Call CSVs from REST API script
Time to read: 6 minutes
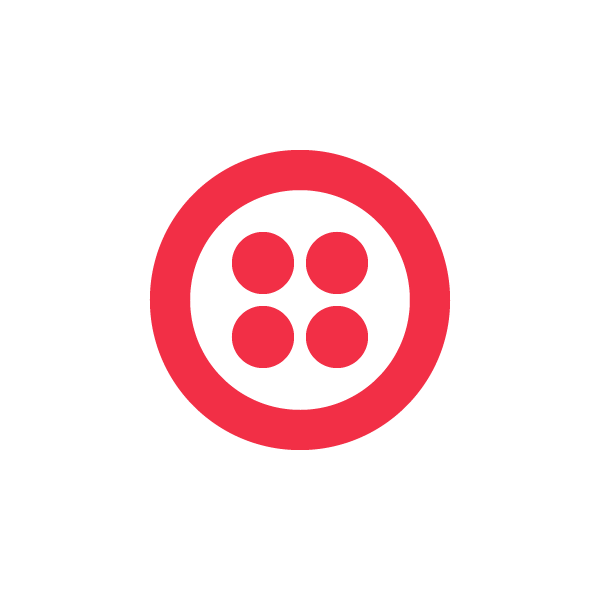
A customer asked today if our Voice API can provide call records in a CSV format. It’s a perfectly reasonable request, and something we could clearly support in the future. But in the meantime, I put together a quick PHP script to pull XML call records from our REST API, and write a CSV to stdout.
/*
Copyright (c) 2008 Twilio, Inc.Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the “Software”), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED “AS IS”, WITHOUT WARRANTY OF ANY KIND,EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
# relies on the TwilioRest Helper Library located at
# http://www.twilio.com/resources/sdk/twilio-rest-sdk-php-1.0.tar.gz
require “twiliorest.php”;
function usage($msg = null) {
if(strlen($msg))
echo “$msgn”;
echo “Usage: calls-to-csv.php –account-sid accountsid –auth-token authtoken [–caller caller-phone-number] [–called called-phone-number] [–records #]n”;
echo “Writes a call-record CSV to stdout in the format:n”;
echo “CallSidCallerCalled StartTimeEndTimeDurationCostn”;
die;
}
// init num pages to fetch
$records = 50;
// start list of URL Query String params to send with the request
$params = array();
array_shift($argv);
while($arg = array_shift($argv)) {
switch($arg) {
case “–caller”:
$params[“Caller”] = array_shift($argv);
break;
case “–called”:
$params[“Called”] = array_shift($argv);
break;
case “–records”:
if(!is_numeric($records = array_shift($argv)))
usage(“Invalid number of records: $records“);
break;
case “–account-sid”:
$accountSid = array_shift($argv);
break;
case “–auth-token”:
$authToken = array_shift($argv);
break;
default:
usage(“Unknown parameter: $arg“);
break;
}
}
// validate accountsid and authtoken were given
if(!strlen($accountSid))
usage(“AccountSid is required”);
if(!strlen($authToken))
usage(“AuthToken is required”);
// calculate the # of pages with 50 each
$pages = ceil($records / 50);
// create Rest Client
$client = new TwilioRestClient($accountSid, $authToken);
// output a header row
fputcsv(STDOUT, array(“Sid”,“Caller”,“Called”,“StartTime”,“EndTime”,“Duration”));
// for the number of pages, fetch and output csv
for($i=0; $i<$pages; $i++) {
// add the desired page to the Query String
$params[“page”] = $i;
// do the request and parse the XML response
$response = $client->request(“/2008-08-01/Accounts/$accountSid/Calls”, “GET”, $params);
if($response->IsError)
die(“Error talking to Twilio API: {$response->ErrorMessage}“);
// output as CSV the desired params
foreach($response->ResponseXml->Calls->Call AS $call)
fputcsv(STDOUT, array($call->Sid, $call->Caller, $call->Called, $call->StartTime, $call->EndTime, $call->Duration));
// if we’ve reached the total number of pages, break prematurely
if((int)$response->ResponseXml->Calls[‘page’] == (int)$response->ResponseXml->Calls[‘numpages’]-1)
break;
}
?>
- Download the script (copy and paste)
- Download the Twilio Helper Library for PHP here, if you haven’t done so already. Put in the same directory as the above script, or modify the script above to point to the location of the twiliorest.php file on your server
- Run the script with the named arguments. For help, just run with –help flag. The –account-sid and –auth-token are required args, for obvious reasons. Other args are optional.
- You’ll probably want to make a file of the CSV, just redirect standard out to a file: php calls-to-csv.php –account-sid XXX –auth-token XXX > my-calls.csv
Have fun!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.