Sending Emails in Python with Twilio SendGrid Dynamic Templates
Time to read: 3 minutes
Whether it's for a Marketing Campaign or simply because you want to send more complex, professional-looking emails where you don't have to write HTML by hand, Twilio SendGrid has a feature called Dynamic Templates that you can use to send rich emails with dynamic content which you can update anytime in the Design Editor. In this post, I'll show you how.
Prerequisites
If you do not already have one, create a SendGrid account. For this tutorial, you can choose the free tier.
We will use the example of sending a marketing email to let people know about the Mars Rover taking a selfie.
Sending an email with Twilio SendGrid
We'll start by using the basic example of sending an email with inline HTML rather than a Dynamic Template. Before you can write code for emails using the Twilio SendGrid API, you'll need to create an API key if you don't already have one.
On your command line, set your API key to an environment variable named SENDGRID_API_KEY
, and install the Twilio SendGrid helper library for Python using the command pip install sendgrid
before moving on.
Using this code, we can send an email with a link to a picture taken by the Mars Rover:
That's great… but nobody wants to write HTML by hand. Let's move on using Dynamic Templates.
Creating a Dynamic Template
In your SendGrid Dashboard, click on Dynamic Templates under the Email API section:
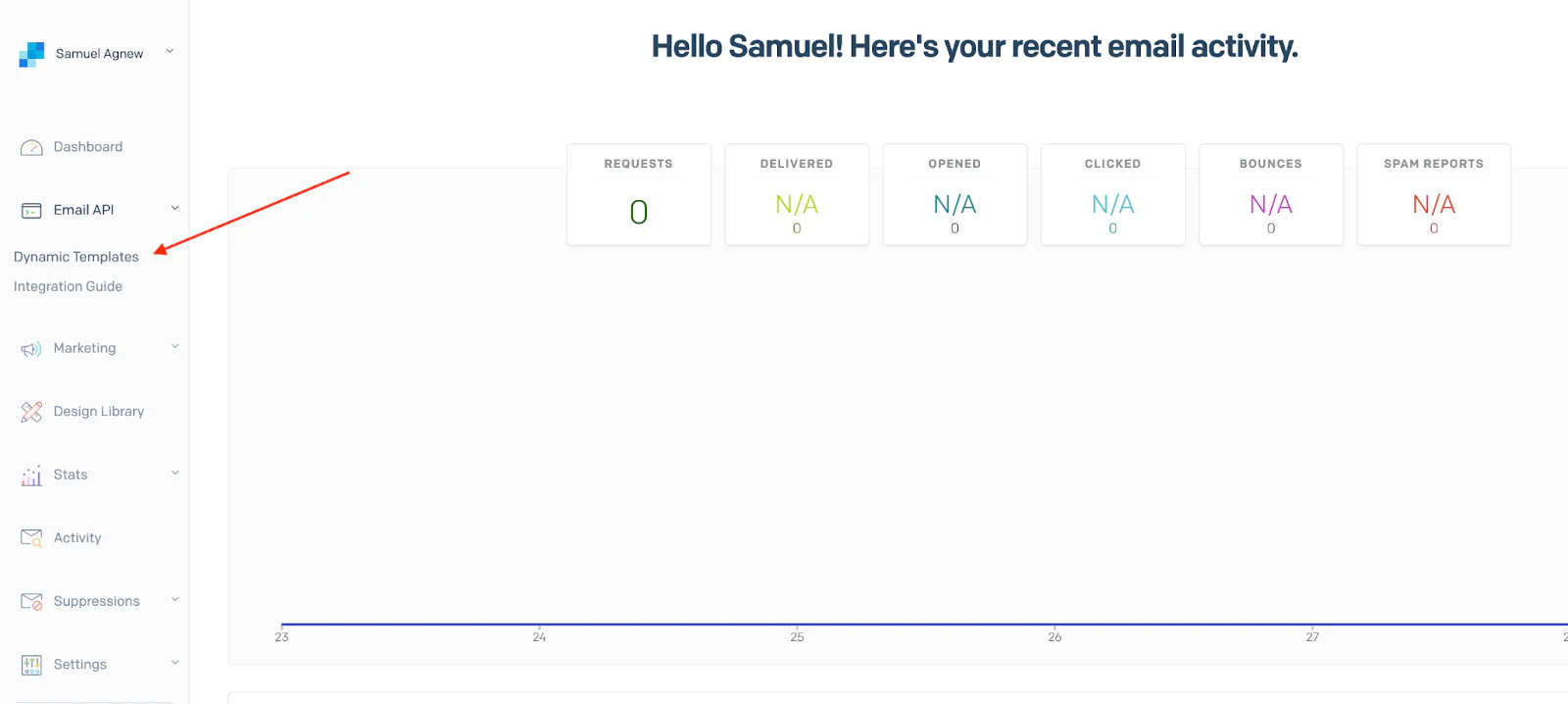
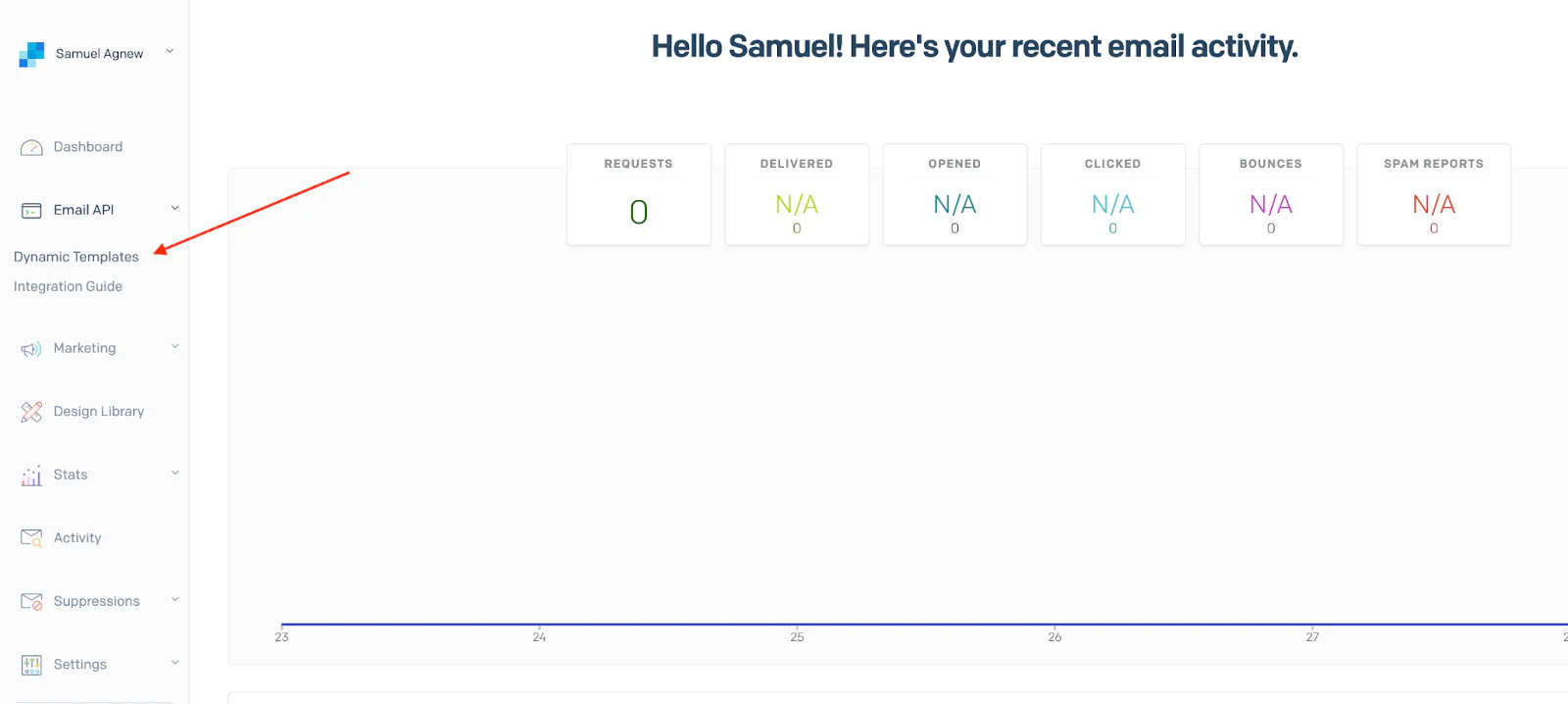
Click Create a Dynamic Template and give it whatever name you want. Once you have the template created, click to add a Version to it, so you can start editing the content of the email. You can update