Secure Your Twilio Account: Monitoring and Alerts for Suspicious Activities
Time to read:
As telecom criminals evolve, businesses need to evolve with them to secure communications and protect against increasingly sophisticated attacks. Twilio has a suite of products and APIs which help you build communications experiences, but we also offer powerful tools that provide real-time insights and facilitate proactive threat detection.
In this blog post, we'll explore how to harness our APIs to set up robust monitoring and alerts, enhancing your security posture and helping you stay one step ahead of potential threats.
Prerequisites
The code examples in this post are provided for demonstration purposes. However, if you wish to follow along, you should have the following ready:
- A Twilio account - sign up here if you haven’t yet
- Python 3.x
- Node/ Express app
- Try my plum-bob demo TwiML app
- Python/ Flask app
- Get started with my flask_template app
- Twilio Helper Libraries
APIs and Features
Twilio Account activity can be broken down into three basic categories:
- Usage - Twilio products used/consumed by your application
- Events - Actions performed using a Twilio Account SID or Console User
- Alerts - Errors and Warnings that occur as a result of Usage and Events activity.
These are used in concert to keep track of what is happening behind the scenes of your application.
Usage Records API
Message segments, Programmable Voice Minutes, Phone Numbers, Lookups, and more are all tracked and can be reviewed via the Usage Record API.
The results can be fed to your own reporting or analytics tool for tracking, or you can use a Helper Library to make your own reports and scripts.
A GET
to /Usage/Records/Today.json
will produce all the usage from the current day.
Twilio provides multiple other time based endpoints for Usage Records:
- /Usage/Records/AllTime.json
- /Usage/Records/Daily.json
- /Usage/Records/LastMonth.json
- /Usage/Records/Monthly.json
- /Usage/Records/ThisMonth.json
- /Usage/Records/Yearly.json
- /Usage/Records/Yesterday.json
Usage Triggers
Twilio can send an email or webhook when certain defined usage related conditions are met.
Usage Triggers can be set up in the Console.
- Enter the Usage Trigger properties.
- Determine which action should be taken when the trigger value is exceeded.
- Click Save!
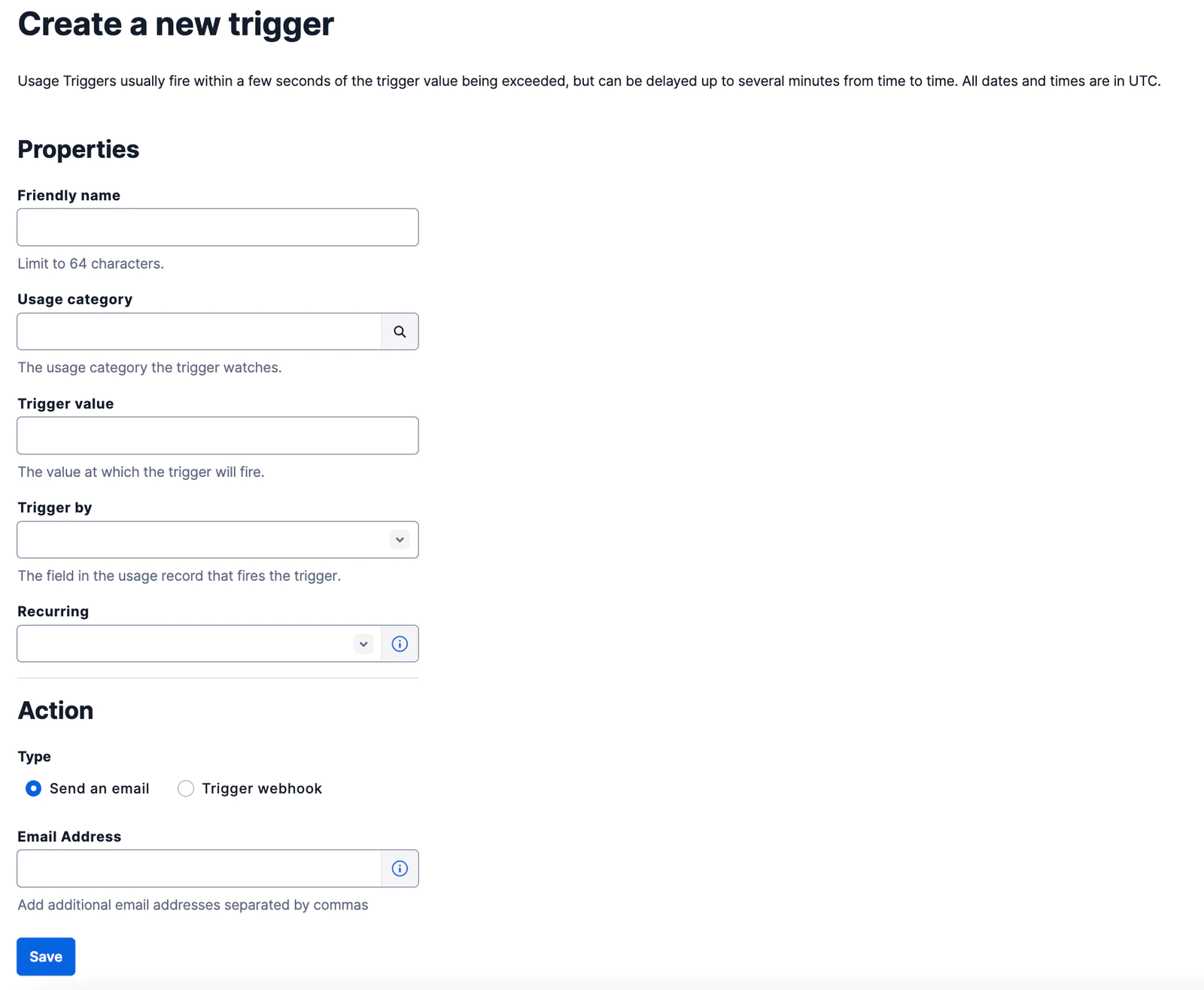
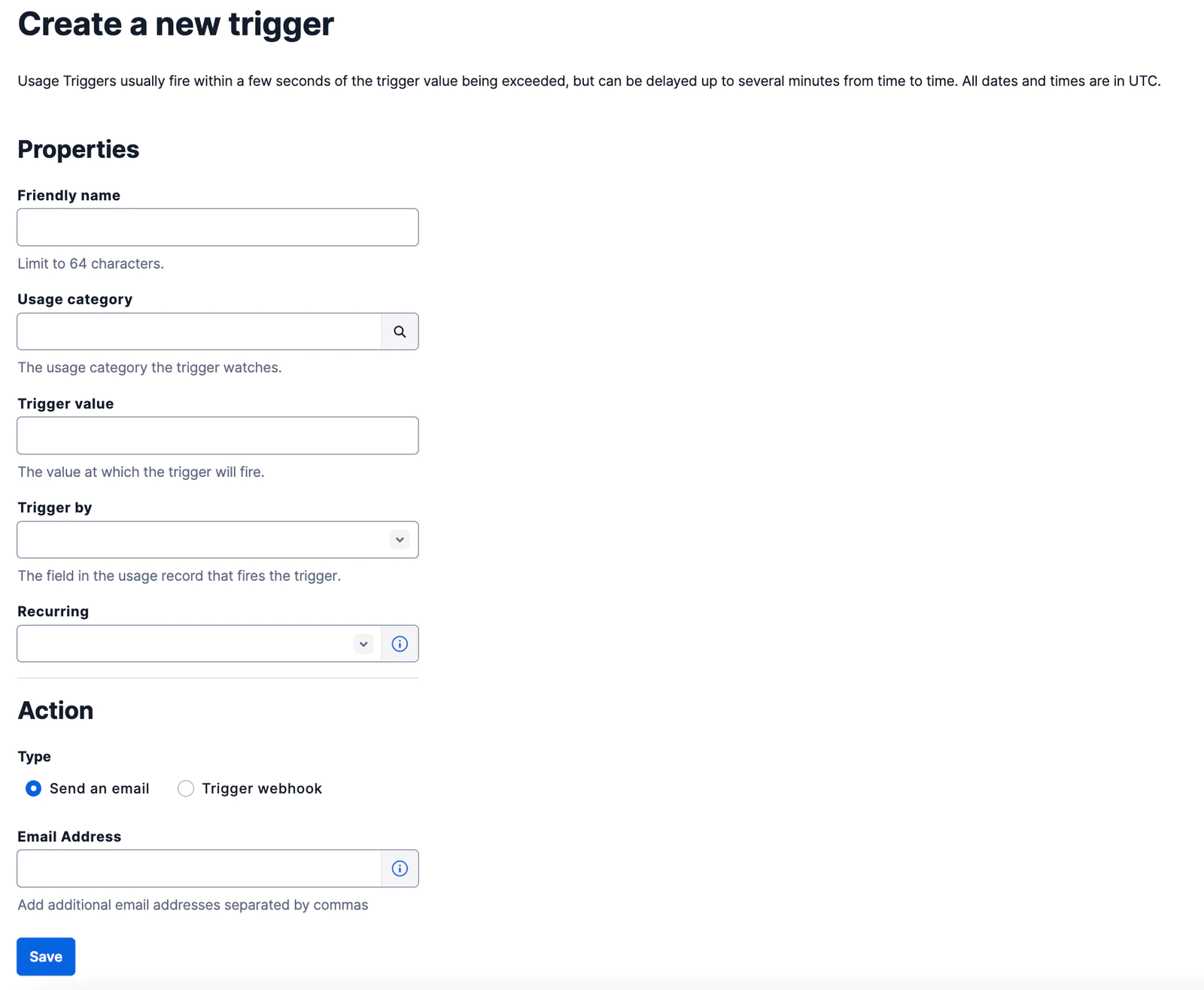
There is also an API for that. To create a trigger, POST
to /Usage/Triggers with your desired parameters. The CallbackUrl is what Twilio will hit when the usage threshold is reached.
When the usage value is exceeded, the webhook result should look something like this:
Debugger Webhook
In Console, users can define a Debugger Webhook that Twilio will hit whenever an Error or Warning occurs on your account.
