Create a Real-Time Twitter Stream in PHP using Pusher and Laravel
Time to read: 7 minutes
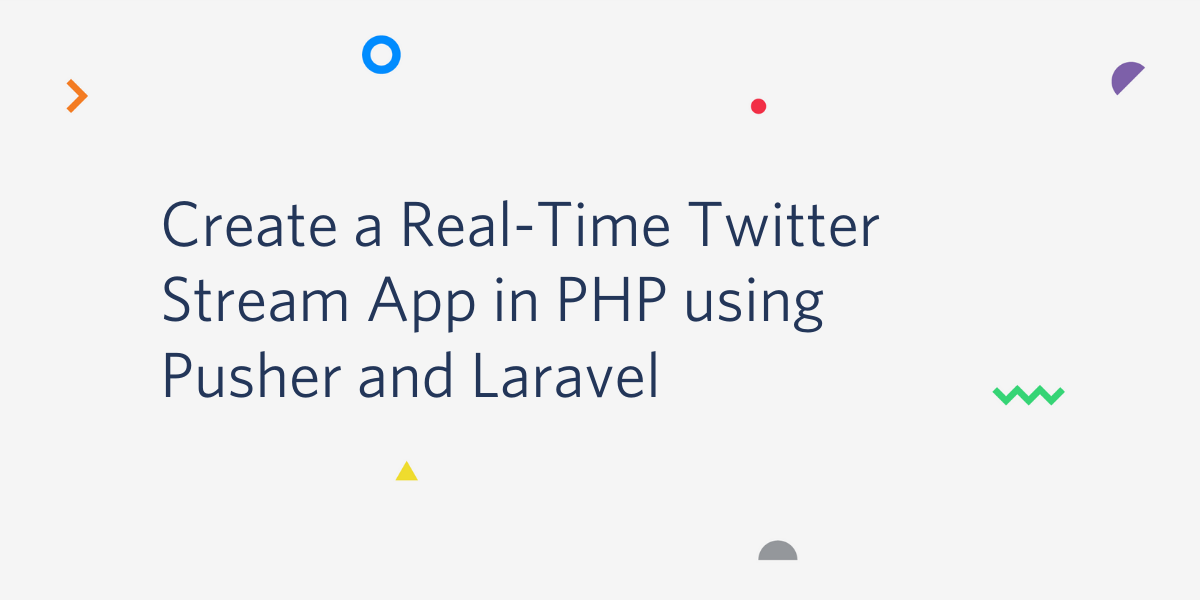
Introduction
Laravel is one of the most famous PHP MVC frameworks, largely due to its commitment to modern architecture and active community. Laravel 7, the latest release, has added the coolest new features like Blade-X, Stubs, Cache optimization, and much more.
Pusher is the hosted service that enables developers to add real time data and functionality easily and securely by taking care of all the heavy lifting under the hood.
Laravel supports Pusher integration very gracefully, which makes it very easy for developers to add real-time functionality to their application. In this tutorial, we will be using Twitter APIs to fetch tweets based on a specific hashtag, and create a dashboard to display them in real-time using Pusher.
How does Pusher work?
Pusher uses Channels to provide real-time communication between the server and the client. What are these Channels? Pusher Channels are based on the popular Publish/Subscribe Model. In the publish-subscribe model, senders (called publishers) don’t send messages directly to receivers (called subscribers), they send messages into a specific categorized channel without knowledge of the subscribers. Anyone who is a subscriber to that specific channel will get the message.
Pusher acts as a proxy and sits in between the client and the server. It maintains the persistent connection to the clients over WebSockets and HTTP if the browser doesn’t support WebSockets. The server sends data directly to Pusher through HTTP which Pusher then forwards to the client over WebSockets. This is how Pusher accomplishes real-time communication.
Requirements
To complete this tutorial you will need the following:
- PHP development environment with Laravel installed
- Composer globally installed
- Pusher Account
- Twitter Developer Account
- Passion :D
Set Up a New Laravel Project
If you don’t have Laravel installed in your system, a quick download from the Laravel Official Documentation will get you started. A setup guide can be found in the Laravel docs.
Let’s start with setting up a new Laravel project named pusher-tweets
. Remember, you can give your project any name you want. Run the following command in your terminal to begin:
This will install Laravel v7.0, along with all the dependencies required by it. Composer post-create
scripts automatically create the .env
file for us and set the APP_KEY
environment variable. If you’re unaware, the .env
provides secure, non-cacheable storage of our application security credentials.
