How to Send a Picture on WhatsApp Using Twilio and Python
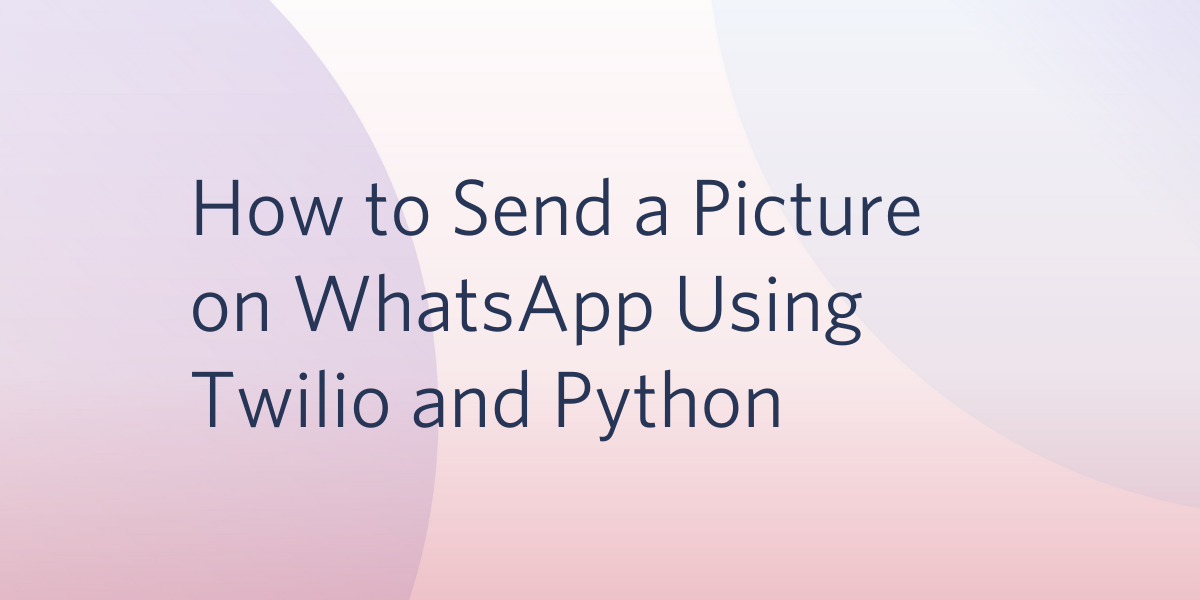
The WhatsApp Business API from Twilio is a powerful, yet easy to use service that allows you to communicate with your users on the popular messaging app.
In this article, we’ll walk you through how you can develop a functional Python program to send an image to a user through WhatsApp.
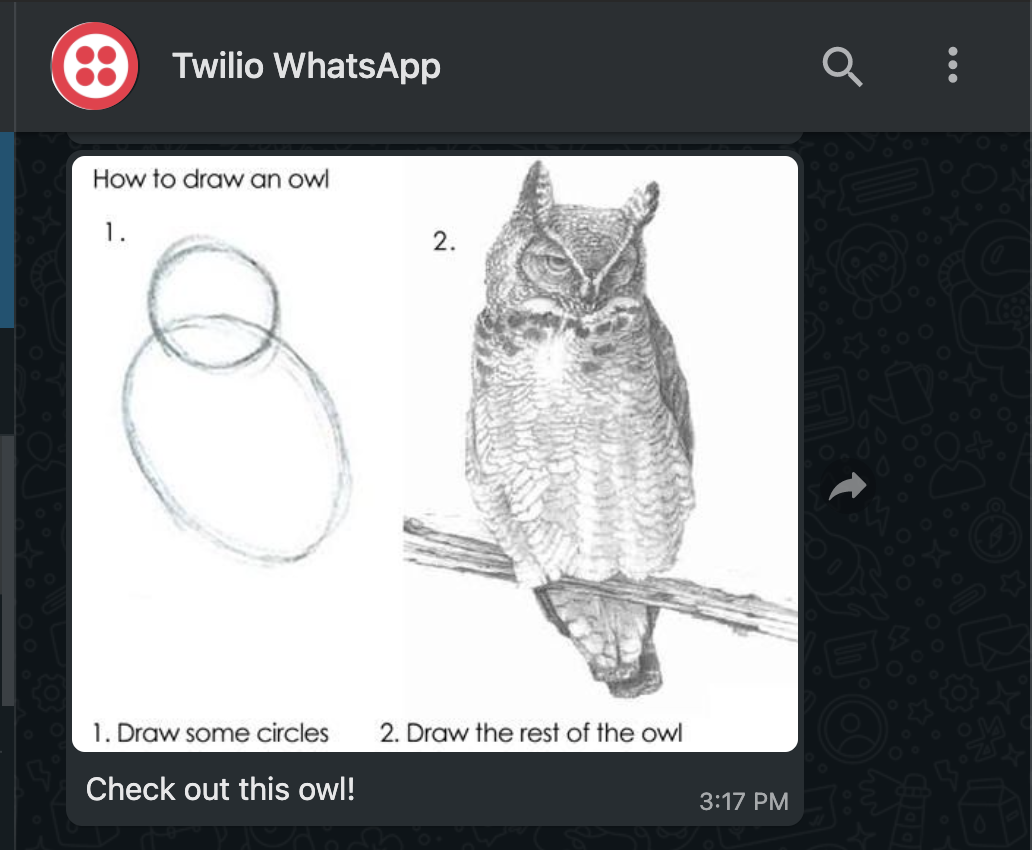
Prerequisites
To follow this tutorial you need the following items:
- Python 3.6 or newer. If your operating system does not provide a Python interpreter, you can go to python.org to download an installer.
