Building Daily SMS News Notifications with NodeJS
Time to read: 6 minutes
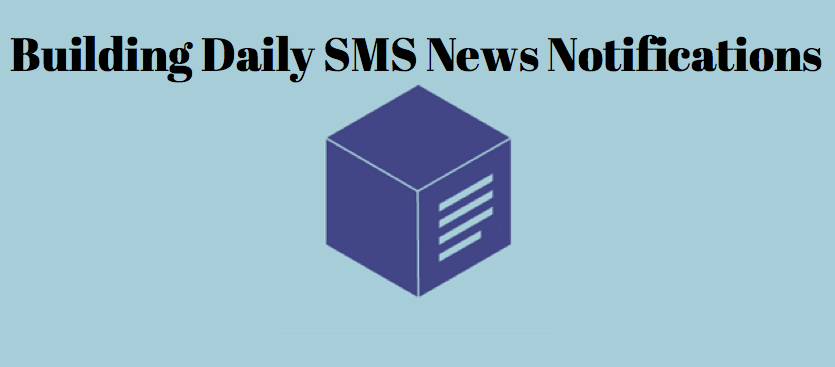
First they showed you how to build SMS weather alerts in Node, now the boys from Stamplay are back showing you how to build a daily SMS news service, of course, in Node.
Stamplay helps automate development steps that you’d have to otherwise have to code. Let’s dive into the tutorial, the code, and all that code you’re saved from writing.
Read the original post right here.
Building An SMS News Notification Service with Twilio and Stamplay
Our service will work in the following steps
- A user sends an SMS to a number provided by Twilio to subscribe to our service or they can just provide their phone number from a web page.
- A Stamplay Task will catch the SMS using the Twilio integration and store a subscription record in the database. After the subscription is stored a confirmation message is sent back to the user.
- A Scheduled task will run once per day and trigger a Code Block who will process the subscriptions. For every active subscription the Code Block will call another code block in charge to fetch the news that will be sent to our user.
- A final task will execute once the Code block execution is complete, and using the output to send the daily message to the subscriber.
Try it out by sending a text to +1 617-863-2088, with the word “subscribe” to get daily inspirational quotes. You can also unsubscribe by texting “stop” or ask for quotes on demand with “quote”. Also a Web UI to allow users to subscribe for the service by with an online form is available at https://inspirationbot.stamplayapp.com.
Connecting Twilio
Signup for a Twilio account and then navigate to your Account Settings to get your API credentials, your AccountSID and AuthToken. You can find them here.
Inside your Stamplay app configuration, on the left hand side select Tasks then components. Choose the Twilio component within the list.
Next inside the Twilio component configuration, add the Twilio credentials to their corresponding fields.
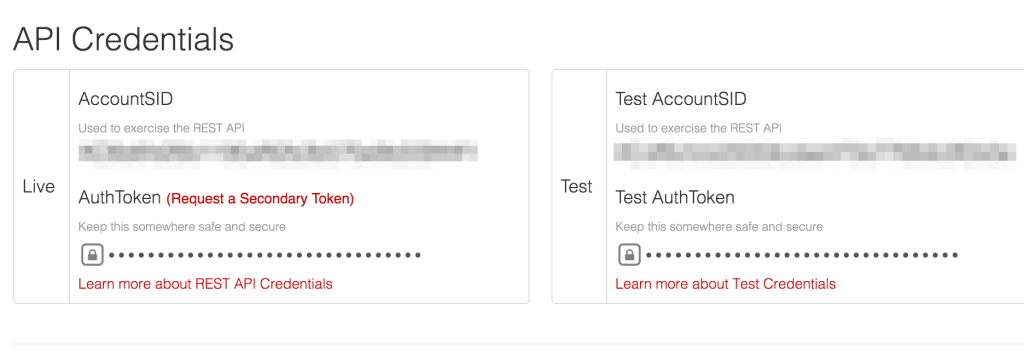
Keeping Track Of Phone Number Subscriptions
We need to keep track of the phone numbers who subscribe to our service, to do this we’ll use the Object component that allow us to easily store data in the cloud.