Add the APP_KEY
in the .env
file by running this command, if it is not configured automatically.
Add the Tweets Page
Let’s add the frontend where we will integrate pusher for real-time communication to exchange data related to the latest tweets. I have coded a small frontend for tweets which you can find also find on codepen “Twitter gradient animation card”.
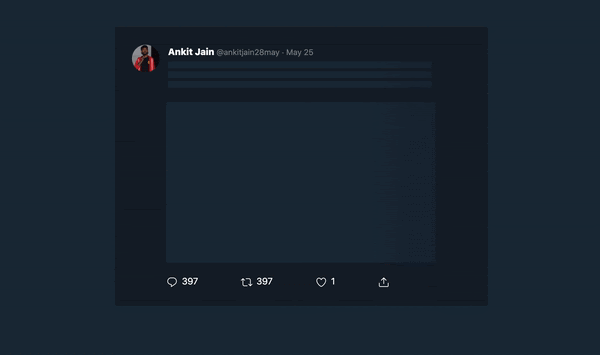
Create a new file tweets.blade.php
under resources/views
directory and copy and paste the code below.
We have added the asset reference for custom CSS and JS on line 8 and 16 in the code above. We can also see that on lines 14, 16, reference to JS is commented. We will uncomment that, later on while integrating with Pusher at the client-side.
Let’s now add the assets to the public directory.
In the public/css
folder create a new file style.css
. Copy the code below into your style.css
file. You will need to create the css
folder inside of the public
directory.
Awesome, let’s now add the route for the tweets.
Create Route for Tweets
Implementing Authentication in Laravel is very simple, as everything is already configured for us out of the box. We are not putting this route behind Authentication for demo purposes, so we don’t need to set up the database.
