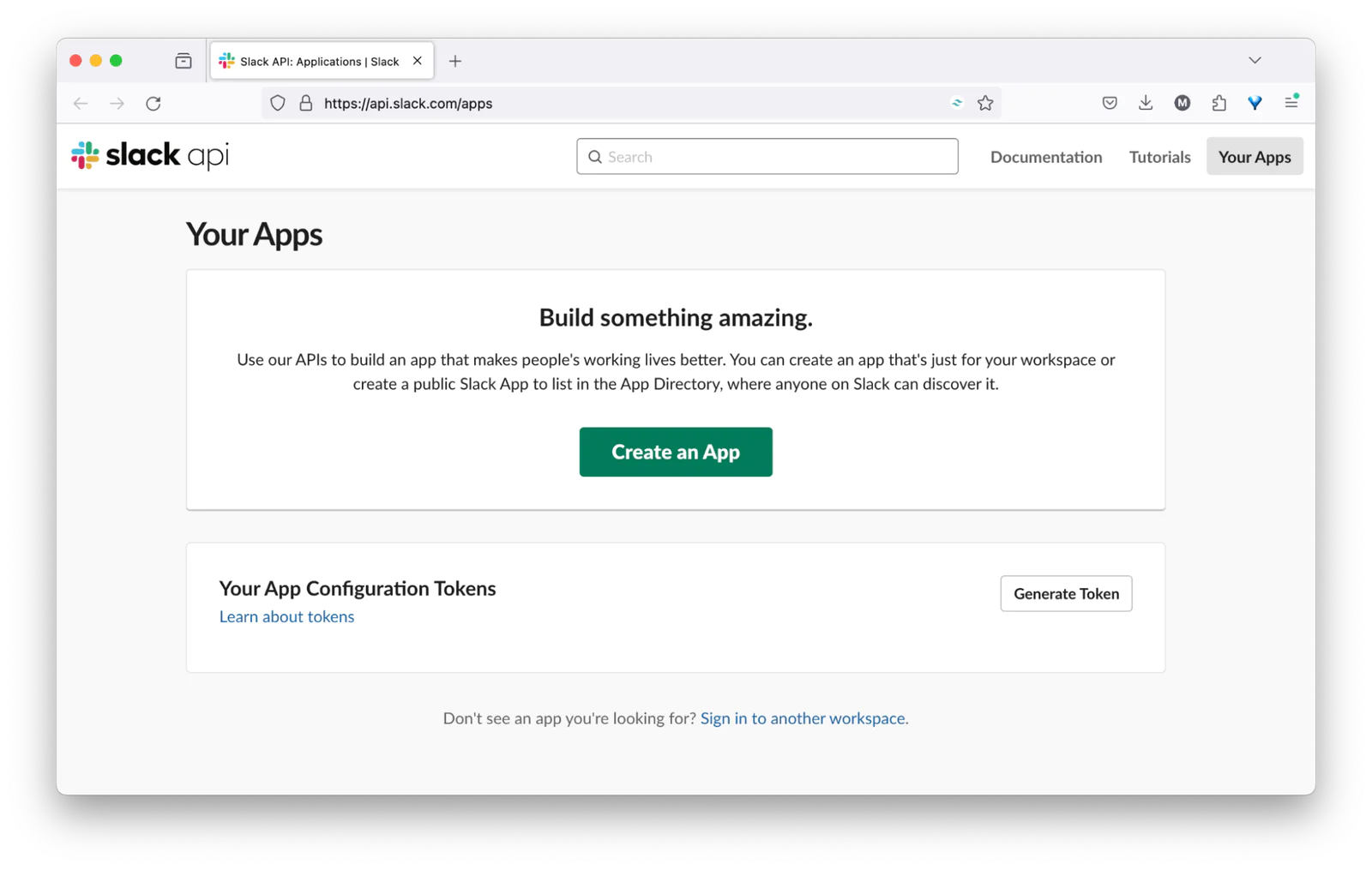
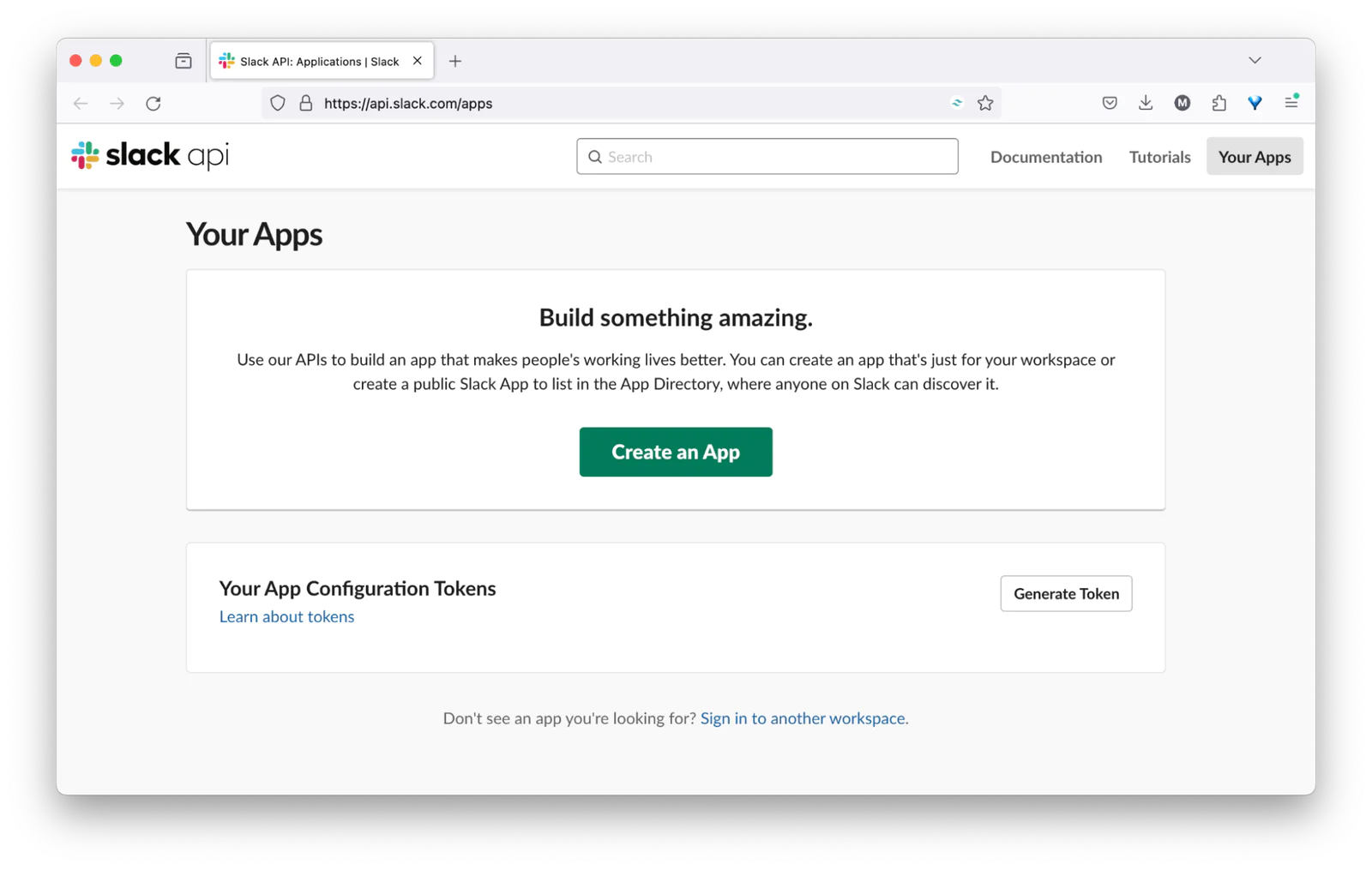
A modal box will pop up asking you to select the app configuration method. Click on the From scratch option, as shown in the screenshot below.
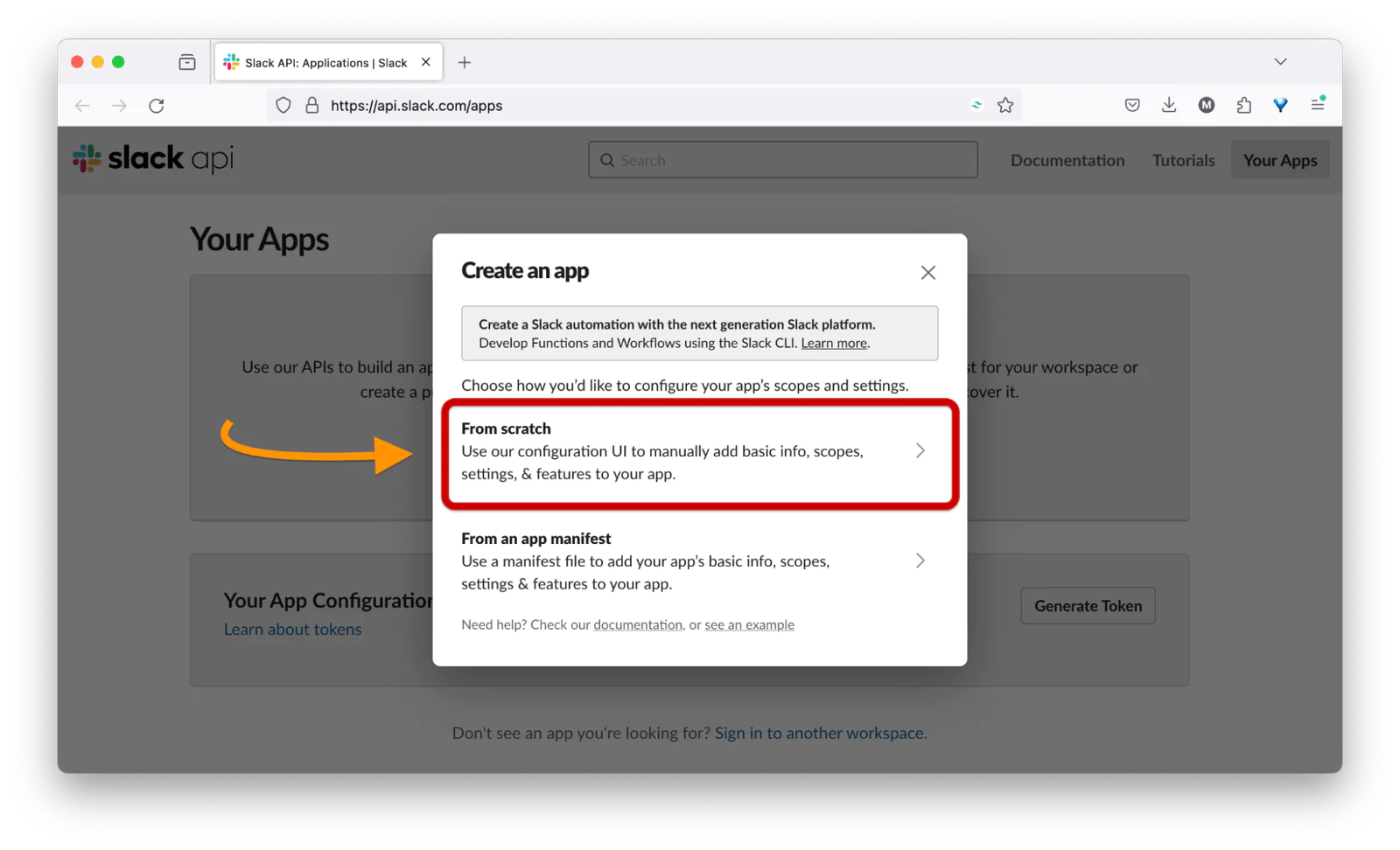
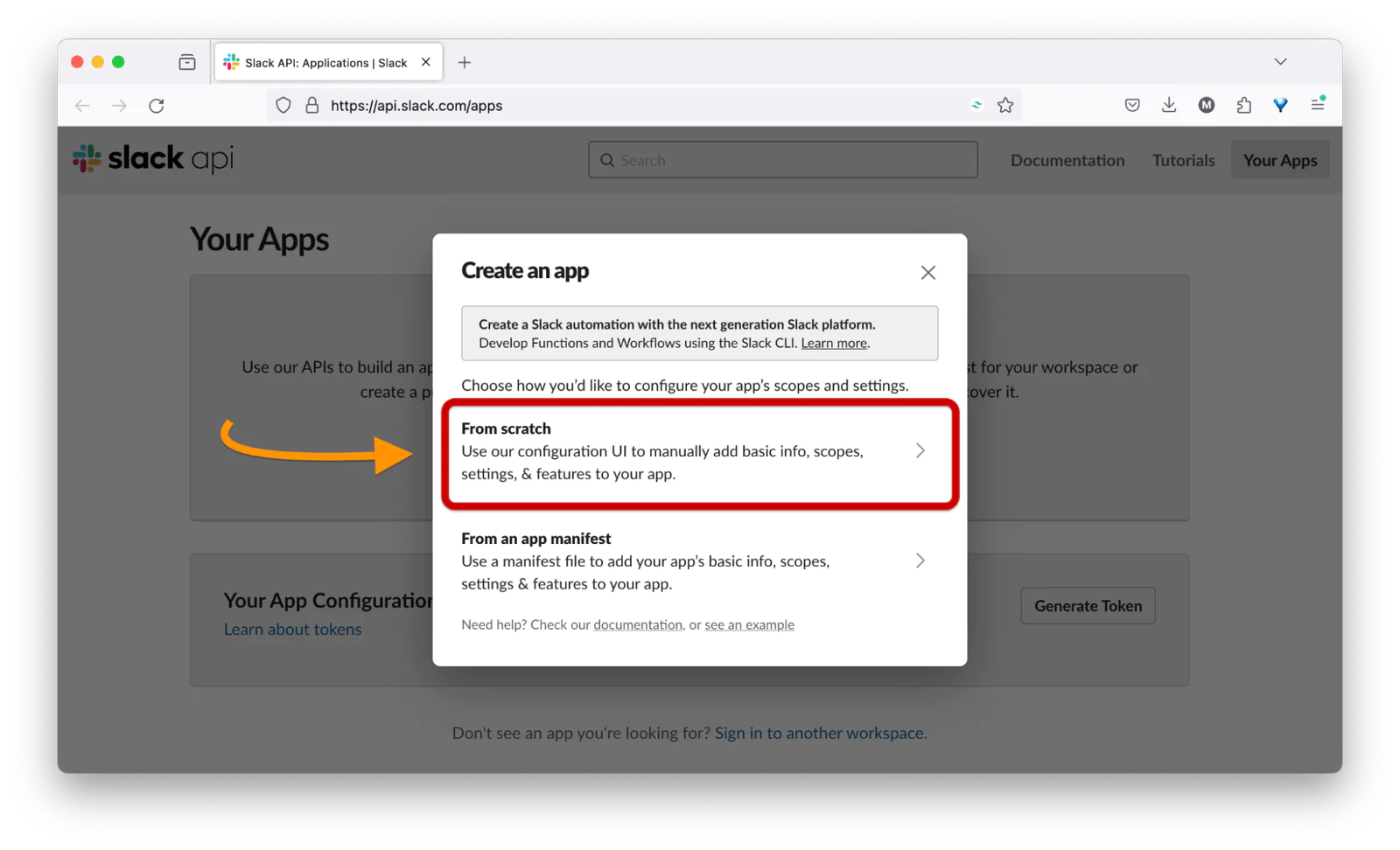
Next, you will be prompted to name the app and choose a Slack workspace. Enter the app name and select your workspace, as shown in the screenshot below.
