How to Send an RCS message with Twilio and Python
Time to read: 5 minutes
Rich Communication Services (RCS) is revolutionizing digital communication by bringing together the best of SMS and modern messaging apps. The recent addition of RCS support on iOS 18 has opened up exciting possibilities for reaching Apple users. As developers worldwide begin exploring this powerful channel, the potential for creating engaging messaging experiences has never been greater.
In this tutorial, we'll show you how to get started with RCS using Twilio and Python.
Comparing RCS to SMS and WhatsApp
Think of RCS as the natural evolution of SMS, enhanced with features you'd typically find in modern messaging apps. Unlike WhatsApp or other messaging platforms, RCS works directly through your phone's default messaging app - no additional downloads required. You get all the powerful features: rich media sharing, typing indicators, read receipts, verified business profiles, and interactive buttons. For businesses, this means higher engagement rates and better analytics, while users enjoy a seamless, app-like experience without the friction of installing another messaging app.
What sets RCS apart is its ability to maintain the universal reach of SMS while delivering these enhanced capabilities. For a deeper dive into how RCS stacks up against other messaging channels, check out the Guide to Rich Communication Services: What Developers Need to Know.
Implementing RCS with Twilio and Python
Twilio makes it easy to explore and implement RCS. In this section, you’ll learn how to set up an RCS sender, create a messaging service, and send your first RCS message using Python. Follow these step-by-step instructions to get started.
Prerequisites
- A Twilio account (sign up here).
- A Twilio phone number for sending SMS, as a fallback to your RCS service.
- A device capable of receiving RCS business messages.
- Python installed on your machine.
Step 1: Create RCS Sender
- Log in to the Twilio Console.
- Navigate to the Explore Products section and click RCS.
(If RCS isn’t available, you may need to request access.)