NOTE: I highly recommend using authentication for production, or before making the application live to keep the data safe.
Open the web.php
file under the routes
folder and add the following code:
We can check whether everything is working perfectly or not by running our application with a simple Artisan command. Make sure that you’re in the project root and run this command to start your local server:
The Laravel development server will run at http://localhost:8000
. Open the browser and navigate to this URL http://localhost:8000/tweets. You should see something similar to the screenshot below:
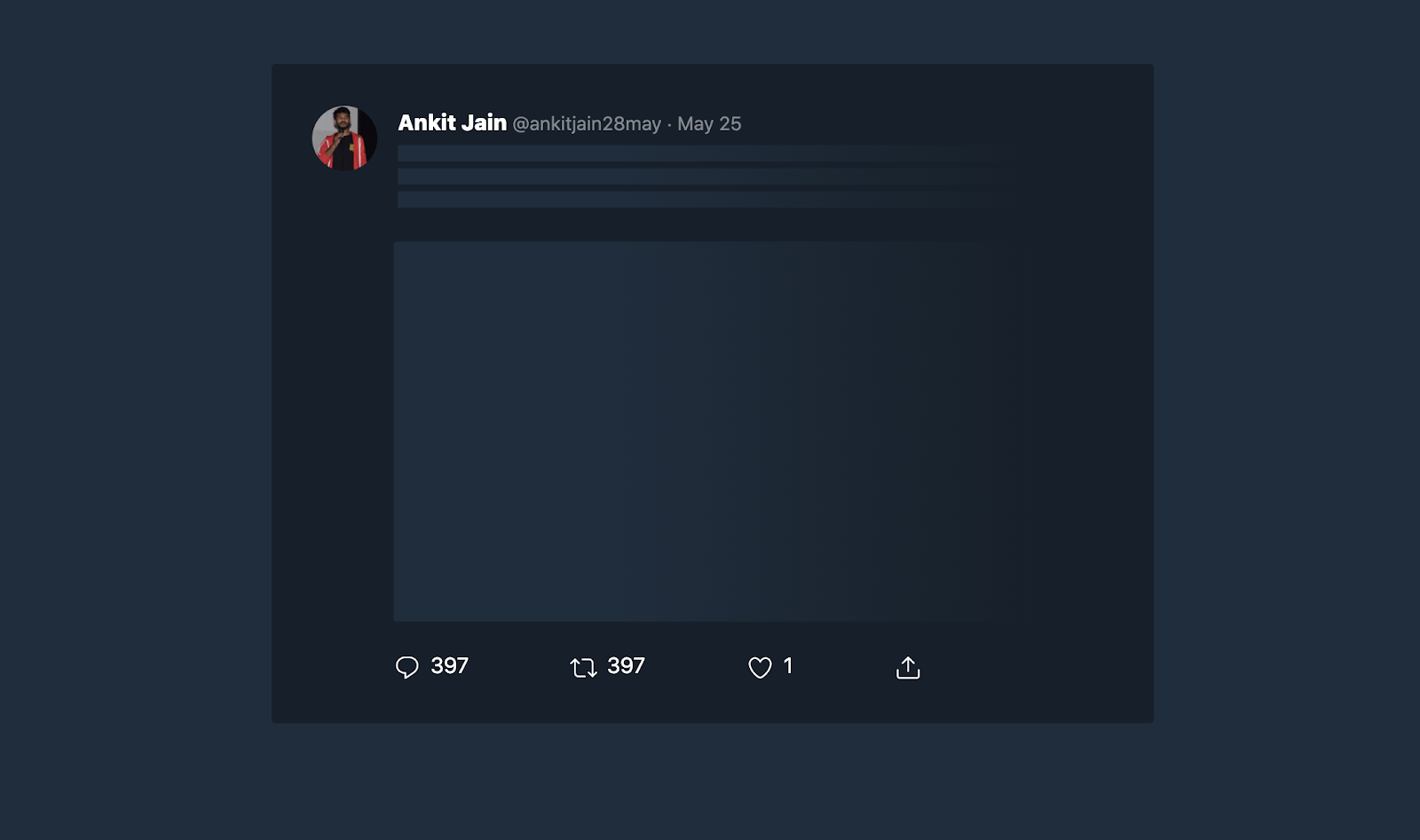
Our client-side is almost ready and working fine. Let’s add the next part; fetching tweets from Twitter using Twitter APIs.
Setting up the Twitter API
We will be using the laravel-twitter-streaming-api composer package to easily integrate Twitter Streaming API with Laravel.
Install the package by running the following command inside the pusher-tweets
directory.
Once the Composer package is installed and the config has been created, we need to add the following environment variables in the .env
file.
In order to obtain the credentials above, we need to create a new developer app called “Pusher Tweets” in the Twilio Developer dashboard. You can read more about creating an app from the Twitter Official Page.
