How to Implement a Magic Link in CakePHP Using Twilio
Time to read: 5 minutes
How to Implement a Magic Link in CakePHP Using Twilio
A magic link is an authentication method that allows users to log in to an application or website — without using a password. Instead, the user provides their phone number or email address, and the system sends them a unique, time-sensitive link to that phone number or email address. When the user clicks on the link, they are then automatically logged in.
In this tutorial, you will learn how to implement and send a magic login link to users via SMS in a CakePHP application.
Requirements
To complete this tutorial, you will need the following:
- PHP 8. 3 or higher
- Composer installed globally
- Access to a MySQL database
- A free or paid Twilio account with an active phone number
- Ngrok
Set up a new CakePHP project
Let's get started by creating a new CakePHP project. To do this, open your terminal and navigate to the directory where you create your PHP projects. Then, run the commands below to create the project and change into the new project directory.
Once the project is created, you will be prompted with "Set Folder Permissions? (Default to Y) [Y, n]?". Answer with "Y".
Retrieve your Twilio access credentials
Now, let’s retrieve our Twilio access credentials by logging into your Twilio Console dashboard. You will find them (the Account SID and Auth Token) under the Account Info section, as shown in the screenshot below.
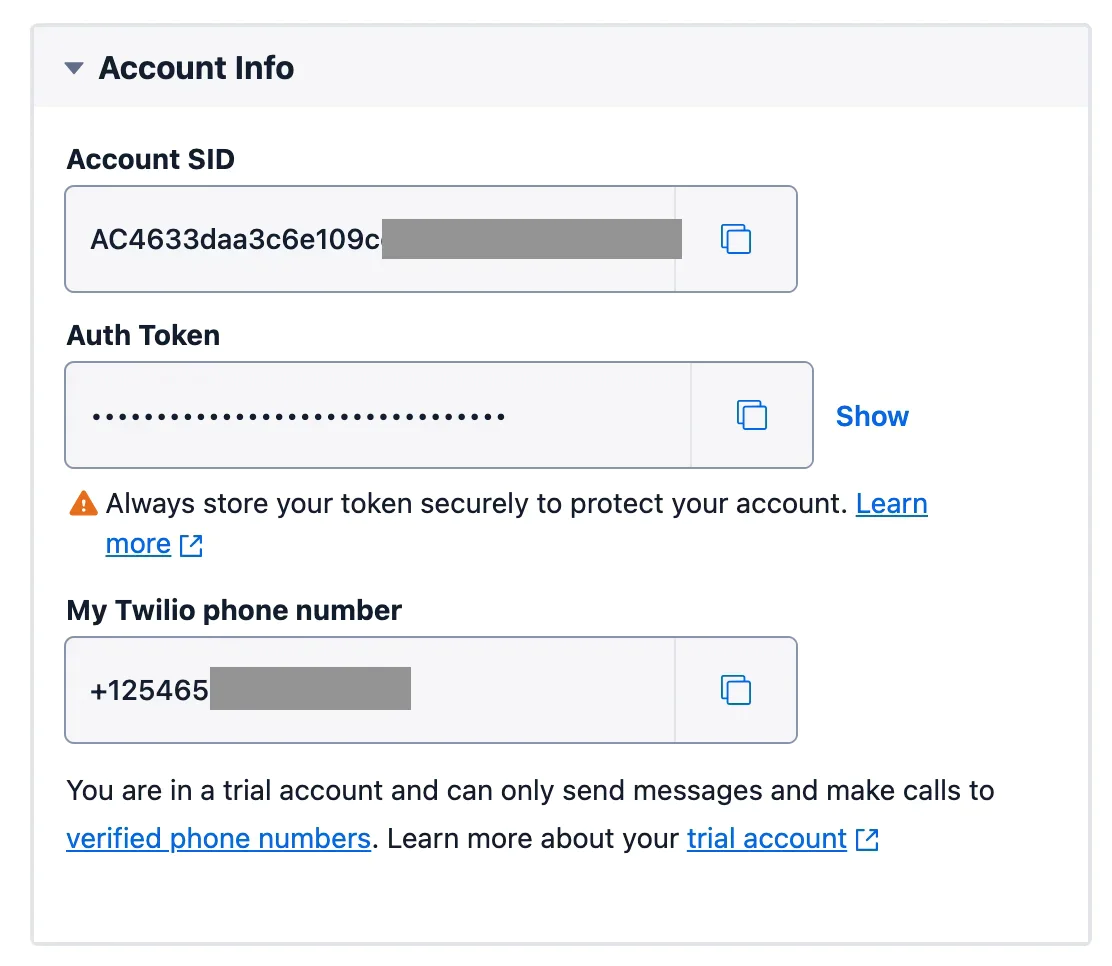
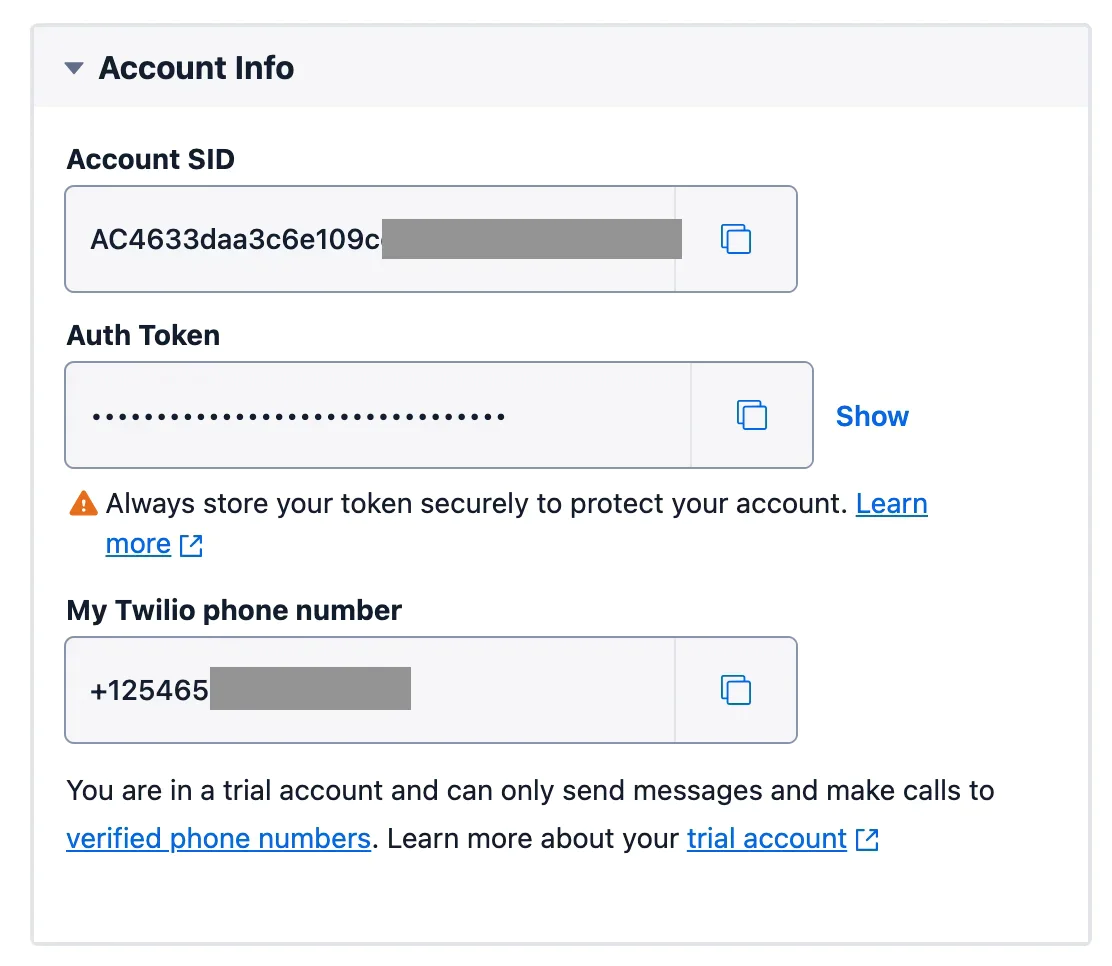
Set the credentials as environment variables
Let’s now add the Twilio credentials as environment variables. To do this, you will first need to create a .env file using the command below.
