Monitoring Email Delivery/Open Rates in .NET Using Twilio SendGrid Webhooks
Time to read: 4 minutes
Monitoring Email Delivery/Open Rates in .NET Using Twilio SendGrid Webhooks
Introduction
Communication through emails is a priority for almost every business. The ability to send outgoing emails is important. But it is equally important to be able to measure the metrics around those emails. What is the number of users who actually opened the email? Was the email even delivered successfully or not? In this tutorial, you’ll learn how to monitor email delivery and open rates for a .NET application using Twilio SendGrid Webhooks.
Prerequisites
Building the app
First, log in to your SendGrid Account. You’ll need to create a new SendGrid API Key. For this tutorial, you can choose restricted access when you create your key. On the Restricted Access tab, be sure your API Key is set to allow Email Sending access.
Create a New .NET Web API Project
Open the terminal in VS Code and use the following command to create a new ASP.NET Core Web API project:
Install SendGrid NuGet Package
In your terminal window in VS Code, run the following command to install SendGrid’s package.
Configure SendGrid in Your Application
Open the file appsettings.json and add the API Key you created for SendGrid. Replace the placeholder below with your API key that you copied earlier.
Implement Email Sending Functionality
Create a new file called SendGridSettings.cs. Add the following code.
Now, load the added configuration to Program.cs. Under the first line of code that contains the builder’s definition, add this code:
Set Up the Webhook Endpoint
In order to receive event notifications from SendGrid, you need to set up an endpoint that will be triggered with the SendGrid event webhooks. Create a new folder called Controllers. Then create a new file called WebhookController.cs. Add the following code to implement the endpoint and capture the event data from SendGrid. It will then write it in a log file called webhook_events.log.
In order to enable the controller’s mapping and the routing to the API, you need to edit the file Program.cs. Find the line var app = builder.Build();
and add following code:
Run The App and Expose localhost With Ngrok
Run the app with the following command:
Then notice which localhost port is the one running the API. For example http://localhost:5255.
You will use Ngrok to generate a URL for you to expose your localhost port while testing the webhook API. Another alternative, if you don’t want to map to your localhost, is to deploy your API to a testing server then use the deployed endpoint’s URL for testing.
If you have not already configured ngrok, set it up now. Do this by signing into ngrok and going to your setup dashboard. Here you will find the download and installation steps, and your account’s token, which you must use in your CLI. The setup dashboard will look like the image below:
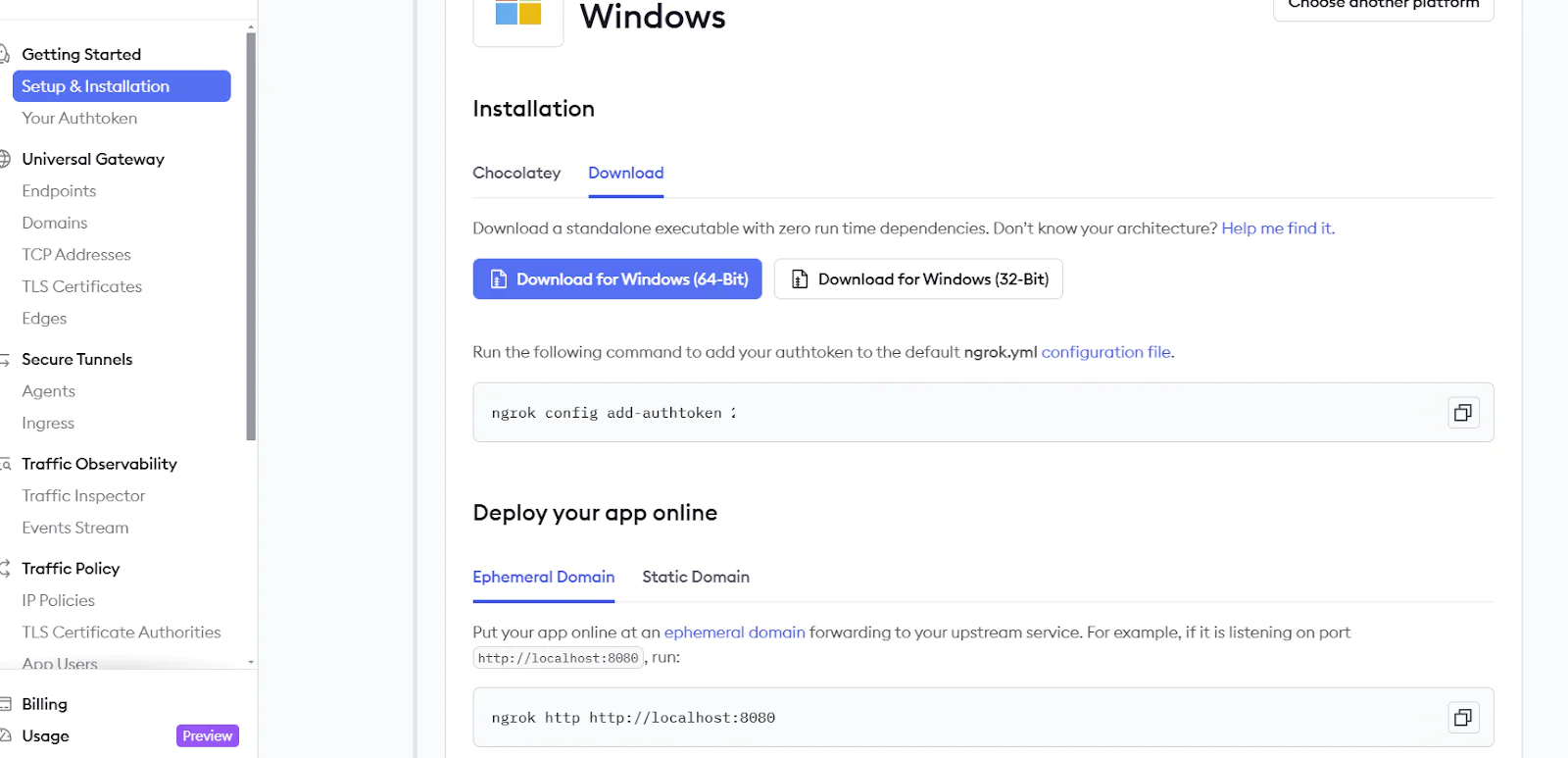
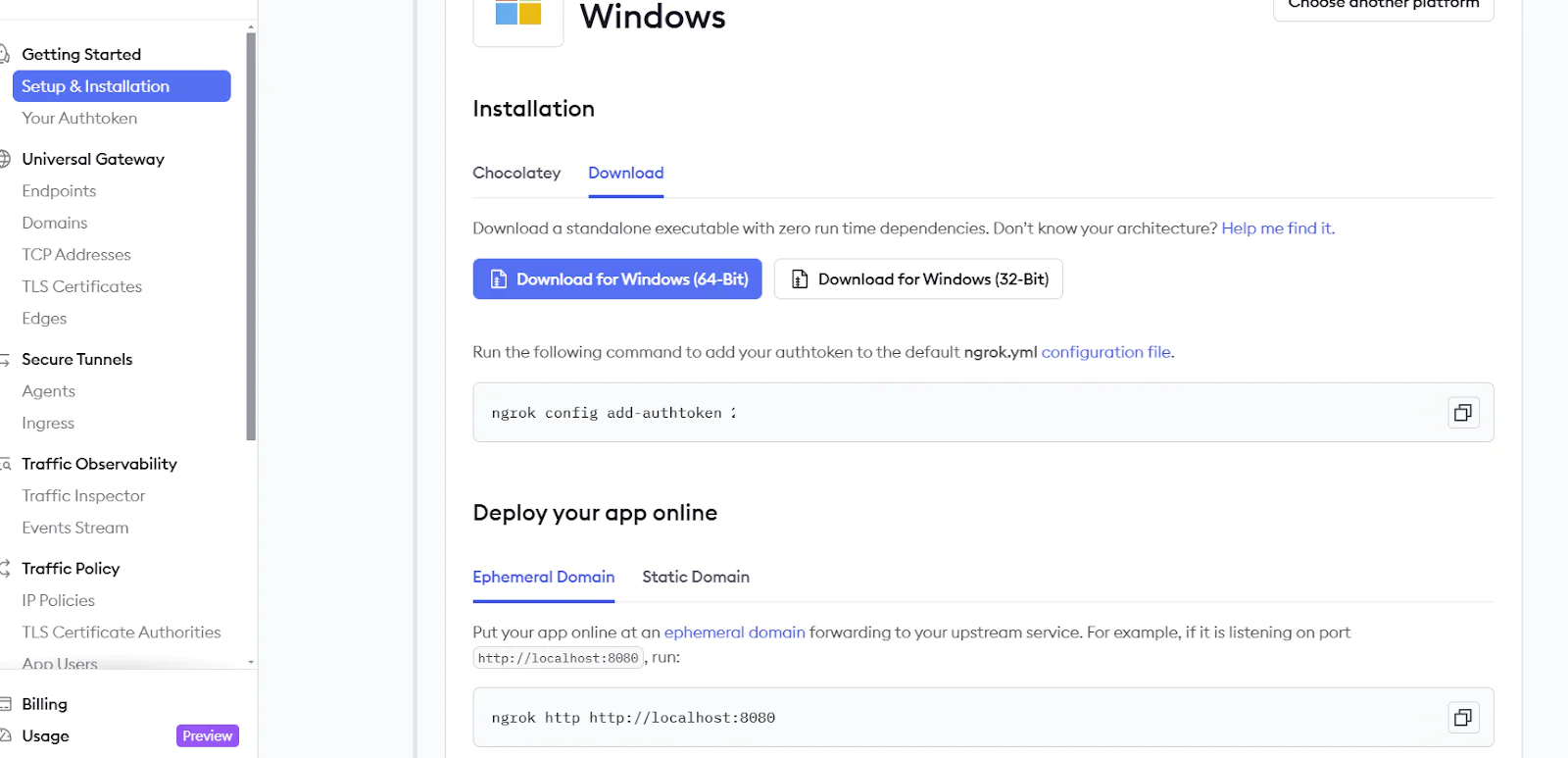
Run the following command in ngrok CLI to generate your URL, replacing the placeholder with your port.
