How to Create a Voice Call Meeting Reminder App with CakePHP and Twilio
Time to read: 5 minutes
How to Create a Voice Call Meeting Reminder App with cakePHP and Twilio
Effective communication is vital for any team's success. In today's fast-paced environment, staying on top of schedules and deadlines can be challenging. Missed meetings can disrupt workflows and hinder progress. This is where integrating Twilio Programmable Voice with your CakePHP application can be a game-changer.
By leveraging this powerful combination, you can automate team meeting call reminders, ensuring everyone is informed and prepared beforehand. This not only improves punctuality and participation but also fosters a culture of accountability and organization within your team. In this tutorial, you will learn how to integrate Twilio Programmable Voice with a CakePHP application to create an automated meeting reminder system for your team.
Benefits of the app
An automated meeting reminders app offers numerous benefits for both team leads and members, including the following:
- Improved Attendance: By sending automated voice call meeting reminders, team members receive a reminder call. This ensures they are notified even if their phone is not connected to a network.
- Reduced Missed Meetings: Automated voice call reminders minimize the chances of team members missing out meetings, leading to better attendance and participation.
- Streamlined Communication: This allows the team lead to convey important messages to team members before the meeting starts.
- Increased Productivity: By reducing scheduling conflicts and ensuring everyone is on the same page, team productivity can be significantly boosted.
Prerequisites
- PHP with a minimum version of 8.2
- Composer installed globally
- Access to a MySQL database and a database client, such as phpMyAdmin or Navicat
- A Twilio account (either free or paid) with an active phone number. If you are new to Twilio, click here to create a free account.
Create a CakePHP project
To begin, let’s create a new CakePHP project using Composer by running the command below:
