Enhancing WordPress Comments Using Twilio and WebServices
Time to read: 9 minutes
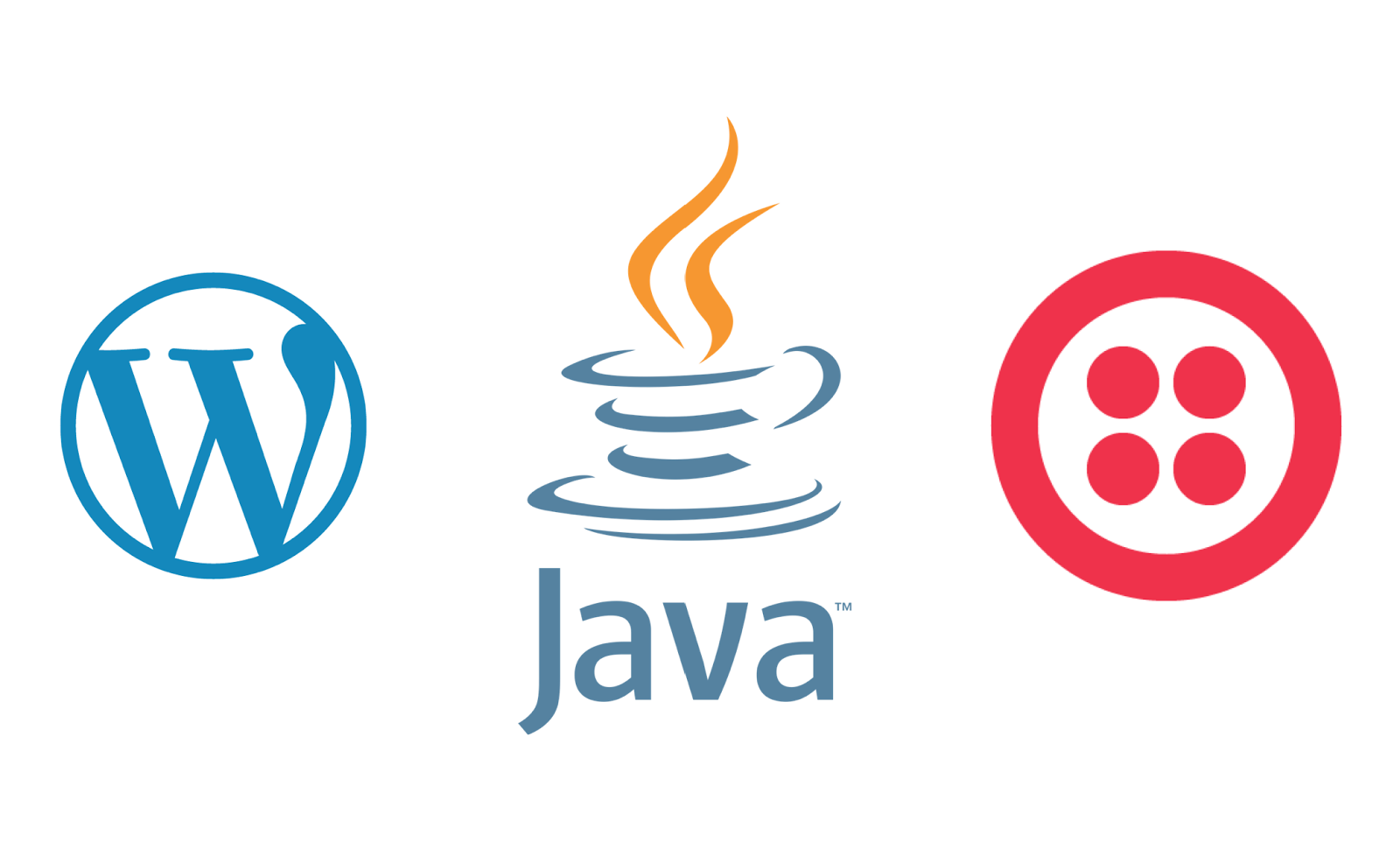
Getting users to interact with your blog is essential. It’s also easy using Twilio. In this post you will learn how to write a WordPress plugin that will send your users a text message when someone replies to their comment. If you want to take it a step further you can enable the user to comment on the post via a text message by building a Java REST webservice.