this template by creating new versions in the future, so you can tweak it for different campaigns.
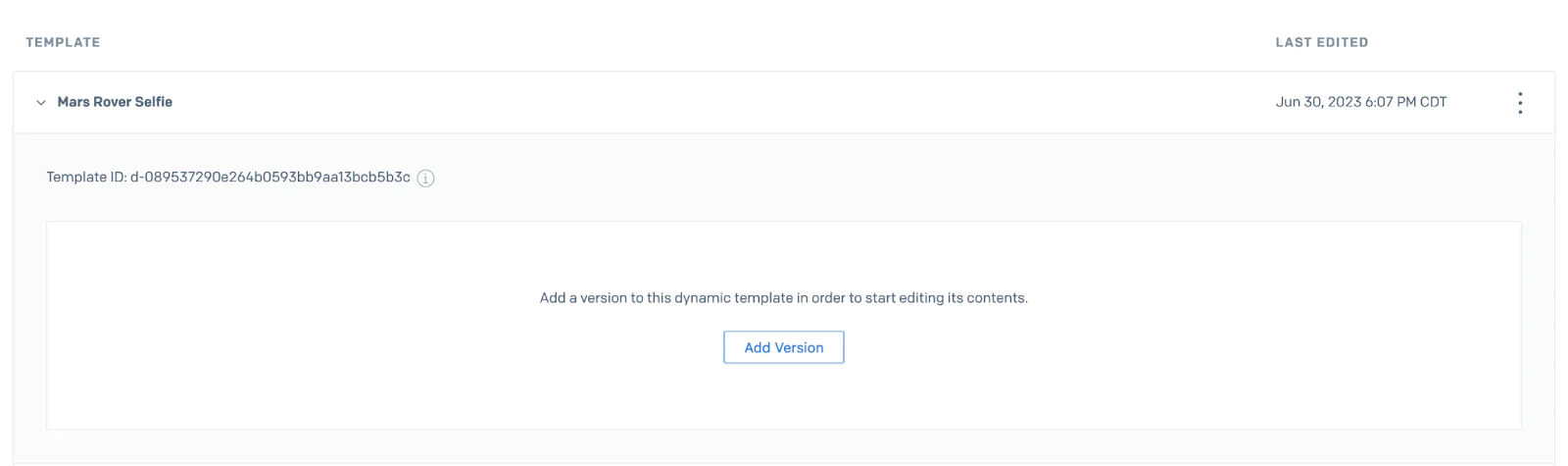
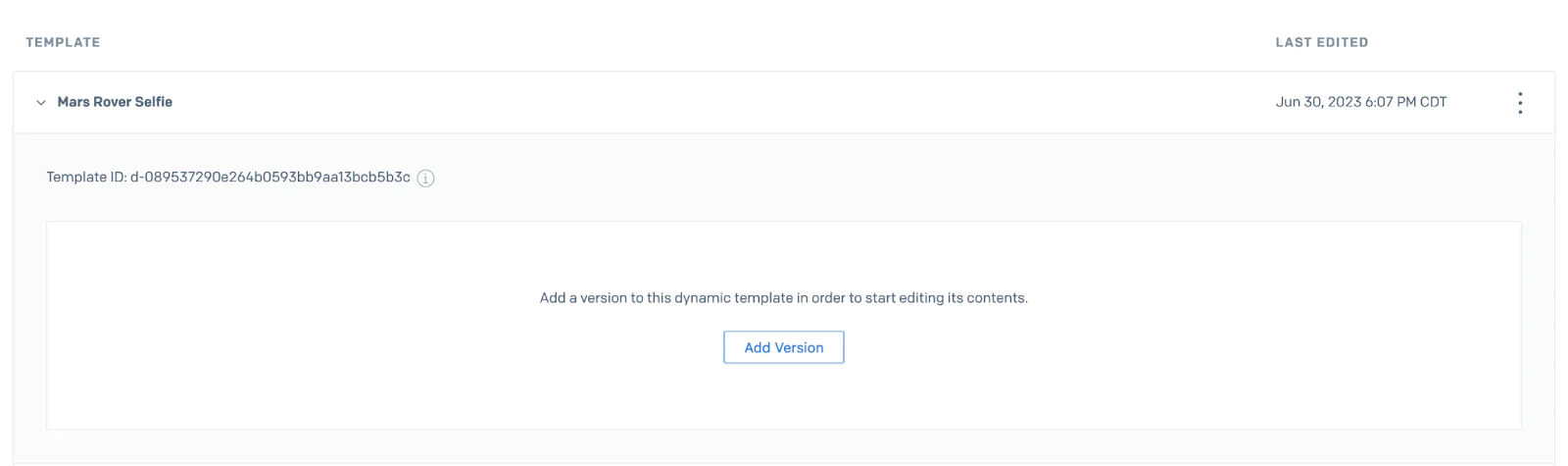
Designing your Template
You can edit the email with the Design Editor or directly through code. We will use the Design Editor for this example, but you can get more granular by tweaking the HTML when needed.
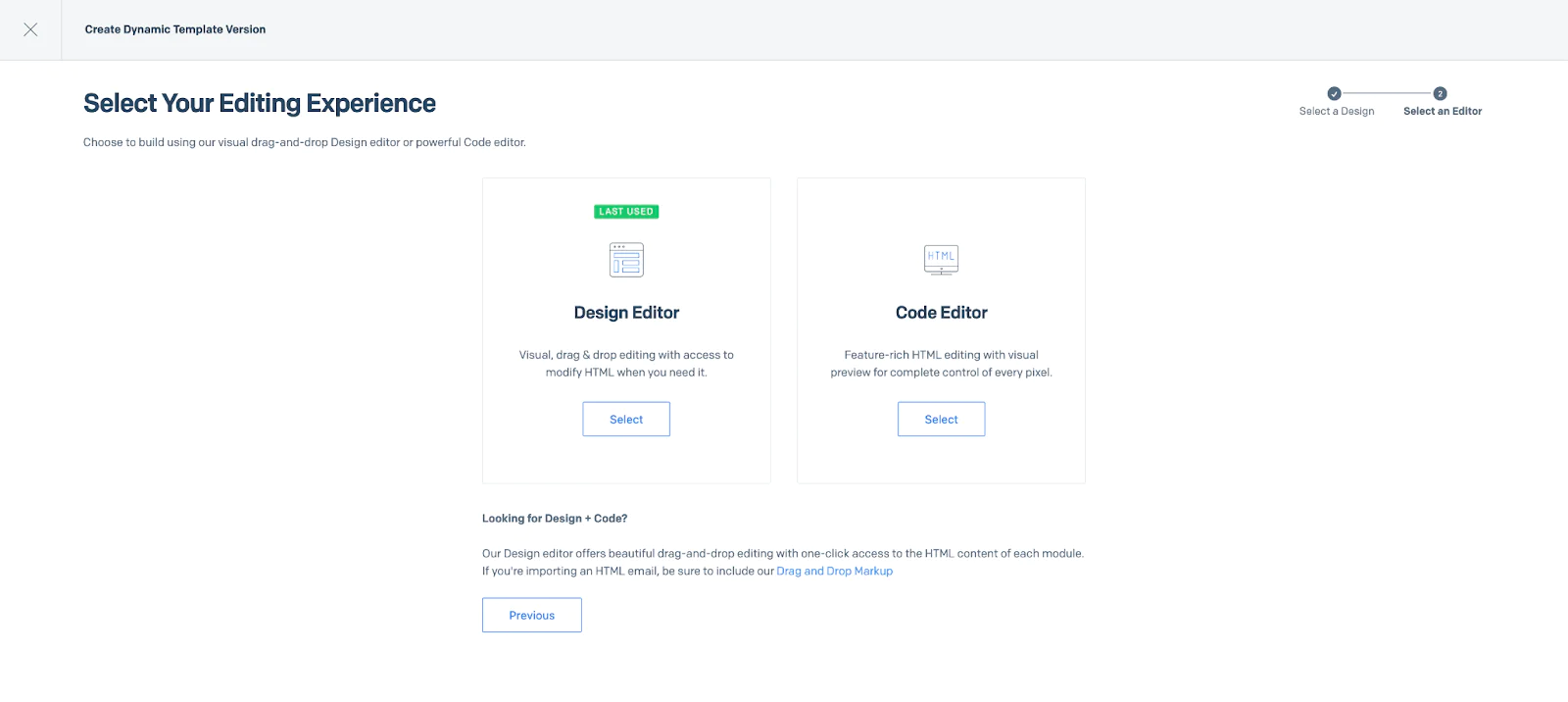
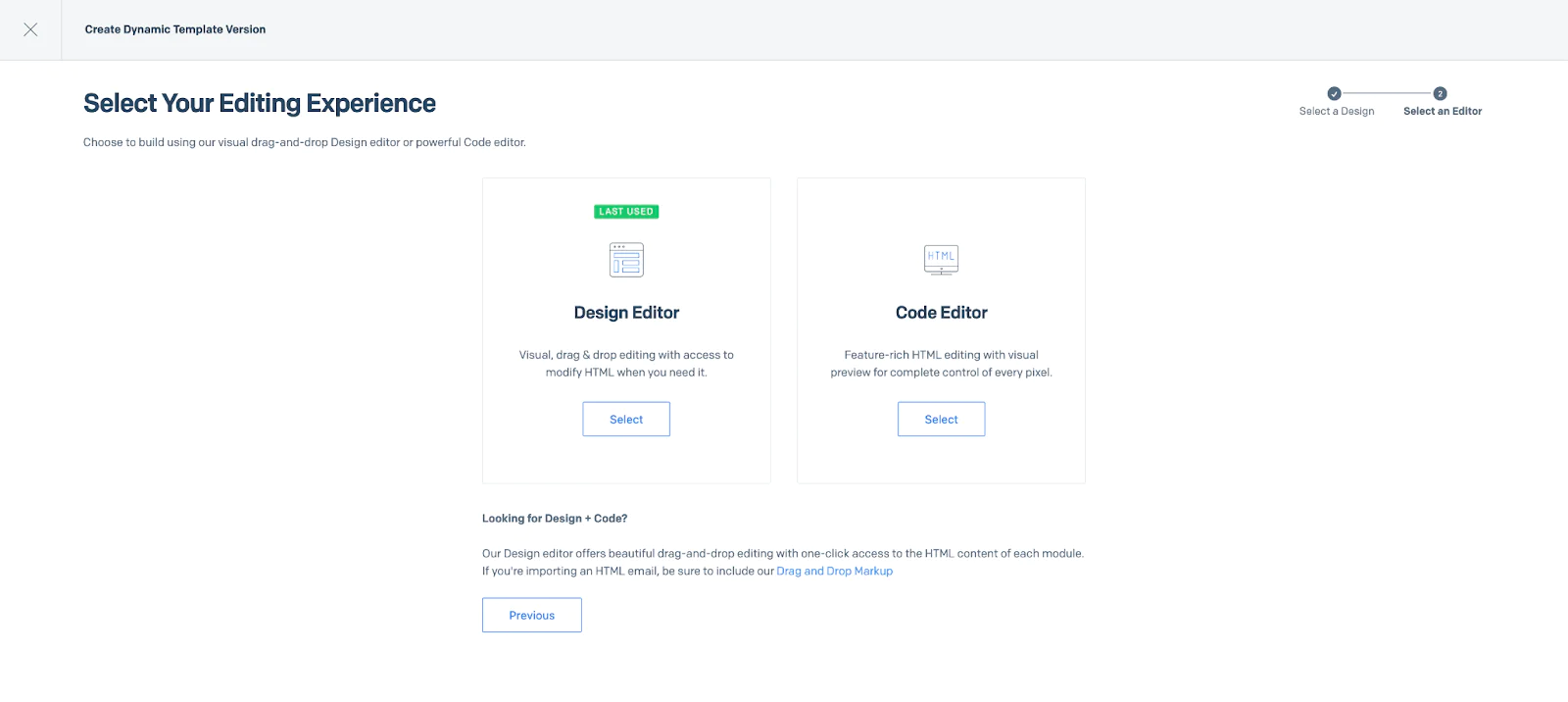
Give your template a Version Name and a Subject line, which will be the email's subject when it is sent.