Inside your Stamplay app configuration, on the left hand side select Objects then “+ New”. Type “subscription” as object name and then add the following properties:
- number as a string
- status as boolean
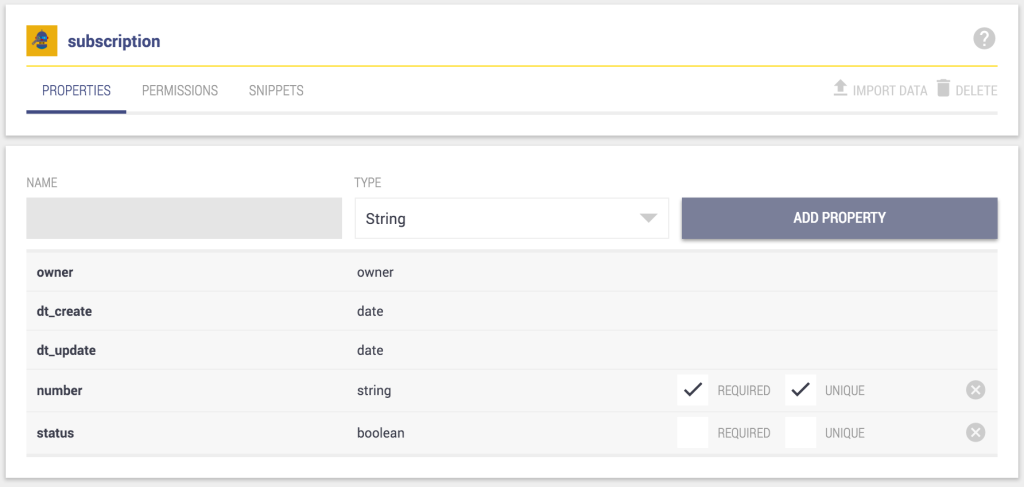
After this one let’s build the database to store the quotes that we’ll send once a day to our subscriber, for this we’ll create a new object called “quotes” with the following structure:
- quote as a string
- author as a string
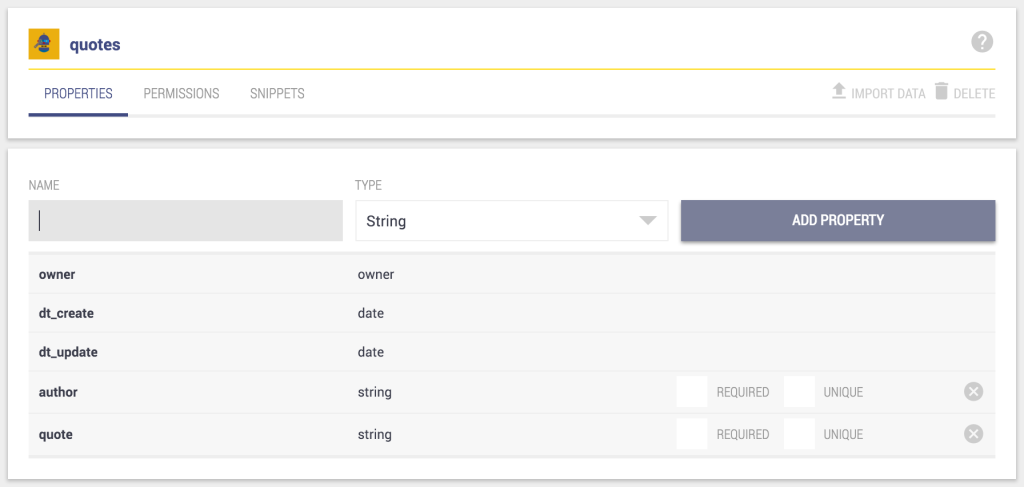
Building Subscription Functionality
Let’s build the first task that will create an instance of Subscription when a SMS that contains “subscribe” will