Create Dynamic Data Visualizations in Laravel with D3.js
Time to read: 6 minutes
Create Dynamic Data Visualizations in Laravel with D3.js
In today's fast-paced technological environment, where data flows in from every direction, it's more important than ever to have accessible ways to view and understand this data. One effective way to do so is with dynamic data visualizations.
In this tutorial, you'll learn how to create dynamic visualizations of the top five most populated countries by combining Laravel's exceptional data management capabilities with D3.js's sophisticated visualization techniques.
Understanding Data Visualizations
Data visualization is the practice of converting raw data into graphical or visual formats to make the information more accessible, understandable, and usable; as in the image above. It represents data using standard graphics like charts, plots, infographics, and even animations.
By leveraging your visual perception, data visualization, usually found on dashboards, has become a go-to tool for users to analyze and communicate information. These visual tools help reveal patterns, trends, and insights that might be difficult to discern from raw data alone.
In the world of Big Data, data visualization tools and technologies are important for analyzing vast amounts of information and making data-driven decisions. They provide a powerful means to present complex data to non-technical audiences clearly and effectively.
Benefits of dynamic data visualizations
Dynamic data visualizations provide numerous benefits, ranging from revealing hidden patterns and trends to making complex data accessible to everybody. These visual tools help you make better decisions and foster a data-driven culture, which enables you to act quickly and intelligently.
Let's look at the several benefits that they bring to the table.
- Effective Communication: they clarify complex data for non-technical audiences through interactive charts and graphs, making information more accessible and easier to understand.
- Enhanced User Engagement: they allow users to explore data interactively through hovering, clicking, and zooming, making the data more engaging, and help maintain user interest.
- Improved Decision-Making: By presenting complex data sets interactively, they enable faster and more accurate decision-making, helping organizations respond swiftly to changing conditions.
- Cost and Time Efficiency: Automating data updates, modifications, and dynamic visualizations saves time and money compared to manual data processing and static reports.
- Scalability: they can handle big datasets, making them ideal for enterprises dealing with large volumes of data. They scale as the data grows, maintaining consistent performance and reliability.
