How to Implement a Robust Voice Authentication with Go and Twilio
Time to read: 4 minutes
Implementing A Robust Voice Authentication with Twilio and Go
Voice OTP (One-Time Password) authentication is an increasingly popular method for secure user verification — especially in situations where SMS-based OTPs might not be ideal.
This approach generates a temporary password and then delivers it via voice call, which helps provide an additional layer of security to the user account.
Leveraging Twilio’s powerful voice API alongside Go, this tutorial outlines a streamlined process to create a voice-based OTP system. With it, developers can enhance their applications with a robust, accessible, and secure verification method.
Requirements
To follow along with this tutorial, ensure you have the following:
- Go 1.23 or higher
- Access to a MySQL database
- A free or paid Twilio account with an active phone number
Create a new Go project
To get started, you need to create a new Go project. To do that, open your terminal and run the following commands where you create your Go projects:
After running the commands above, open the newly created project folder named go-registration-app in your preferred code editor.
Create the environment variables
Let’s create environment variables to store your Twilio credentials. To do this, create a .env file inside the project folder's top-level directory, and add the following code to the file.
Retrieve your Twilio credentials
Now, let’s retrieve your Twilio credentials. To do this, log in to your Twilio Console dashboard. In the Account Info section, you’ll find your Account SID, Auth Token, and Twilio phone number, as shown in the screenshot below.
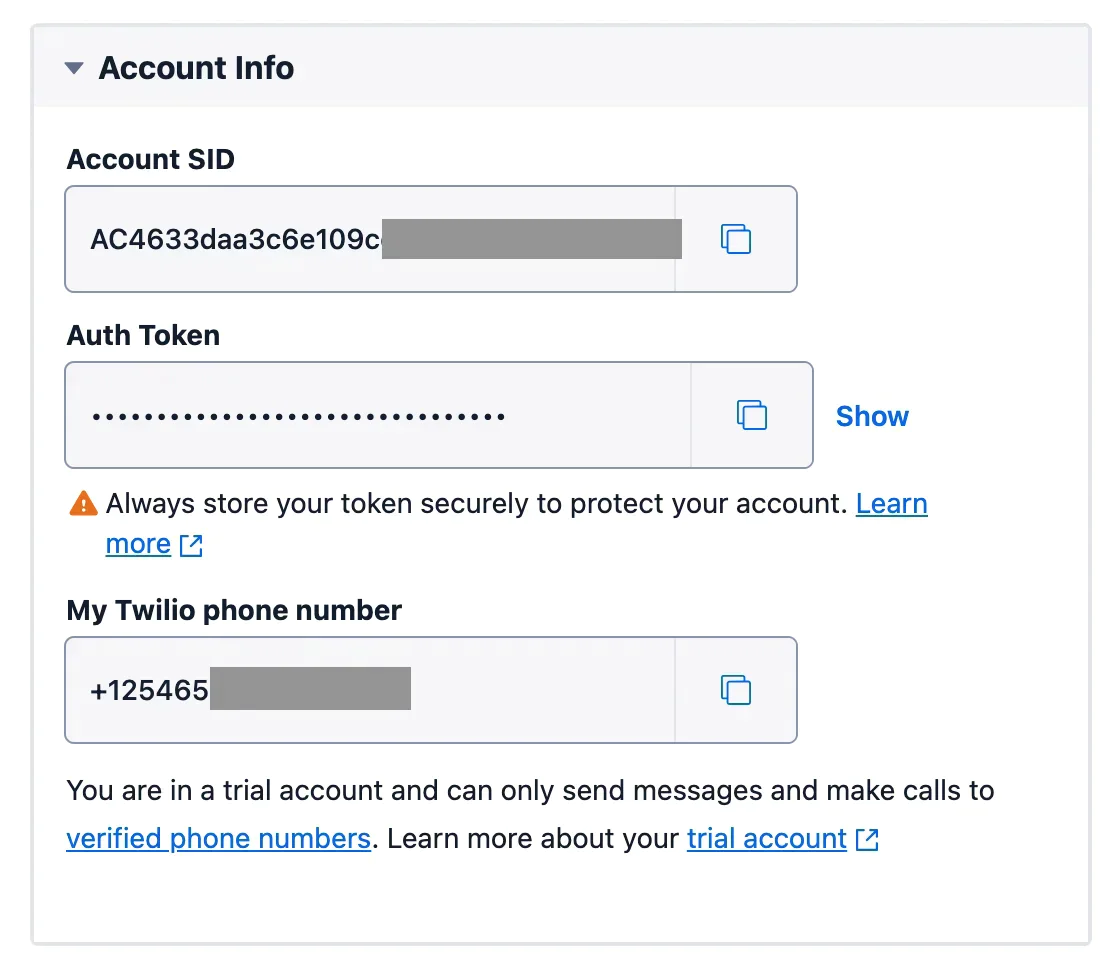
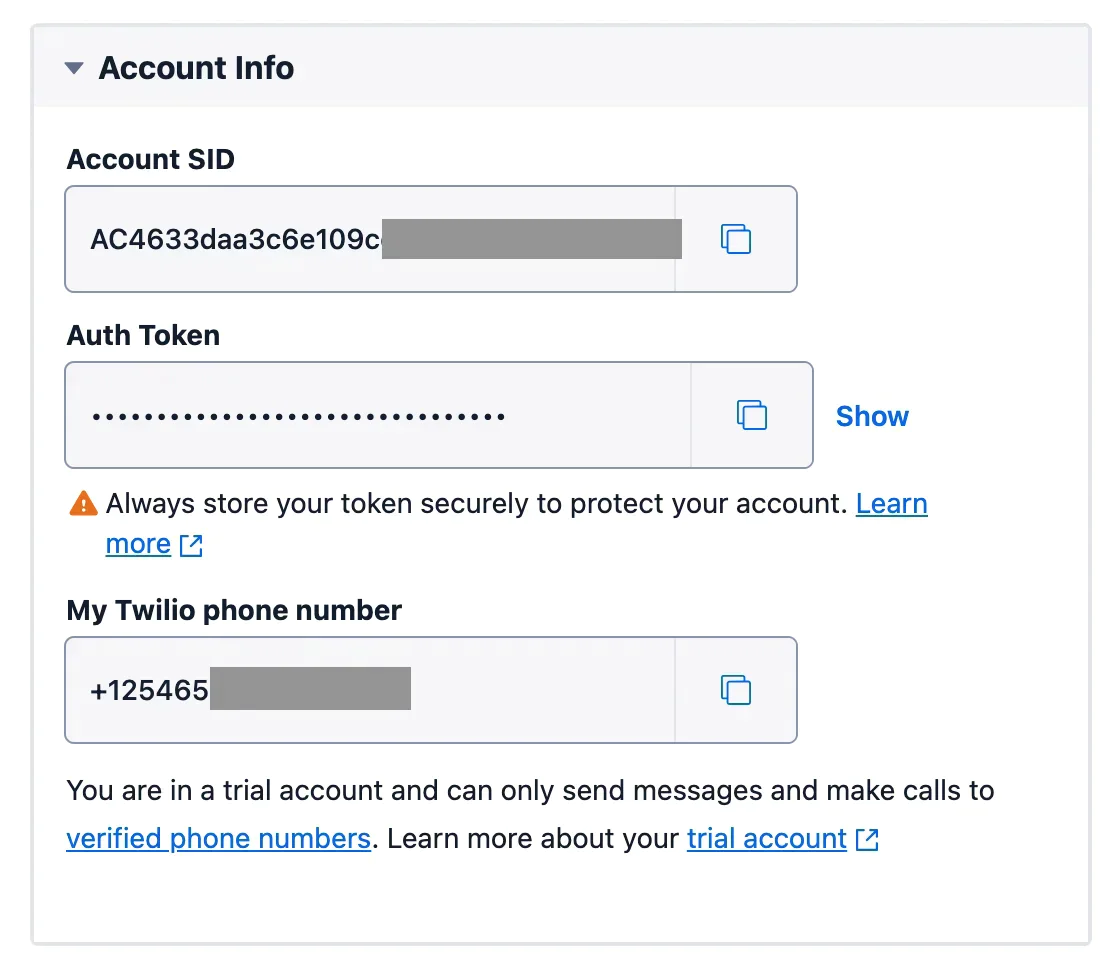
Copy them and replace the corresponding Twilio environment variables in .env, respectively, with them.
Install Twilio's Go Helper Library
To interact with Twilio Programmable Voice in your Go project, let’s install Twilio's Go Helper Library using the command below:
Create the database schema
Let’s create a database schema to store registered user information. To do this, log in to your MySQL database, create a new database named "userinfo", and then create a "users"table with the following schema.
After creating the table, your table structure should look like the screenshot below.
