Build a Movie Recommendation App with Python, OpenAI, and Twilio SendGrid
Time to read: 9 minutes
In an era of information overload and decision fatigue, tools that simplify our choices while catering to our emotional needs are invaluable. Sometimes, all it takes is the right movie to turn your day around. Feeling down, anxious, or just stuck in a funk? Movies can tap into your emotions and lift you out of those moods, giving you a sense of escape or even a fresh perspective. Whether you're looking for something to brighten your spirits, ease stress, or channel your excitement, the Mood-Based Cinematic Curator is here to help. It’s more than just a movie suggestion tool. It’s a way to sync your emotions with the perfect film, offering a remedy for whatever mood you're in.
This innovative app doesn't just recommend movies. It curates a personalized cinematic experience tailored to your current emotional state. By leveraging the power of natural language processing, machine learning, and AI, our app understands the nuances of human emotion and translates them into spot-on movie suggestions.
In this tutorial, you’ll learn how to build this Mood-Based Cinematic Curator. You'll use Flask for backend, harnessing the power of OpenAI's GPT-4 for intelligent movie recommendations. Tapping into The Movie Database (TMDb) API for rich movie details, and implementing Twilio SendGrid to keep users engaged with personalized email notifications.
Prerequisites
Before you start building, you will need the following:
- Python 3.7 or later installed.
- A Twilio account. If you don’t have an account, you can sign up for a free account.
- An OpenAI account and API key creation. You can sign up for a new account here.
- A TMDb API key. You can sign up to create an account.
- Basic understanding of Python.
Set up the Project
To create a new directory for your project and navigate to it. Open your terminal and run the commands below:
mkdir
: This creates a new directory called mood-based-cinematic-curator.
cd
: This changes your working directory to the newly created folder, so all further commands are run within this project directory.
Next, you create a virtual environment for the project and activate it. A virtual environment helps isolate the project's dependencies so they don’t interfere with other Python projects on your machine. Run the command below:
python -m venv moodBooster
: This creates a virtual environment named moodBooster
.
moodBooster\Scripts\activate
: This activates the virtual environment on Windows.
Install the Required Libraries
With the virtual environment activated, run the command below to install the necessary Python libraries using pip
:
flask
: A lightweight web framework for Python.
openai
: The library to interact with OpenAI's API.
python-dotenv
: To load environment variables from a .env file.
requests
: For making HTTP requests.
transformers
: A library by Hugging Face for natural language processing (NLP) tasks, useful for working with OpenAI models.
sendgrid
: A library to send emails via SendGrid.
torch
: The transformers
library uses torch as its backend. Without torch
installed, you'd get an import error when trying to use the emotion classification model
Create a .env
file
The .env file stores your API keys and sensitive configuration data in an easily manageable and secure way. Create this file in your project’s root directory and add the following keys:
Replace the placeholder values with the actual API keys, which will be loaded in your application. For you to be able to acquire the services needed from the third party applications.
This setup prepares the foundation of the project. Which allows you to build an application that can integrate with OpenAI, TMDB, and SendGrid APIs for AI-based film recommendations and notifications.
Now proceed to acquire the required API keys that will allow the communication between the application and the third party applications.
Obtain the API Keys
Configure your OpenAI Account
To set up your OpenAI account and obtain an API key, visit the OpenAI website and log in to your OpenAI dashboard. Follow the provided instructions to generate an API key tailored to your account. Securely copy and add it to the .env file. It will be used for authentication of requests to the OpenAI API from your Flask application. It enables it to leverage OpenAI's natural language processing capabilities effectively.
Click on Create new secret key. You are prompted to enter the name of the API key and then click again on Create new secret key to generate the API key. Copy the API key and add it to your .env file under the OPENAI_API_KEY
.
With the above in place, you are now able to analyze and recommend films.
Configure your TMDb Account
After obtaining the OpenAI credential, you will need to get your API key from TMDB to make the interaction. Create an account on the TMDB signup page, and then verify your account by clicking the blue button labeled Activate My Account in the verification email you receive. Log in to your account and then request an API key by clicking the link under Request an API Key on this page.
Register as a Developer and fill out the API request form completely with the respective details, and then click submit. Once you’ve done so, you should land on a page that contains your API Key, as shown in the screenshot below. Copy the API key and paste it in your .env file under the TMDB_API_KEY
value.