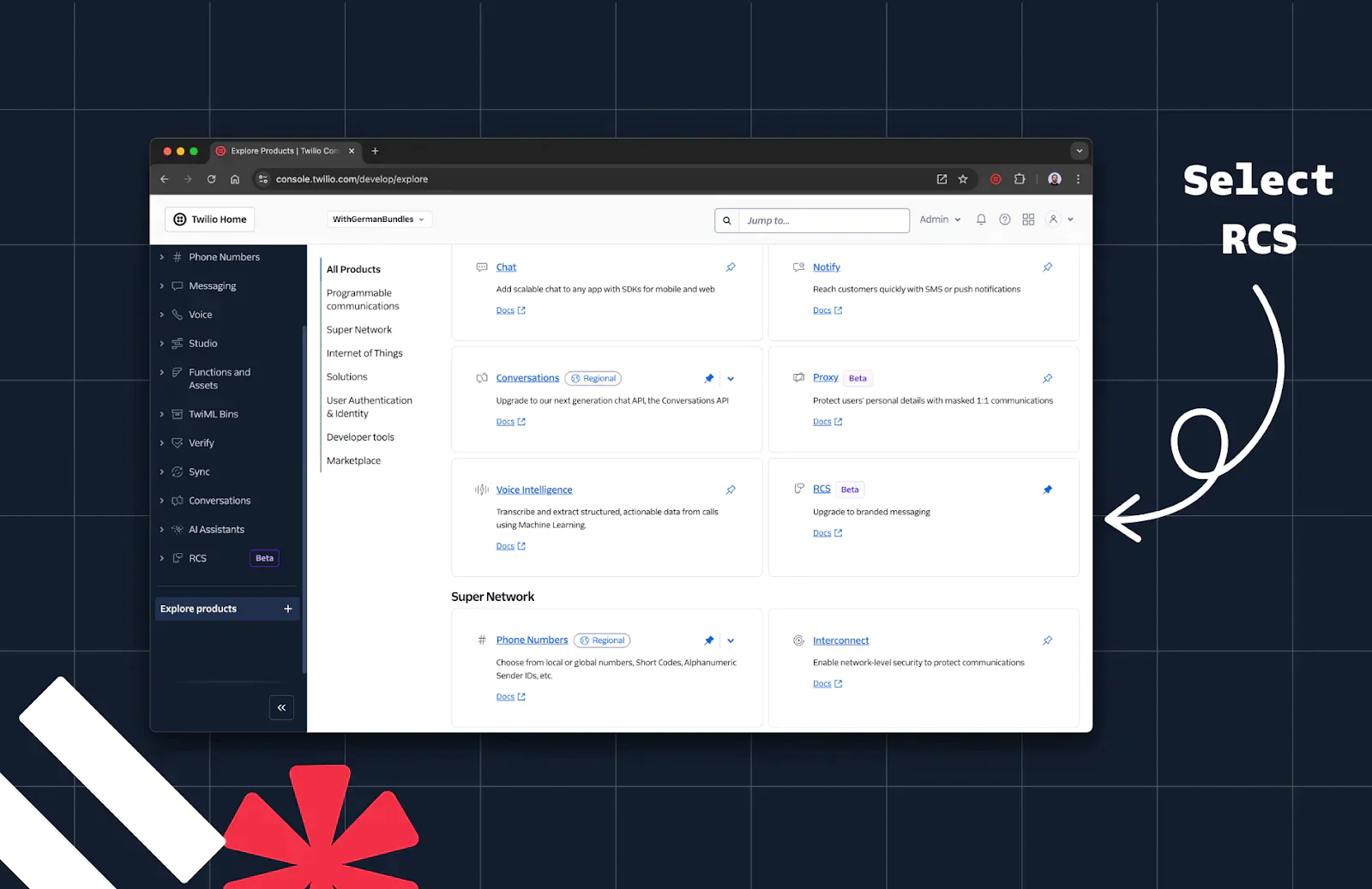
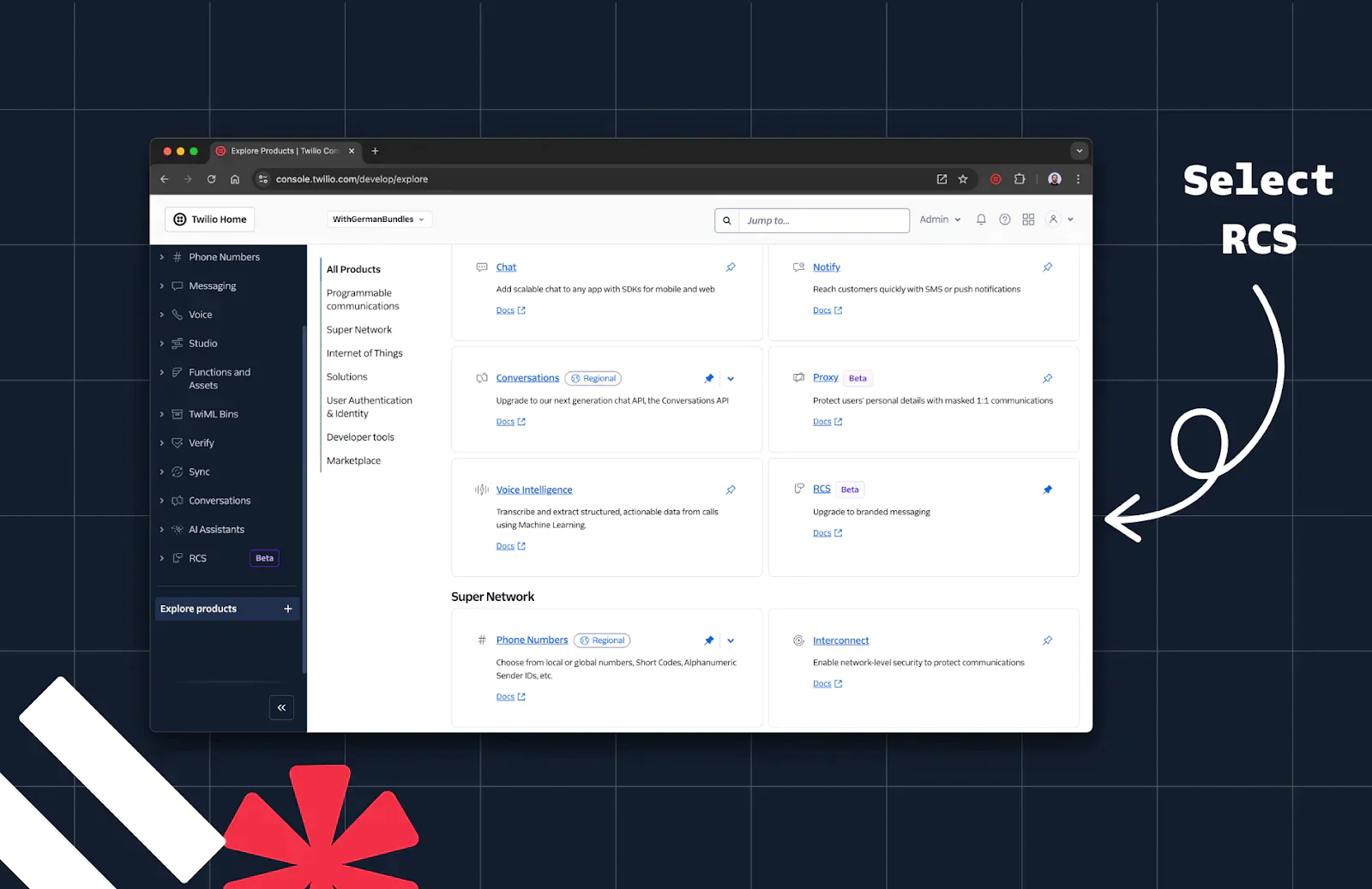
3. In the side panel, navigate to the Senders section.
4. Click Create new sender.
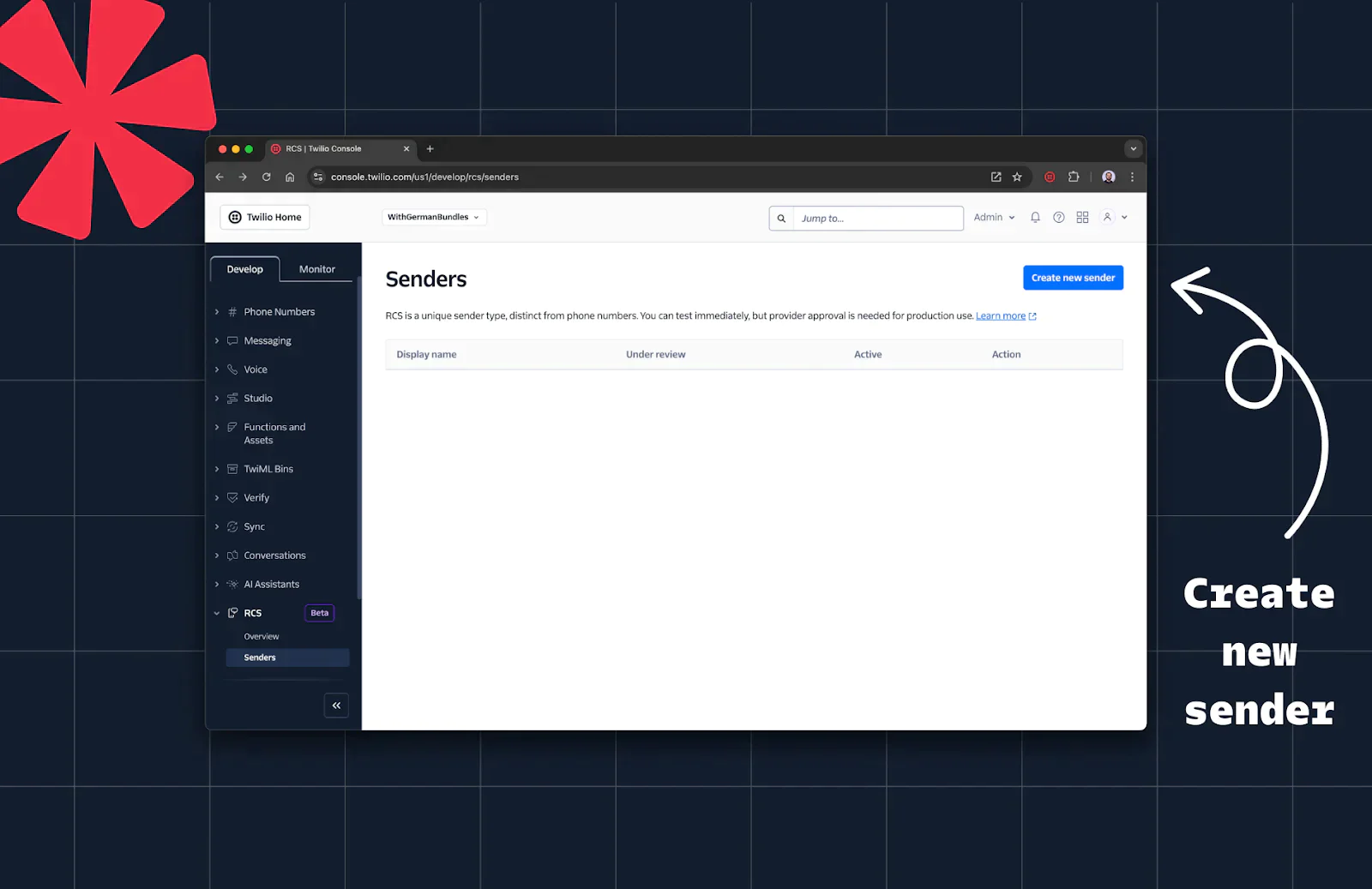
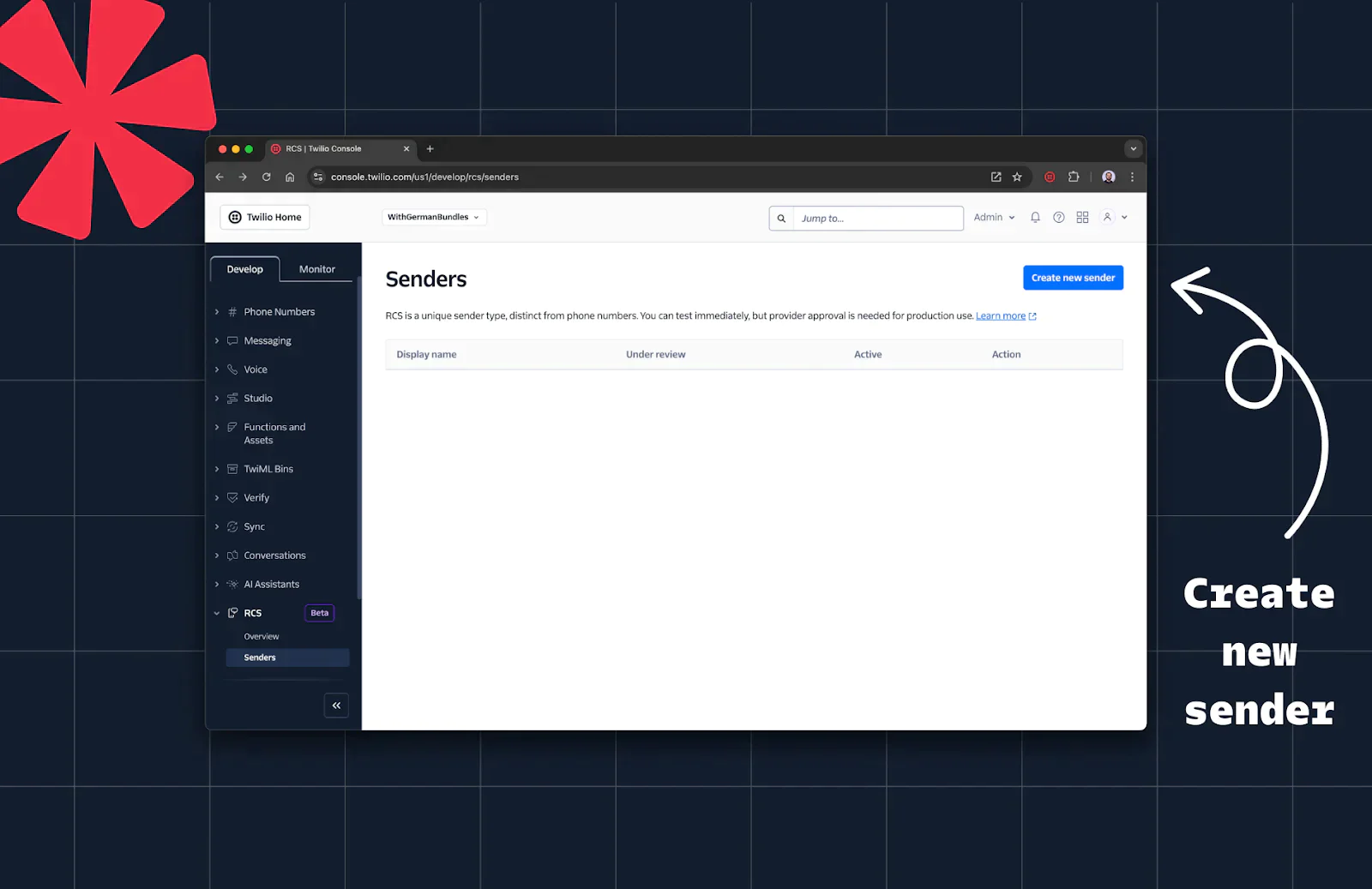
5. Provide a name (for example: “My First RCS Sender”). Click the continue button.
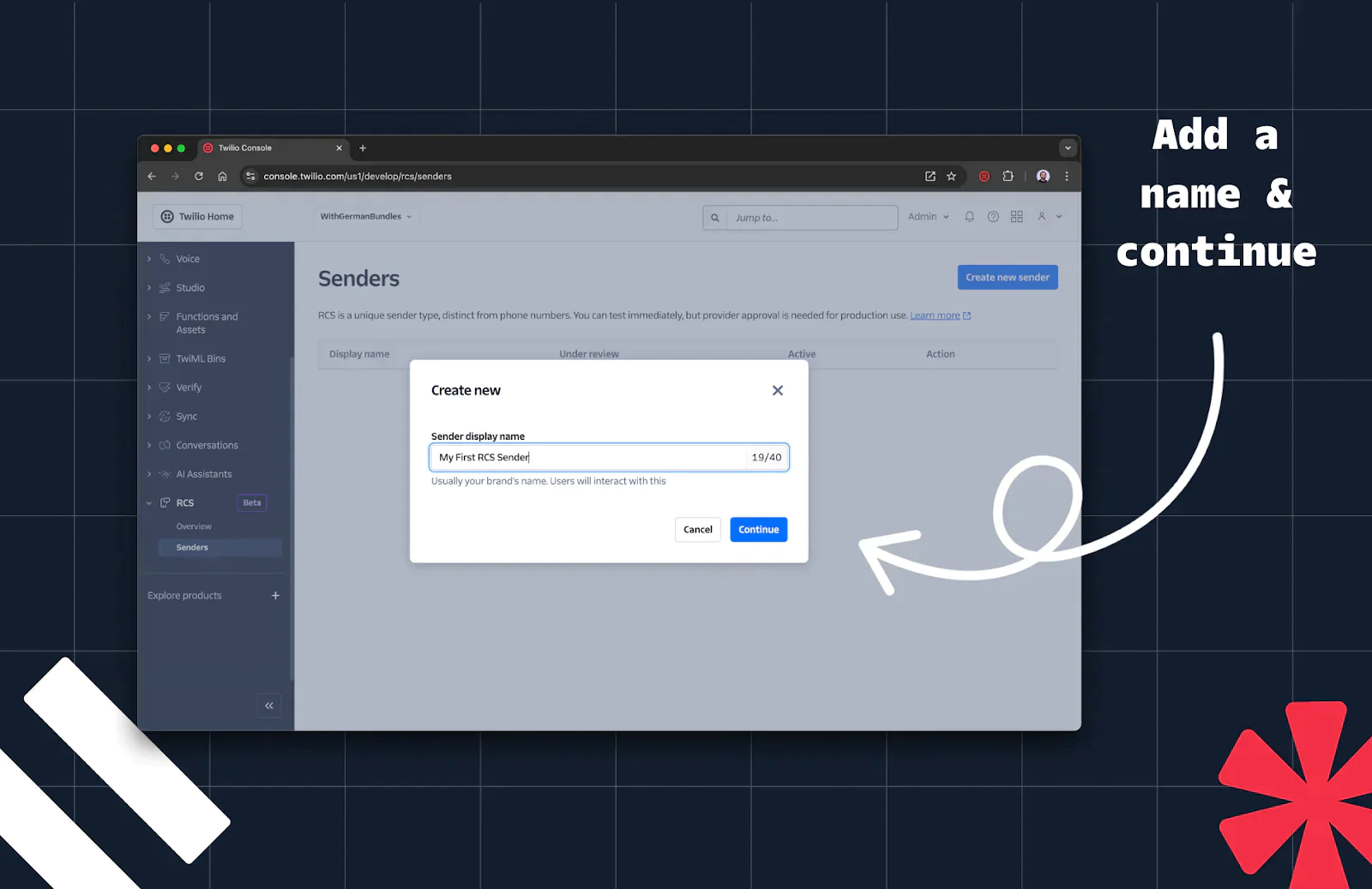
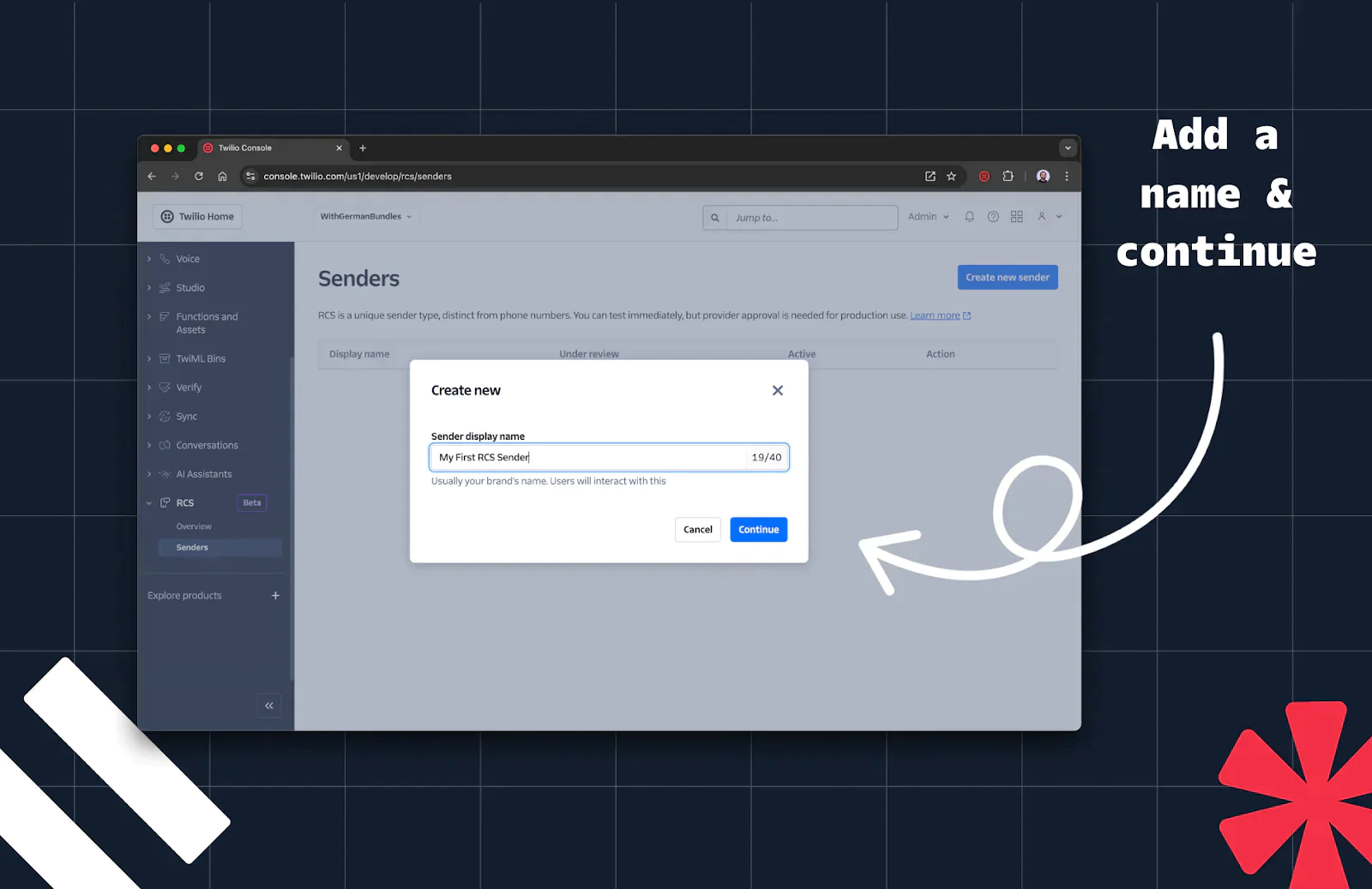
Step 2: Add Profile Information
Next, you’ll add profile information for your RCS sender. Use the following:
- Sender display name: Choose a recognizable name for your sender. Usually, this is your brand name. For our example, we will use “My First RCS Sender”.
- Description: Describe your brand. This description will be displayed just under your display name at the top of your user’s RCS interactions.
- Border color: Keep in mind the minimum contrast ratio. For testing purposes, start with
#000000
to keep it simple. - Logo: Your brand logo should meet the following requirements: • Dimensions: 224x224 pixels • File size: Max 50 KB • Image type: JPEG/JPG/PNG • For testing, you can use this example image:
https://corn-lobster-7338.twil.io/assets/twiliodevs-logo-rcs.png
- Banner: Your brand banner should meet the following requirements: • Dimensions: 1440x448 pixels • File size: Max 200KB • Image type: JPEG/JPG/PNG • For testing, you can use this example image:
https://corn-lobster-7338.twil.io/assets/twiliodevs-banner-rcs.png
- Contact Details - Phone number: A phone number associated with your RCS sender is required. Optionally, you can also add an email address.
- Link to privacy policy and Link to terms of service: For testing purposes, you can enter links to arbitrary information here. When you begin building for production usage, make sure to create an RCS sender with real information about your business.