be sent to our Twilio number. Go on “Task” and then “Manage” and start configuring the automation as follows:
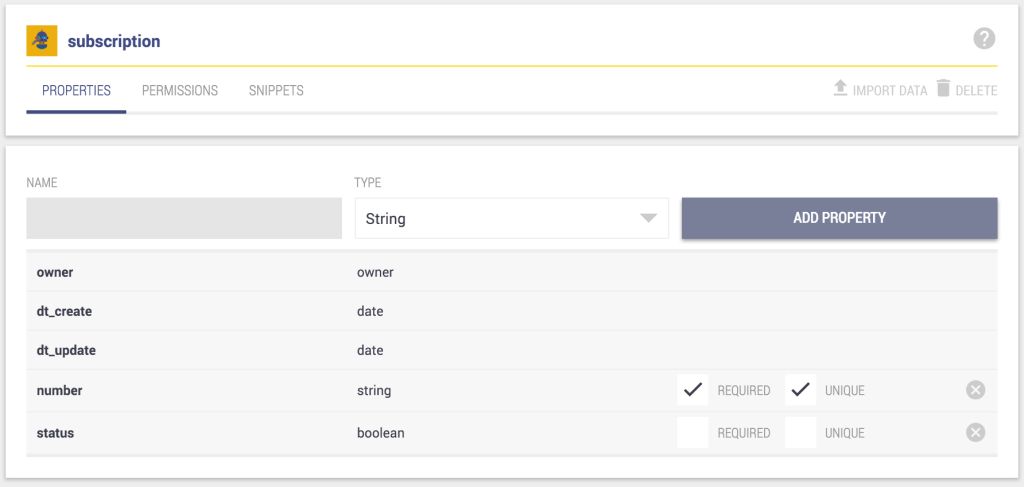
Select a number from the dropdown you wish to trigger this Task when the number selected receives an SMS.
When you created a Twilio account, you receive a Twilio number. You can see your numbers here, if you do not have one then add one.
Below the dropdown where we selected the Twilio number, copy the input field containing the callback url and head over to your Twilio phone numbers page.
Select the number you used to trigger the Task in Stamplay.
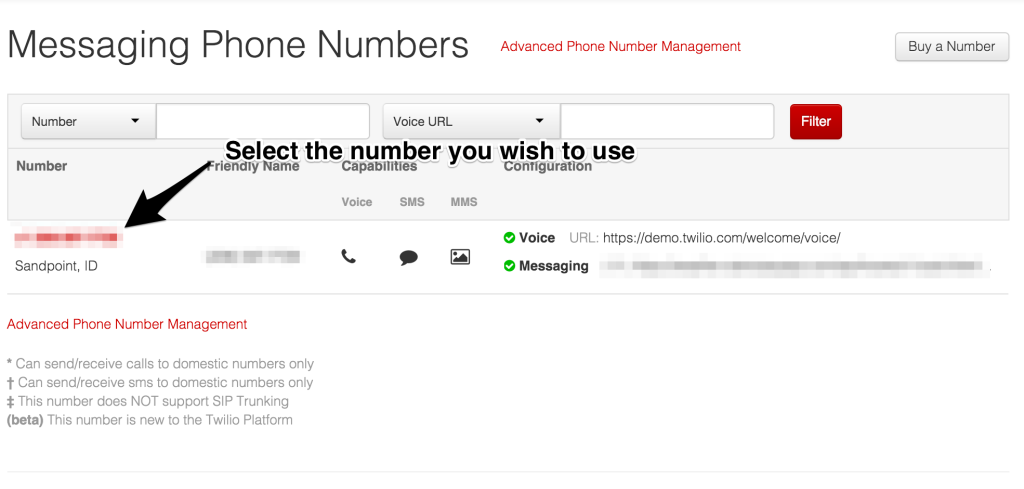