Next, open the project directory in your preferred code editor or IDE and navigate to the config folder. Inside the folder, open the .env file and add the following three variables, replacing the placeholders with their corresponding values.
Now, you need to enable the josegonzalez\Dotenv library to load the environment variable into the application. To do this, open the bootstrap.php file inside the config folder and uncomment the code below.
Install the Twilio PHP Helper Library
To simplify how the CakePHP application interacts with Twilio's Programmable Messaging API (allowing it to send a magic login link to users via SMS), you need to install Twilio's PHP Helper Library. To install the library, run the command below.
Connect to the database
Let’s connect the application to the database. To do this, navigate to the config folder and open the app_local.php file. Inside the file, locate the default
subsection of the Datasource
section. In this section, replace the values for the host
, username
, password
, and database
settings with your corresponding MySQL database values, as shown in the screenshot below.
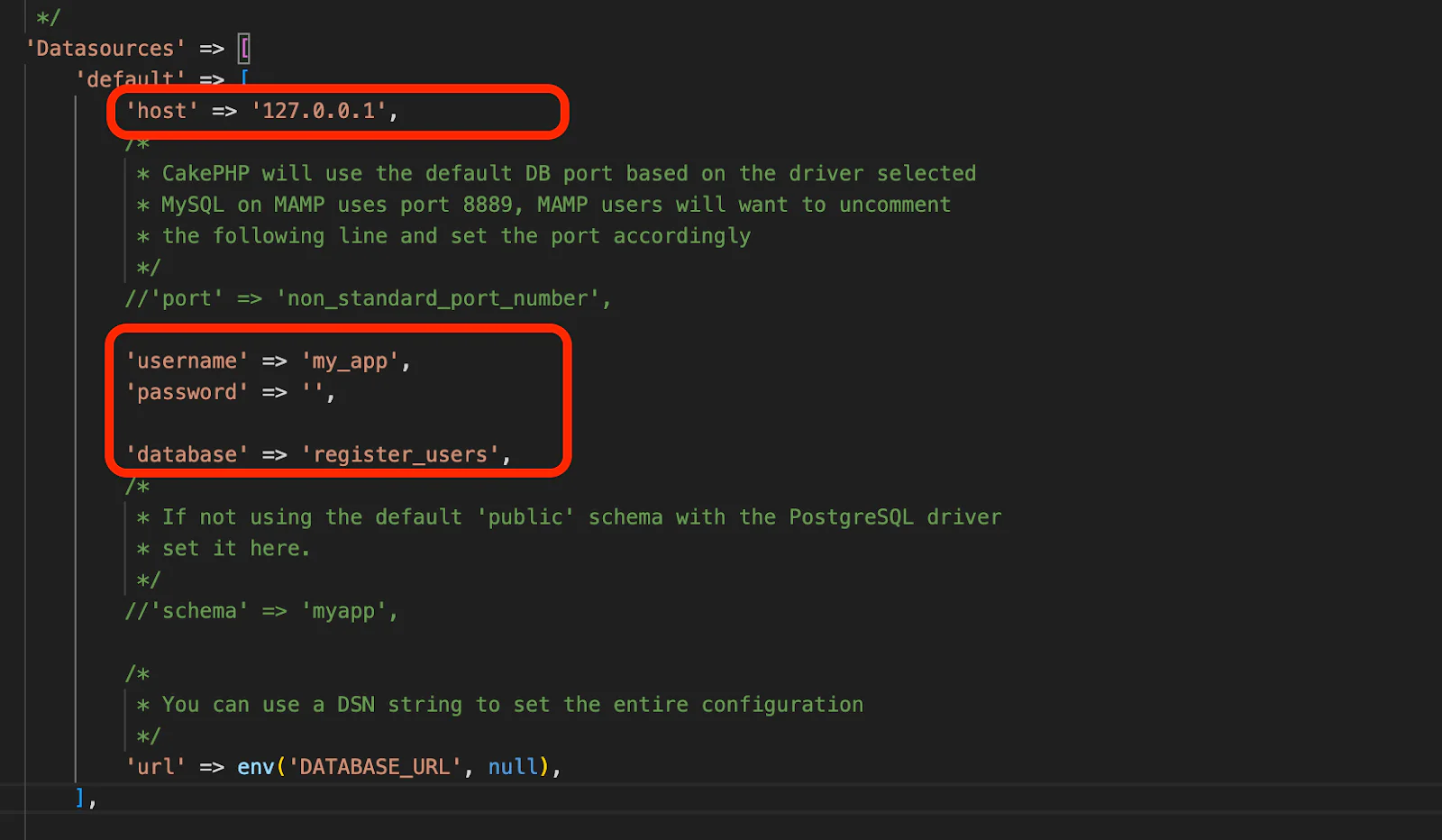
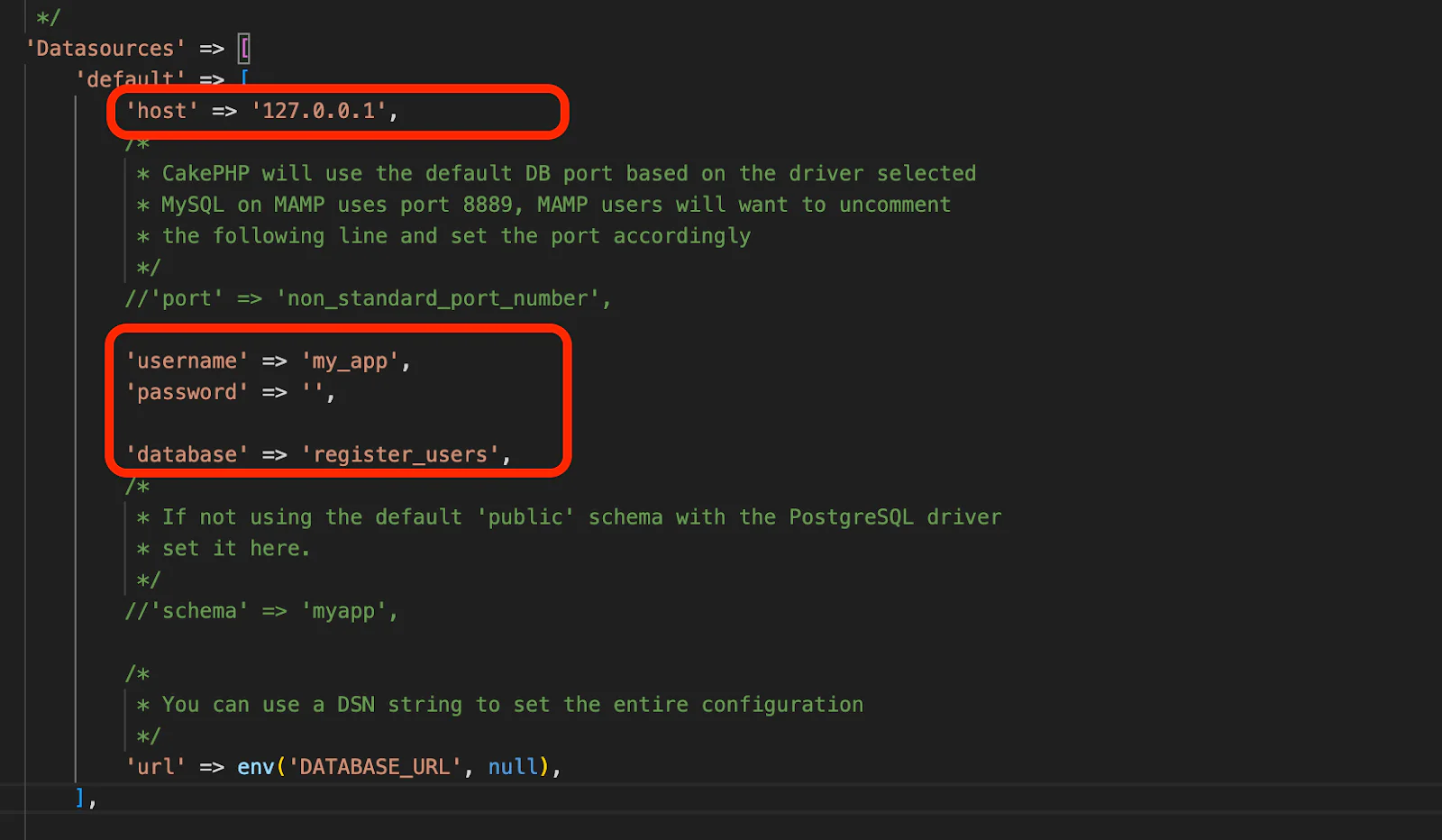
Now, start the MySQL database server and create a new database named register_users
.