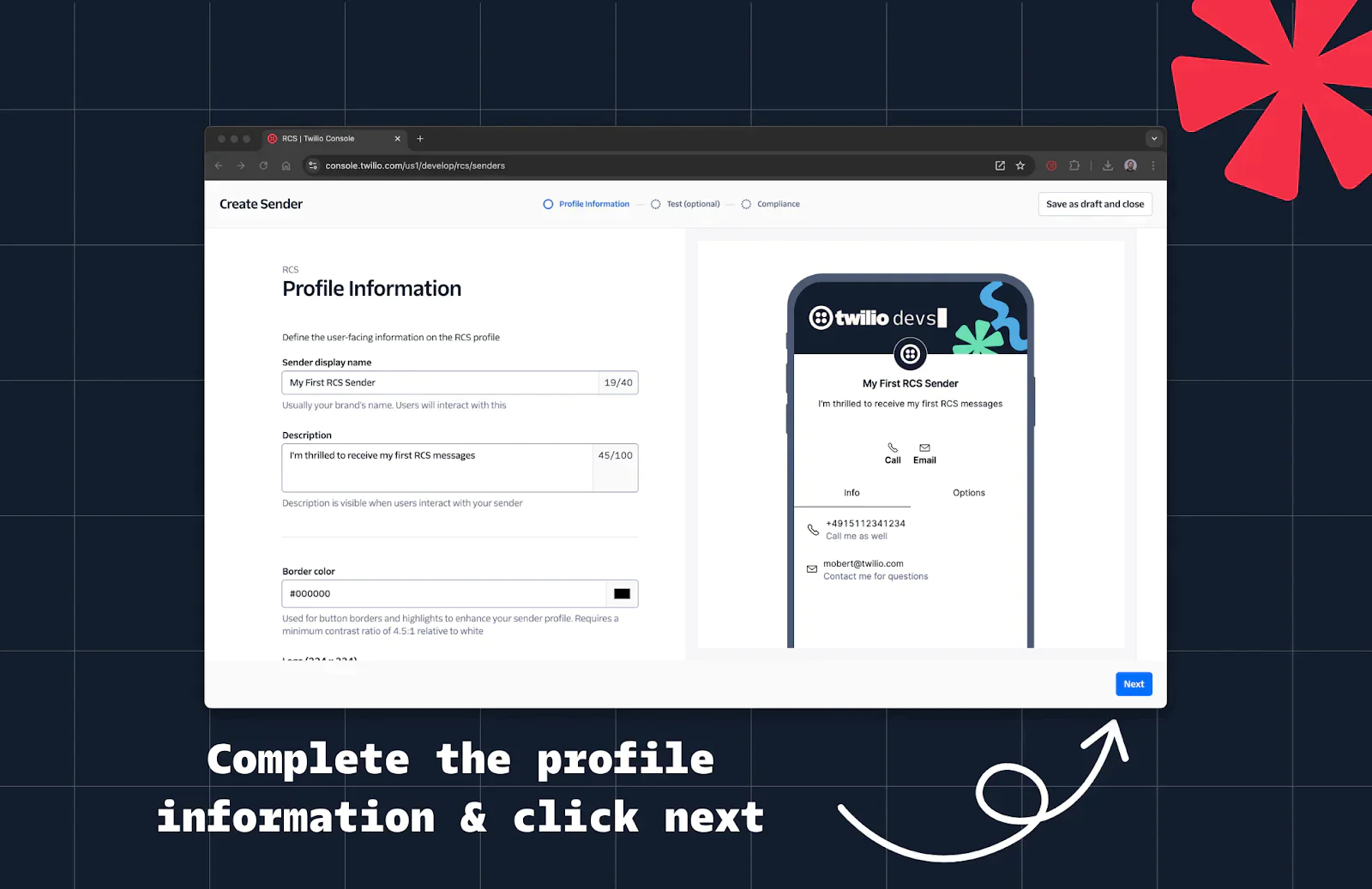
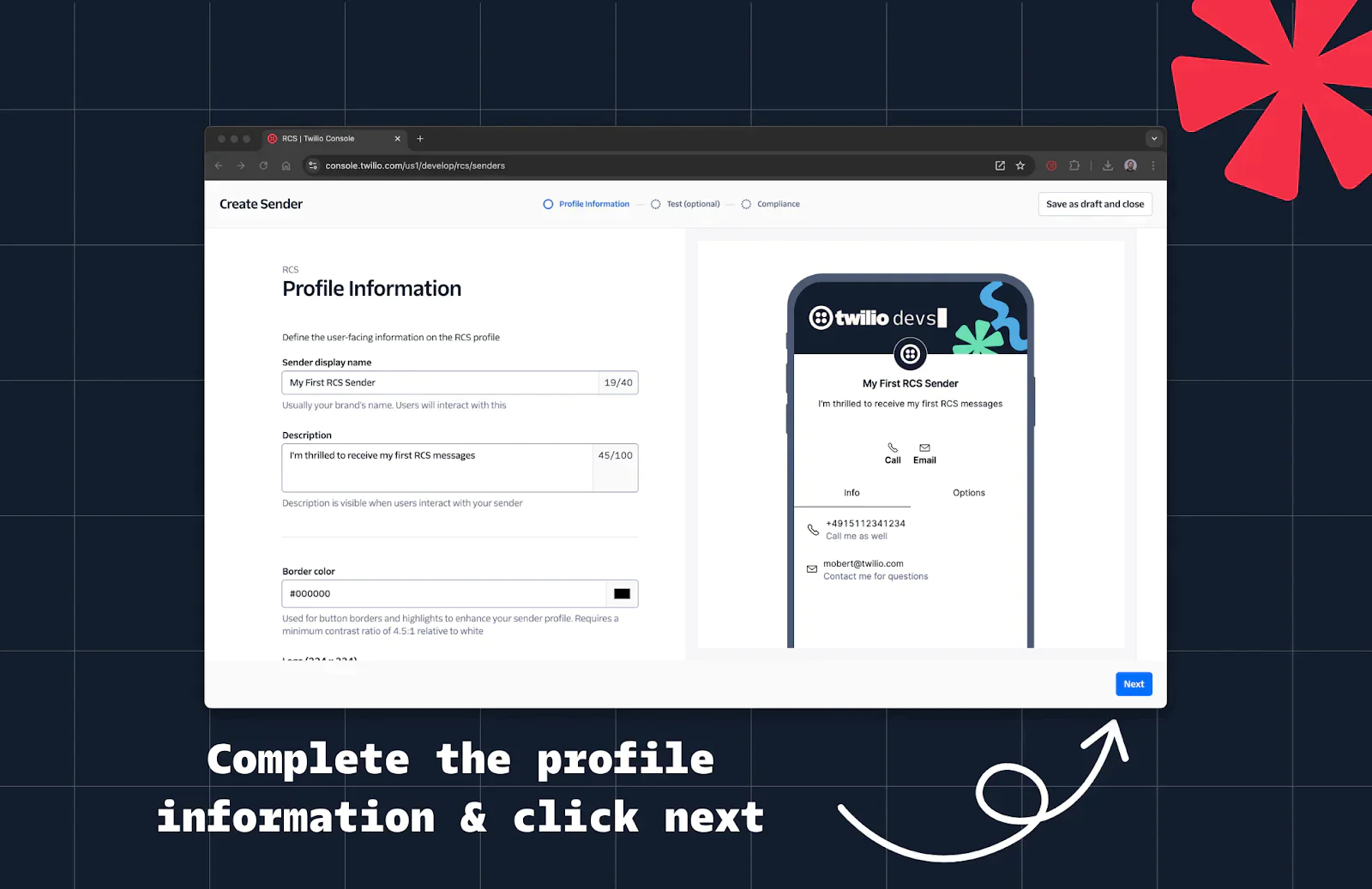
Step 3: Test your RCS Sender
1. On the optional Test tab, click Add device to test this sender.
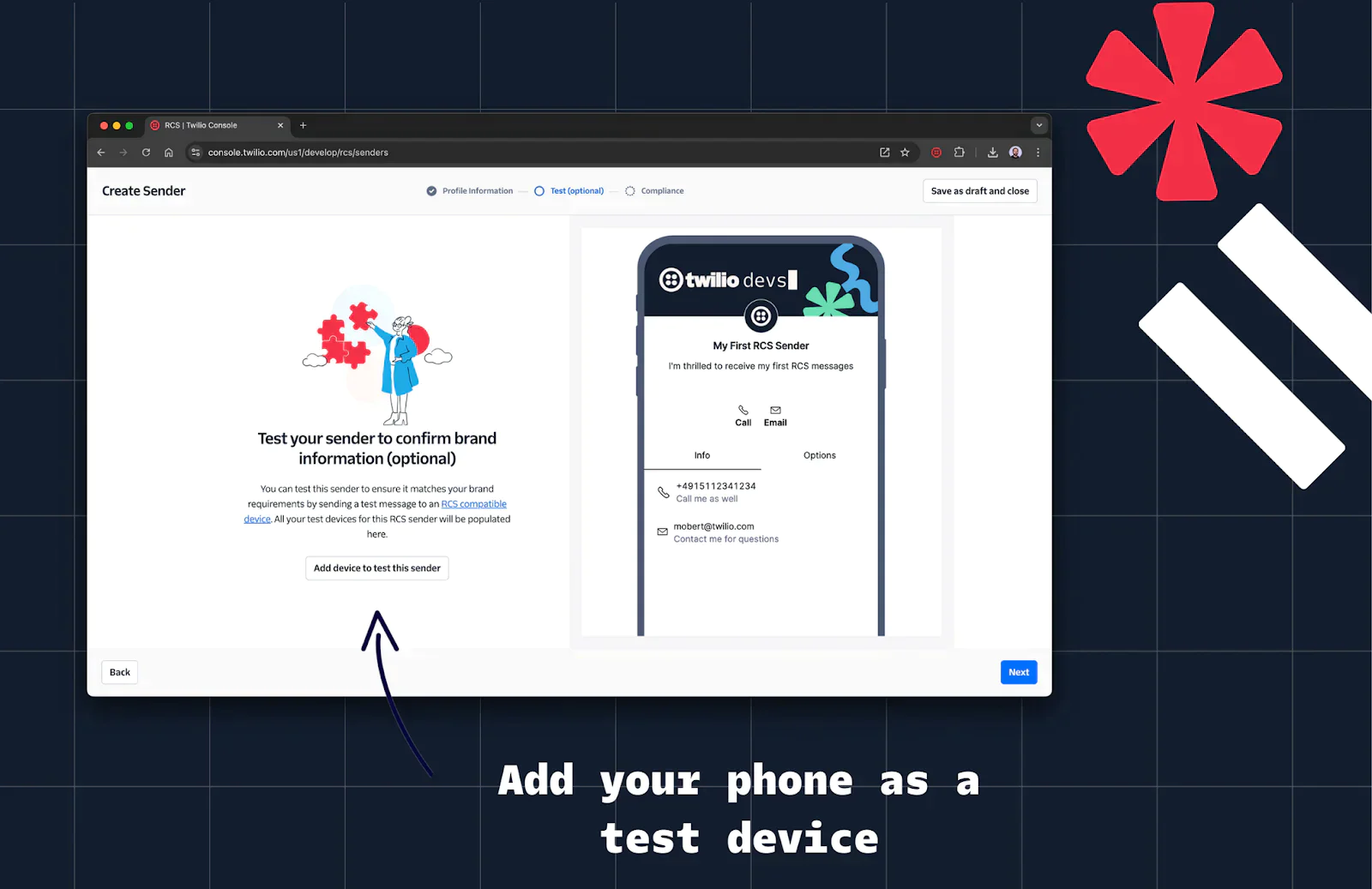
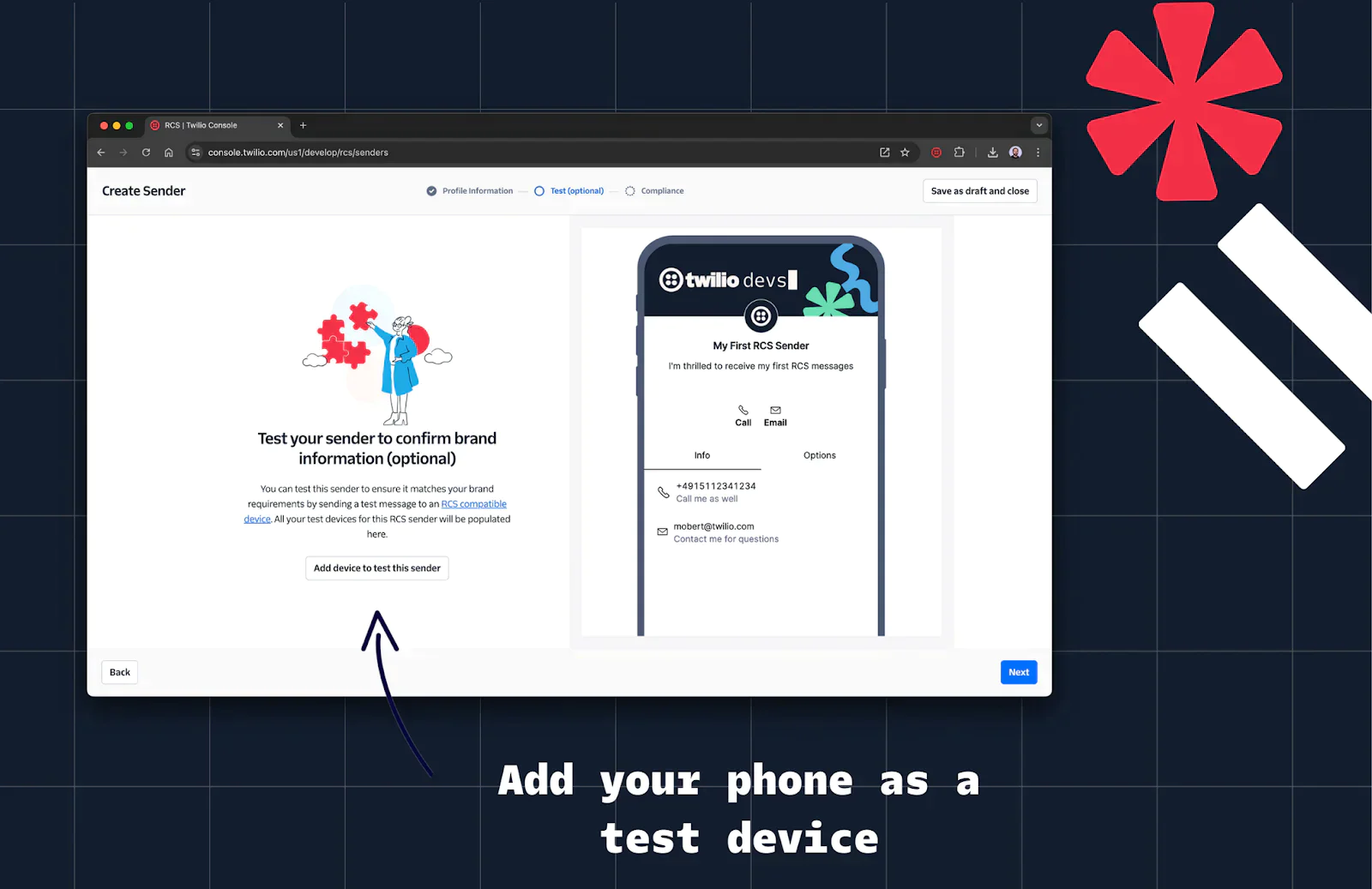
2. Enter the phone number of your RCS-capable device and click Invite.
3. You should receive an opt-in message from Twilio’s RCS agent on your device.
4. Confirm the opt-in on your device by selecting Make me a tester.
5. Check the dialog box in the Twilio Console, and send a test message.