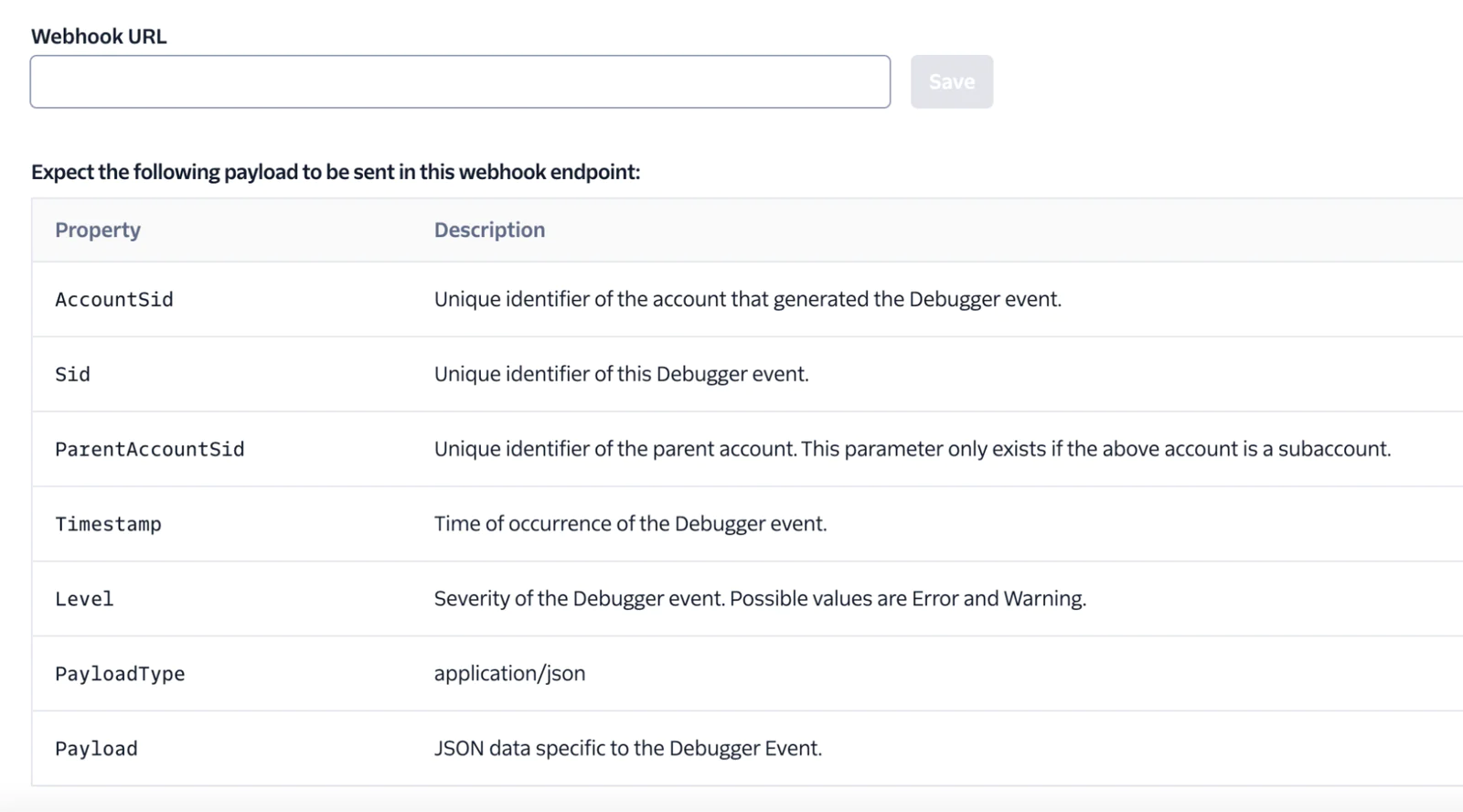
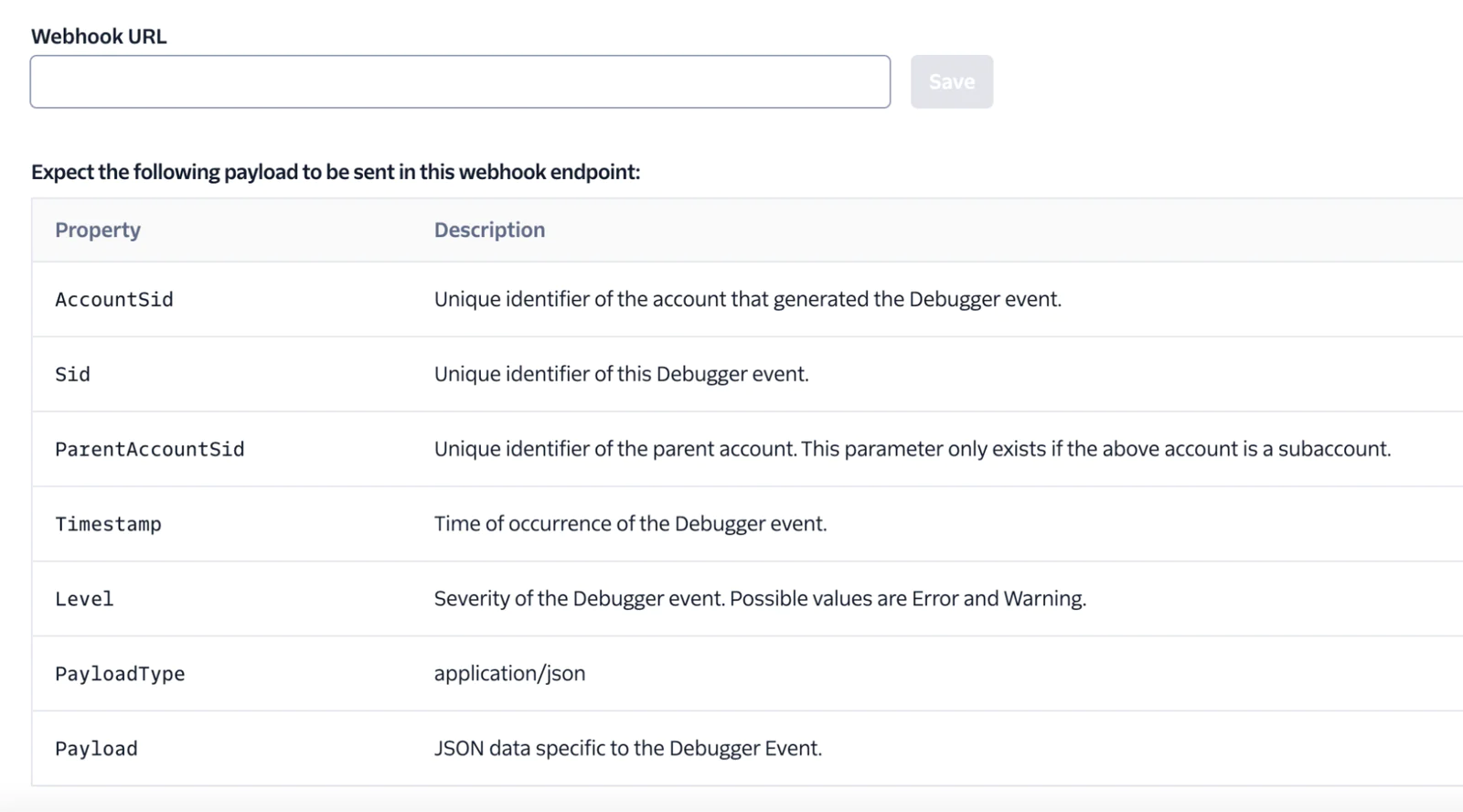
The application receiving the webhook from Twilio will be hit with every Error and Warning, so specific logic should be included to handle various cases. This node/express example route can be used to handle Invalid CallerID and Invalid Phone Number Format errors differently than everything else.
Console Alarms
Users can define different webhooks for specific warnings or errors, offering a more flexible alternative to the Debugger Webhook. Multiple alarms can be created to handle different Errors or Warnings with different behavior. Additionally, users can define different methods of how the Alarm will notify them - webhook, email, console notification, or all of the above.
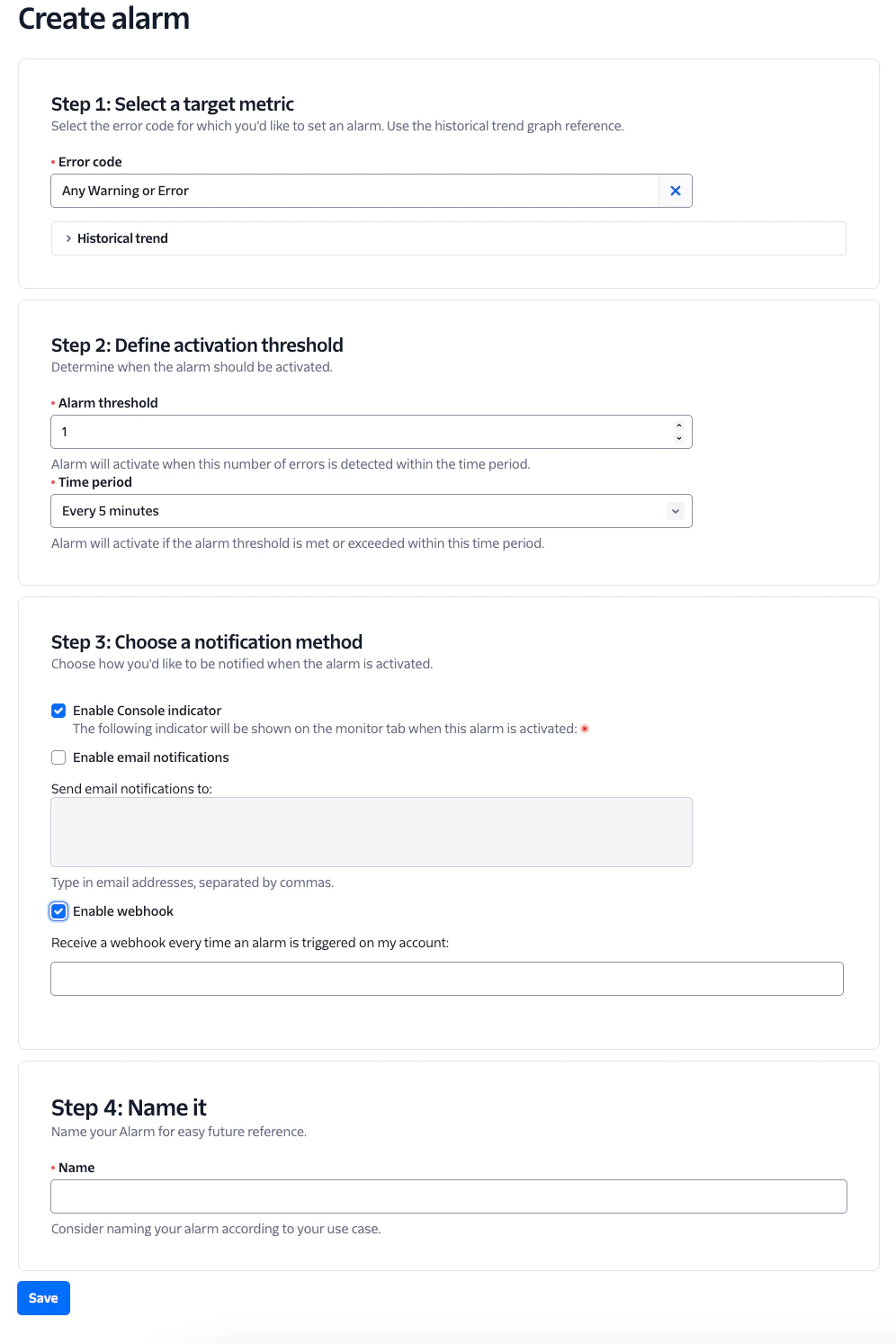
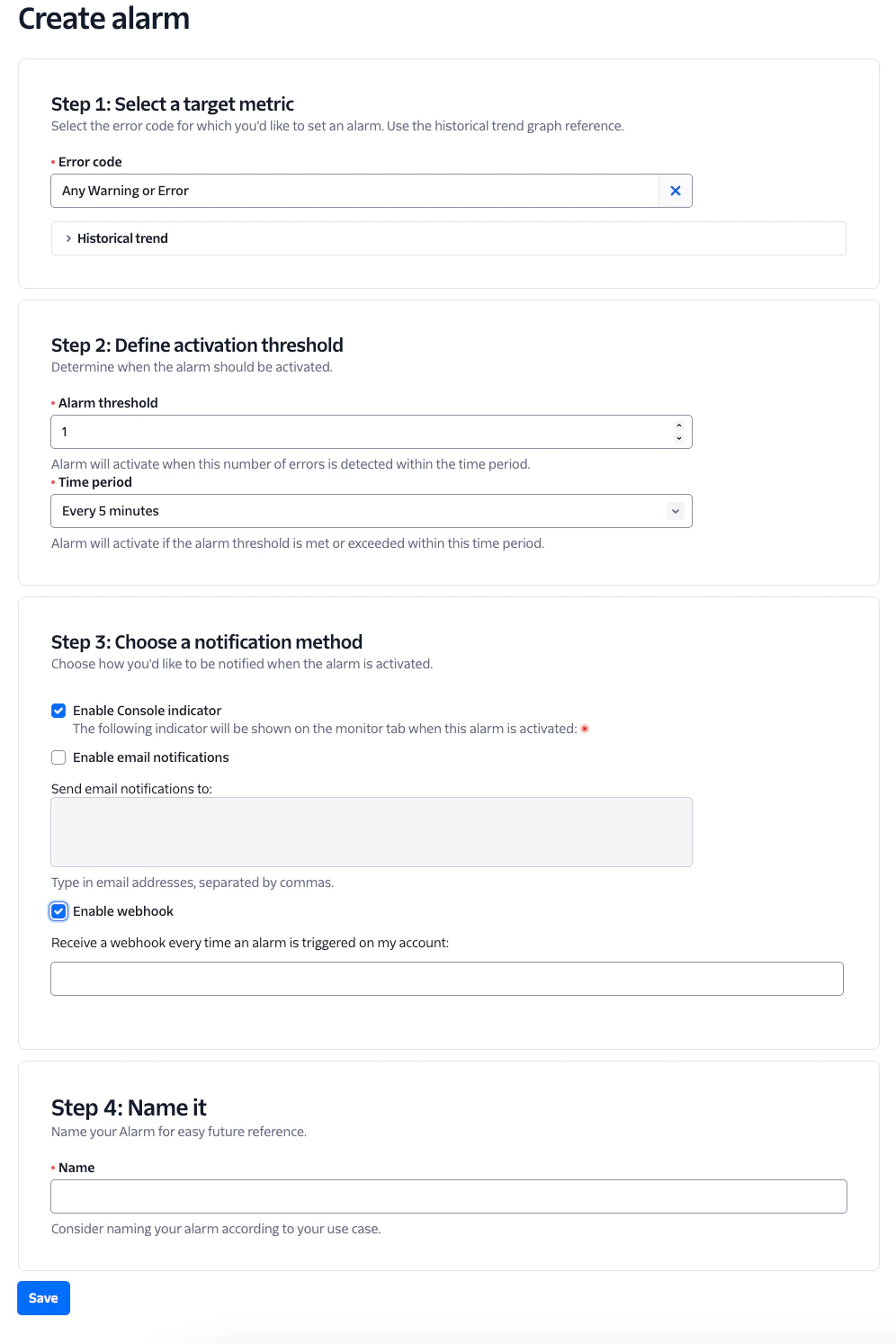
Monitor Alerts API
The Debugger Webhook and Console Alarms are designed to actively notify you of an Error or Warning as it occurs in real time. The Monitor Alerts API is used to retrieve historical information on these Errors and Warnings, after they occur.