It will return a URL that you can use for your localhost port that your app is running on as shown below:


Copy the URL as you will need it with the route to the webhook endpoint in SendGrid.
Enable Event Webhooks
In order to be able to use webhooks in SendGrid, you need to enable the event webhooks manually in the settings by navigating to Settings > Mail Settings > Event Webhooks in the SendGrid dashboard.
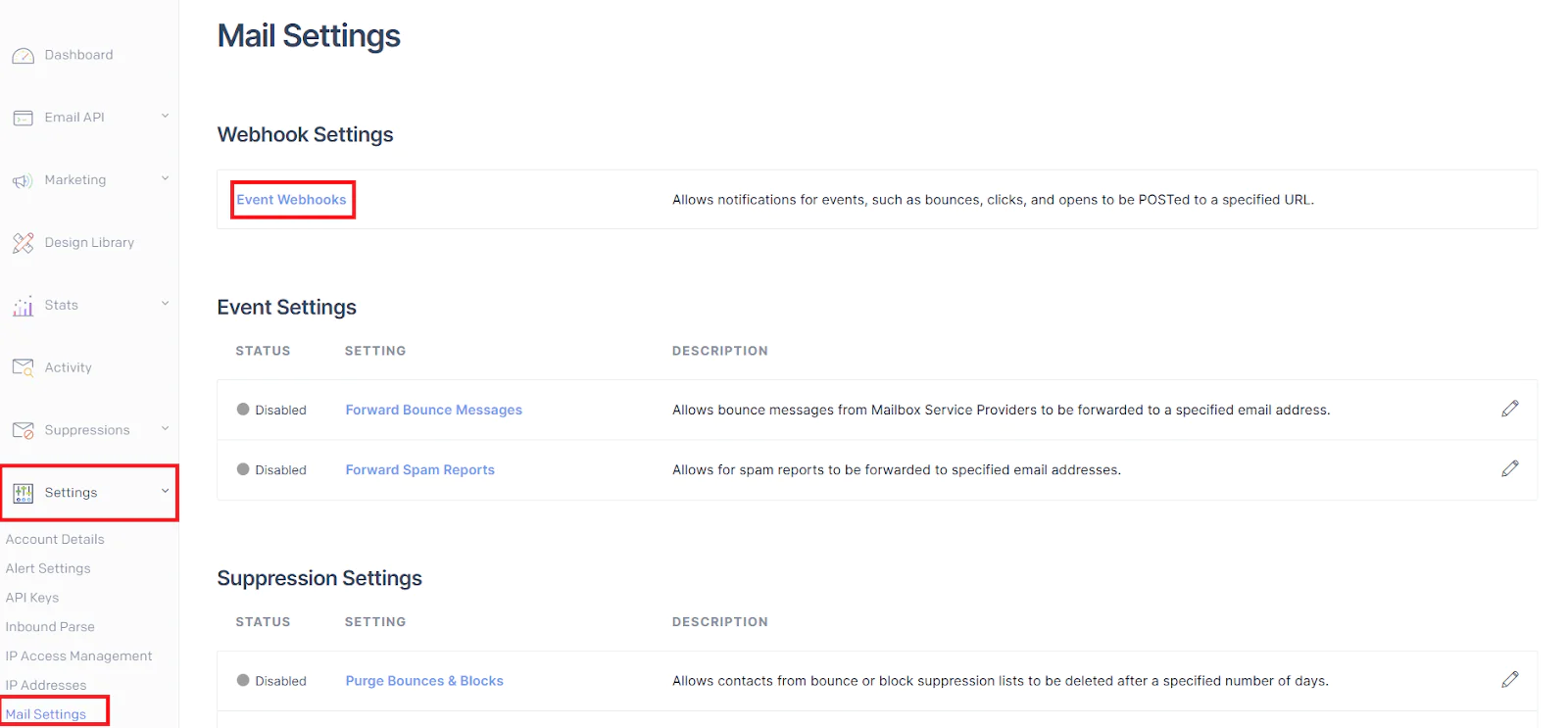
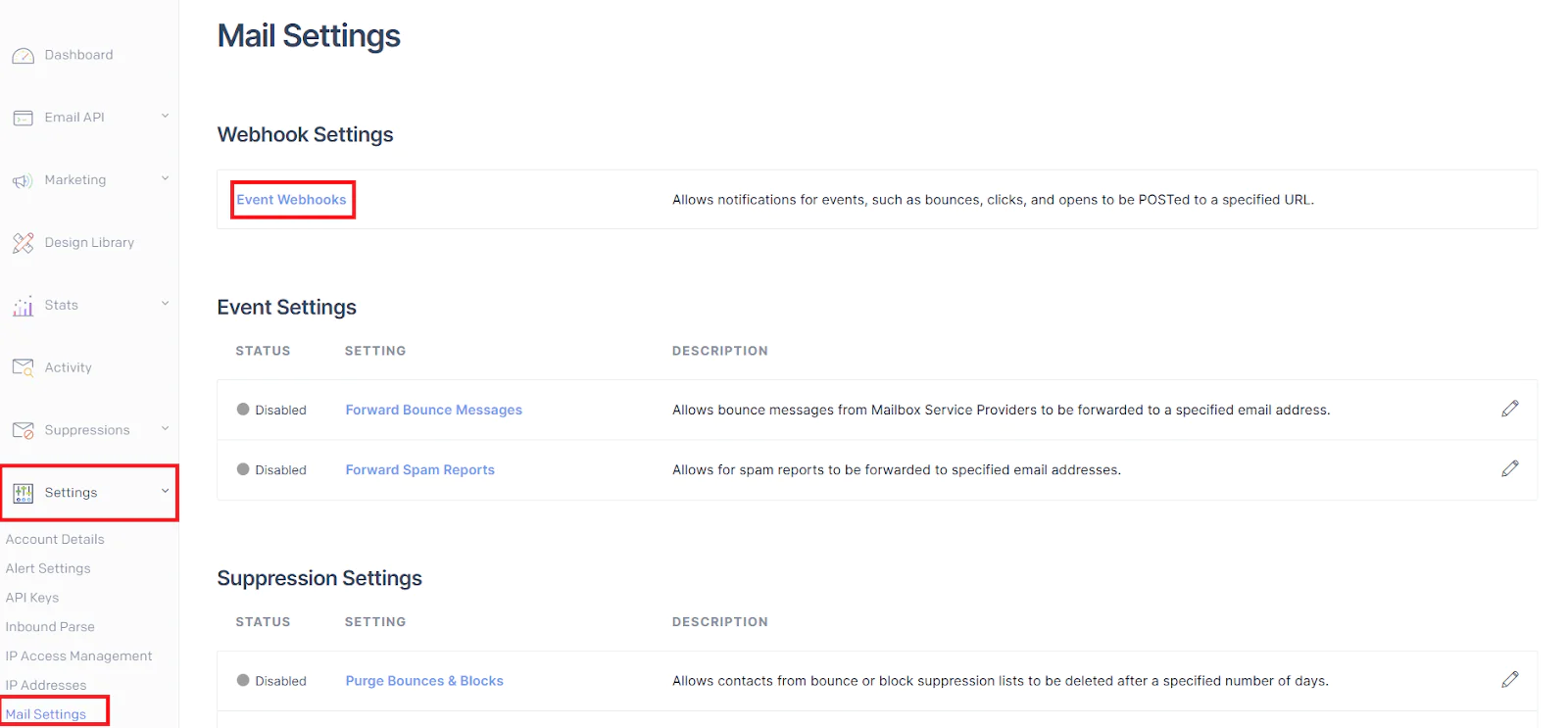
Click Create new webhook
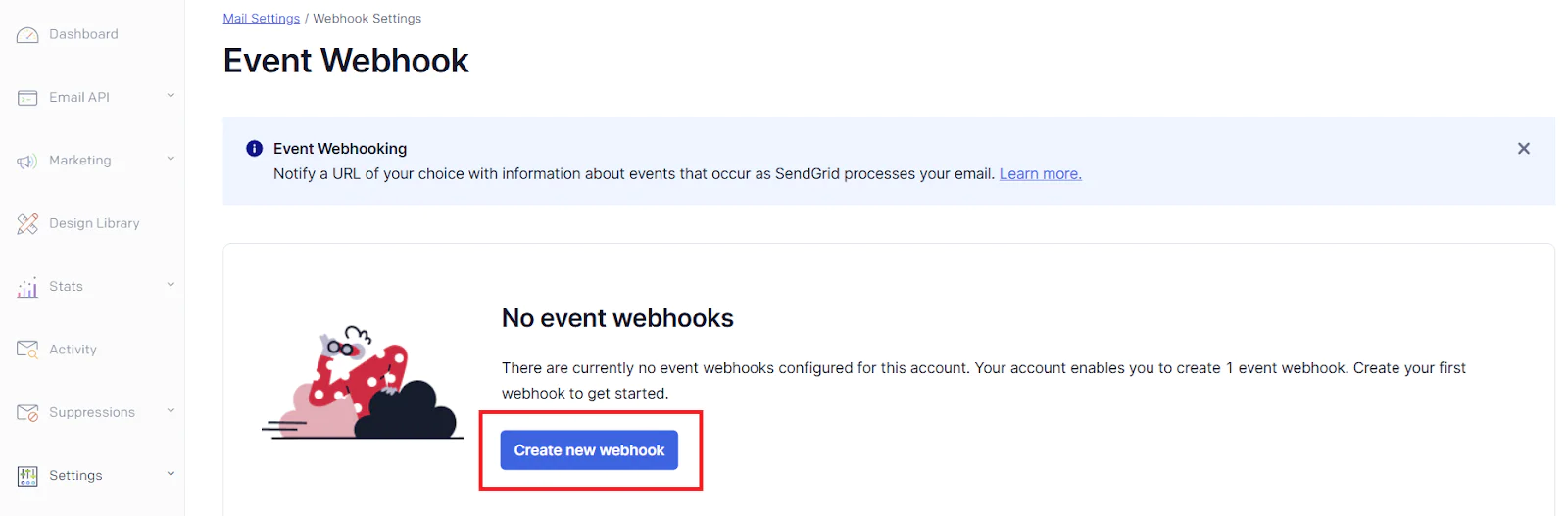
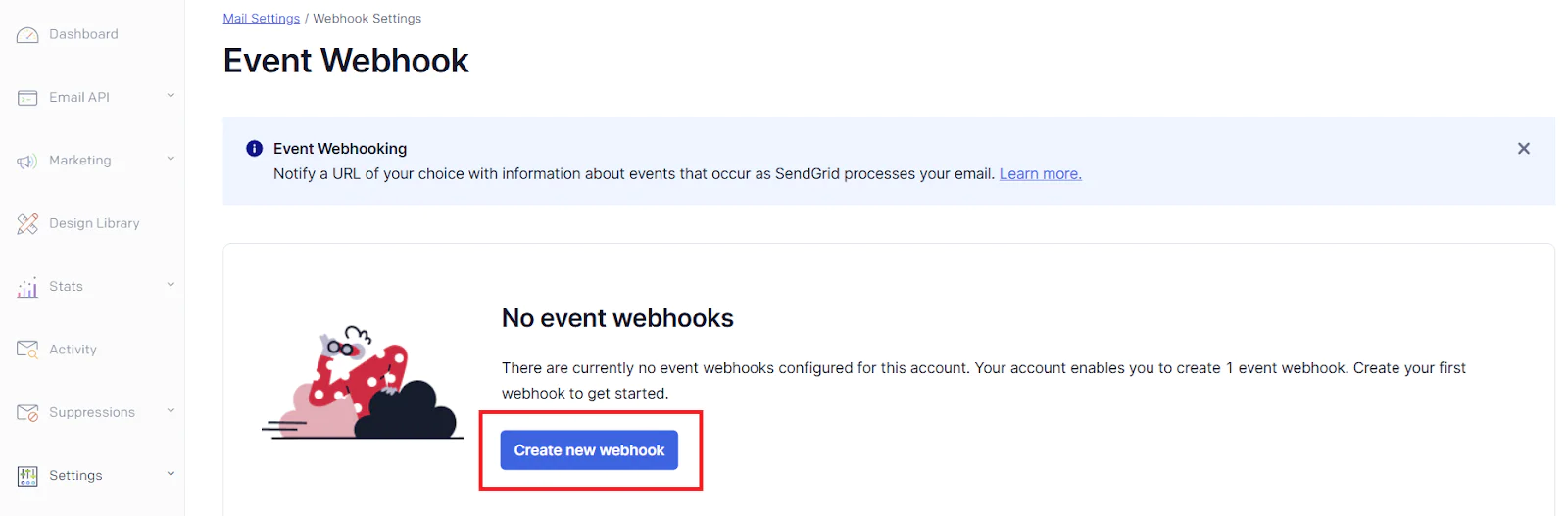