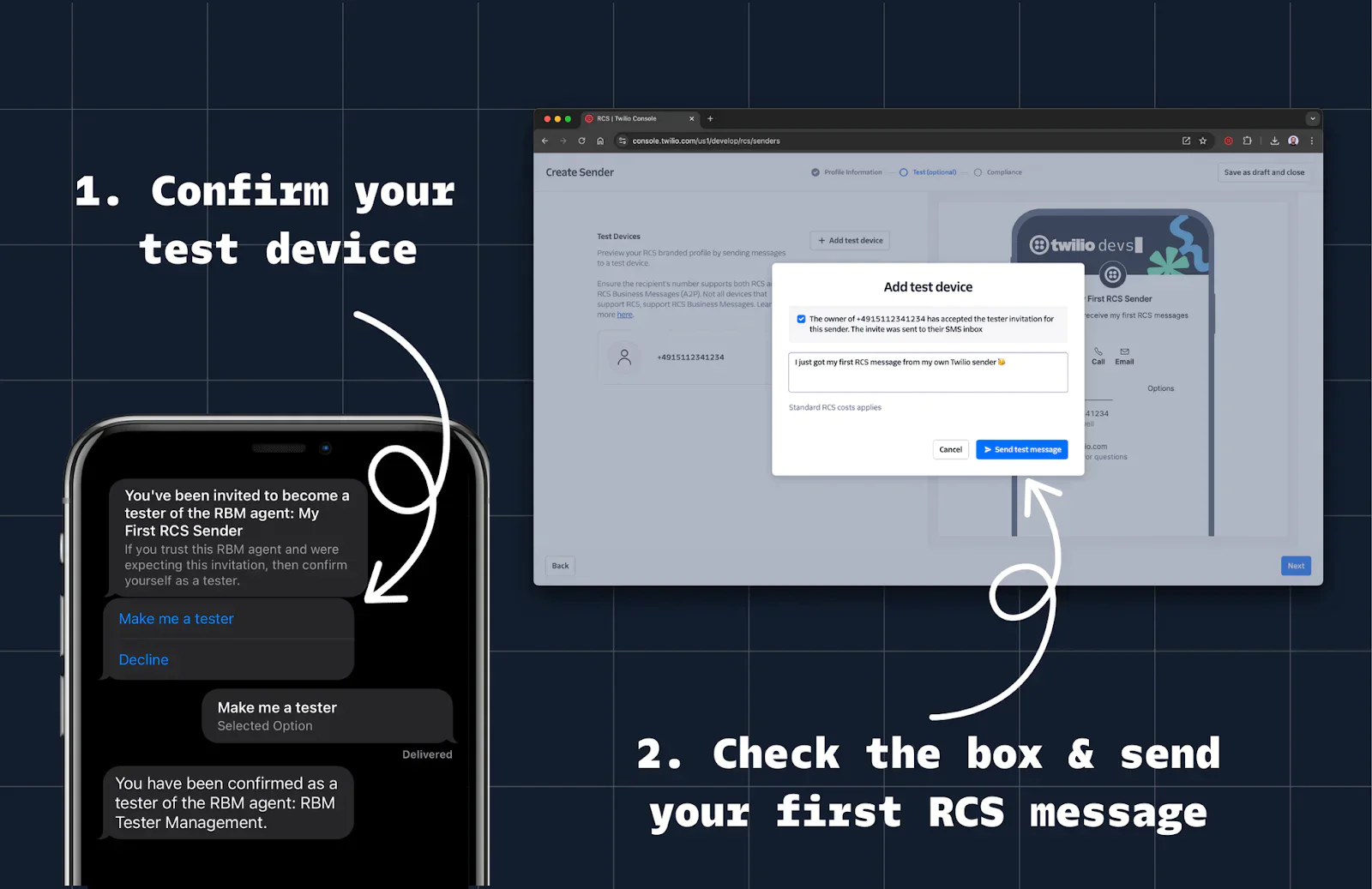
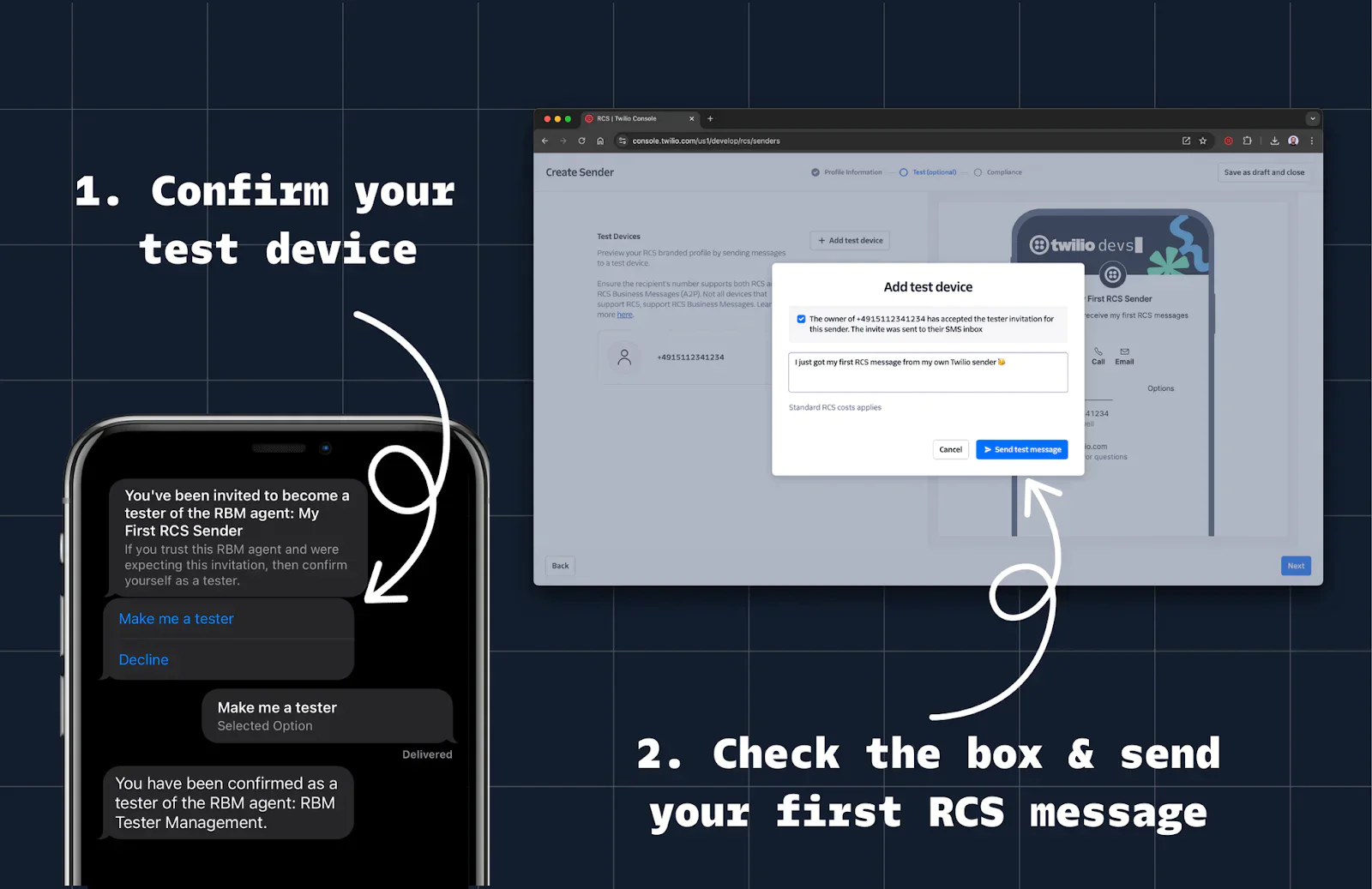
6. Excellent! You’ve just sent your first RCS message!
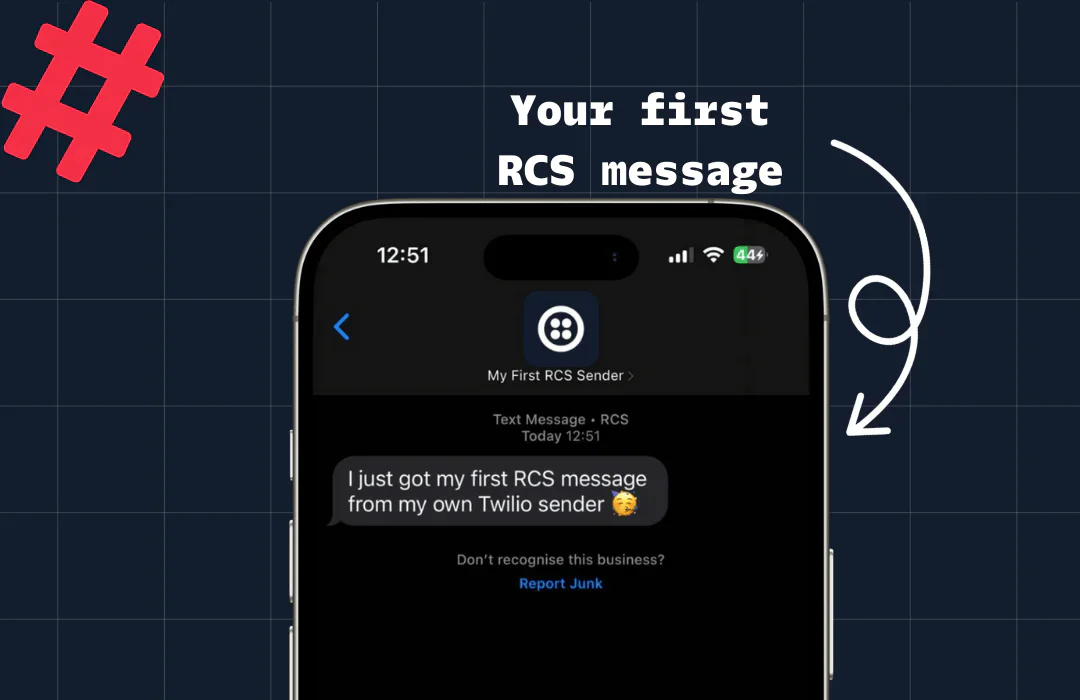
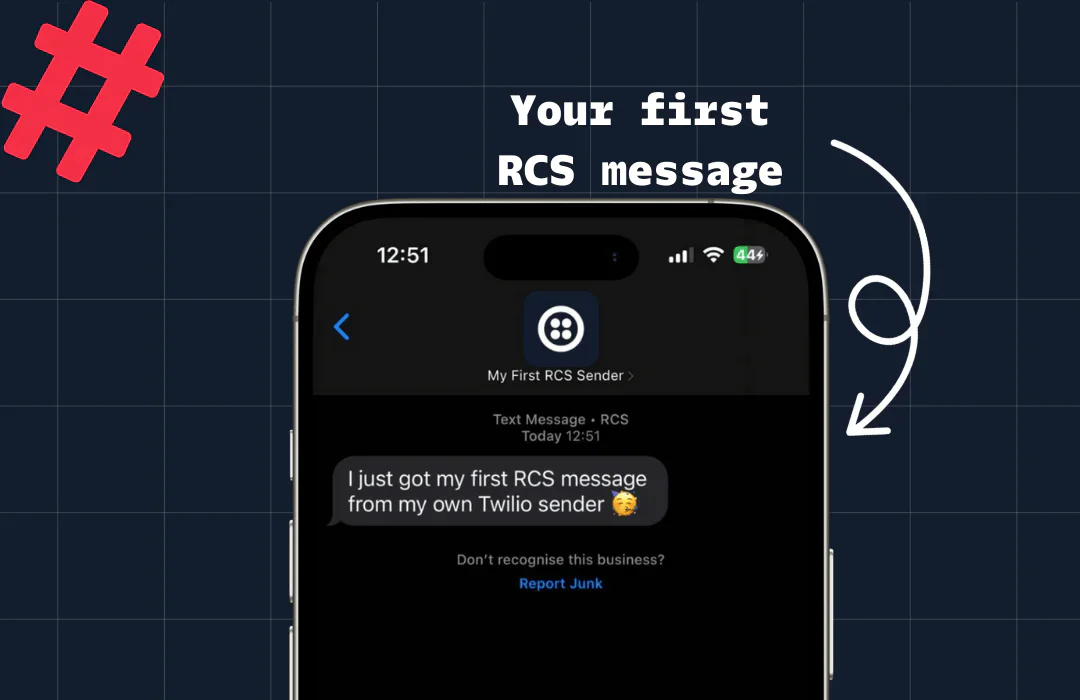
7. Since this is just a test sender, click Save as draft and close.
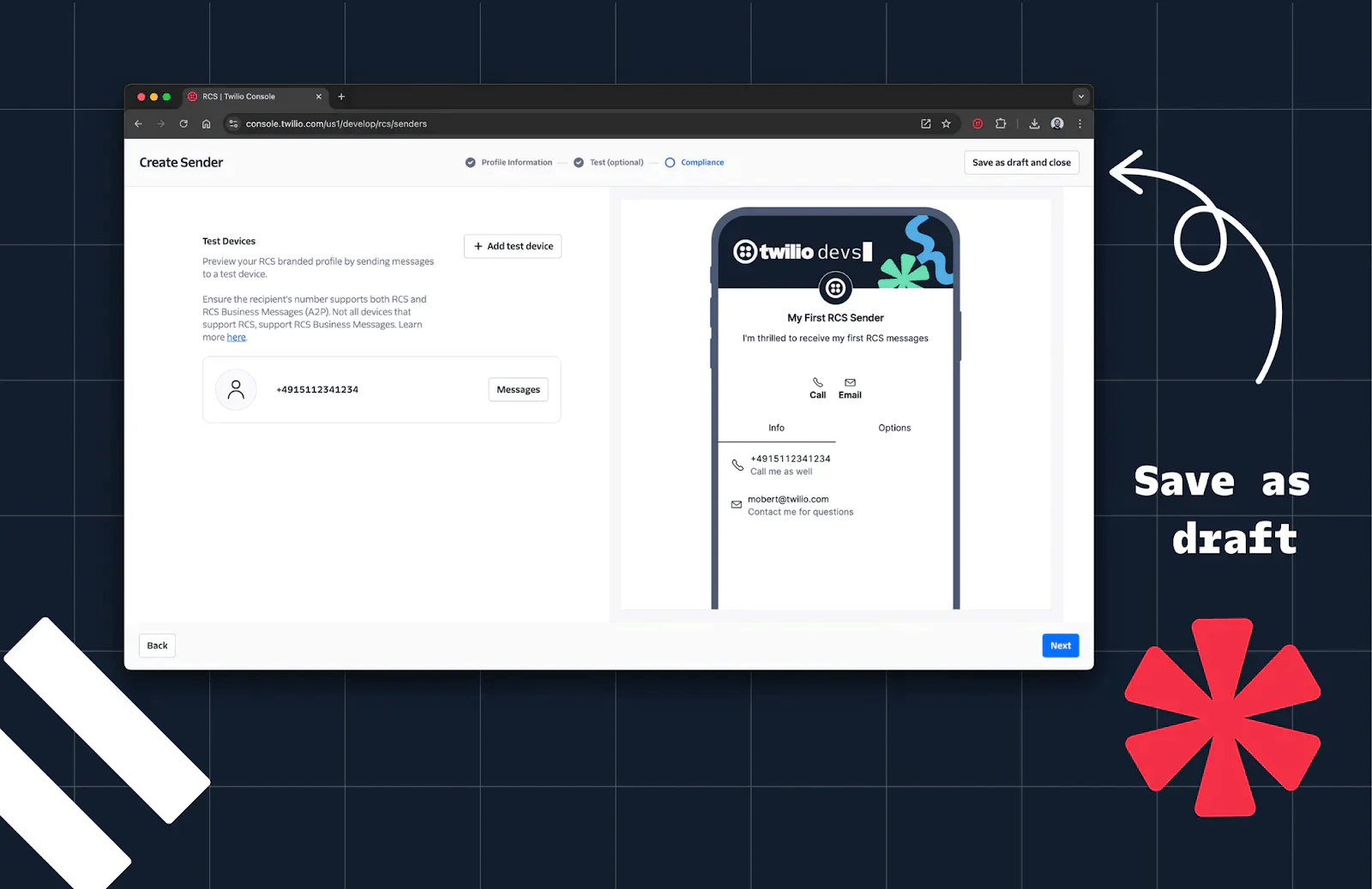
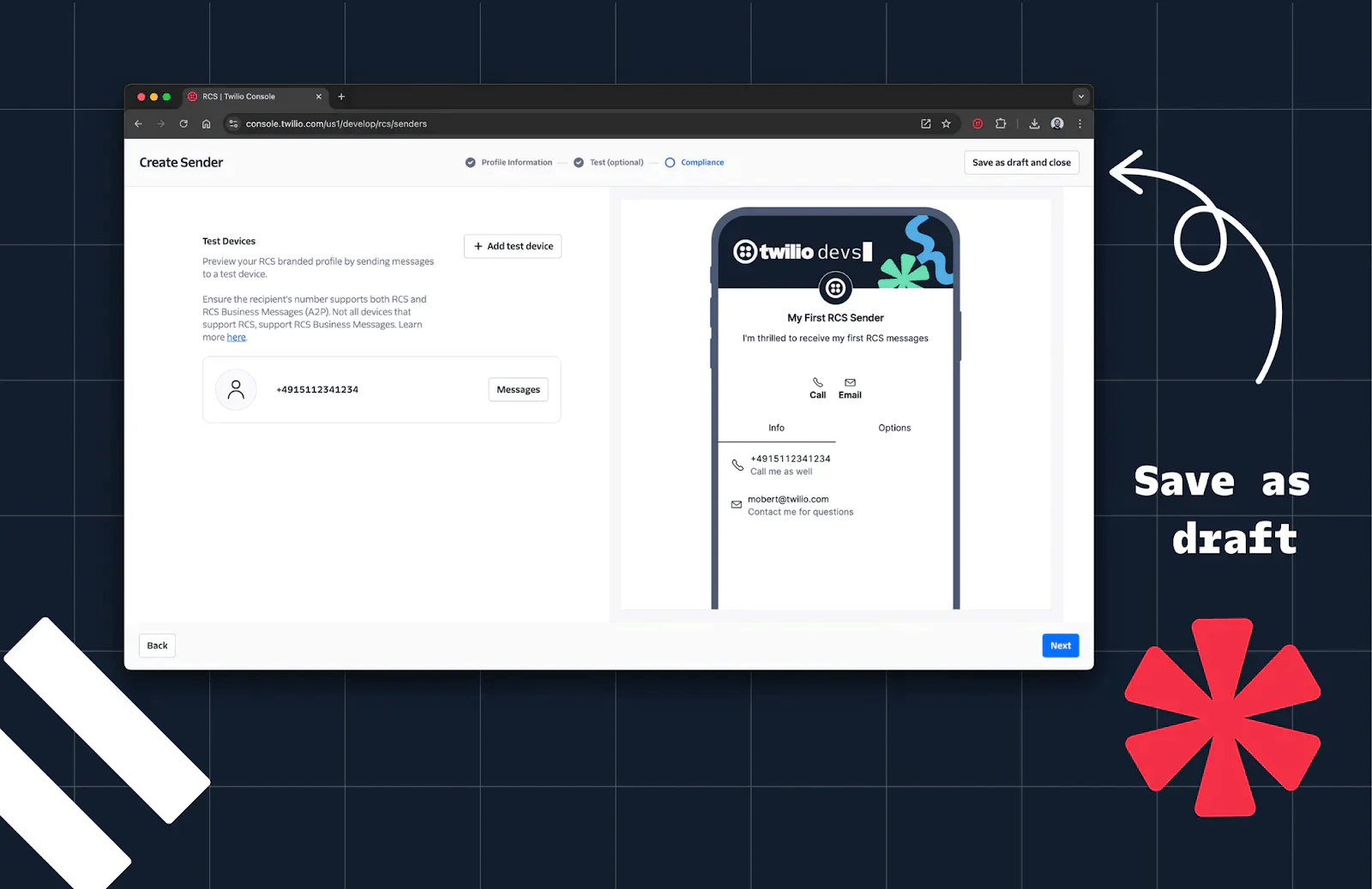
Step 4: Create a Messaging Service
- In the Console, navigate to Messaging > Services.
- Click Create Messaging Service.