This example will return details on errors that occurred in 2025 so far.
This example produces a bar graph with a total count of each error code in the defined time period. First, the alerts that have occurred this year are listed via the API. The results are then parsed and each unique error code is counted and plotted.
Visualizing this data could be very useful for establishing a baseline of error counts for more effective anomaly detection.
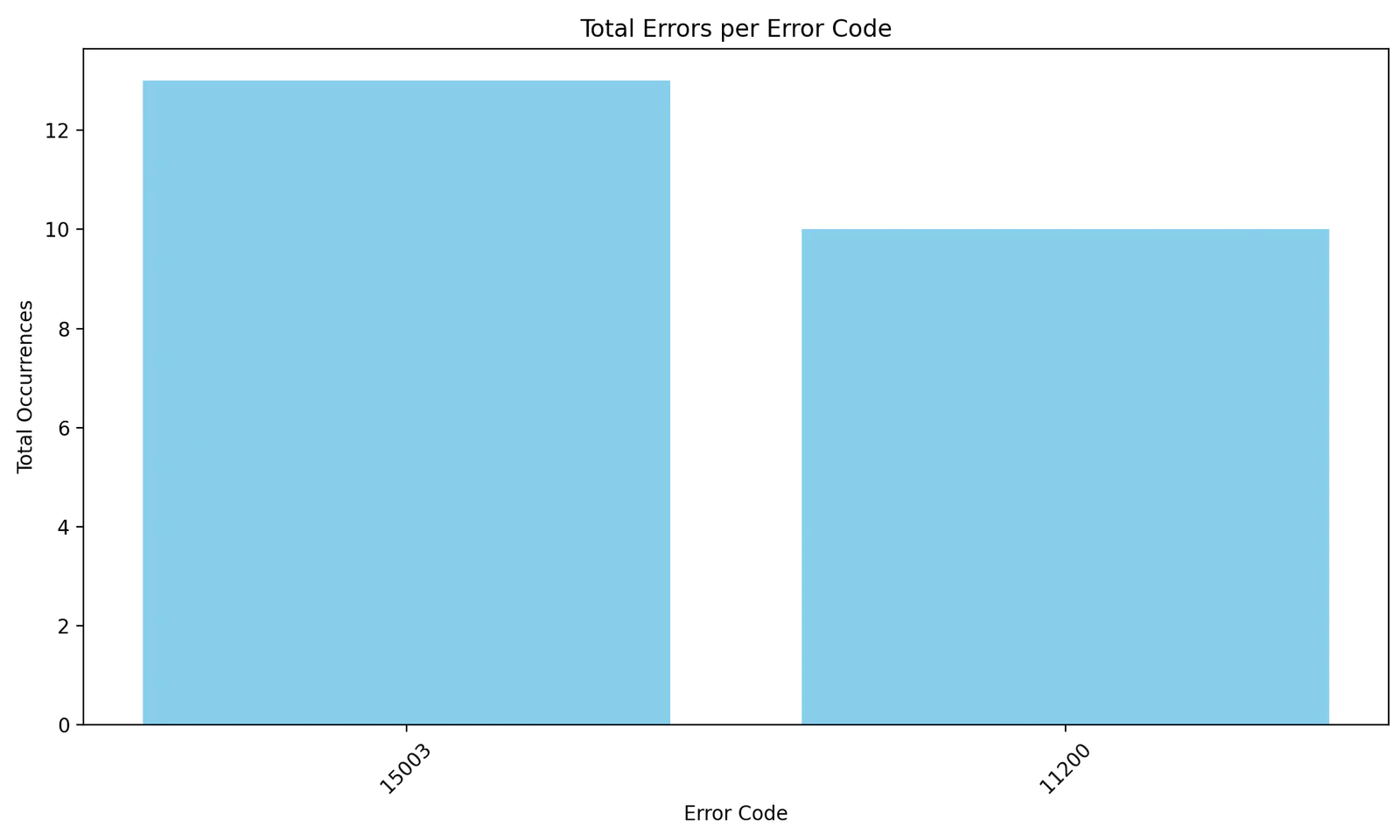
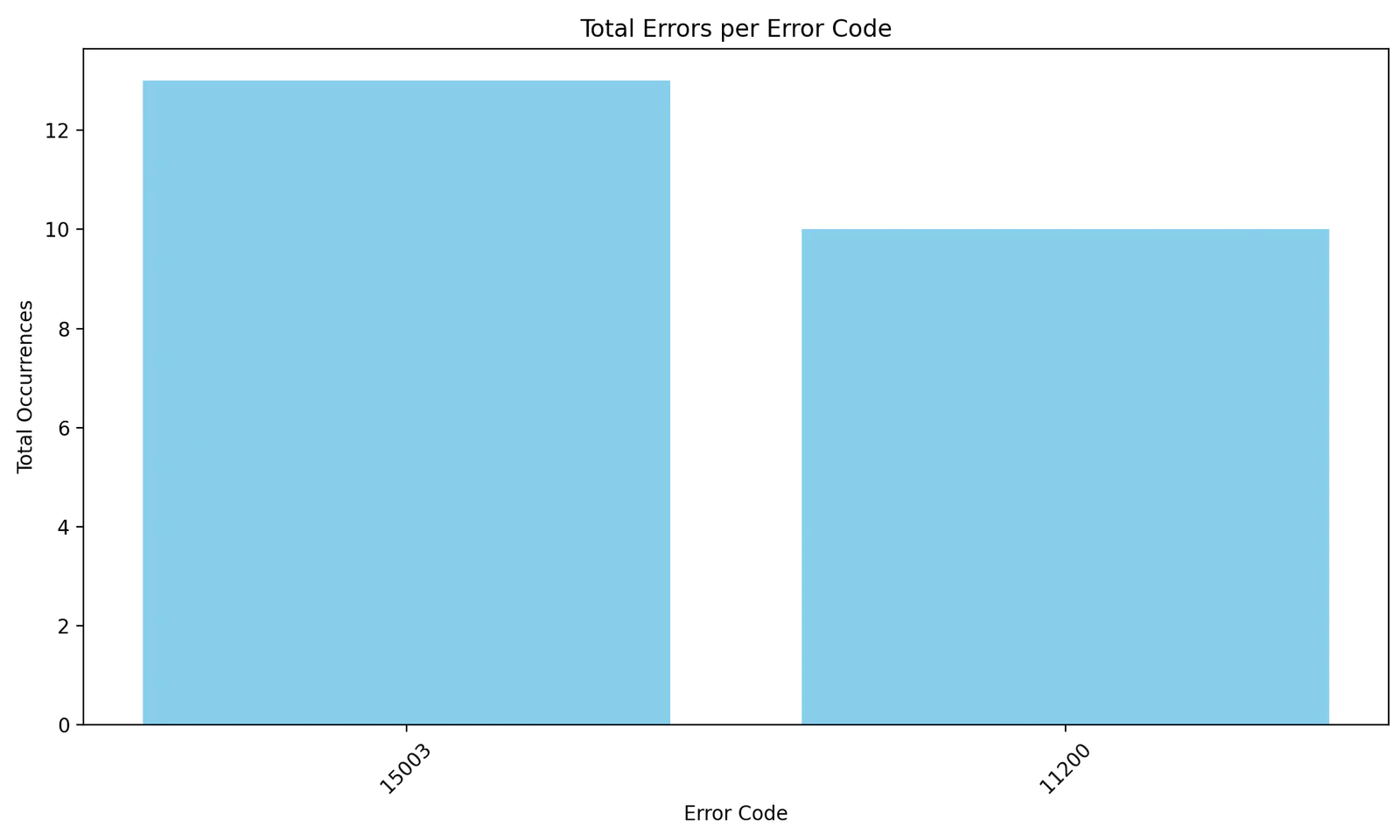
Monitor Events API
The Events platform offers detailed logging and change-tracking for all Twilio resources, like provisioning phone numbers and modifying security settings. The Monitor Events Resource allows you to access this log and can be integrated with your existing log management solution. This API is commonly used for periodic monitoring, post mortem investigations, and building a baseline of expected activity.