- A Twilio account. If you are new to Twilio click here to create a free account now and receive $10 credit when you upgrade to a paid account. You can review the features and limitations of a free Twilio account.
- A smartphone with an active WhatsApp account, to test the project.
The Twilio WhatsApp sandbox
Twilio provides a WhatsApp sandbox, where you can easily develop and test your application. Once your application is complete you can request production access for your Twilio phone number, which requires approval by WhatsApp.
In this section you are going to connect your smartphone to the sandbox. From your Twilio Console, select Messaging, then select Try it Out on the sidebar. Open the WhatsApp section. The WhatsApp sandbox page will show you the sandbox number assigned to your account, and a join code.
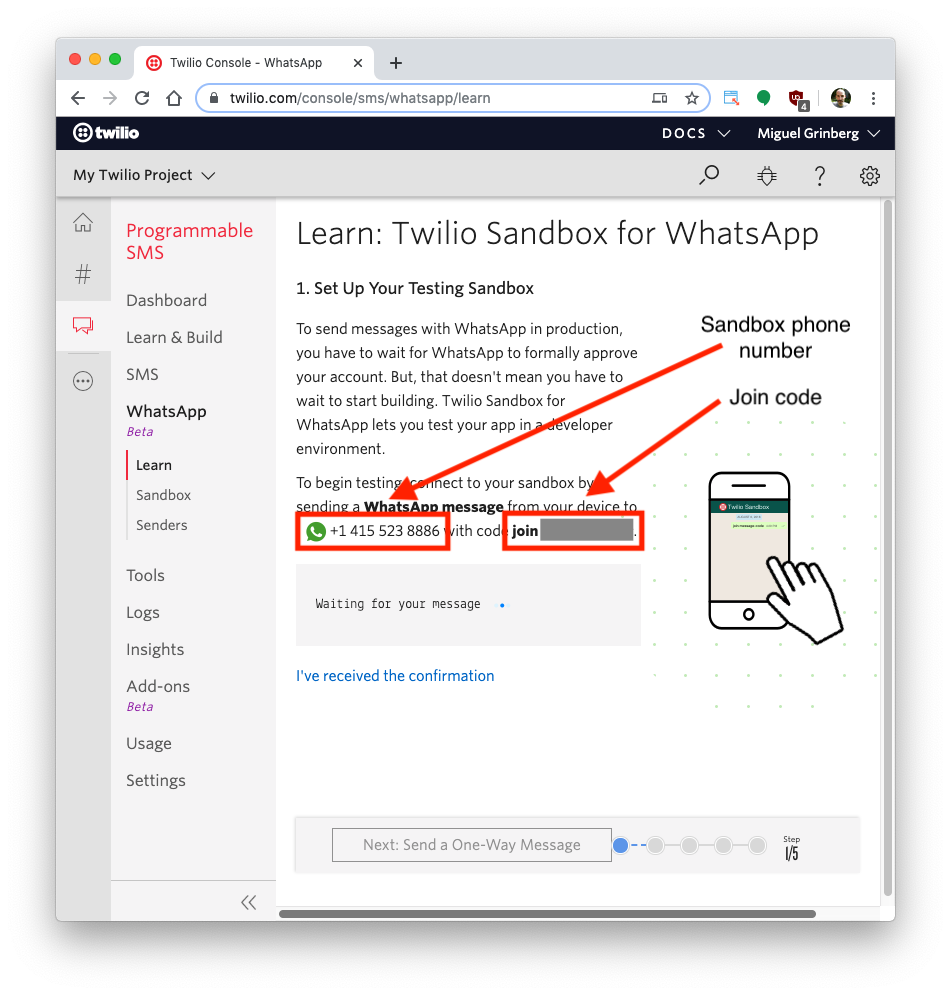
To enable the WhatsApp sandbox for your smartphone send a WhatsApp message with the given code to the number assigned to your account. The code is going to begin with the word "join", followed by a randomly generated two-word phrase.