Add the details as shown below. Make sure the Post URL contains the ngrok url you exposed for your localhost, then add the route to the API to the end of this url: /api/webhook/
. Also select the events: Opened and Delivered.
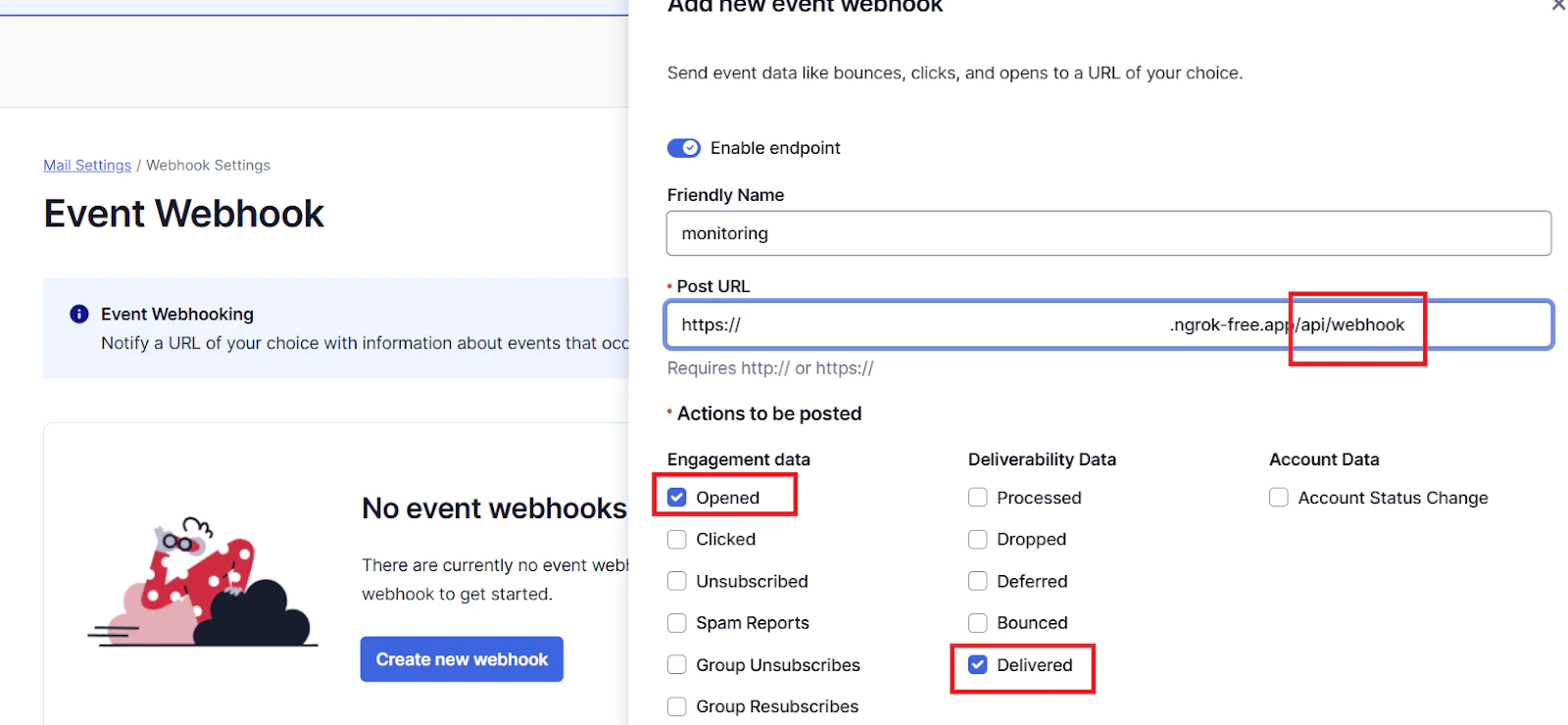
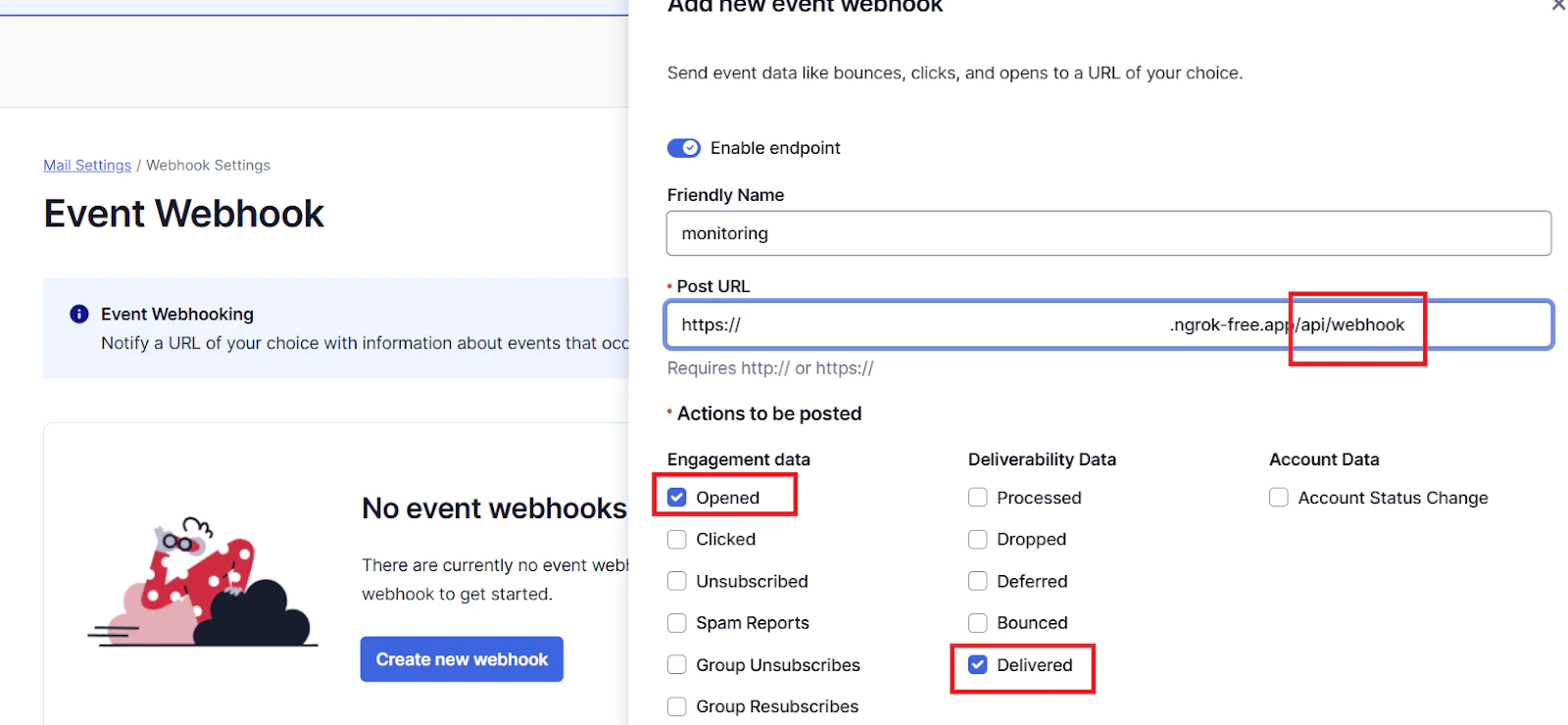
Testing your application
Now you will test your application's integration. Make sure your application is still running on the same port you created the ngrok link for.
Login to SendGrid’s dashboard. Go back to the event webhook you created and click the gear icon, then select Edit.
