How to Create a Customer Satisfaction Survey using Twilio Programmable Voice and PHP
Time to read:
Create a Customer Satisfaction Survey Using PHP and Twilio Programmable Voice
You’ve successfully shipped multiple products and/or rendered services to your customers. But, how do you get rating feedback from them?
We can use Twilio Programmable Voice to do this. Using voice provides reach, because customers may not actively come online to rate us. What's more, with voice we can initiate the feedback process.
Sounds interesting?
Then in this tutorial, we will build a system where we can create questions for which we would like feedback. When called, customers can press digits 1 - 5 on their phones to give us their feedback. In addition, it will have a user interface (UI) to see the feedback.
Prerequisites
For this project, we will need:
- Composer installed globally
- PHP 8.3 or higher, with the PDO and PDO_MySQL extensions installed and enabled
- Access to a MySQL (or MariaDB) database
- MySQL's database client or Navicat
- A Twilio account (free or paid) with a Twilio phone number. If you are new to Twilio, click here to create a free account
- ngrok
Create the core of the application
The first thing to do is to create a new directory for the project and install the required dependencies. So, wherever you store your PHP projects, run the following commands to create the project directory and navigate into it.
Then, install Twilio's PHP Helper Library and PHP Dotenv to enable us to load our Twilio credentials from a dotenv file ( .env, which will be set up next). To do that, run the following command.
Set the required environment variables
Let's add our database and Twilio credentials as environment variables. Saving them there will ensure we can use them anywhere in our application. Create a new file in your project's top-level directory, named .env, and then paste the configuration below into it.
Next, log in to your Twilio Dashboard and obtain your Account SID, Auth Token, and Twilio phone number in the Account Info panel, as seen below.
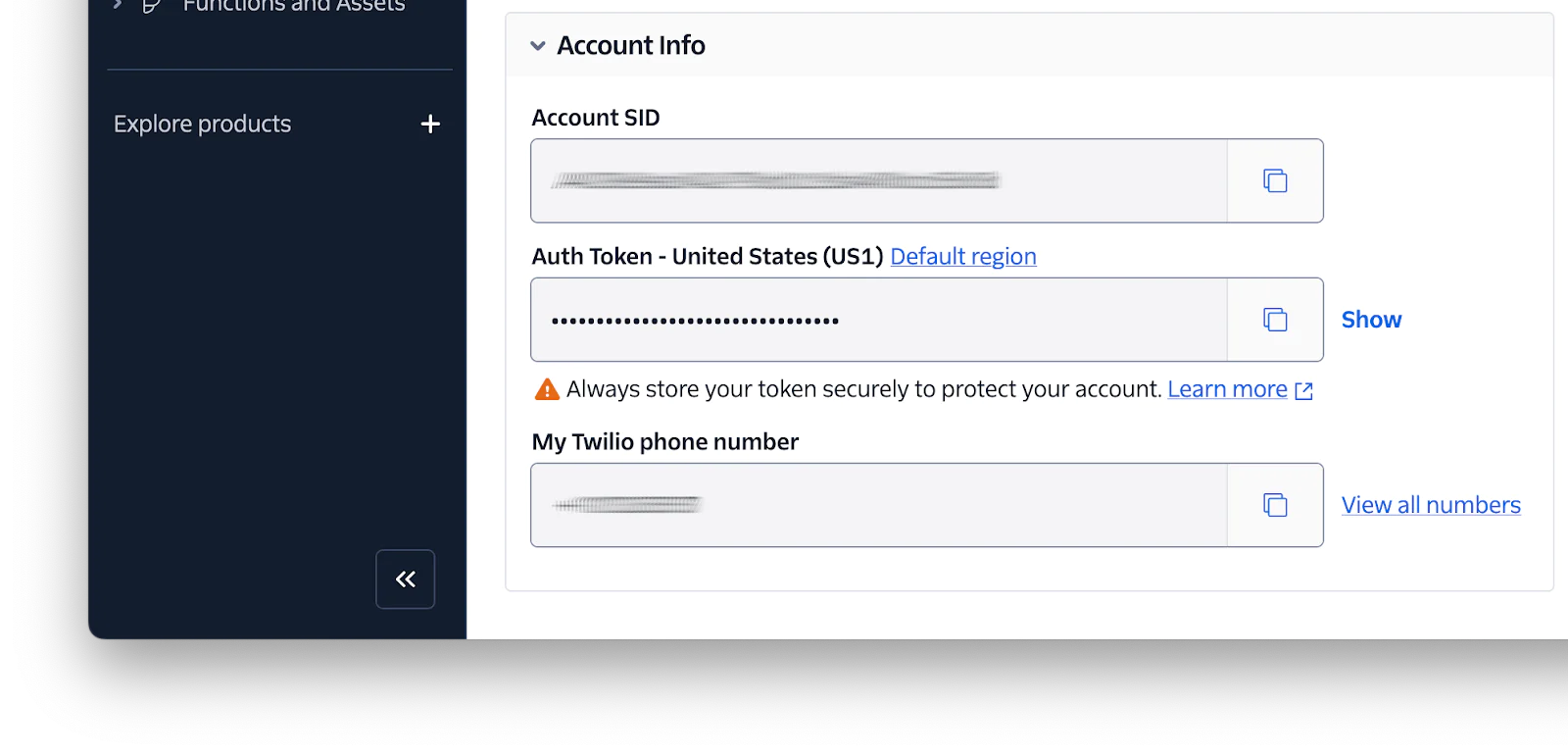
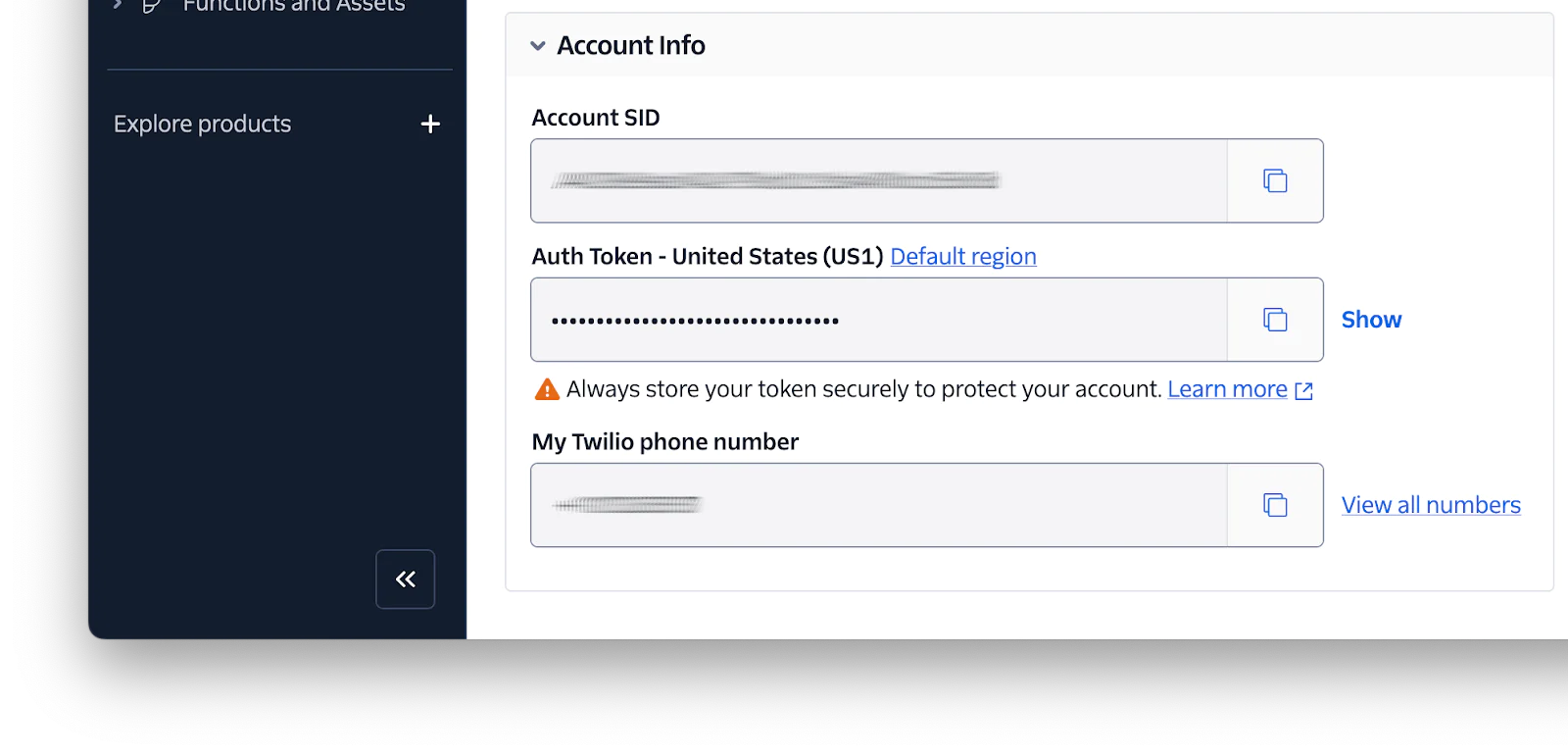