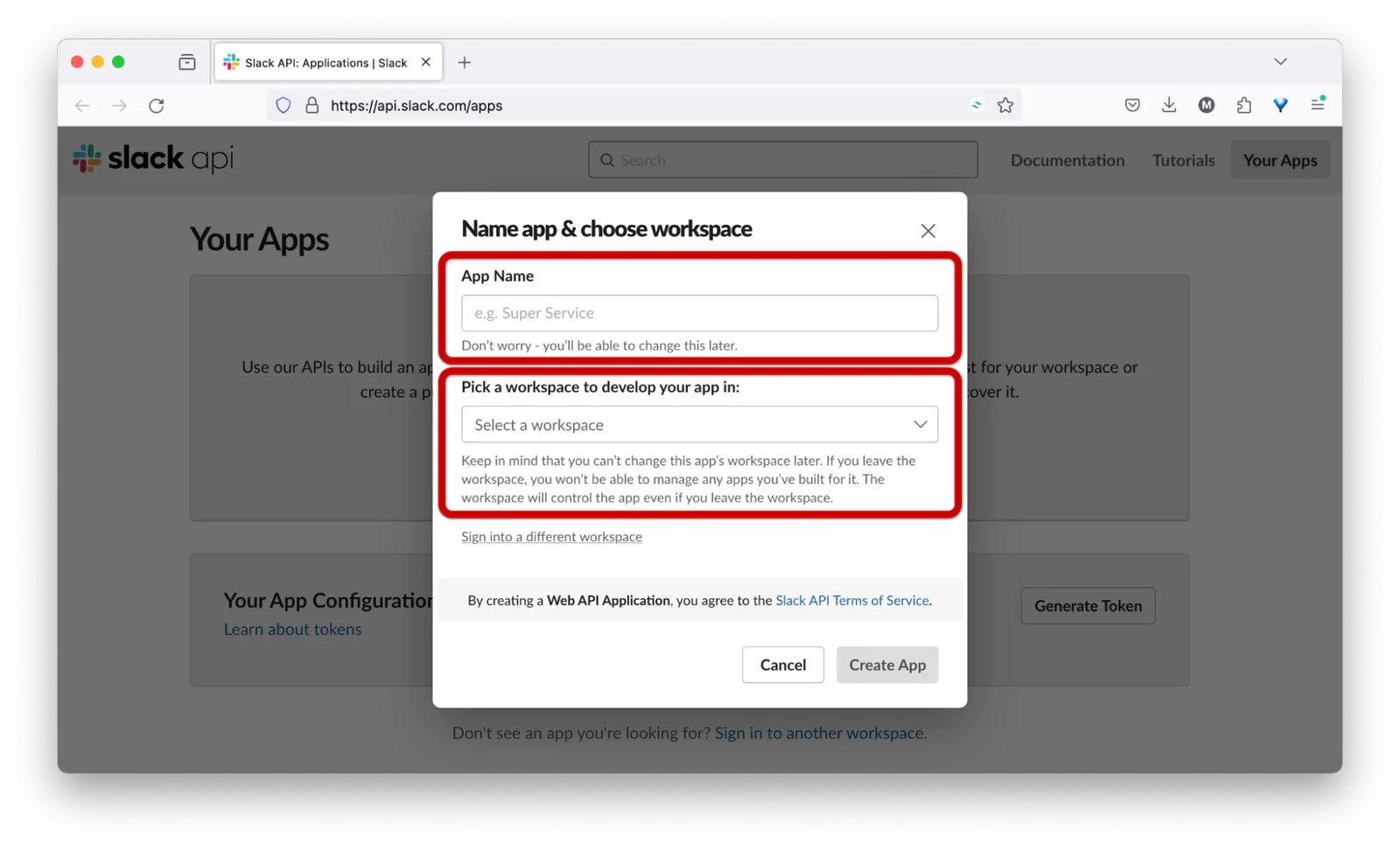
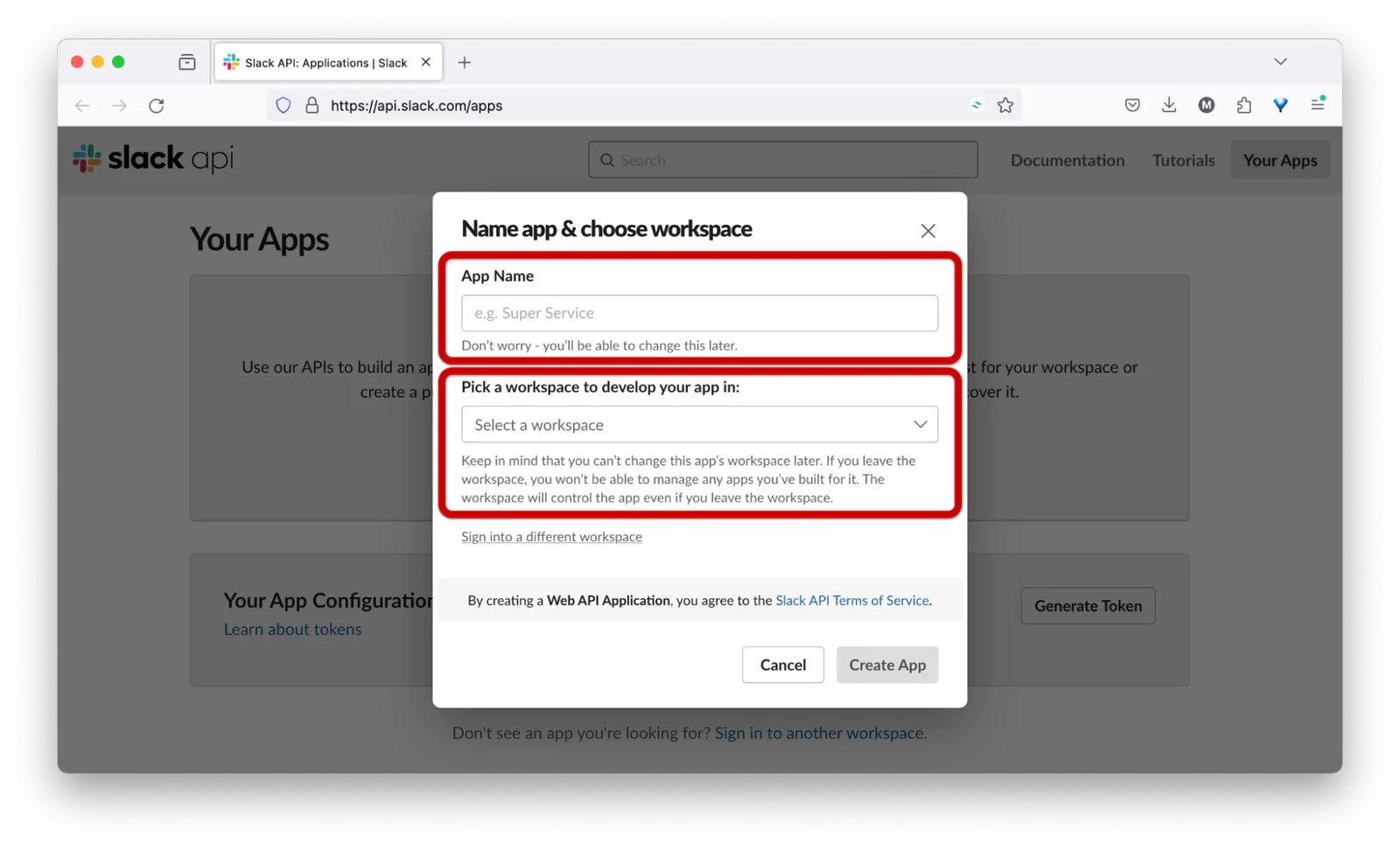
Create a new CakePHP application
Now, let’s start creating the CakePHP application. To do that, open your terminal and navigate to the directory where you want to create the project. Then, run the command below.
When the installation is complete, you will be prompted with "Set Folder Permissions? (Default to Y) [Y, n]?". Then, answer "Y" to set the folder permissions.
Install the Twilio PHP Helper Library
For the application to interact with the Twilio WhatsApp API, you next need to install the Twilio PHP Helper Library using the command below:
Next, run the command below to start the application development server listening on port 8765.
Configure the Slack app webhook settings
Now, let's configure the Slack app to allow external applications to post messages into the workspace channel. To do so, from the created Slack app menu, click on Incoming Webhooks. Then, enable the Activate Incoming Webhooks option and click on the Add New Webhook to Workspace button, as shown in the screenshot below.