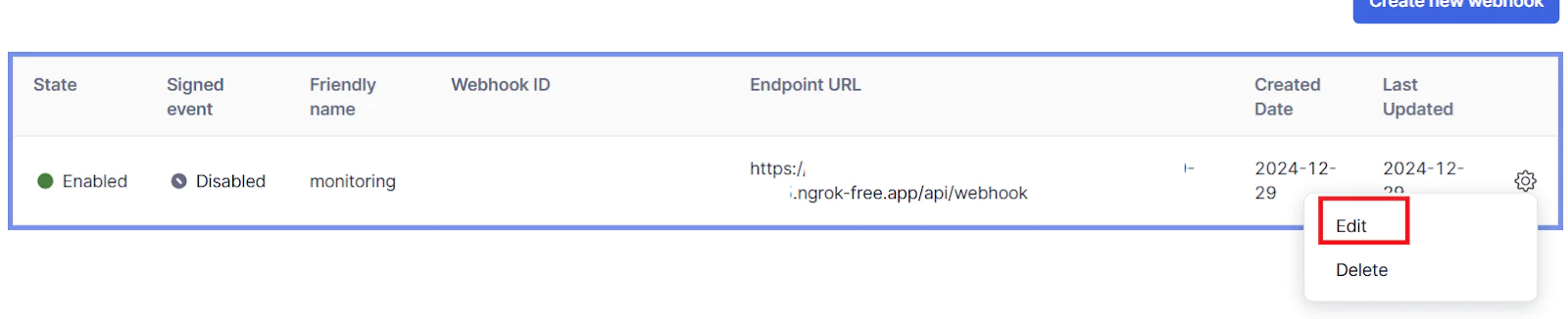
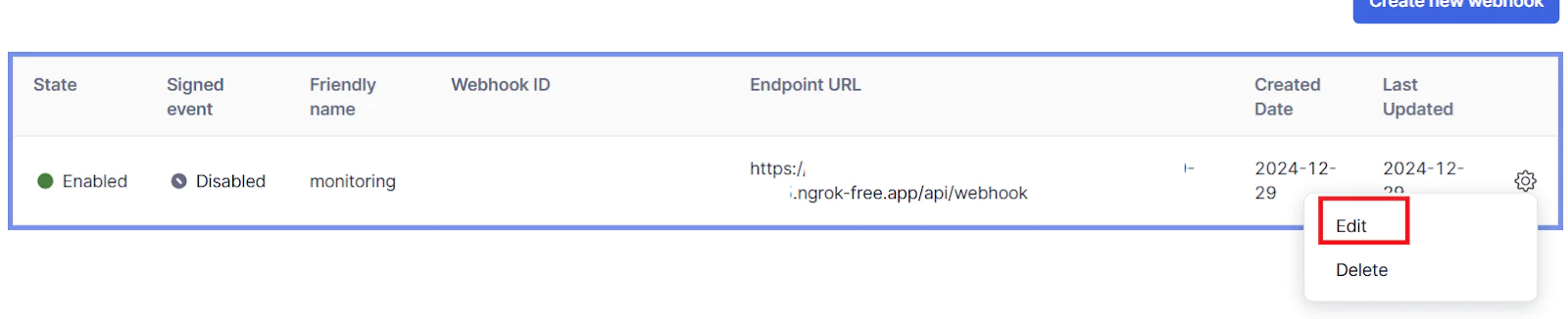
Then scroll down and click on Test Integration as shown below:
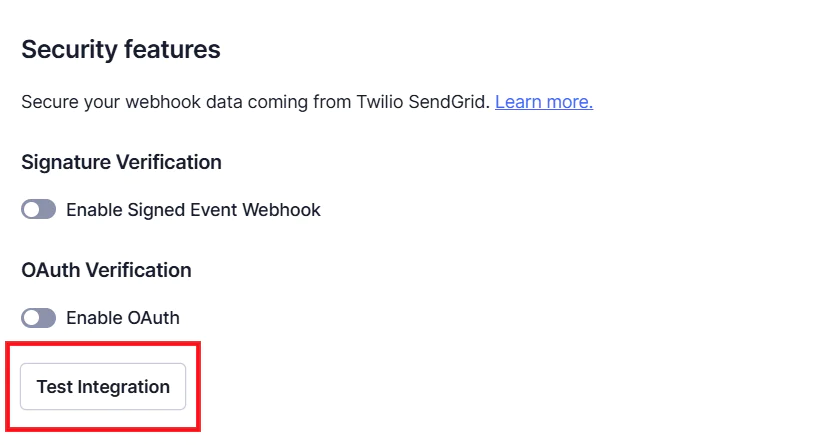
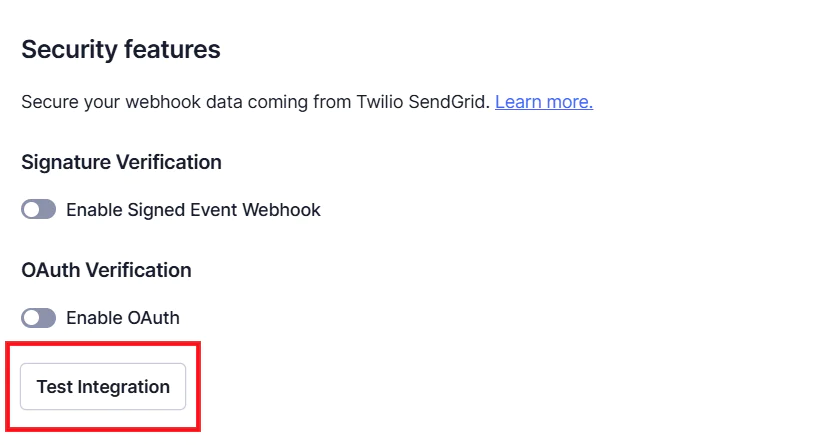
Then go back to the still-running ngrok CLI. You should see a trigger to the URL, and the status of the request as shown below:


If you go back to your project’s folder, you should find a log file created called webhook_events.log
. It contains the log of the triggered events that SendGrid sends in the test.