Then add the callback url into the Request URL input field:
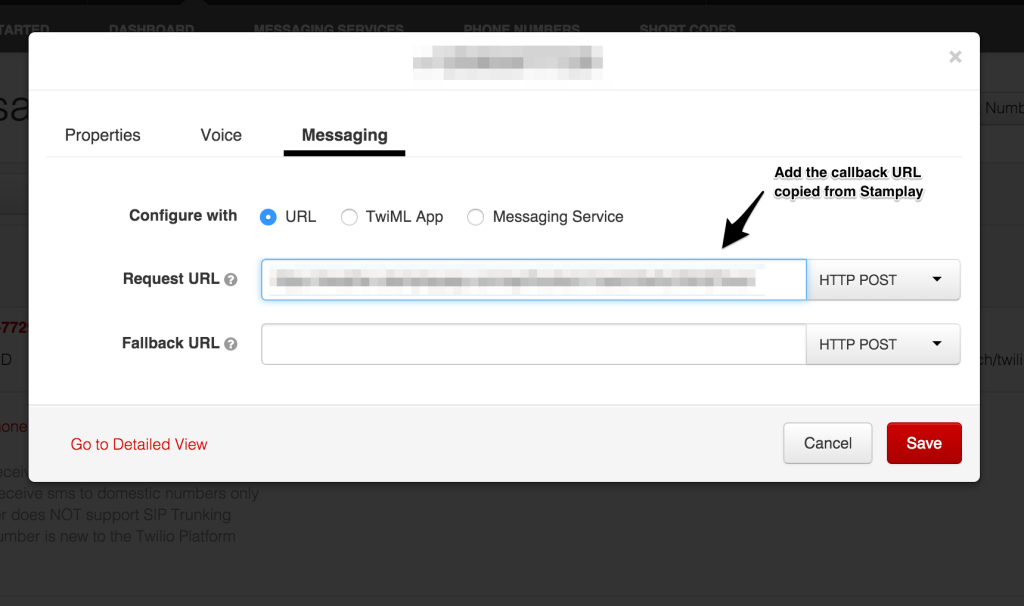
Now back in Stamplay, we need to finish our Task setup. Proceed to Step 3 where we’ll select “subscription” as the type of object that we want to create, “status” will be set to “true” and the number will be dynamically taken from the trigger by using the Handlebars-like signature {{sms.body.From}}”
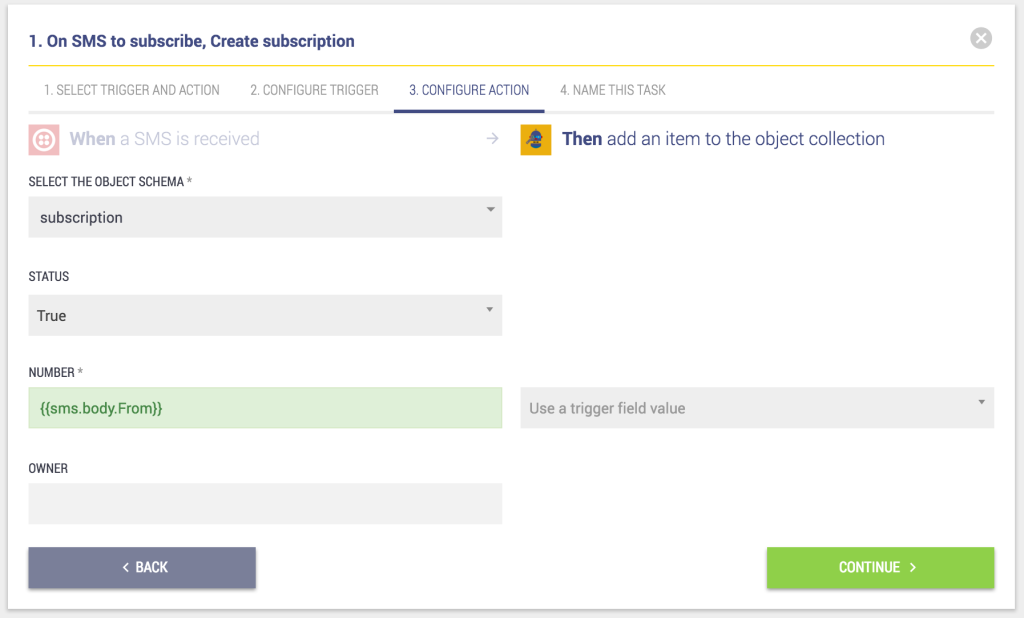
Verifying The Subscription Task
Now try to send the text message to your Twilio number, if everything has been configured correctly, by going to the “Database” section on the left sidebar and then selecting “subscription” you should see one entry.