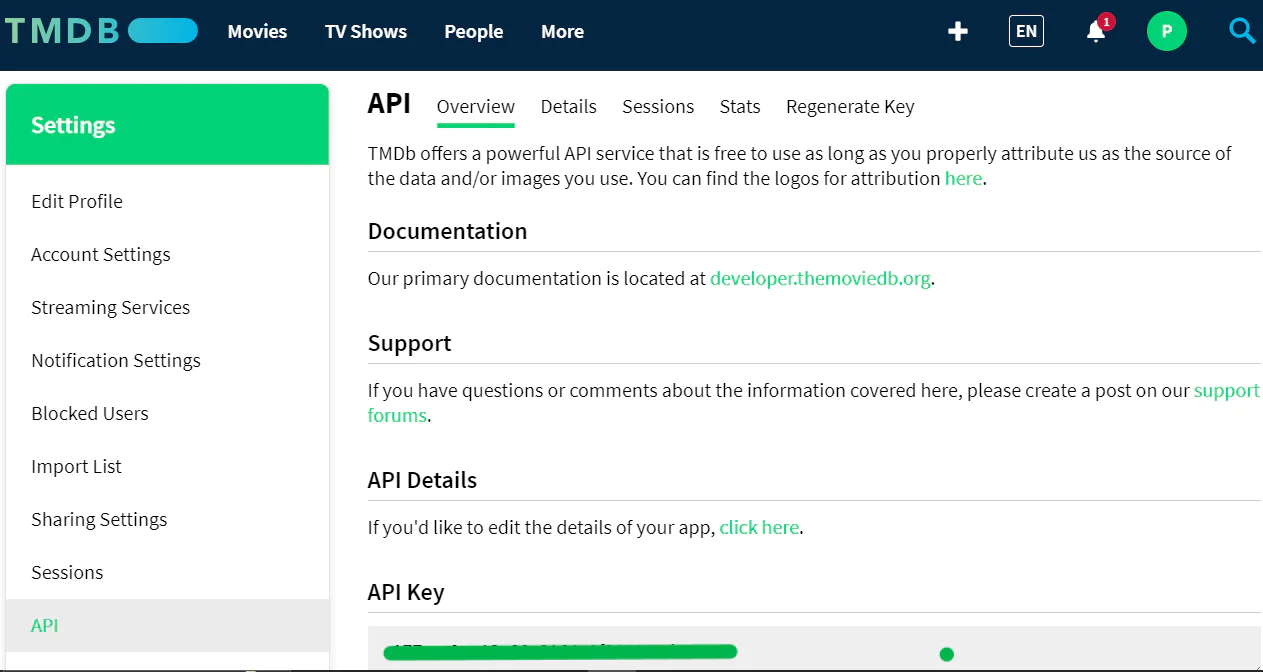
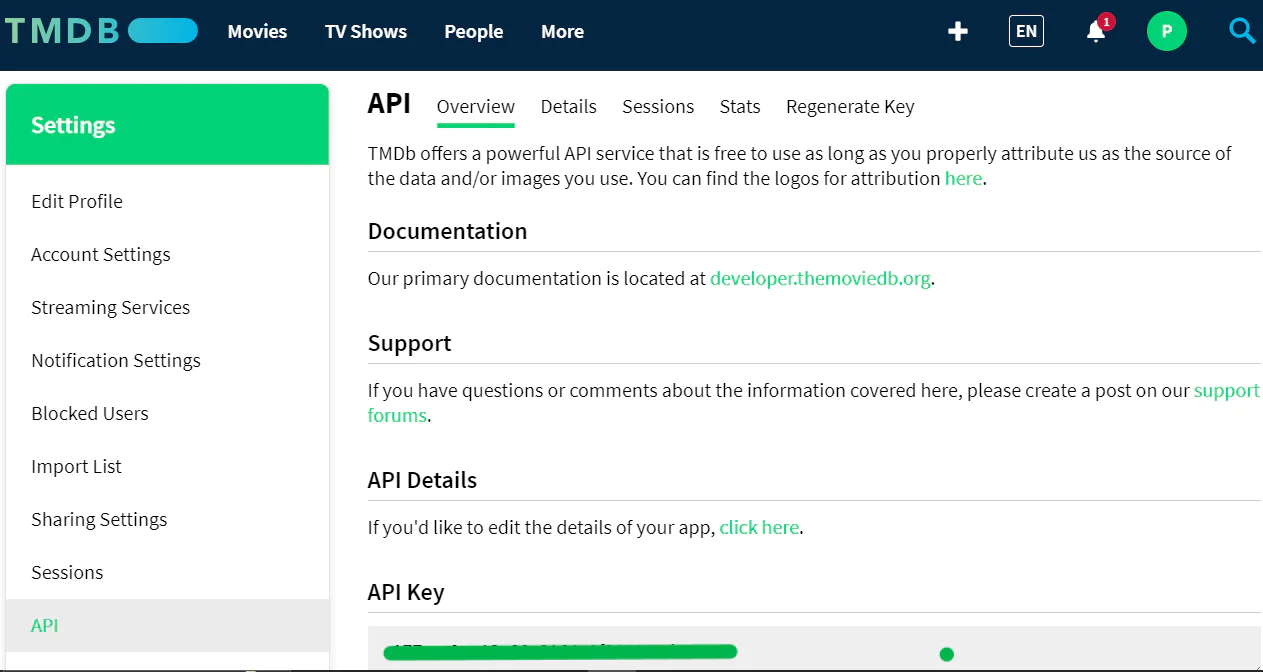
With the above in place, you are now able to query for movies.