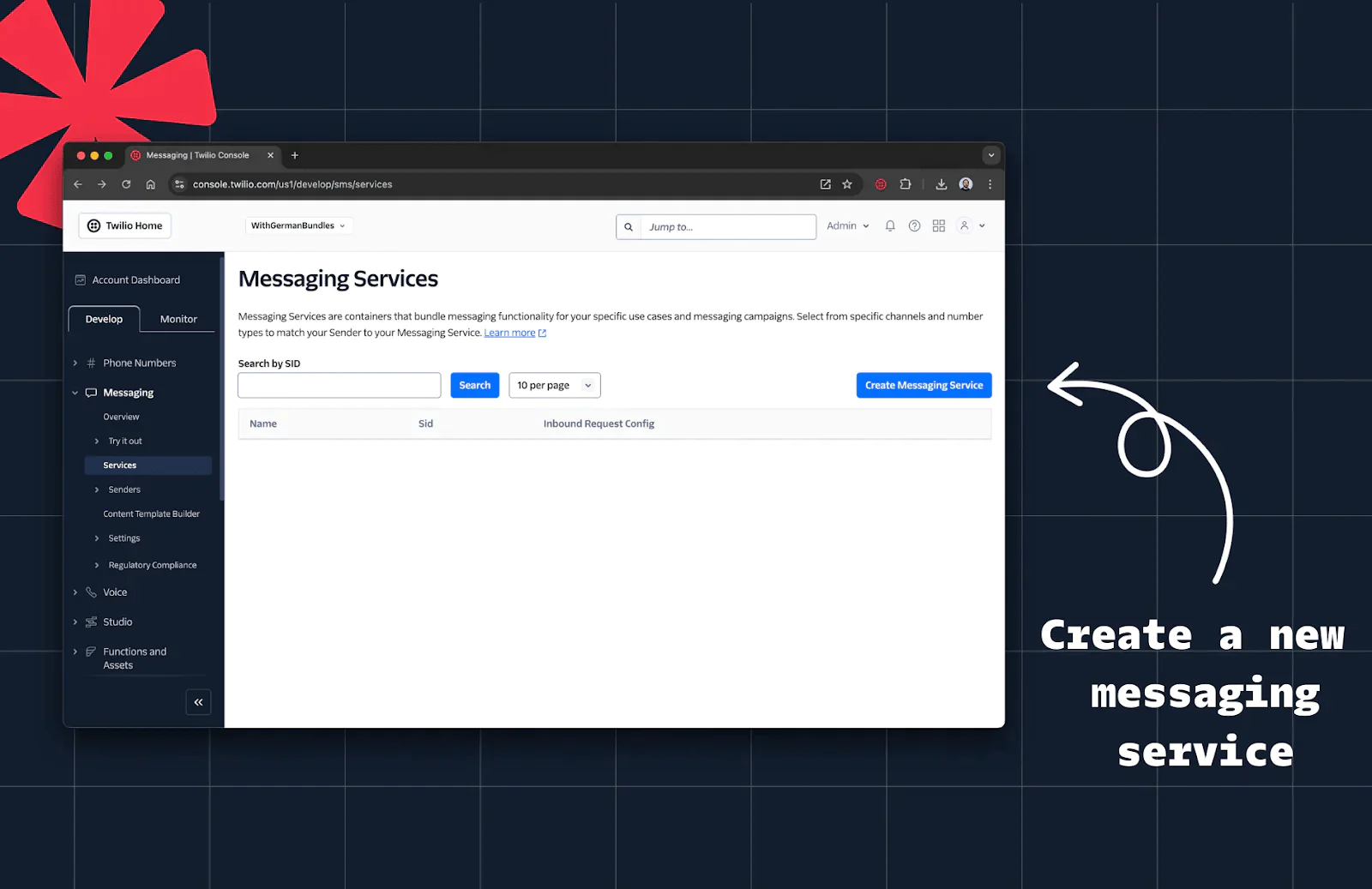
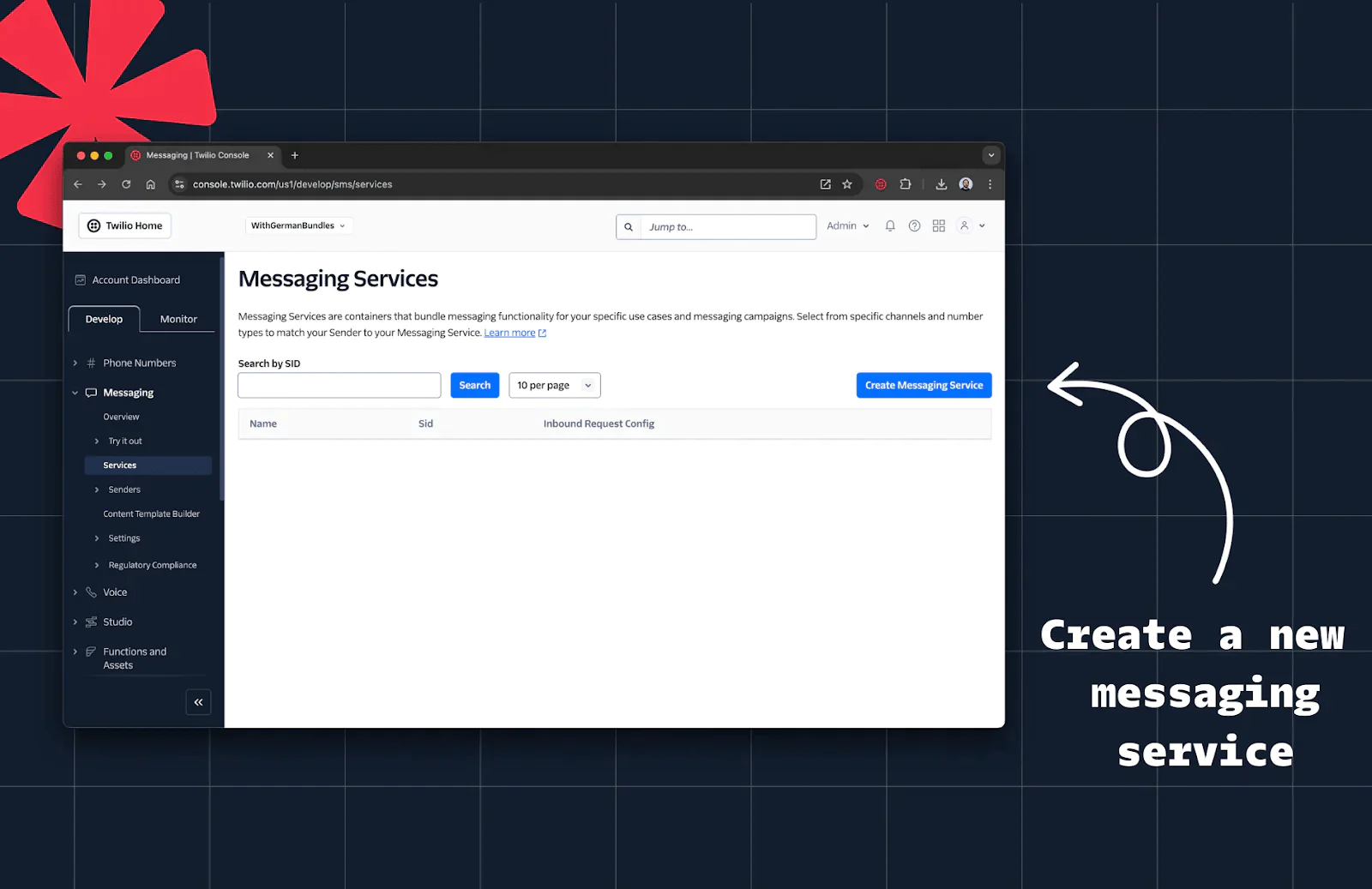
3. Give the new service a name (for example: “My RCS Service”) and click Create Messaging Service.
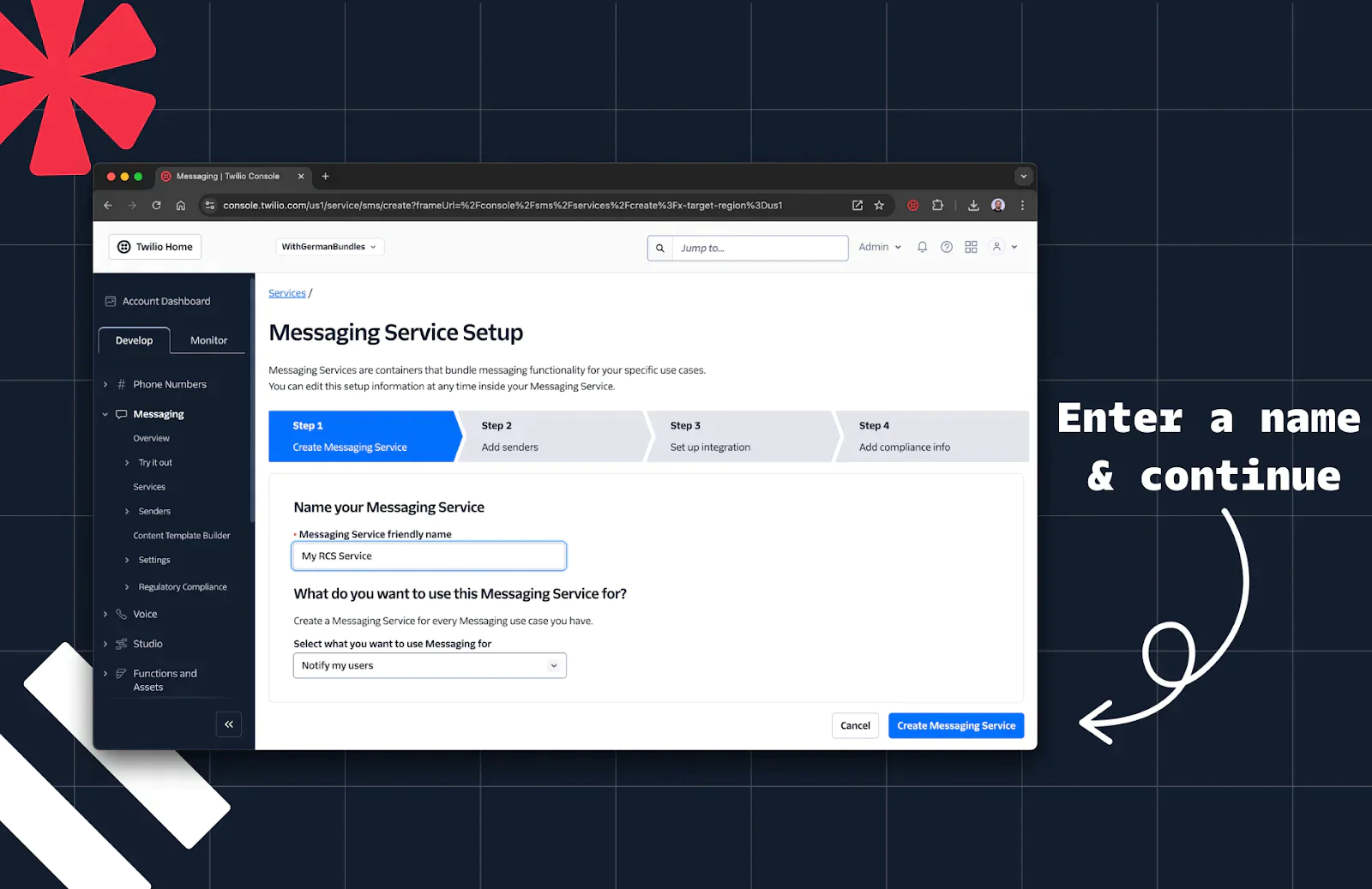
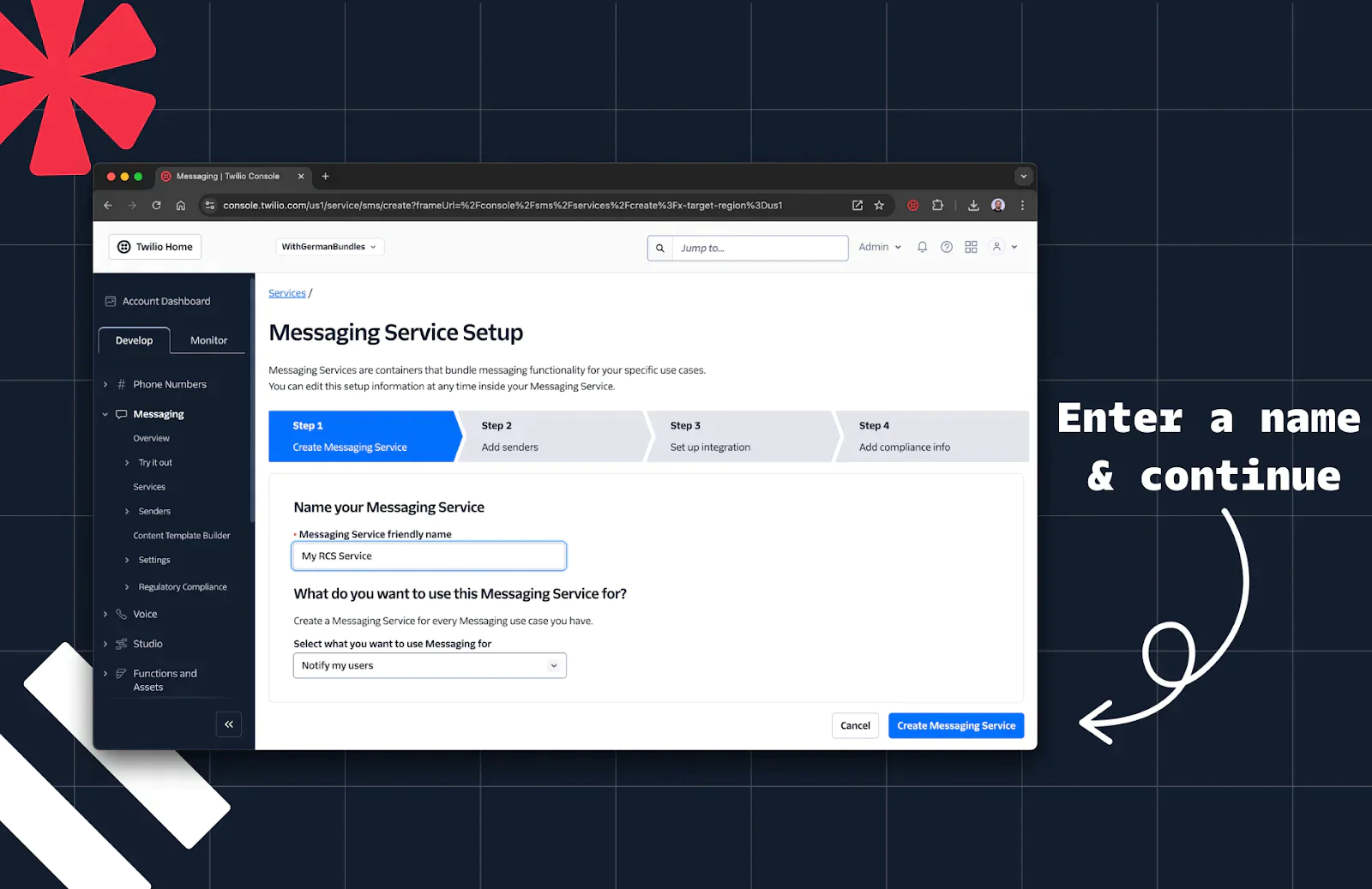
4. Add your RCS sender.
5. Click Add Sender, select RCS Sender.
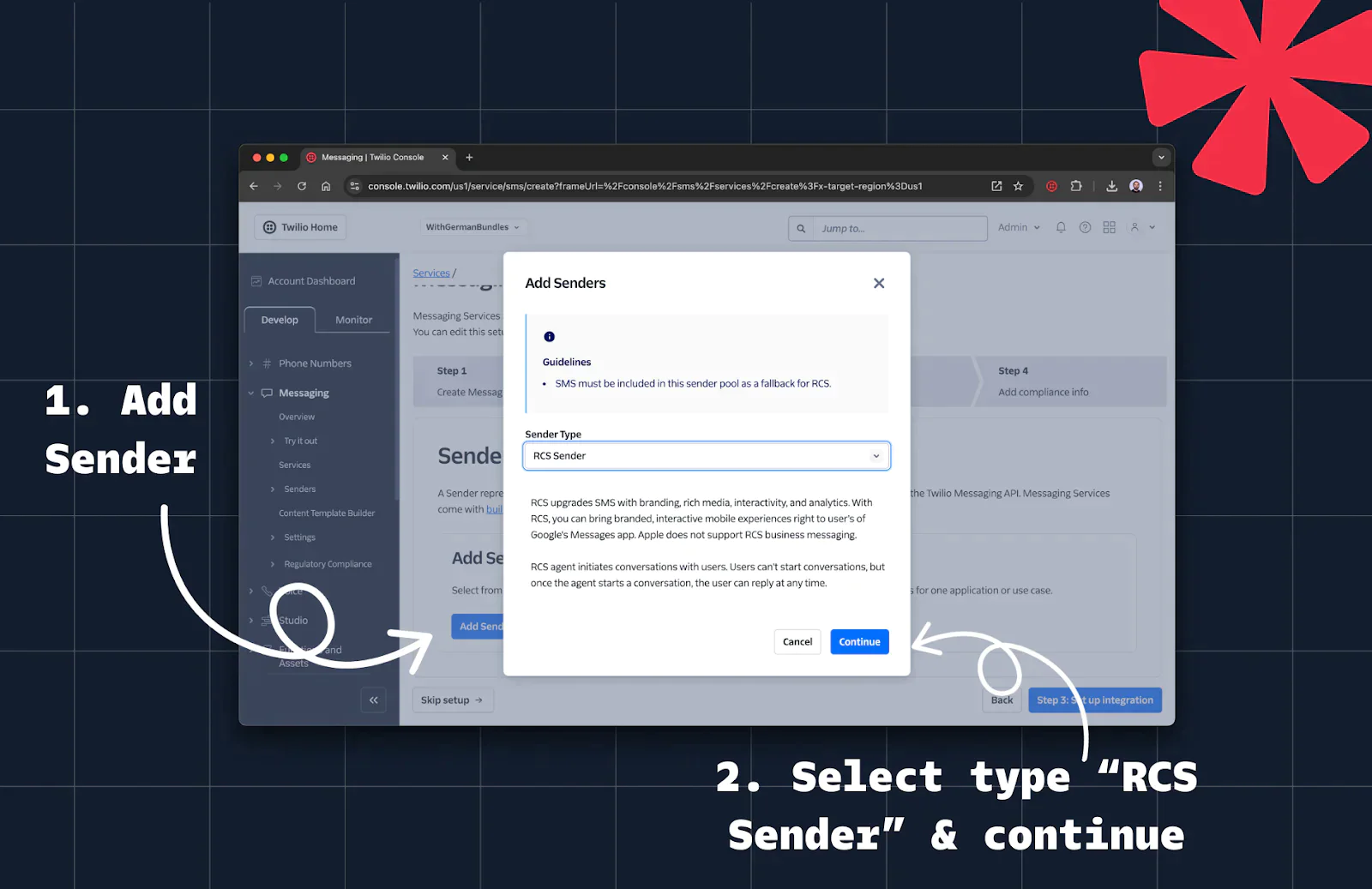
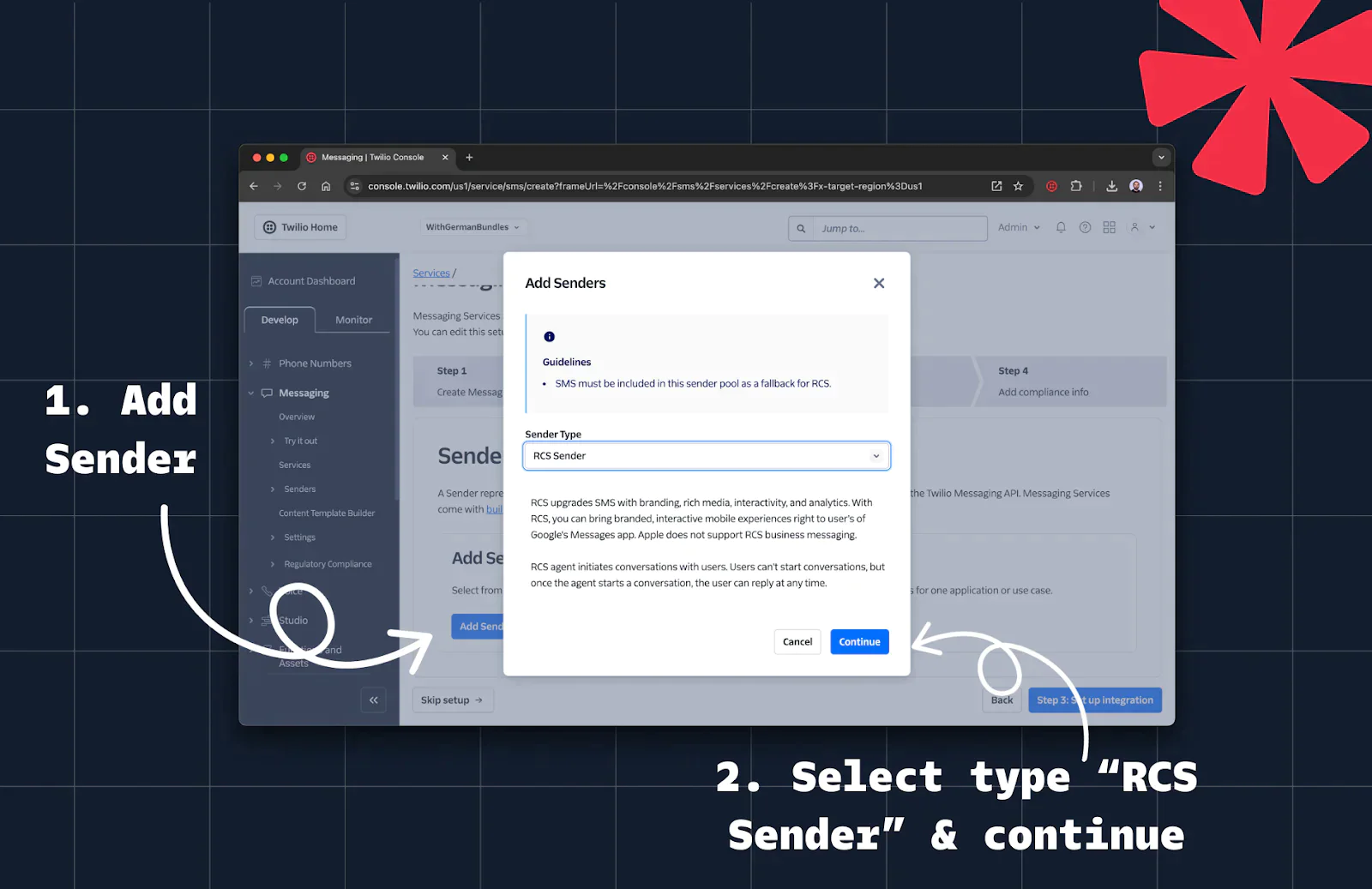
6. Add the RCS sender you just created.
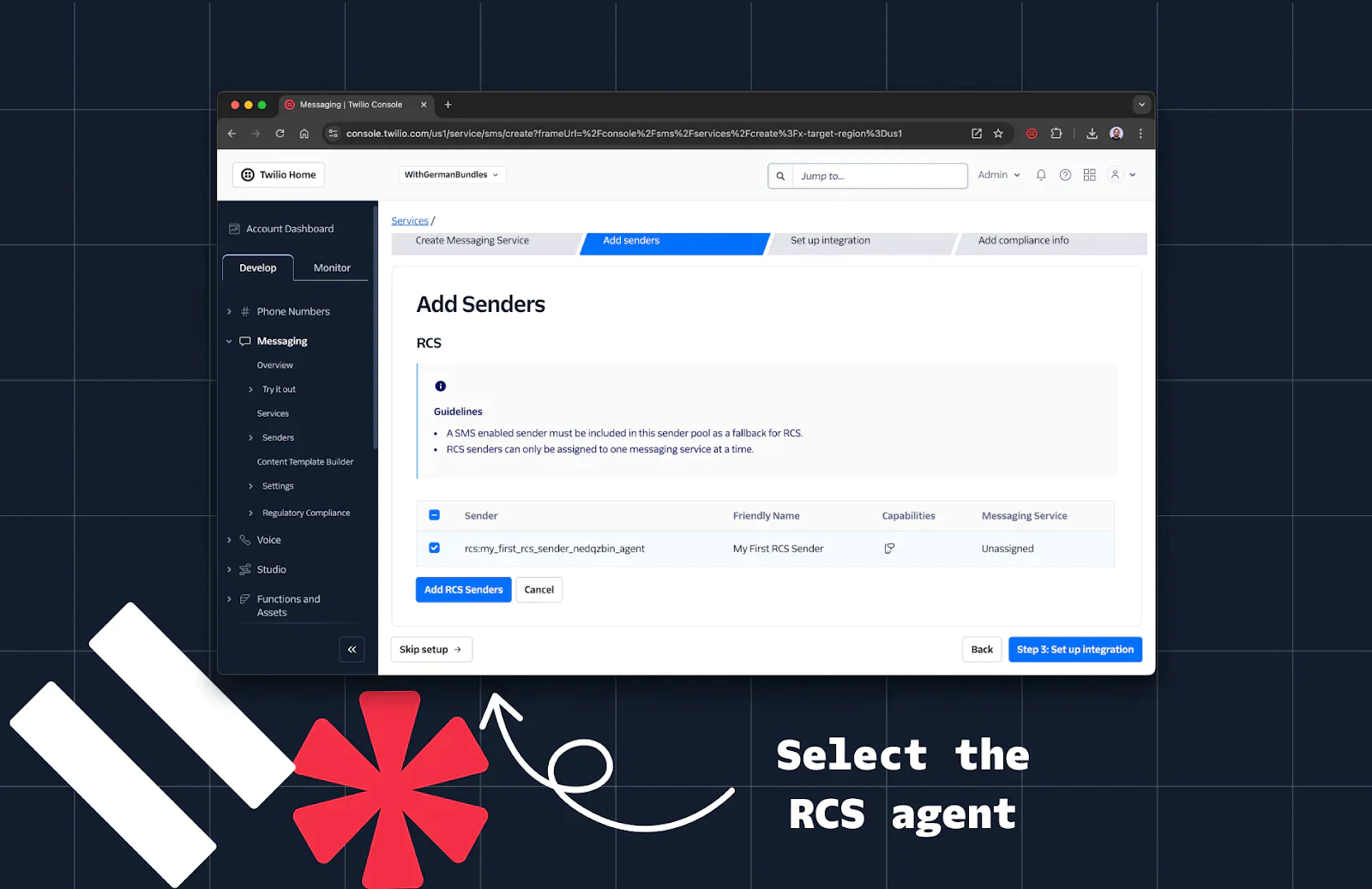