Twilio offers numerous Event Types which are available to audit via the Console and API.
Event Streams
The Event Streams REST API gives access to a flow of all interactions sent or received on Twilio. It allows streaming to multiple destinations, such as Twilio Segment, Amazon Kinesis, or webhooks, and includes features like consistent metadata, event queuing, and delivery from various Twilio products.
Setting up an HTTP sink is as easy as making a POST to /Sinks.
Test Cases
With a firm understanding of Twilio’s various Monitoring and Usage related APIs and features, we can apply these concepts to real situations.
Account Takeover (ATO)
An Account Takeover is when a bad actor assumes control of online accounts owned by other people. With control over your Twilio account, a bad actor can send malicious emails, make expensive calls, or launch smishing campaigns at your expense.
The best way to avoid an ATO is to keep your Twiliio account secure.
- Use strong passwords
- Protect your credentials
- Implement periodic rotation where applicable
- Use roles and permissions specific to jobs/ tasks
See the Anti Fraud Developers guide for more info on developing with security in mind.
However, prevention is only part of the equation. You should consistently monitor for unauthorized usage, changes to services, and other suspicious activity on your account, and compare them to a normal baseline to identify potential issues quickly.
Auth Token/ API Key Rotation
One of the first things a bad actor might do when they gain access to an account is to change the credentials so the original owner has a harder time stopping the malicious activity.
If your Auth Token is rotated without your knowledge, requests will begin to fail. Keep track of these errors by setting an Alarm. The 20003 Error is generated when your Account SID is used to make an API request with an invalid Auth Token.
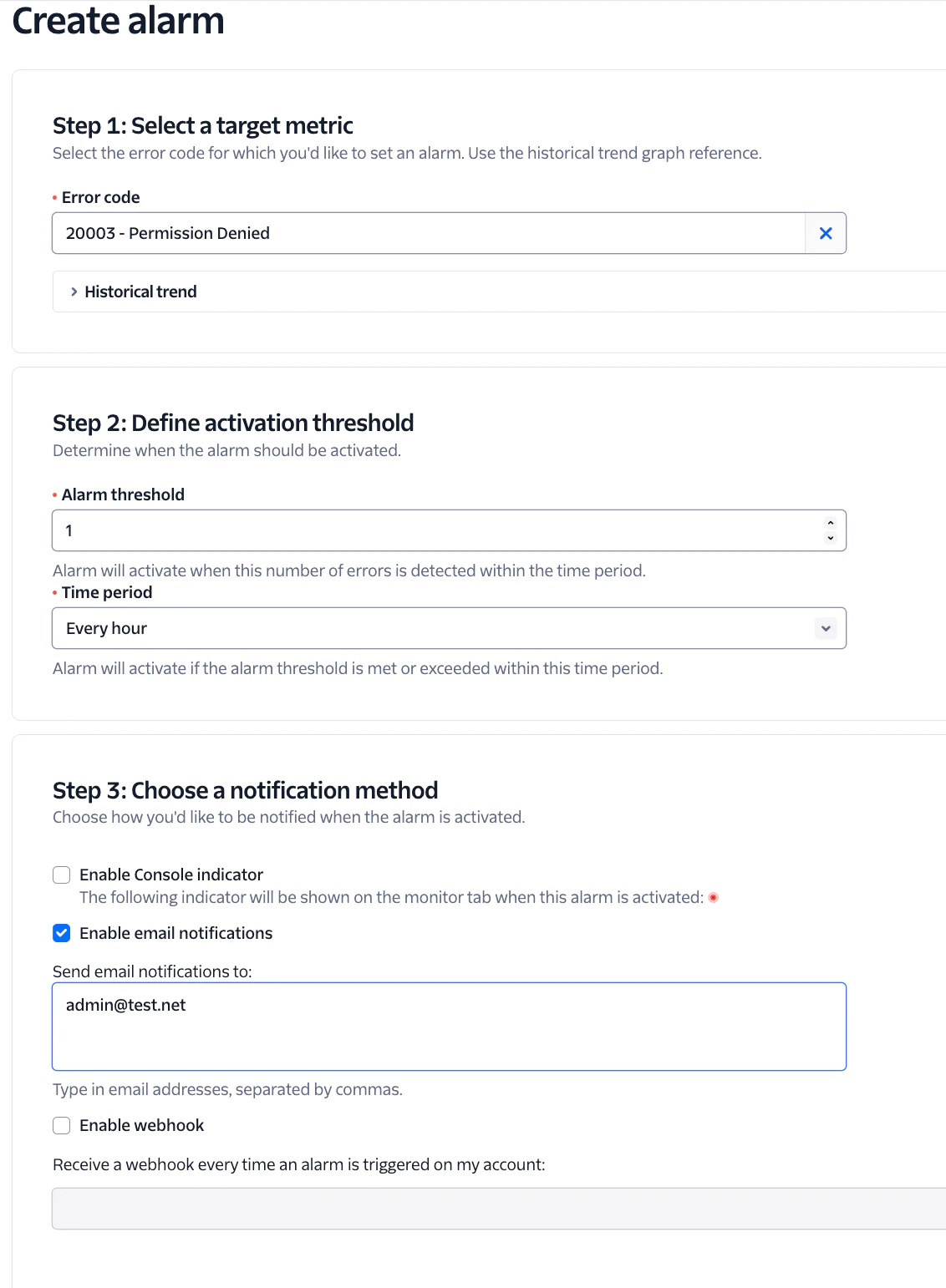
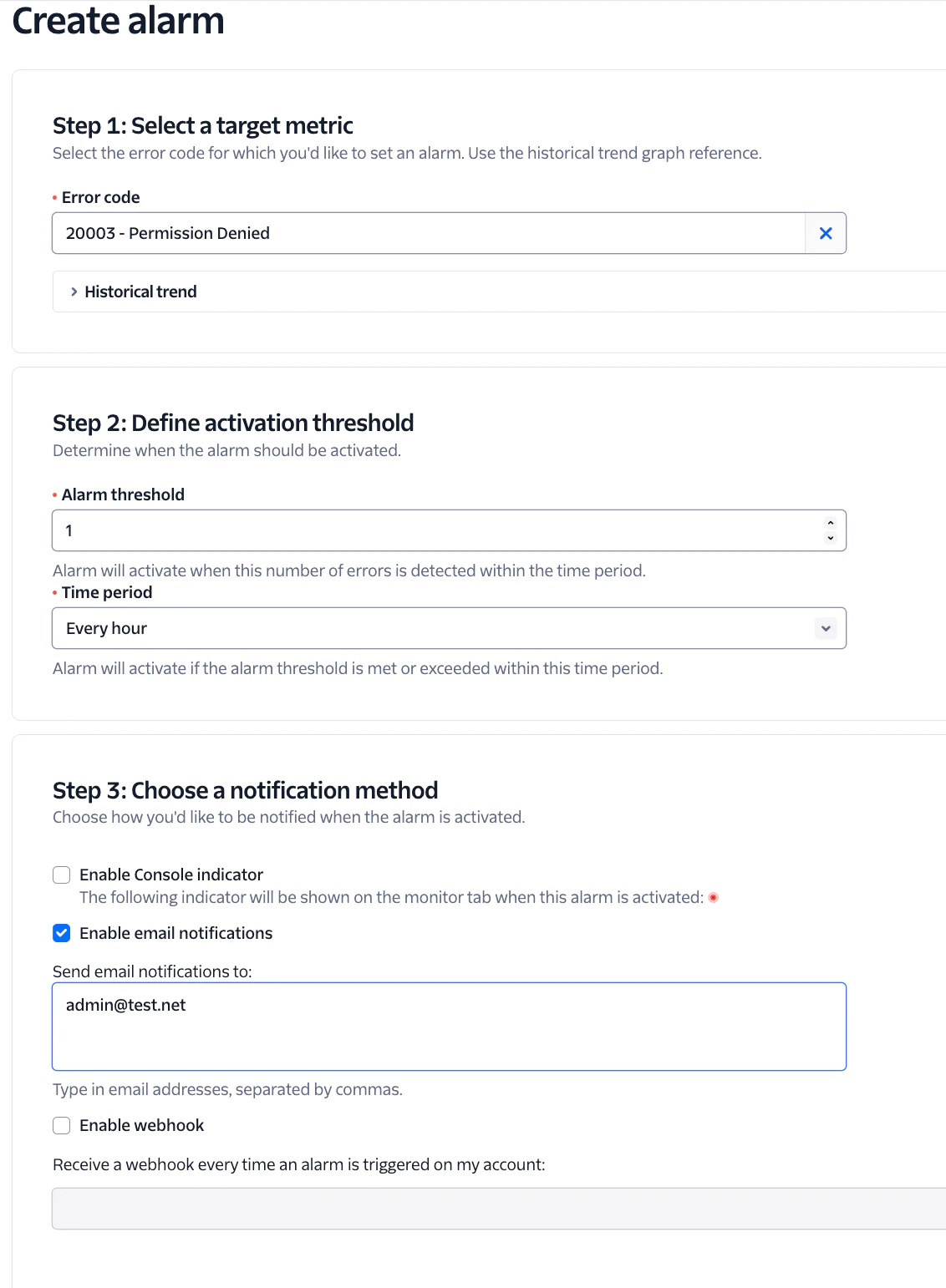
In the event a subaccount is compromised, the Parent account credentials can be used to scrape the Monitor Events Resource for auth token related events. Something like this can be periodically run as a cron job to notify an admin via SMS if unexpected auth token rotations have occurred.
Changes in Activity
Being familiar with the expected behaviors of your app, even during extreme conditions, is extremely important when trying to detect suspicious activity. If your Twilio app does not utilize Lookups, Lookup usage may be a sign of an attacker gathering information on potential targets. This Usage Trigger will shoot a webhook every day where at least 1 Lookup was performed.
