Building Video Chat with IBM Watson, Twilio Video, and WebRTC
Time to read: 2 minutes
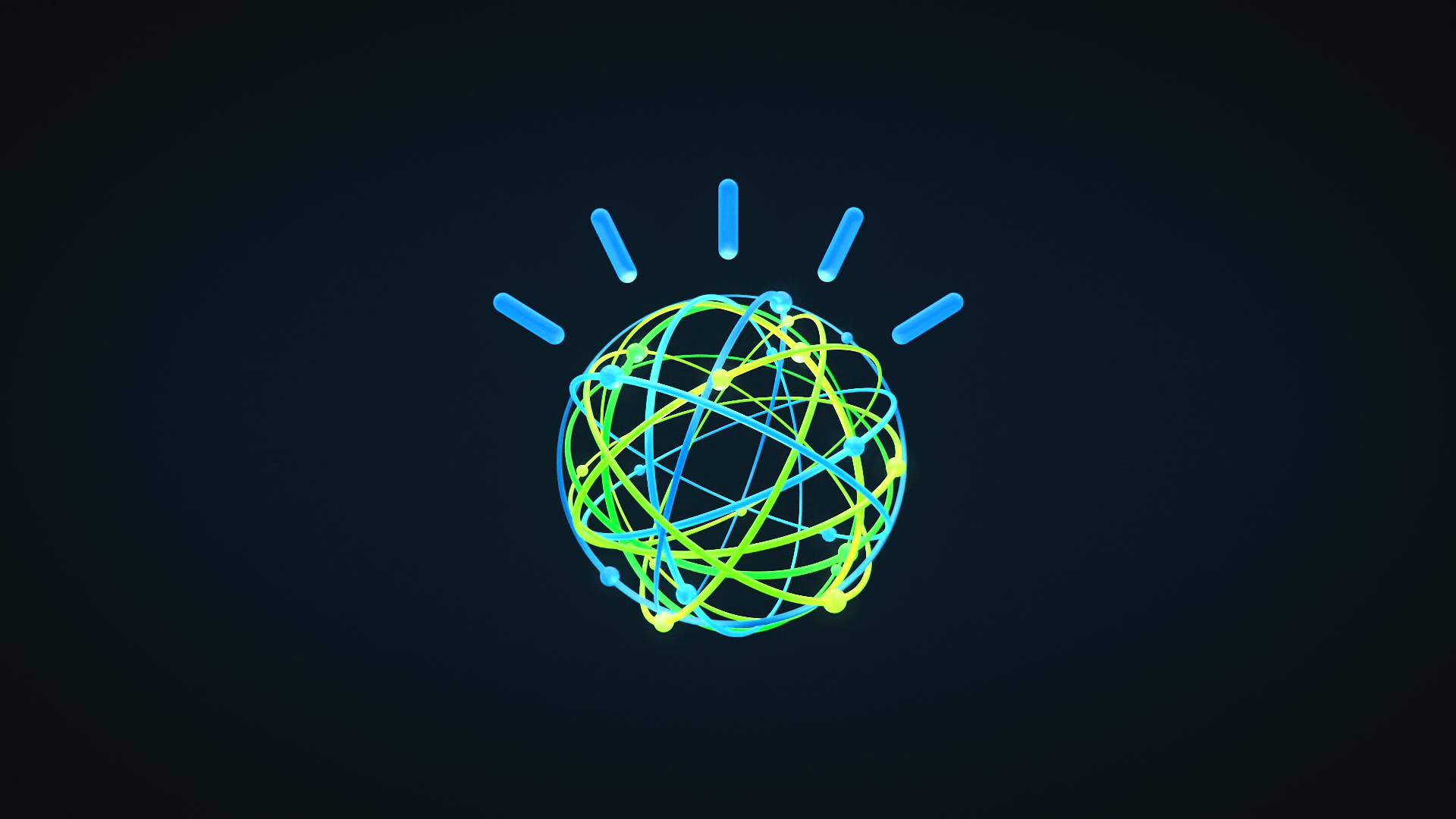
In Houston,TX patients drive from all over the state to receive treatment at a leading cancer clinic. Some patients commuted six hours each way. Last year, that cancer clinic completely eliminated their patients’ commute, letting patients communicate with doctors from the comfort of their own home via video chat.
IBM Developer Advocate, Jeff Sloyer, shared the story of the Texas Clinic at last year’s Kranky Geek Conference. Sloyer breaks down how the component parts of video chat work together, covering IBM Bluemix, Twilio Video and WebRTC integration.
Jeff live-demos the app and runs through everything from setting up a video call to initating a websocket connection from Node to IBM Watson in the following video.
If you’re interested in seeing what you can do with IBM Bluemix and Twilio, catch us at the IBM Interconnect Conference at Booth #158. We’ve also got a ton of technical talks showing how developers can easily embed communications into their apps. That schedule is right here.
Building Video Chat with IBM Watson, Twilio Video, and WebRTC
Read Jeff’s full breakdown of the app on the IBM Bluemix blog. Jeff explains how WebRTC comes into play here:
WebRTC basically abstracts all this really low level and complex tech into simple and reusable API’s. While WebRTC has its issues right now (most maturity and adoption) its an amazing technology that aims to make video conferencing a breeze.
With WebRTC you can basically call someone with the following lines of Javascript
Honestly, just that and you can setup a video call! What if we wanted to take it a step further and allow someone to call us? Well, we already did that, the function above endpointConnected gets called when someone calls us to a video chat. So lets look at that:
With the above code we are basically waiting for someone to call us; in tech terms, it’s an invite. When the invite comes through, we accept the invite prompt in the browser then the conversation is started. Again, basic WebRTC stuff here.
Next we need handle the event when the conversation actually starts. Below is the code:
Wow, only 3 lines to actually connect the video call, crazy! If you tried doing this with a VoIP infrastructure you would literally hate your life and have lots of gray hair. In the above function there are 2 function calls, showLocalVideo and showRemoteVideo. These functions basically attach the video and audio streams to a DOM element in your webpage.
Attaching the local stream (if you called someone then you are the local stream) is pretty simple. See below:
Yes, I know the above selector uses an id and it’s incredibly bad but it exemplifies a point that you need to attach the video to a specific element in the DOM.
OK, so cool we have the local video showing, let’s show the remote video in the browser as well. To do this we can use the following code:
Basically the above code attach’s the remote audio and video to an element in the DOM.
So all in all let me count it out real quick… It’s only 26 lines of code to setup a video call through your web browser. Again, if you did this through conventional VoIP means you probably would not have any hair left at this point, that stuff is really really really hard to setup; let alone maintain.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.