How to Send a Text Message with Python
Time to read: 2 minutes
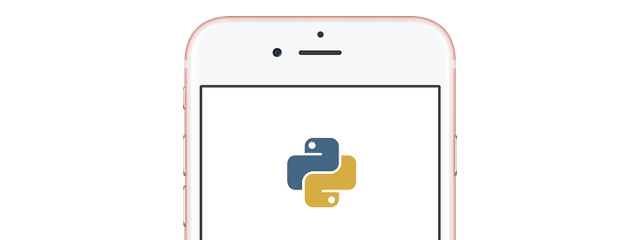
You’re building a Django or Flask app and you need to send text messages. Did you know you could do it in only 44 seconds? Here’s a video to show you how quick it is to get started:
Video: How To Send a Text Message with Python in 44 Seconds
You can’t copy and paste code from a video, so maybe that’s not very helpful. Here’s all of the code you would need:
How do I run this?
If you want to run that code, open a file called send_sms.py
and copy and paste that code into it. Don’t forget to replace the to
and from_
phone numbers with their appropriate values.
Grab your Account SID and auth token from your Twilio account Console. Set these in your environment variables by entering this in your terminal:
If you had trouble setting your environment variables or are running Windows, check out this blog post. Now enter this in your terminal to install the Twilio Python library and run your code (from the same directory the file is saved in):
What just happened?
Let’s walk through the code in the video step by step.
First you import the Twilio Rest Client
Instantiate a REST client using your account sid and auth token, available in your Twilio account Console:
In the video and earlier in the post, these are stored in environment variables.
You’ll now need three things:
- The number you are sending the message to
- The Twilio number you are sending the message from
- The body of the message
With these you can now send a text message by calling client.sendMessage()
:
Now just wait for the magic to happen!
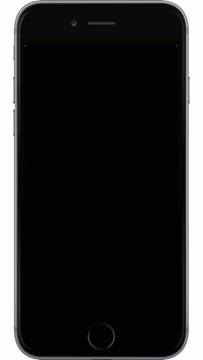
We can’t wait to see what you build
You’ve sent a text message and now you’re ready to take on the world. Check out the Twilio REST API documentation and the documentation for working with the Python helper library to see what else you can do.
You can also take a look at our tutorials to see more examples such as: sending SMS notifications, masking phone numbers for user privacy or two factor authentication for user security.
I’m looking forward to seeing what you will build. Feel free to reach out and share your experiences or ask any questions.
- Email: sagnew@twilio.com
- Twitter: @Sagnewshreds
- Github: Sagnew
- Twitch (streaming live code): Sagnewshreds
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.