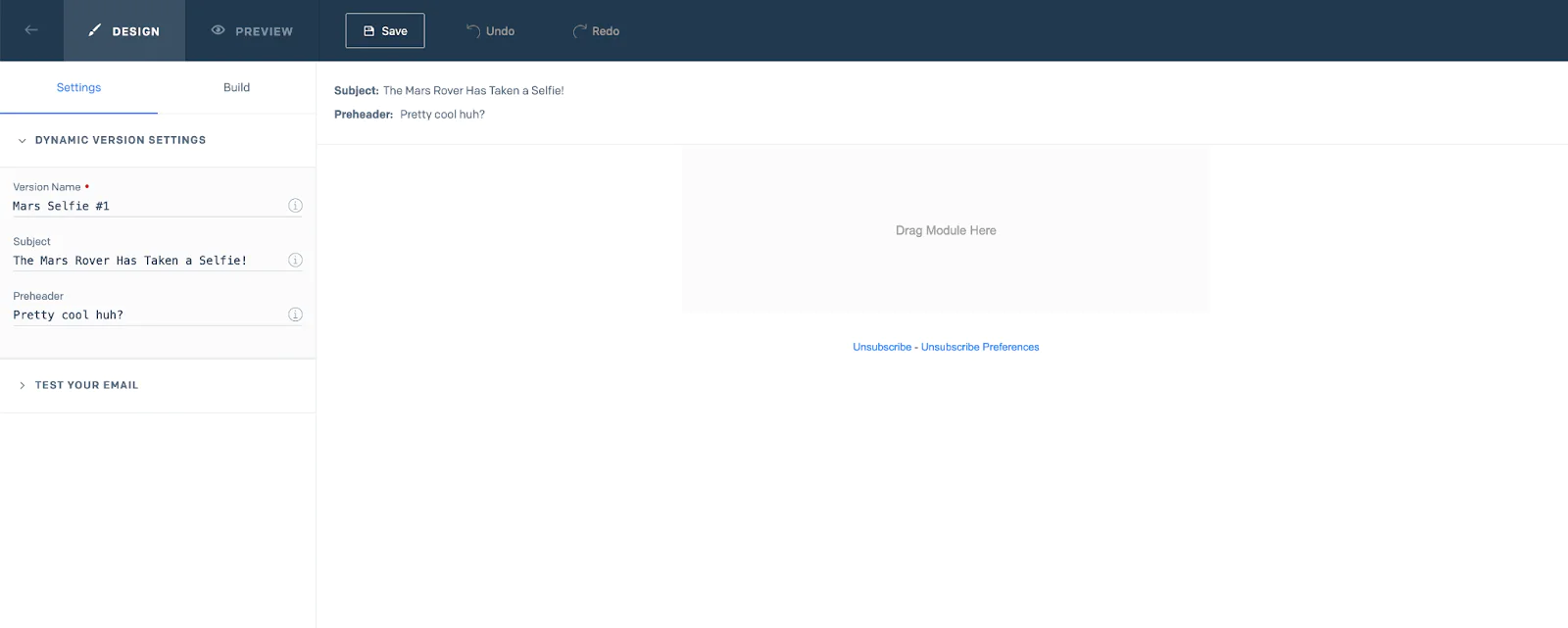
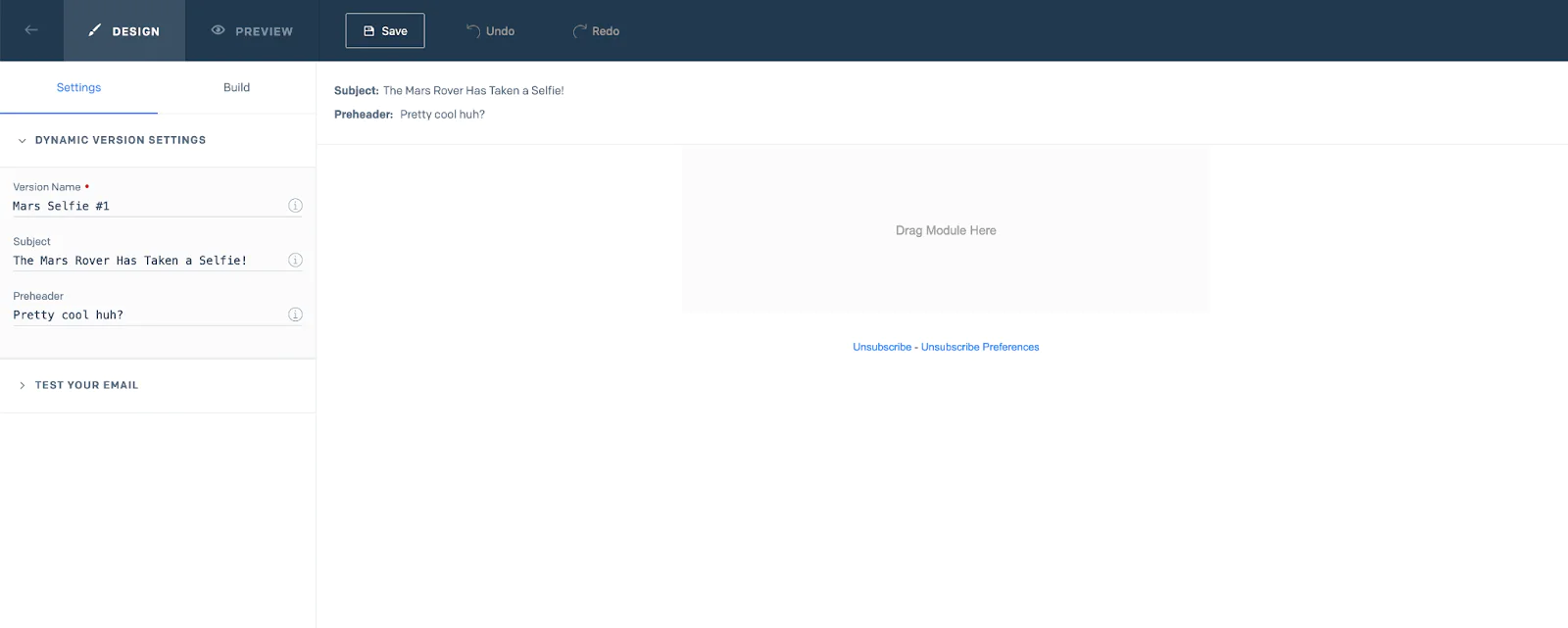
The Design Editor has various features that you can use via a drag-and-drop interface, for example, inserting images and text, buttons, code blocks, unsubscribe preferences, and so on. The GIF below demonstrates these features in action.
