Respond to Incoming SMS with Python and GCP Cloud Functions
Time to read:
Respond to Incoming SMS with Python and GCP Cloud Functions
Have you ever wanted to build an app that automatically responds to text messages? Since I love working with serverless technologies, I wanted to share how you can create a serverless SMS responder using Google Cloud Functions.
What We'll Build
We're going to create a serverless function that handles incoming text messages. The best part? You don't need to manage any servers - Google Cloud Functions takes care of all that for you.
By the end of this tutorial, you'll have:
- A working SMS responder using Twilio's API
- A serverless function deployed on Google Cloud
- A foundation for building more complex messaging applications
Prerequisites
Before we begin, make sure you have the following:
- A Twilio Account: Sign-up here
- A Google Cloud Account: Register at Google Cloud's website to open an account
- Python 3.9+ installed locally
- Google Cloud CLI installed and configured
- A code editor (VS Code, PyCharm, etc.)
Once you have all these prerequisites in place, you're ready to start building.
Understanding the building blocks
Let's break down the key components we'll be working with:
Twilio's Webhook system
Think of webhooks as real-time notifications between systems. When someone texts your Twilio number, Twilio immediately pings your application with all the message details. Your application then decides how to respond.
Twilio uses two main types of webhooks for SMS:
- Incoming Message Webhook: Gets triggered when someone texts your number