Create the database schema
CakePHP allows developers to easily create database tables using migrations, which generate migration files which define the database schema. To generate a migration file to define the users
table, run the command below.
Now, let’s define the table properties. To do that, navigate to the config/Migrations folder and open the generated migration file that ends with _Users.php. Then, update the change()
function with the code below.
To complete the database migration and generate a model file for the application, run the commands below.
Next, start the CakePHP application by running the following command.
After starting the development server, open http://localhost:8765 in your browser. You should see the application's default page, as shown in the screenshot below.
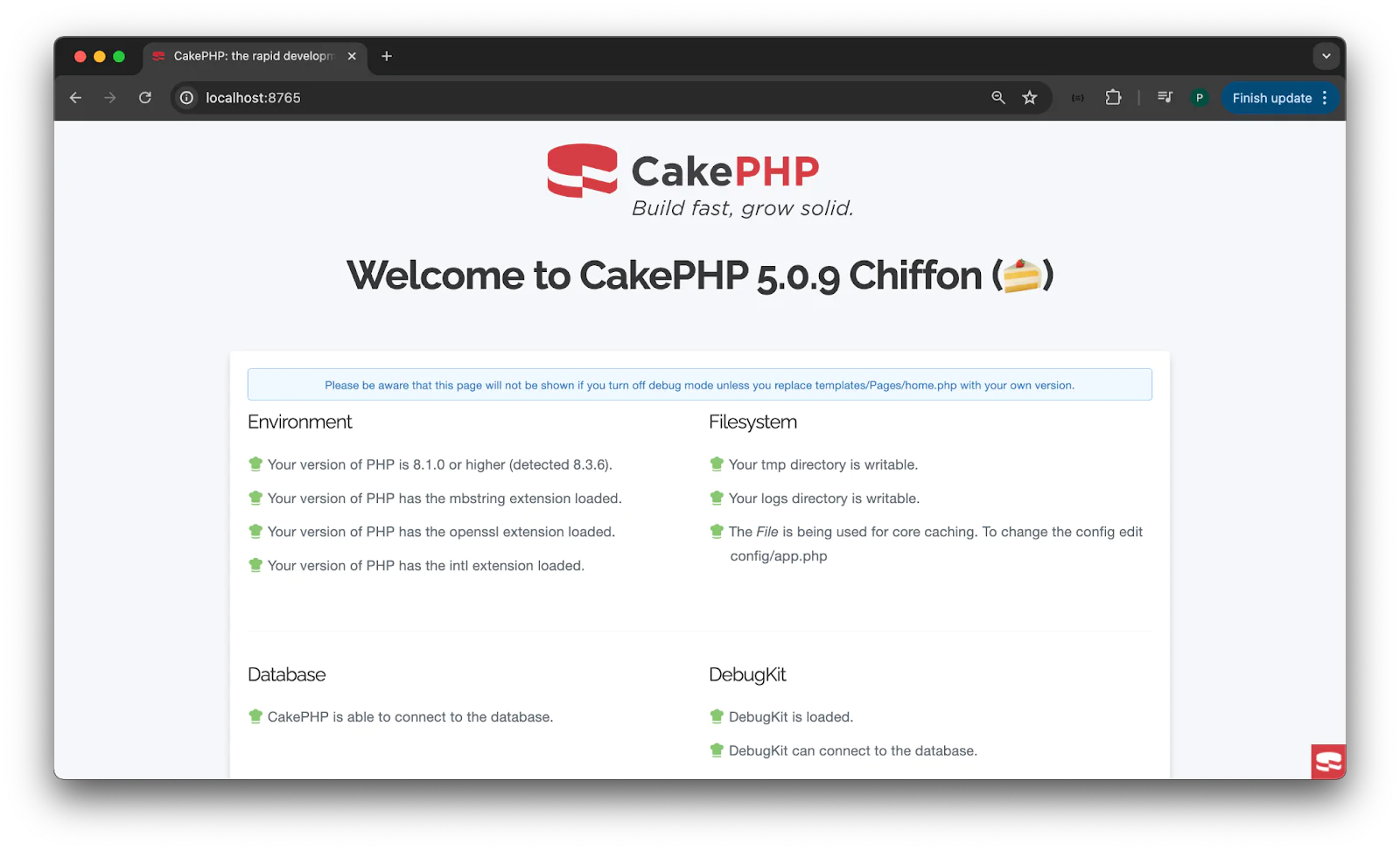
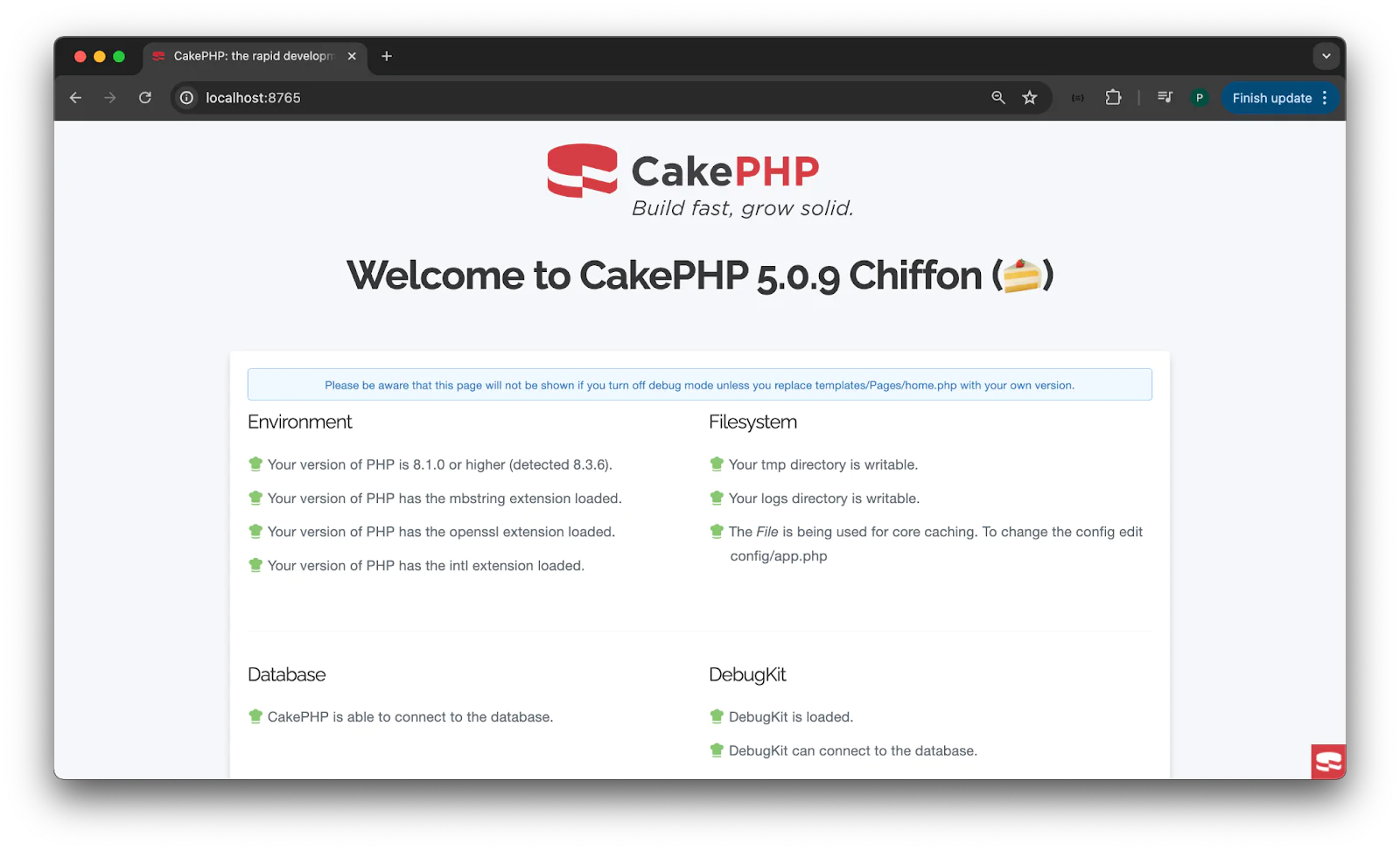
If you see the Security.salt
error notice, you can clear it by adding the environment variable below to the .env file.