Toll Fraud/ Toll Pumping
Toll Fraud, Traffic Pumping, Call Pumping, International Revenue Share Fraud (IRSF) are all different names for the same basic concept - fraudsters generate calls to premium-rate numbers they own to generate income from the call charges. If an ATO takes place, the bad actor may begin to attempt Toll Pumping at your expense. Similarly, SMS Pumping is when bad actors send messaging traffic to numbers they control, for a share of the generated revenue.
In V2 of the Lookup API, Twilio provides the SMS Pumping Risk Score to determine a particular phone number’s history and help prevent fraud.
For Verify customers using the SMS channel, Fraud Guard is enabled by default at no additional cost. When creating your Verify Service, you will have the opportunity to set a Protection level based on your risk appetite. Twilio establishes a baseline of expected activity to help identify unusual traffic patterns and filters out fraudulent activity.
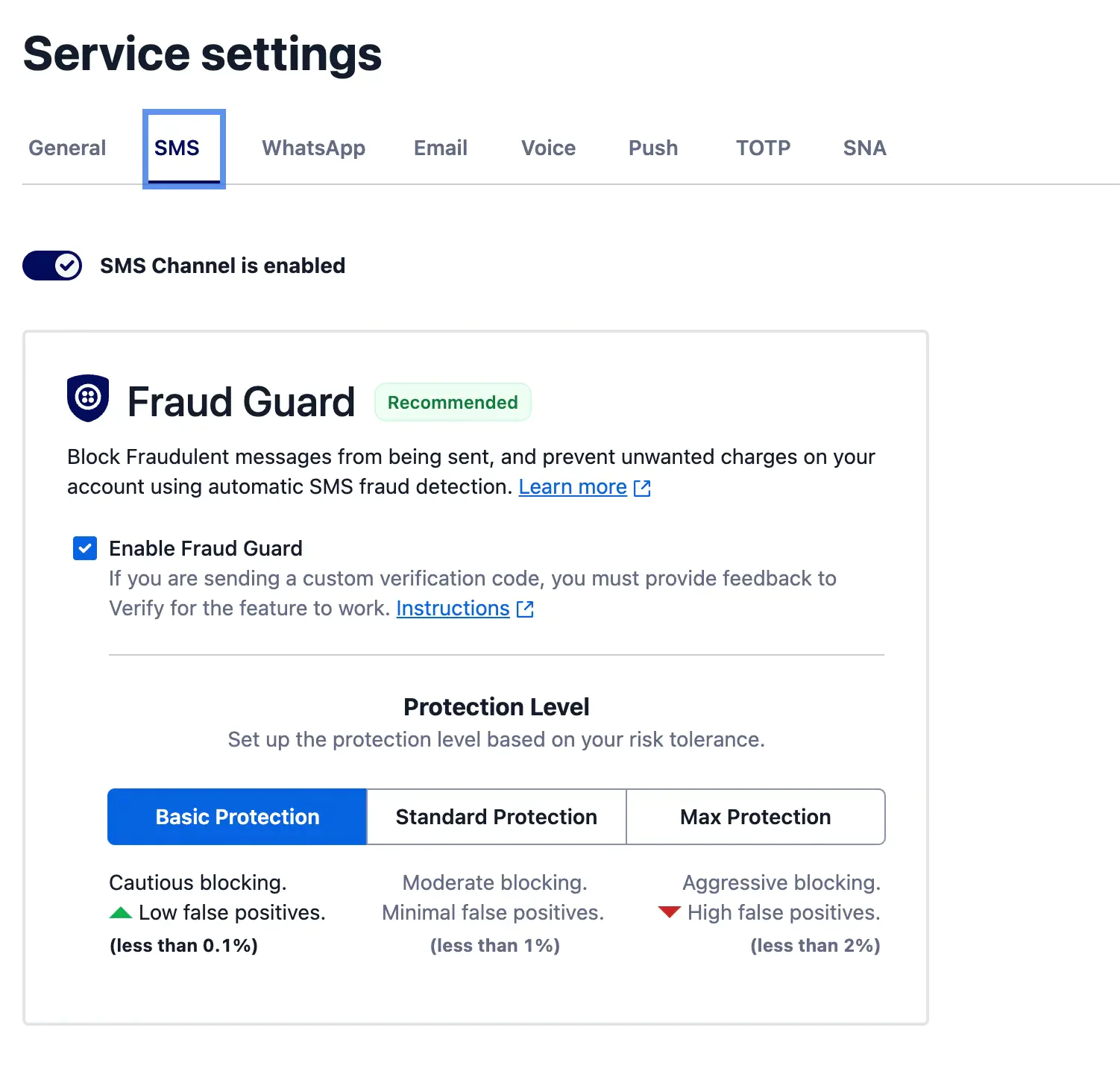
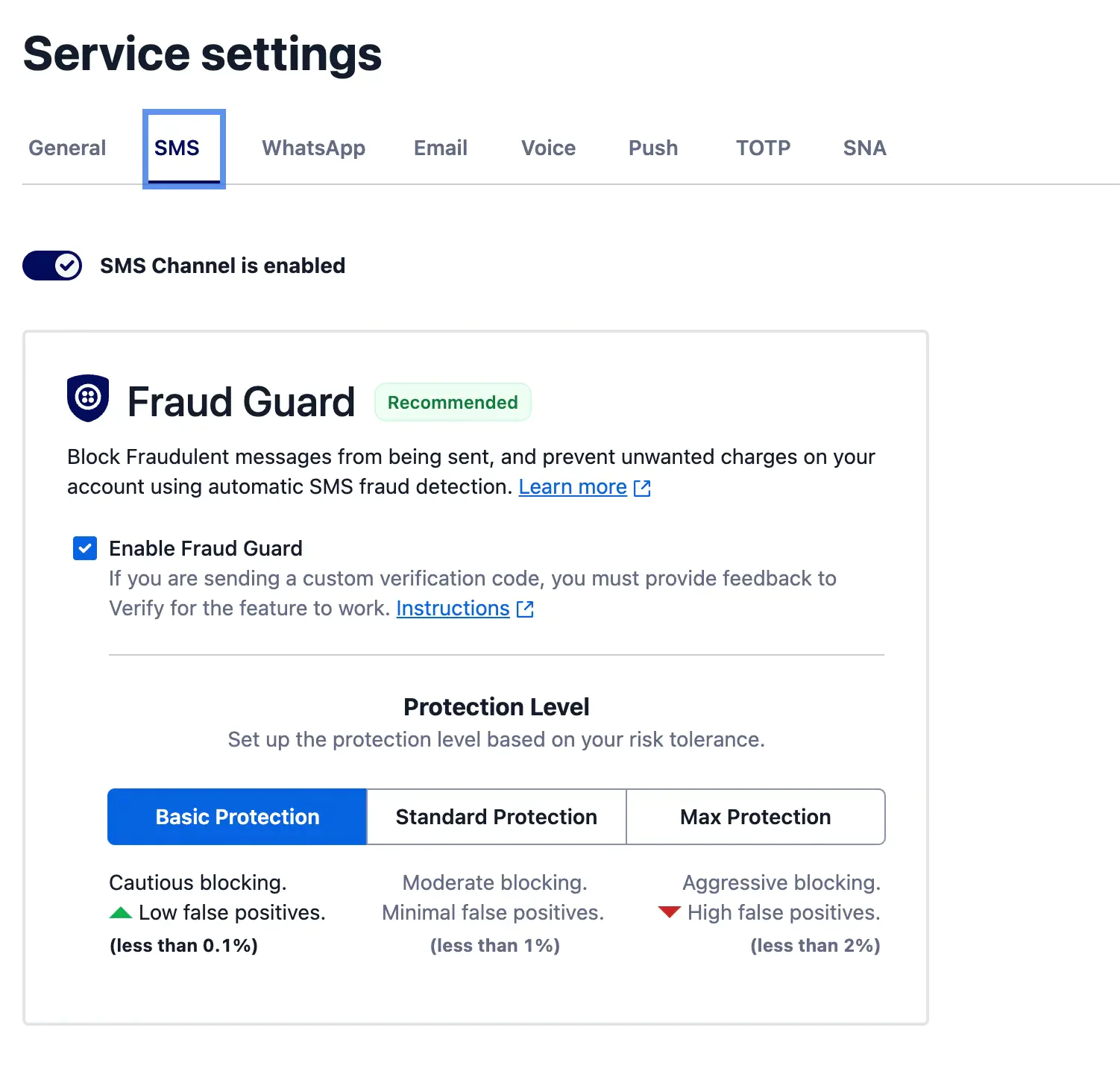
Monitoring your account activity and alerting on strange or unexpected behavior can further help you detect and act on malicious behavior before it spirals out of control.
Geo Permissions
Twilio offers location based permissions for communications using Programmable Voice, Elastic SIP Trunking, Programmable Messaging, and Verify. Taking the time to set Geo Permissions based on expected activity, and auditing them periodically, are crucial security measures to keep in mind when building. For example, if your application is designed for users in certain locations, it is likely safe to disable other locations entirely.
Once access to an account is gained, Toll Pumpers will sometimes try to open a bunch of high risk geo permissions before initiating calls and messages.
This example scrapes Monitor Event Resource and produces a line graph to visualize call volume over time.
In the resulting graph, the red lines indicate at least one voice-geographic-permission
event took place on that date, and the blue line tracks the number of daily calls.
In this hypothetical example, we can see a steady increase of calls since the geo-permission event on March 1.