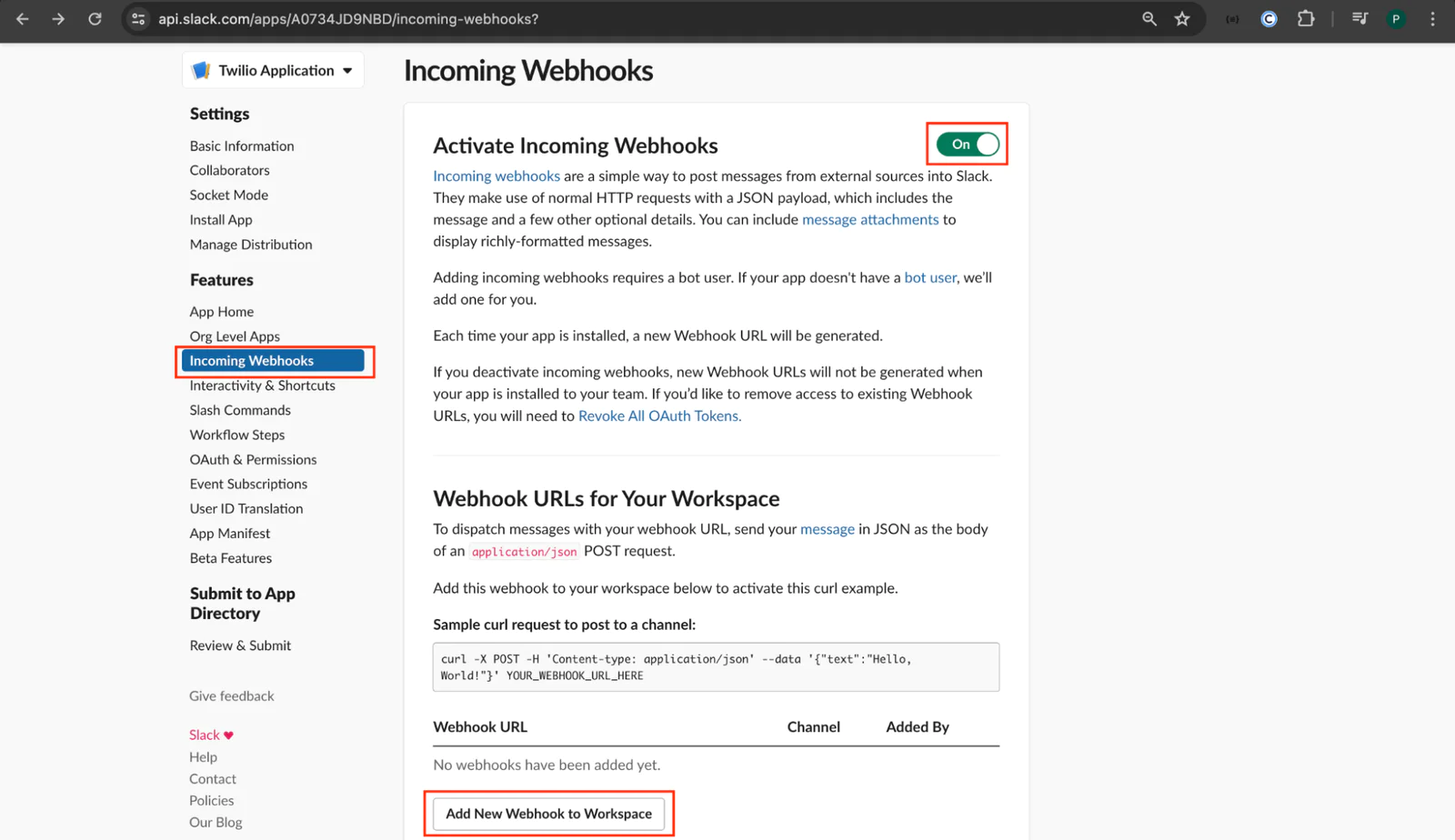
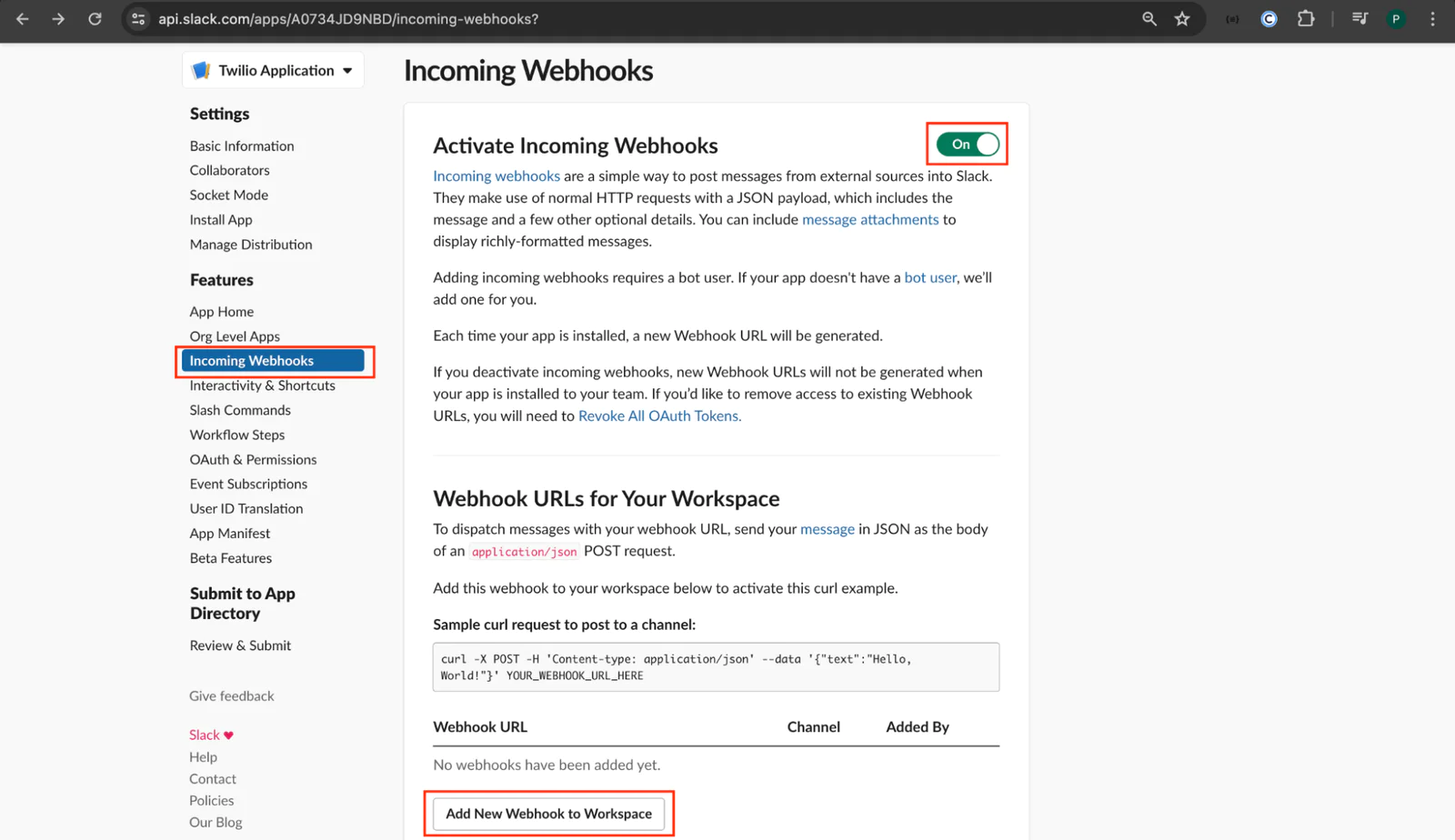
Next, you will be required to select the channel to which you want to send messages. Go ahead and select the appropriate channel. Then, click on the Allow button to grant the application access to the channel, as shown in the screenshot below.
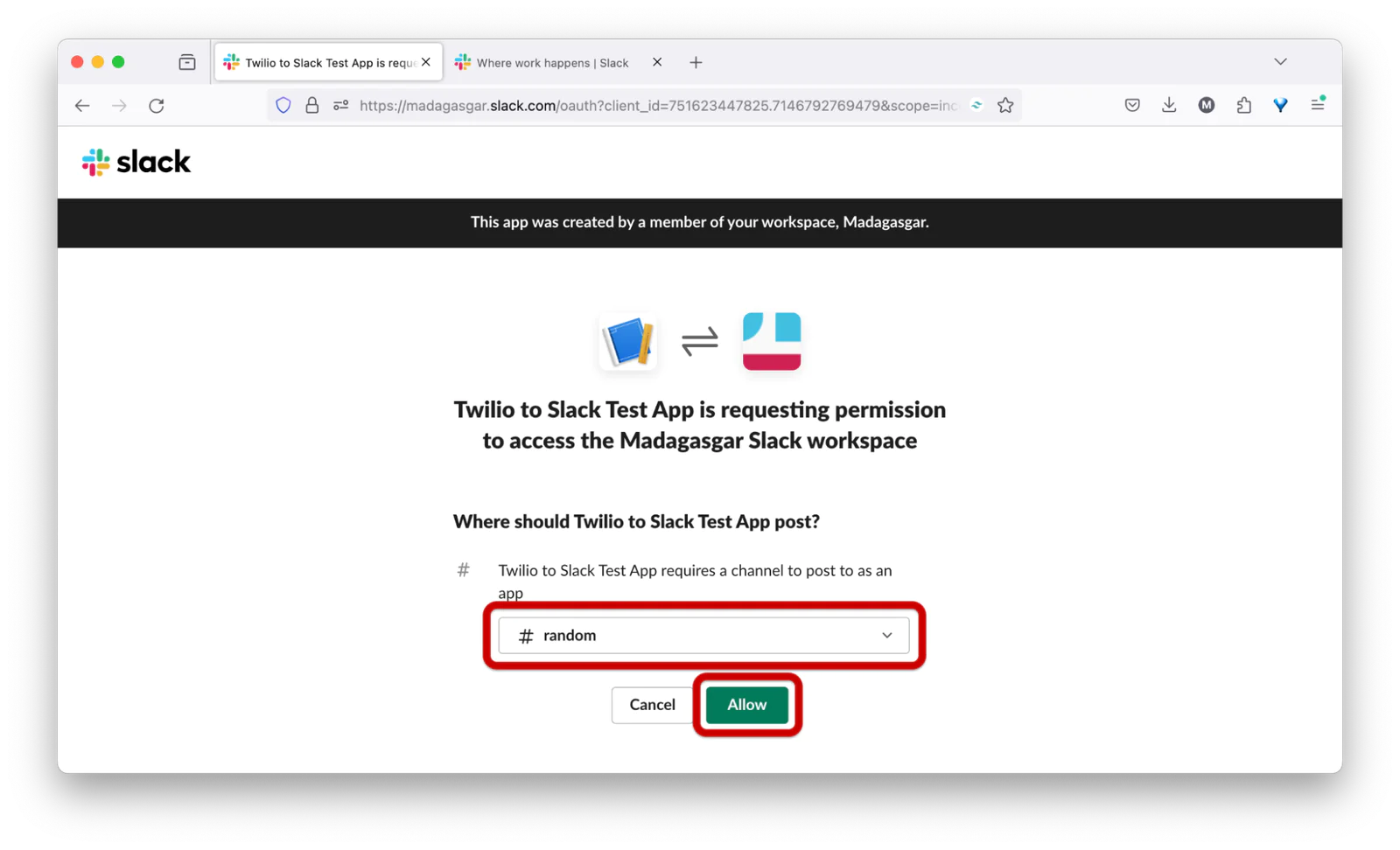
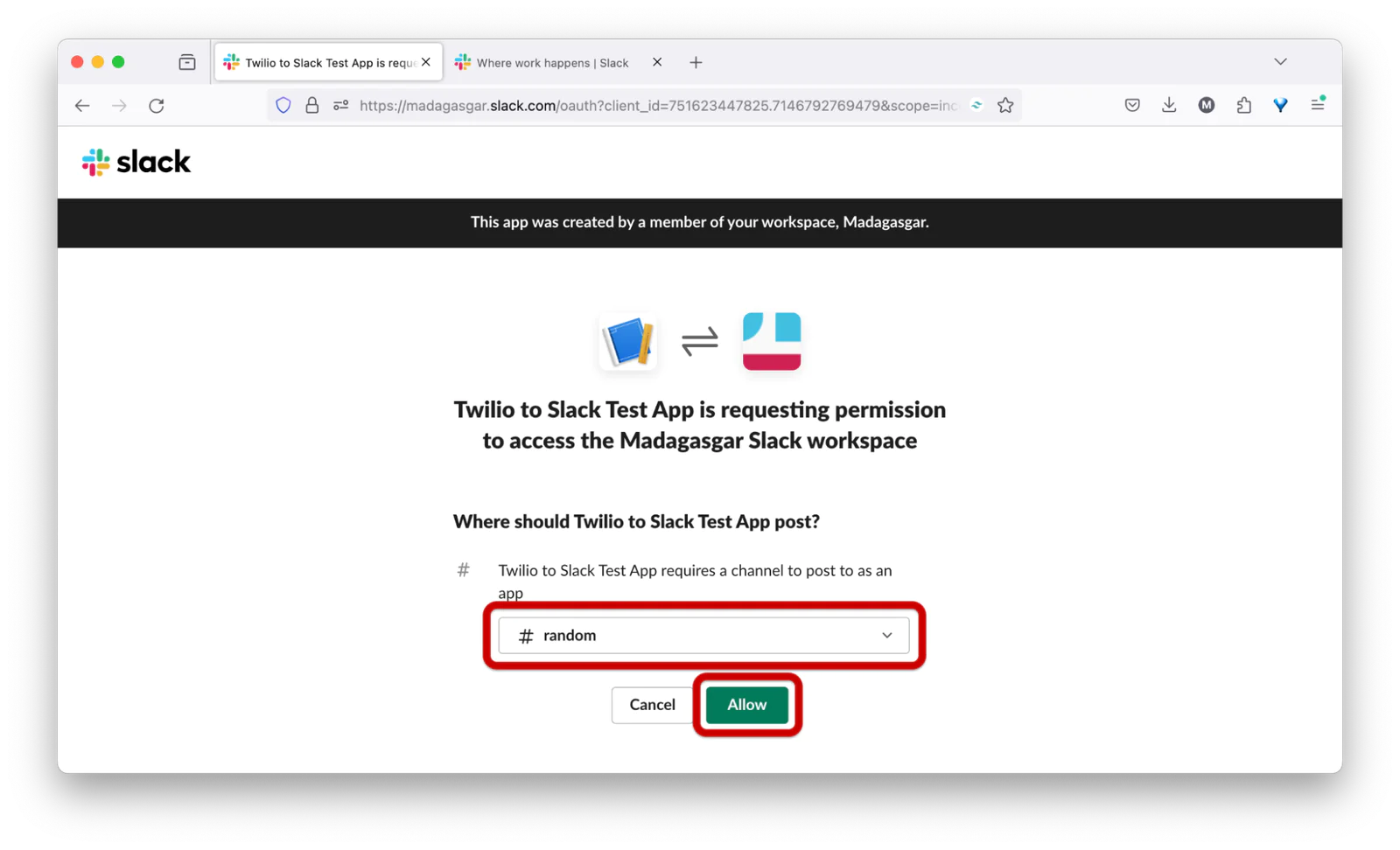
At the bottom of the Incoming Webhooks page which you'll see next, you will see the generated webhook URL for the application, as shown in the screenshot below.