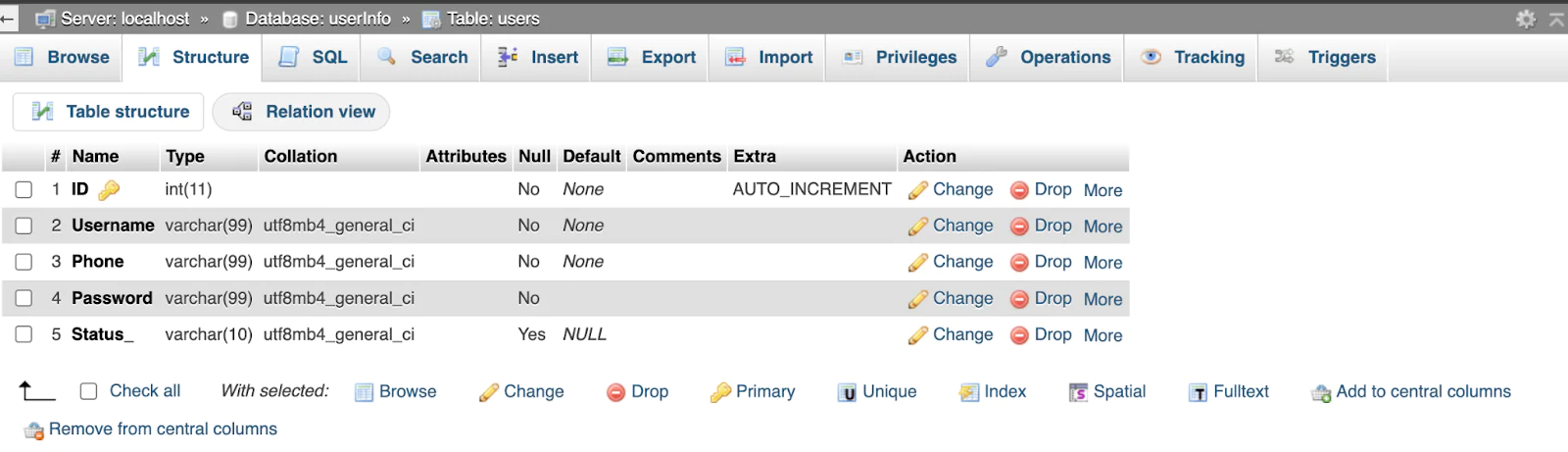
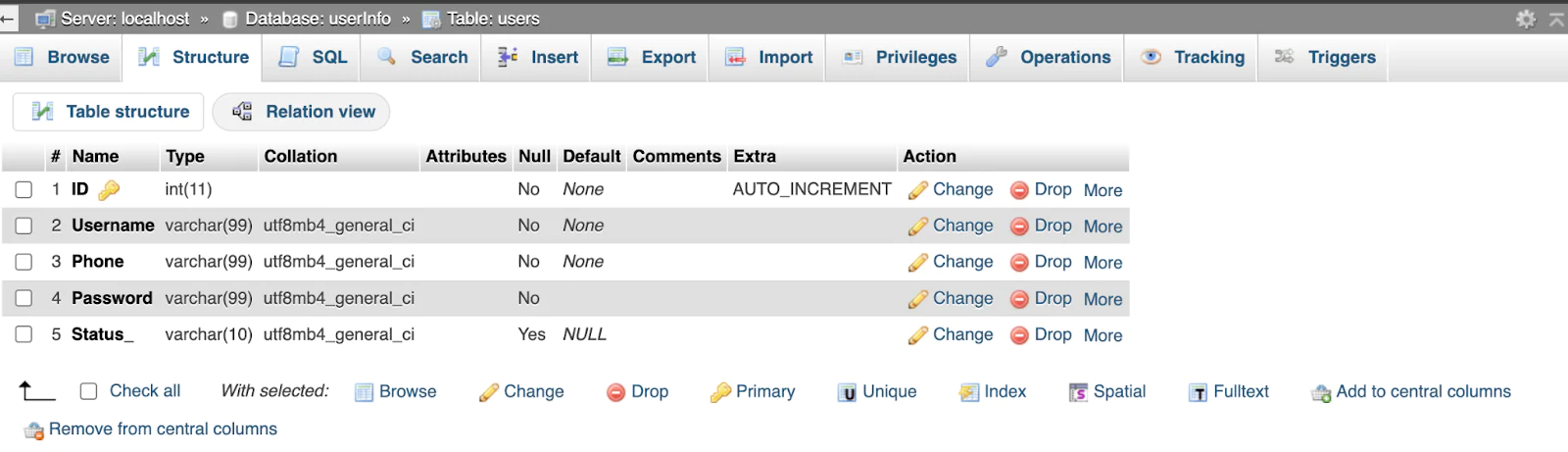
Install other packages
In our application, we will use the mysql package to interact with the database, gorilla/sessions to manage user sessions, and godotenv to load environment variables into the application. To install these Go packages, run the commands below.
Create the authentication logic
Now, let’s create the application’s registration, login, and verificationpages and implement OTP verificationusing Twilio Programmable Voice to confirm user accounts. To do this, create a file named main.go in the project's top-level directory and add the following code to the file.
From the code above:
- The
InitializeDB()
function is used to connect to the MySQL database where we store the user information. Make sure you replace the<bd_user>
and<db_password>
with the corresponding database value. - The
registerHandler()
function handles the user registration details and saves them into the database using theSaveUserToDB()
function - The
verifyOTPHandler()
function processes the user OTP code and changes the status of the user to "verified" if the OTP code is valid - The
profileHandler()
function loads the user profile details after the user successfully verifies their account - The
main()
function defines the application routes and connects the necessary functions together
Create the HTML form template
Next, we'll create the HTML templates for the application's pages. In the project's root folder, create a templates directory. Inside this folder, create a file named register.html and add the following code to it.