Shortly after you send the message you should receive a reply from Twilio indicating that your mobile number is connected to the sandbox and can start sending and receiving messages.
If you intend to test your application with additional smartphones, then you must repeat the sandbox registration process with each of them.
Configuration
In this section you are going to set up a brand new Python project. To keep things nicely organized, open a terminal or command prompt, find a suitable place and create a new directory where the project you are about to create will live:
Since we will be installing some Python packages for this project, we need to create a virtual environment.
If you are using a Unix or MacOS system, open a terminal and enter the following commands:
If you are on a Windows machine, enter the following commands in a prompt window:
We’ll use the python-twilio package to send messages through the Twilio service.
Authenticate against Twilio services
We need to safely store some important credentials that will be used to authenticate against the Twilio service.
You can obtain the TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
credentials that apply to your Twilio account from the Twilio Console:
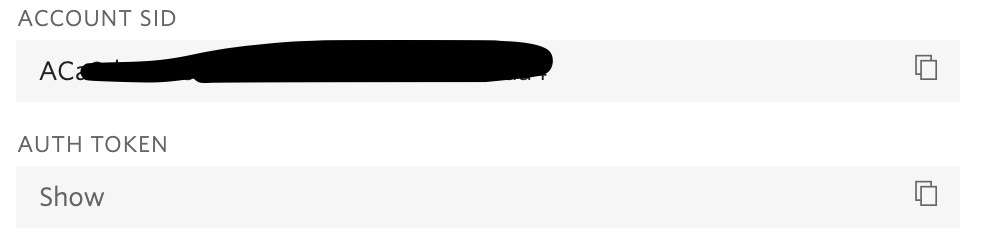
Once you have located the Account SID and Auth Token, we’ll be setting environment variables by typing the following commands into your terminal:
If you are following this tutorial on a Windows computer, replace export
with set
in the commands above.
Send a picture message with Twilio
Create a file in your project directory named app.py and copy and paste the following code in it:
The client
object initializes a Twilio client using the TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
variables set above. Replace <YOUR_WHATSAPP_SANDBOX_PHONE_NUMBER>
and <YOUR_WHATSAPP_PHONE_NUMBER>
with your actual numbers, using the E.164 format.
Your Python program creates a new message
instance of the Message
resource as provided by the Twilio Messaging API for WhatsApp in order to send a WhatsApp message to your personal phone number from the Twilio sandbox number. Make sure to replace those placeholders with the appropriate values.
The message
instance also allows you to include the body
of a message as well as a URL of an image you want to send. Feel free to change it to another image URL as you wish.
After the message is sent, the message SID is printed on your terminal for your reference.
In just a moment, you will see a ping from WhatsApp on your personal phone sent directly from your computer!
What's next for sending an image on WhatsApp with Python?
Congratulations on writing a short Python file and using the command line to send a quick image to your WhatsApp enabled device! This was so wickedly quick, can you even believe it was possible?
There's so much more you can do with Twilio and code without even picking up your phone. Here are some other neat WhatsApp media projects that you can check out:
- Build an image recognition app on WhatsApp using Twilio WhatsApp API, Clarifai API, Python, and Flask
- Learn Data Visualization with WhatsApp, Google Maps, Python, Twilio, and Clarifai
- Send SVG Images by SMS and WhatsApp with Python and CairoSVG
Let me know about the projects you're building with WhatsApp, Twilio, and Python by reaching out to me over email!
Diane Phan is a Developer Network editor on the Developer Voices team. She loves to help programmers tackle difficult challenges that might prevent them from bringing their projects to life. She can be reached at dphan [at] twilio.com or LinkedIn.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.