- Status Callback Webhook: Lets you know if your message was delivered successfully
These webhook calls expect responses in TwiML (Twilio Markup Language), which looks like this:
Google Cloud Functions
Instead of setting up and maintaining a server to handle these webhooks, we'll use Google Cloud Functions. It's a serverless platform that lets you run code in response to events - perfect for handling incoming messages!
Ready to start building? Let's dive into the setup!
Project Setup
First, let's set up our local development environment:
Create a new project directory:
Create a virtual environment”
Create project files:
Add the following to your .gitignore:
Add the required dependencies to requirements.txt:
Install the dependencies:
Implementing the Function
Create your main.py file with the following code:
This implements the function by using the twilio
package to create a TwiML response that includes a random Programming Joke.
Note: For production deployments, you should validate that requests are genuinely from Twilio using the request signature. See Twilio's Security Documentation for implementation details.
Testing the Function locally
To test locally using the Functions Framework, run the command:
Now you can use curl
or go to localhost:8080
in your browser.
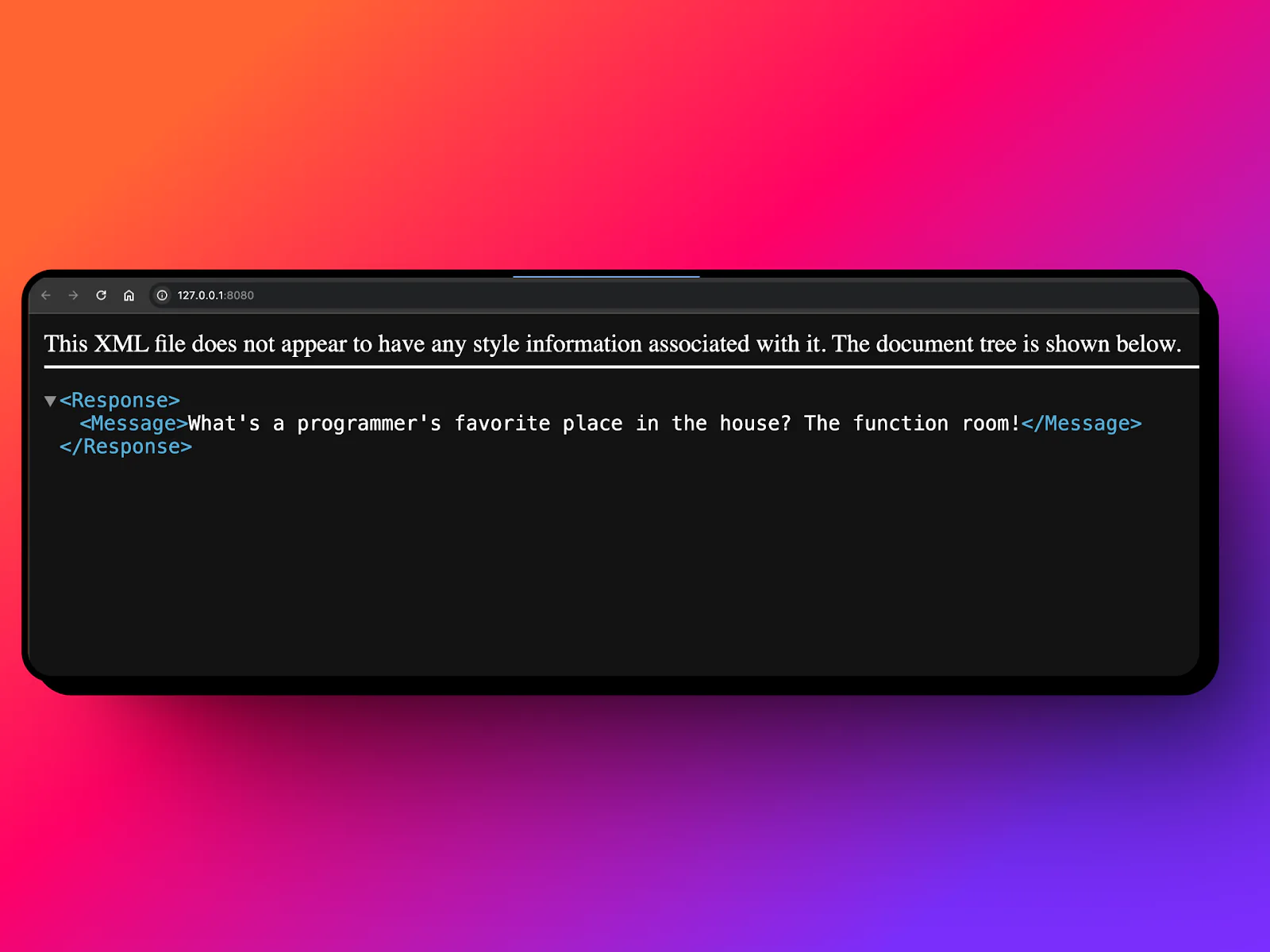
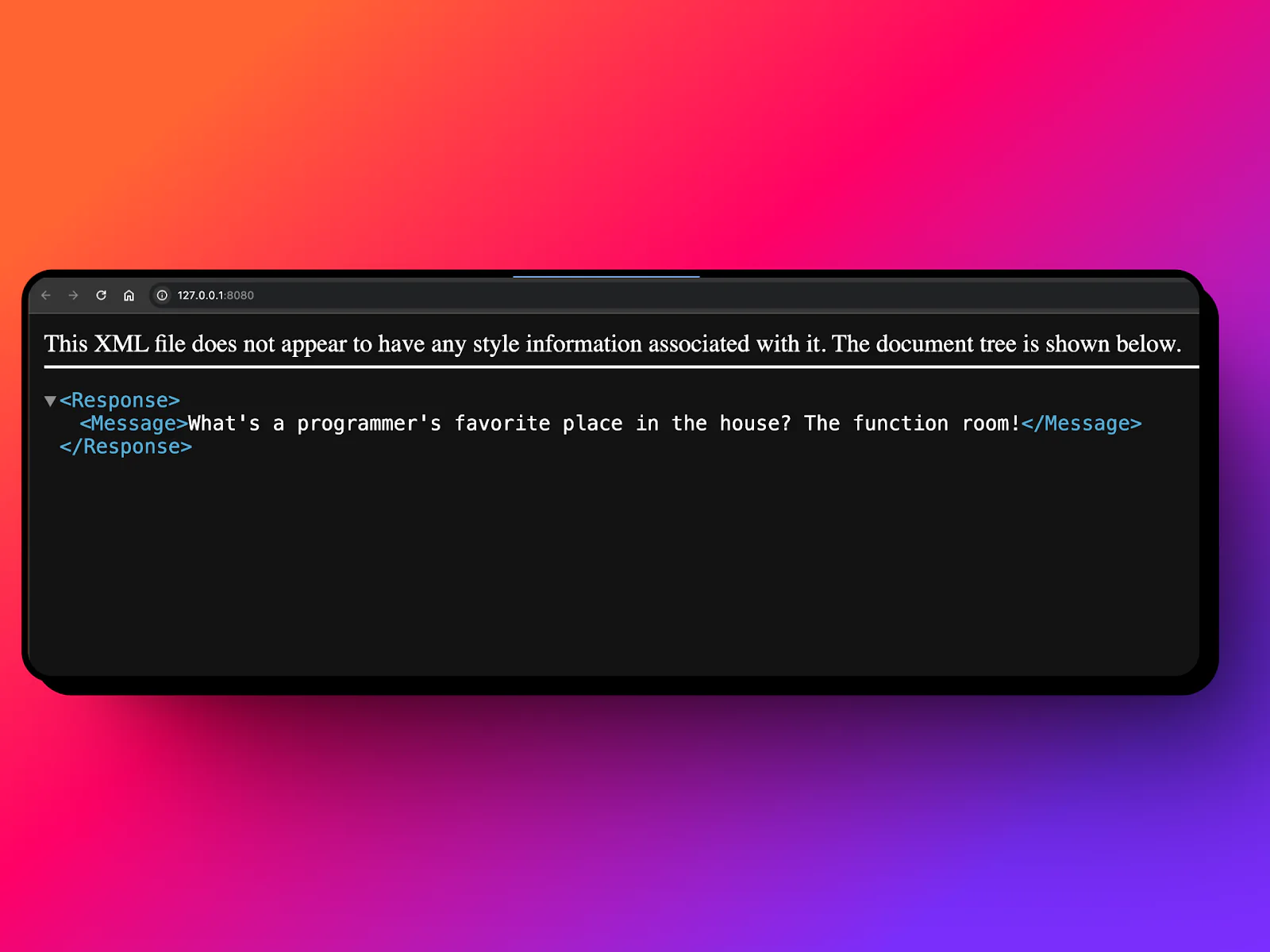
Deploying to Google Cloud
To deploy the function to Google Cloud, make sure you have gcloud CLI installed and configured. Alternatively, you can do this in the Google Cloud Console as well.