Prerequisites
- Composer installed globally
- PHP 8.2 or higher with the PDO extension installed and enabled
- A database tool, such as SQLite's Command Line Shell or the Visual Studio Code SQLite extension, would be beneficial
- Node.js installed
Create a Laravel project
To get started, let's create a new Laravel project. Open your terminal, navigate to the directory where you want to create the project, and run the following command:
Next, navigate to your newly created project by running the command below:
Then, to start your server, run the following command below:
Now, open your browser and navigate to http://localhost:8000. You should see the default Laravel welcome page, confirming that the base application is ready, as shown in the screenshot below.
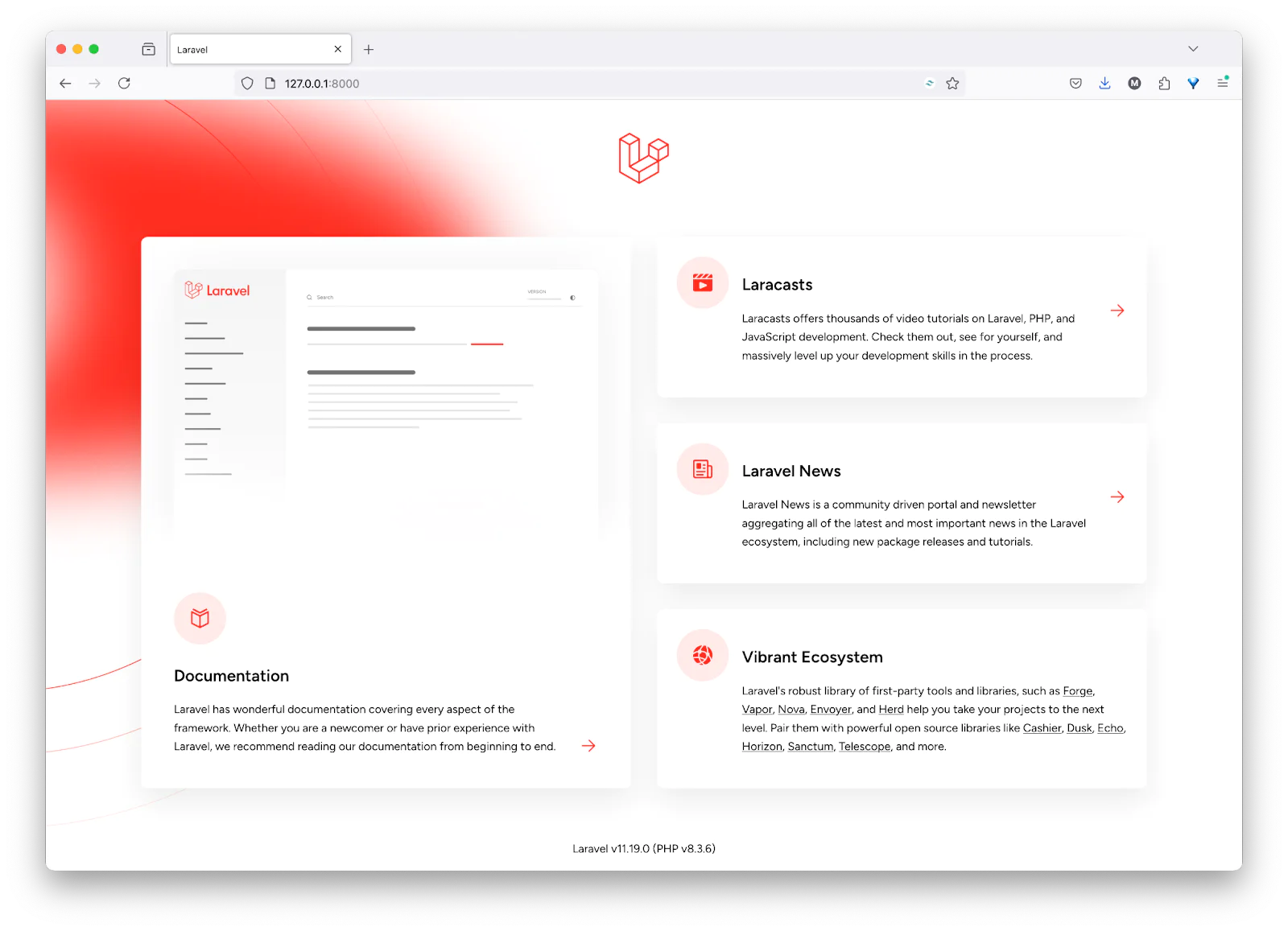
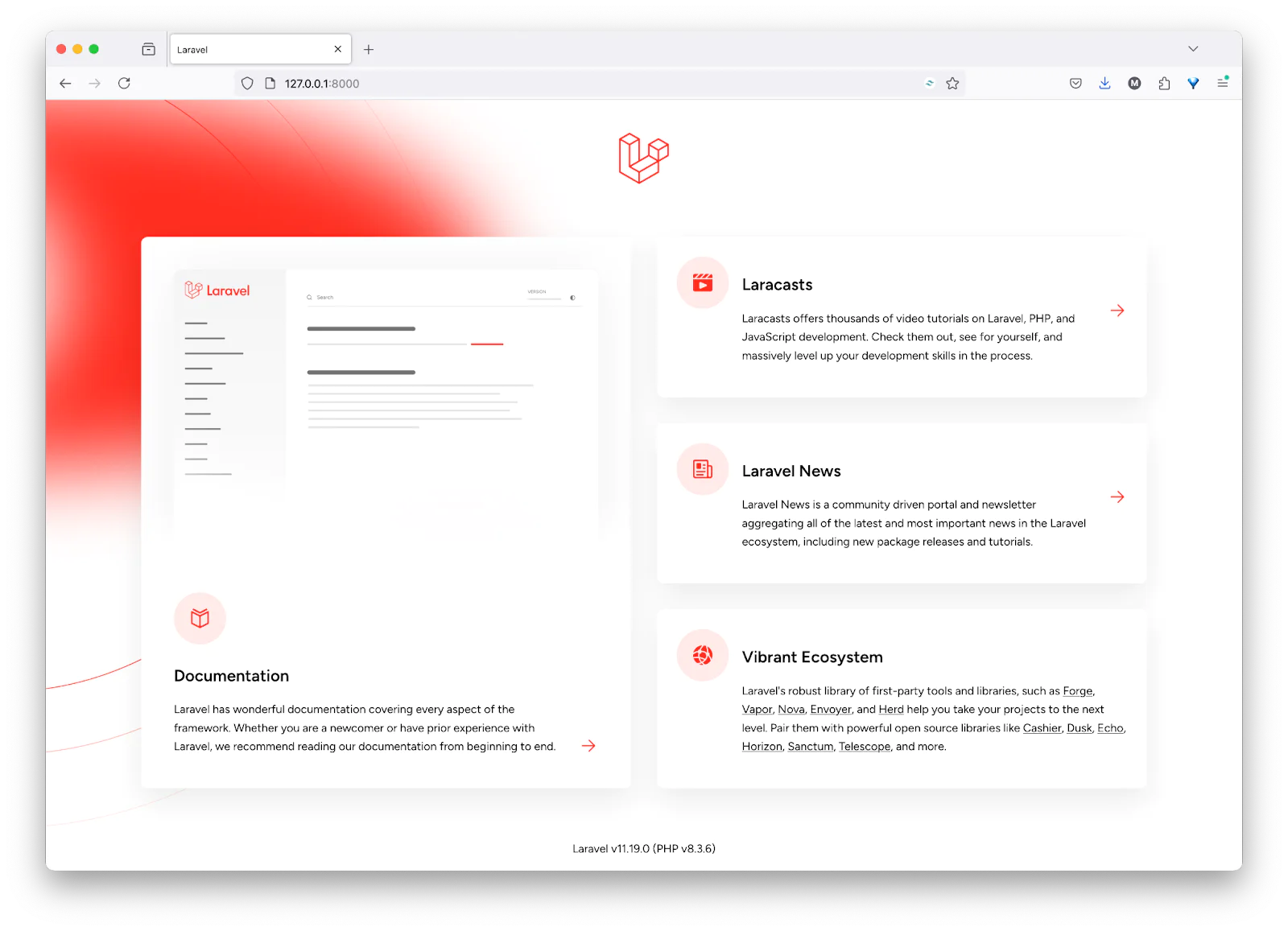
Create a model and migration table
Models and migrations are essential for defining and managing your database schema. The model represents your data, providing a convenient interface to interact with the database, while migrations allow you to create and modify database tables. Migrations are also essential for version control, ensuring your database structure is consistent and more easily manageable across different development environments.
To generate them for a country table, run the following command in a new terminal tab or session:
Next, open the migration file you just created (it ends with create_countries_table.php and is located in the database/migrations directory) and replace its content with the following code:
The code above defines the table structure and outlines the functions for creating (up()
) and dropping (down()
) the table.
Next, run the command below to run the migration:
Now, navigate to app/models/Country.php and update the file to match the code below:
As previously mentioned, the model provides an object-oriented interface for interacting with the database table and ensures that the attributes (color
, latitude
, longitude
, name
, population
) can be mass-assigned, providing security and control over data manipulation operations.
Seed the database
With the database ready to go, let's now use the database seeder to quickly populate the database with sample data of the five top most-populated countries, to provide a consistent starting point.
To create a seeder run the command below:
Next, navigate to database/seeders/CountrySeeder.php, open the file, and update with the code below:
The code above will populate the "countries" table with five records. Each record represents a different country and includes attributes such as name, population, latitude, longitude, and color.
