Detect Cellphones and Verify Phone Numbers in Laravel PHP using Authy
Time to read: 3 minutes
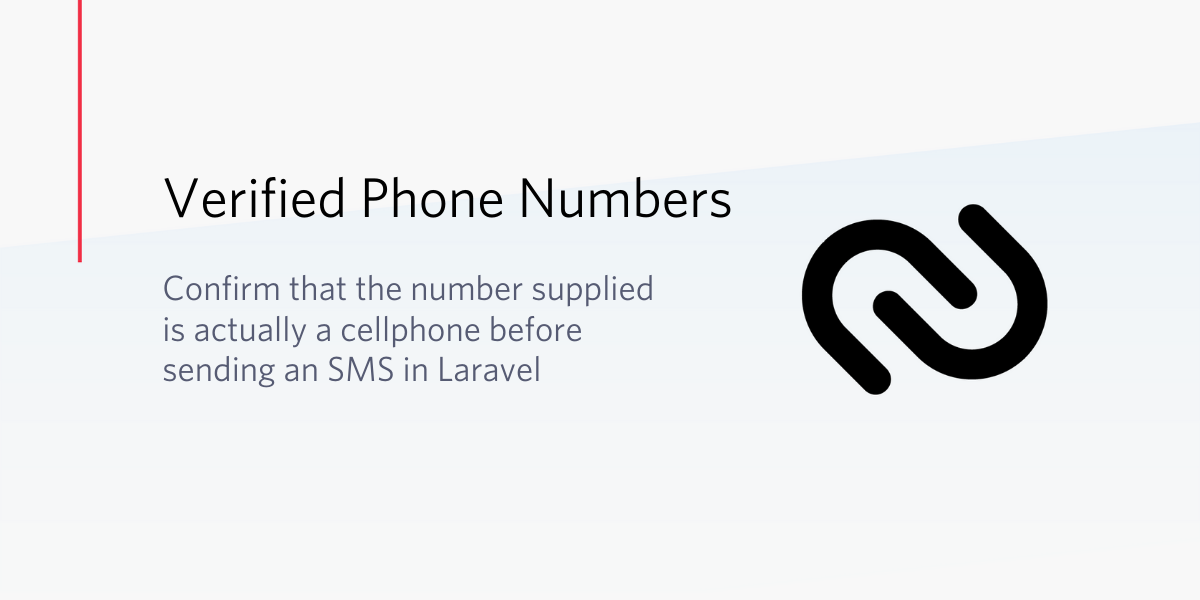
Introduction
There may be times in building your app that you will need to send an SMS to the phone number of a user. Wouldn't it be awesome if you could confirm that the number supplied is actually a cellphone? Or maybe you want to only send SMS to verified users. With Twilio's Authy API, these use cases are easy to implement. This tutorial is aimed at helping developers to build a phone number validator to verify users phone numbers in any PHP application and check if a number is a cellphone or landline before sending an SMS.
Technical Requirements
For this tutorial, it is expected that you have basic knowledge of the following:
NOTE: Don’t forget to craft a Laravel project for this purpose. Here’s a link to a guide on the installation process for installing Laravel.
Phone Number Verification
First, we need to install the Twilio PHP SDK which makes available the functions we would need to carry out the task of verifying a phone number. In the root folder of your Laravel project, run the following command.
After successfully installing the Twilio SDK, we need to configure the client application to be able to communicate with our Twilio account. To do this, please add the following to your .env
file. This should be after you have created an Authy application in your Twilio Console:
NOTE: Your "AUTHY_API_KEY” can be retrieved from the Settings tab of the Authy application you just created and “TWILIO_PHONE” is your Twilio Phone Number.
Now add the code below in config/app.php
to access the Twilio configuration variables globally.
Now that we are done with the basic installation setup, we will begin building the controller methods to process our Twilio requests. This can be achieved by using the following command and should be done inside your project directory:
Open the newly created controller file located in app/Http/Controllers/TwilioController.php
and import the Twilio AuthyApi class which will be used for phone number verification and checking verification codes.
Next, we will initialize the constructor, define the needed variables, and make them accessible within the controller. Add the following code:
Now create the controller method to verify the user’s phone number. Upon validation, it will send out a verification code to the user which will be used in the next step. Next, create another method to verify the verification code that was sent to the user.
Open up web.php
located in the routes
folder and paste in the following code for the phone verification code verification methods:
Next, create a file in the resources/views
directory called phone-verification.blade.php
which will be used to receive user input. Add the following to the file you just created.
Sending the SMS
We have created and defined the necessary variables we need for this app to verify our phone number. We will now proceed to create the logic to send our SMS.
Create a file in the resources/views
directory called send-sms.blade.php
and add the following code to it.
Route:
TwilioController:
Here’s the complete TwilioController code.
Testing
With all this done, you will want to see how this works. All you need to do is start your development server using the command below in your terminal(Unix)/Command Prompt(Windows).
Now, using your preferred browser, visit http://localhost:8000/verify (for phone verification) or http://localhost:8000/send-sms (for sending SMS). Fill in the forms and submit. On /send-sms
, input a landline number to test Authy’s phone information API. On submit, it should return an error.
Conclusion
Following this, you can see how easy it is to verify phone numbers and also send out SMS using the Twilio service. Now you can go ahead and build awesome communication-based projects.
Ugendu Martins Ositadinma
Email: ugendu04@gmail.com
Twitter: https://twitter.com/ohssie_
Github: https://github.com/ohssie
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.