How to Send an Email with Go and SendGrid in 30 Seconds
Time to read: 3 minutes
SendGrid is all about delivering exceptional email experiences. In this tutorial, you'll learn how to send your first email with Go and SendGrid in 30 seconds. To do this, you'll use the official Twilio SendGrid Golang API library to save yourself a lot of time and effort.
Ready? Let's get started!
Tutorial requirements
To follow along with the tutorial, you're only going to need three things:
- A SendGrid account. If you are new to Twilio Sendgrid you can create a free account, which allows you to send 100 emails per day.
- Go, version 1.18 or newer. You can download an installer from the official Go website.
- An email address to receive the email.
- An email address to send the email which has a verified identity.
Create the project directory
Create a new directory named send-email-with-sendgrid in your Go workspace (somewhere inside $GOPATH/src
), and change into it by running the following commands.
Import the required dependencies
The code will make use of two external libraries, ones not part of Go's standard library. These are GoDotEnv and SendGrid's Golang API library. To import them, in the terminal, run the following two commands.
Write the code
Then create a new file named send-email.go and paste the code below into the new file.
The code starts off by importing all of the required libraries. Then, it uses GoDotEnv to import the required environment variables from .env, which you'll create shortly. Following that, it initialises a series of variables, one for the recipient, sender, and email subject and body, both in plain text and HTML formats.
After that, it initialises a new email message with those variables. With the email message ready, a SendGrid Client
object, which simplifies interacting with SendGrid's API is initialised and an attempt is then made to send the email.
If sending the email fails, "Unable to send your email" is printed to the terminal along with the specific reason why, returned from the API. Otherwise, if the status code from the response is 200, 201, or 202, the email is considered sent, and "Email sent!" is printed to the terminal.
Retrieve the required environment variables
The last thing to do is to retrieve the environment variables which the code relies upon. There are five of them:
SENDGRID_API_KEY | This authenticates your requests to SendGrid's API, allowing the code to interact with it. |
SEND_FROM_NAME | The human-readable name of the sender. |
SEND_FROM_ADDRESS | The email address of the sender. |
SEND_TO_NAME` | The human-readable name of the recipient. |
SEND_TO_ADDRESS` | The email address of the recipient. |
As previously mentioned, they'll be retrieved from .env by using the GotDotEnv library. So, create a new file named .env, and paste the following code in it.
Then, set the sender and recipient's details to the names and email addresses that you want to use.
Create a SendGrid API key
Now, you need to create a SendGrid API key. To do that, login to your SendGrid account. Then, in the SendGrid Dashboard, under "Settings > API Keys", click "Create API Key", in the upper right-hand corner.
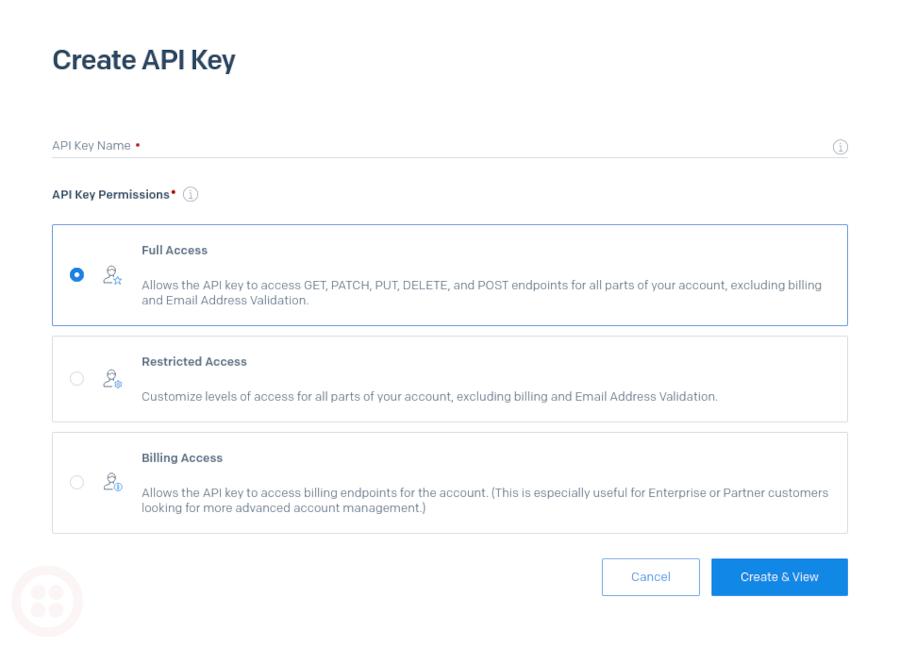
From there, add a meaningful name for the API key, such as "Send an email with Golang", and click "Create & View" in the lower right side of the page.