Now, make sure you have the right project selected:
After you have configured your project, deploy the function:
This command deploys our local function to cloud with configuration:
- Using Python 3.9 runtime
- HTTP Triggered function
- Unauthenticate requests are allowed for the function
- Entry point is
receive_sms
If this is your first time using Google Cloud Functions on the GCP project, you may get a prompt about the API not being enabled, enter Y
to enable the API:
Once enabled, it will deploy your local function to the cloud. You will receive a URL for your function. Save this URL as you’ll need it for the Twilio webhook configuration.
[Note]: Based on your Organization restrictions/policies, you might not be able to generate/access the Function URL.
Configuring Twilio
To send a message, first you will need to buy a phone number, ideally from the region you are based in to avoid roaming charges. To do this, go to the Twilio Console and search for a number you’d like to buy.
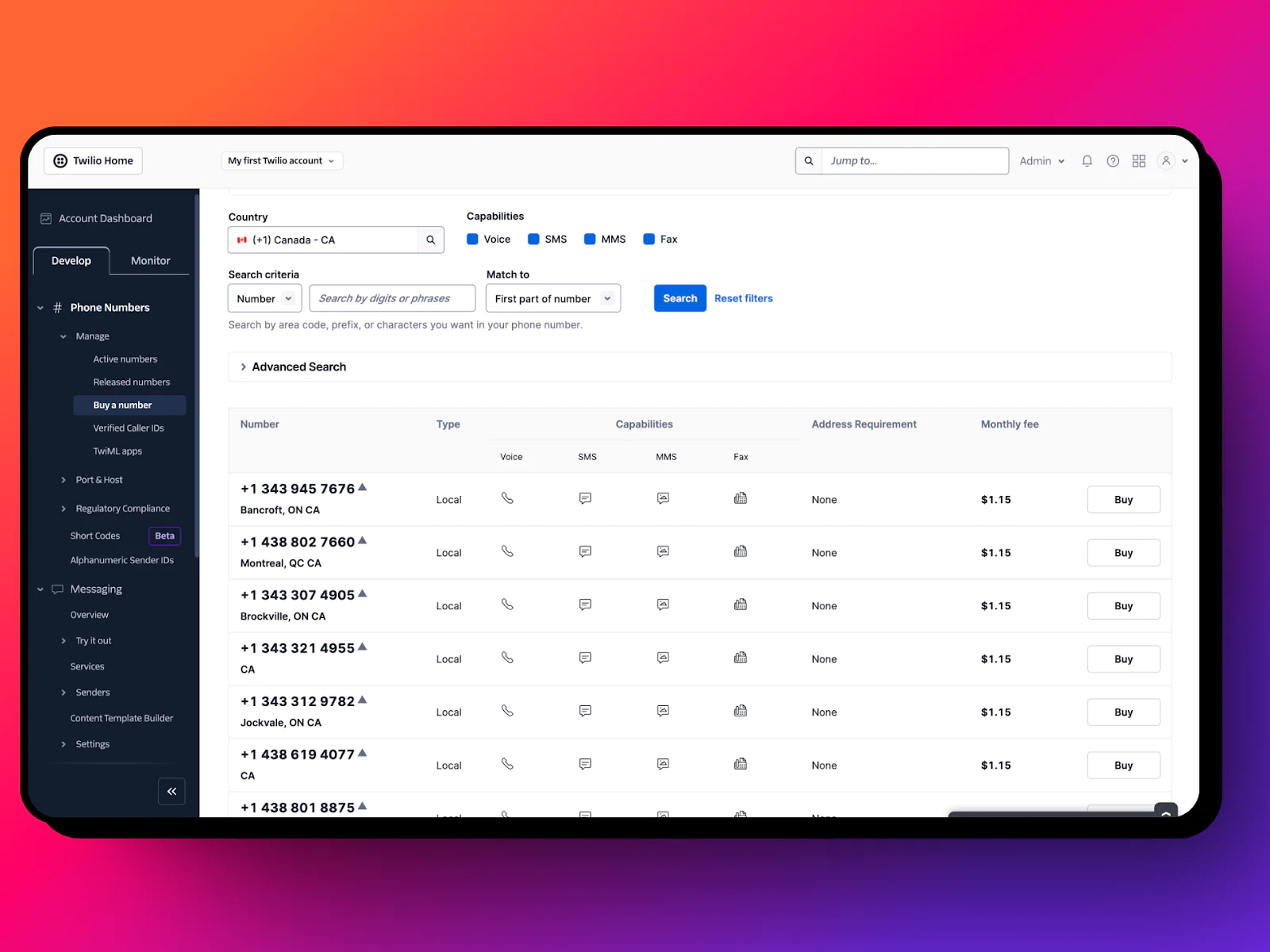
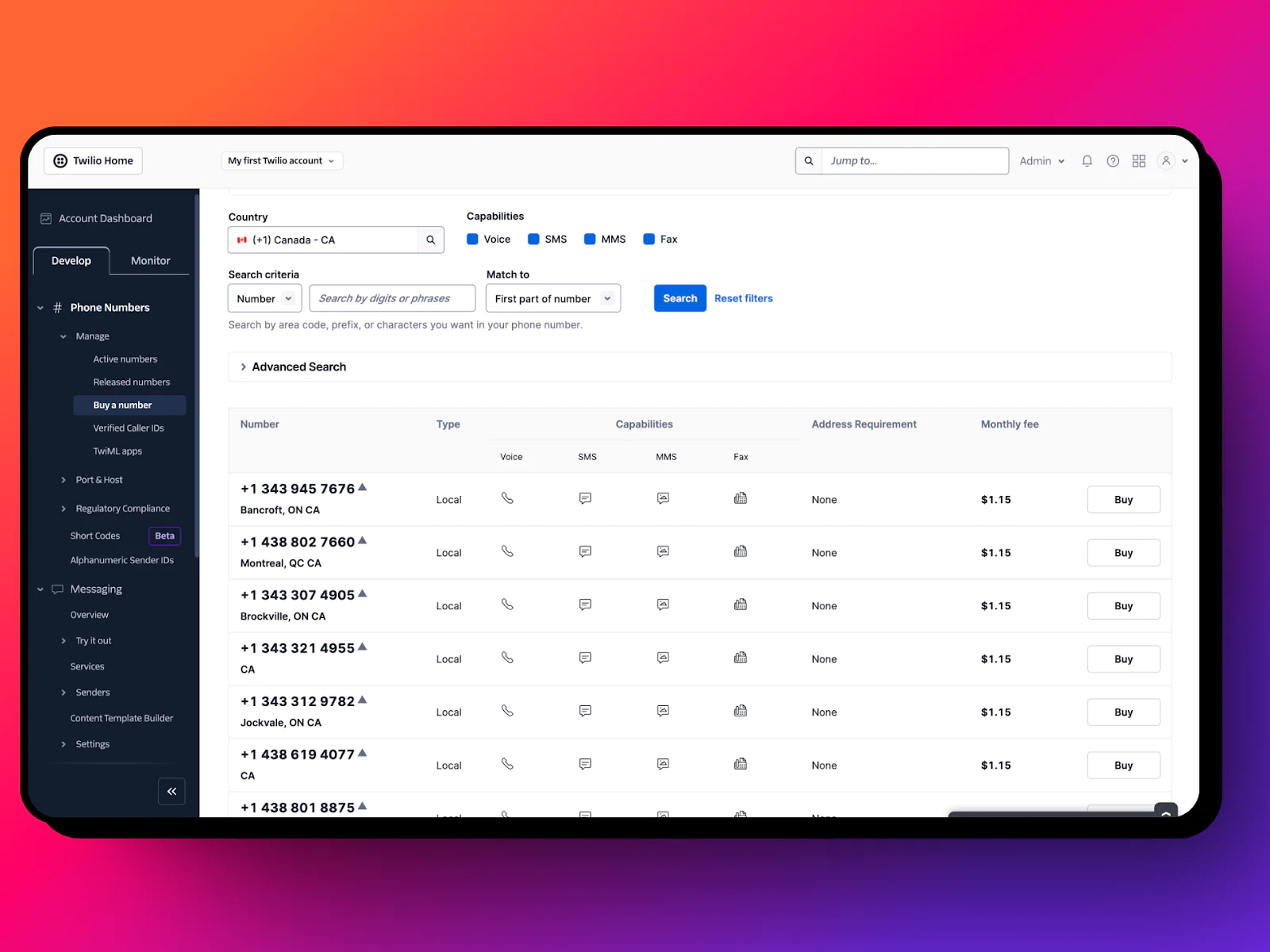
Now let’s configure the number.