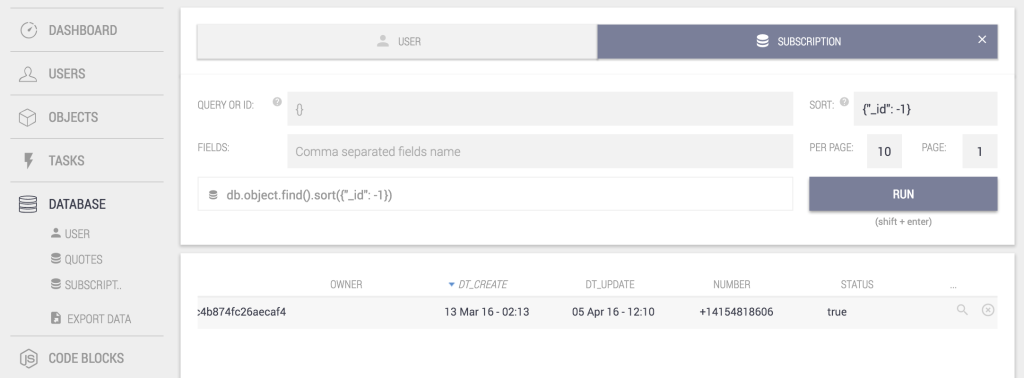
Last thing we want to address is to actually create subscriptions only when the SMS body contains the word “subscribe”. To do this let’s go back to our Tasks, click the Cog icon and then “Edit”. Go to Step 2 and expand the filtering section below the callback URL input field.
Here we will add a filter as the one below where we tell Stamplay to run this Task only if the message body contains the word “subscribe”.
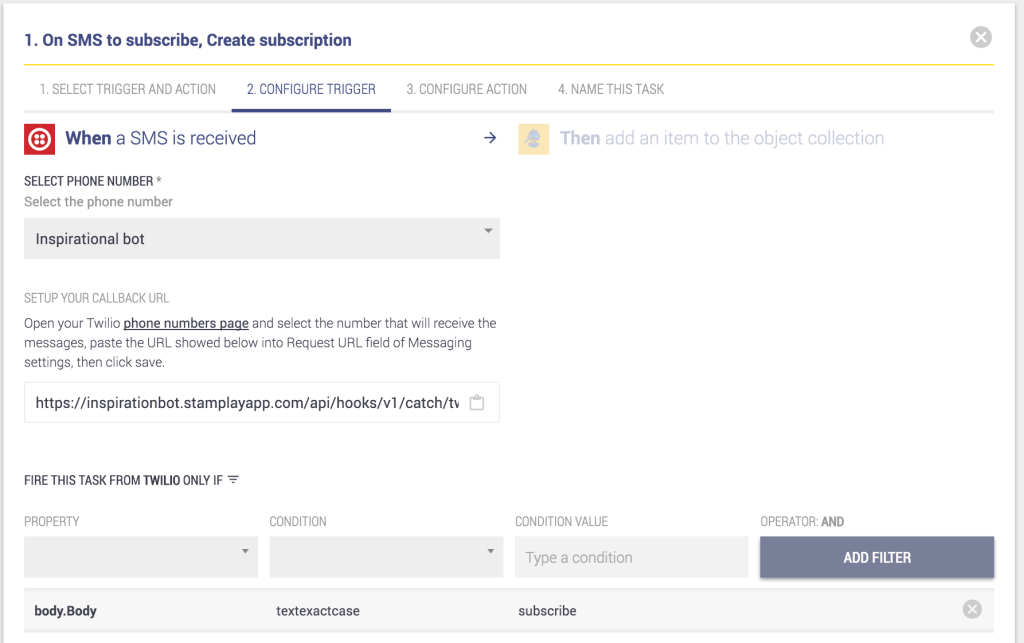
How To Process Subscriptions
To process the subscriptions we’ll have to use a two Code Blocks. One will be in charge of fetching all the active subscriptions, iterate through them and for every phone number pass it to the second Code Block which will fetch a quote and pass it along with a number to Twilio, using a Task.
The first one will be called processSubscriptions and will look like this:
The Code Block above uses the Stamplay NodeJS SDK to request all the subscriptions with status set to “true” and then iterate on the list. For each subscription it calls the FetchToSend Code Block passing as a parameter the phone number of the subscriber.