Plugin Setup
You are going to need a self-hosted WordPress site so you can create and install a plugin. You will also need to download the Twilio SDK and set up a Twilio number.
Plugin Development
We are going to create a plugin in its entirety outside of WordPress and then install and activate it. First, create a directory in your development file system called sms-twilio-comment-notifier
and in that directory create sms-twilio-comment-notifier.php
. This is your main plugin file. You need to add metadata to define this class as your plugin file and the code will come after that.
Next, tell the plugin where to find the Twilio SDK you downloaded earlier. Unzip the SDK into the folder and add the following to sms-twilio-comment-notifier.php
:
Now we can access the Twilio API. In order to authenticate to Twilio, we need to know a few key elements. We will store them in a custom database table. When we activate the plugin it will check to see if the table is there, and if not, it will create it. The following code will be run when the plugin is activated to create a table that will store the Account SID, Auth Token, and Twilio number you set up earlier, again in sms-twilio-comment-notifier.php
:
We are going to need a way to clean up the table if it is deleted, so let’s take care of that now.
Create a file called uninstall.php
and add the following:
This class will get called when the plugin is uninstalled and will drop the table from the database and delete the database version option from WordPress.
How does data get into our new table? We need to create an admin page for that. Add the following to sms-twilio-comment-notifier.php
:
We are adding a menu page where only users with the edit_pages
role can see the page. Now let’s put something on the page. Keep it simple and only allow one row of the table so we have a very basic form to collect our three pieces of data. Register an action to capture the form submission and grab the contents from the table so we can prepopulate the fields with the current values from the table. Set a nonce
field to verify the form submission. Add the following to sms-twilio-comment-notifier.php
:
Here is the completed page:
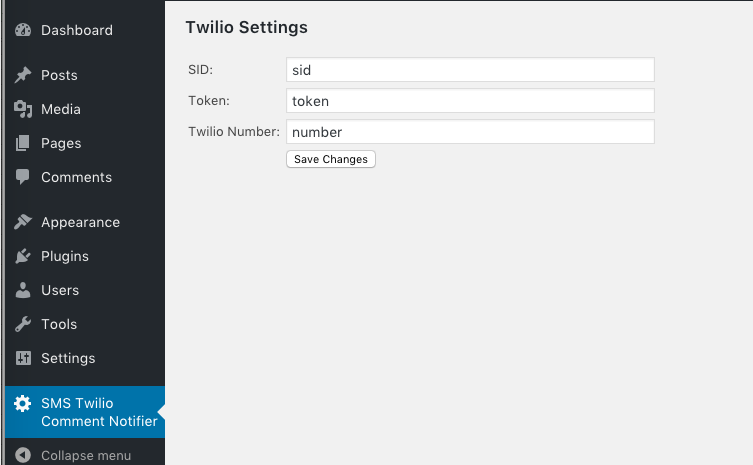
Once the user saves their Twilio account values, persist them to the table. First we’ll verify the nonce
to make sure the request has not been spoofed. We need to sanitize the inputs for security, then we can store them in our table and refresh the page. Add the form below to sms-twilio-comment-notifier.php
:
Now that we have that setup done so we can talk to Twilio, we need to be able to store the user’s mobile number. Add a field to the User Profile page so the user can enter their number and save the fields to the user metadata. Still in sms-twilio-comment-notifier.php
, add:
This is what your profile page will have now:

We are almost there! We can authenticate to Twilio and we have a number we can send a message to. We need to add some hooks into the comment functions. When a comment is published or updated, first check to see if this comment is a reply to another comment. If it is, make sure the user is not replying to themselves. If they’re replying to someone else, send the user of that parent comment a notification. Add the following to sms-twilio-comment-notifier.php
:
Time for the fun part – using Twilio to send the message. First, get the Twilio authentication fields from the table we created, then get the mobile number from the parent comment to send that user the message. We’ll send a message including the link to the comment to make it a useful message. You could stop here and have yourself a nifty little notification plugin.