- Go to the Twilio Console and navigate to your phone number
- In the Messaging Configuration section, paste your Cloud Function URL as the webhook for incoming messages
- Ensure the HTTP method is set to POST
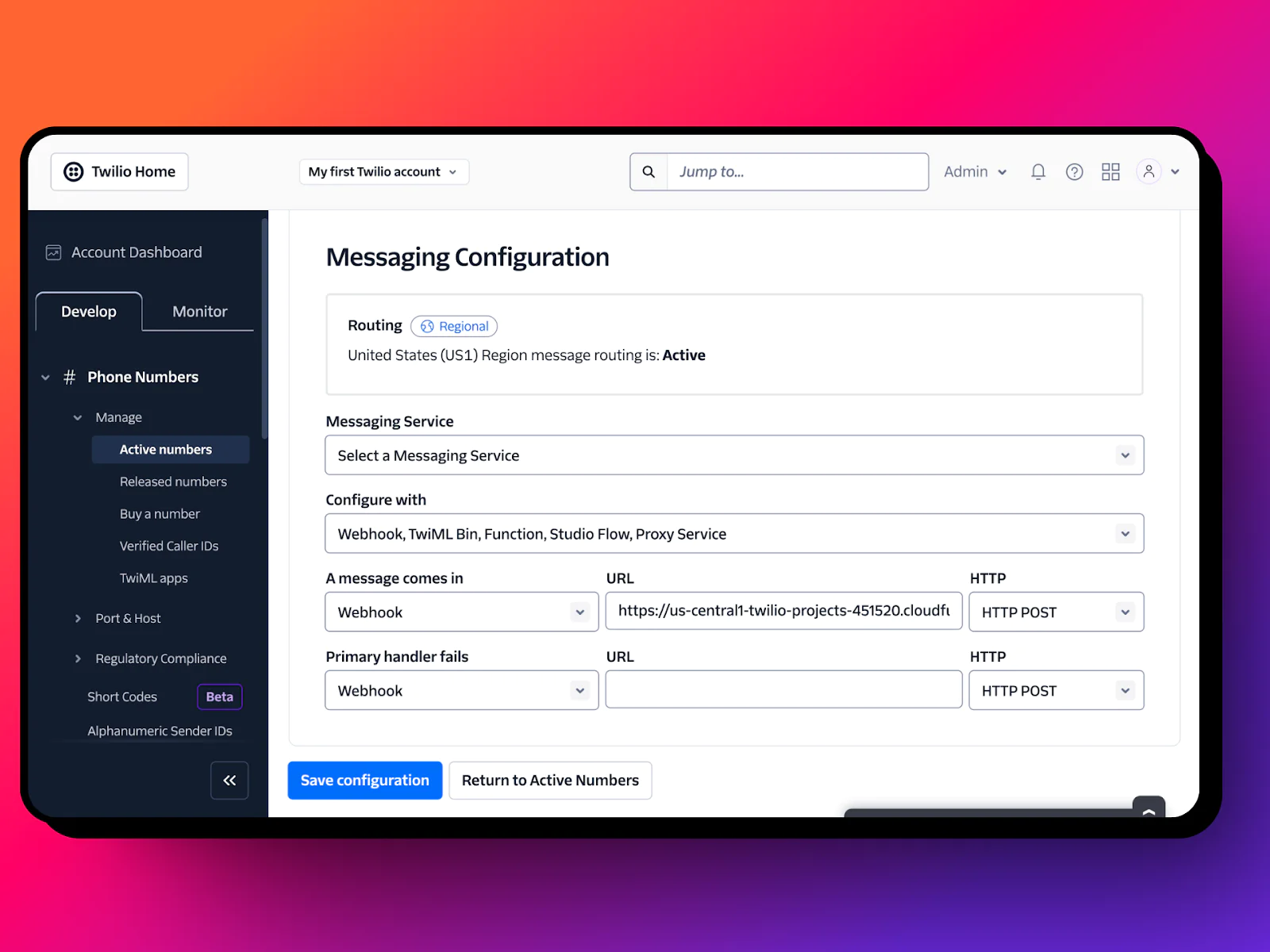
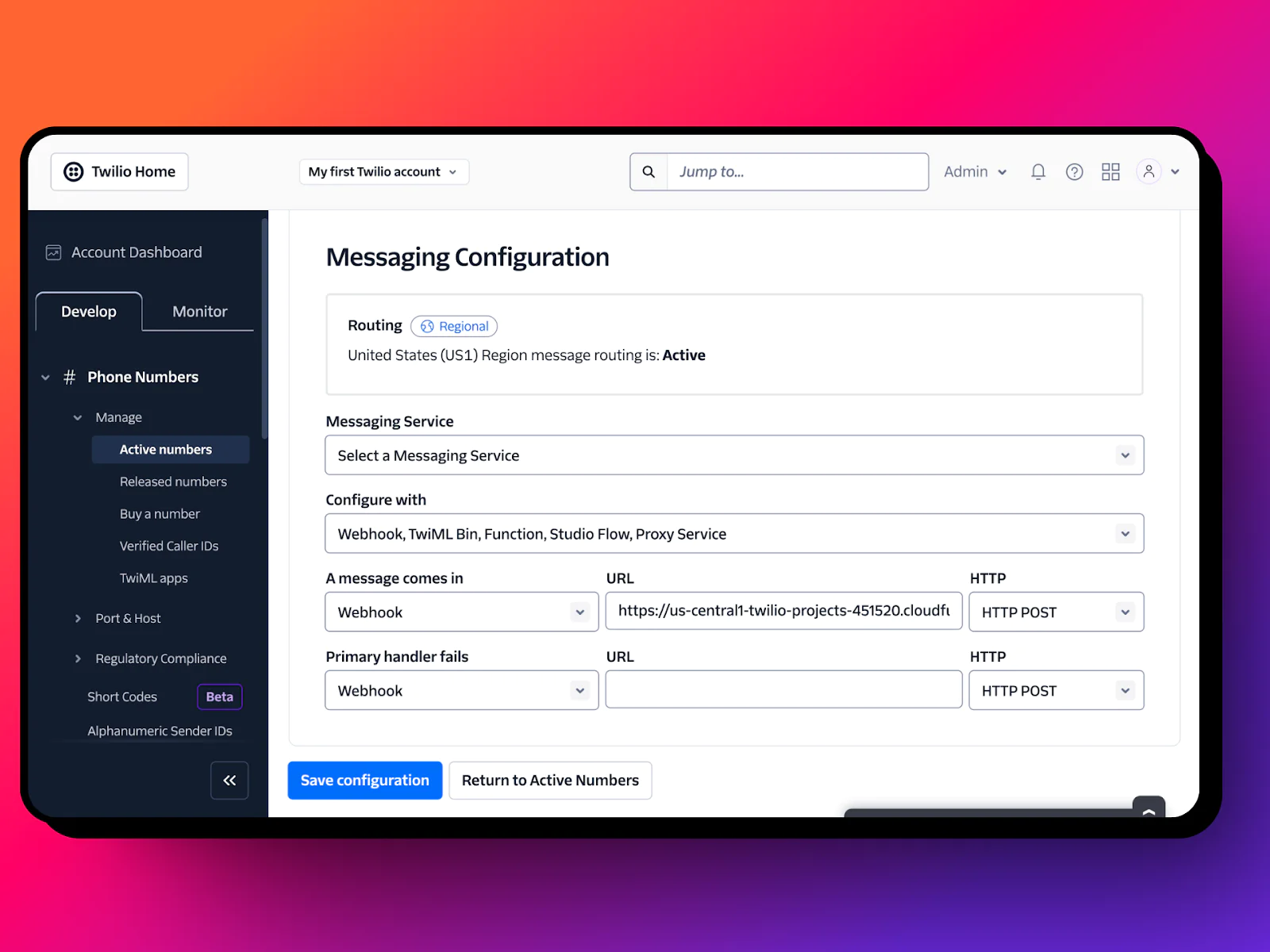
Now, let’s test if it all works. Grab your phone and send a text message to your Twilio number, you should receive back a random programming joke!