Configure your SendGrid Account
Secure your SendGrid credentials needed to work with your application. This allows you to authenticate your requests and send emails through following the process below.
To begin, access your SendGrid dashboard and navigate to Settings > Sender Authentication. Look for and click on the Verify a Single Sender button to start the verification process. You'll need to fill out the sender details form with your From Name (your name or company name), From Email Address (the email you'll send from), Company Name and Address, and Website. After completing the form, click Create and check your email inbox. You'll receive a verification link that you'll need to click to complete this process.
Once sender verification is complete, return to your SendGrid dashboard. Navigate to Settings > API Keys in the left sidebar. Click on the Create API Key button, then choose Full Access as shown in the screenshot below.
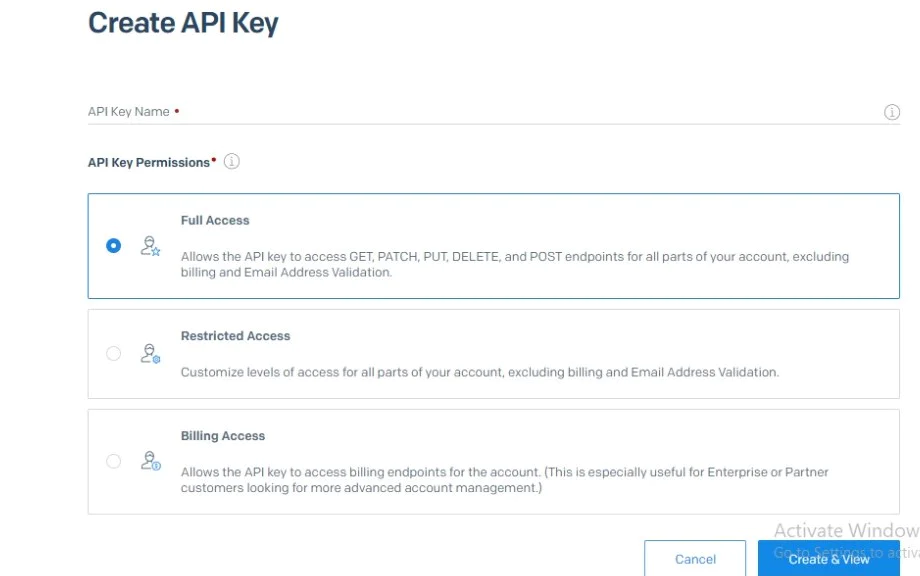
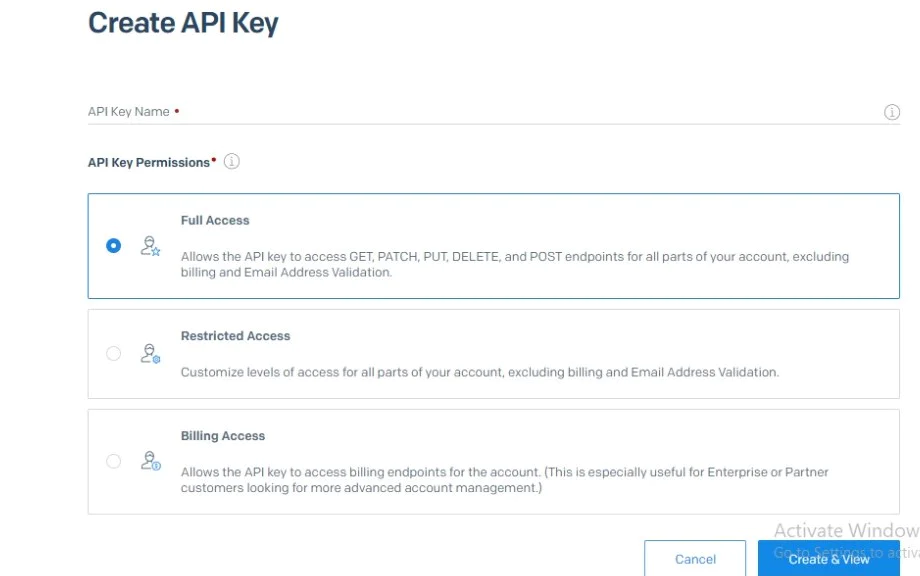
Next, give your API key a name. Feel free to give it a name of your choice, then click Create & View for the API generation to take effect.
