How to Check your Twilio Account Balance in PHP
Time to read: 3 minutes
How to Check your Twilio Account Balance in PHP
Through a large array of APIs, Twilio makes it easy to add a broad array of communication functionality to your applications, such as SMS, MMS, and email. But, to use that functionality, your account balance must be in credit.
You can check your account balance at any time via the Twilio Console. After logging in to the Twilio Console, click Admin in the top right-hand corner, then click Account Admin > Account Billing.
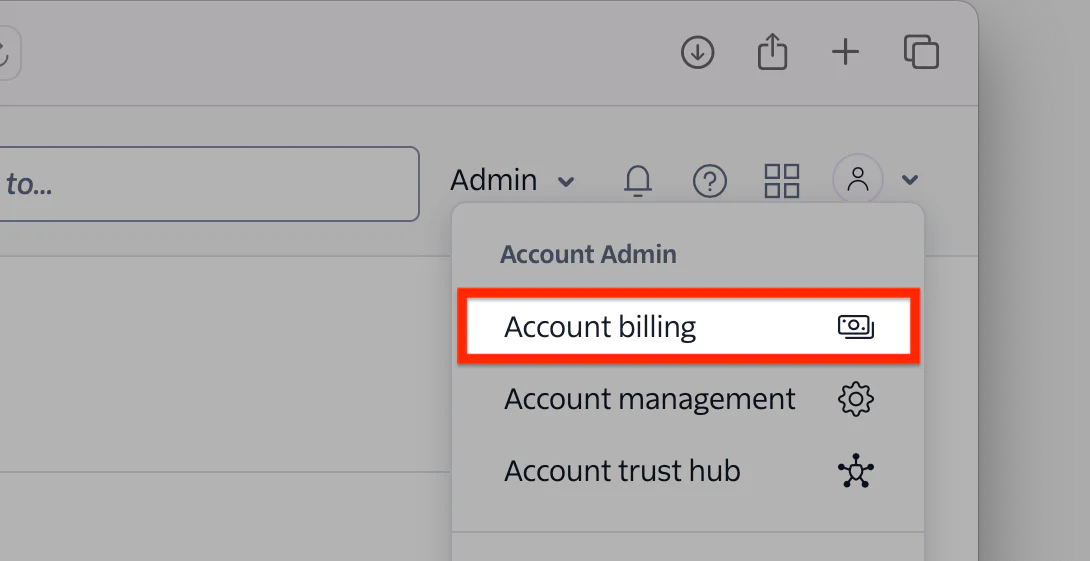
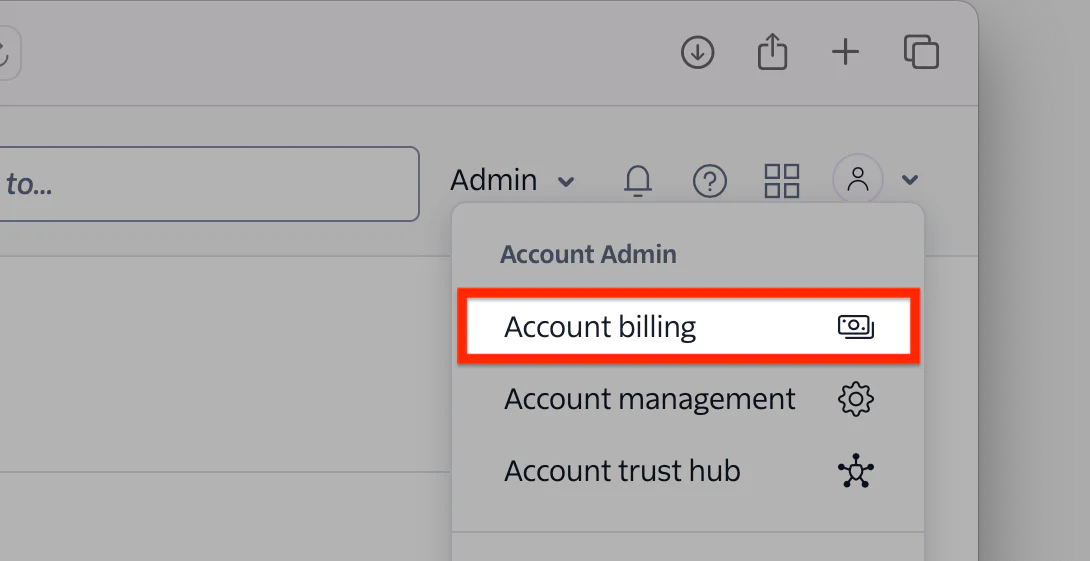
While this is good for checking occasionally, doing this each time is both manual and quite inconvenient. Gladly, you can keep track of your account balance via an API call.