The “Consumer API keys” and “Access token & access token secret” will be generated after your app is created, as shown in the image below:
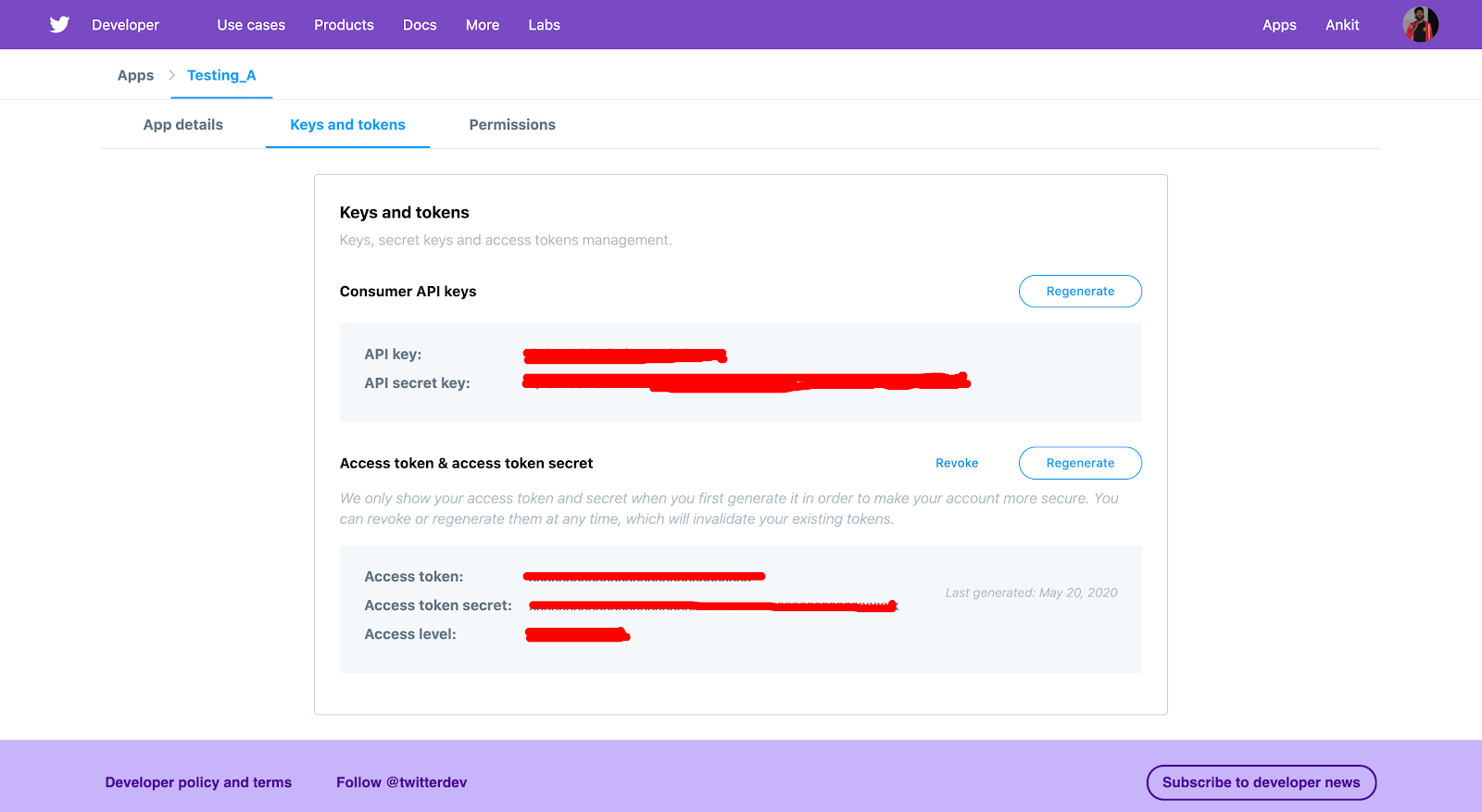
Now that these credentials have been generated, copy their values to the .env
as outlined in the previous step.
Let’s continue to the next part of writing functionality to fetch tweets in real-time using the above composer package. For this, we will be creating a console command to run independent to our Laravel application.
Creating a Console Command
By default, all of the console commands for our application are stored in the app/Console/Commands
directory. If the app/Console/Commands
directory doesn't exist, it will be created when we run the make:command Artisan command
. We can generate the TweetStream Class using the following Artisan CLI:
Let’s add the code to stream the tweets in real-time in the TweetsStream.php
file created in the app/Console/Commands
directory.
In the code above, we will be listening for hashtag “#twilio”, we can listen to as many hashtags as we want and pass them as a comma (,) separated string into the TwitterStreamingApi::publicStream()->whenHears()
function.
When we generate any console command using Artisan, it adds our command to the php artisan