Replace the <new-random-string-generated>
placeholder with a random value of at least 32 characters.
Create the application controller
Now, let’s generate a controller file for the application, named authentication
, run the command below.
Next, let’s add the application logic to the authentication controller. To do that, navigate to the src/Controller folder and open the AuthenticationController.php file. Then, update the file with the following code.
Here is the break down of the controller code above:
- The
register()
method handles the registration form, stores the user's data in the database, and then redirects the user to the login page if the registration is successful. - The
login()
method handles user login authentication by sending a magic login link to the user via SMS. - The
magic()
method validates the magic login link by checking whether the link is valid or expired. If the link is valid, the user is automatically logged in and directed to the profile page. - The
profile()
method displays the user's profile page if the user is logged in. If the user is not logged in, they are redirected to the login page.
Create the application's templates
Let’s now implement the application's user interface by creating the template files for the authentication controller’s methods. To create the controller's template files, navigate to the templates folder and create a new folder named Authentication. Inside the Authentication folder, create the following files:
- register.php
- login.php
- profile.php
Now, inside the register.php file, add the code below.
Inside the login.php file, add the code below.
Lastly, inside the profile.php, add the code below.
Update the application's routing table
To set up the application's routes, navigate to the config folder and open the routes.php file. Inside the file, locate $routes->scope()
and add the following code there, before $builder->fallbacks()
.
Make the application accessible on the internet
Finally, let’s make the application accessible over the internet, allowing us to use the magic login link on any device. Using Ngrok, open a new terminal tab (or session) and run the command below.
The command generates a Forwarding URL in the terminal, as shown in the screenshot below.
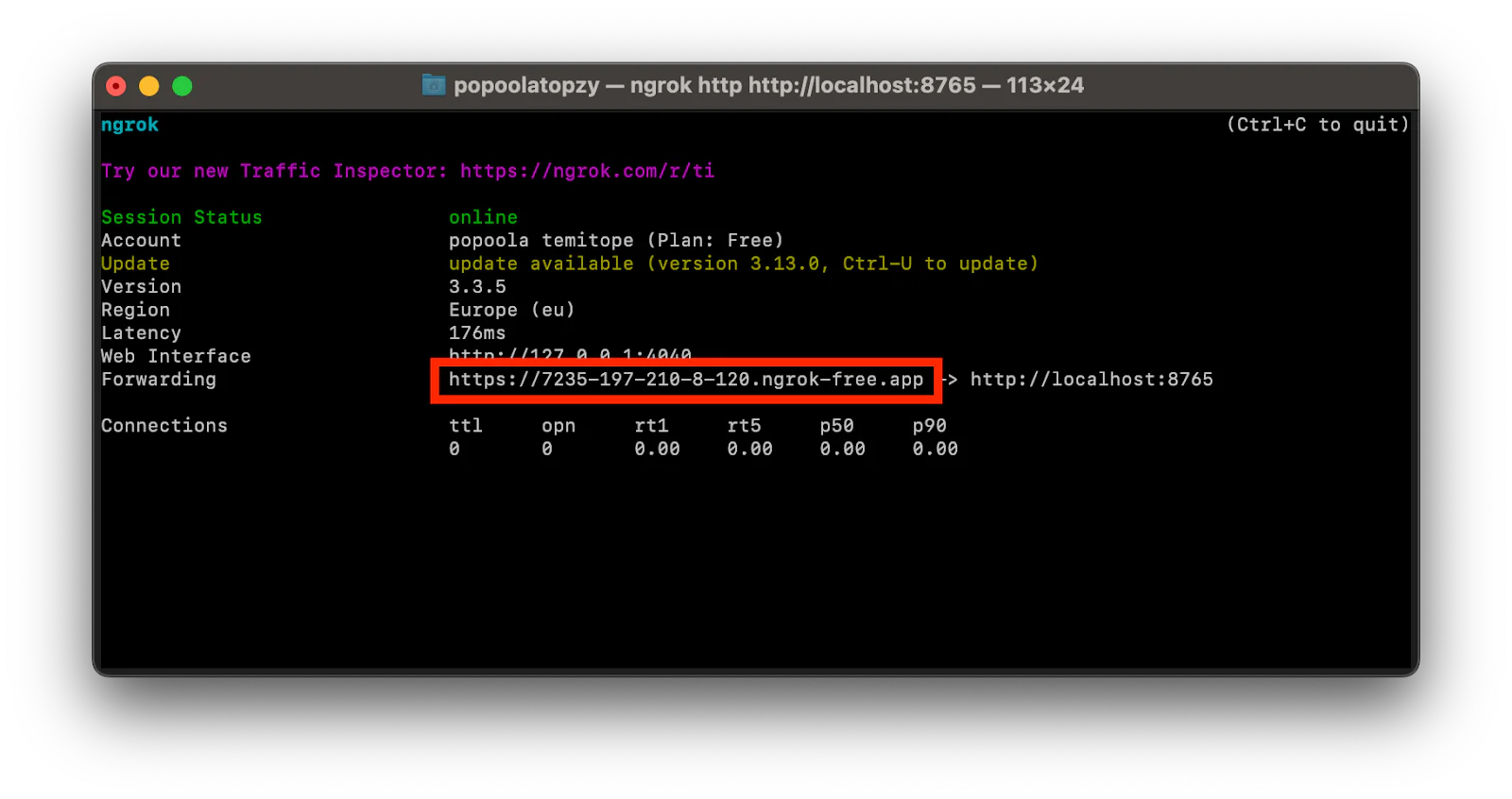
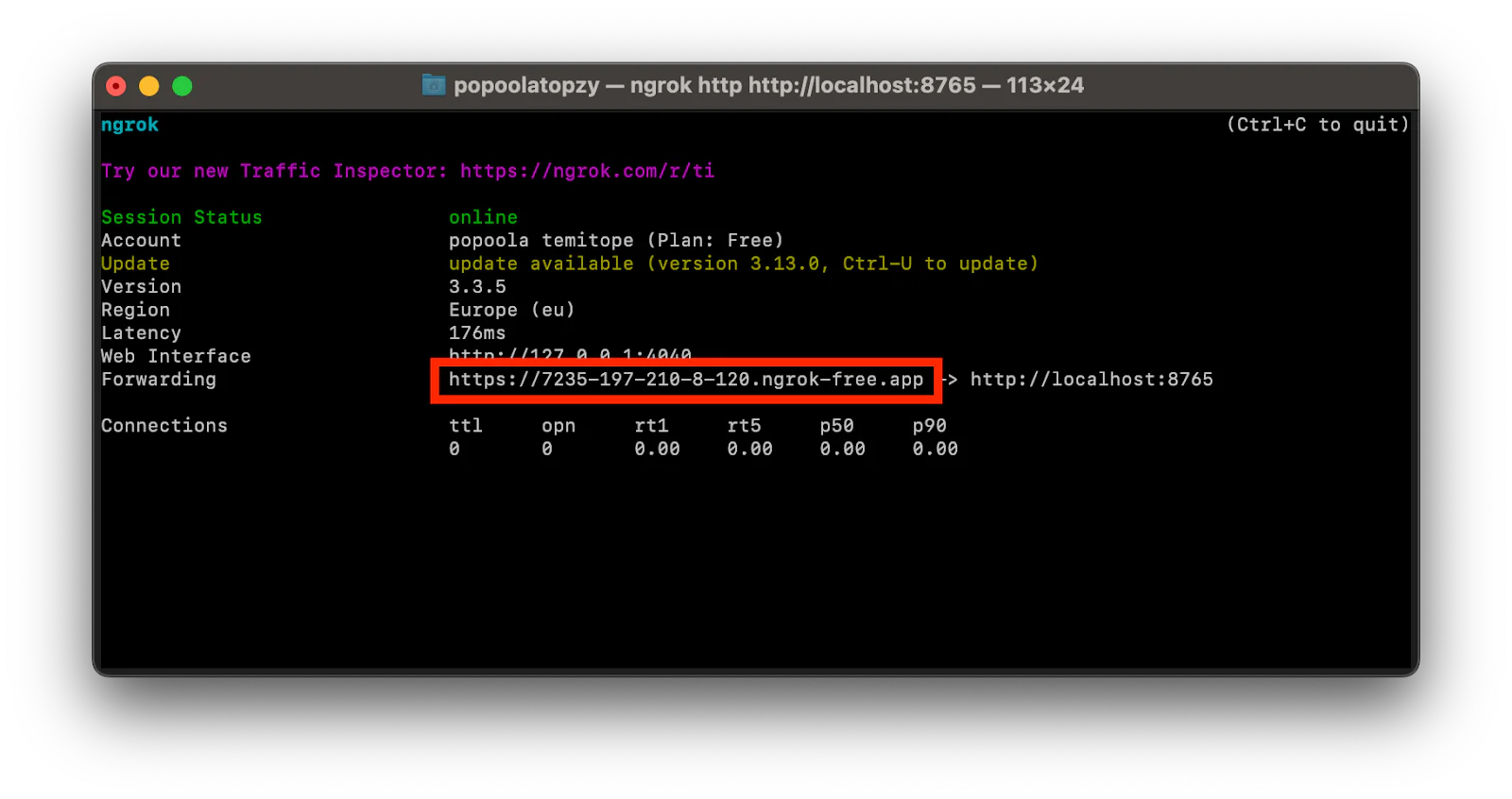
Next, add the Forwarding URL to the environment variables. To do that, navigate to the config folder and open the .env file. Inside the file, add the environment variables below, replacing <forwarding_url>
placeholder with the Forwarding URL generated by ngrok.