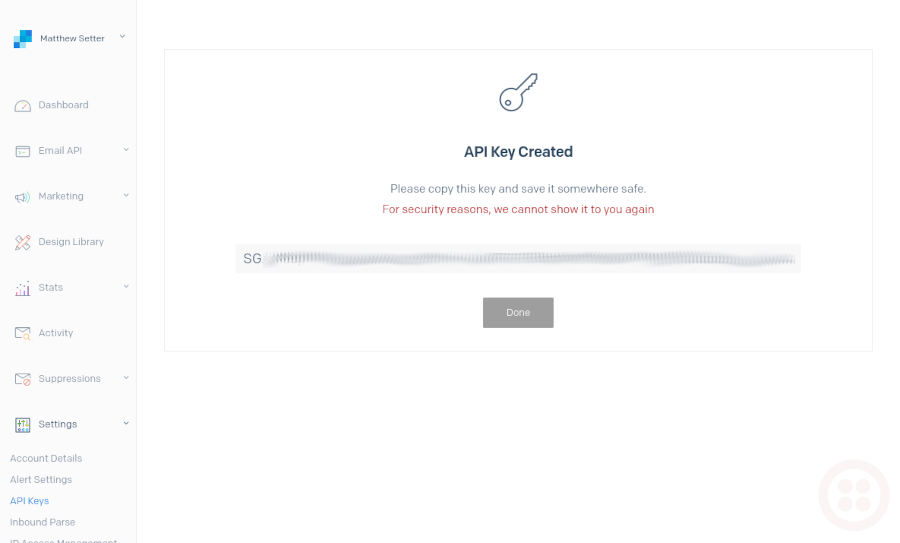
You'll then be shown your API key. In .env, replace <SENDGRID API KEY>
with the new API key. It's important that you do this now, as once you leave the page you won't be able to see or copy the key again.
Send the email
Now, you're ready to send an email. To do that, run the following command from the command line.
If all went well, you'll see "Email sent!" written to the terminal, similar to the screenshot below.
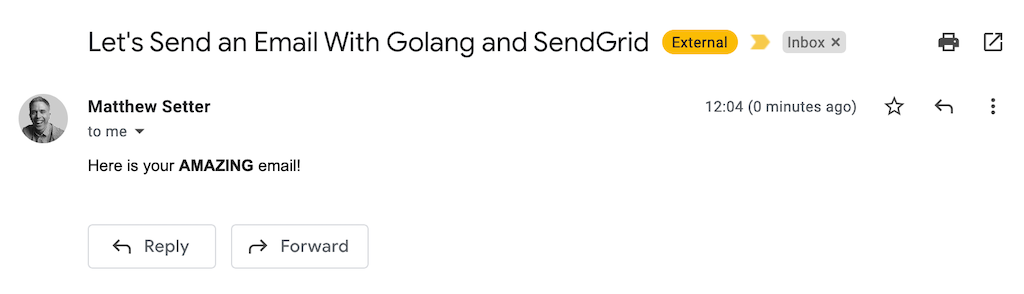
If not, from the SendGrid Dashboard, under "Suppressions > Blocks", have a look in the Blocks table to see if you have any records listed with the sender's email address, as in the example below. If so, then you're likely not allowed to send emails with that email address yet.
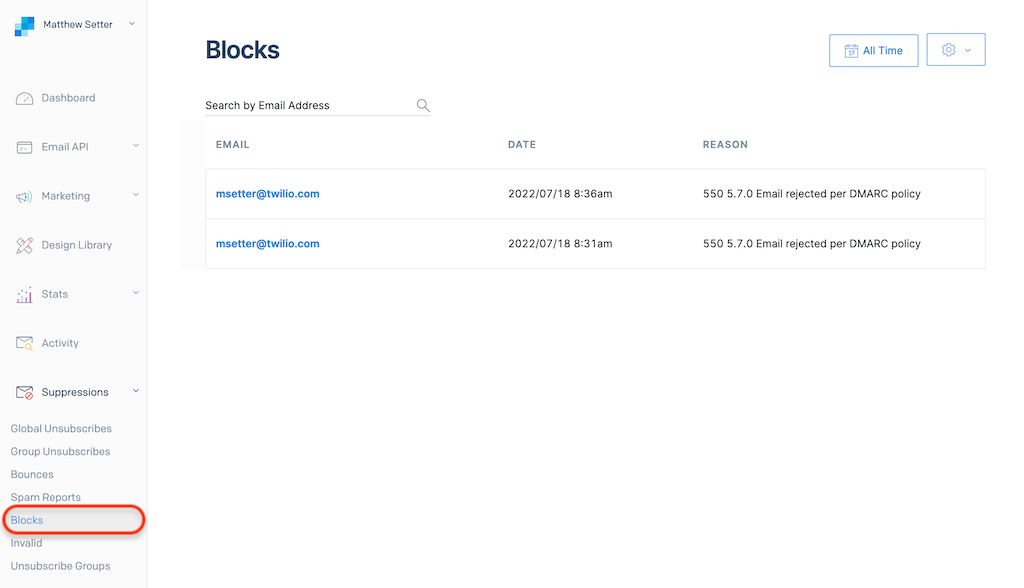
That's how to send an email with Go and SendGrid in 30 seconds
I hope that this tutorial has given you a taste for just how simple it can be to send an email when using the official Twilio SendGrid Golang API library. Have a deeper look at the library and the API documentation to get a fuller understanding of the available functionality.
Otherwise, I can't wait to see what you build with Golang and SendGrid!
Matthew Setter is a PHP and Go editor in the Twilio Voices team, and a PHP and Go developer. He’s also the author of Mezzio Essentials and Deploy With Docker Compose. You can find him at msetter@twilio.com. He's also on LinkedIn and GitHub.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.