After creation, SendGrid will display your API key. This is the only time you'll see the full key, so make sure to copy it immediately and add it to your .env file under the SENDGRID_API_KEY
value.
With the setup done right, proceed to the coding section, which binds everything together.
Create the Flask Application
Start by building the basic structure of your Flask application. Create a folder in the root directory and name it templates. Inside the templates directory, create a file and name it mood_check.html. This will contain the code of your user interface. Next, create a file named app.py for the main function in the root directory and update it with the code below.
The code imports the necessary libraries and initializes the Flask app. It then uses load_dotenv()
to load the environment variables from the .env file. The OpenAI API key and other keys are retrieved using os.getenv()
. Next, the emotion classifier model is initialized using Hugging Face’s transformers
library. This model will classify the user's mood based on their input.
Fetch Movie Details from TMDB
To retrieve movie details such as the title, poster, and link from TMDB add a function that queries the TMDB API. Copy this code to your app.py file.
The get_movie_details
function constructs a URL to query the TMDB API for movies that match the movie titles suggested by OpenAI's GPT model. If the response is successful, it extracts the movie's details, including the title, poster image URL, and a link to the TMDB page. If no results are found, it returns None
.
Write the Flask Route to Display the Main Page
This route renders the HTML template mood_check.html, which is the main page where users can express how they are feeling, add their email, and get a recommendation. Copy the code below and add it to the app.py file.
The form on this page collects user input and submits it to the /analyze
route for processing.
Analyze the User’s Mood and Provide Recommendations
In this step, you create the analyze route which is the core route in this application. Copy the code below and add it to the app.py file.
This route handles the POST request sent when a user submits their mood description and email. It's responsible for processing the user's input, determining their mood, generating movie recommendations, and sending an email.
First, the app extracts the user's input and email from the form data. Then, it uses a pre-trained emotion classification model to determine the user's emotional state based on their input.
With the emotion classified, the application taps into the power of OpenAI's GPT-4o model. You provide the AI with a carefully crafted system message that essentially says, "You're a movie recommendation assistant. Based on this emotion, suggest three appropriate movies." You then feed it the detected emotion and await its response.
Once we receive the movie recommendations from OpenAI, you don't stop there. For each recommended movie, you reach out to The Movie Database (TMDB) API to fetch additional details like the movie poster and a link to more information. This enriches our recommendations, providing users with more context and visual appeal.
With the user provided email address, you take an extra step to enhance their experience. You use your Twilio SendGrid integration to email them containing their detected mood and movie recommendations. This allows users to revisit their personalized recommendations later, even after closing their browser.
This analyze
function serves as the heart of our Cinematic Curator. It orchestrates a complex dance between emotion analysis, natural language processing, and multiple external APIs, all to deliver a uniquely personalized movie recommendation experience.
Send Email with Twilio SendGrid
Using OpenAI’s GPT-4, you’ll generate film recommendations based on the user’s mood. This function sends a prompt to GPT-4 to request mood-specific movie titles. Copy the code below and add it to the app.py file.
This function constructs an email using the SendGrid Mail helper. The from_email
is set to the sender's email address, which is retrieved from the . env file. The body of the email contains the detected emotion and a list of film recommendations. The SendGridAPIClient(SENDGRID_API_KEY)
sends the email, and the function prints the status of the email send operation. If an error occurs, it prints the error and returns False
.