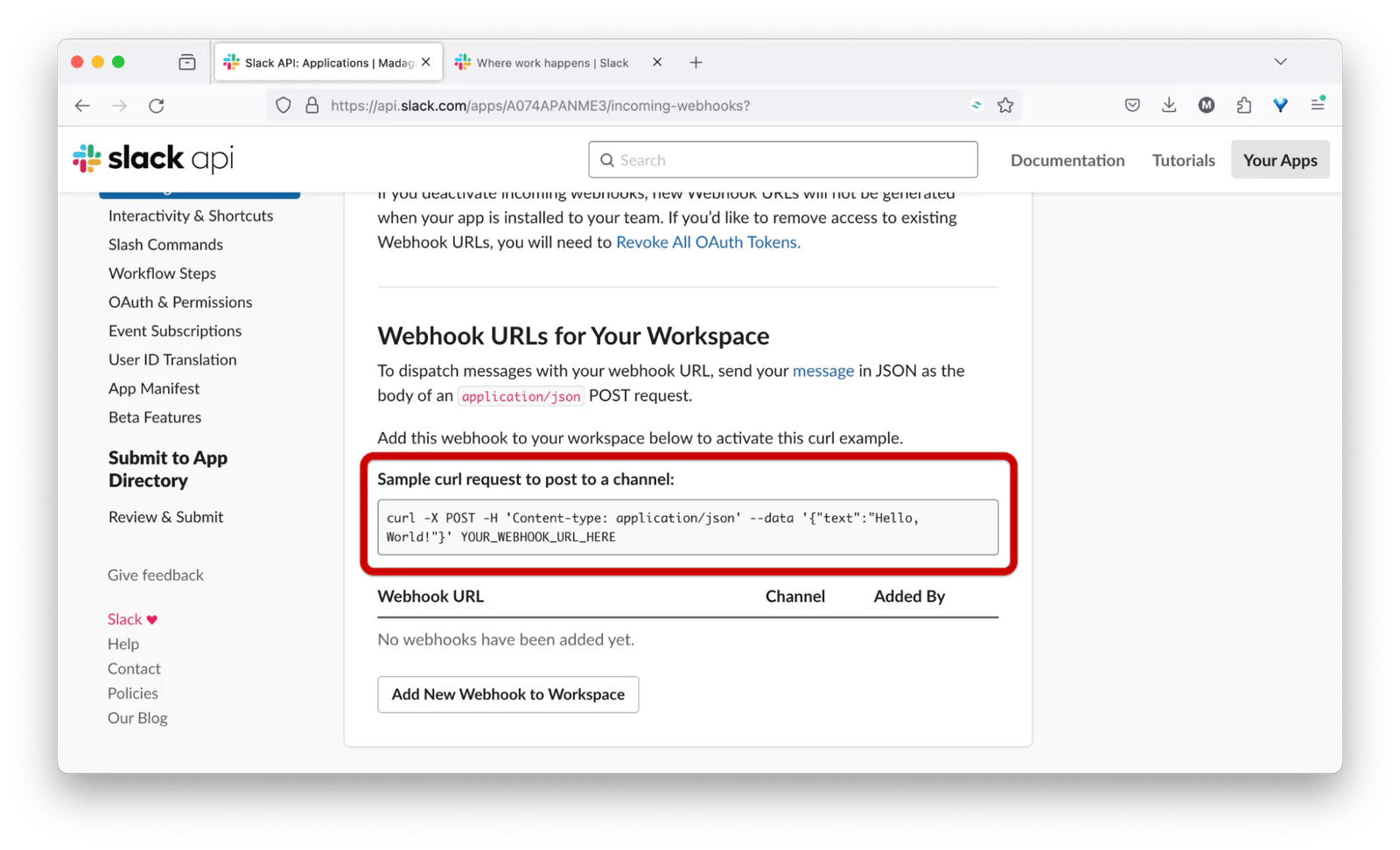
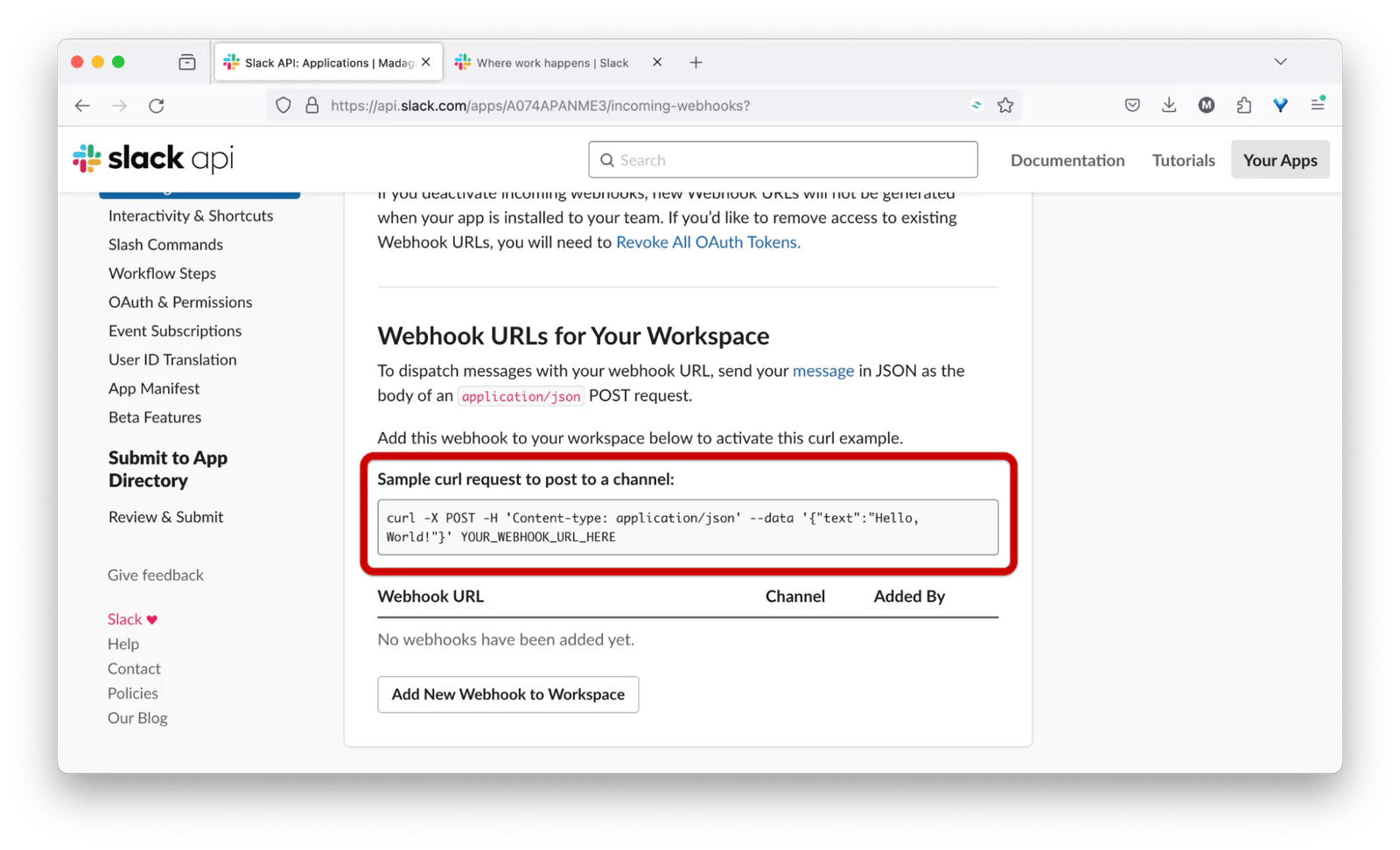
Retrieve your Twilio credentials
To retrieve your Twilio access credentials, log in to your Twilio console dashboard. Under the Account Info section, you will see your Account SID and Auth Token, as shown in the screenshot below.
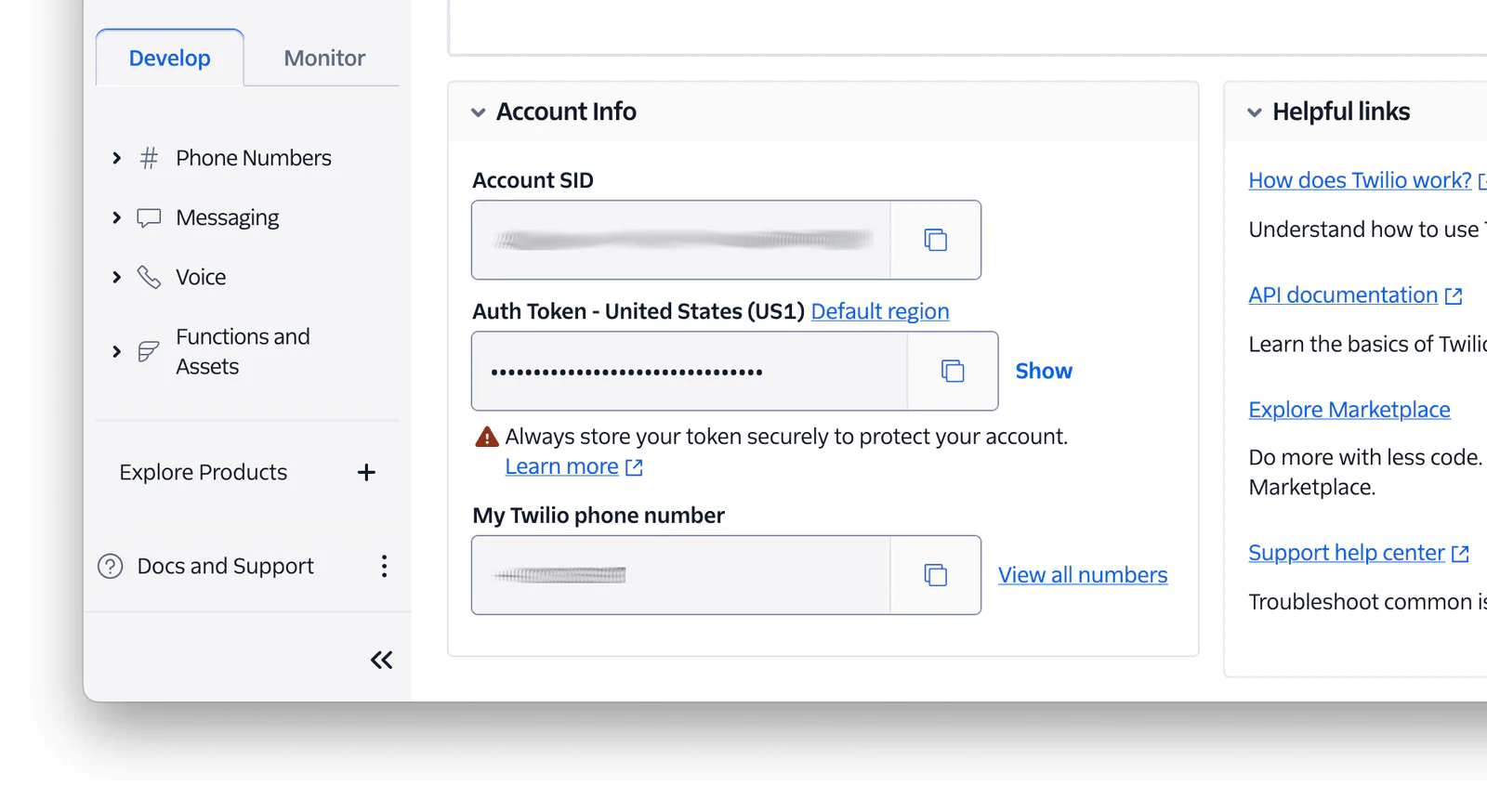
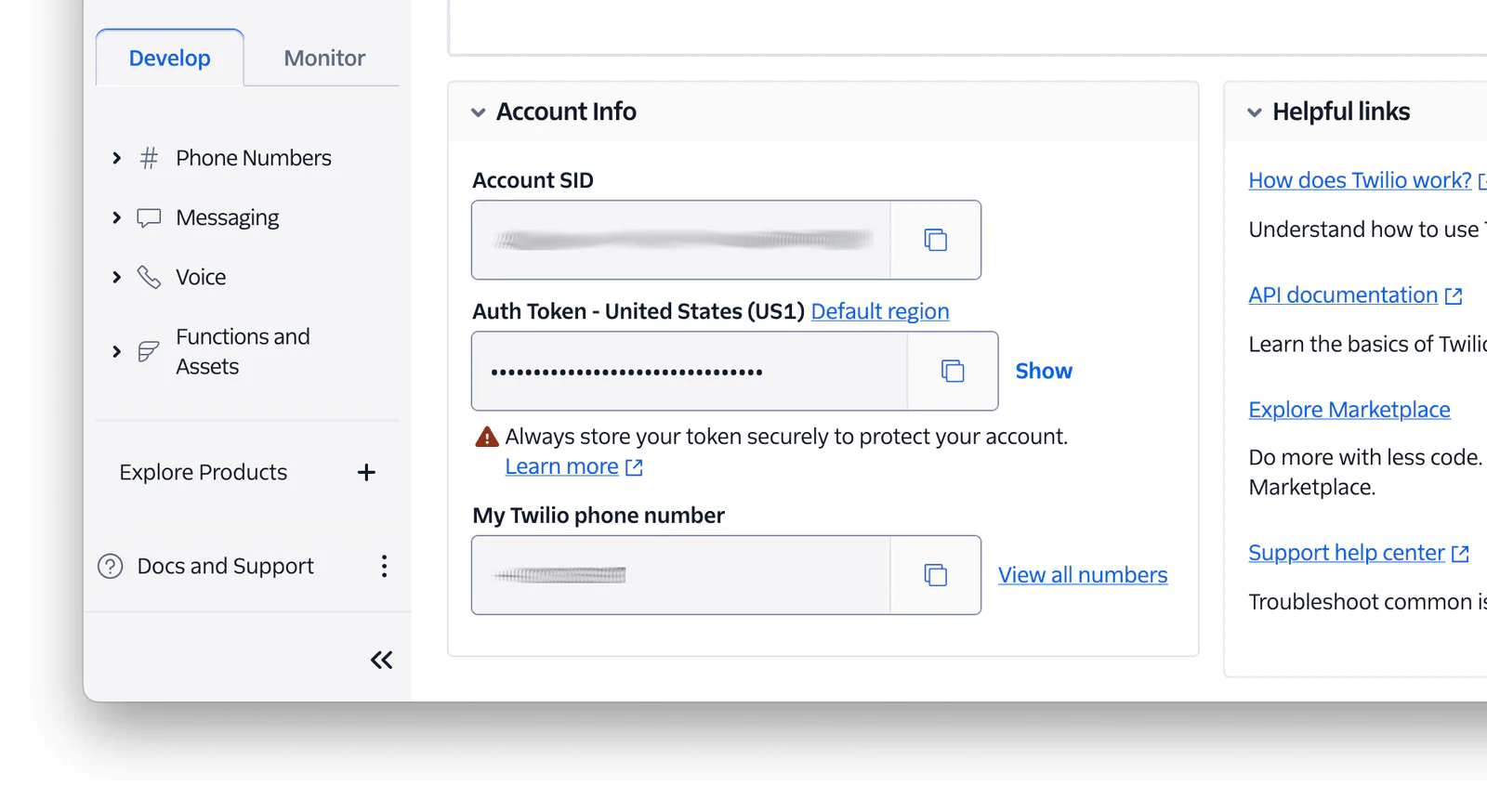
Store the application's configuration settings
To store the Slack access token and Twilio access credentials within the CakePHP application's environment variables, you need to create a .env file from .env.example inside the config folder. You can do this by running the command below in a new terminal session or tab:
Now, open the project folder in your code editor, navigate to the config folder, and open the .env file. Inside the file, add the following environment variables.
