Enhance Customer Engagement with Go and Twilio SMS
Time to read: 5 minutes
Enhance Customer Engagement with Go and Twilio SMS
Engaging customers effectively — especially during promotions — can significantly boost traction and drive profits for your business. Imagine having a list of loyal customers, or even new ones who have expressed interest or consented to receive updates about your latest products or special offers. How can you efficiently communicate these promotions to them?
This is where SMS marketing excels. Studies show that people rarely go anywhere without their mobile phones, making SMS a powerful channel for staying connected. So, by leveraging SMS marketing as a core part of your customer engagement strategy, you can maintain a consistent presence with your customers, driving sales, and fostering loyalty, ultimately increasing your business's bottom line.
In this tutorial, we will build a marketing campaign service in Go that utilizes Twilio’s Programmable Messaging API to send targeted, promotional SMS messages to customers. We will set up a Go-based backend, integrate it with Twilio to send personalized promotional messages, and allow customers to opt out by replying with a STOP word. The end result will offer businesses an effective way to enhance customer engagement through SMS marketing, helping them connect with their audience meaningfully and profitably.
Prerequisites
You will need the following for this tutorial:
- A basic understanding of and experience with Go (version 1.22.0 or newer)
- A Twilio account (free or paid). Sign up for a free trial account, if you don’t have one
- A Twilio phone number capable of sending SMS