Next, you’ll see how the frontend works in association with the backend.
Build the Frontend of the application
Create a new file named mood_check.html in a templates folder and add the following code content:
The HTML structure includes a form for users to input their mood and provide an email address.
It uses Tailwind CSS classes to style the page, ensuring a visually appealing and responsive layout. The template includes elements to show loading status and potential error messages. JavaScript is used to handle the form submission, fetch data from the backend, and update the user interface with the movie recommendations with results asynchronously or any errors that may occur.
Test the Application
Find the full source code in this GitHub repository.
Navigate to the project directory in your terminal. Run the Flask application by executing the command:
This will start the web server locally. Open a web browser and navigate to the URL provided by Flask, typically http://localhost:5000
. You should see a homepage as shown in the screenshot below.
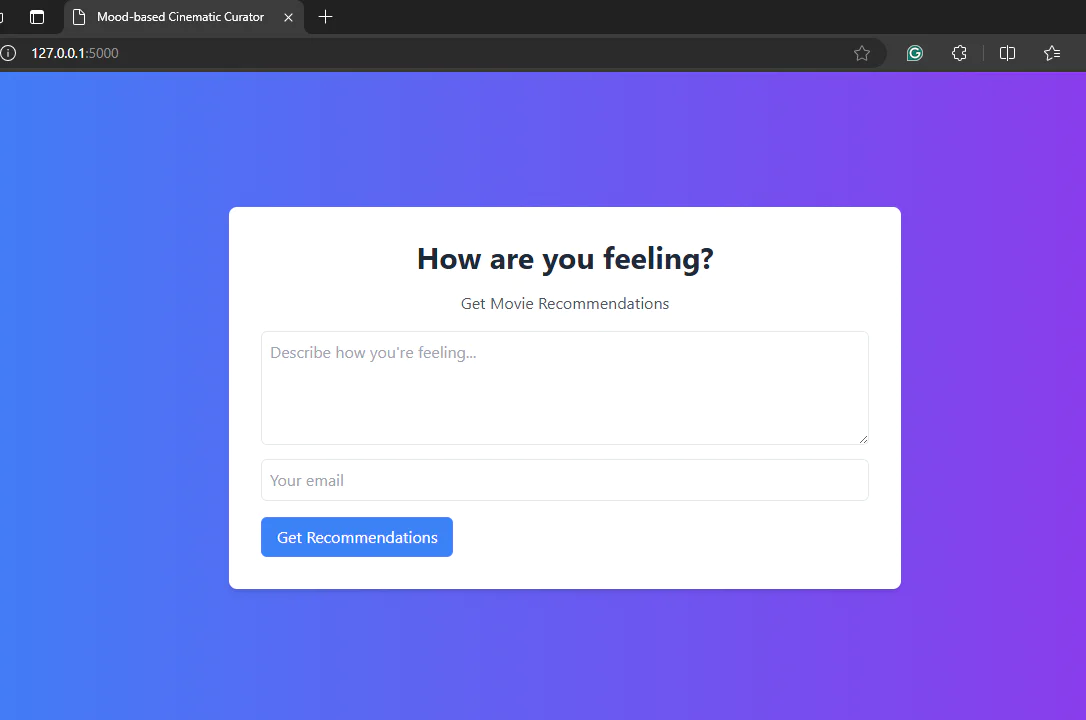
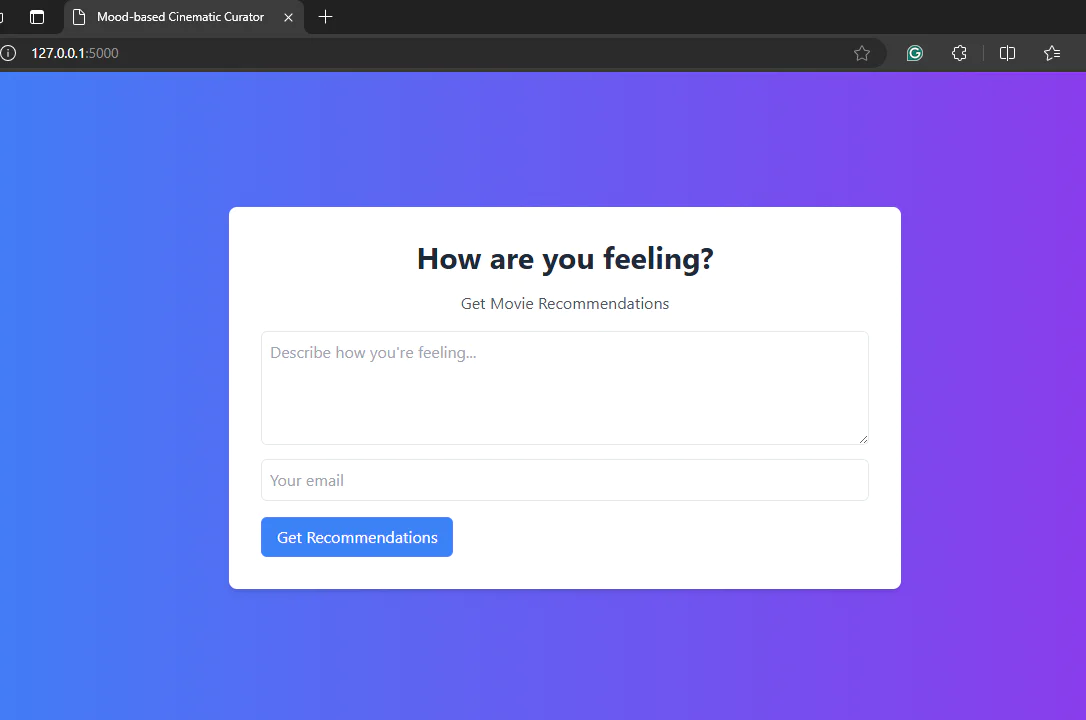
On the webpage, express yourself in the provided text area and provide your email too. Then click the Get Recommendations button to submit. As show in the screenshot below.
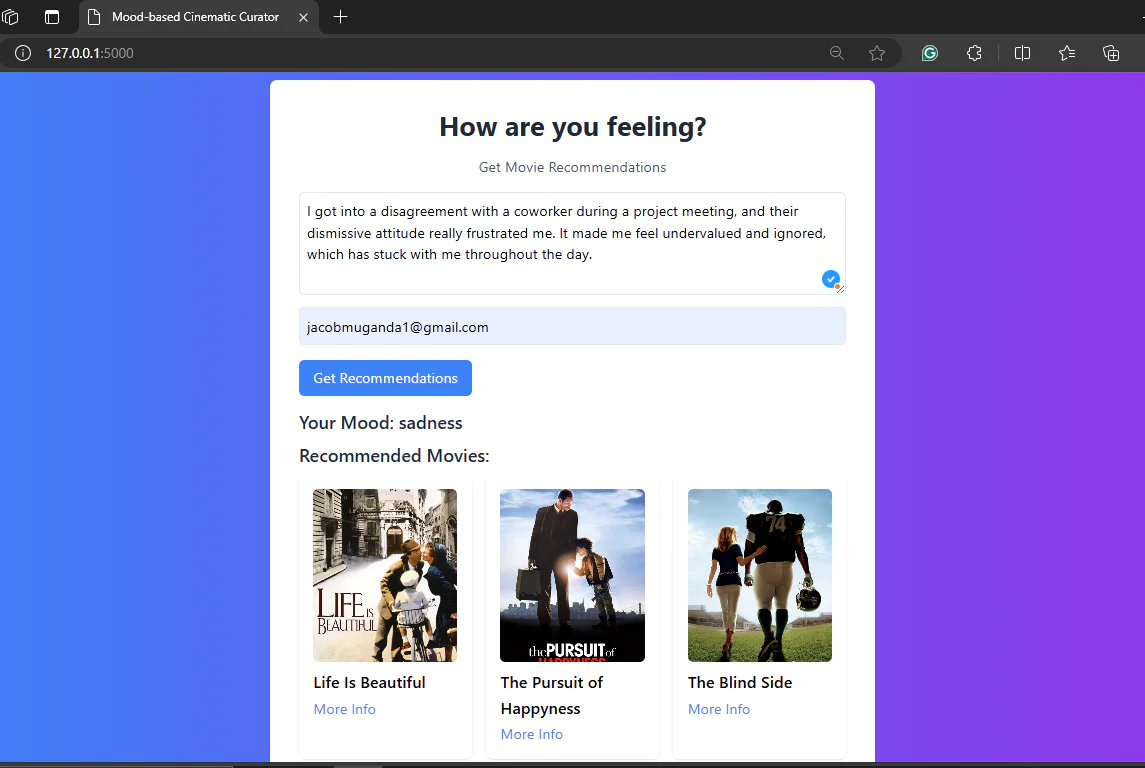
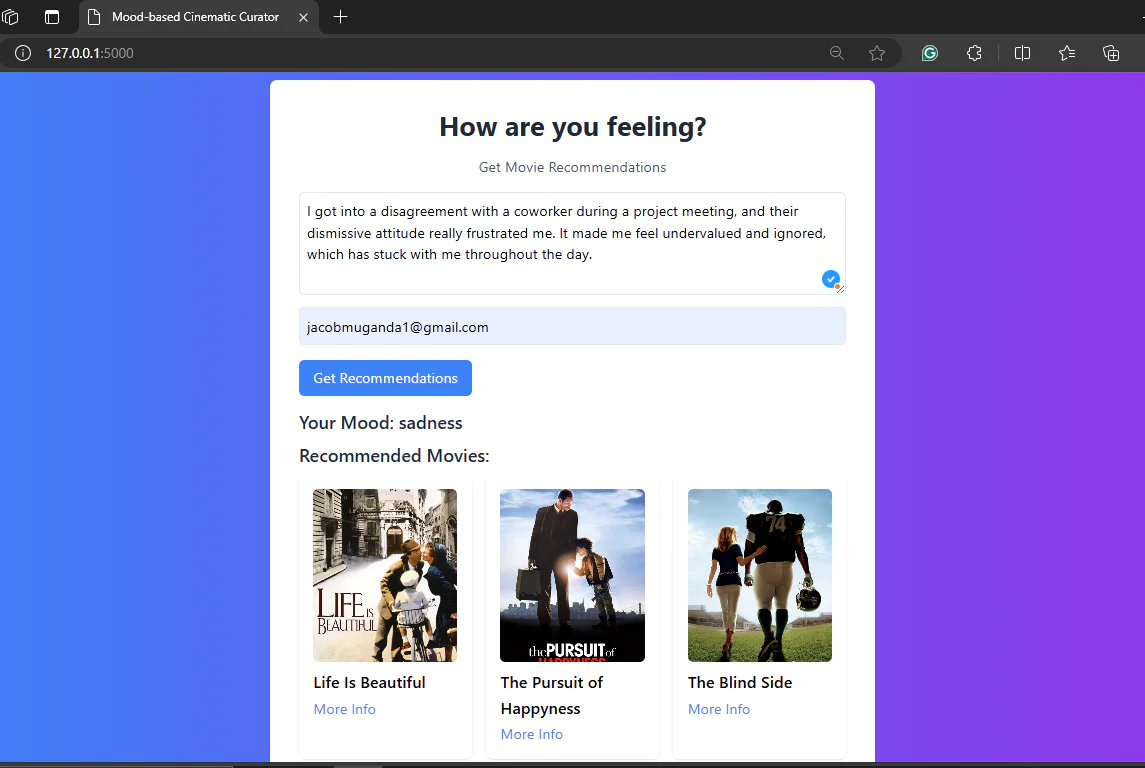
Now with that in place, check your email. It should contain the links suggested, as shown in the screenshot below.