usage list. Run the console command to test:
I made the dummy tweet “Hello World #twilio” to test it out. Write your own tweet using the #twilio hashtag and monitor the output. You should see something similar to what is shown in the image below:
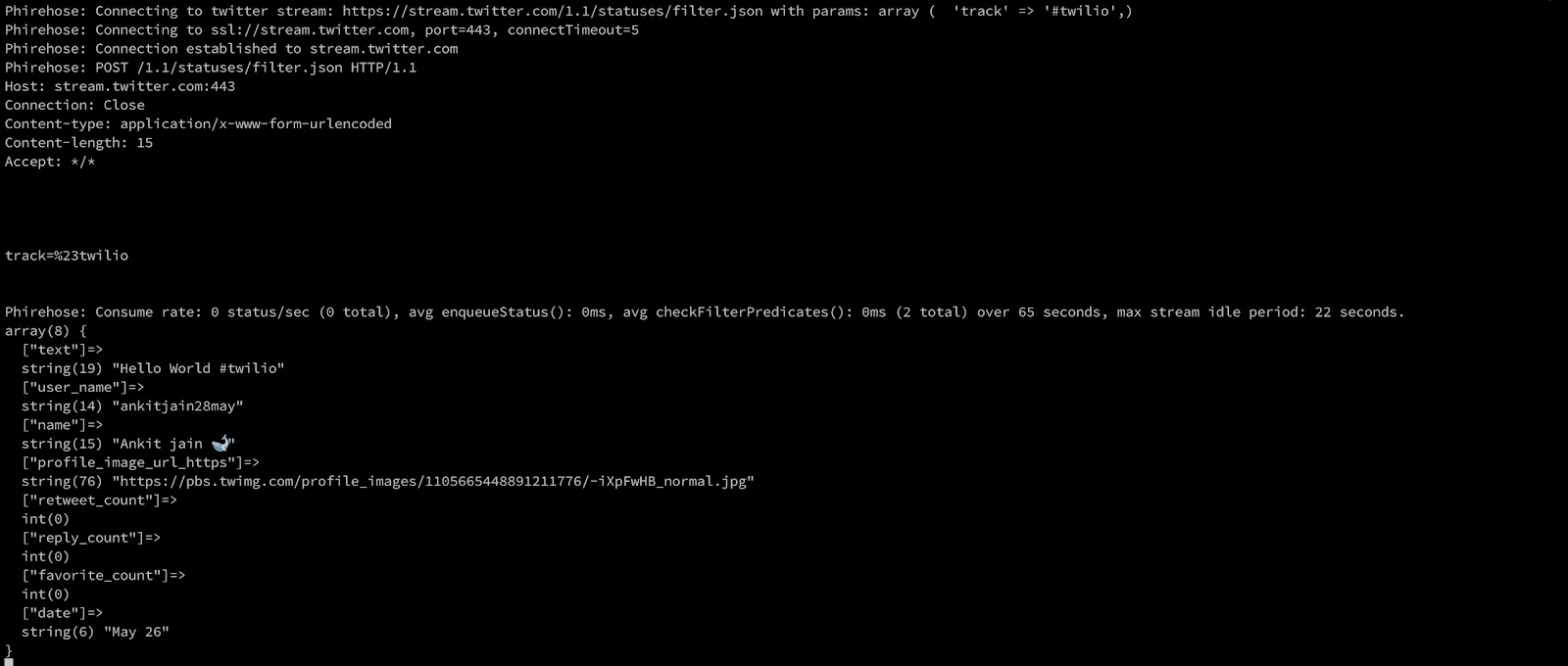
If this is working then everything is going as expected. Now let’s integrate Pusher within our application to render tweets in real time.
Create a Pusher Channel
As a reminder to our introduction,Pusher uses channels for real-time data communication. We will leverage this logic to build out the next steps.
Login to Pusher and create a new pusher channel app as shown in the image below:
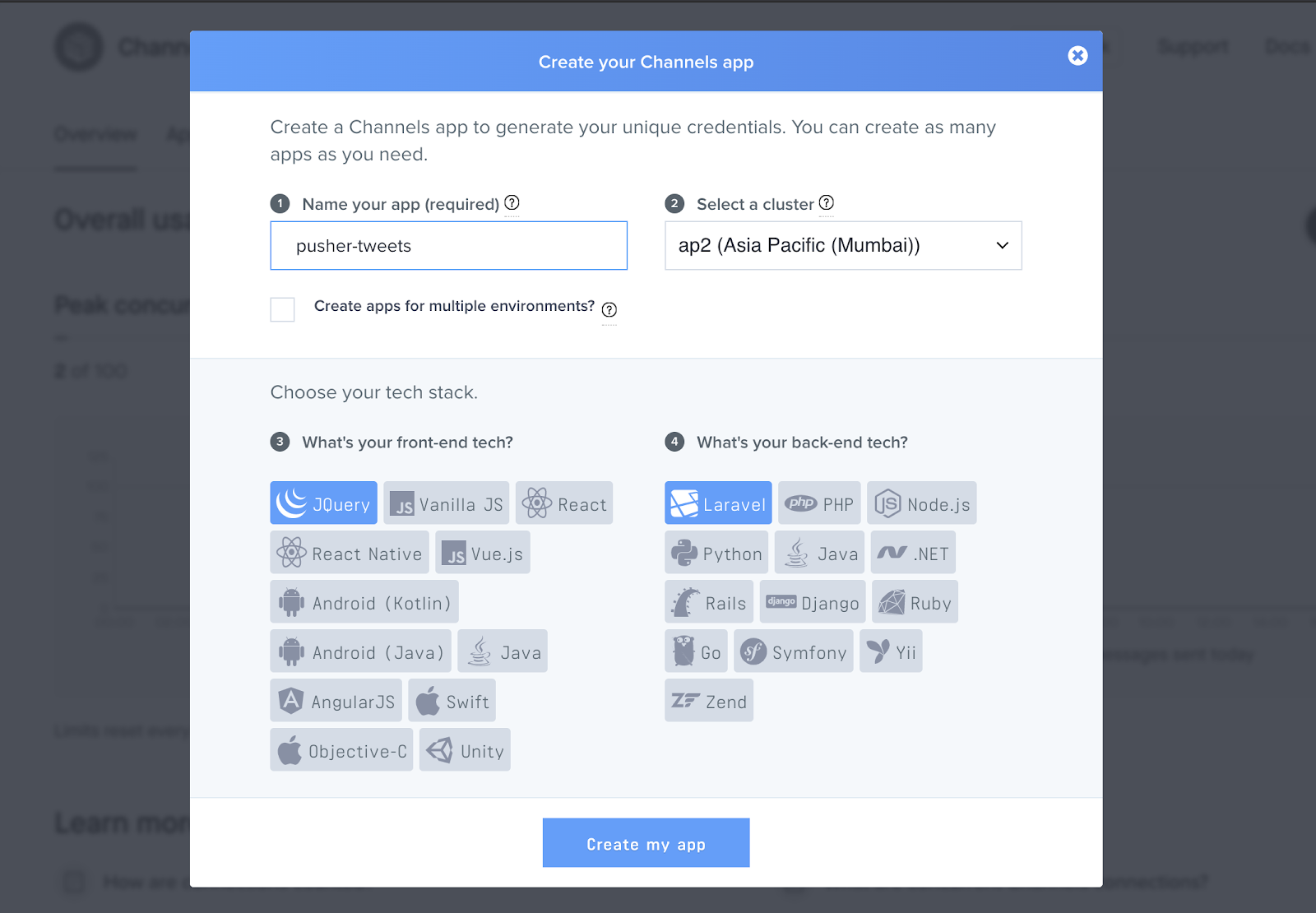
Once we create a channel app, we will get the “Pusher App ID, App Key, App Secret, and App Cluster”. Open your .env
file and replace the values respectively.
Let’s go back to our tweets.blade.php
where we have commented lines 14 and 16. Uncomment those lines and subscribe to “tweets” event in “pusher-tweets” channel as shown in the image below:
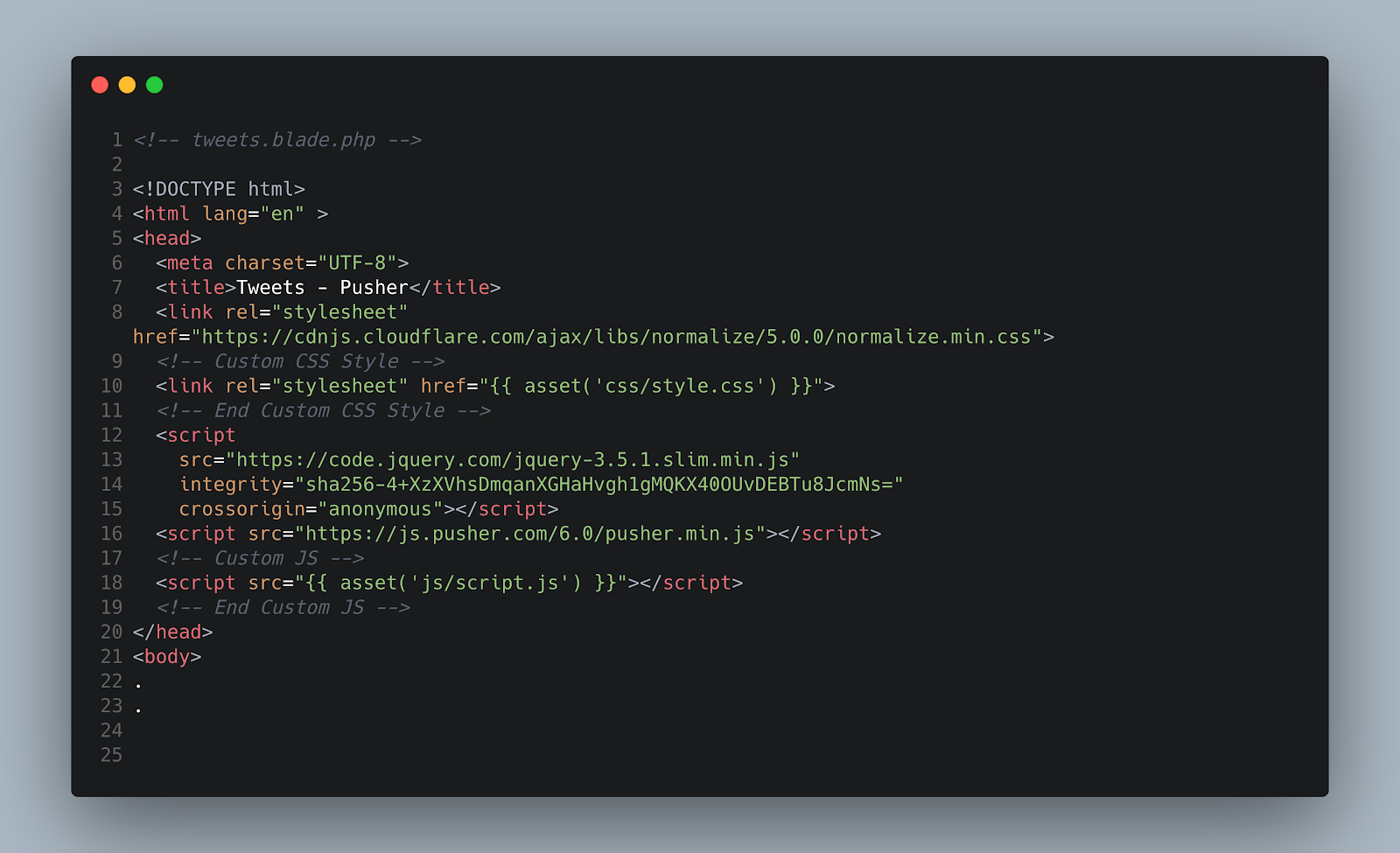
Next in the public/js
folder create a new file script.js
. Copy the code below into your script.js
file.
Make sure to replace the PUSHER_APP_KEY
and PUSHER_APP_CLUSTER