- A phone that can send and receive SMS
- curl for testing the application
Set up the project
To begin, create a new project directory and change in to it by running the following commands:
While you are within the sms-marketing-twilio folder, create a new Go module by running the following command.
This will create a go.mod file in the root of your project which defines the module path and lists all dependencies required by your module.
Install the project's dependencies
This project will require two packages:
- Twilio's Go Helper Library: Simplifies interaction with the Twilio API
- GoDotEnv: A popular package for managing environment variables
Install them using the following command:
Next, create a .env file to store your environment variables:
Then, replace the placeholder values with the details retrieved from your Twilio Console, by following these steps:
- Log in to the Twilio Console
- Copy the details from the Account Info panel
- Replace
<YOUR_TWILIO_ACCOUNT_SID>
,<YOUR_TWILIO_AUTH_TOKEN>
, and<YOUR_TWILIO_PHONE_NUMBER>
with the corresponding values
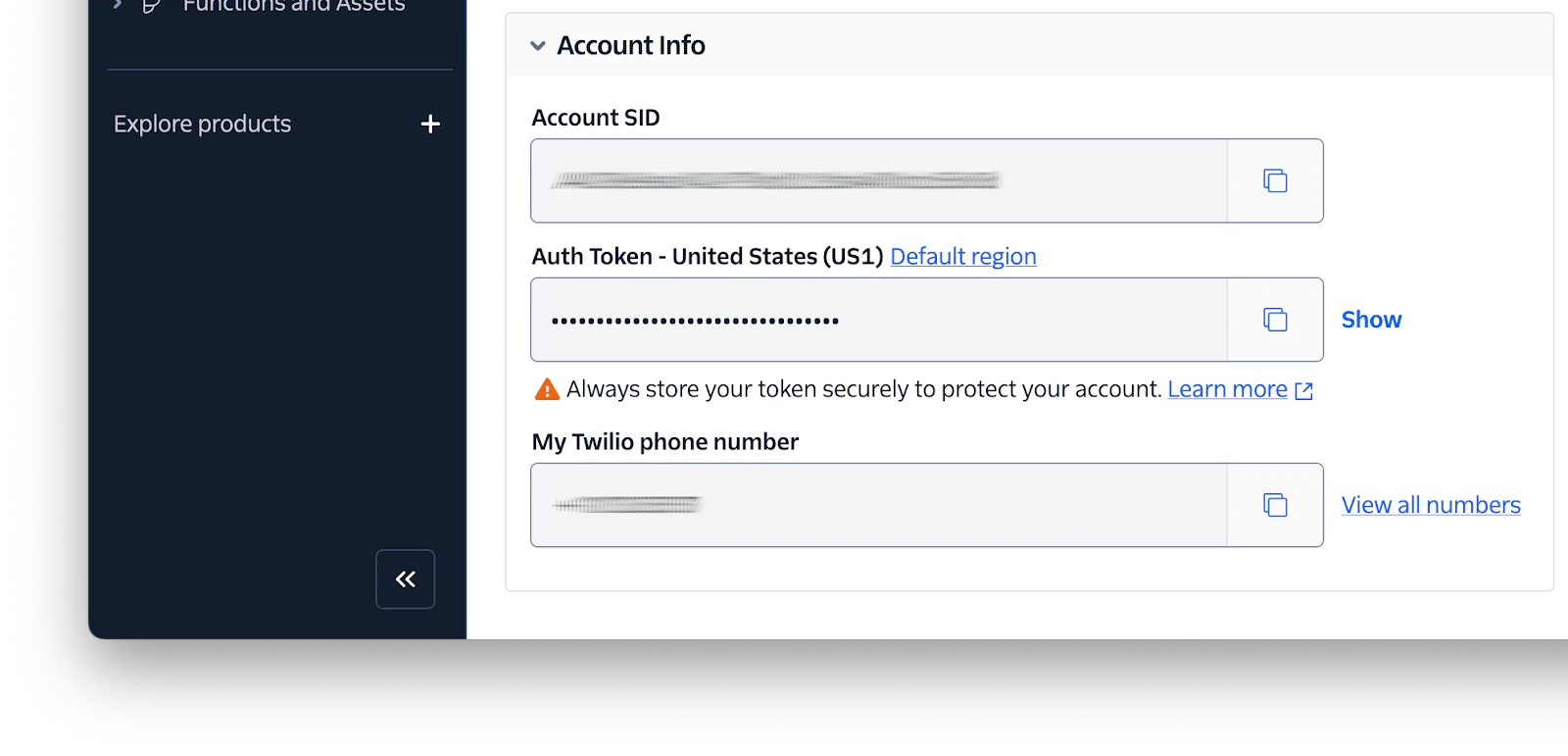
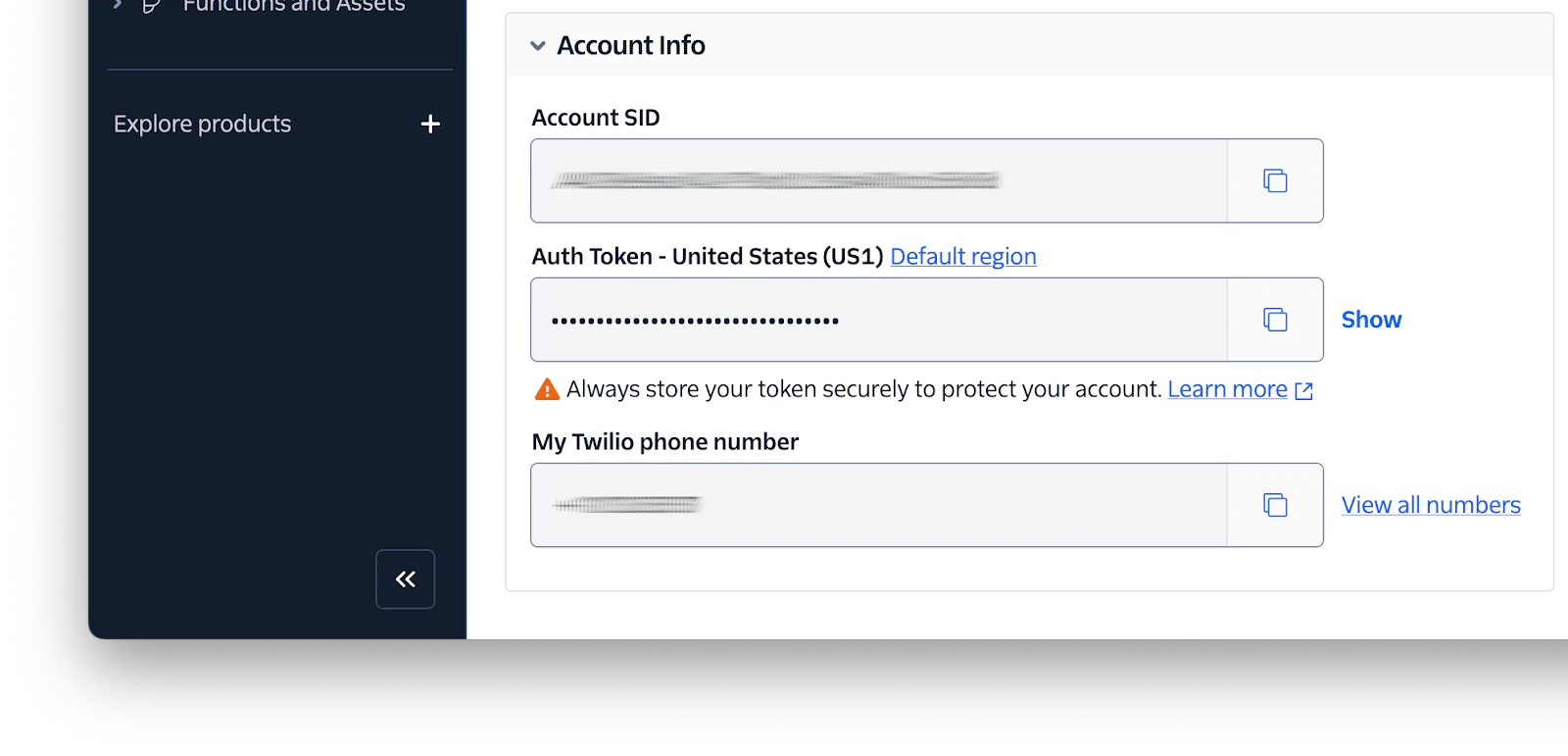
Load the environment variables
Next, you will create a helper function to load the environment variables defined in the .env file and make them accessible throughout the application. To do this, create a folder named config, and a file called config.go within it. Following that, paste the following code into the new file:
This loads the environment variables from the .env file using os.Getenv()
, so that they can be accessed anywhere in the Go code. The program will log an error and terminate if the file is not found.