For this email, we will add an image of the selfie that the Mars Rover took, a text block explaining the image, and a link to a technical blog post about how to use the Mars Rover API with SendGrid.
Sending an email with a Template ID
Before you can send an email, you'll have to create a Sender. This requires you to enter things such as your contact information and mailing address to comply with anti-spam regulations. If your email address matches one of your verified domains, you don't have to validate that email address at this step.
Once you have a Sender, you can send this email using the SendGrid Dashboard or with code. If you want to send this email via the API, use the Template ID in your code. You can copy and paste the Template ID by viewing your Dynamic Templates in your SendGrid account.
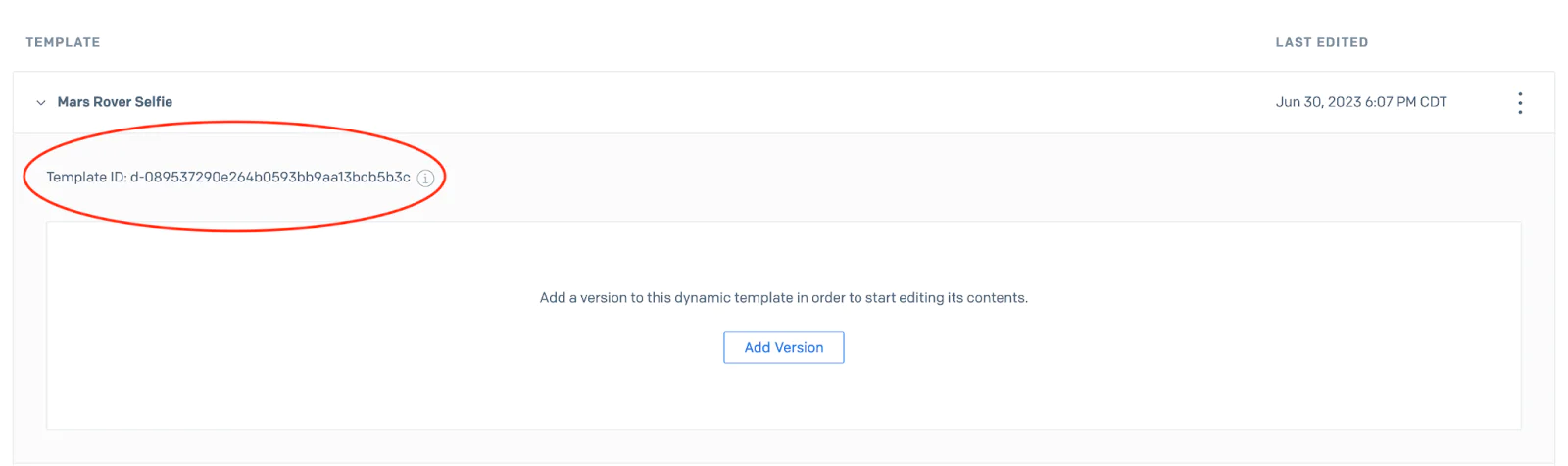