After the installation is successful, you will be asked to "Set Folder Permissions ? (Default to Y) [Y,n]?", answer with Y. then run the command below to navigate to the project's working directory.
Connect to the database
To set up the application database, open the project in your code editor and navigate to the config folder. Inside the folder, open the app_local.php file, and in the Datasources > default section, update the database options with the following:
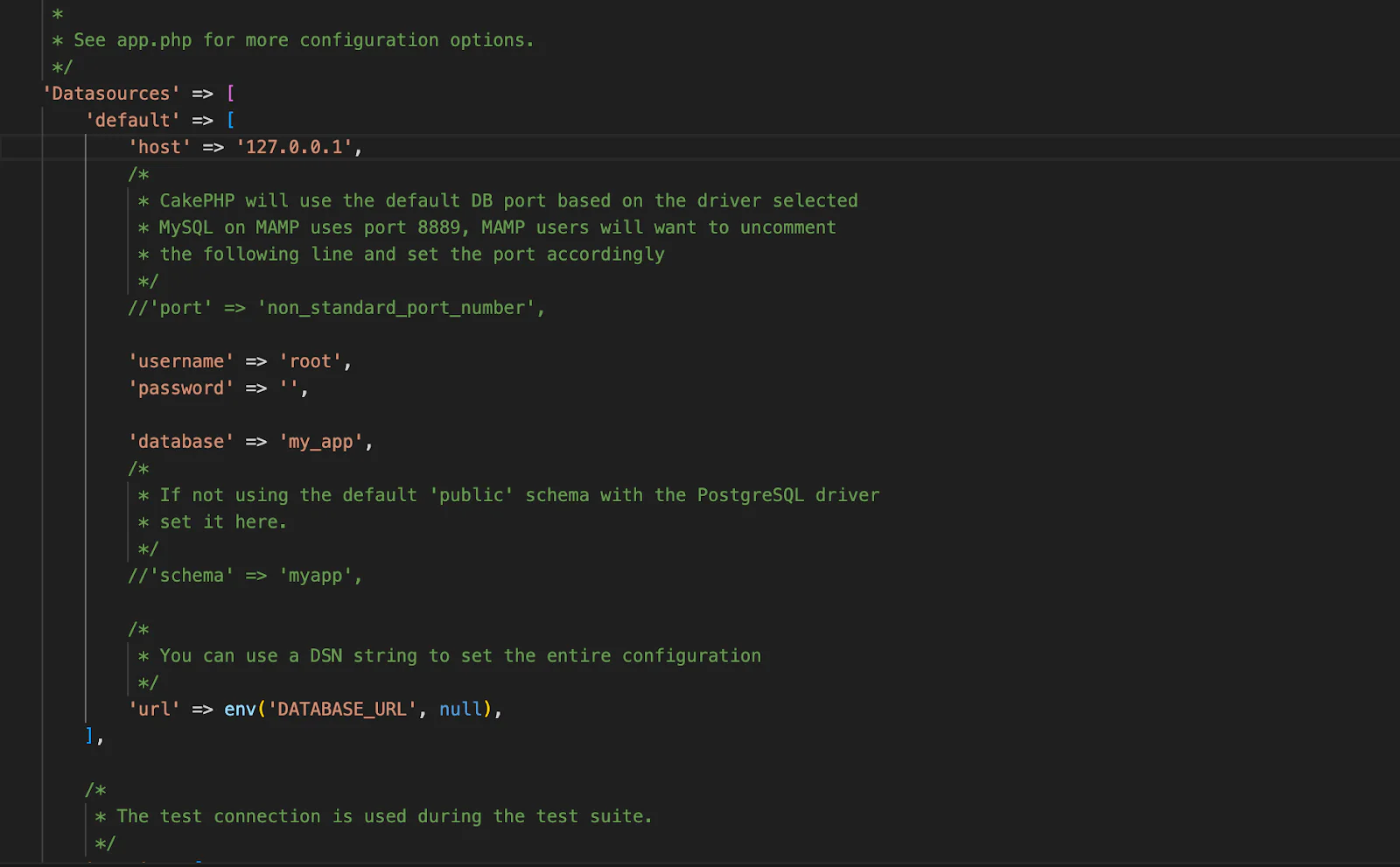
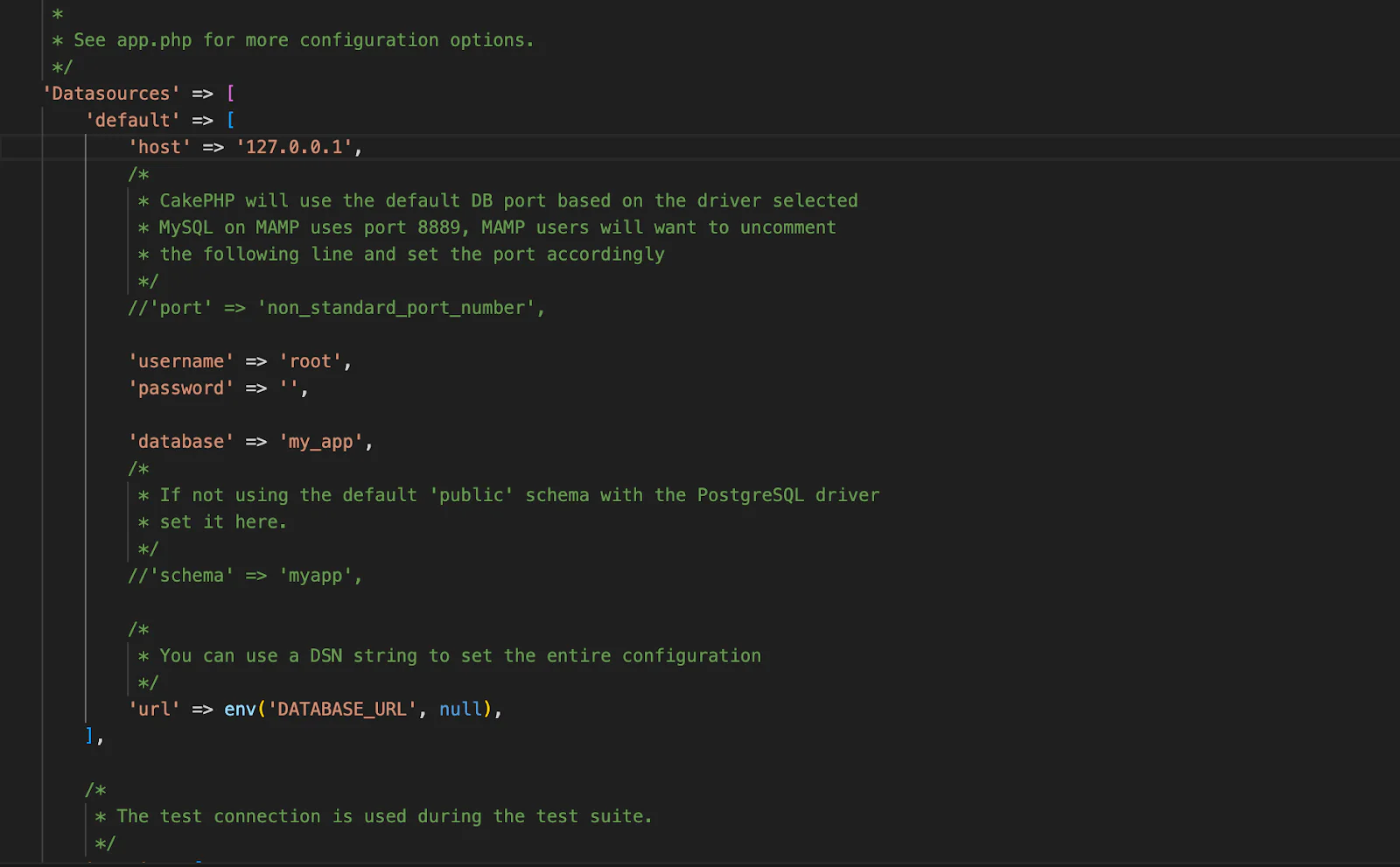
Create the database
To start, we need to create a database that will store the team member's information. To do this, in your database server, create a database named my_app
.
After creating the database, run the CakePHP migration command in your terminal to generate the migration file for the database.
Then, in the config/Migrations/ folder open the migration file ending with _Members.php, and update the change()
function to match the following code:
Then, run the database migration by running the command below:
Now, check the MySQL server. You should have a members
table with the id, email, username and phone fields, as shown in the screenshot below with phpMyAdmin:
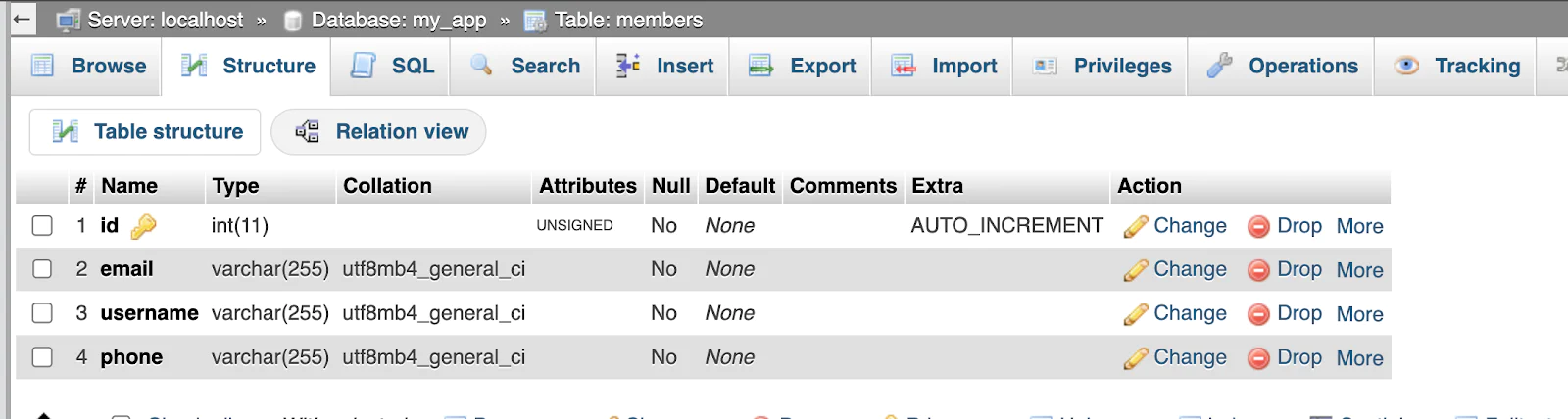
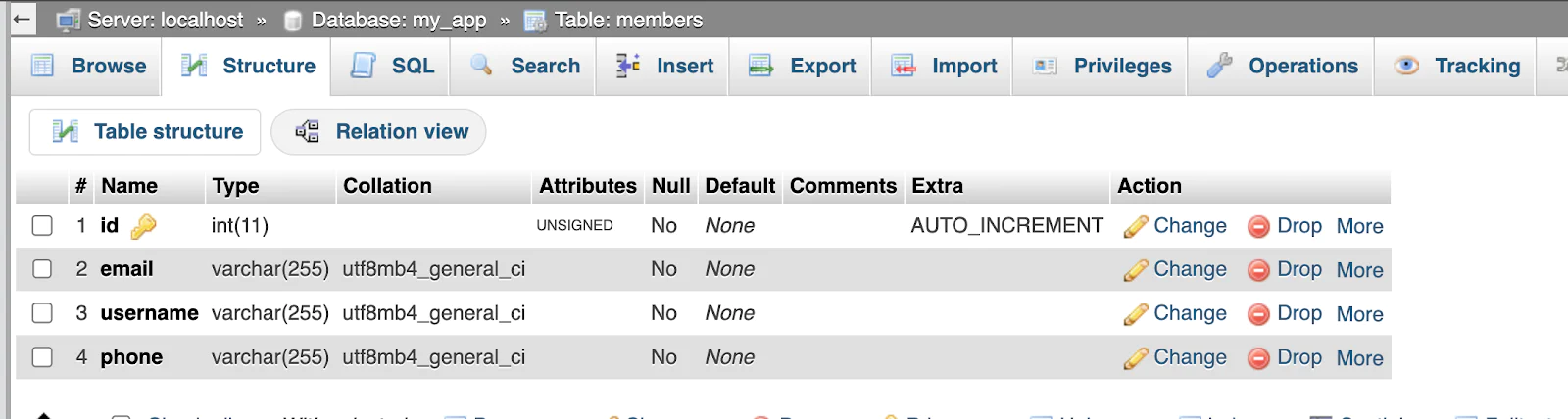
Next, start the application by running the command below:
After starting the application, open http://localhost:8765 in your browser. It should look similar to the screenshot below.
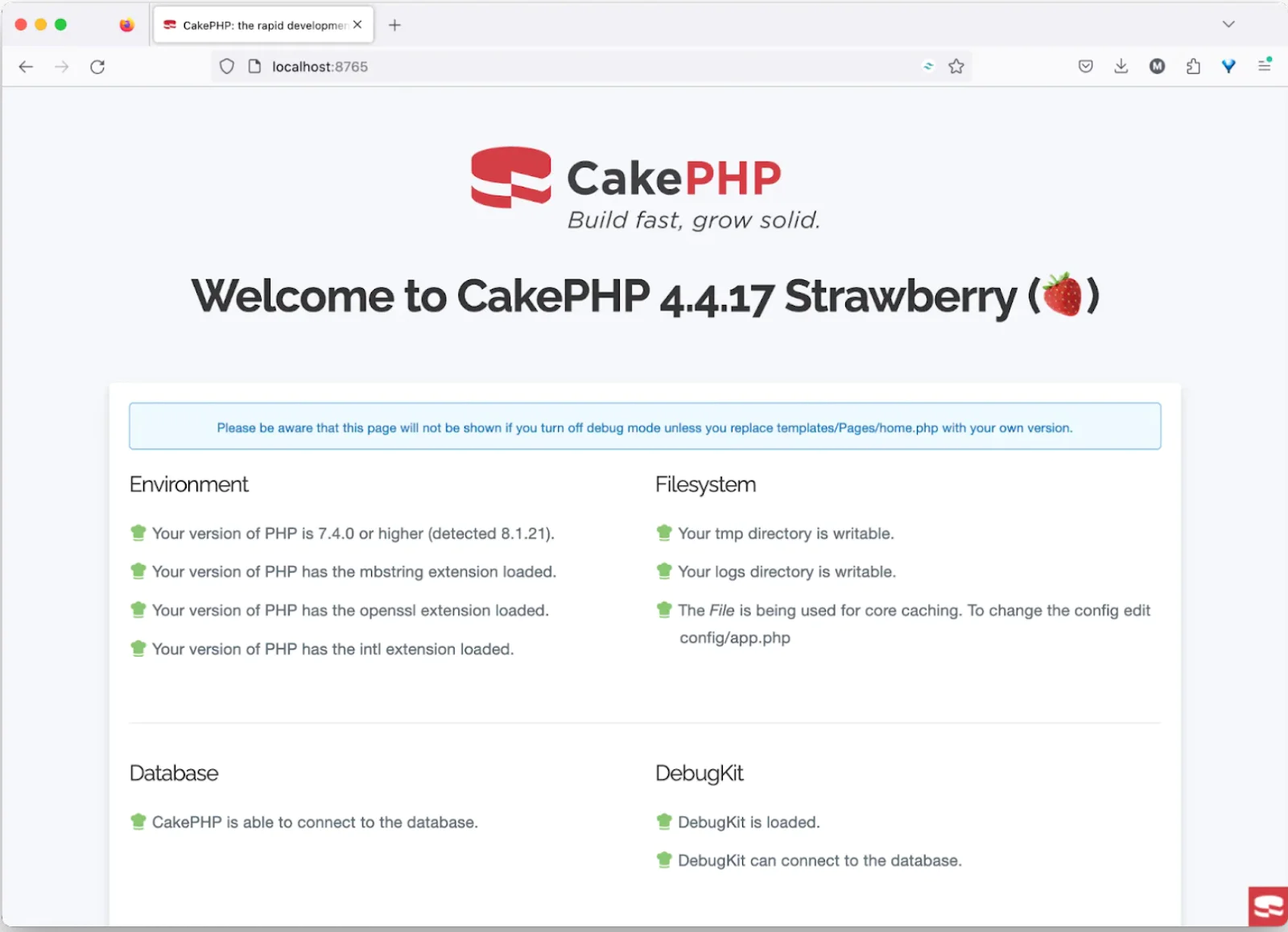
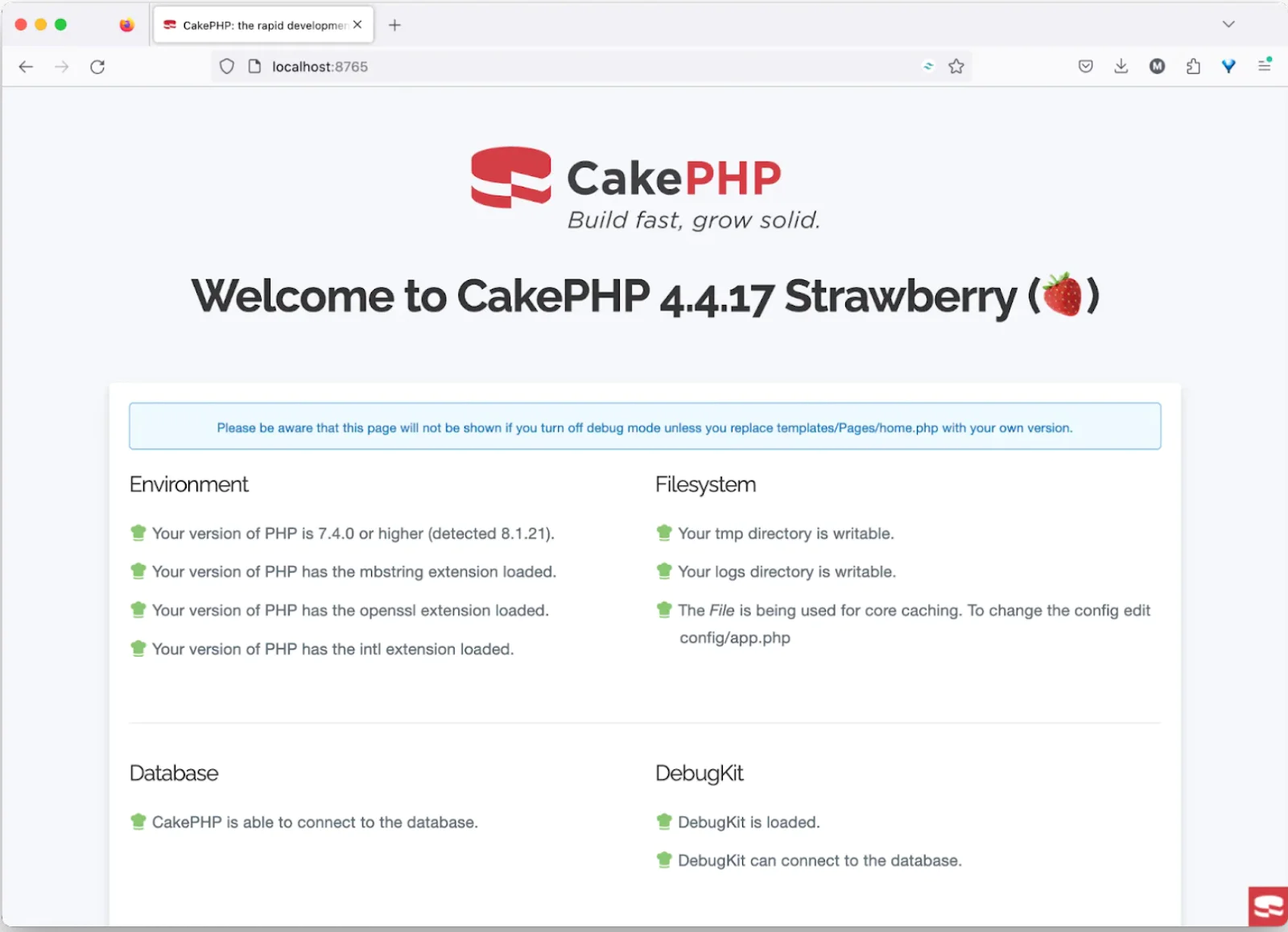
Create the application model and entity
To create a model and entity, open up a new terminal window or tab and run the command below:
The entity file Member.php and the model file MembersTable.php will be available inside the /src/Model/Table and /src/Model/Entity folders, respectively.
Retrieve your Twilio credentials
To retrieve them, open the Twilio Console dashboard. There, in the Account Info section, you will see your Account SID and Auth Token, as shown in the image below.