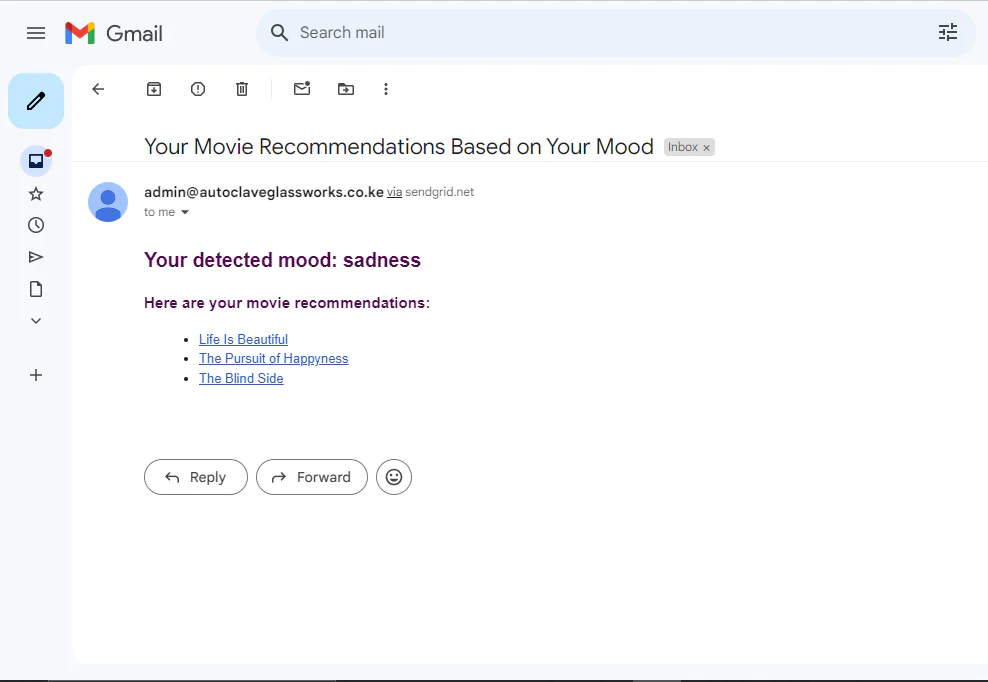
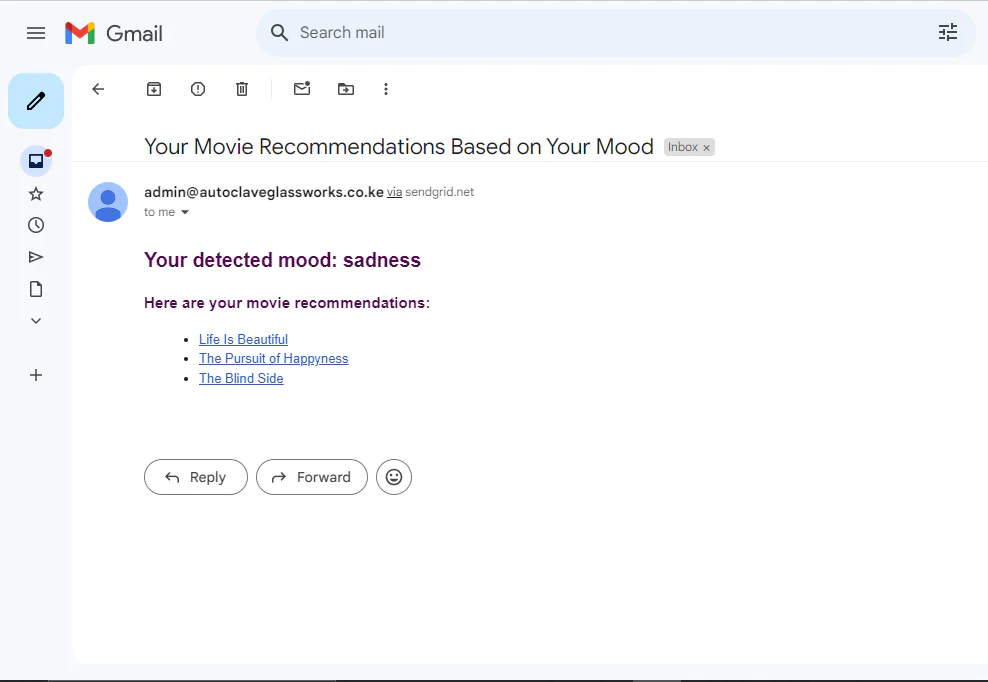
What's next for OpenAI and SendGrid projects?
Congratulations! By following this tutorial, you've built an AI-powered movie recommendation system that understands users' emotions and delivers personalized suggestions right to their inbox. Throughout this journey, you've learned how to analyze sentiment using AI, generate contextual movie recommendations with OpenAI's GPT, and send beautiful, personalized emails using Twilio SendGrid.
This project not only showcases the potential of integrating advanced AI models with modern web applications, but also emphasizes the importance of emotional well-being through tailored recommendations.
If you are looking for ways to further enhance your application, check out these articles for inspiration:
- Hype Someone Up This Christmas With Laravel Sail, Twilio Programmable Voice, and SendGrid
- How to Send Emails in Python with Sendgrid
- Crafting a Unified Voice: Creating the next step in virtual agent evolution using AI
Jacob Muganda is a full-stack developer and Machine Learning enthusiast. He enjoys leveraging AI solutions in software applications.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.