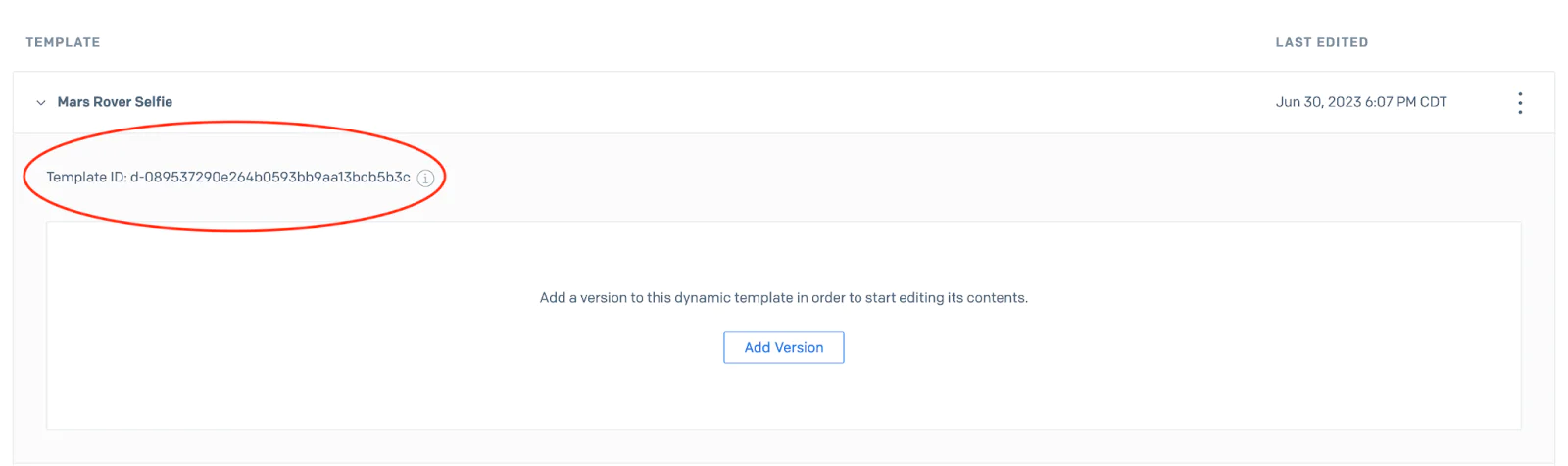
The following code sample displays how to send an email using a Dynamic Template in Python using this Template ID:
Sending an email with dynamic content in it
This previous example does not contain any changing content, but what if we wanted to send an email with sections that change based on certain factors, such as who it is being sent to or when it is being sent? Don't worry; I've got you covered.
You will, however, have to choose the dynamic sections up front. You use Handlesbars syntax to make the content dynamic in your templates.
In the previous code example, the dynamic_template_data
in the message are "key: value" pairs representing data you want to display in the email. You can read more about this on the SendGrid docs.