Once the user successfully registers, they are redirected to the account verification page to verify their phone number by entering the OTP sent to it. To create the OTP HTML template, in the templates folder, create a file named otp.html, and add the following code to the file.
To create the profile HTML template, in the templates folder create a file named profile.html, and add the following code to the file.
Add style to the application
Lastly, let’s style the application pages. To do this, create a static folder in the top-level directory of the project. Inside the folder, create a file named styles.css and add the following code to it.
Start the application
Let’s start the application . To do that, run the command below in your terminal.
Test the application
Let’s now test the application to confirm it’s working as expected. Open your preferred browser and navigate to http://localhost:8080/register. The user registration page should appear, as shown in the screenshot below.
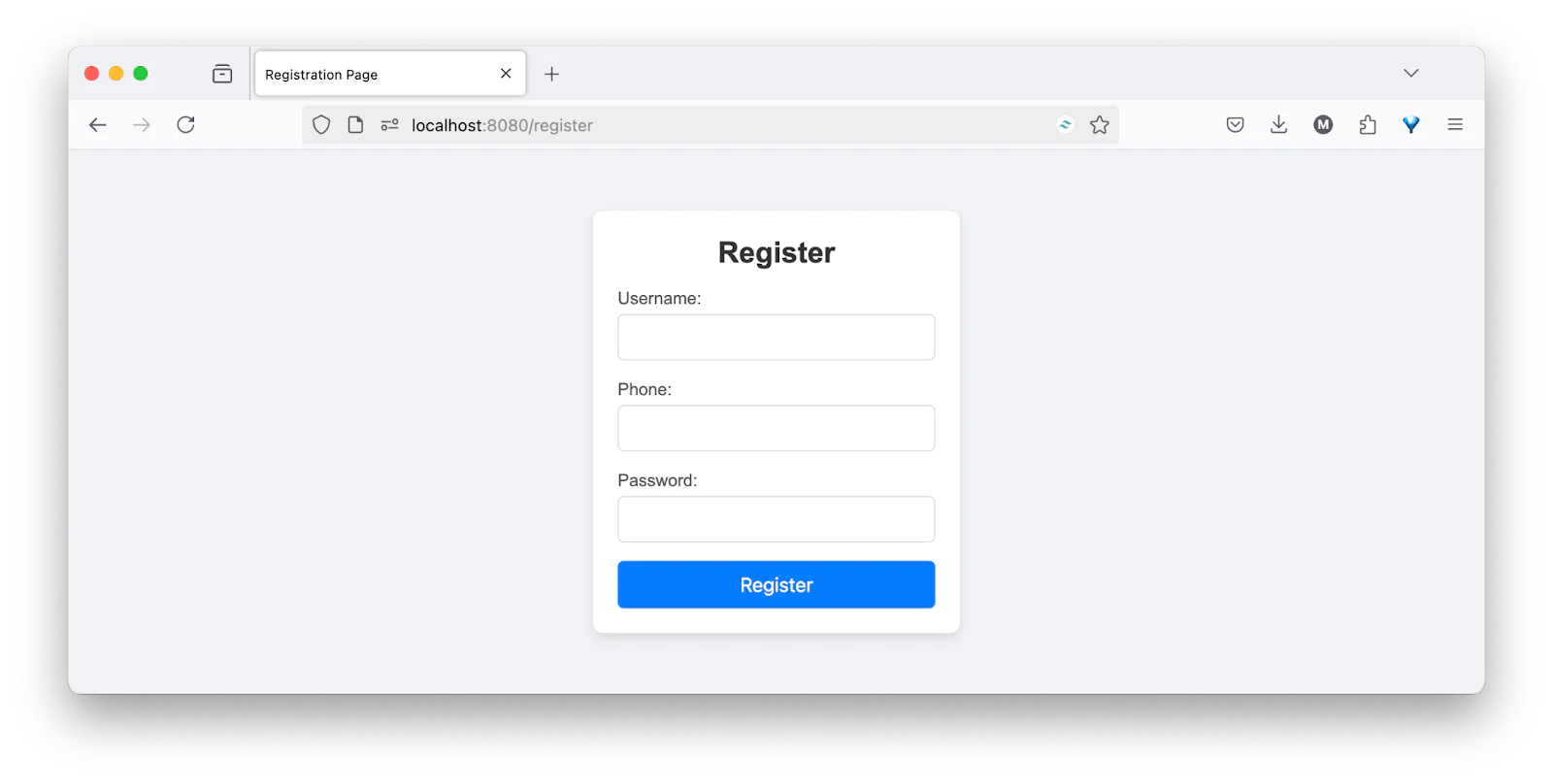
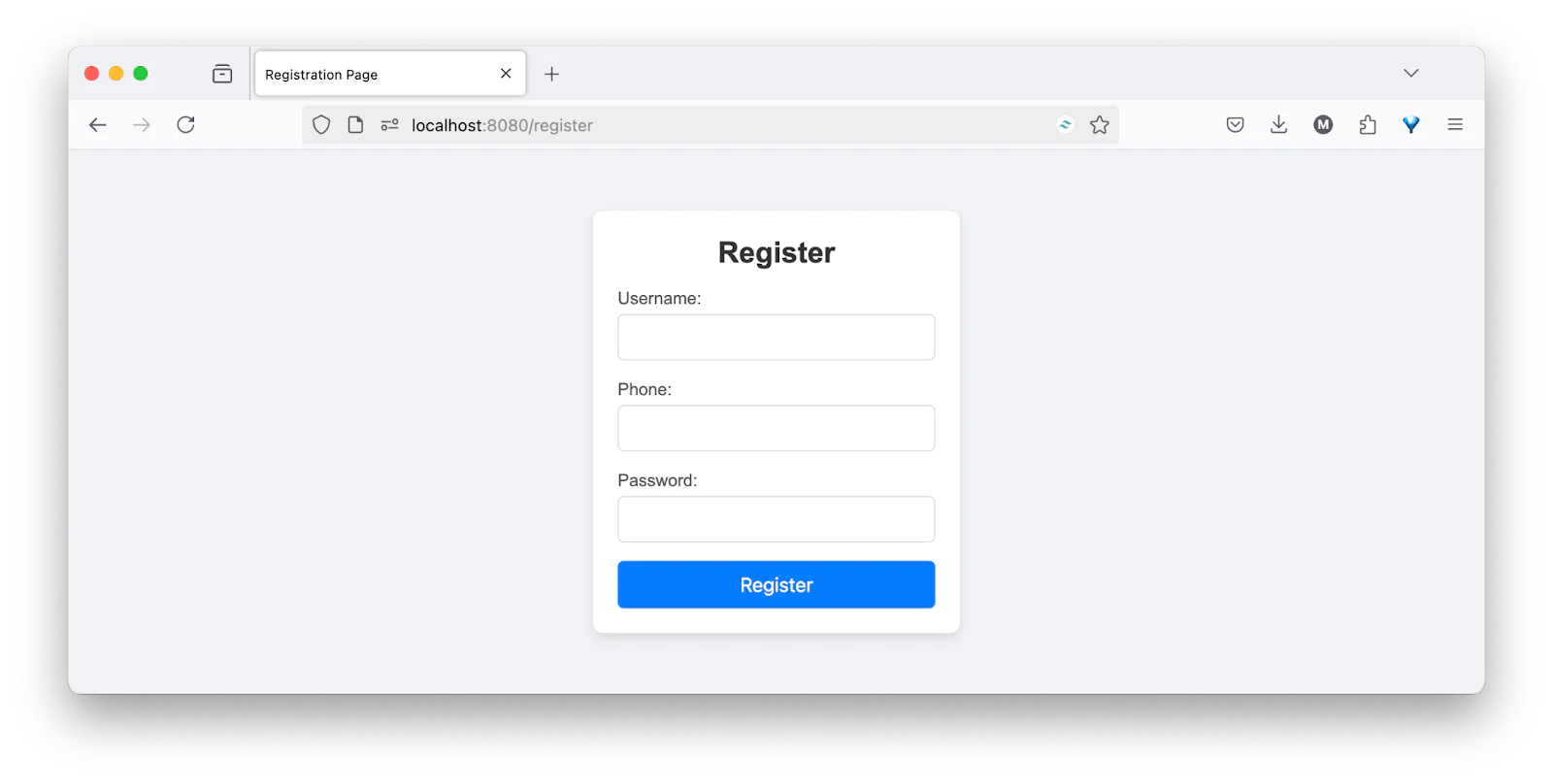
After completing the registration, the user will be redirected to the account verification page to enter the OTP code received via phone call, as shown in the image below.