placeholders with the appropriate values as seen in your .env
.
In JavaScript, we have subscribed to the channel “pusher-tweets” and bound the “tweets” event so that any data sent to this channel with this particular event will be available to us directly at client-side in real-time.
With the data, we will be doing some DOM (Document Object Model) manipulation like updating the image, username, name, date, and user reaction.
Awesome! We have now integrated the Pusher channel at the client-side. We will also need to do the same in our Laravel Application in order to broadcast our tweet data.
Broadcasting Tweets Data
Laravel supports the integration of Pusher using Events out of the box. Let’s install the Composer package for Pusher by running the following command:
If we check the config/broadcasting.php
file, we can see that multiple drivers like "pusher", "redis", "log", "null" are supported by Laravel for the broadcasting of events. Let’s set the driver to Pusher by setting this environment variable as follows:
Let’s create an event class named “Tweets”. We can generate the Tweets Class using the following Artisan CLI command:
Open the newly created Tweets.php
file, located in the directory app/Events
. Add the following code:
In the Events Class above, we have defined the channel which our application will publish the events to, the name of the events, and the data that we will be broadcasting. In Laravel, we can broadcast the message simply by using the broadcast()
function.
The next important step is to modify the console command to broadcast the Tweet data.
Open the TweetsStream.php
file in app/Console/Command
directory and add the following code in place of var_dump
at line 63.
Our file TweetsStream.php
will look like this:
Congratulations!. You have completed integrating Pusher with your Laravel Application to communicate Tweet data in real-time. Let’s test it out.
Testing
Open two terminals. One for running the Laravel development server and one for running the console command. Run these commands in each terminal respectively.
Laravel Development server will run at http://localhost:8000
so let’s open the browser and browse http://localhost:8000/tweets
.
Let’s add more hashtags so we can have more data before running our console command. In TweetStream.php
, replace #twilio
with #covid19
.
It will look similar to this:
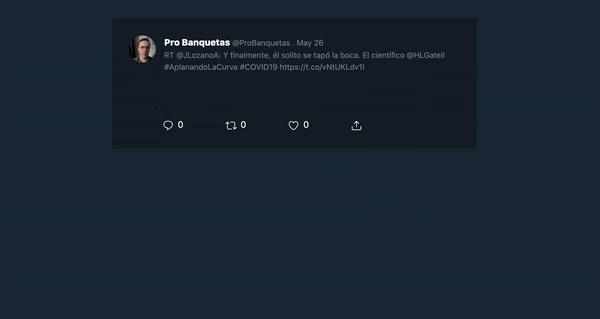
As you can see, our page is now updating with the latest tweets in real-time. We can check the overview page on the Pusher Dashboard for the messages/data that we have sent using pusher.