Test the application
To test the application, open http://localhost:8765/register in your browser and register a new account, as shown in the screenshot below.
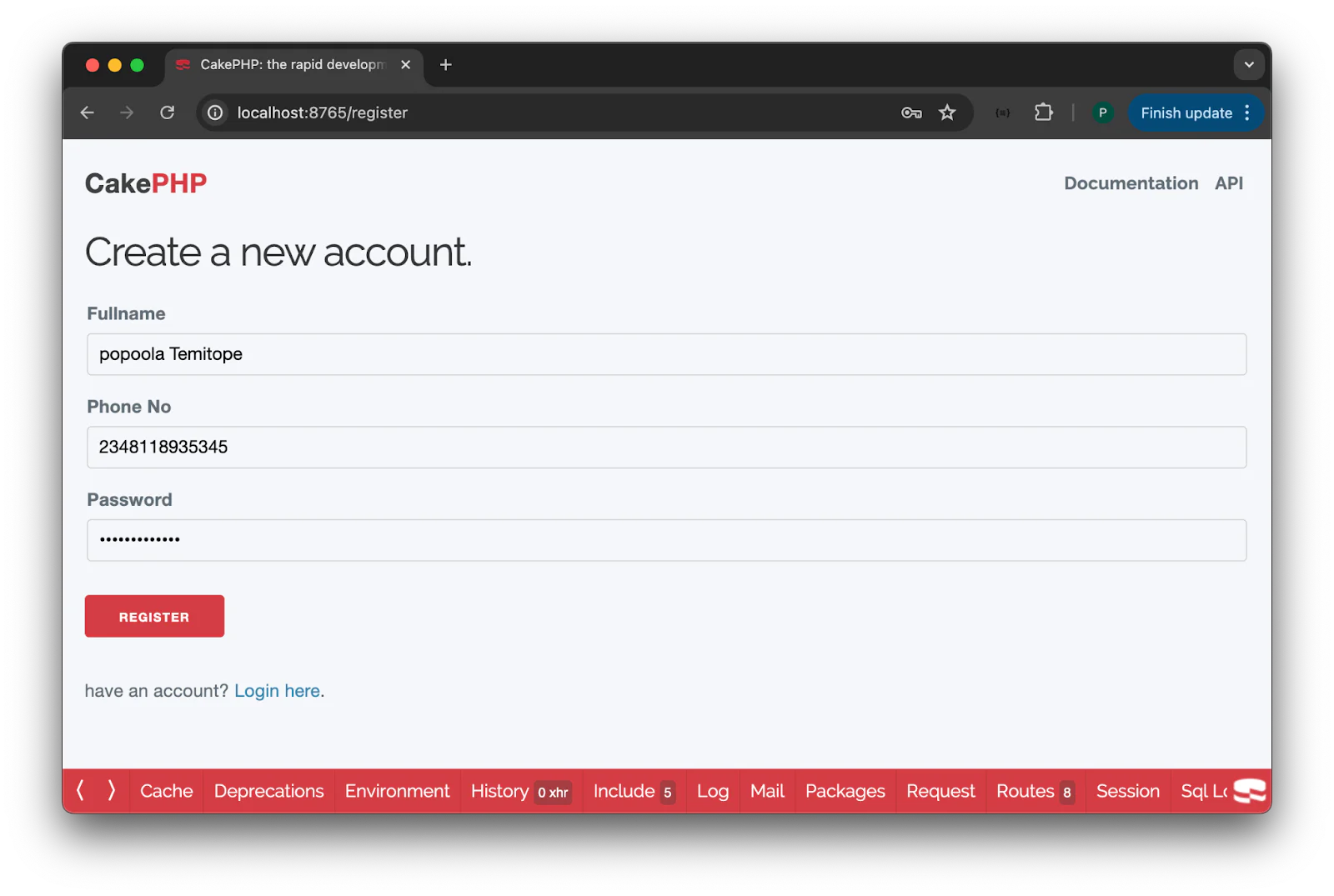
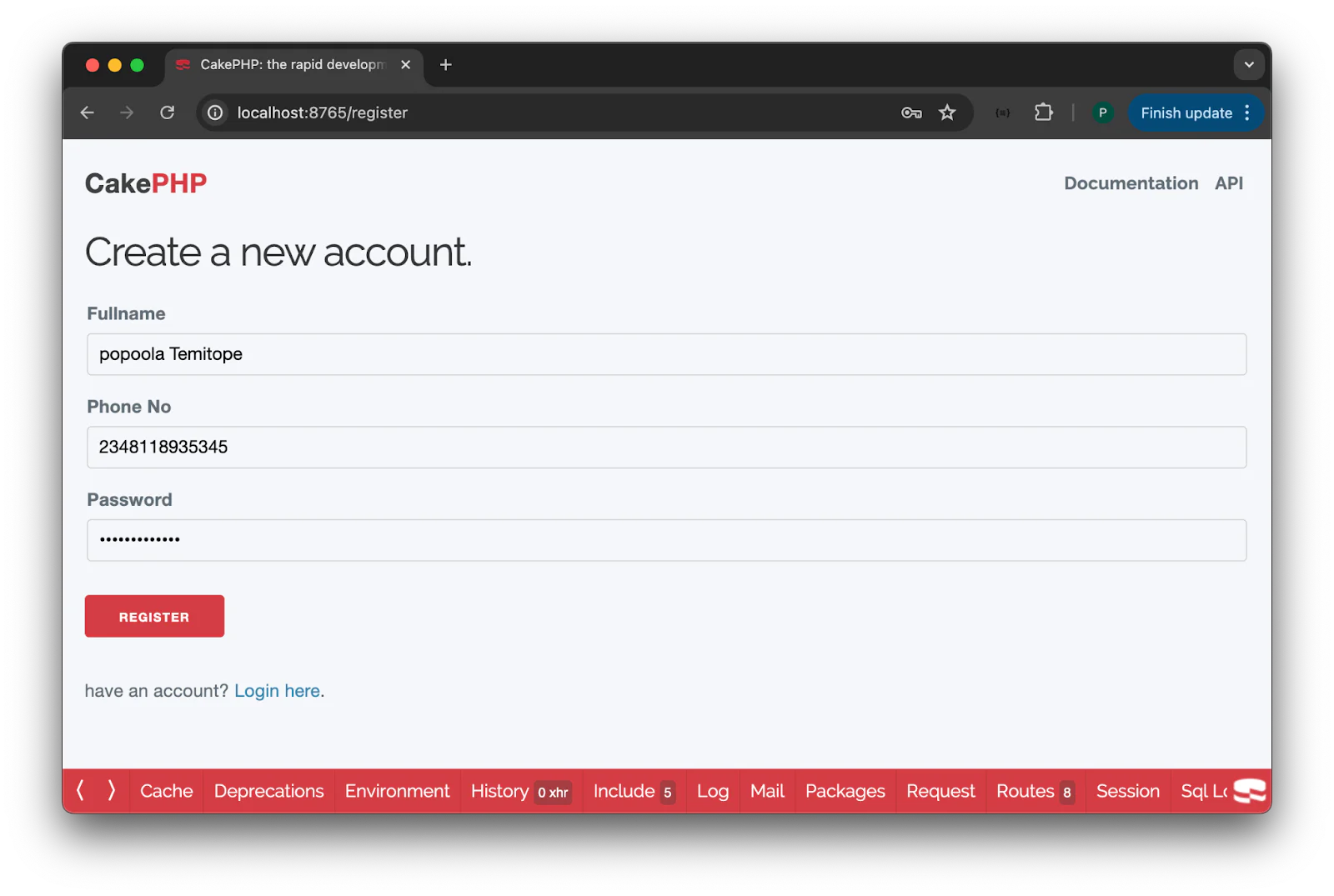
After creating a new account, you will be redirected to the login page where you can log in using a magic login link, as shown in the screenshot below.