Replace <actual_slack_webhook_url>
with your actual Slack webhook URL, and <actual_account_sid>
and <actual_auth_token>
with your corresponding Twilio values.
Next, let's load the environment variables from the .env file into the application's environment using the josegonzalez\Dotenv library. To do this, open the config/bootstrap.php file and uncomment the following code:
Connect to the Twilio WhatsApp Sandbox
To configure Twilio WhatsApp, navigate from your Twilio Console dashboard to Explore Products > Messaging > Try it out > Send a WhatsApp message, following the steps shown in the screenshot below.
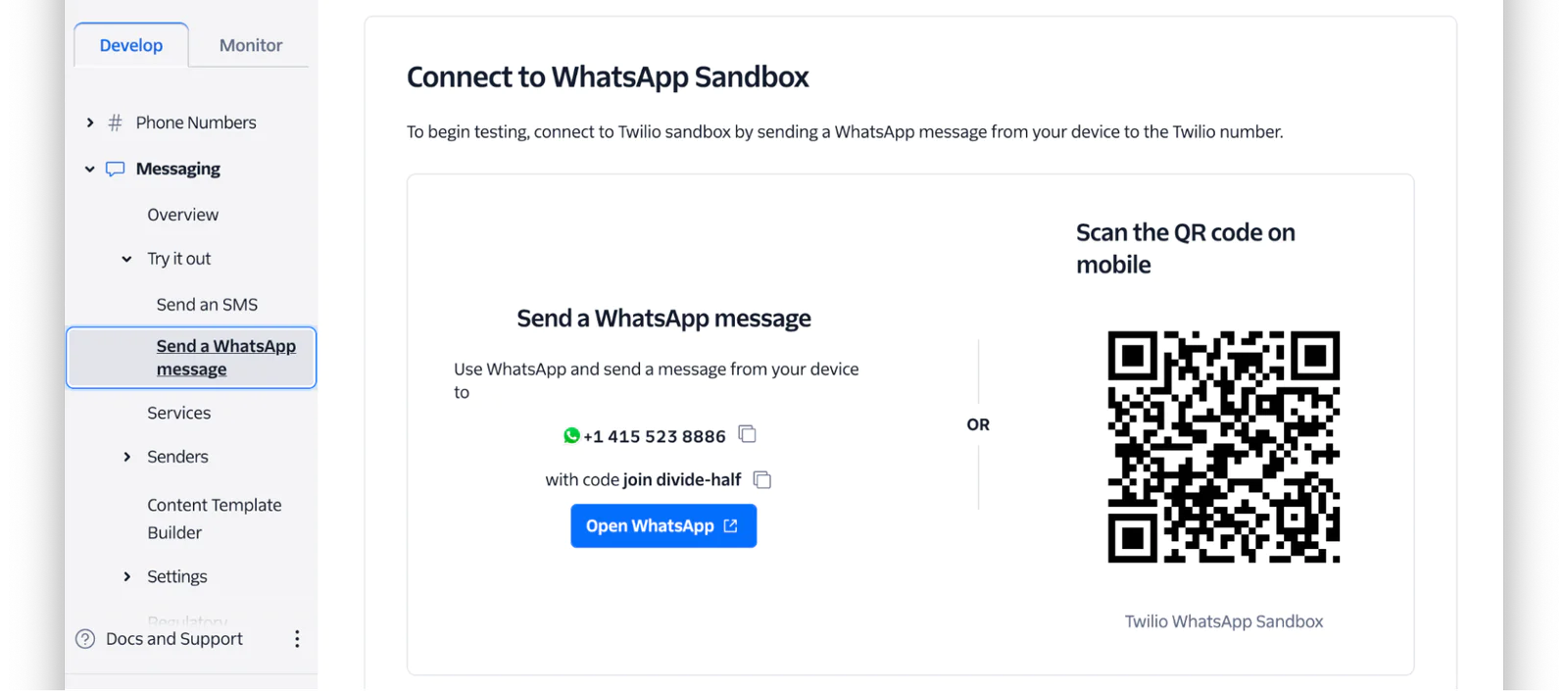
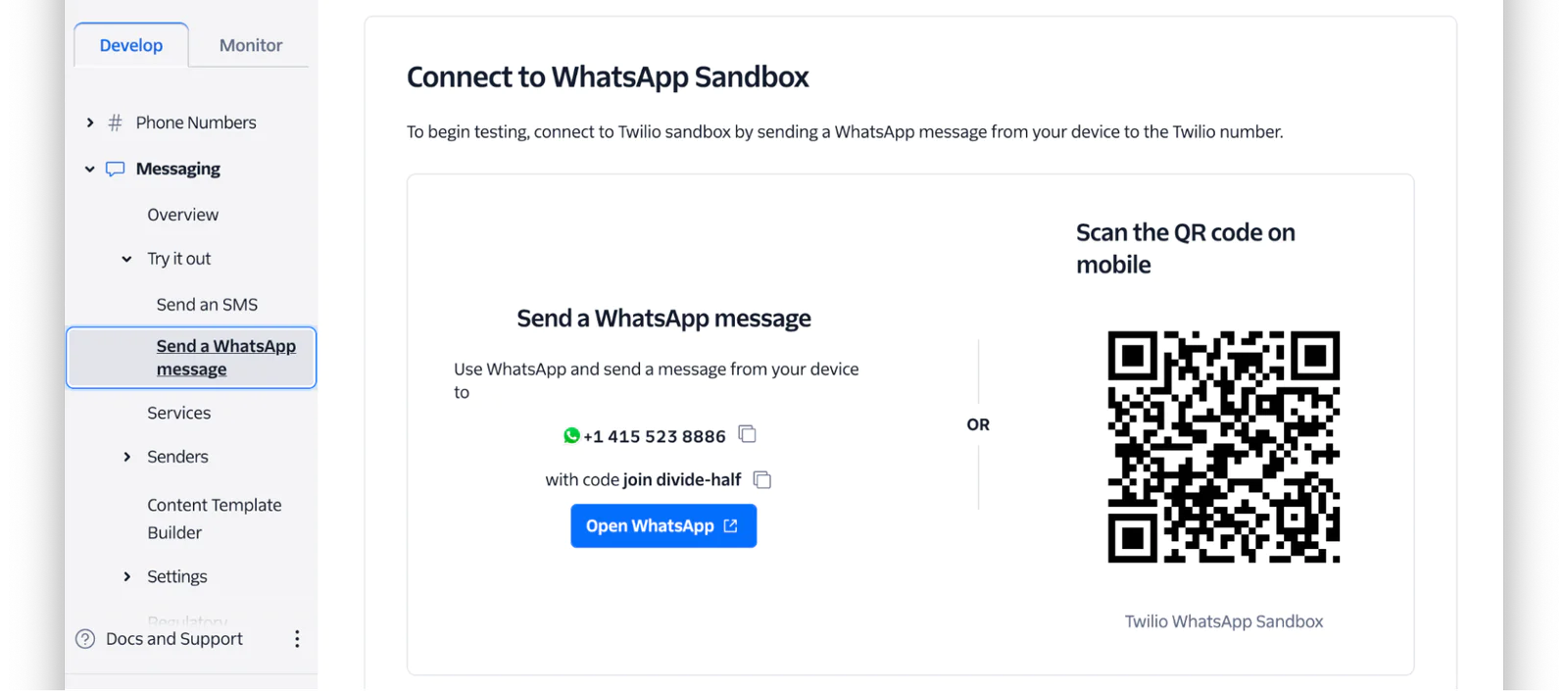
Next, follow the stated instructions by sending the join message to the displayed Twilio WhatsApp number from your own device's WhatsApp number, as shown in the screenshot below.