In this short tutorial, you'll learn how to do so in PHP so that you can add automated account balance monitoring to your applications.
Prerequisites
To follow along with this tutorial you'll need the following:
- A Twilio account (whether free or paid); if you don't have one, get a free account
- PHP 8.4 with the Intl (Internationalization) extension
- Composer installed globally
Set up the project
Let's begin by creating a project directory and changing into it by running the commands below in your terminal:
Then we'll add the third-party packages that we'll need; these are:
- Twilio's Helper Library for PHP: This greatly simplifies interacting with Twilio's APIs
- PHP dotenv: This loads environment variables (such as credentials and other code configuration details) from dotfiles, such as .env, into PHP's $_ENV and $_SERVER superglobals
To add them, run the command below.
Write the PHP code
Now, it's time to write PHP code. Open your preferred IDE or text editor, create a new PHP file named index.php, and in it paste the code below:
The code starts by using PHP dotenv to load the environment variables in a file named .env (which we'll set up shortly) into PHP's $_ENV
and $_SERVER
superglobals. If TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
are not defined and set, it will throw an exception.
Then, it initialises a new Twilio\Rest\Client
class with your Twilio Account SID and Auth Token. This object is the central class in the PHP Helper Library that coordinates requests to Twilio's APIs.
After that, it retrieves the account's balance (internally making a call to the account's Balance resource) and formats it using the NumberFormatter class, rounding the amount to three decimal places for better readability.
Retrieve your Twilio credentials
The final thing to do is to retrieve your Account SID and Auth Token from your Twilio account and store them for the code to load as environment variables. To do that, create a new file named .env in the top-level directory of the project. Then, in that file paste the following code:
Now, log in to the Twilio Console and, in the Account Info panel, copy your Account SID and Auth Token. Paste the two values into .env in place of <TWILIO_ACCOUNT_SID>
and <TWILIO_AUTH_TOKEN>
respectively.
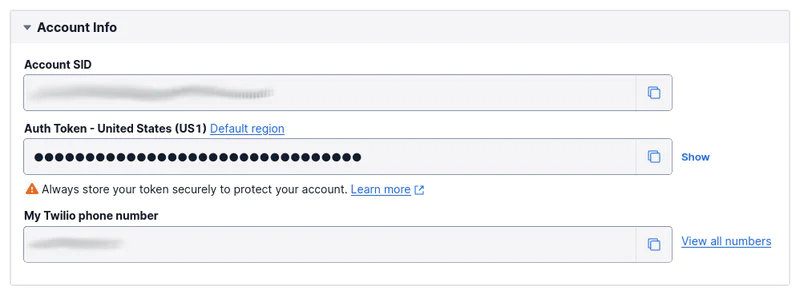
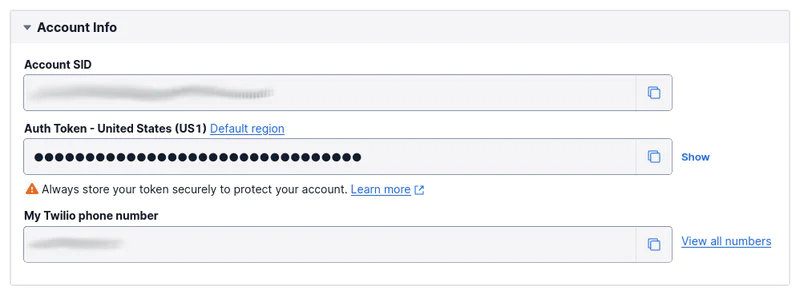
Run the code and retrieve your account balance
With everything done, you're ready to retrieve your Twilio account balance. To do that, run the following command:
You should see output similar to the below:
That's how to retrieve our Twilio account balance with PHP
Thanks to Twilio's Helper Library for PHP, there's very little that you have to do, making it trivial to integrate automated account balance checking into your application. If you were so inclined, you could refactor it to notify you via SMS if the balance falls below a defined amount.
I hope this improves your experience integrating with Twilio.
Matthew Setter is a PHP and Go editor in the Twilio Voices team, and a PHP and Go developer. He’s also the author of Mezzio Essentials and Deploy with Docker Compose. You can find him at msetter@twilio.com. He's also on LinkedIn and GitHub.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.