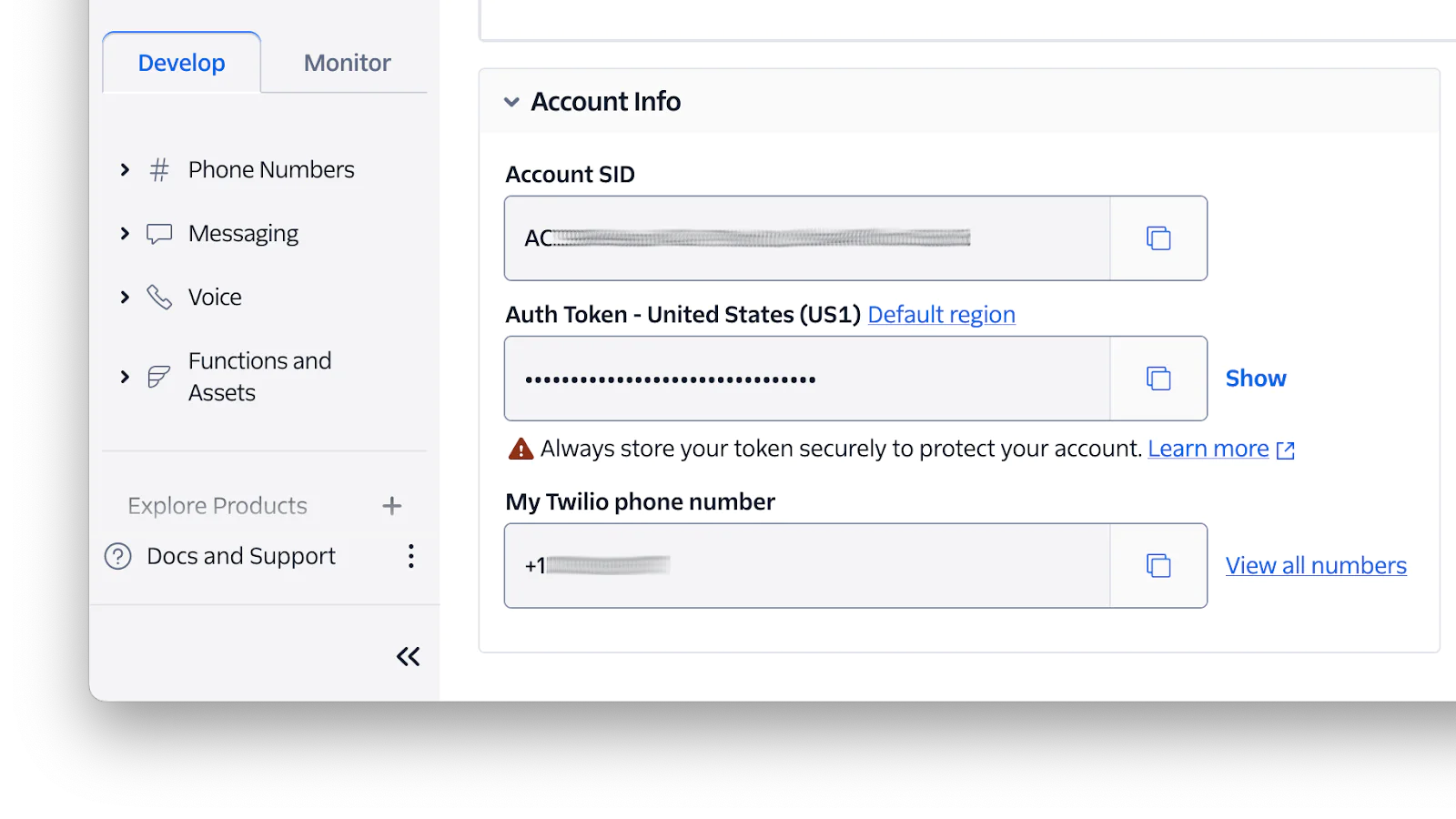
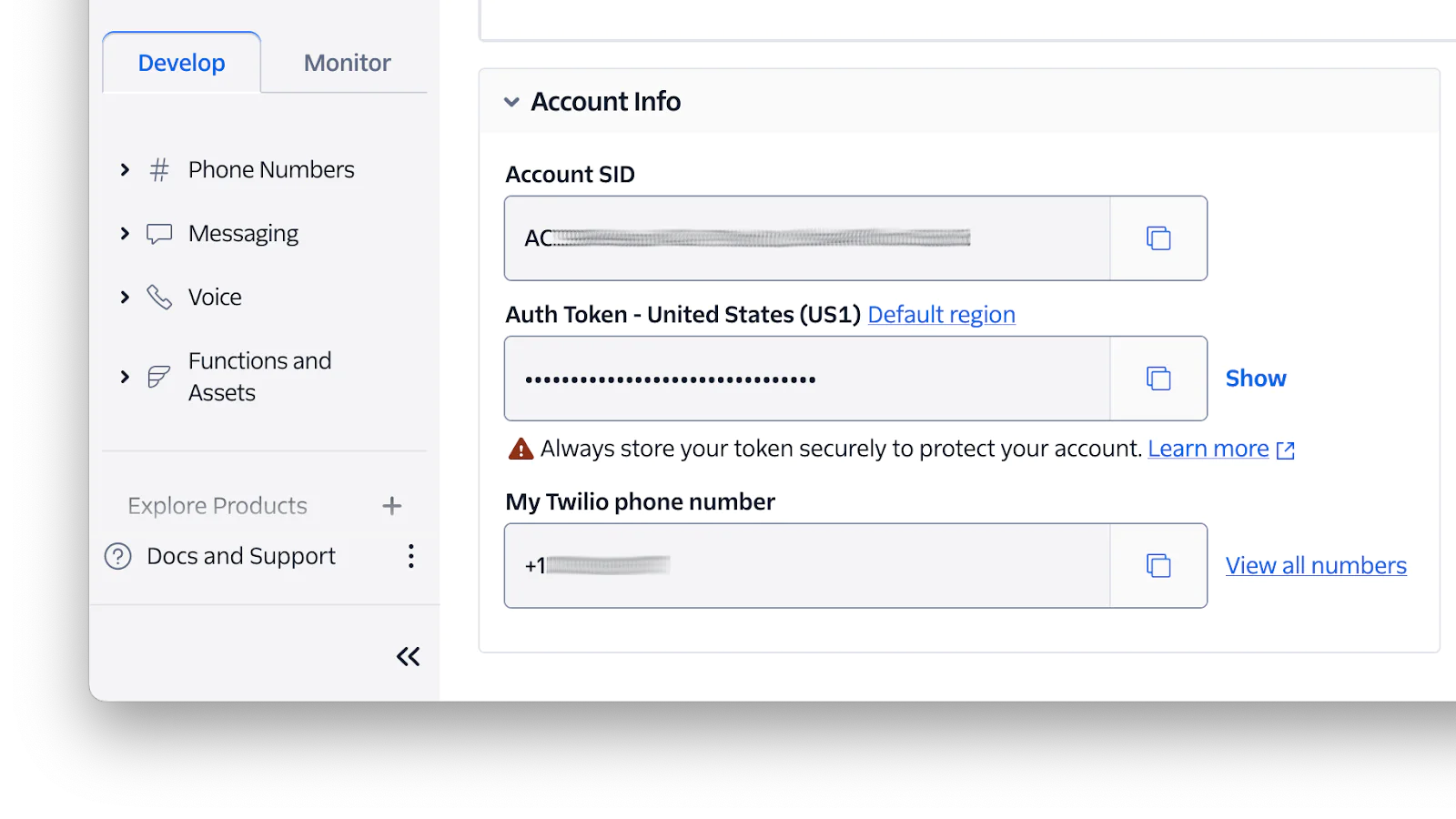
Store the access token in .env file
To use environment variables in CakePHP applications, you need to create a .env file from .env.example. This can be done by running the command below.