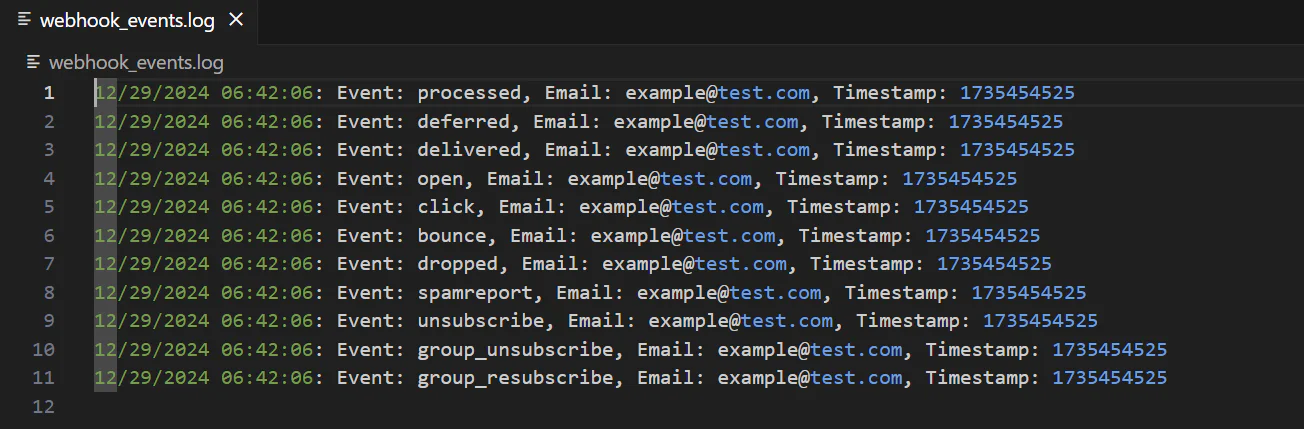
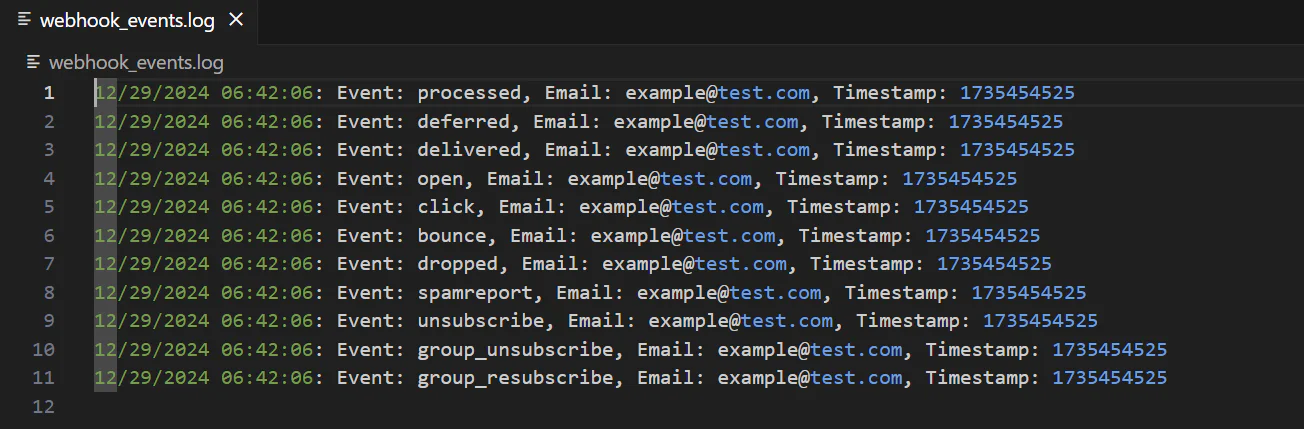
A recommended practice is to save these logs in an analytics database that would help you get good insights on your emails’ delivery and open rates over time.
Wrapping Up
Twilio SendGrid’s event webhooks are very powerful and can help you evaluate the success of your email campaigns and make informed decisions upon accurate results. The most important thing to remember is that you need to design your webhook endpoint correctly to make sure it accepts the event’s data format without errors and make sure it is enabled for the webhook to be able to send the request to.
Eman Hassan, a dedicated software engineer and a technical author, prioritizes innovation, cutting-edge technologies, leadership, and mentorship. She finds joy in both learning and sharing expertise with the community. Explore more courses, code labs and tutorials at https://app.pluralsight.com/profile/author/eman-m.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.