How to Make a Morse Code Application
Time to read: 8 minutes
Telegraphs change the way humans communicate by allowing long-distance communication.
Many implementations were done before the electrical version using systems such as optical and fire. It was with the evolution of electricity that the telegraph system became famous, commercialized, and widely used. Morse code is the standard encoding for telegraph transmission. It is an effective communication system, but can we still have telegram messages like in an electric telegraph with our modern device - a mobile phone?
In this tutorial, you will discover and learn how you can send a telegram message encoded with Morse code to a mobile phone using Twilio Programmable Voice to replicate a telegram message that our grandfather in 1900 would have received.
Prerequisites
To complete this tutorial, you will need:
- A Twilio account with phone numbers. Create an account if you don’t have one already.
- A Python3.6 or higher installed in your preferred operating system.
- An internet connection: to install Python packages and also to make the application accessible from outside your local network.
Understand the history of the telegraph system and Morse code
A telegraph is a system or a device that allows one to transmit a message over a distance. It was in 1791 that Claude Chappe designed the first version of this telegraph system. It is based on optical principles and is generally called semaphore. Below is an illustration of the Chappe telegraph device.
With the evolution of electricity, the electrical telegraph arrived In 1832 with the invention of Pavel Schilling. In 1844 a single-wired version of the electrical telegraph appeared, designed by Samuel Morse and Alfred Vail. This uses Morse code, which became the standard for telegraph communication in Europe.
Morse code is a system that encodes text characters with a succession of dots and dashes. When transmitting a message with a telegraph, the operator translates the text message into a dot and dash (Morse code) and then types it on the telegraph device. In the receiver, dots and dashes are received and then translated to text to have the original text.
Morse code was used in many fields of application and was first designed to be written on paper tape which rotated as long as messages were received. The movement of the telegraph device when moving on and off the paper tape produces a sound that leads to the change from written code to audible code. A trained operator can recognize the produced sound and translate it to letters without the need for paper tape.
Fun fact: “dot” was known as “dit” because of the sound it makes on the device. Same thing for dash with “dah”. There is also some implementation of Morse code that uses visual signals like light or eye blink.
Each letter has its specific code, and there is no distinction between upper case and lower case.
Here is an illustration of the international version when encoding the text “Morse code”.
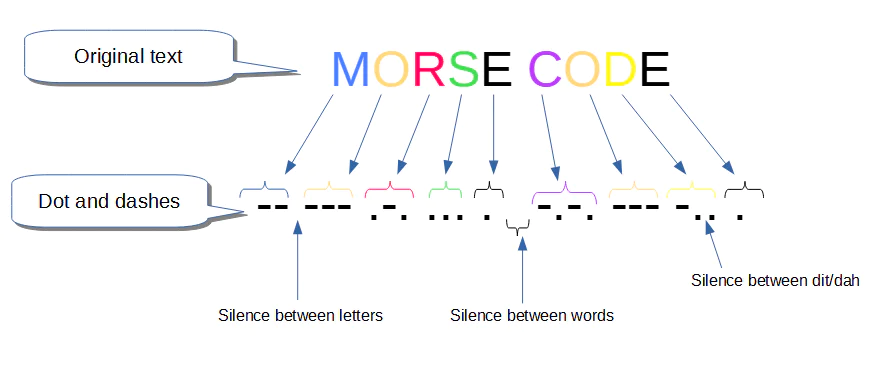
Build a telegraph system with Twilio Programmable Voice
In this tutorial, you will work with the Twilio Programmable Voice API which allows you to make a call and play audio files during the running voice call. The audio can be taken from a web server accessible by Twilio.
We can assemble every sound for each letter to be a unique file sound that can be later played by the Twilio Programmable Voice API.
So here is how our system will work:
- A text message is written.
- Each letter in the text message is converted to Morse code.
- The Morse code is then converted and compiled into one single audio file.
- The compiled audio file will be streamed by a web server.
- Create a call with the Twilio Programmable Voice API.
- The encoded audio message will be streamed through the call using TwiML.
The system can run under any platform that supports Python3.6+. You need to install the required packages in order to build and run it.
Install the necessary requirements
Start by creating a directory named “twilio_morse_code”. It will contain the code for the system.
Create a virtual environment to isolate the package for your application. In the root folder of the project, run the following command. It is always better to create a virtualenv so that your application runs in a freshly separated environment.
Activate the virtualenv so you can have a separate library installation in it.
Then install the required libraries.
In order to make your app accessible from the internet you need to install Ngrok. It allows you to expose your local service to the internet so that the Twilio Programmable Voice can communicate with it.
Download it from the official website for free: https://ngrok.com/. Install it by using the command line indicated on the website.
Build the Morse code encoder
Start by adding a Python package named ` morse` inside the root directory.
You need to translate or encode a given text to a Morse code sound. Each letter has its corresponding Morse code. Create a file named “ code.py” inside the morsepackage and paste the following code that defines the dict:
Note the presence of the “ONE_UNIT” variable initialized with 0.5. This will correspond to the unit time as described above in the Morse code section.
"SEVEN_UNITS" represents space between words and "THREE_UNITS" defines the pause between each letter.
On the other hand, you need Morse code sound for each letter and digit. A prepared list of sounds is available on this GitHub repository from GitHub user cptdeadbones.