The second one will be called FetchToSend and will look like this:
The FetchToSend function takes a phone number as an input, collects a Quote object from the database and return as a response an object containing the recipient’s number, the author and the quote. We will use this data later on a Task that will send Twilio’s SMS and will be fired everytime the FetchToSend function runs.
The output of the Code Block will have the following structure:
{"to": "+11112223333","quote" : "Lorem Ipsum","author" : "Someone famous"}
Scheduling Triggers For A Desired Code Block
Now it’s time to set up a scheduled Task that will be executed once a day. To do this we will create a new Task using the Scheduler component.
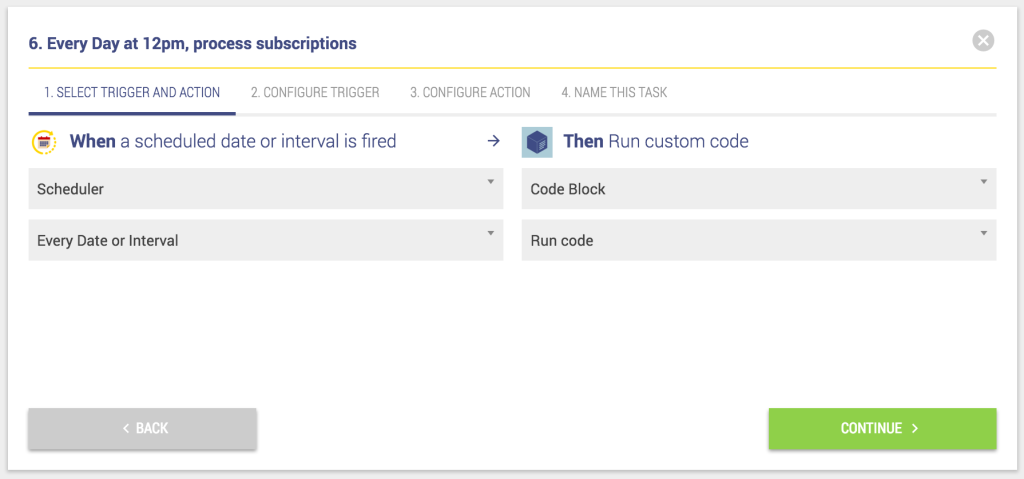
The time interval can be described with a crontab string. Crontab strings can be easily composed using Crontab guru who has an extensive list of examples, after defining your cadence pick the timezone where for this task and click “Continue”.
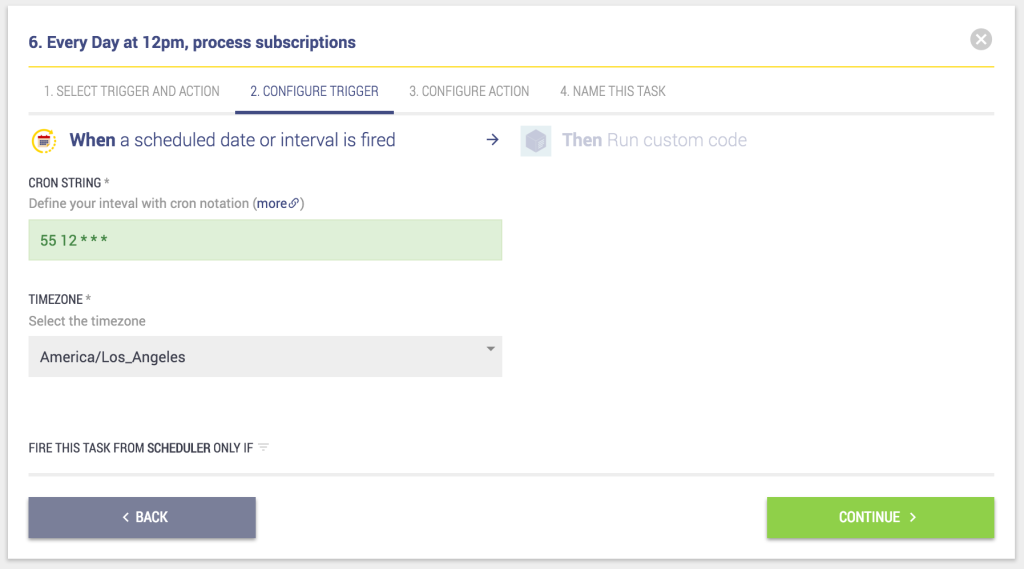
Select what Code Block we want to execute and provide a basic JSON input. After this let’s name our Task and save it.