Add the ability to send SMS with Twilio
Now, you will create another helper function for interacting with the Twilio API to send SMS. Create a folder named sms and a file called send.go within it, then paste the following content into the file:
The SendSMS()
accepts to
which is the recipient’s phone number and message
which is the content to be sent. It uses the Twilio Go Helper Library to send the SMS. If the message is sent successfully, it logs a success message; otherwise, it returns an error.
Add the ability to send campaign SMS
Next, create a new file named campaign.go within the sms folder. This file will hold the list of customers’ details, a function to retrieve a specific customer’s details and the logic to send marketing campaigns to customers who have not opted out.
Paste the following code into the file:
The code first defines a Customer
struct that stores a customer’s details with two fields: PhoneNumber
and OptedOut
. Next, an array of Customers
is defined, with two phone numbers hardcoded for this tutorial. This can be replaced with database integration for more complex use cases if desired. Make sure you update the array with your phone number.
Lastly, the FindCustomer()
function searches for a customer by their phone number, and SendMarketingCampaign()
sends the promotional message to customers who have not opted out, using the SendSMS()
function from earlier.
Let users opt out of the campaign
To allow customers to opt out of the campaign, create a new file named optout.go within the sms folder and paste the following code within it:
The HandleIncomingSMS()
function handles responses from customers. It checks if the message body is “STOP”, and if so, marks the customer as opted out. Otherwise, it logs the received message. This function simulates how the system can process opt-out requests.
Put it all together
Create a main.go in the project’s root directory and then paste the following code in it:
The main.go file is the application's entry point. It sets up the HTTP routes for sending campaigns and processing replies. Running the application will start a server on port 8080, enabling you to interact with the campaign and opt-out functionality.
Test the code
With the code completed, it's time to test that the application works as expected. First, start the server with the following command:
This will start the server on port 8080.
Then, in a new terminal session or tab, run the command below to send a campaign request.
You will see the following output printed to the terminal:
And, if the message is successfully received, it should look similar to the screenshot below.
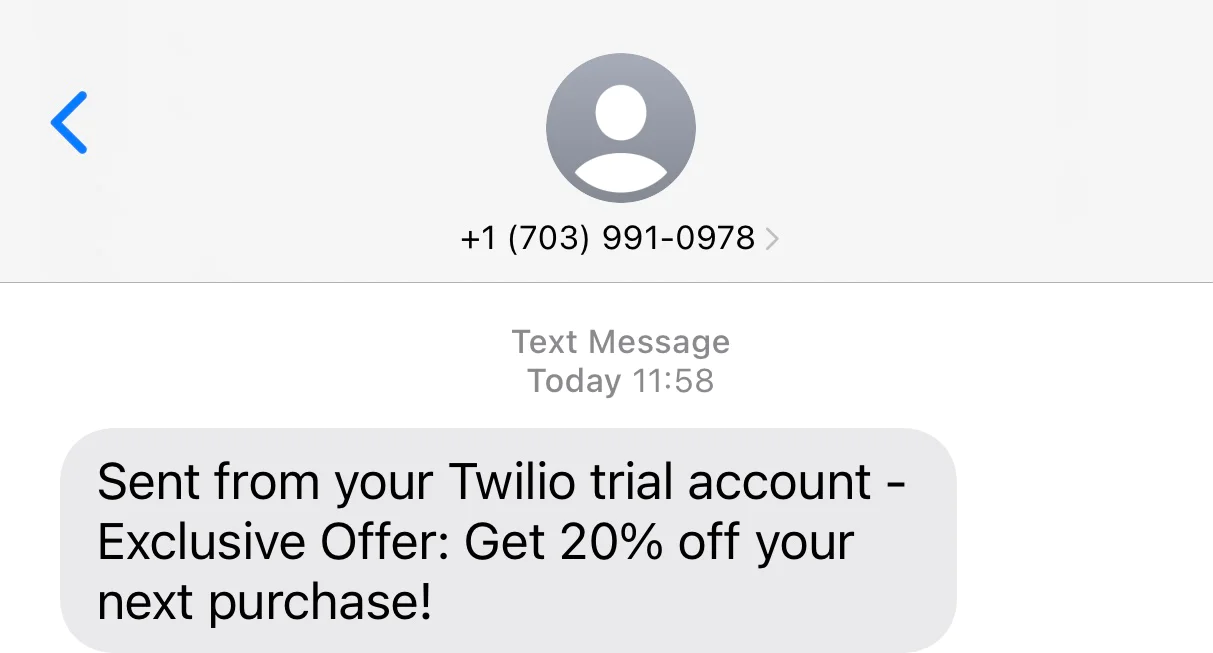
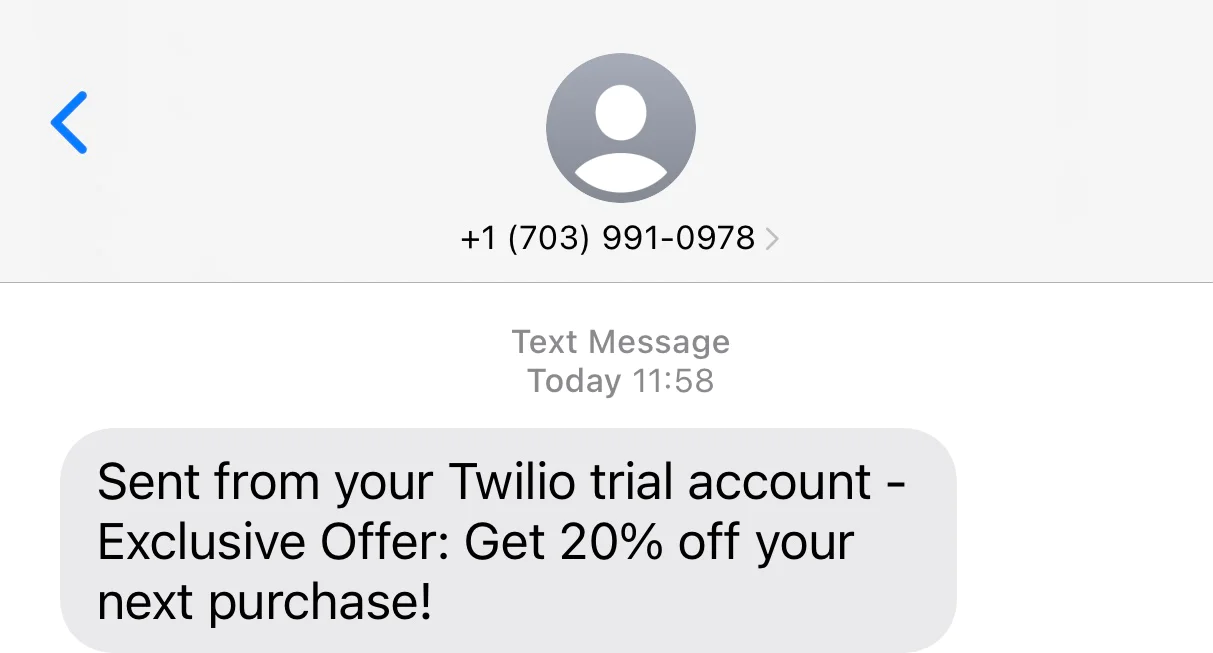
You can opt out of receiving future messages by sending the appropriate HTTP request to the "/process-reply" endpoint with the command below.
That's how to enhance customer engagement with Go and Twilio's SMS Marketing API
The flexibility of this implementation makes it applicable across various industries that depend on effective customer communication and targeted promotions. Whether retail, e-commerce, healthcare, hospitality, or events and entertainment, businesses can leverage this SMS marketing service to engage their audience and drive sales.
What we've built here is a solid foundation that can be extended and enhanced. For instance, while this example uses hardcoded customer details, a more robust solution would involve integrating a database to manage customer data efficiently. Adopting a more organized folder structure would improve scalability and maintainability, making extending the application with new features more accessible.
I hope you found this tutorial helpful. Check out Twilio's official documentation to explore additional products and services that can help grow your business. The complete source code can be found here on GitHub. Happy coding!
Oluyemi is a tech enthusiast with a background in telecommunications engineering. With a keen interest to solve day-to-day problems encountered by users, he ventured into programming and has since directed his problem-solving skills at building software for both web and mobile.
A full-stack software engineer with a passion for sharing knowledge, Oluyemi has published a good number of technical articles and content on several blogs on the internet. Being tech-savvy, his hobbies include trying out new programming languages and frameworks. You can find him at: https://twitter.com/yemiwebby and https://github.com/yemiwebby.
The SMS icon in the main image was created by Freepik on Flaticon.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.