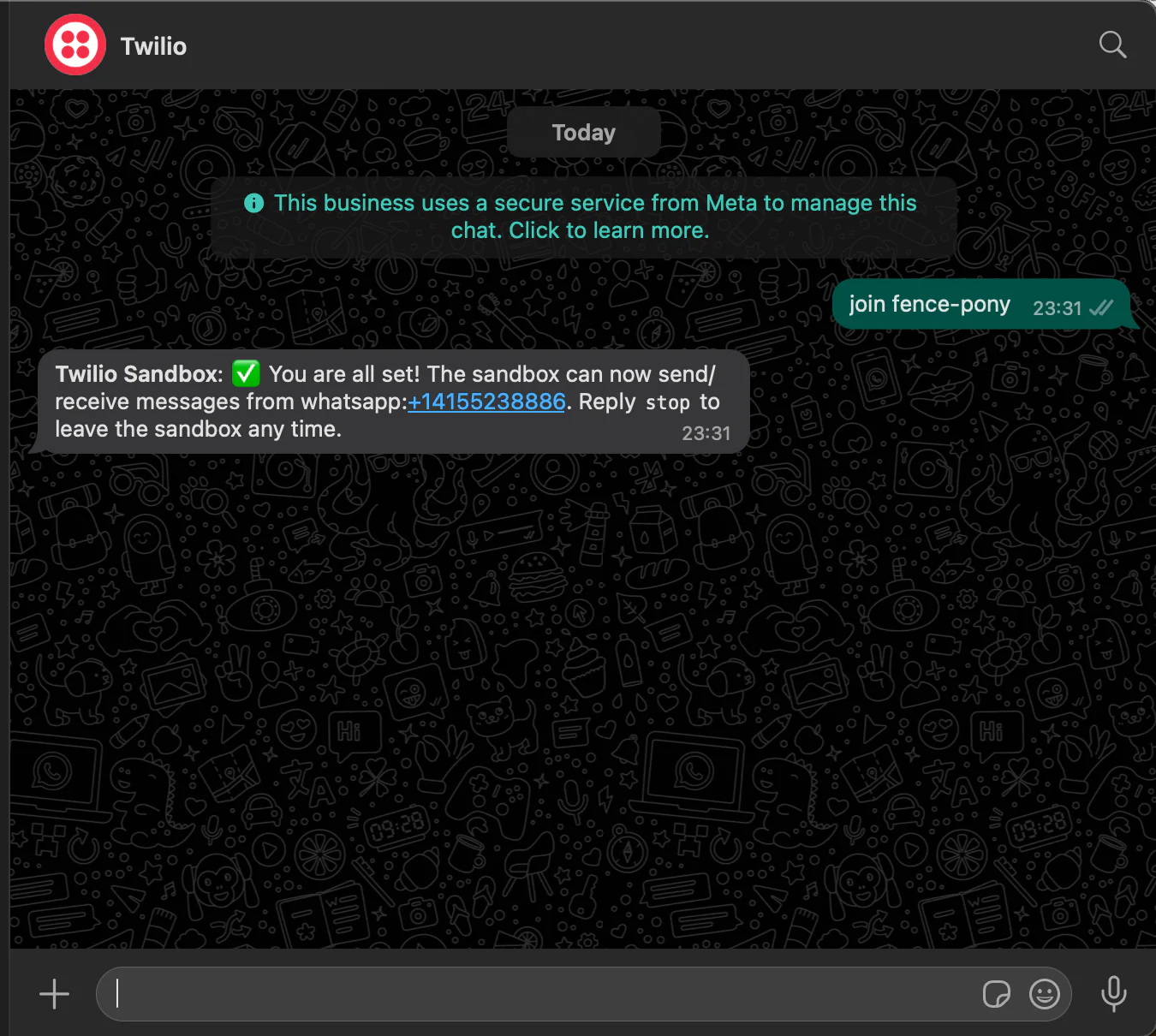
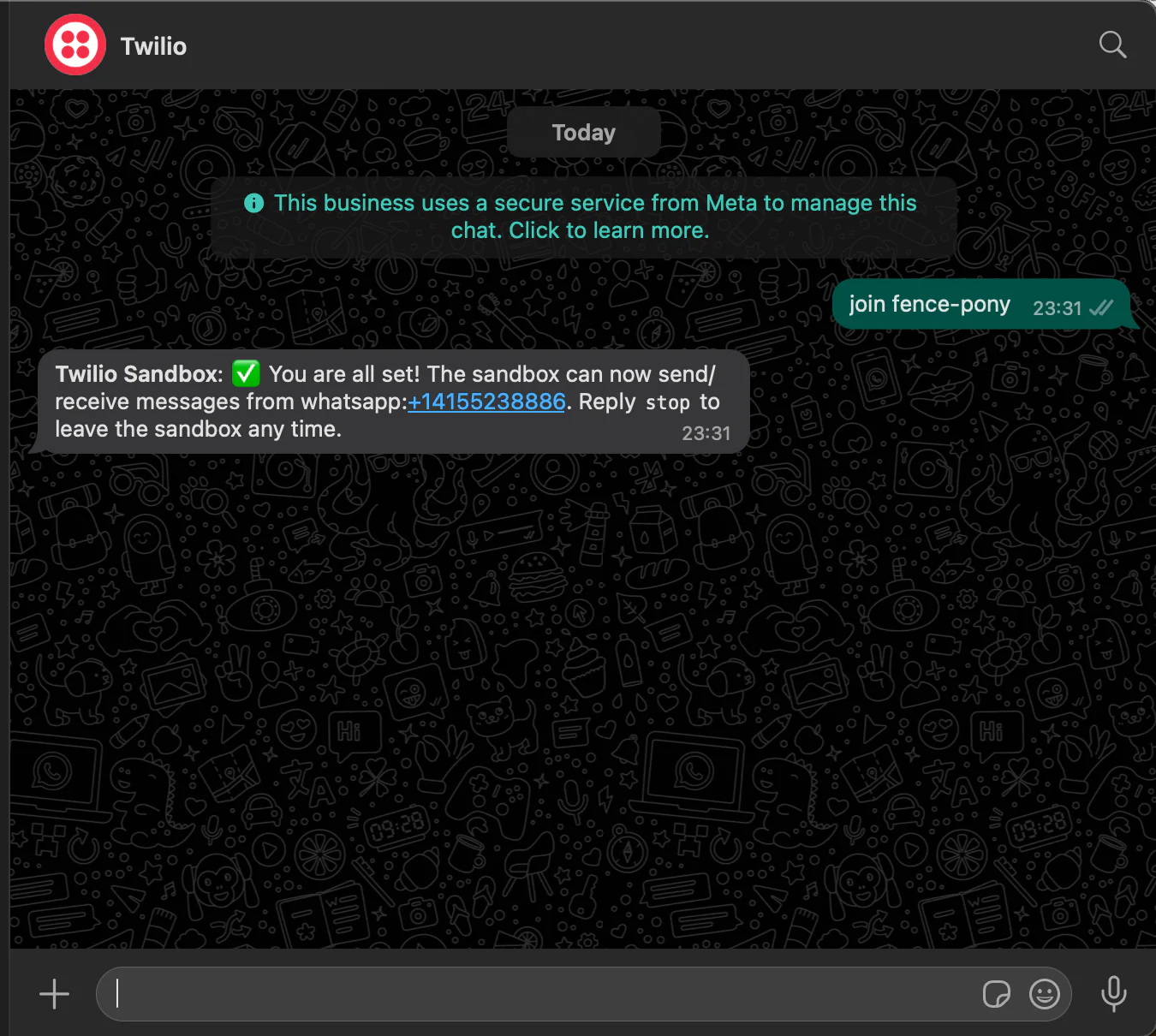
Now, let's add the Twilio WhatsApp number to the environment variables. To do that, add the following line of code to the .env file, replacing the <twilio_whatsapp_number>
placeholder with your actual Twilio WhatsApp number (the value of the From field).
Create the application's core controller
Now, let’s create a controller that receives incoming messages from WhatsApp and sends the messages to the nominated Slack channel. To create the controller, run the command below.
The command above will generate a controller file inside the src/Controller directory named SendSlackMessageController.php. Navigate to this directory, open the file, then update it with the following code.
In the controller code above, we use the receiveMessage()
method to handle incoming WhatsApp messages. The message is then sent to the Slack channel using CakePHP's HTTP client. Once the message is successfully sent to Slack, a success message is sent to the sender's WhatsApp number.
Set up the application's route
To configure the application's sole route, navigate to the config folder and open routes.php. Inside the file, locate $routes->scope()
and add the following code before $builder->fallbacks()
.
Since the CakePHP application is an API endpoint, it is advisable to disable CSRF security. To do this, navigate to the src folder, open the Application.php file, and update the middleware()
method with the following code.
Set up a Twilio WhatsApp webhook
A Twilio WhatsApp webhook is a URL endpoint on your application server that Twilio uses to send HTTP requests containing information about incoming messages and events from WhatsApp. To set up the Twilio WhatsApp webhook, first make the CakePHP application accessible over the internet using Ngrok. To do that, run the command below in your terminal.
Then copy the generated Forwarding URL from the terminal, as shown in the screenshot below.
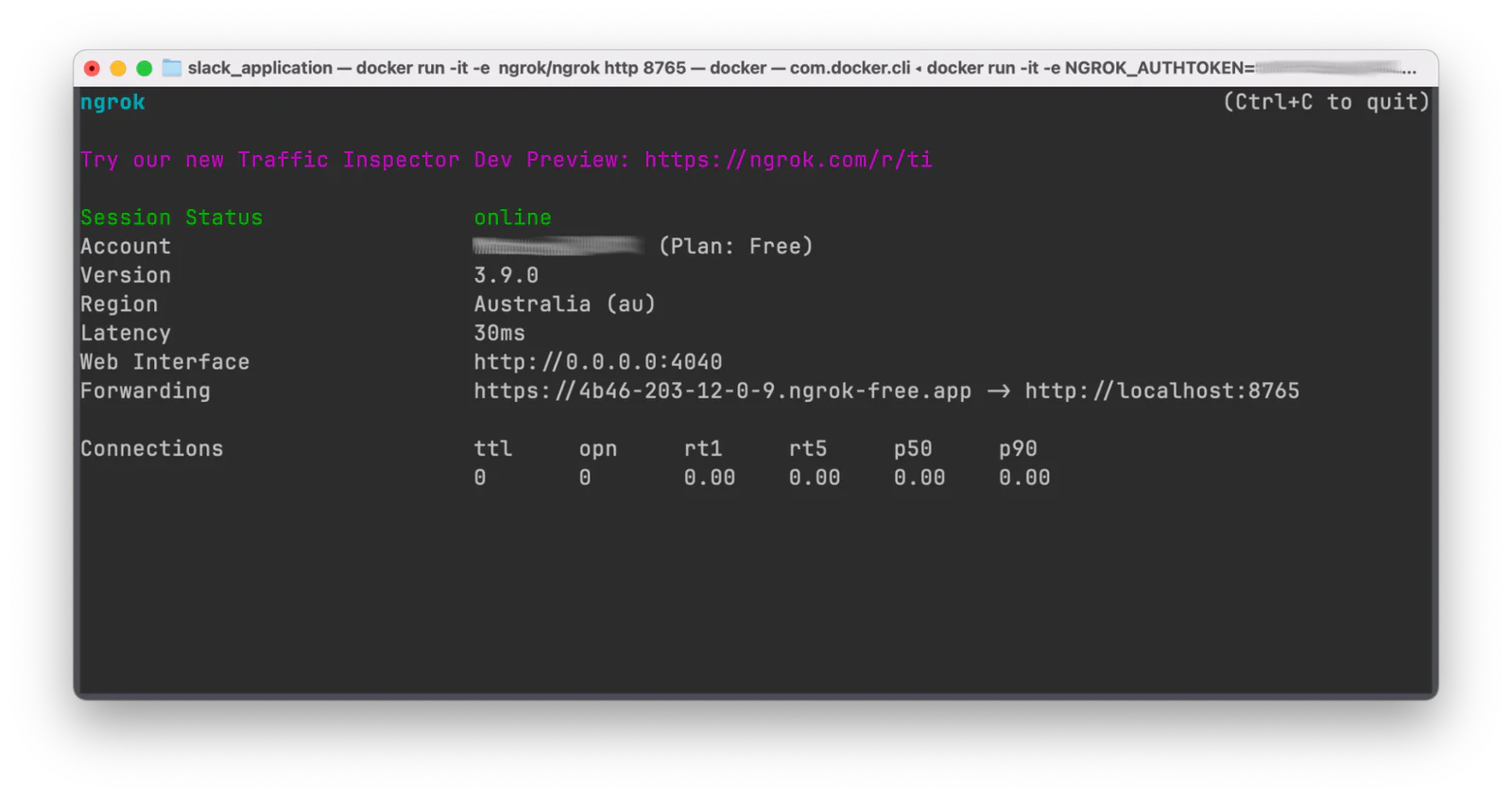
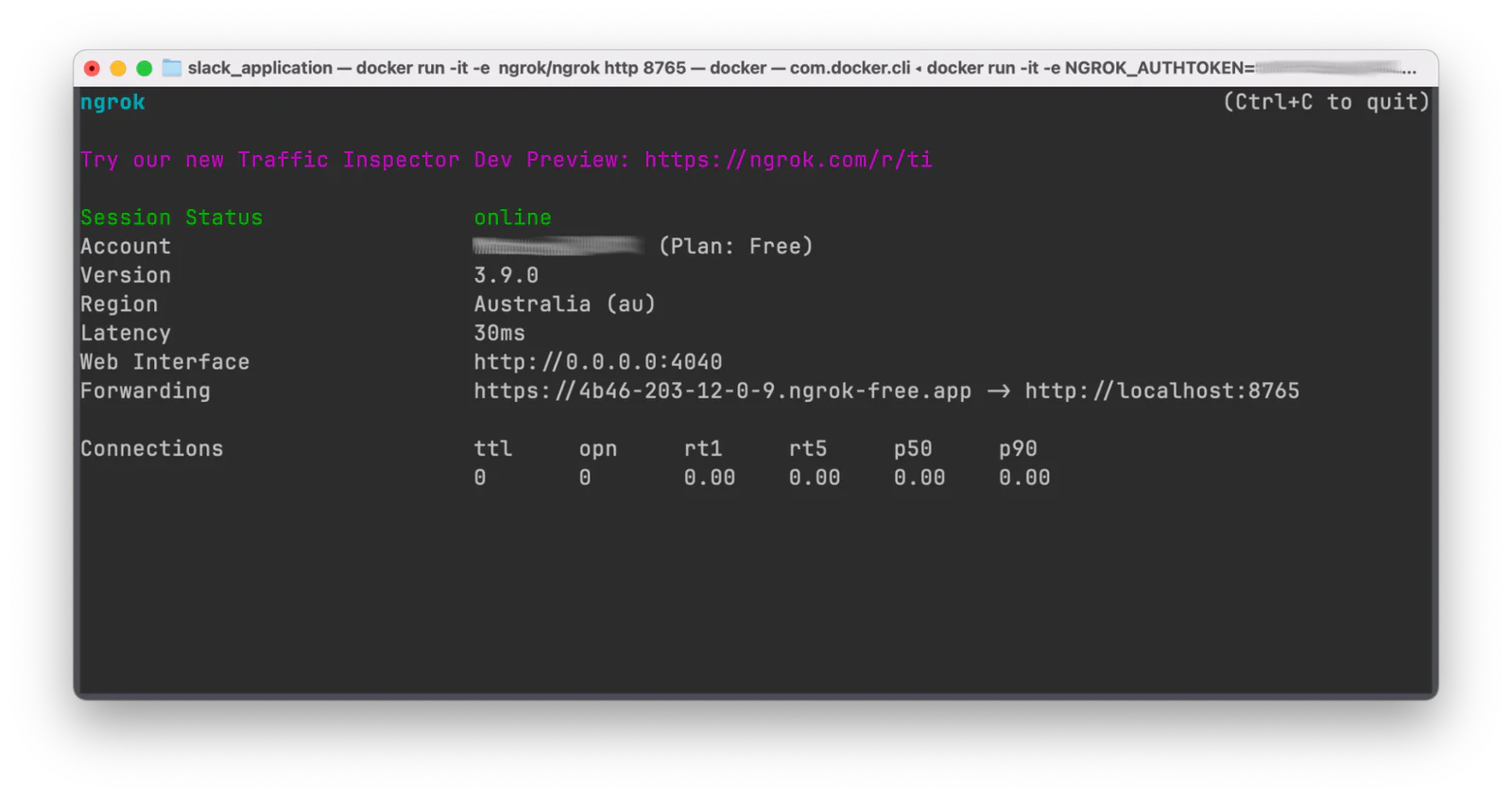
Next, on the Twilio Try WhatsApp page, click on the Sandbox Settings option, and set the sandbox configuration as follows.