Comment Notifications via SMS
If you want to take it the extra mile, we’re going to let the user reply to that text message and post the comment to the blog on behalf of that user. In sms-twilio-comment-notifier.php
add:
If you don’t want to let your user reply to the text in order to post a comment, just change the body message.
If you do want to let the user text a comment to the post, it gets a little tricky. WordPress finally has a REST API where you can interact using REST web services, including making posts or comments. However, in order to post a comment as a user you have to authenticate as that user. In order to do that, you would need to have OAuth or something similar set up to allow the user to use a token to authenticate to WordPress. Complicating matters, WordPress does not have out of the box support for OAuth. There are plugins to help but the whole point of replying via a text message is to get in and get out without having to jump through too many hoops. Otherwise, you could just use the WordPress app or log in to a browser. To offer a fast way to comment on a blog post, we are going to set up a “tweener” service that will post anonymously to WordPress on behalf of your user. Since the user isn’t logging in and posting the comment themselves, we will append the user’s display name to the end of the comment so it is clear who wrote the comment.
To do this, enable anonymous commenting. Add this filter to sms-twilio-comment-notifier.php
:
Now your plugin is complete. Zip up your plugin. You can download the example on GitHub sans the Twilio SDK. Go to the plugins screen in your WordPress admin panel, and click Add New.
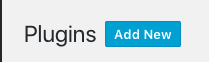
Click Upload Plugin.
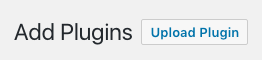
Upload your zipped up plugin file and Activate it.
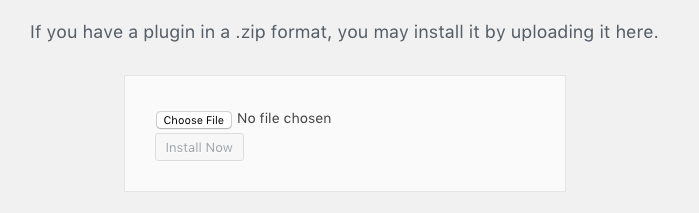
All done!
This is what your text might look like, if someone replies to your comment:
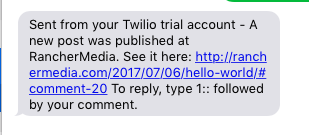
Now, how about that reply feature?
Enter Java
For the next piece you are going to need to be able to install a Java webservice on an application server. You can install one locally and use ngrok to test until you are ready to publish it to the world.
If you don’t already have a Java development environment set up, download Eclipse and Tomcat and unzip them.
Create a new Dynamic Web Project in Eclipse, and name it SMSNotify
. Create a package structure – I use com.company.sms.notify
and your SMSNotify.java
class in the package. To speed things up, create a dao
subpackage and an SMSNotifyDAO.java
class in that package. We’ll get to them in a bit.
We are going to use RESTEasy to expose our REST web services, but you could use your favorite framework instead.
What we need is a webhook in Twilio that will call your webservice any time a message is sent to your Twilio number. The webservice will try to parse out the post id to reply to, make a call to the WordPress database to grab the user meta data, and then submit a POST to the WordPress REST API to actually post the comment.
In your web.xml
you will need to set up RESTEasy by adding the following right before your closing tag.
This will let any class be scanned by RESTEasy to be used to surface a REST endpoint which only accepts a POST call.
First we will set up the web service the Twilio webhook is going to POST to.
Set up your @Path
at the top of your SMSNotify.java
class, and annotate a function to receive the POST
.
This just replies with a 200 OK message.
Install your webservice on tomcat, and use ngrok to surface your local environment publicly.
Set up your webhook in Twilio:

Now whenever someone texts your Twilio number, it will send the message to that web service.