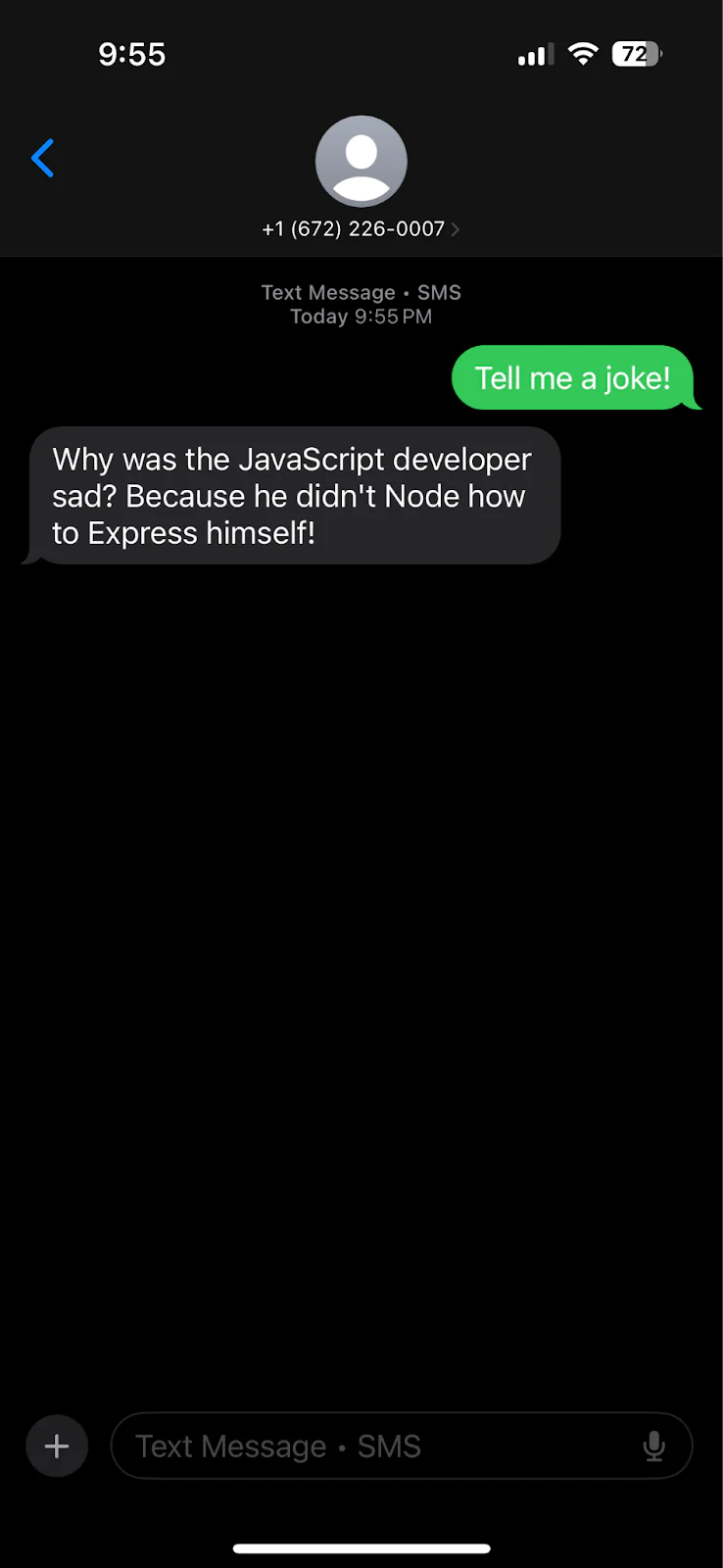
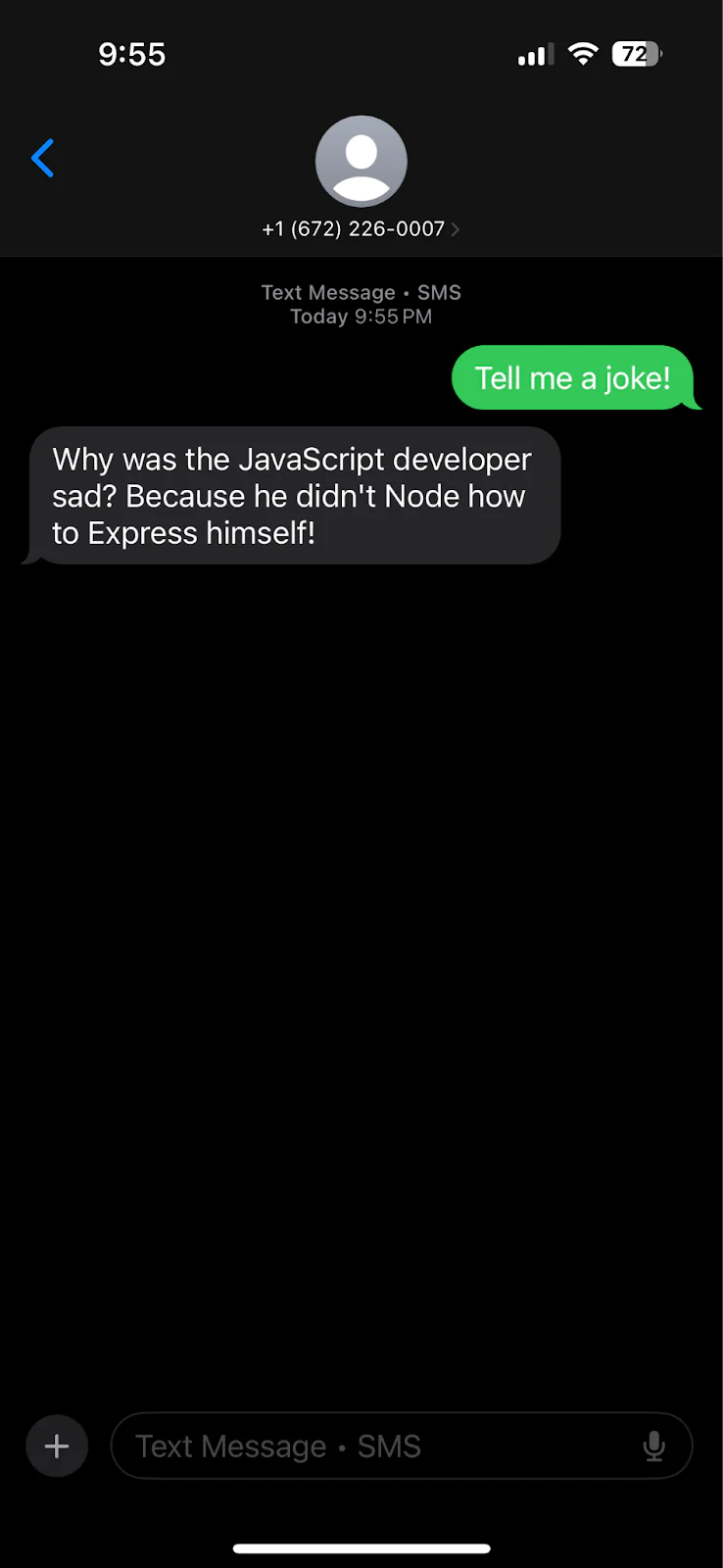
Monitoring and Logging
You can monitor your functions logs via the console or via the CLI as well.To view your function's logs using the CLI:
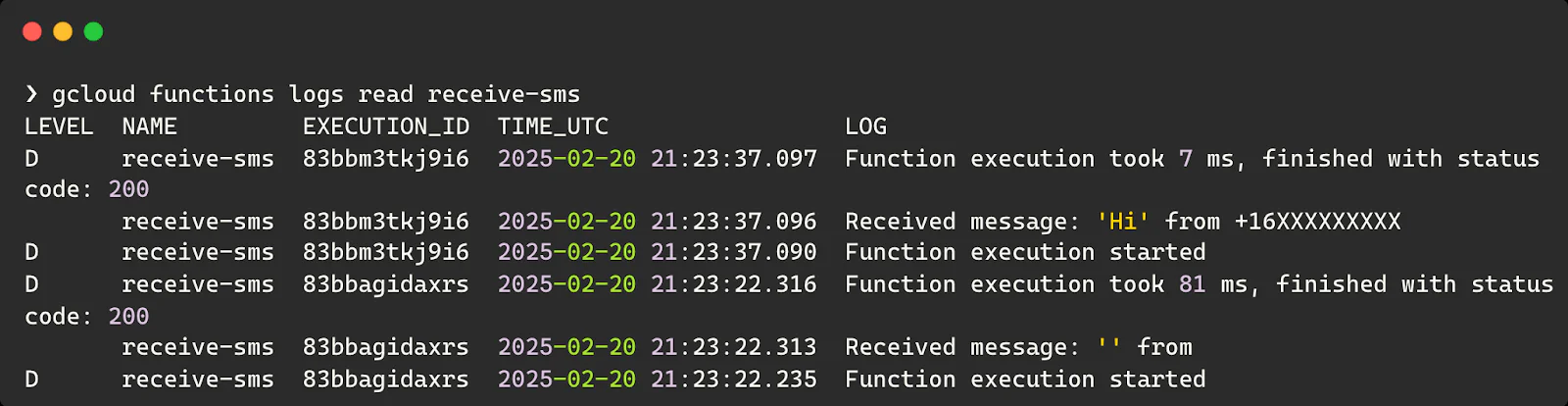
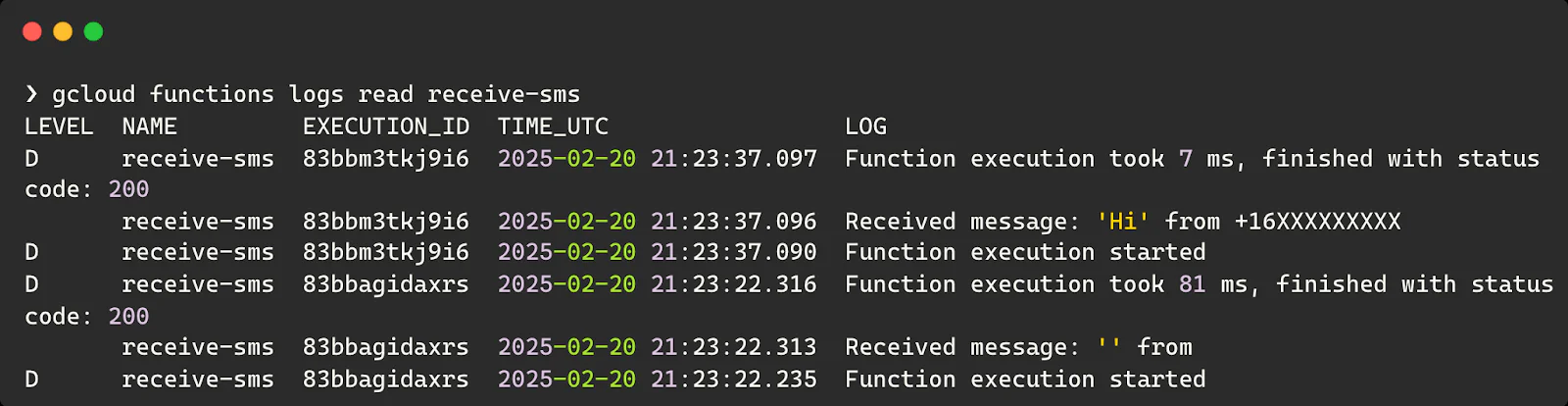
If you want to clean up the resources (cloud function) we deployed to GCP, you can use the following command to delete the function.
Conclusion
That's it! We've built a serverless SMS responder using Google Cloud Functions that can handle incoming messages and respond automatically. Having worked with both Twilio's APIs and Google Cloud, I can tell you this combination is powerful for building scalable communication solutions.
This is just the beginning. Maybe I will do another post where I can show you how to handle long-running processes using Google Cloud Pub/Sub, perfect for scenarios where you need to process messages asynchronously or integrate with AI services.
I'd love to see what you build with this! Feel free to reach out to me on LinkedIn or Twitter/X if you have questions or want to share your project. Keep building, keep learning!
If you found this helpful, check out my other tutorials on Cloud, Python and DevOps and on my YouTube Channel.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.