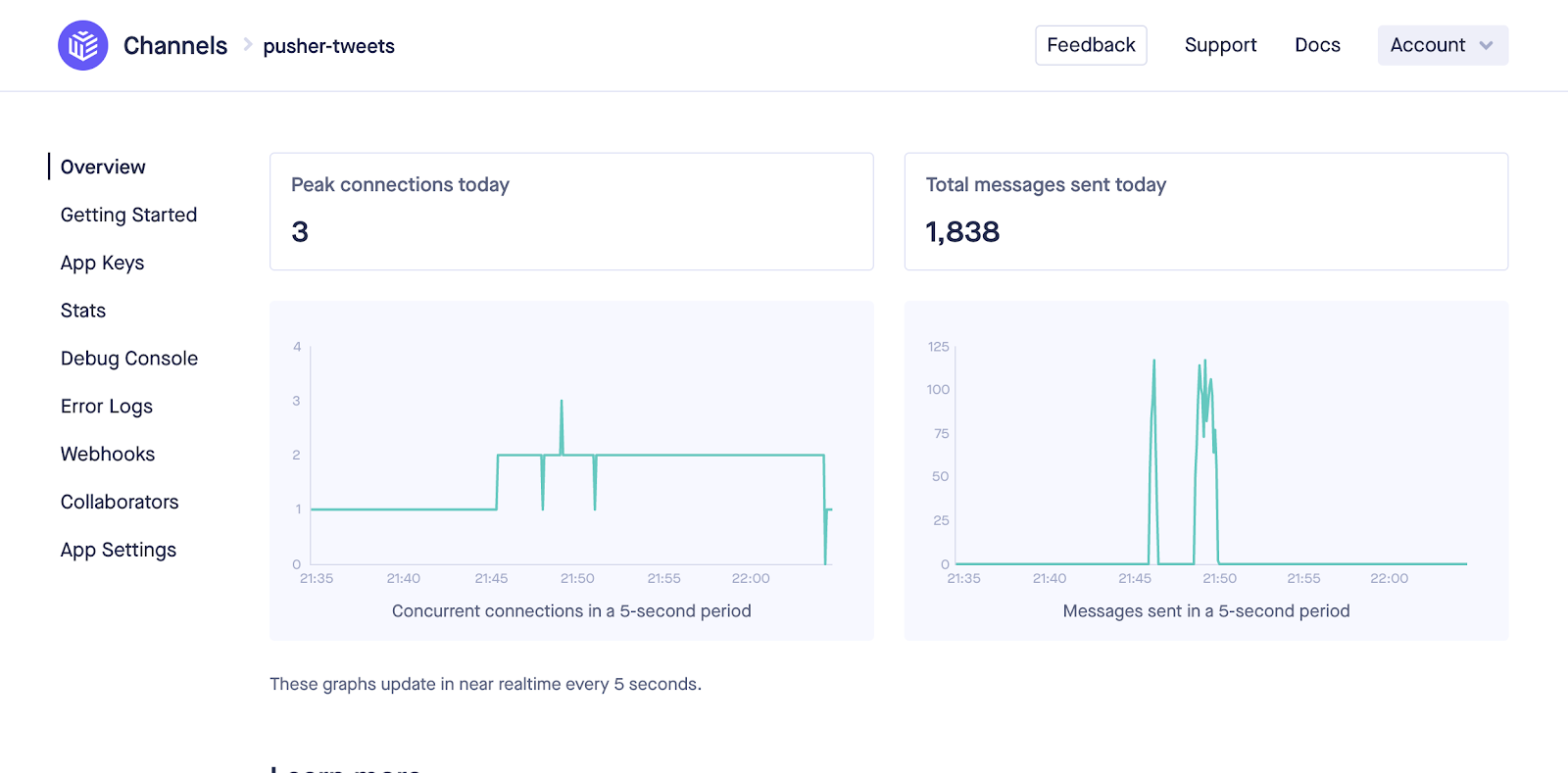
Conclusion
In this article, we learned how to use Pusher for real-time data communication with Laravel. With Pusher, we can do more like add some visualizations and update the graph in real-time, sending messages. We can also extend this to add features like sentimental analysis of user tweets to predict something based on user’s tweets and generate reports.
The complete code for the tutorial can be found on GitHub repo → pusher-tweets
Feel free to comment and ask me anything.
You can reach me at ankitjain28may77@gmail.com and follow me on Twitter @ankitjain28may. You can also read more of my articles on Medium @ankitjain28may.
Thanks for reading!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.