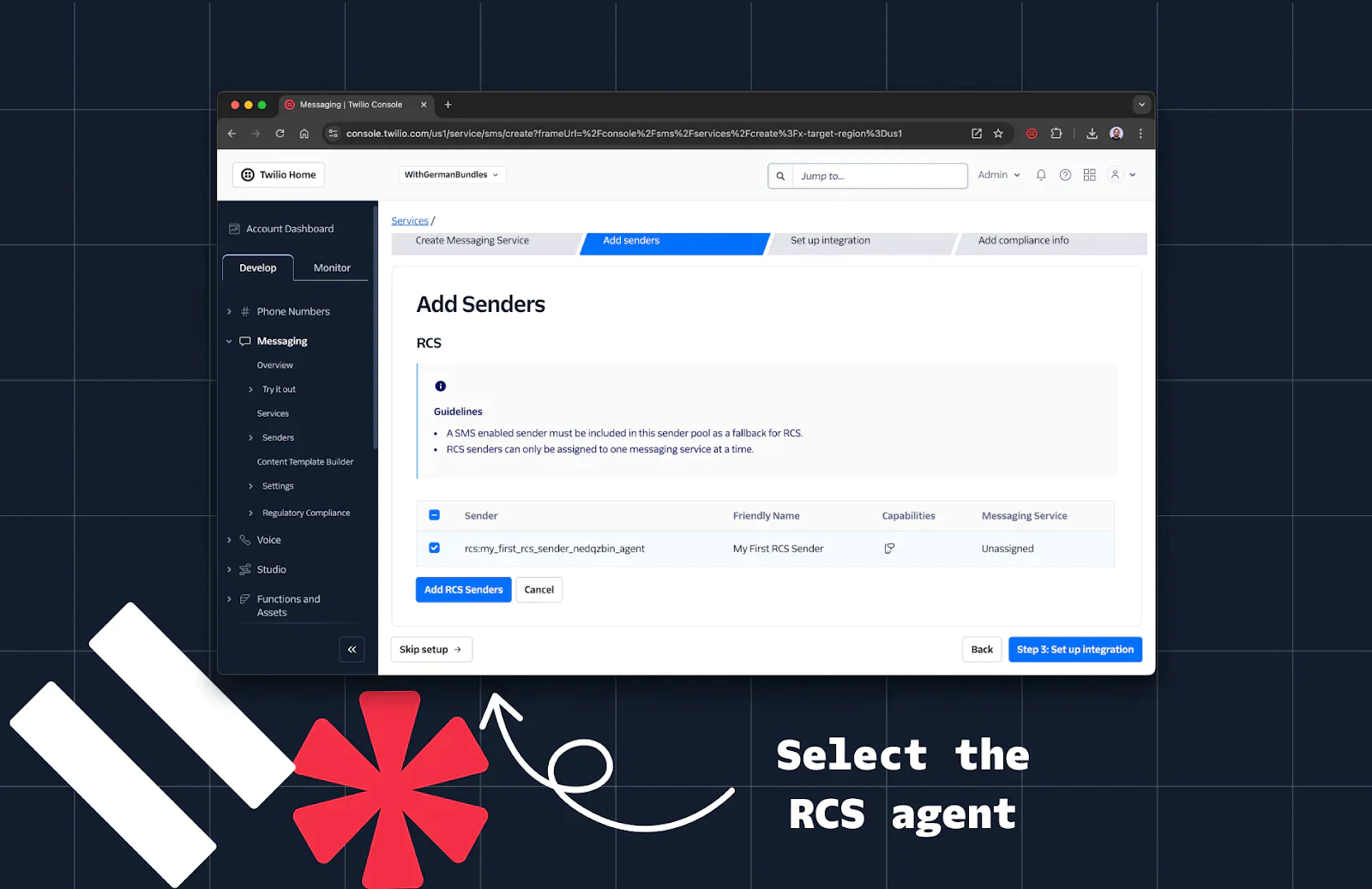
7. Add an SMS fallback sender by repeating the Add Sender process and selecting a phone number (or other SMS-capable sender). Then, click Step 3: Set up integration.
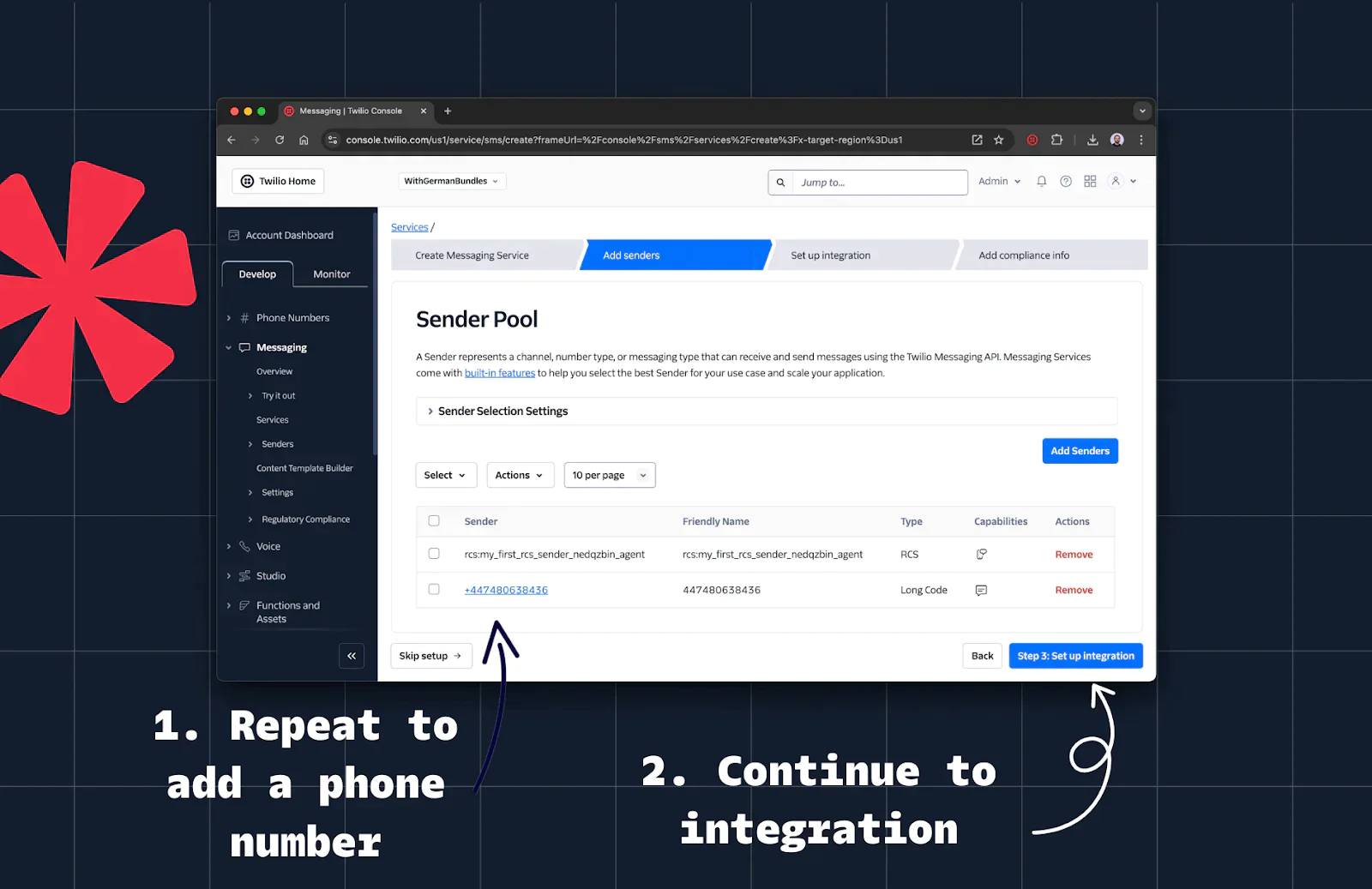
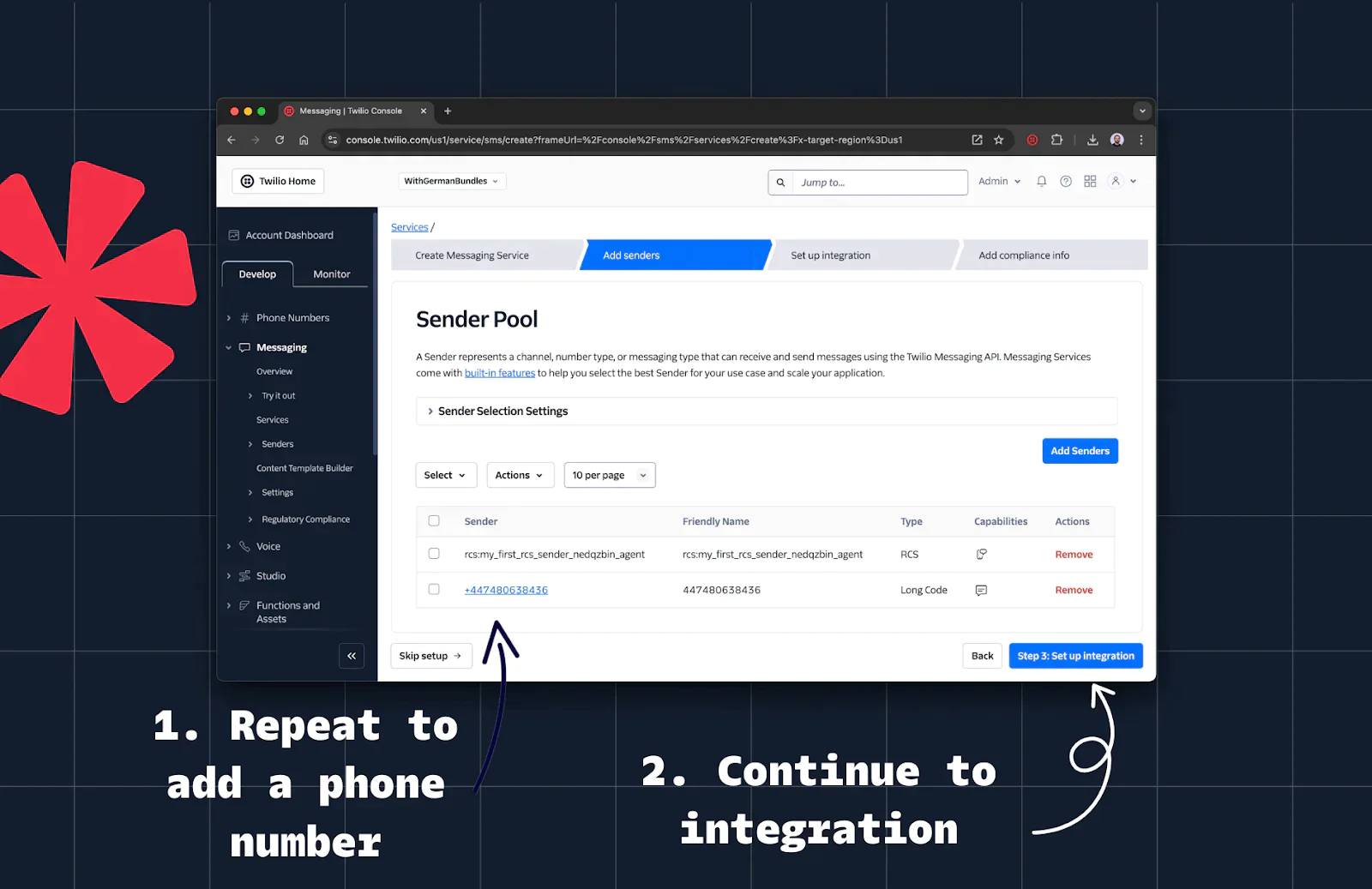
Step 5: (Optional) Set Up Webhook Integration
- Webhooks allow you to handle incoming messages in the same way as SMS or WhatsApp. Learn more in Twilio's docs about Incoming Message Webhooks.