Let's make it so the title of the email gets passed in through code. Go back to your template in the Design Editor, and change the header section at the top of the email to "{{ header }}".
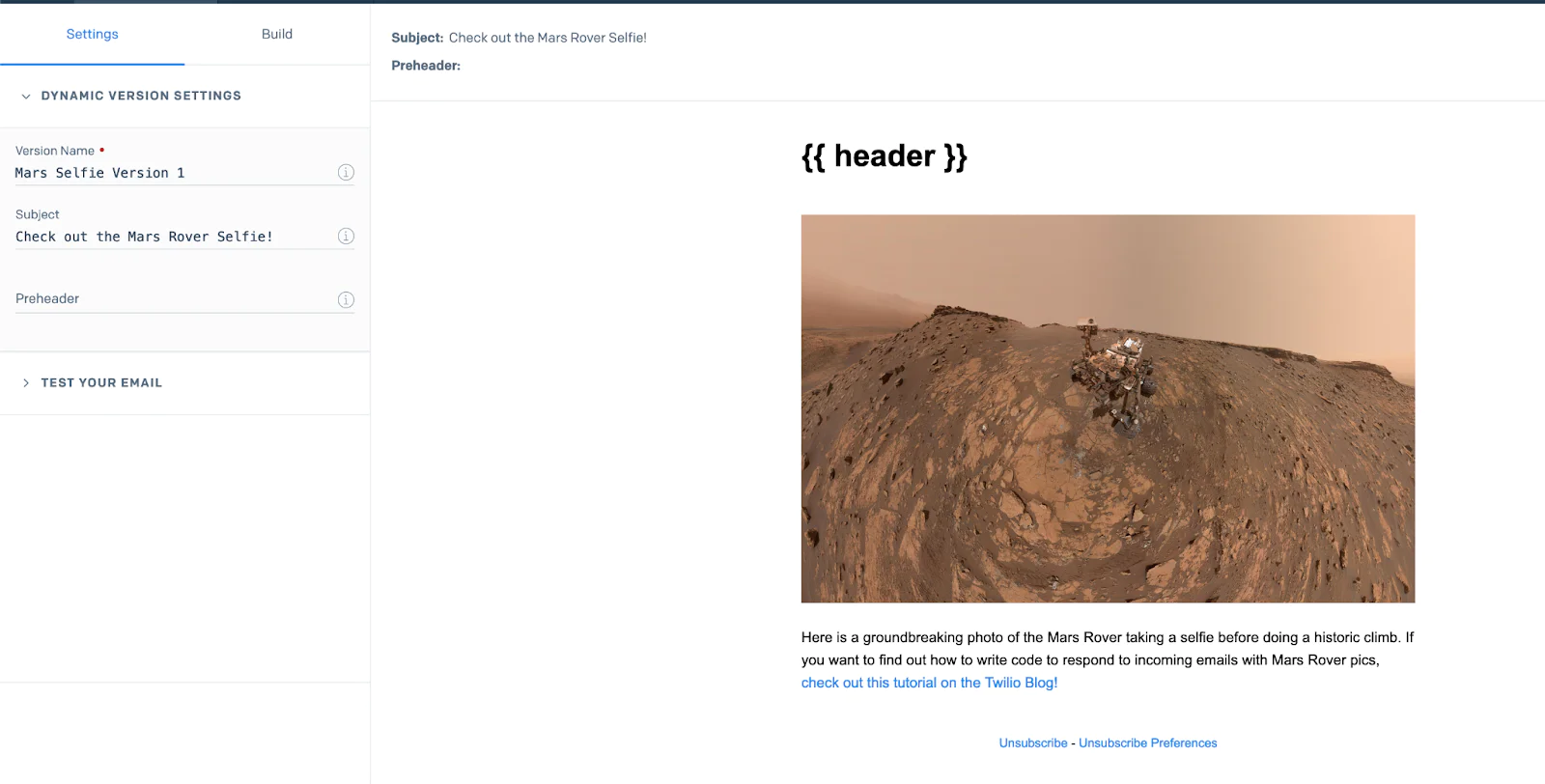
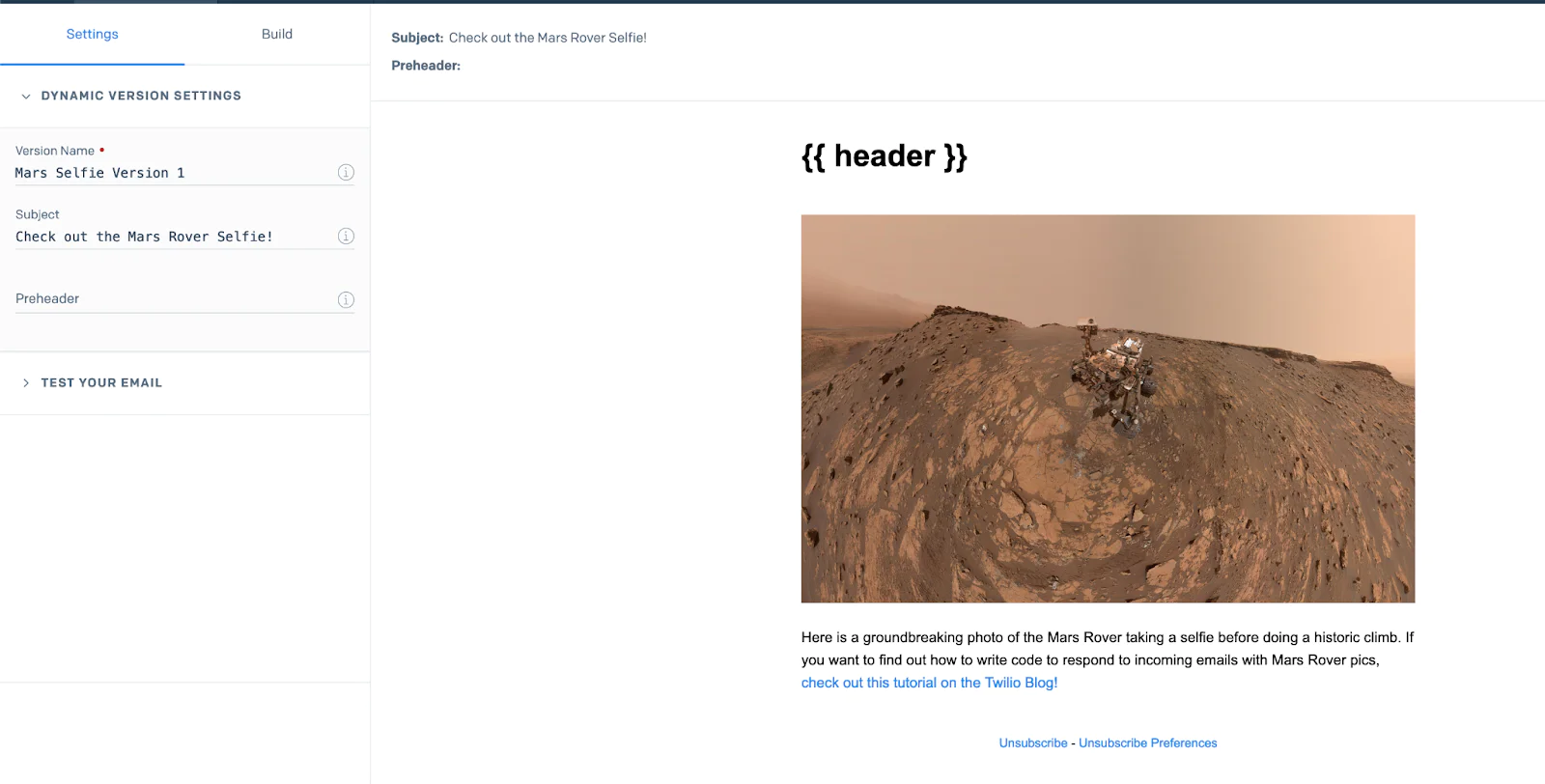
Now, in your code, you can change the line message.dynamic_template_data = {}
to message.dynamic_template_data = { 'header': 'Wow check out this selfie!!' }
and run the code as is. You can change anything in the template, and as long as you pass it through in the dynamic_template_data
dictionary, it can be whatever value you want.
Now that you know how to use the Design Editor and Twilio SendGrid Dynamic Templates, you can create custom emails for marketing campaigns. I can't wait to see what you build.
- Email: sagnew@twilio.com
- Twitter: @Sagnewshreds
- Github: Sagnew
- Twitch (streaming live code): Sagnewshreds
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.