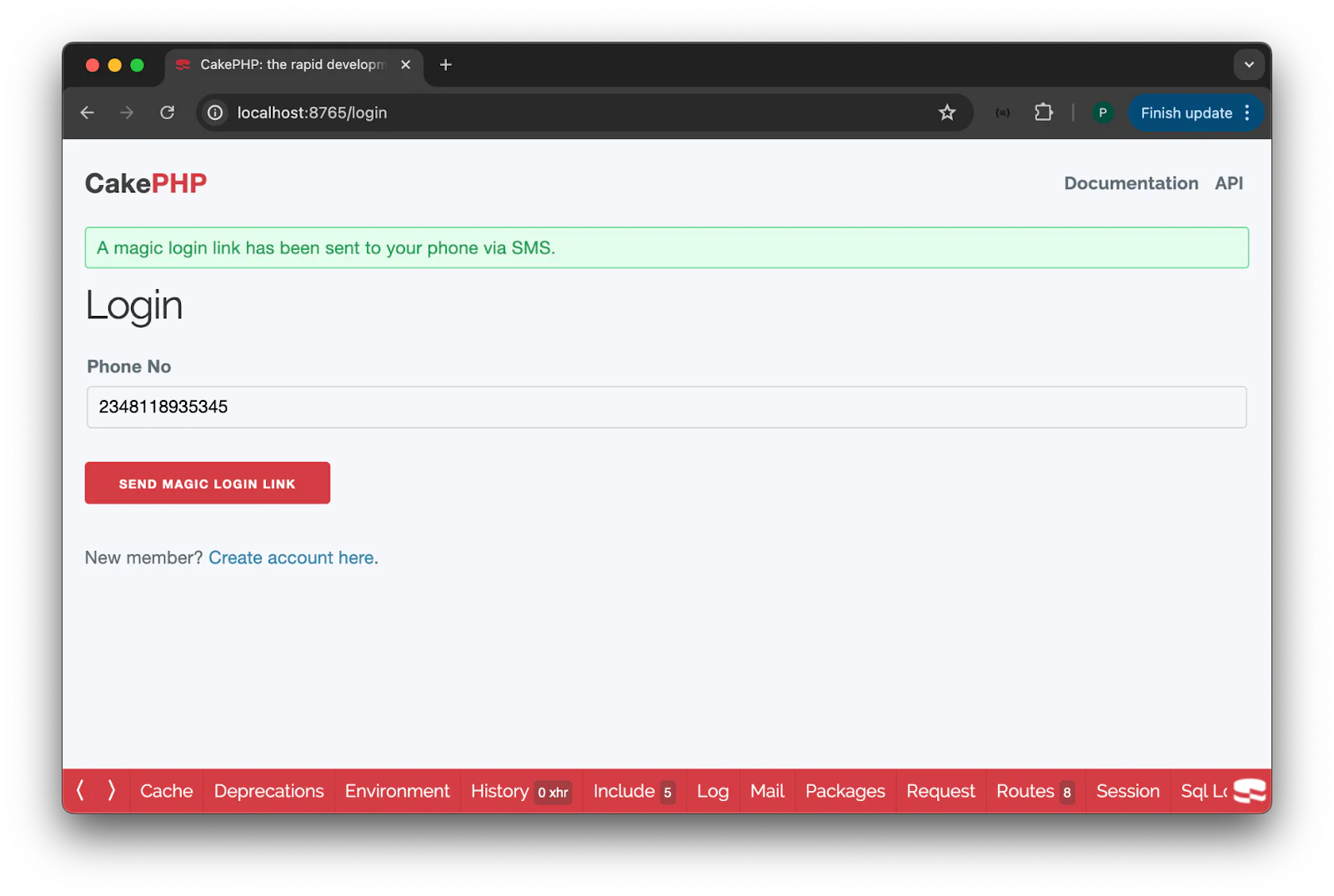
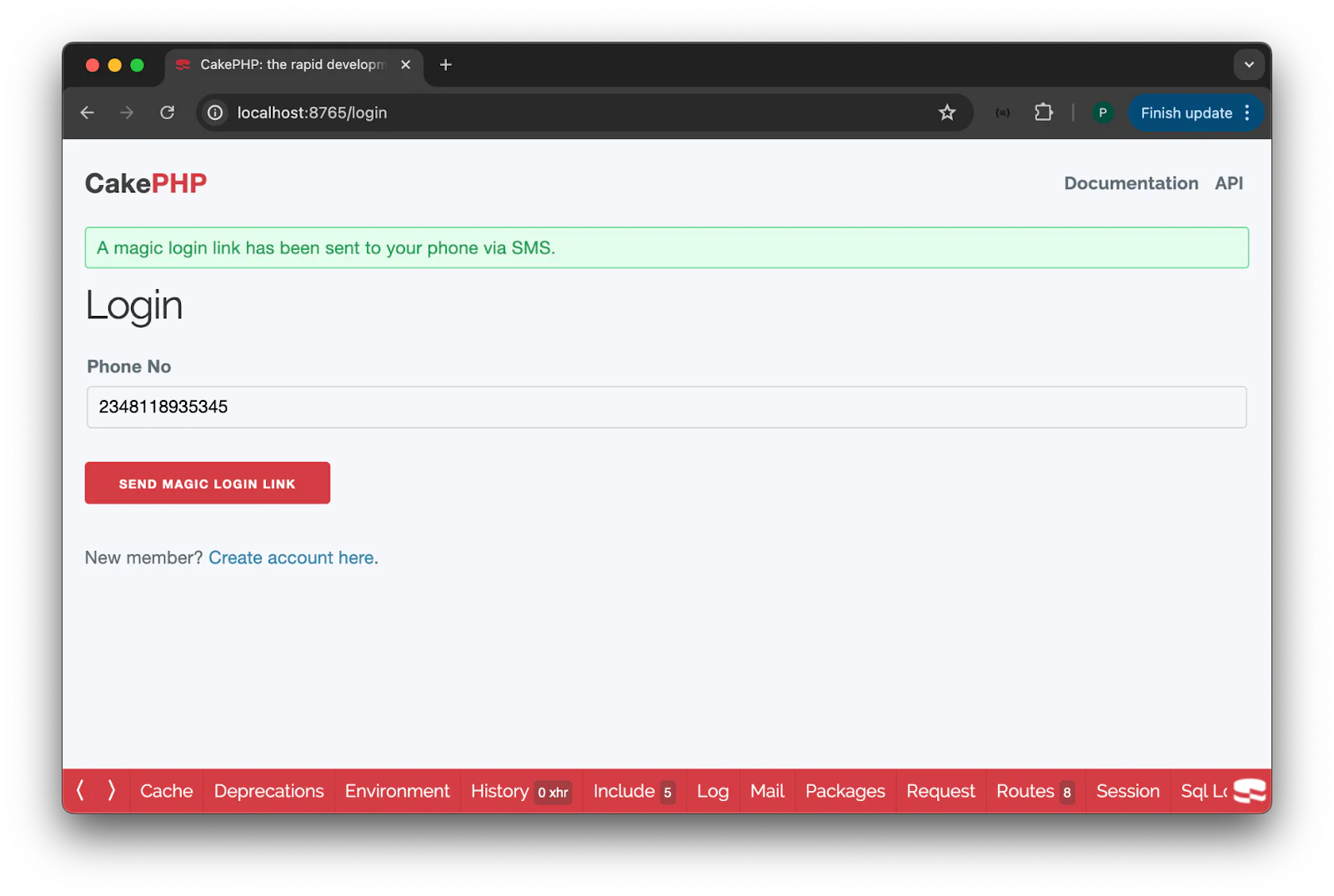
Once you enter your login phone number and click on SEND MAGIC LOGIN LINK, you will receive your magic login link via SMS, as shown in the screenshot below.