- Use the Set Up Integration option to register a webhook. Feel free to reuse an existing one if available.
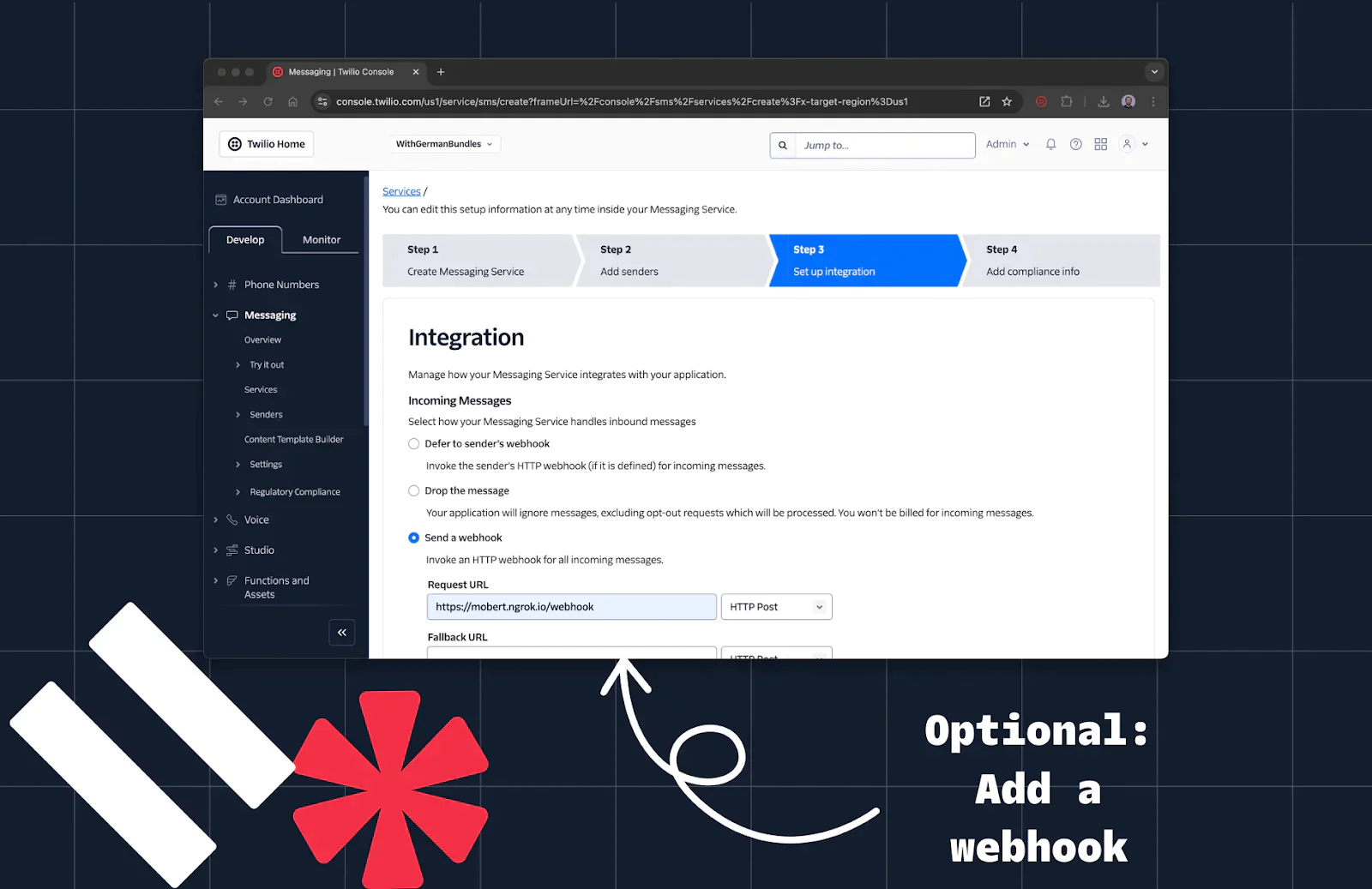
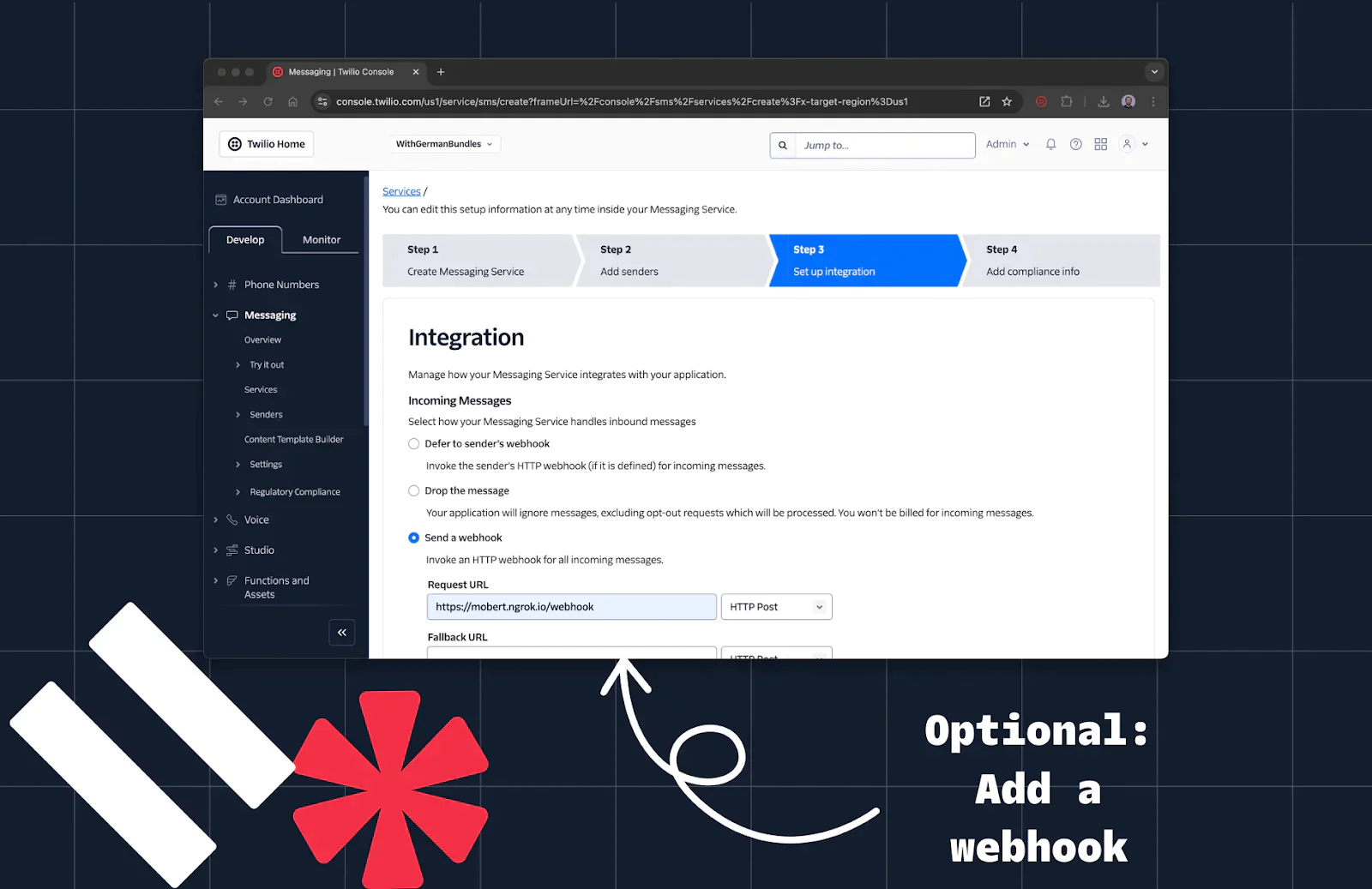
Step 6: Send Your First RCS Message with Python
- Finish the wizard flow by clicking the white Skip setup button.
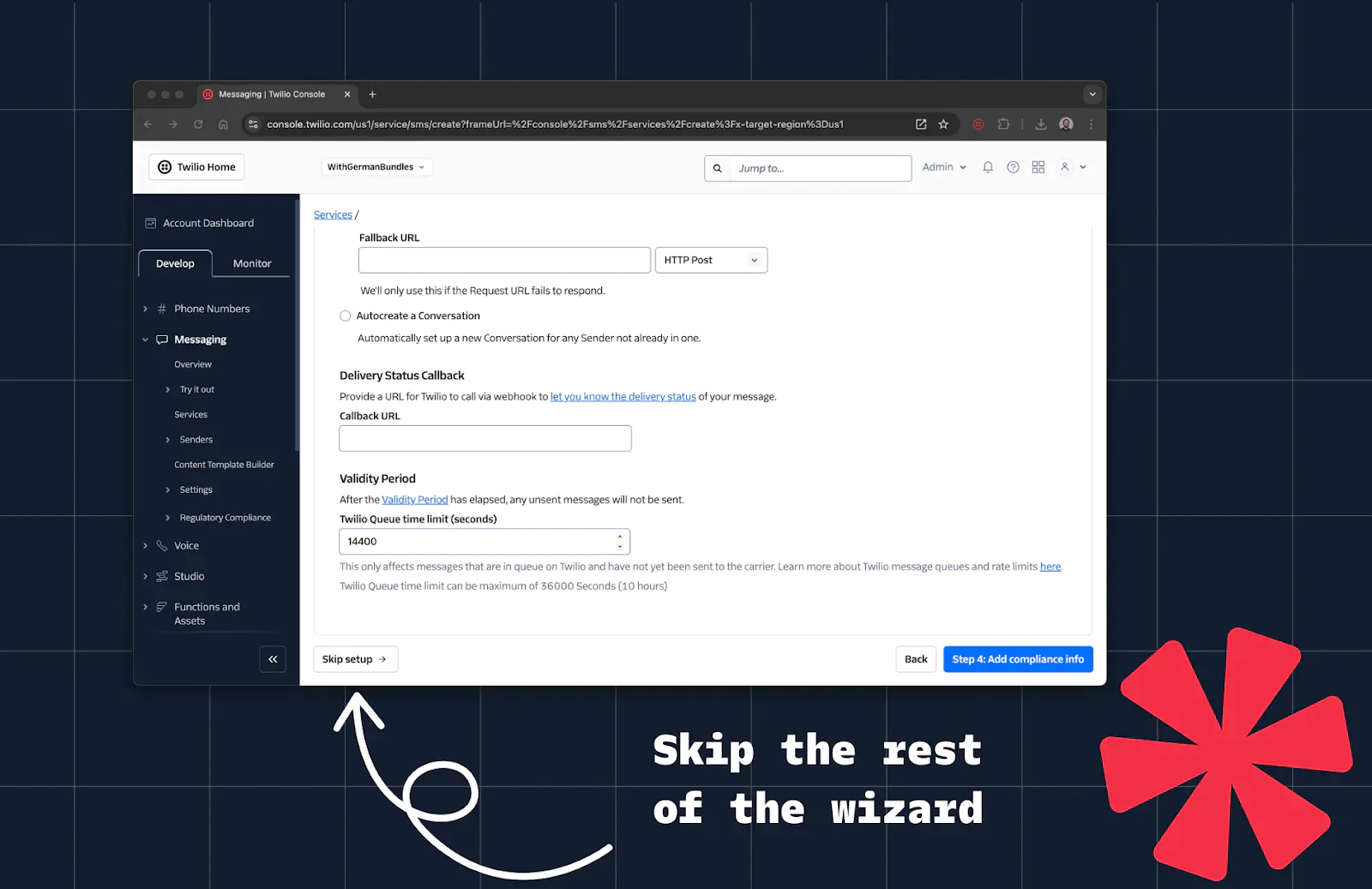
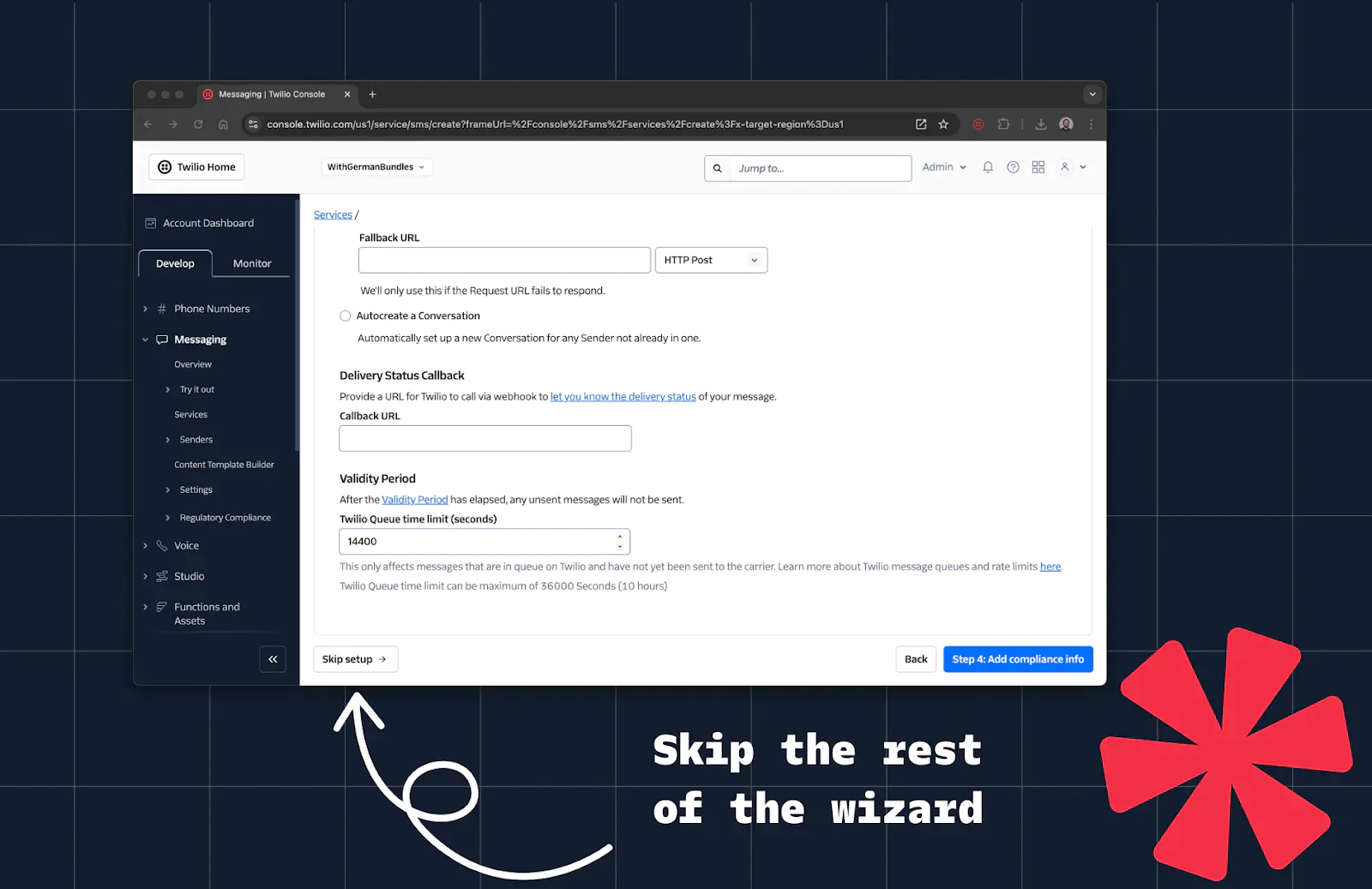
2. Copy the Messaging Service SID (e.g., MGe1fdfb207e9aa5b44f398b1094d88a8b
) from the Console.