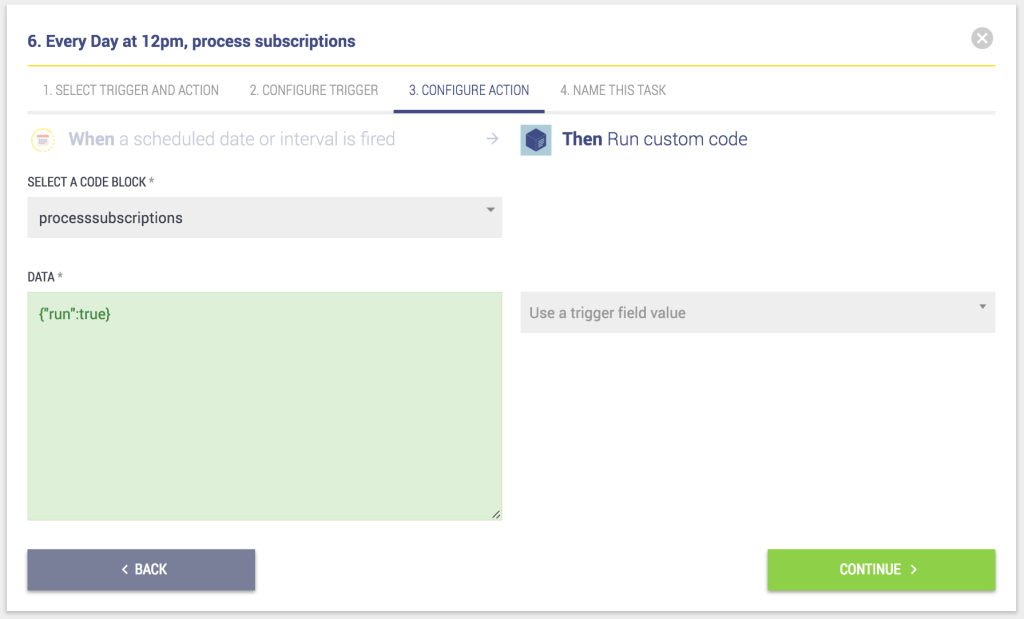
Sending The SMS after FetchToSend Is Executed
To close the loop of our SMS service we need to setup the last task that will take the output of the FetchToSend function and pass it to the Twilio “Send SMS” action.
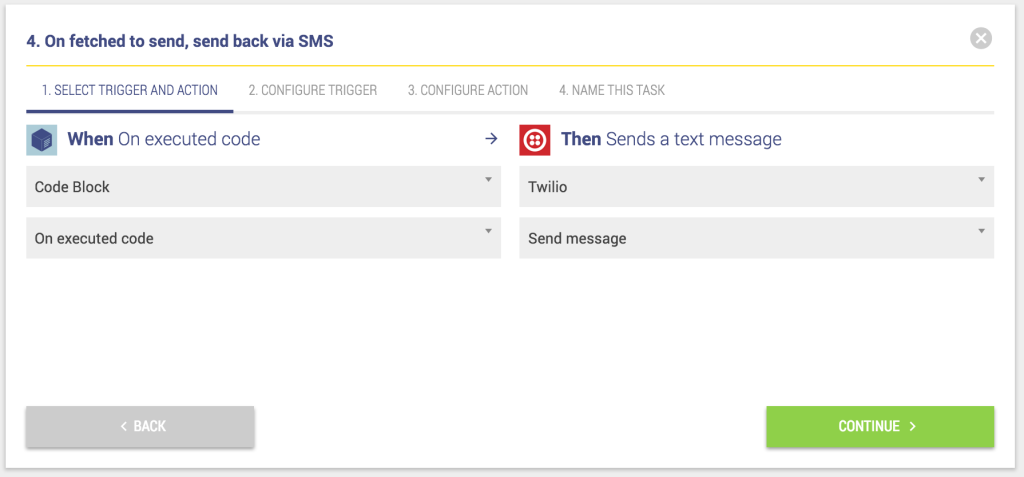
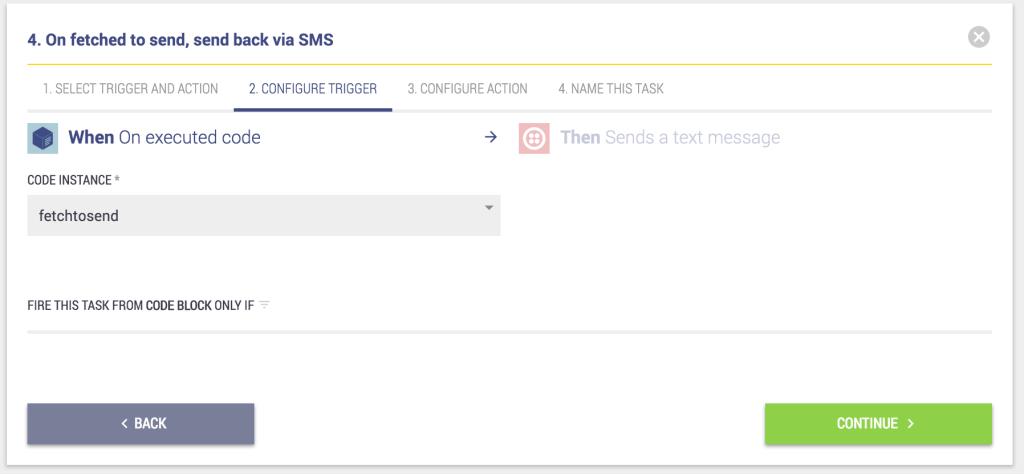