When a message comes in: paste the ngrok Forwarding URL and append /receive-whatsapp-message
Method: POST
After that, click on the Save button to save the sandbox configuration, as shown in the screenshot below.
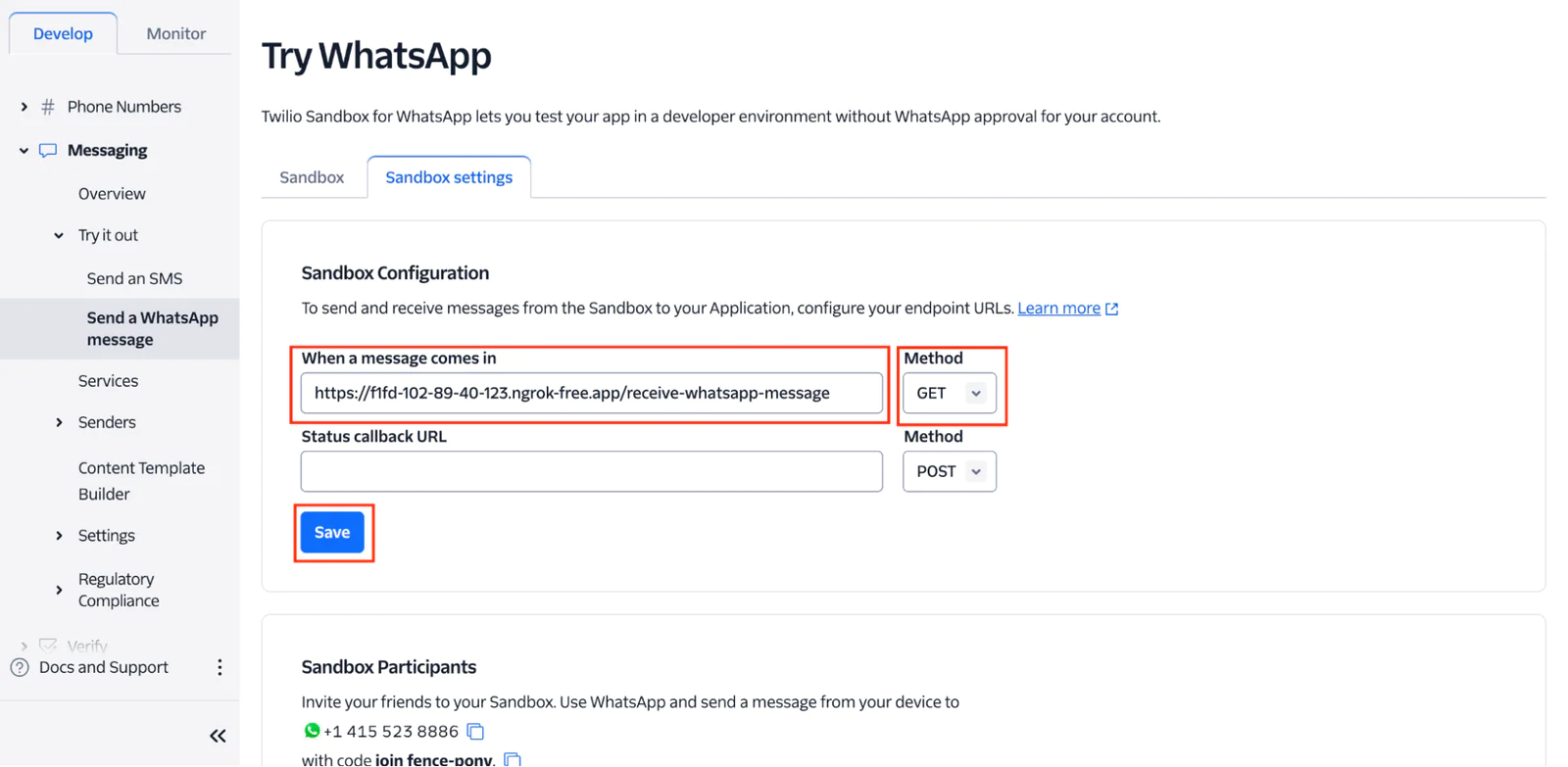
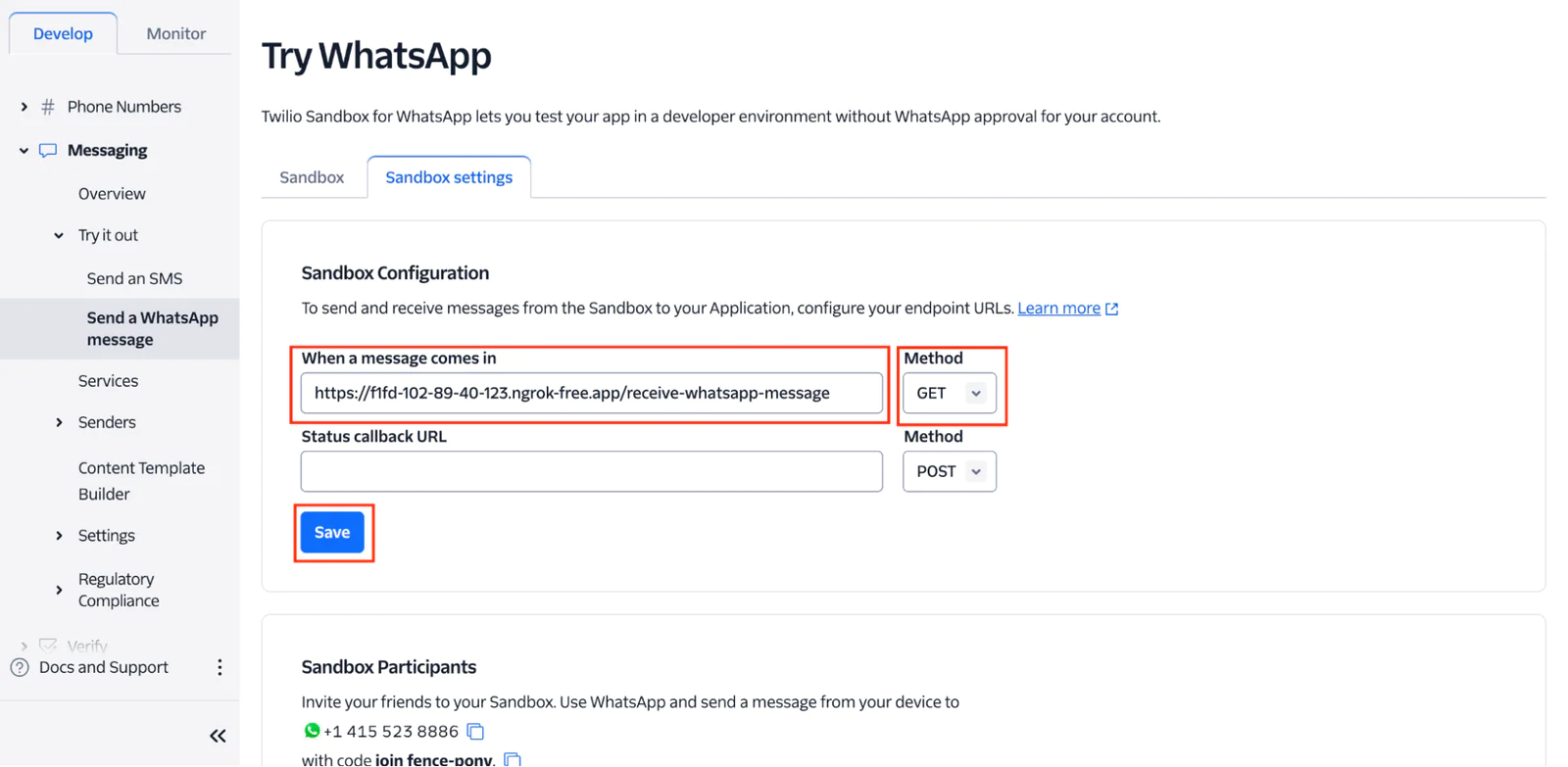
Test the application
To test the application, send a message from your WhatsApp number to the Twilio number, as shown in the screenshot below.