Now, navigate to the config folder and open .env. Then add the following Twilio variables to the end of it.
From the code above, replace <your_account_sid>
, <your_auth_token>
and <your_twilio_phone_number>
, respectively, with your corresponding Twilio values.
To finish the environment variable configuration, next, navigate to config/bootstrap.php and uncomment the following section.
Install Twilio's PHP Helper Library
After retrieving your Twilio credentials, you need to install Twilio's PHP Helper Library, by running the command below:
Create the core controller
Next up, the CakePHP bake console can be used to generate the application controller, by running the command below:
Next, navigate to the src/Controller folder, open the new MeetingController.php file, and add the following code to it:
From the code above:
- The
register()
method is used to handle the team member registration page. - The
sendReminder()
method is used to send the reminder message. Inside this function, we retrieve our Twilio access token from the .env file and send the voice call message using the$twilioClient->calls->create()
method.
Create the application templates
Next, navigate to the templates directoryandcreatea Meeting folder inside it. Then, create a file named register.php in that directory and add the following registration form template code to it.
After that, to create the verification page template, inside the Meeting folder, create a file named send_reminder.php and add the following code to it.
The final step is to add the register
and verify
routes to the application configuration. To do that, navigate to the config directory and open routes.php. Inside it, add the following routes just before $builder->fallbacks();
:
Test the application
To test the application, you need to register your team members' details. To do that, open http://localhost:8765/register in your browser and enter enter member details as shown in the screenshot below:
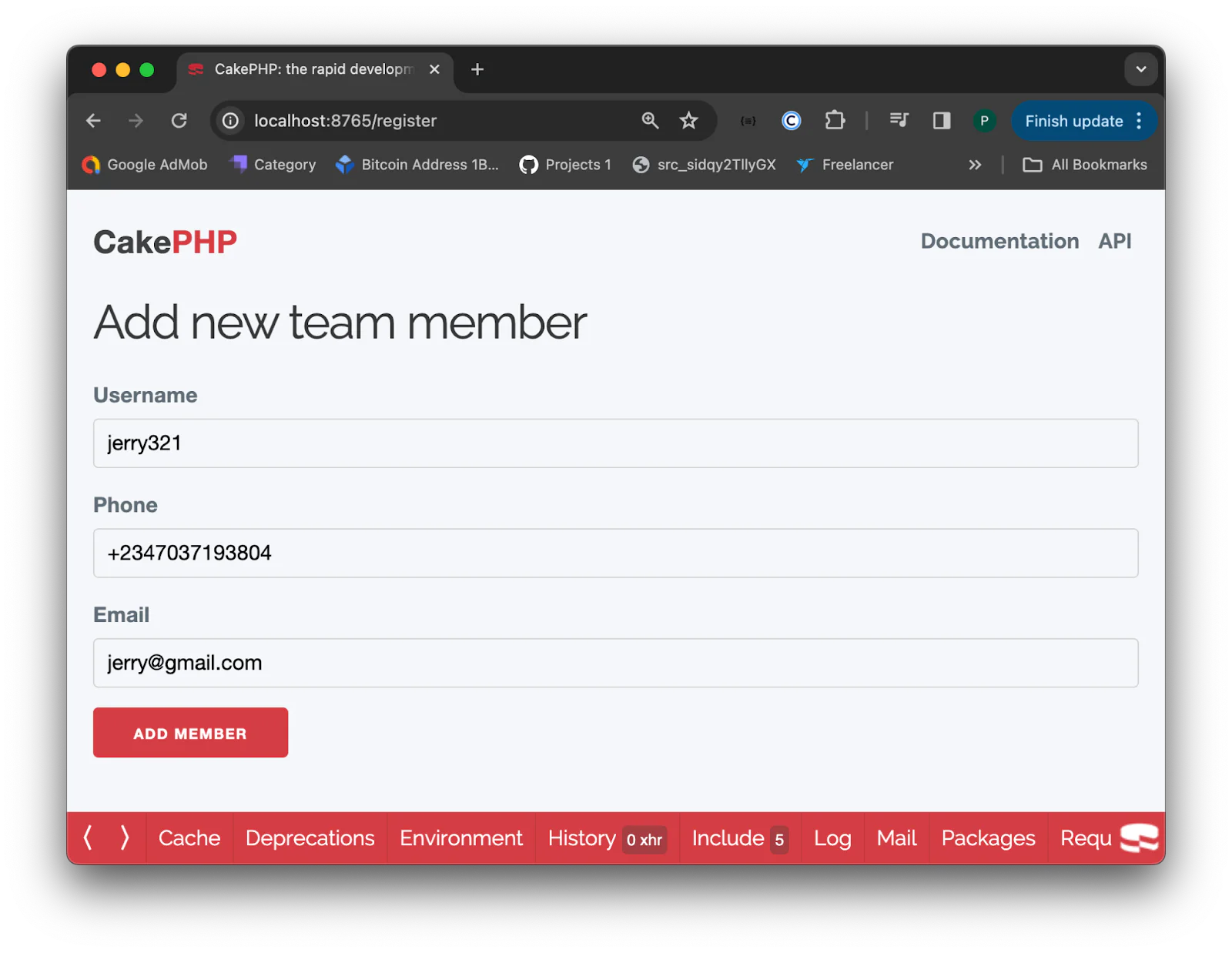
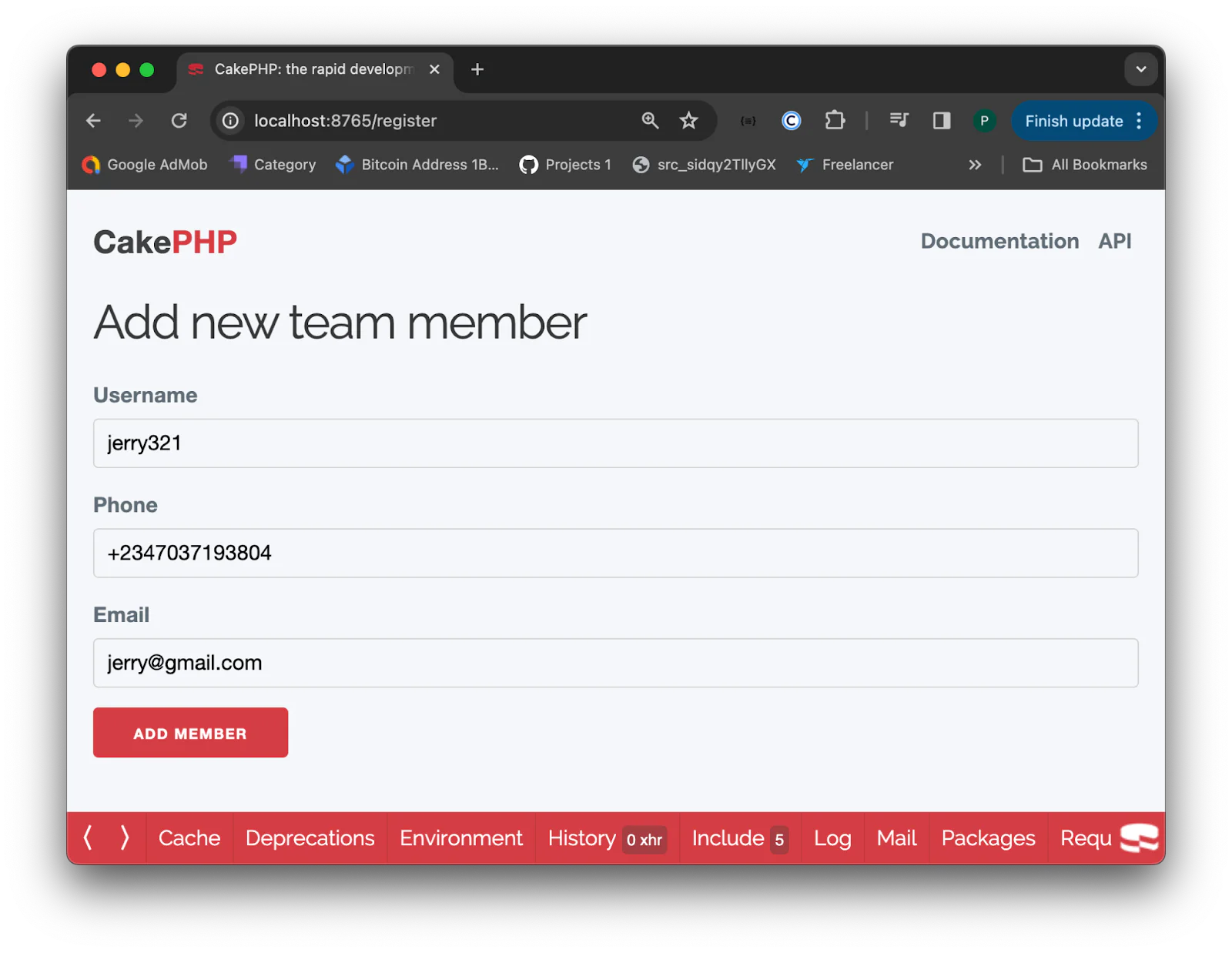
After entering the member's username, phone number, and email address, click on the ADD MEMBER button to register the team member in the database. You can register as many team members as you have.
To send the meeting voice call reminder to registered team members, open http://localhost:8765/sendreminder in your browser and enter the reminder message as shown in the screenshot below:
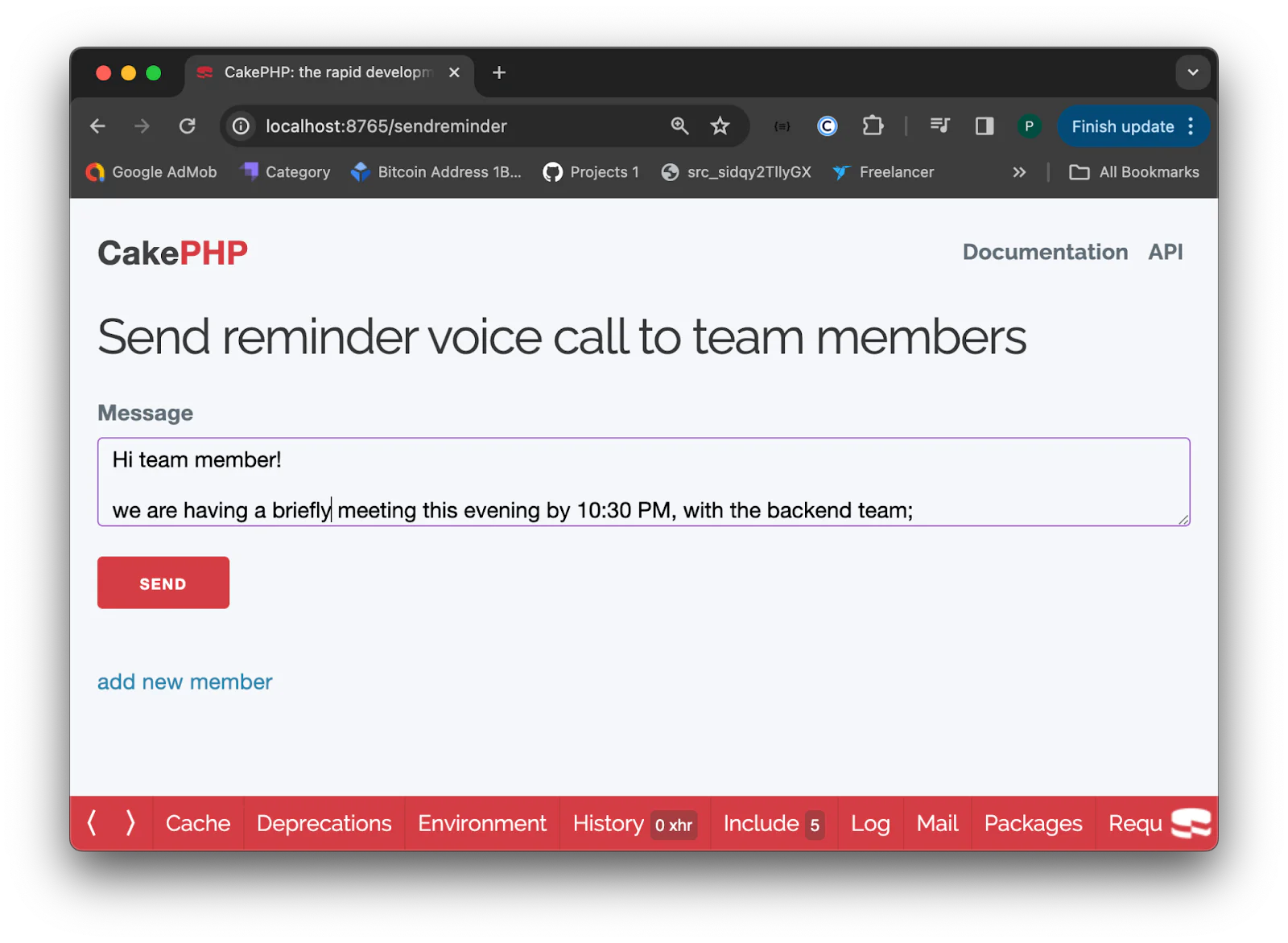
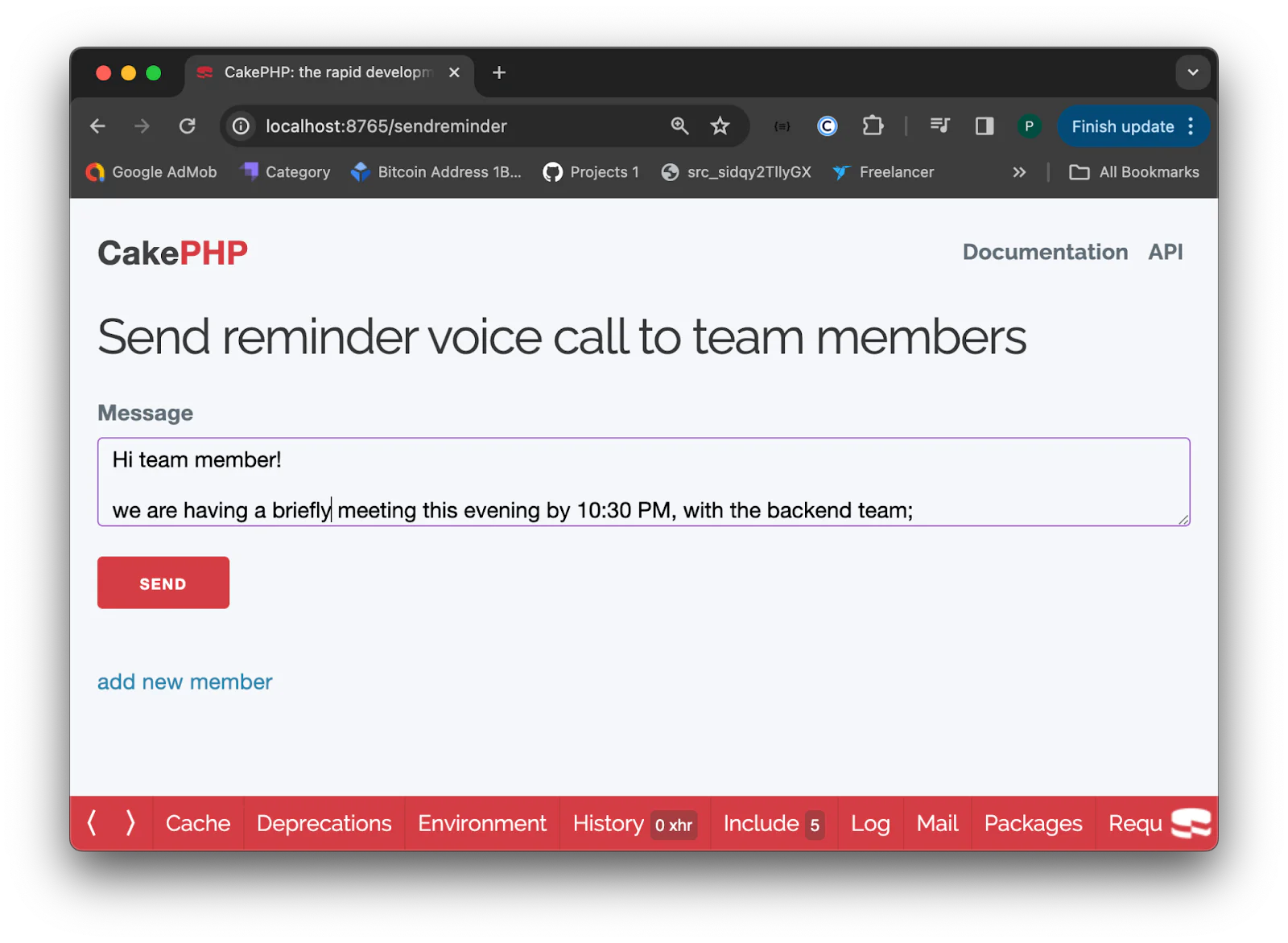
After filling out the meeting message, click on the SEND button. This will place a voice call to all registered team members, providing them with details about the meeting.
That's how to create a voice call meeting reminder app with CakePHP and Twilio
In this article, we discuss how to integrate Twilio Programmable Voice with CakePHP application to build efficient automated team meeting call reminders. This integration streamlines communication and fosters a culture of punctuality, accountability, and improved overall team productivity.
Key takeaways:
- Improved Attendance: By sending automated voice call meeting reminders, team members receive a reminder call. This ensures they are notified even if their phone is not connected to a network.
- Reduced Missed Meetings: Automated voice call reminders minimize the chances of team members missing out on meetings, leading to better attendance and participation.
- Streamlined Communication: This allows the team lead to convey important messages to team members before the meeting starts.
- Increased Productivity: By reducing scheduling conflicts and ensuring everyone is on the same page, team productivity can be significantly boosted.
Remember to customize the reminders to suit your specific needs and consider incorporating additional features like calendar integration or escalation options for missed calls.
I'm Oluseye Jeremiah, a dedicated technical writer with a proven track record in creating engaging and educational content.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.