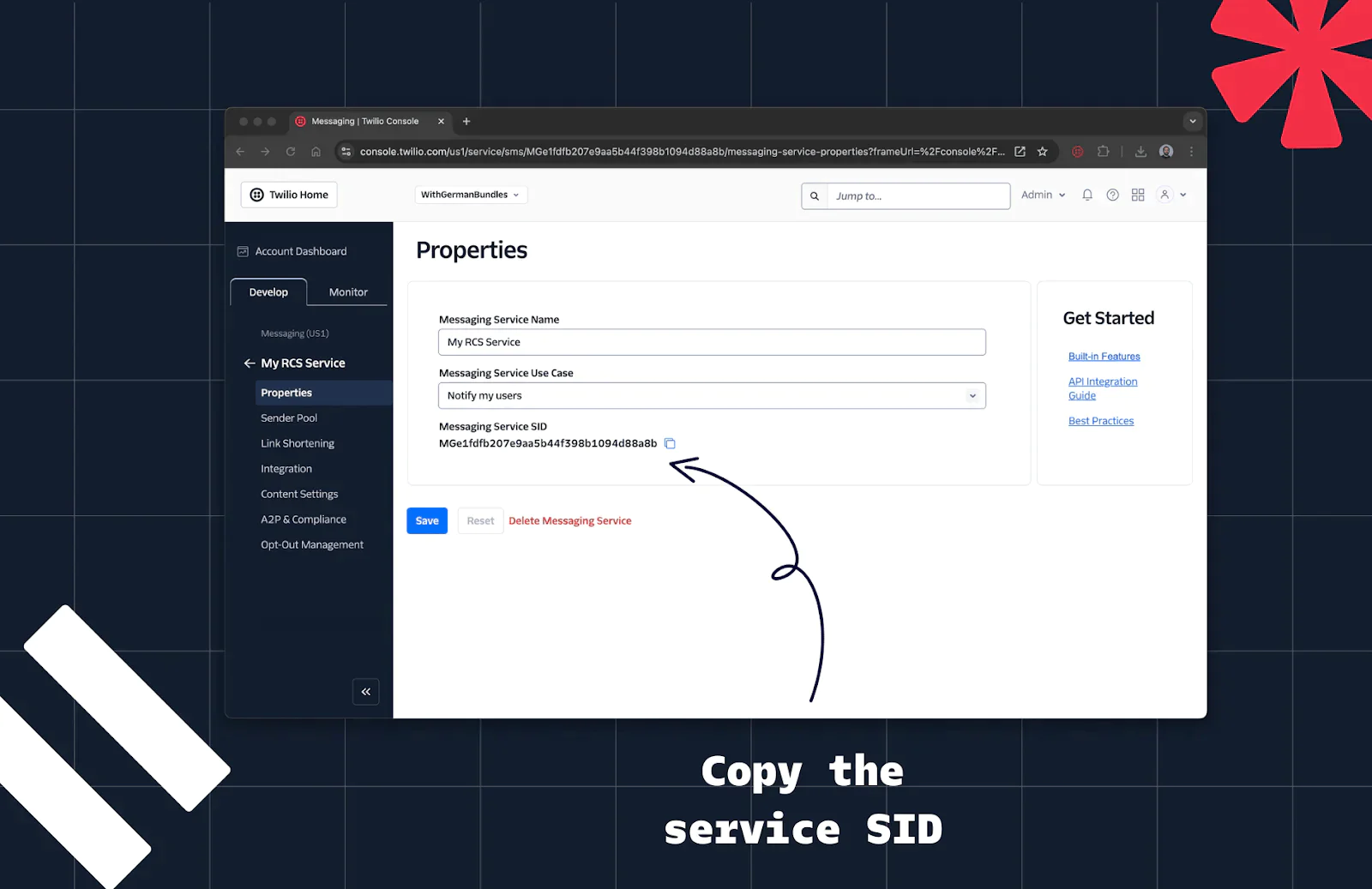
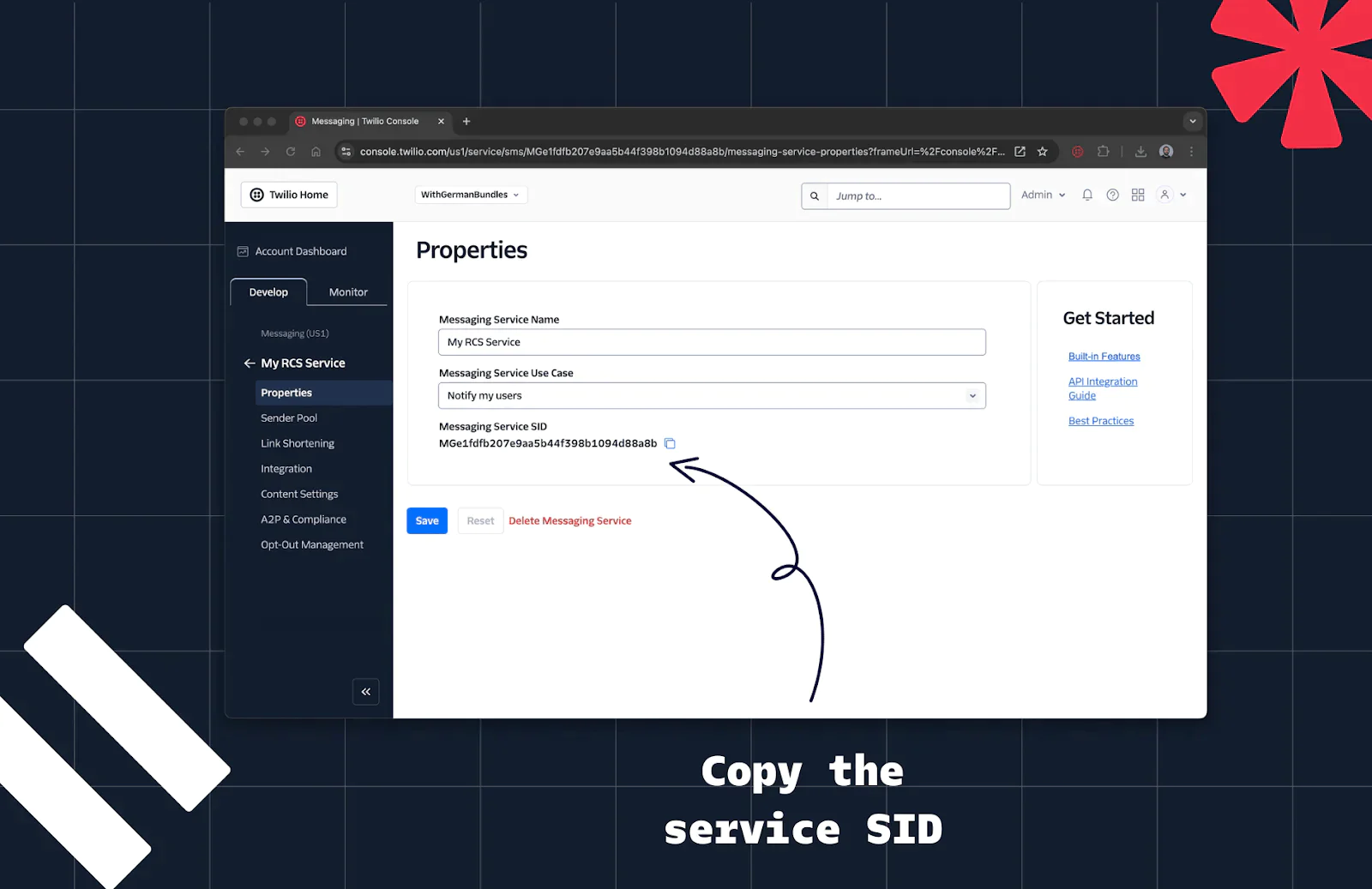
3. Retrieve your Account SID and Auth Token from the Twilio Console.
4. Create a new folder and within that folder create a file .env
. Add the credentials from the previous steps, so that your file looks like this:
5. Create a virtual environment and install the required dependencies:
6. Create a new file named send_rcs.py
with the following code:
7. Run the script using python send_rcs.py
and see the result on your phone.
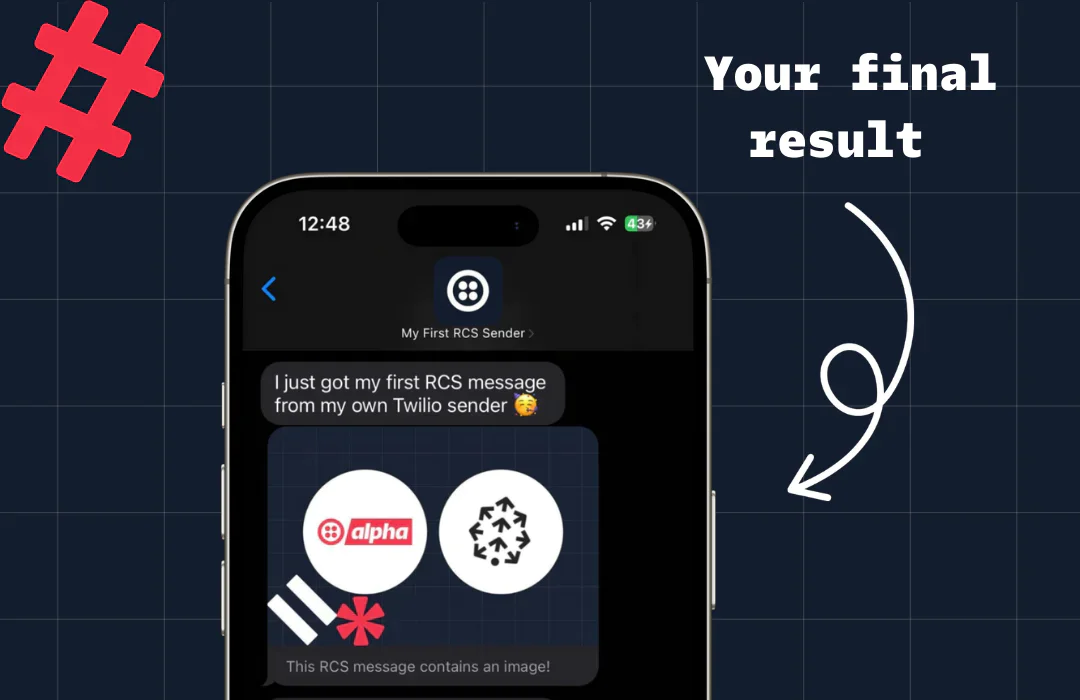
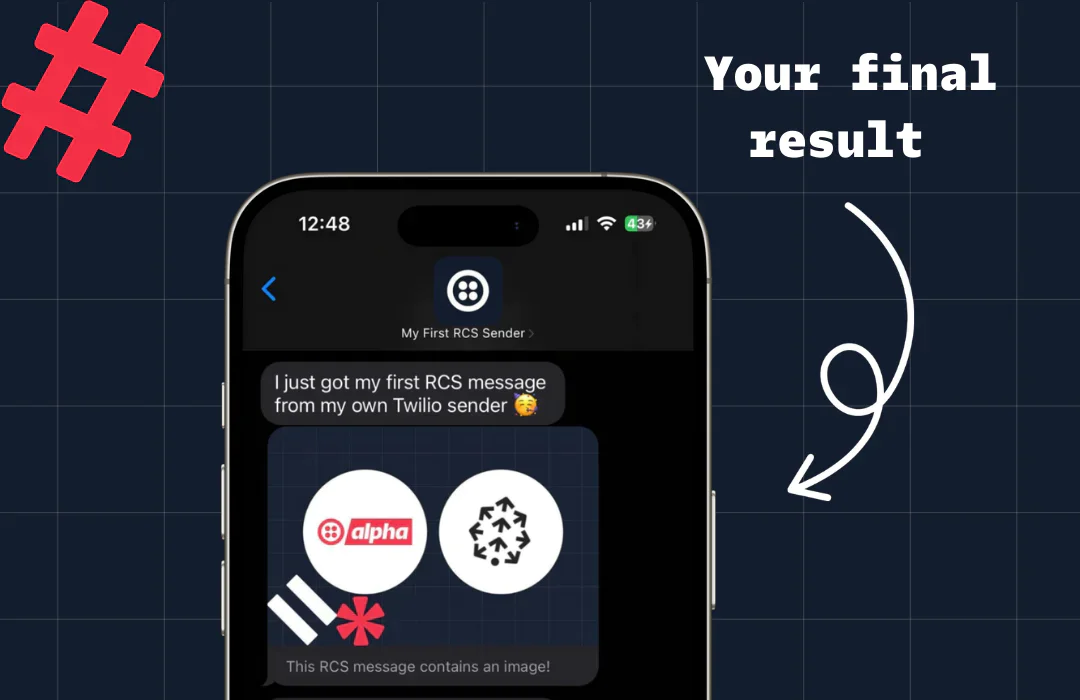
As you transition from sandbox testing to production usage, you’ll need to pass the country-specific verification process we skipped above. This requirement ensures compliance with local regulations and protects users from potential messaging spam. While this might introduce initial friction, it guarantees that messages from reputable sources maintain their integrity, significantly reducing spam and increasing user trust. Twilio and other carriers around the world are committed to fighting spam and avoiding the problems that were caused by the permissionless nature of SMS. For further guidance, check out Twilio's RCS Documentation.
Explore Advanced RCS Features
Congratulations on sending your first RCS message with Twilio and Python! Now, let's explore some features that set RCS apart from traditional SMS:
- Extend your script to use the Content Template Builder for rich message content.
- Explore the Programmable Messaging Logs to check if messages are read and troubleshoot any errors.
- Need a reliable way to send OTP codes with RCS? Twilio Verify makes it easy to deliver secure and engaging One-Time Passwords to your users.
RCS is transforming how businesses communicate with customers, blending the reliability of SMS with the interactivity of modern messaging apps. With Twilio, you can harness the power of RCS to create engaging and rich communication experiences from your applications.
We can’t wait to see what you build!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.