After that, replace <your_twilio_account_sid>
, <your_twilio_auth_token>
, and <your_twilio_phone_number>
, respectively, in .env with your Twilio credentials. Then, update the database variables with your database credentials.
Set up the database
Let's set up our database, using the following SQL commands to create the schema. Run them in your database client of choice.
The above SQL commands create four tables:
- surveys: This table stores information about each survey
- questions: This table stores the individual questions for each survey
- survey_customers: This table stores the phone numbers of customers who will take the surveys
- survey_customers_rating: This table stores the ratings provided by customers for each question in a survey
The tables have the following relationships:
- surveys is our primary table
- questions are linked to surveys
- survey_customers are linked to surveys
- survey_customers_rating links survey_customers, questions, and surveys
Create a database connection
Our database has been initialized, so we can now connect to it. To do that, we will create a Database.php file with the code below in the project's top-level directory. This will load up our database variables and connect to our database.
Set up autoloading for the application's code
Our application will consist of multiple directories and files with classes in line with modern PHP design patterns. To do this, add the following configuration to composer.json file.
The above configuration tells Composer's autoloader how to map namespaces to directories. These directories will be created as we build our project.
Next, you need to update Composer's Autoloader file by running the following command.
Add the application's routing table
To set up routing, create a directory named public in the project's top-level directory, and in that directory create a file named index.php. index.php will bootstrap the application and serve as the entry point of our project, handling requests to URLs in our app.
Once the file and directory have been created, paste the code below into public/index.php.