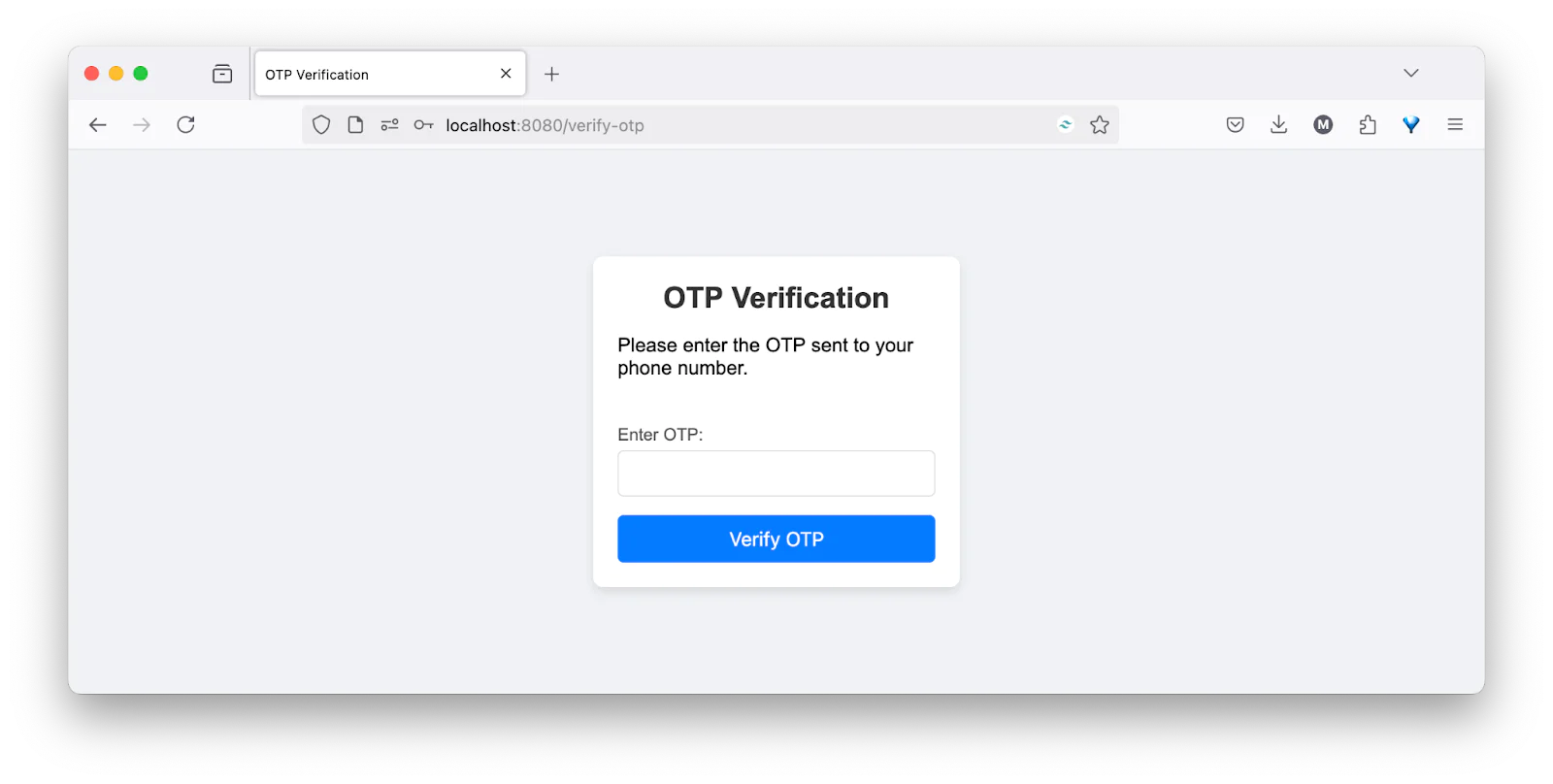
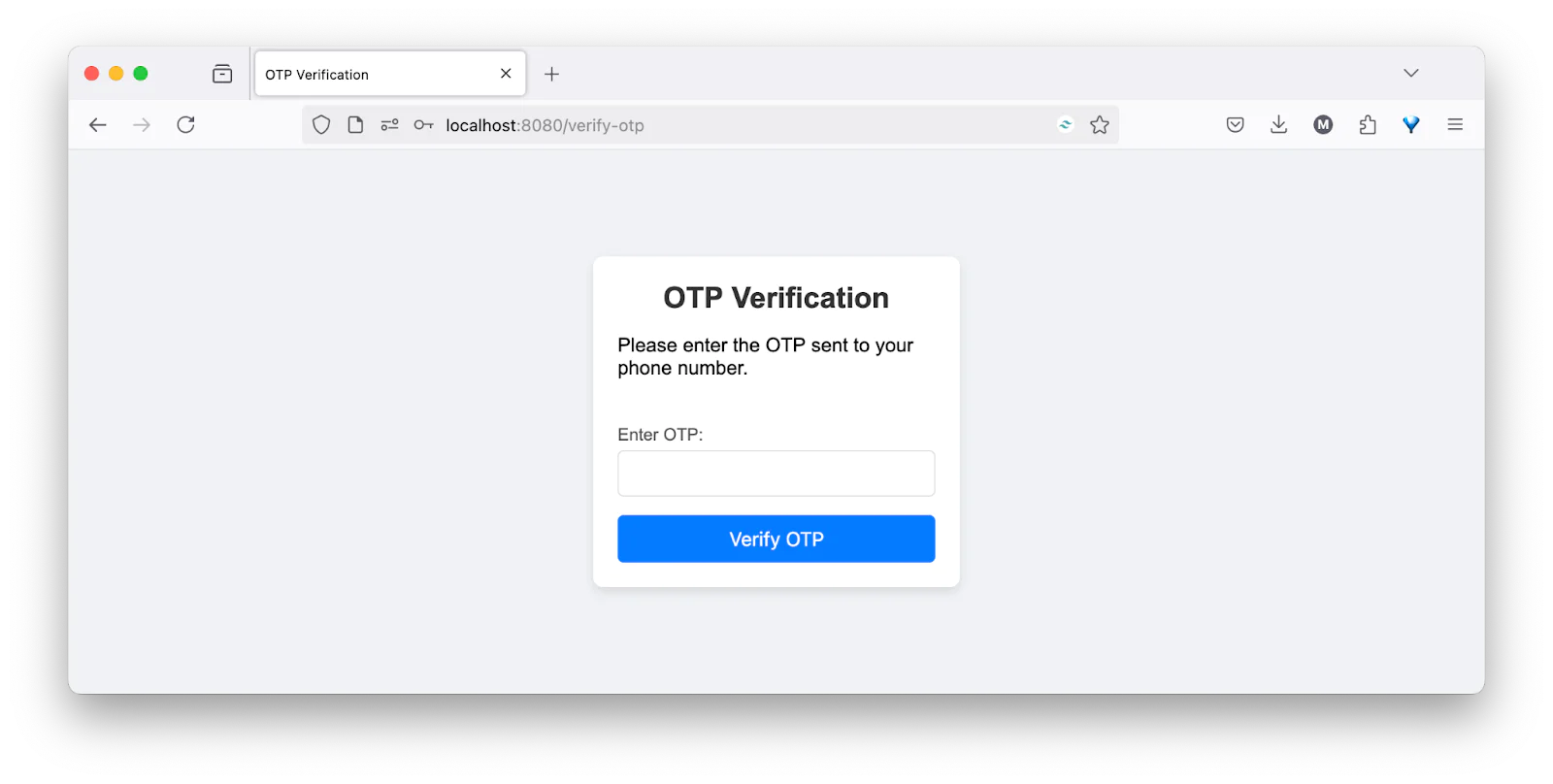
Once the OTP is entered correctly, the user's account status will be updated to "verified",' and they will be redirected to their profile page, as shown in the image below.
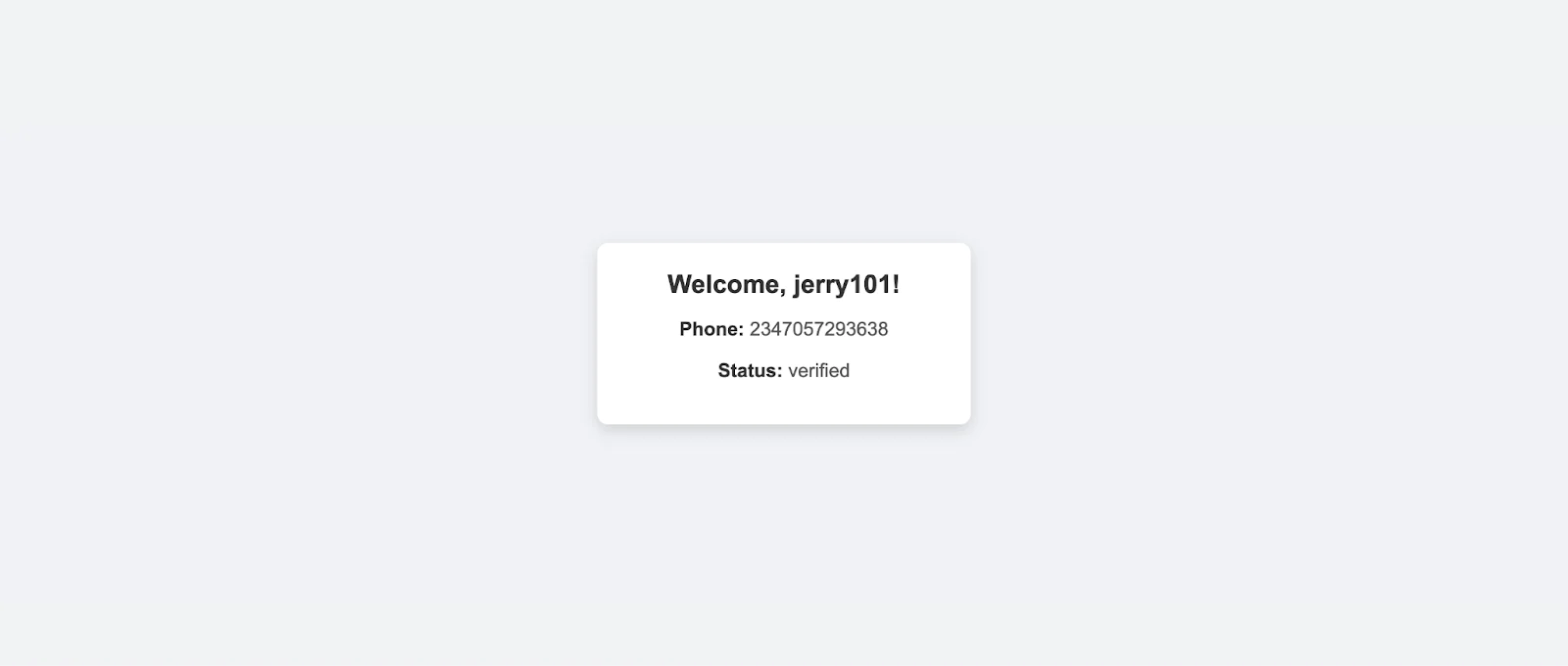
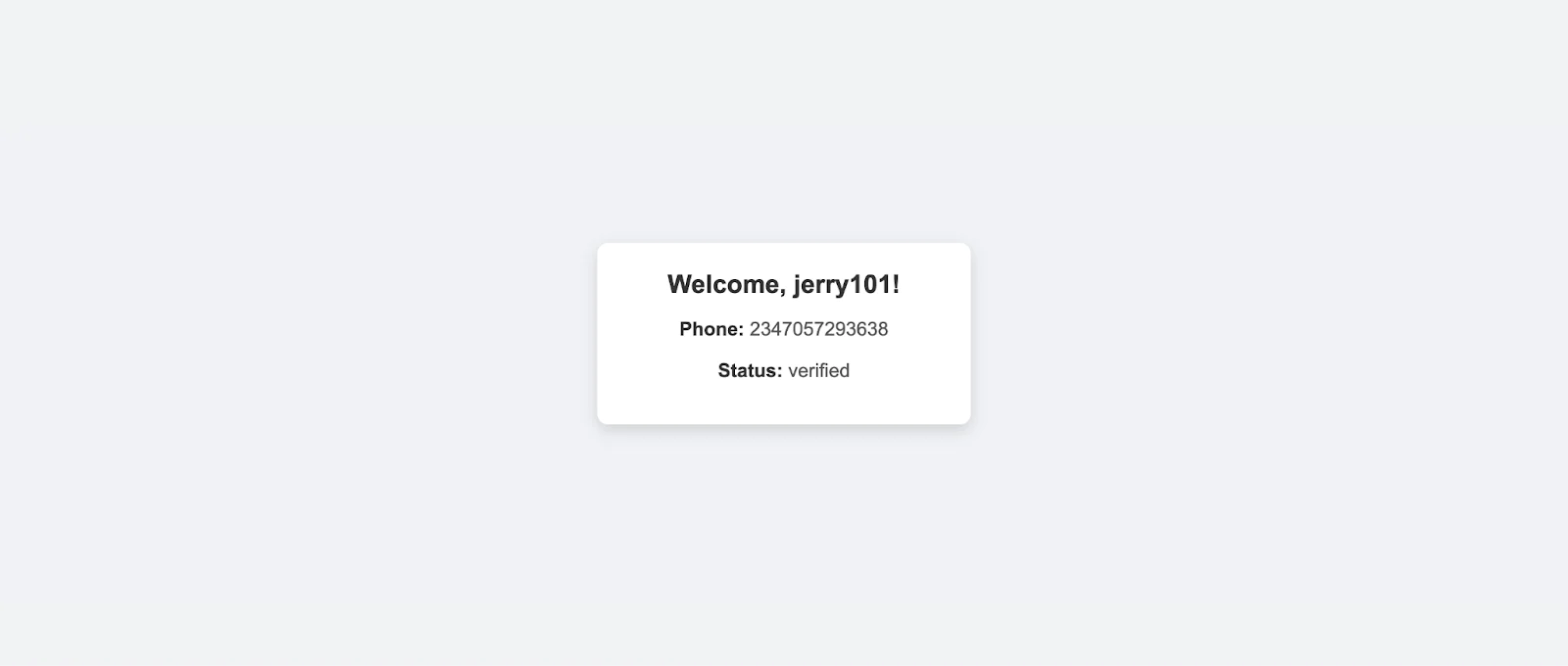
That's how to implement a robust voice authentication with Twilio and Go
Building a voice authentication system using Twilio and Go provides a secure and efficient way to verify users. By combining Twilio's Voice API with Go's speed and simplicity, you can develop a solution that is both reliable and scalable for real-world applications.
This approach enhances security without compromising user experience, whether it's securing user accounts or enabling voice-based multi-factor authentication. By following this guide, you’ve taken the first step toward implementing voice authentication in your applications.
Oluseye Jeremiah is a dedicated technical writer with a proven track record in creating engaging and educational content.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.