Download all of the ogg files and store them inside a subdirectory named “ morse_sound_files” at the same level as the morse package. The directory structure inside “ twilio_morse_code” should look the same as shown below.
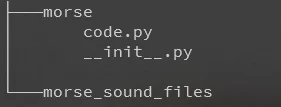
Now you need a way to concatenate the sound for each letter to form a final unique sound that can be streamed later. pydub, a Python extension can be utilized here to manipulate audio files.
Create a function “ message_to_morse_sound” that takes a text message string variable and converts it to a Morse code sound file.
The function takes four parameters:
- a text message string.
- the base path of the Morse code sounds.
- the file name of where the generated audio file should be saved.
- the format of the generated sound file.
Create a new file named encoder.py within the morse subdirectory and copy and paste the following code into the file.
The function verify_message
checks if all characters in the message can be translated to Morse code.
Now you have a way to translate a text message to a Morse code audio file. Let’s move to the way to stream it in a phone call.
Make calls with the Twilio Programmable Voice API
You will need to create an instance of Twilio client in order to create a call. In the running call, you will add TwiML instructions to play audio by pointing to your web server URL.
Navigate to the root folder of the application twilio_morse_code and create a file named web_app.py. Note the import of the message_to_morse_sound
function from the morse.encoder subdirectory for later use. Copy and paste the contents to the newly created file:
Navigate to the Twilio console to obtain your TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
. Set the corresponding value in your environment variable.
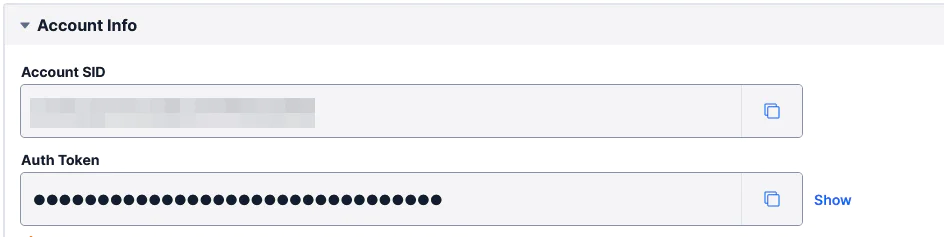
If you are on Linux set the environment variable using /etc/profile so that it can be permanent. Edit /etc/profile with your favorite text editor.
Replace the XXXXX with the appropriate values obtained from the Twilio console.
Build the web server
The web server is the center point of your system because it is where a user enters text to be translated and also where Twilio interacts to retrieve an audio stream.
The Flask framework is used to build the web server. It has the advantage of being lightweight and can give you a way to serve an audio file quickly with static files.
Still in the file web_app.py add the following codes at the end of the file:
The above code shows the basics of your web server with one route (/hello). It will be used to check that your web server is running by returning a “ hello” text when the web server is running.
At the same level as web_app.py, create a folder named static. It will store the audio file generated by your encoder. Flask can stream any audio file in the static folder automatically in the URL path /static/audio_file.xxx.
It’s time to add a way to interact with the system. For that, the web server needs to have more routes.
First, a route where a user can enter text which will be encoded with Morse code. It is called “tomorseaudio” and can be accessed in URL path /tomorsecode. A “ text” query parameter should be set when accessing this route.
Secondly, create a route where a user decides to send the Morse code to a mobile phone via the Twilio Programmable Voice API. Call it “ sendmorse” and available in “ /sendmorse” URL path. Users should give “to” and “from” query parameters when using this route. The values of to and from should be a valid phone number. Twilio uses E.164 standard, but it is better to use the international format like the following number: +4402077651182.
Add these two functions in web_app.py file after the “hello” function.
The complete code can be downloaded from this GitHub repository.
Run the Morse code app
Now everything is in place to run the system.
Use the following command to run the web server:
Expose the web server with ngrok
If you are running ngrok for the first time, you should create an account and get your auth token. Once you get your auth token, run the following command to add it to your local configuration. Do not forget to replace <token> with your auth token:
Now run the following command to expose your web server on the internet: ngrok http 5000
You should get something like the below screenshot on your console. Note the ngrok link shown in it.

Test the system by sending a Morse code message
Open the ngrok URL with a web browser by appending /tomorsecode?text=hello to the end of the URL as seen in the example below:
This will generate a Morse code audio file for the text “hello”.
Now send it to a friend by specifying your friend's phone number in the query parameter as shown below. Note that it is better to follow the international format for the phone number.
Be sure to replace the "to" value with your friend's phone number and the "from" value with your Twilio phone number in the international format "+44XXXXXXXXXXX", as seen below:
Your friend should now receive a phone call and hear the Morse code audio message.
What's next for building telegram apps with Programmable Voice?
This article shows that it is still possible to send messages encoded with Morse code like an old telegraph. In our century, it may sound weird to send Morse code with our powerful device and Twilio Programmable Voice API, but it is fun, and it is constantly enriching to learn from the origin of a technology.
Check out these articles to expand your project further:
- Make a Phone Call Using Python and Twilio Programmable Voice
- How to Transcribe a Voice Message Using Twilio, Python, and Flask
- Setting Up a Conference Call Line with Twilio Programmable Voice, Python and Django
Aina Rakotoson is a lead Python developer, a dad, a handyman, and a vintage mechanic lover. You can find him on Twitter or on GitHub .
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.