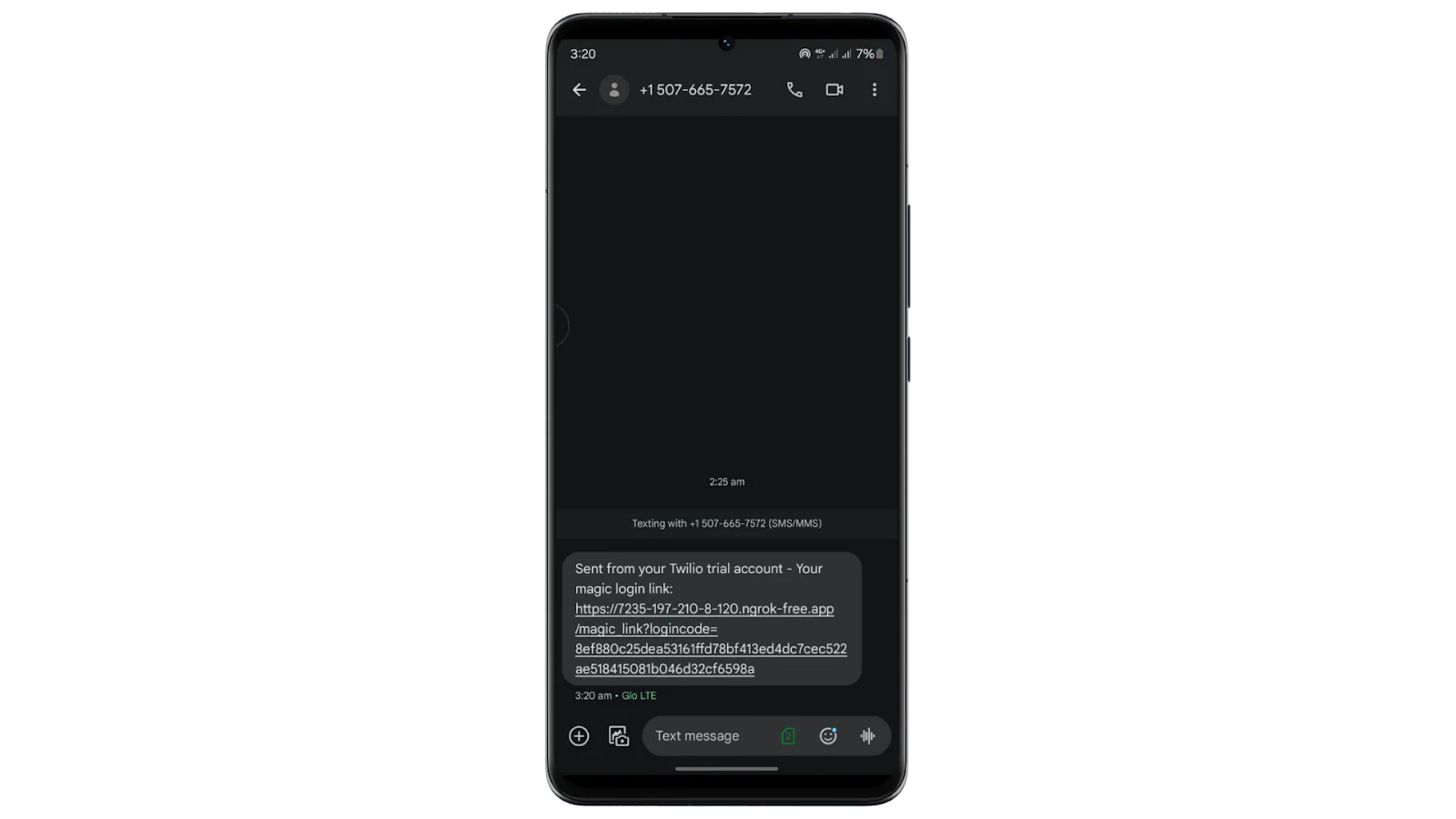
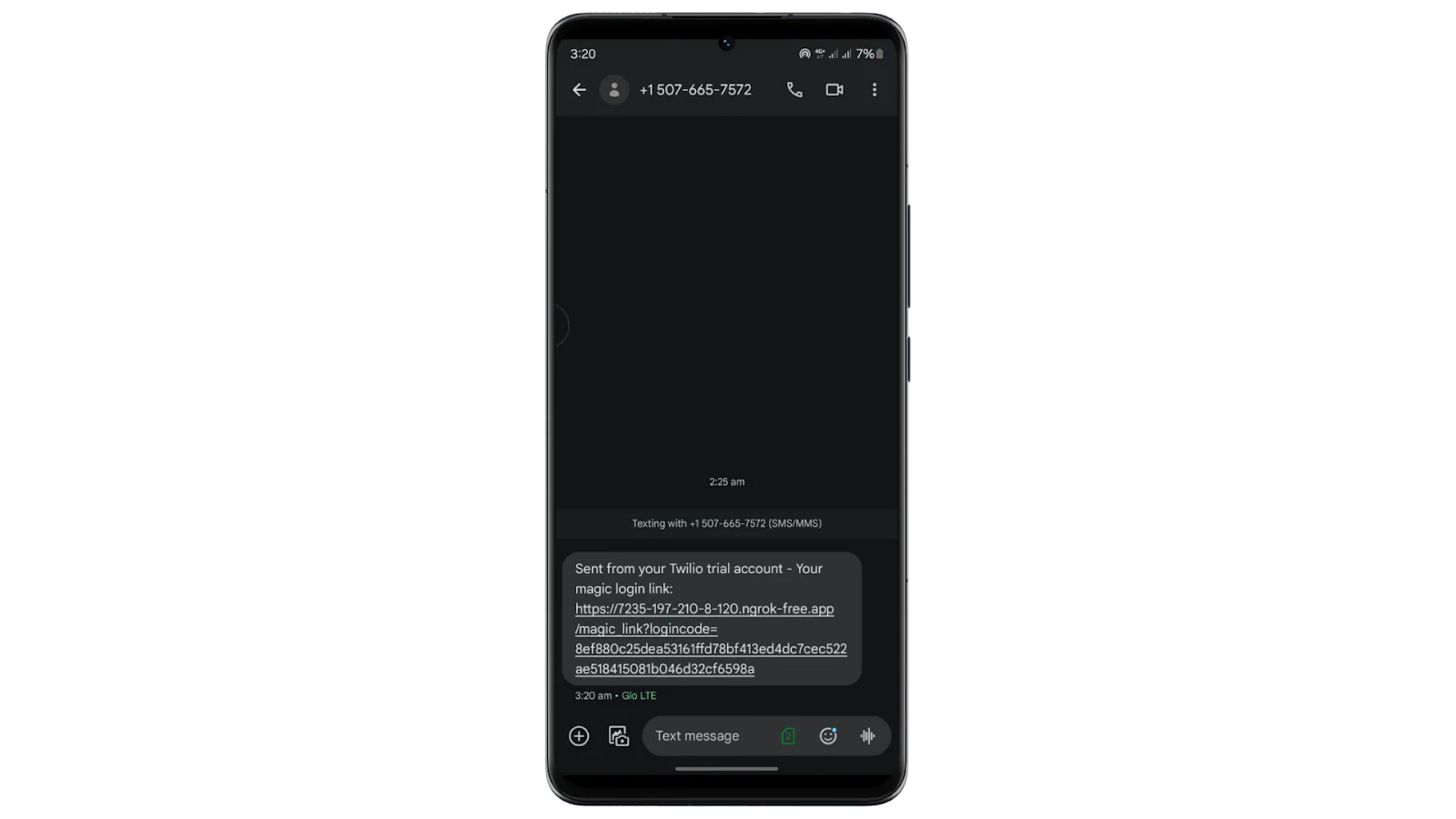
When you click on the received link, you will automatically log in to your profile page, as shown in the screenshot below.