Twilio sends messages in a specific format so we need to parse out the information sent in order to understand the message. We’ll set some constants that we can use to parse the String. We want to verify the incoming message so we’ll need to grab our Twilio credentials from our WordPress table as well. Prepare for that by creating constants for those calls:
This is the format of the message we get from Twilio:
The keys that we need are the AccountSid, Body, To, and From. We are going to make sure the AccountSid
and To
match our Twilio SID and Twilio Number stored in WordPress earlier. So, we need a DAO class to grab them.
In your DAO class, create final Strings to contain your SQL statements. We are using prepared statements and immutable Strings to prevent SQL injection attacks.
Notice we are calling the same table our plugin created earlier and retrieving the values from there. We also connect to the users and usermeta tables in WordPress to match up the mobile number the Twilio webhook is sending us to the user name who texted it. When we post the message we can append the user name so the post is not truly anonymous.
Depending on your webhost you might need to add the IP address of your Tomcat server to allow an outside server to connect to the hosted MySQL server.
We’ll need to set up a connection to the database. You can set up a datasource in Tomcat, but since this is just sample code, connect manually and don’t worry about connection pooling, which you would want to do in a production environment.
Now that we have a connection to the database, we can call to get our credentials.
Notice the close call in the finally method. We need to make sure we close all of our connections.
While we’re here, we can code our method to get the user’s name from their mobile number.
Let’s recap. We have a web service endpoint and a DAO class that can read all the data we need in the webservice. Time to dive in and finish out the web service.
We already created the constants needed for our parsing so we can fill out the method.
Get your credentials from the DAO method we just made. If we can’t find them, we need to error out since we won’t be able to validate the incoming message.
If we made it this far, the credentials were found. Make sure the Account SID posted to the web service matches your Twilio Account SID. We will parse the String sent to the webservice and find the first time we see AccountSid=
. Grab everything between that location in the String and the first time after that we see the &
. If it doesn’t match our Account SID, bail out with an Unauthorized message.
Do basically the same thing with the Twilio number. When the message is sent to us, the text is html encoded. We could use some third party utils to unencode the message but it’s just as easy to replace the text as we do here, changing the %2B
to +
.
Now that the message is validated, we need to grab the mobile number of the user who sent it. Again we are going to replace the encoded %2B with a +.
Hang on to this and we’ll get back to it in a minute.
Get the text message the user sent and parse out the post id from it. We told the user to type <postId>::
. The ::
were encoded when they were sent to us so we have to stick the colons back in order to parse our message:
We have just about everything we need to post the comment so add this at the end of your class:
Now let’s build that method so the call will work. Take the mobile number and call the method in our DAO that returns the user’s name and build up our new message. In our plugin we told the user to reply with <postId>::
. If they did that, we can get the post id from the message. We parse out the number and trim the string so the <postId>::
doesn’t actually appear in the comment.
The message comes to us with plus signs as spaces so quick replace all to change them to spaces so they don’t show up in WordPress looking like This+is+my+reply
. Now, this isn’t a bullet proof approach since some users might actually put a plus sign in their text but it will work for now.
The last step is to call the WordPress REST API to post the message. We need the URL to send it to. You can store this in a local table somewhere, but to speed things up a bit I’m hard coding it in the DAO since it won’t likely change any time soon:
Let’s make the call to the API. We have the post id and the comment text, which we append as query parameters to our WordPress URL. Use RESTEasy to send the message. If there’s an error, we will return false so our webservice knows to return an error to the user, otherwise we will return a success.
Finally, return a response based on whether the postComment worked or not:
Deploy your completed webservice to Tomcat (see the full example on GitHub) and test it out!
You have now enabled two way communication between your WordPress site and your end users. A user can enter a mobile number on their profile which will allow them to receive a text message when someone replies to their comment. If they want to comment on the post again right away, they can text a reply. Your webservice will receive this text and make a comment on the same post and note that it was sent by a specific user name.
Use this as a starting point to expand your user interaction with your WordPress site!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.