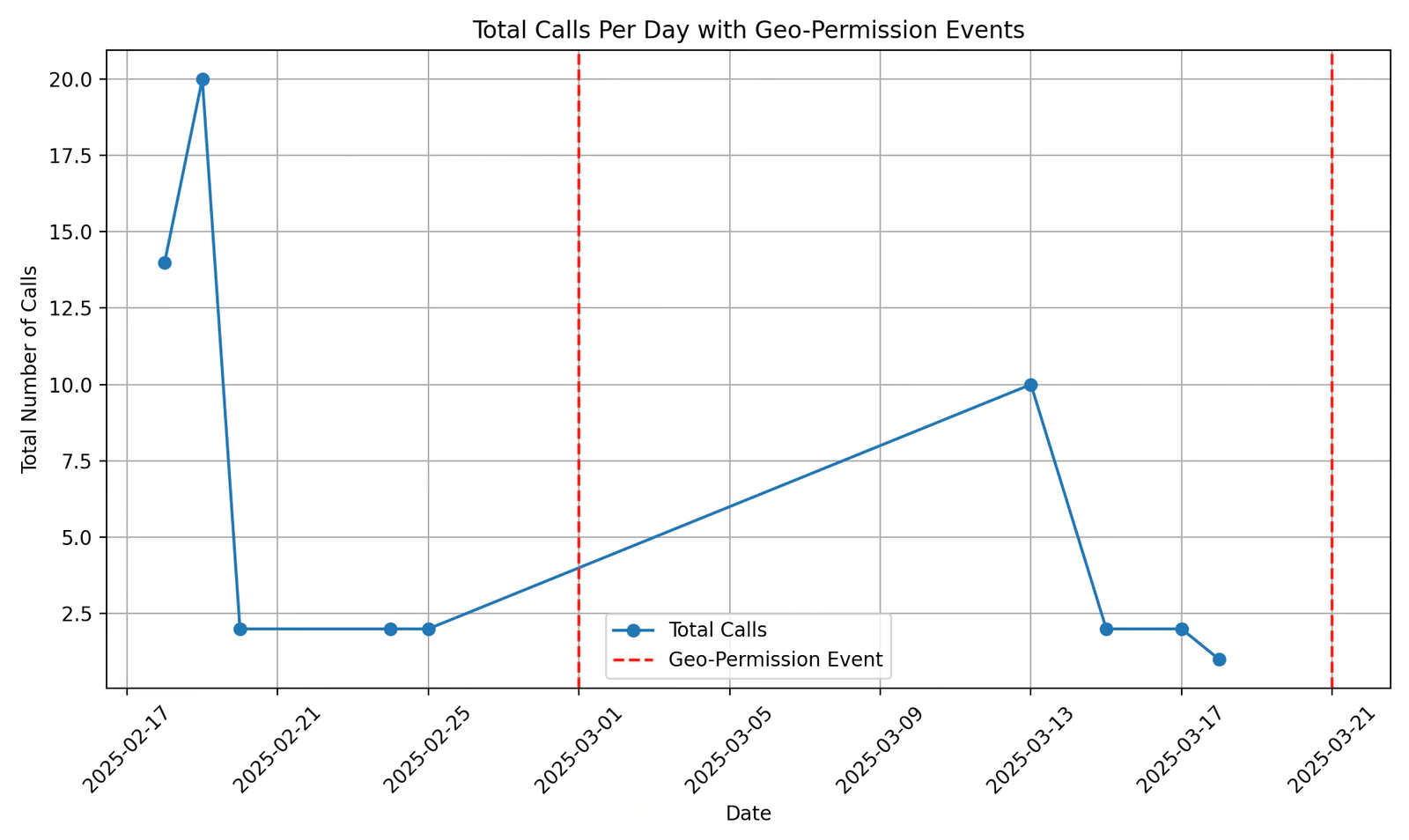
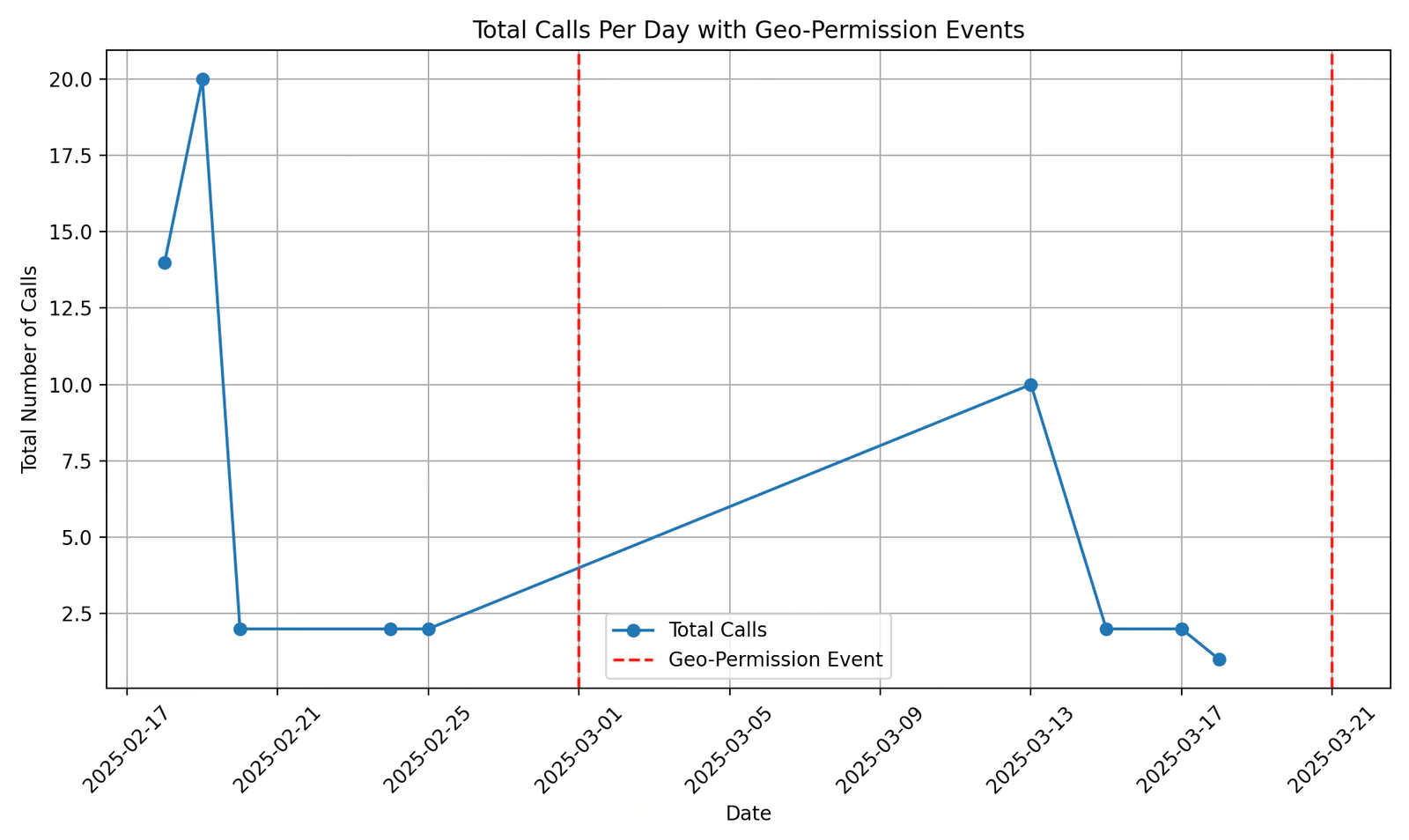
Additionally, you may opt to set up debug Alerts for the Geo Permission related Error Codes.
- 13227 - Geo Permission configuration is not permitting Programmable Voice call
- 32205 - SIP Trunking: Geo Permission configuration is not permitting call
- 21215 - Geo Permission configuration is not permitting call
Or, capture Call related Events in an HTTP sink, and analyze the country codes. Subscribing to the com.twilio.voice.api-request.call.created
event will allow you to analyze every call that is created by POSTing to your Account’s /Calls resource.
Event Streams offers an extensive list of event types to tune your monitoring and alerting. You can also request a new Event Type if the one you need isn’t listed.
Traffic Increase
A sudden increase in call or SMS traffic can be a wonderful thing! Maybe a recent campaign took off, or business is booming after the launch of a great new widget. It could also be a sign of trouble. Telecom criminals expect to be discovered in short order, and will move to their next target. So, once they gain control of an account they often act fast and make large numbers of calls in a short amount of time.
Use the Usage Records API to establish a baseline of activity for your application. Then, Usage Triggers can be implemented to let you know if this baseline is exceeded. It is important to continuously monitor your account and subaccount usage for any unwanted abuse.
This example scrapes Call Logs and determines the average number of calls over a given period of time, and creates a Usage Trigger for when the amount exceeds 20% of the average. Something like this can be implemented as a cron job to update Usage Trigger dynamically as your natural traffic patterns ebb and flow.
Basically, when usage is exceeded a call will be made with a notification explaining the details.
The callback_url
is a Function containing the following code, which will send an outbound call when triggered. The URL is a TwiML Bin containing a <Say>
message to be played when the recipient picks up. Mustache templates can be used with TwiML bins to produce dynamic messages.
From there credentials can be rotated, Geo Permissions can be altered, or other remediation efforts can be taken per your incident response plan.
Messaging Intelligent Alerts
Twilio uses Machine Learning/AI to identify anomalies in your outbound messaging traffic by analyzing multiple data points like error codes and message volume. Intelligent Alerts are similar to Alarms, but are handled by Twilio instead of set manually via the Console or API.