Next, navigate to database/seeders/DatabaseSeeder.php and updated it to match the following code:
The code above calls the CountrySeeder
class within the DatabaseSeeder
so that it will seed the "countries" table with the five records.
Next, run the following command to execute the CountrySeeder
and populate your database:
If you install Visual Studio Code's SQLite extension, you should find this database table in your SQLite Explorer:
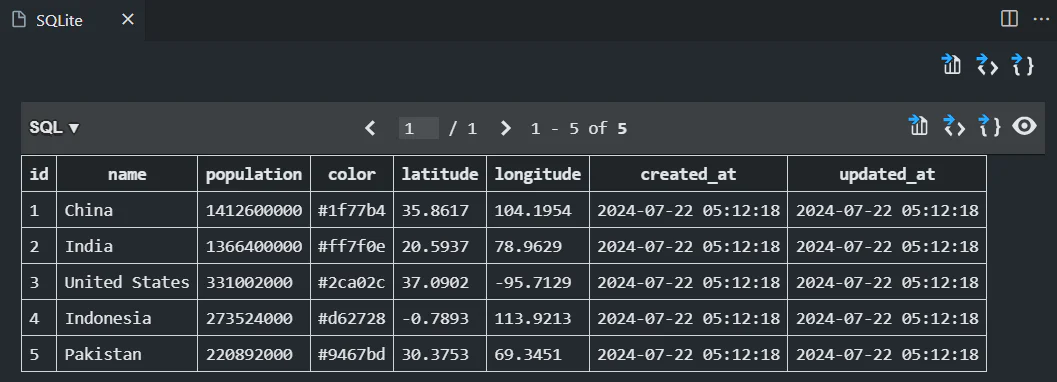
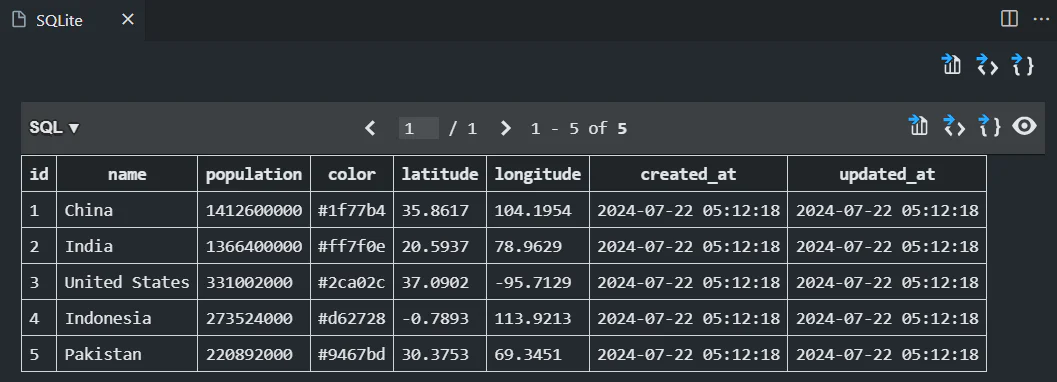
Alternatively, use whatever GUI tool you have installed, or run the following SQL query to confirm that the "countries" table has been seeded:
Create the application's core controller
To generate the controller, run the following command:
Next, open the CountryController.php file you just created (located in app/Http/Controllers/) and update it to match the code below:
This controller is responsible for handling HTTP requests related to the Country model.
Define the routing table
The routing table defines the various endpoints of your application, specifying which functions and views are triggered when the URL is accessed. This routing table is essential for organizing and managing the flow of requests and responses, ensuring that your application can quickly retrieve data, present visualizations, and interact seamlessly with users. It facilitates clear navigation paths, optimizes server-client communication, and maintains a well-structured framework for handling and visualizing data throughout the application.
Now, run the following command to install the API route:
When shown the following prompt, answer with "yes":
Next, navigate to routes/api.php and add the following code to the end of the file:
With this route, when a GET request is sent to the /countries endpoint, the index()
method of the CountryController
is invoked. This method retrieves the specified columns (name
, population
, latitude
, longitude
, and color
) for all records in the countries
table, and returns the data as a JSON response.
Install D3.js
D3.js, or Data-Driven Documents, is a powerful JavaScript package that allows you to create dynamic, data-driven visualizations. D3.js empowers you to craft highly customizable and dynamic data visualizations using Scalable Vector Graphics (SVG), HTML5, and Cascading Style Sheets (CSS).
To get started with the installation process, run the following command:
Create the view
To create the view, navigate to resources/views/welcome.blade.php and update it to match the following code:
Now, let's style the interface. Start by creating a new directory called css within the public directory. Inside this directory, create a new file named style.css. Then, paste the following code into it:
Implement data visualization with D3.js
To integrate D3.js, create a new directory named js within the public directory. Inside the newly created js directory, create a file named chart.js. Then, add the following code to the file:
Next, add the following code to create the pie chart:
The provided code creates a pie chart visualization. It utilizes D3.js for pie layout calculations, arc generators to define slice shapes, tooltips to display additional information on hover, and helper functions that enhance interactivity and improve the visual presentation of data.
Now, add the following code to your chart.js file to create a bar chart:
The code above creates an interactive bar chart visualization using D3.js. It leverages scaling functions ( scaleBand and scaleLinear) to map data to visual attributes, constructs axes to provide context and readability and adds interactive elements (rectangular bars) with dynamic color changes and tooltips to enhance data insights.
Next, add the following code to your chart.js file to create the map visualization:
The code above fetches data and creates an interactive world map visualization. It displays countries and their associated data, including name, population, latitude, longitude, and percentage. The visualization is created dynamically after the data is successfully retrieved.
Test the application
To test the application, openhttp://127.0.0.1:8000 in your browser. You will see the pie chart, bar chart, and map data visualizations as shown below:
That's how to create a Dynamic Data Visualization in Laravel with D3.js
The impact of interactive data visualization is outstanding. It enables you to access valuable information more frequently, enhancing decision-making and minimizing reliance on hunches and guesses in time-sensitive situations.
This tutorial demonstrated how to dynamically visualize the top five most populous countries using pie charts, bar charts, and an interactive map, integrated within Laravel and rendered using D3.js.
This approach can be extended to various datasets and visualization types, allowing you to present data in ways that are both informative and visually appealing. As you continue to explore and utilize these tools, you'll be able to create even more sophisticated and impactful data visualizations. Happy coding!
Lucky Opuama is a software engineer and technical writer with a passion for exploring new tech stacks and writing about them. Connect with him on LinkedIn.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.