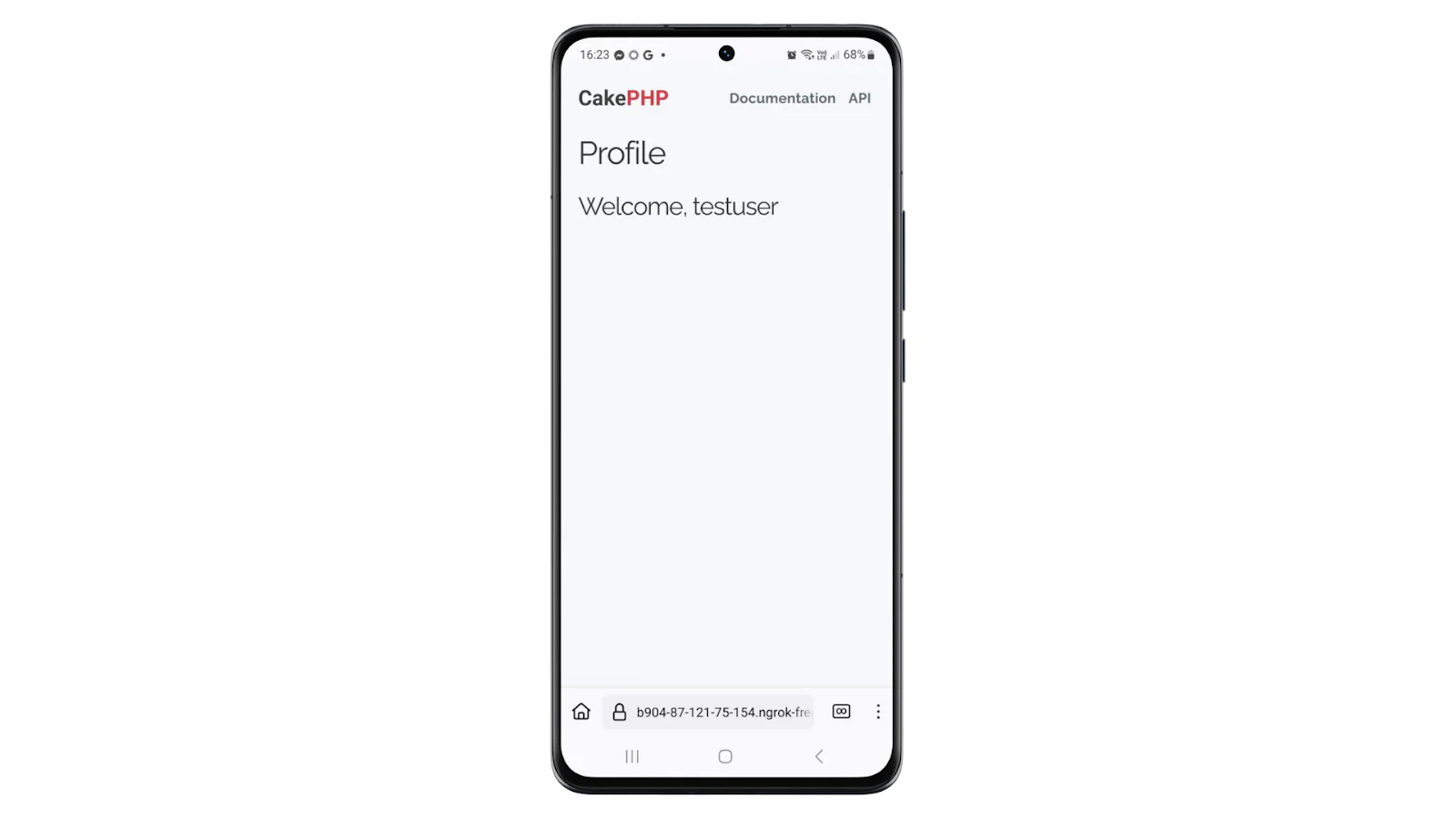
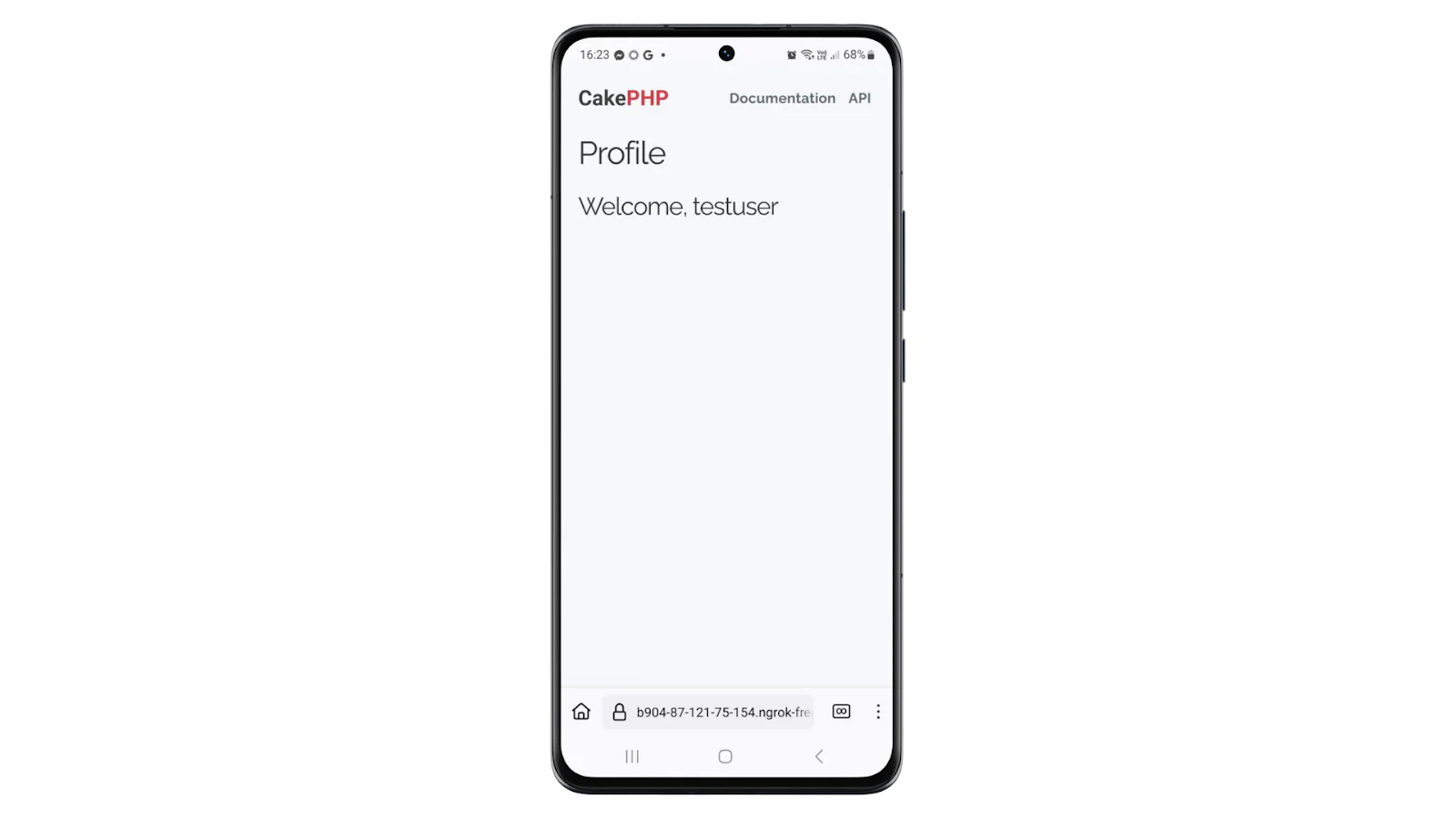
That’s how to implement magic links in a CakePHP application
Magic Link provides a seamless and secure authentication system that enhances the user experience by allowing users to log in without a password. In this tutorial, we explored how to implement a magic login link system in a CakePHP application using SMS.
Popoola Temitope is a mobile developer and a technical writer who loves writing about frontend technologies. He can be reached on LinkedIn.
The link icon in the tutorial's main image was created by Freepik on Flaticon.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.