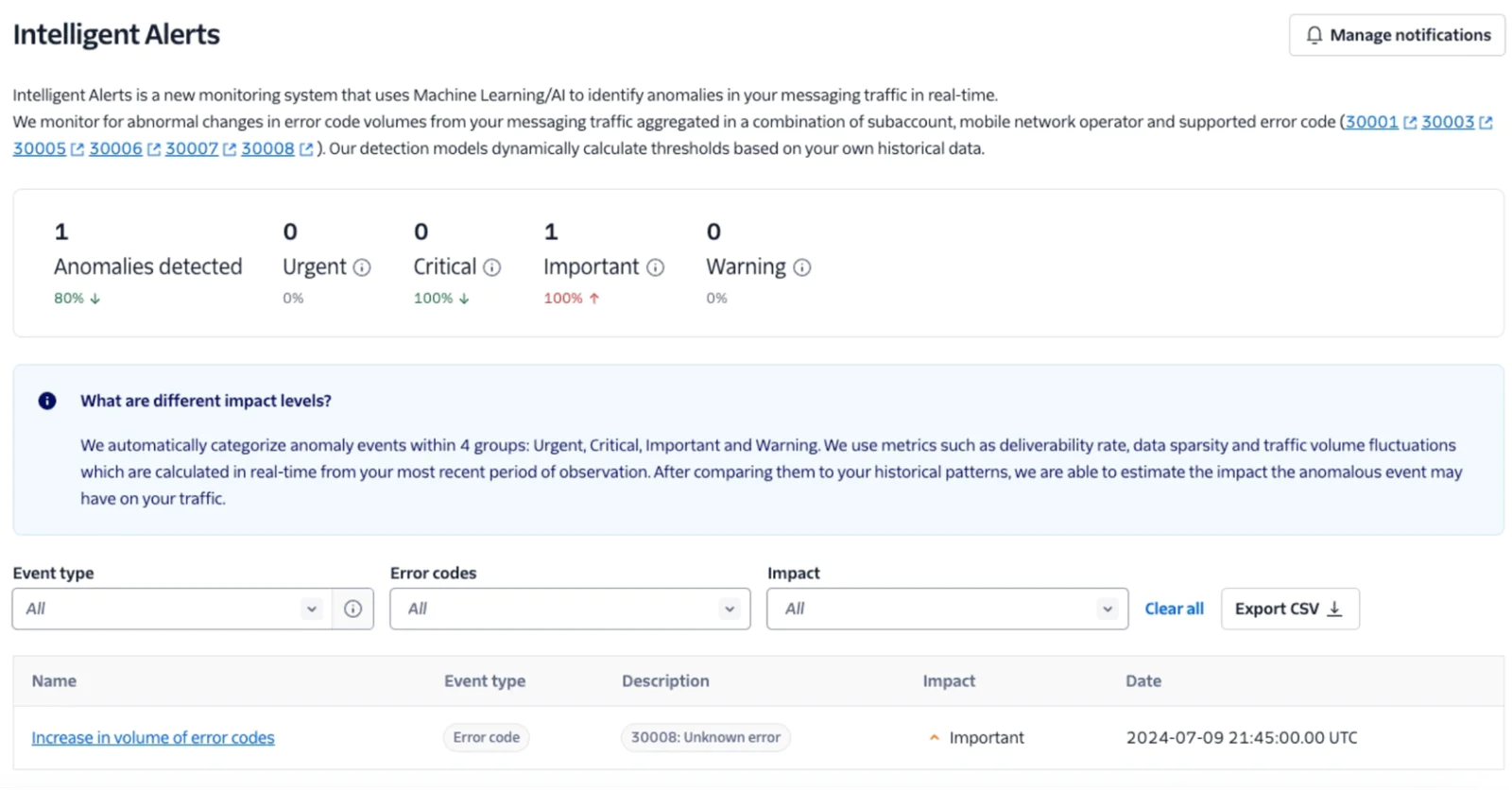
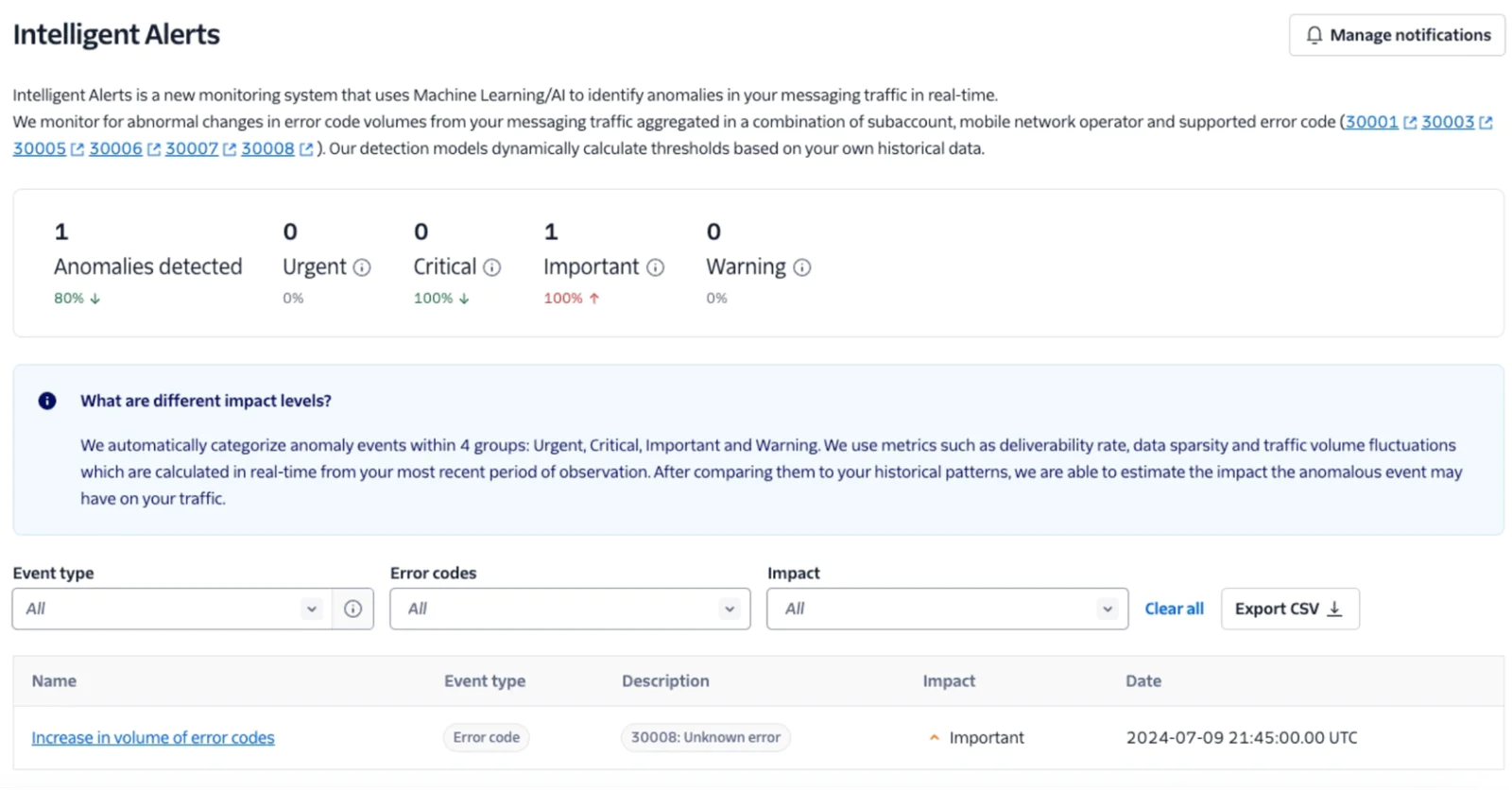
DDoS/Server Side Hacking
While the Twilio side of your app may be locked up, the provider hosting your app’s logic may not be. In the event your server is compromised or otherwise unavailable, Fallback URLs will come in handy.
The most common use of a fallback URL is when the primary handler for an incoming call or SMS fails. To maintain continuity you can duplicate your application logic, or choose to provide temporarily scaled down backup services via this secondary URL.
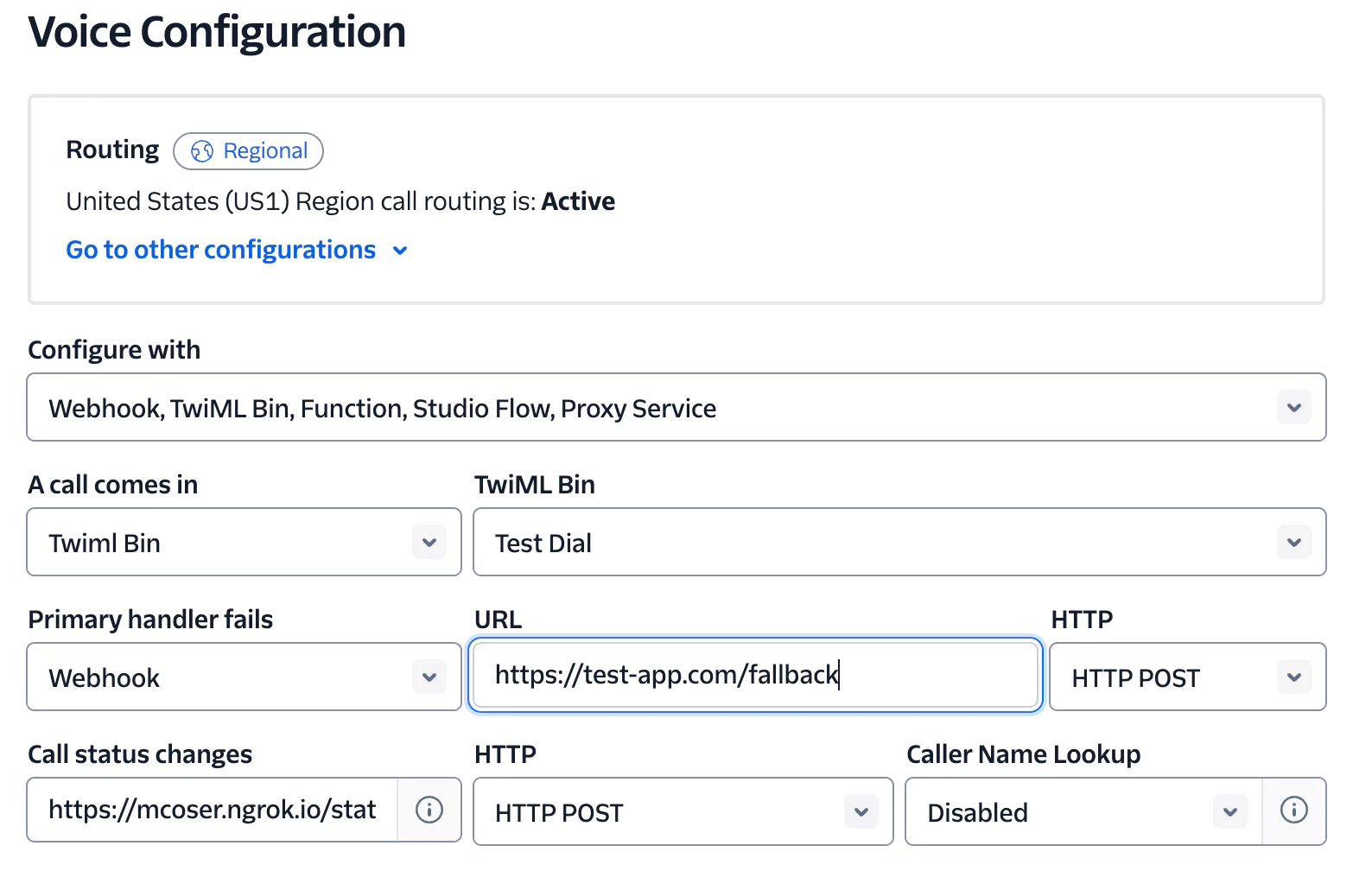
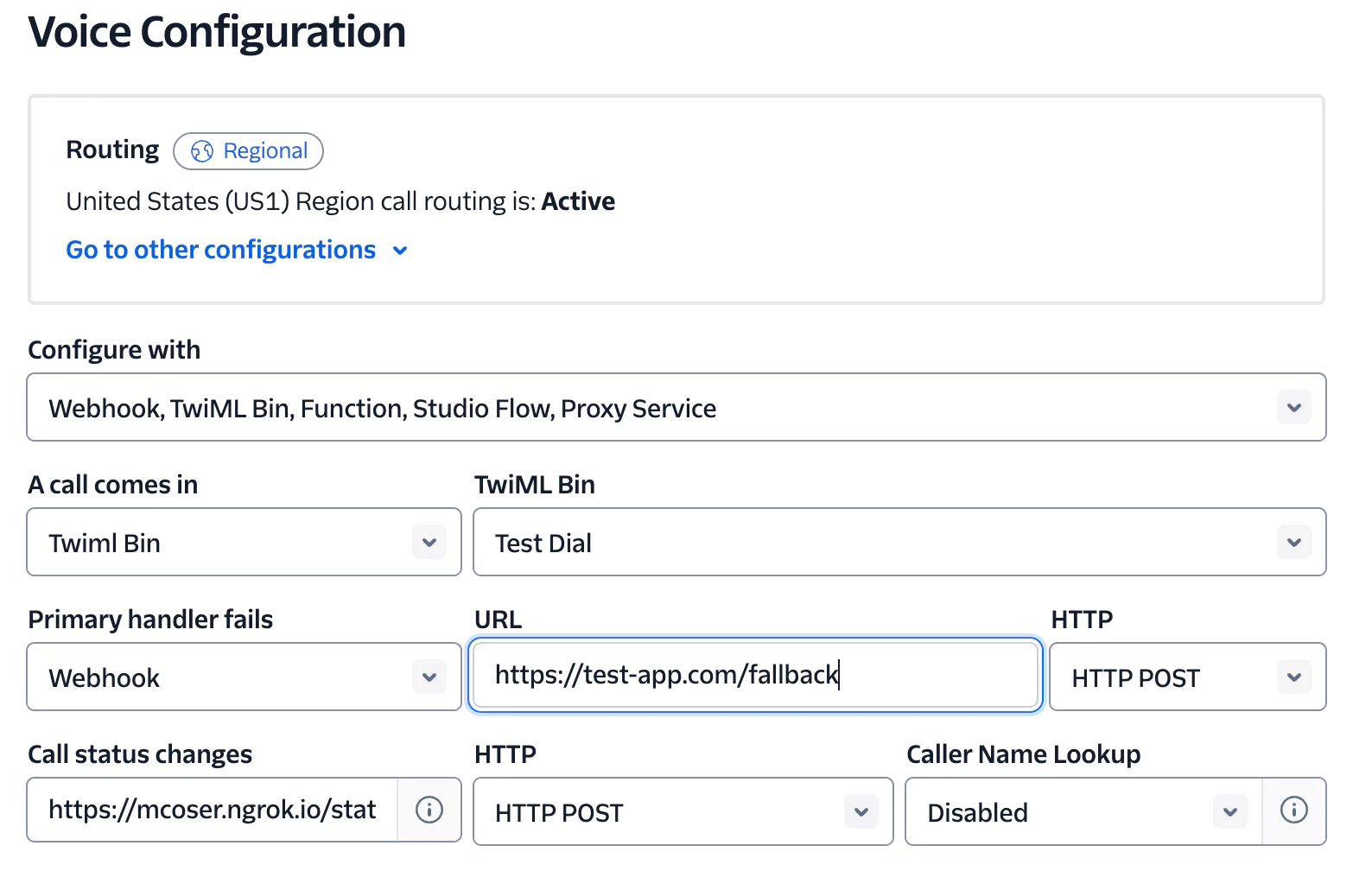
Fallback URLs can also be utilized when making an outbound call, in case the primary URL that provides the TwiML instructions fails.