Now we will use the output of the Code Block to fill in the Twilio SMS action fields.
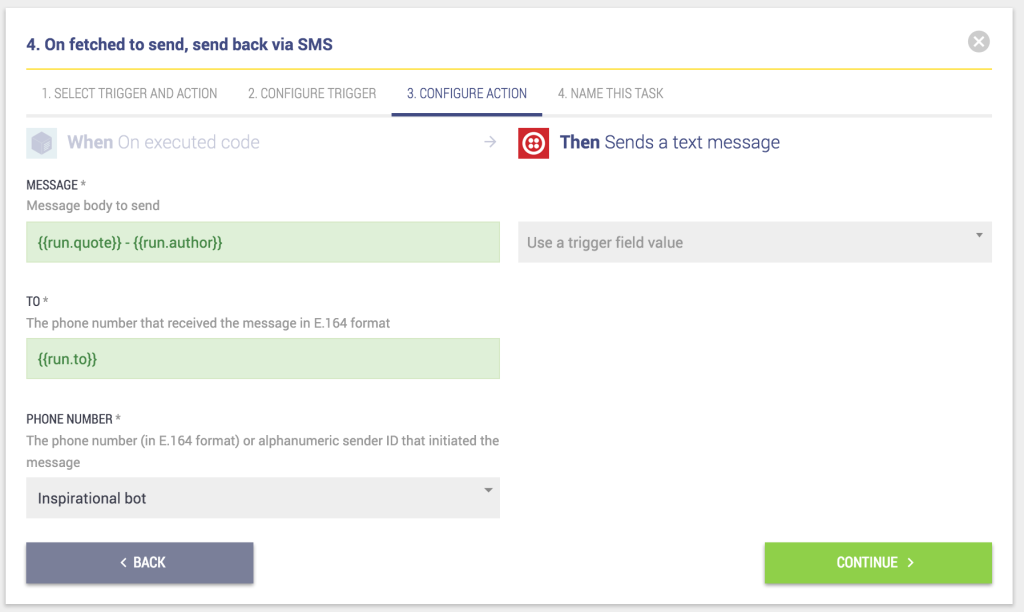
Blam! You’re done. You now have a daily SMS news service ready to rock. You can learn more about how to build unsubscribe functions to the app right here.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.