The code above splits the request URI and deduces the method to execute in the corresponding application controller, which we will create later.
Set up the application's user interface
Next, let’s set up the application's user interface (UI) for creating surveys and adding our customers' phone numbers and questions for which we would like to get a rating.
To do that, create an app directory at the root of the project. Then, inside that directory, create three further directories: Controllers, Models, and views. These will be used to separate concerns in our project.
After that, create a file named layout.php in the app/views directory. This file will contain our UI navigation, and the dynamic content of our pages will be injected into the layout so we do not repeat ourselves. With that done, add the following code to app/views/layout.php.
Next, is the form for creating a survey. In our app/views directory, create a directory named survey; inside that directory, create a file named create.php. This file will contain the form where the surveys will be created.
The form will have the following inputs:
- Title of the survey
- Description
- Our opening message
- Survey questions
- Customer phone numbers
Inside app/views/survey/create.php, paste the following code below.
Now, let's add the following JavaScript to the end of the create.php file to enable us to add survey questions and customer phone number input fields using DOM manipulation.
Add the application's database models
Now, let's create our models, which will have methods to save surveys, questions, and customers' phone numbers to our database.
Create a Survey.php file in our app/Models directory. Then, add the code below to the file.
The above code defines a Survey
class. It has a create()
method containing our SQL statement to save a survey to our database, and a find()
method which uses a survey ID parameter to get a survey by ID from our database.
Now, create a Question.php file in our app/Models directory, and add the following code into the file.
The above code has a create()
method to save questions, and a findBySurvey()
method to find all questions for a given survey.