If you don’t have backup or alternate hosting set up yet, set Alarms (or set logic in your Debugger Webhook) to keep track of HTTP related Errors which may be a sign that your app is not functioning as expected. This Alarm will send an Email and fire a Webhook after detecting over ten 11200 Errors per hour.
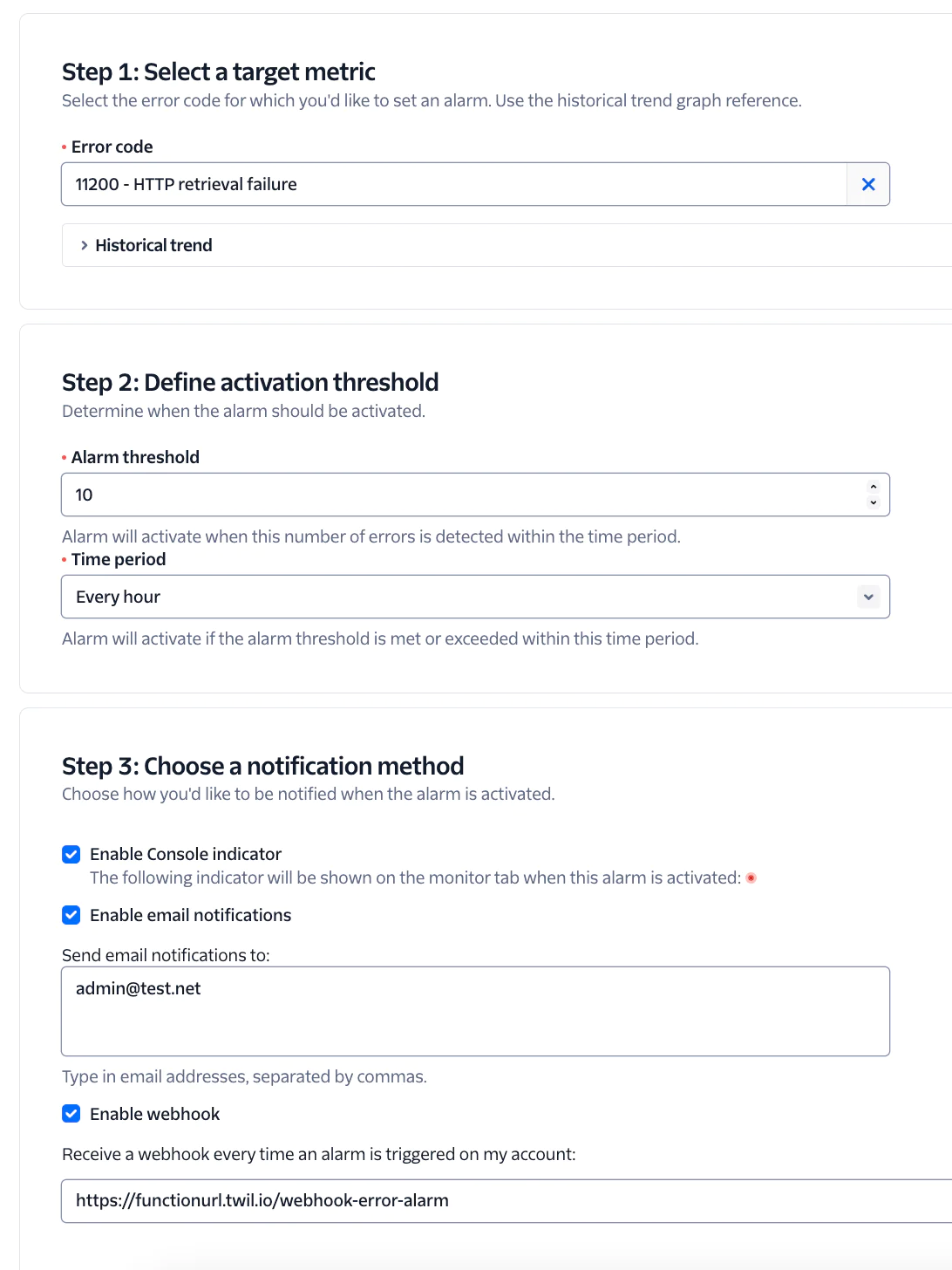
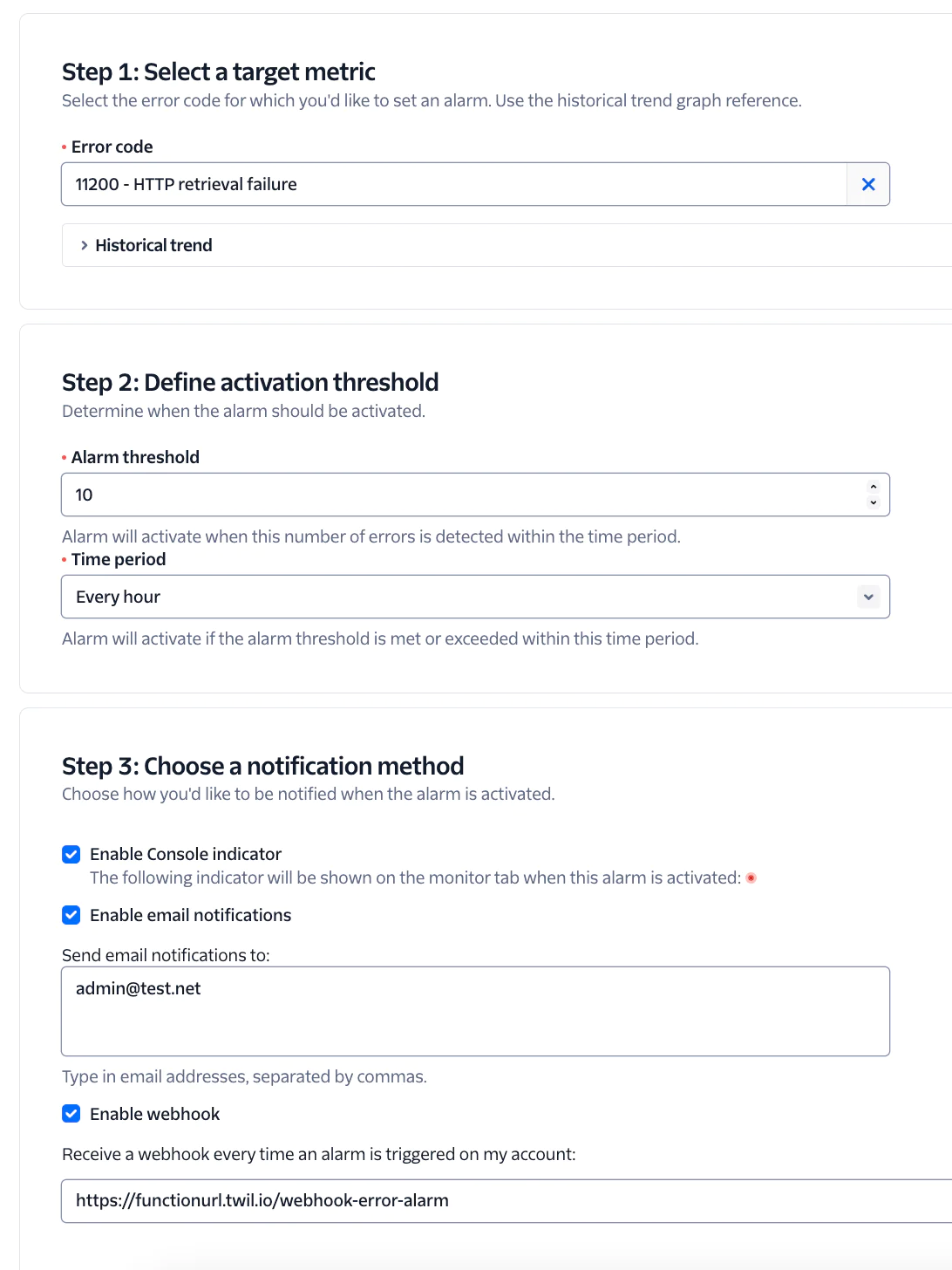
See our docs for more on availability and reliability for more on building with contingencies in mind.
Conclusion
Twilio's Usage and Monitor APIs for monitoring and alerting can help your business maintain robust oversight of your communications infrastructure. By tracking usage patterns and account activity, you can identify and address anomalies then react appropriately. Moreover, the ability to customize alerts and integrate with existing reporting and SIEMs enhances responsiveness and supports your informed decision-making.
Found my post interesting? Please take a moment to browse these additional resources:
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.