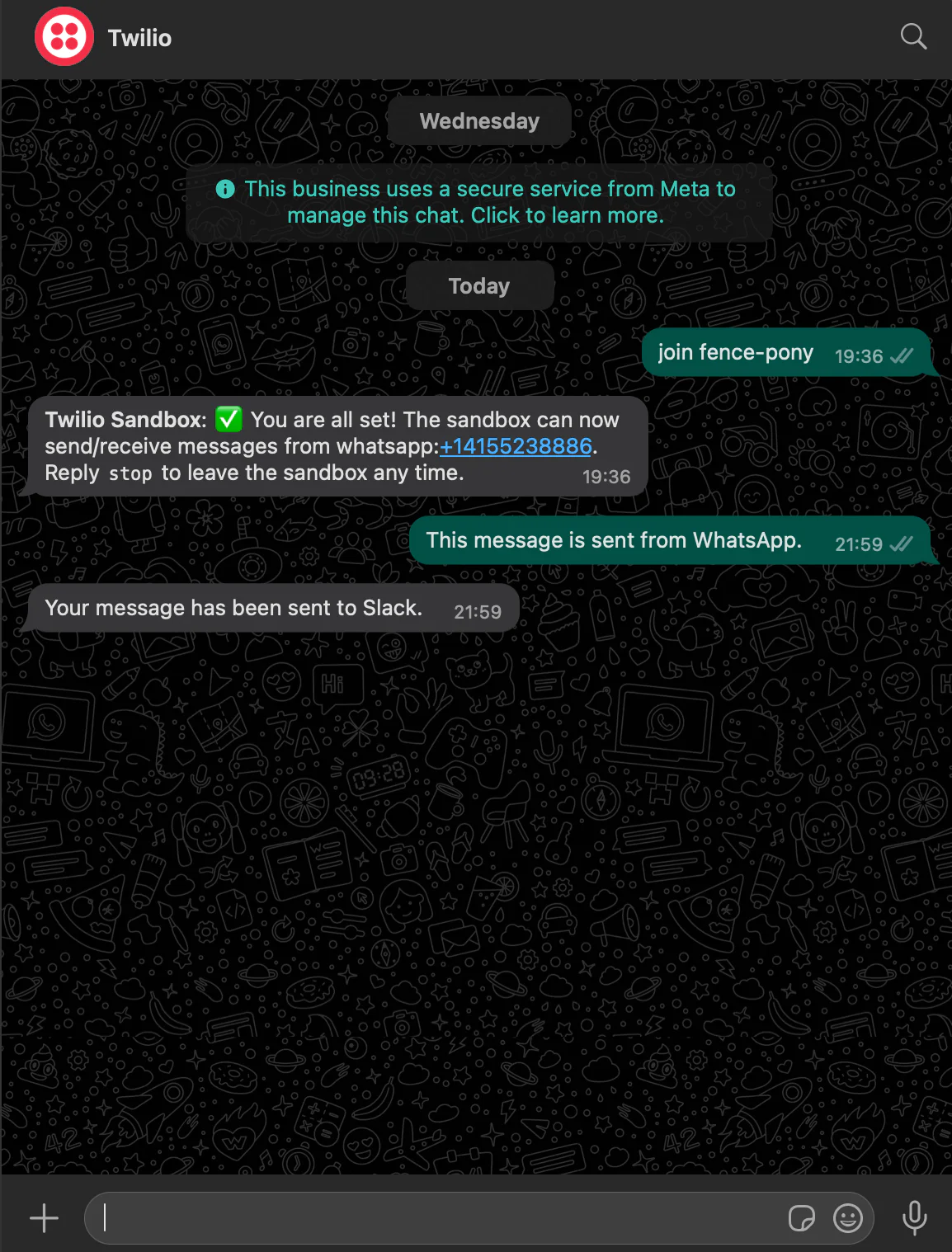
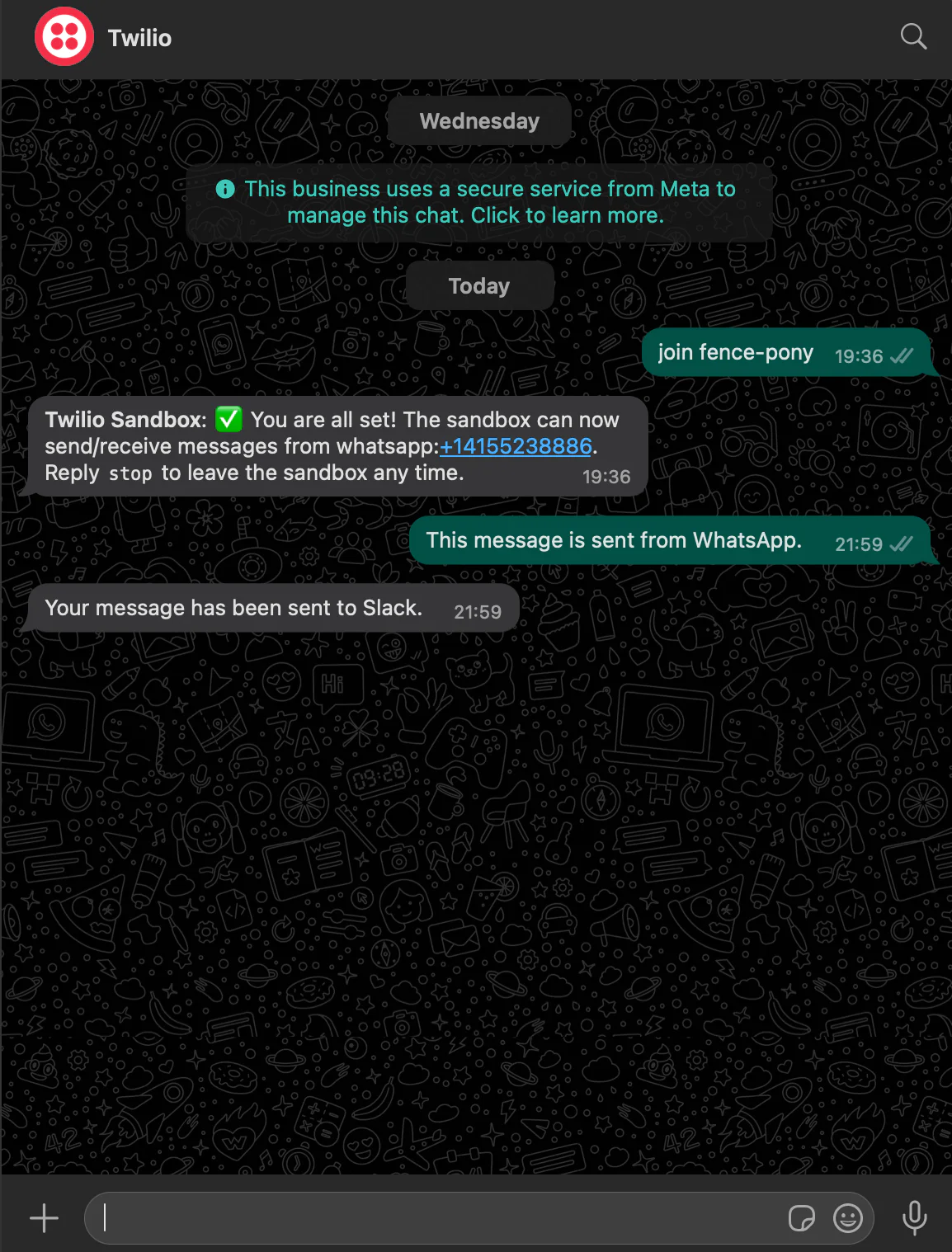
After sending the message, go to your Slack workspace. Under your selected channel, you will see the message sent from WhatsApp, as shown in the screenshot below.
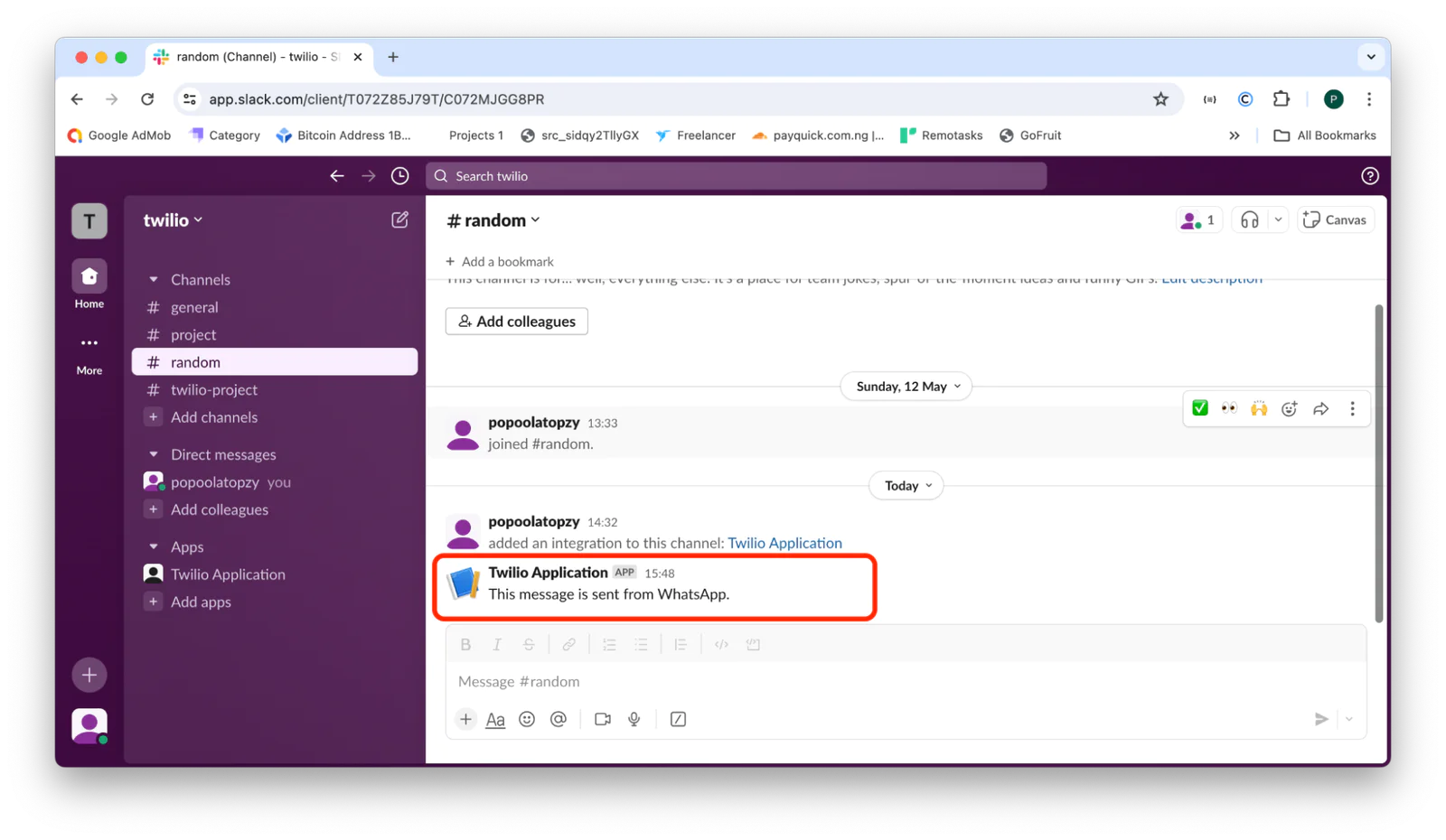
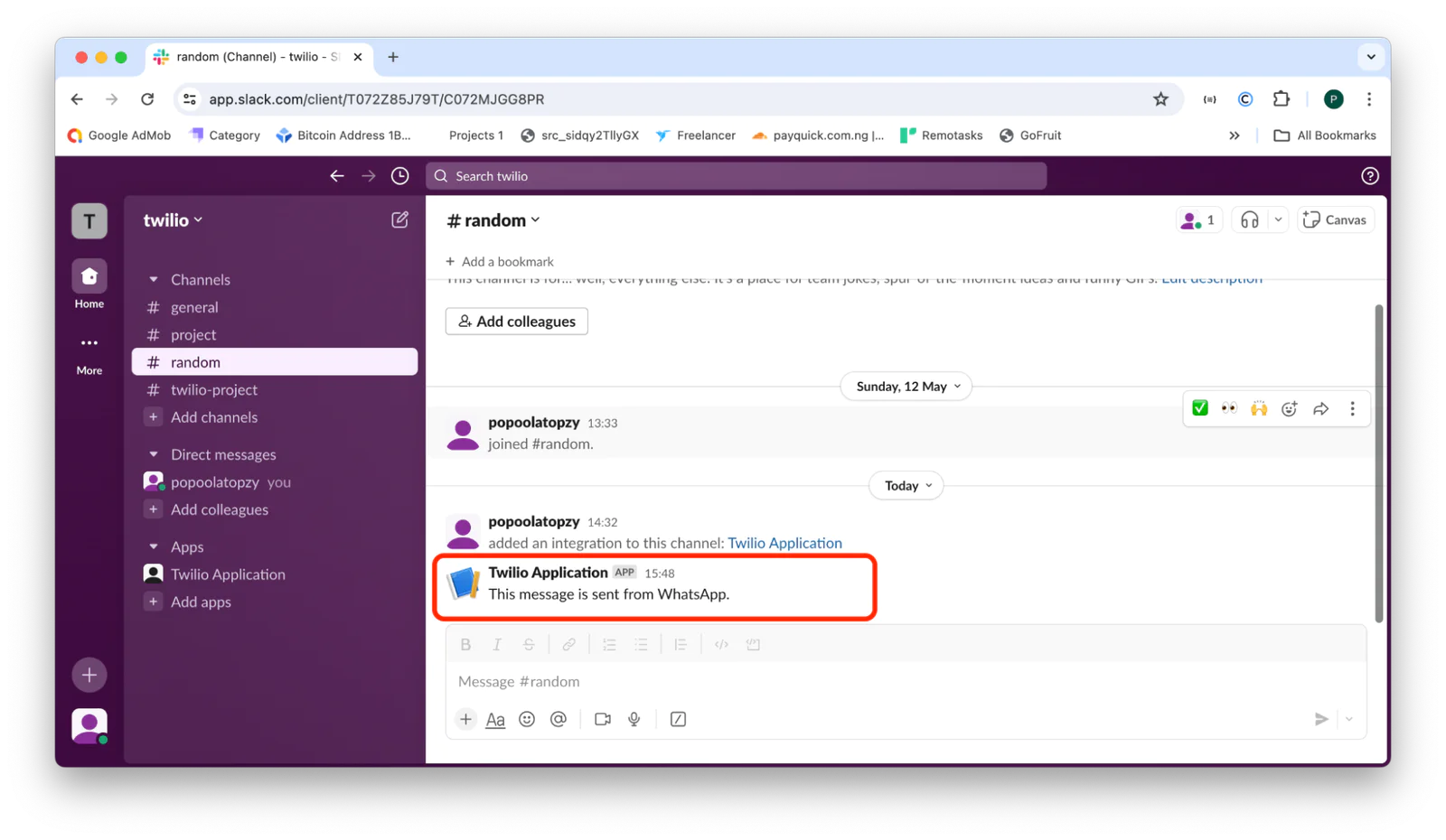
That is how to send messages from WhatsApp to Slack using Twilio and CakePHP
In this tutorial, you learned how to set up a CakePHP application to send messages from WhatsApp to a Slack channel using the Twilio WhatsApp API. This integration showcases the power and flexibility of combining Twilio, Slack, and CakePHP to streamline communication workflows across different platforms.
Popoola Temitope is a mobile developer and a technical writer who loves writing about frontend technologies. He can be reached on LinkedIn.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.