Now, create a file named SurveyCustomer.php in the app/Models directory and insert the following code into the file.
The above code has a create()
method that saves the customer phone numbers we want to call into our database, and a findByPhoneAndsurvey()
method to help us get a particular customer by phone number and survey ID.
Finally, we need a model to save our customer feedback to a question for a given survey. To do this create a file named SurveyCustomerRating.php in the app/Models directory. Then add the following code to the file.
Set up the survey controllers
Controllers in a web application are responsible for showing the appropriate view, handling user input, and updating models.
Create a SurveyController.php file in the app/Controllers directory to handle our survey routes, and paste the code below into the file.
This class will serve as a handler to show our survey form and handle form submissions.
Add functionality for viewing and creating surveys
In our SurveyController
class, we will add a create()
method to show our create survey form in response to GET requests, by pasting the following code at the end of the class.
The create()
method will save the survey when the form is submitted. After saving the survey, we can initiate phone calls with our customers.
Add the ability to initiate phone calls
First, create a new file named TwilioController.php file in our app/Controllers directory, then paste the code below into the file.
The above code imports and instantiates our database models. We also imported our Twilio helper classes we will need later on.
Add helper methods
Some helper methods, appUrl()
and buildQueryString()
, will be added to our TwilioController
. The appUrl()
method will assist us in generating a complete URL based on the requested URI and the current server environment. The buildQueryString()
method will take in an associative array and return a query param string from the array values.
Insert the following method into app/Controllers/TwilioController.php.
The above code determines the protocol (http or https), gets the domain name, constructs the full URL and returns the URL.
Then, also in app/Controllers/TwilioController.php, insert the following method:
The above code uses PHP's built-in http_build_query function to generate a query params string from an array.
Add the ability to initiate calls
Since our TwilioController
will handle all our Twilio actions and routes, we will create a initiateMultipleCalls()
method that will call our customers. The /twilio/voice
route will be used to handle when they respond. We will also pass the customer's phone number and survey ID as query parameters; they will be used when handling the call.
In our TwilioController
, let’s add a method to initiate the calls which will require two parameters: the survey ID and our customers' phone numbers
With our initiateMultipleCalls()
method created, we will add it to the create()
method of our SurveyController
. We will first import our TwilioController
into SurveyController
.
Then, our initiateMultipleCalls()
method will be added just after the code block for saving the survey and customers' phone numbers. We will pass the survey ID and phone number to the initiateMultipleCalls()
method. To achieve this, replace the create()
method in the SurveyController
with the following definition.
With the initiateMultipleCalls()
added, our create()
method in SurveyController
should be like the above code.
Handle when a customer answers
When the customer answers, we need to send them our opening line and add an action URL to gather their feedback on whether or not they want to proceed with the survey. Using the survey ID from the GET request, we will query our database for the opening message for a particular survey.
Add the following voice()
method to our TwilioController
with an action URL to gather the feedback.
From the above code, $response->gather()
uses an associative array to specify what response we want from our customers when they answer; 'numDigits' => 1 and 'input' => 'dtmf'
signified that our customers are required to answer by pressing digits on their phone.
The digit response will be processed by our action URL /twilio/gather
, where we also send some data via query params. Using the $this->buildQueryString()
, we created a query string containing the survey ID, customer phone number, and a boolean indicating it is the opener.
Add the ability to gather feedback
Let's see how we can gather our customer input during the call. We will start by creating a feedback gathering method named gather()
, for our action URL (/twilio/gather
) in our TwilioController
. It will handle two application states:
- Opening message response
- Customer feedback for a question
Opening message response
If it's the opening question, which is indicated by the "opener" key in the $_GET
array, we check if the user pressed 1 or 2. Pressing 2 closes the call, while 1 redirects them to our first question via a URL.
Insert the following code in our TwilioController
to handle the opening message response.
The gather()
method retrieves the customer response, i.e., which number they pressed on their phone, then handles if they want to proceed or not. If they proceed, we will redirect them to the first question.
Handle redirect to a question
Our question redirect handler will get our questions from our database for the given survey using the survey ID in the query parameters. We will also get the current question to ask using the question index from our query parameters. When our customer hears our question, our action URL (/twilio/gather
) we created earlier will handle their feedback when they answer the question with a digit from 1 - 5.
Add the following question()
method to the TwilioController
. The method will pick the appropriate question and use the $response->gather()
to handle our customers' feedback.
Gather customer feedback for a question
Now we'll update the gather()
method to handle subsequent customer responses to questions they hear. It will first save the digit they entered, which serves as a rating to the database, and then redirect to the next question. We will also check and close with a message if they are at the last question.
The following code will replace the gather()
method of our TwilioController
. It will now contain the block to be executed when we are processing a question that is not the opener.
The new code can be seen at the else
block of the if ($opener == '1') {
statement.
With the second part of our gather()
method, we can now see the complete process for handling our two states: the opener and subsequent question responses. We can now run the full process of creating a survey via the form and initiate phone calls.
View survey and customer feedback
We will need where we can see the surveys we created, our customer phone numbers, the questions, and their feedback. Let's add some methods to query our database for this purpose in our Models directory.
In app/Models/Survey.php add the following method to query the database for all surveys.
Then in the file app/Models/SurveyCustomerRating.php create a new method named findBySurvey()
to get our customer's feedback ratings from our database for a particular survey. Include the following code which gets the customers' ratings and the questions asked for a given survey.
Create the UI to view the surveys
We have our SQL queries ready, so we will need methods in our SurveyController
to get our queried data, using the methods created prior, into our UI templates.
Create an index()
method in our SurveyController
, by pasting the code below into the class.
This method will get all our surveys, which will be used to generate the content for an index.php view file we will create.
From the above method, using ob_start()
before including the index.php file will hold the generated content in an internal buffer. The ob_get_clean()
function then captures the buffered content and stores it in the $content
variable, which also ends output buffering. Then, our content is inserted into our layout.php file.
Let’s create an index.php view file in app/views/survey directory that will contain an HTML table. Since this file has access to the surveys, we will use php foreach to loop through our survey records, each iteration will return a table row containing the survey attributes. Add the following code to the file.
Create the UI to view the feedback responses
From the content in our index.php, every survey in our table has a link generated with the survey ID to view our customers' feedback responses. Let’s create the resulting page by following how our index.php was generated.
We will create a responses()
method in our SurveyController
to handle the route when we want to see the feedback responses for a given survey. Then, we will create a response.php file in our app/views/survey directory, which will receive the rating data, loop through each rating and render it in a tabular form.
Add the following method to our SurveyController
in the app/views/survey directory.
Create a response.php in our app/views/survey directory and insert the following code.
Test the application
To test the application, ensure the application is running, if not start the application by running the following command.
Now, you need to make the application publicly accessible over the internet. Do that by running the ngrok command below in a new terminal session or tab.
With the application running and accessible, follow the steps below to create, test, and view the responses for your survey.
- Check your terminal for the generated ngrok Forwarding URL, then copy and paste it into your preferred browser.
- Navigate to the survey creation page by appending
/survey/create
to the ngrok URL (e.g.,<<ngrok_url>>/survey/create
). - Fill out the survey form, including your mobile number in the customer's phone input, and submit it.
- You will receive a call. Answer the call to hear the opening message.
- Press 1 to accept the survey request
- The application will move to the questions you added during the survey creation.
- Answer each question with digits 1–5 on your phone. At the last question, the application will respond with a thank you message, and end the call.
- Once the call is over, navigate to
<<ngrok_url>>/survey
to view your created survey and any subsequent ones. - There is a "View Responses" button for each created survey. Clicking it will take you to a page displaying the questions and responses you provided.
Troubleshoot the application
If you are not getting your customer's response, use this Twilio help guide for further troubleshooting.
That's how to create a customer satisfaction survey using Twilio Programmable Voice and PHP
We have seen how to build a customer satisfaction survey using Twilio's Programmable Voice API and PHP. The code repository is available here.
You can reach me, Godwin Agedah, on the following channels: Email: agedah99@gmail.com, Twitter: @GodwinAgedah, and Github: Godwin9911.
Survey icon in the post's main image was created by Uniconlabs - Flaticon.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.