How to Build a Subscriber WordPress Plugin With the Twilio SendGrid API
Time to read: 8 minutes
How to build a subscriber Wordpress plugin with Twilio Sendgrid API
Every business, blogger, and entrepreneur needs a way of collecting the names and emails of prospective customers for email marketing. A newsletter subscriber form is a perfect way to do this.
Newsletter subscriber forms are online forms embedded on a website, designed to collect information from visitors who wish to receive regular updates, news, promotions, or other content via email. Typically, this form requests basic details such as the subscriber’s name and email address.
Every business needs a newsletter subscriber form for several compelling reasons. Firstly, it enables direct communication with potential and existing customers. Unlike social media platforms, where algorithms control visibility, email newsletters land directly in subscribers' inboxes, ensuring that the intended audience receives the message. This direct line of communication is invaluable for building and maintaining customer relationships.
Secondly, an email list comprises individuals who have expressed interest in the business, making them more likely to engage with its content and convert into paying customers. Regular newsletters can drive traffic to the website, promote products or services, and increase sales.
And thirdly, collecting data through a subscriber form enables businesses to segment their audience and personalize communication. Tailored content increases engagement rates and enhances customer satisfaction, as subscribers receive information relevant to their interests and needs.
Now, let’s build a WordPress subscriber form plugin with Twilio’s Sendgrid API.
How will the Sengrid API-powered WordPress plugin work?
The plugin will do the following:
- Be available with any WordPress theme that has Widgets enabled
- Allow the user to enter their name and email address to subscribe to the newsletter
- Use the Twilio Sendgrid API to handle the form submission of the plugin
Prerequisites
To follow along you’ll need the following:
- A basic understanding of and experience with developing in PHP
- PHP 8.3 with the MySQLi extension installed and enabled
- Composer installed globally
- A free or paid Twilio account with a verified phone number. If you are new to Twilio, click here to create a free account
- A SendGrid account
- The free GeneratePress theme
- MYSQL 8.3 and a client for interacting with MySQL, such as MYSQL’s command-line client, or your preferred database tool
- Your favorite code editor or IDE, such as Visual Studio Code or PhpStorm
Provision the WordPress database
The first thing to do is to provision the database. Using your MySQL client, run the following command to create a new database named wordpress_plugin.
To confirm that the database has been created, run the SQL command below.
You should see the name of your newly created database.
Configure WordPress
Next, you’ll need to download and unzip WordPress (version 6.6 at the time of writing) where you create your PHP projects. Then, change the directory name from wordpress to twiliopress.
As this folder already contains the files and folders required by WordPress, it will also serve as the project’s root directory.
Open the project directory in your favourite code editor or IDE. Find the folder named wp-config-sample.php and rename it to wp-config.php.
Then open wp-config.php and update the database settings (DB_*
) to suit your MySQL database's configuration. I've listed the four key settings in the code block below.
Next, you need to add the authentication unique keys and salts, so find the section labeled: Authentication unique keys and salts.
The authentication unique keys and salts are the code that encrypts your login details. Click here to generate them. Then copy and paste them in place of the existing values. To finish, save wp-config.php.
Run the WordPress install script
Switch to the project's root directory from your terminal and run the following command to start the application.
Then, in your browser of choice, open http://localhost:8080/wp-admin/install.php. From here, you’ll begin the steps to completing your WordPress installation.
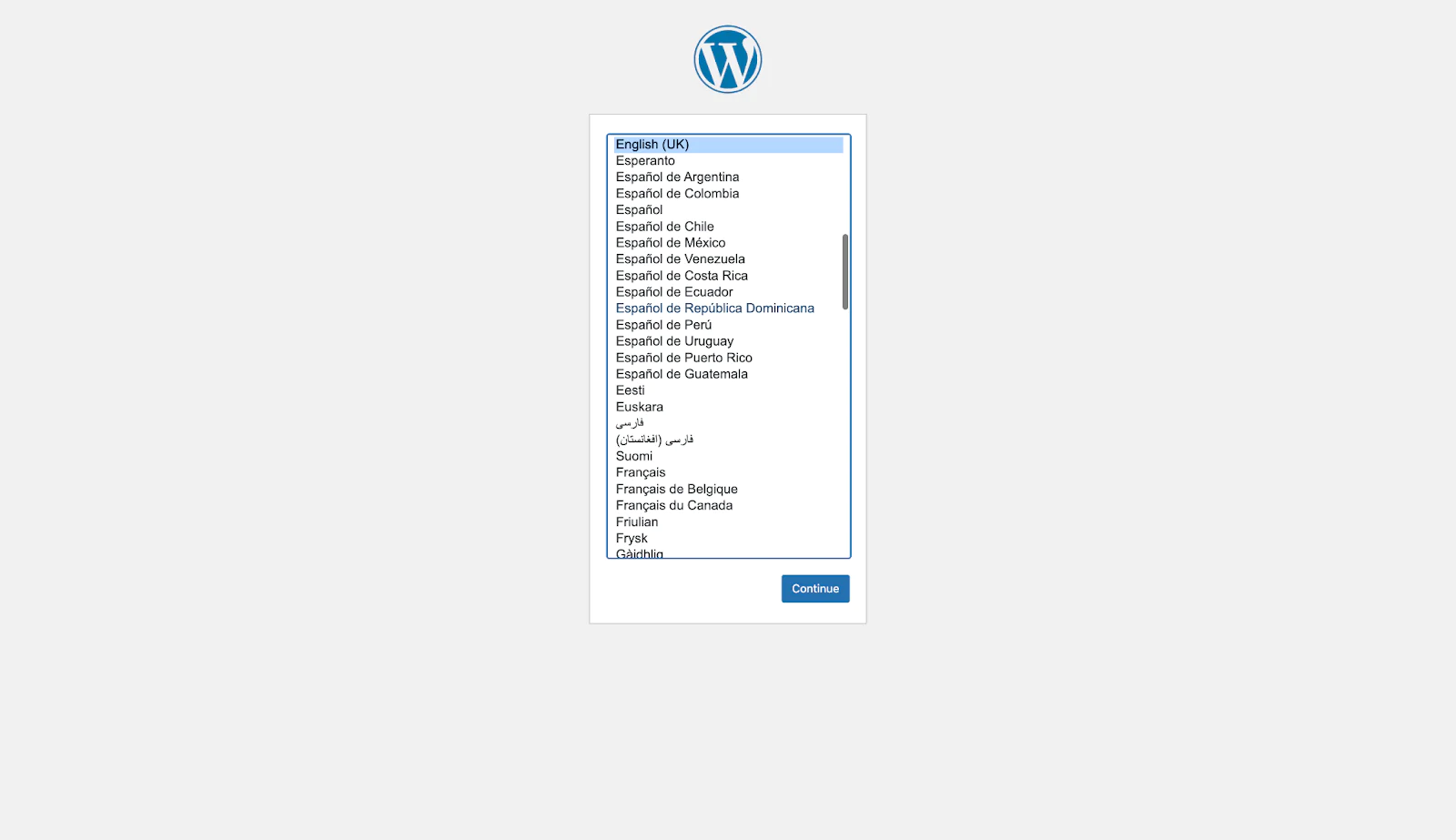
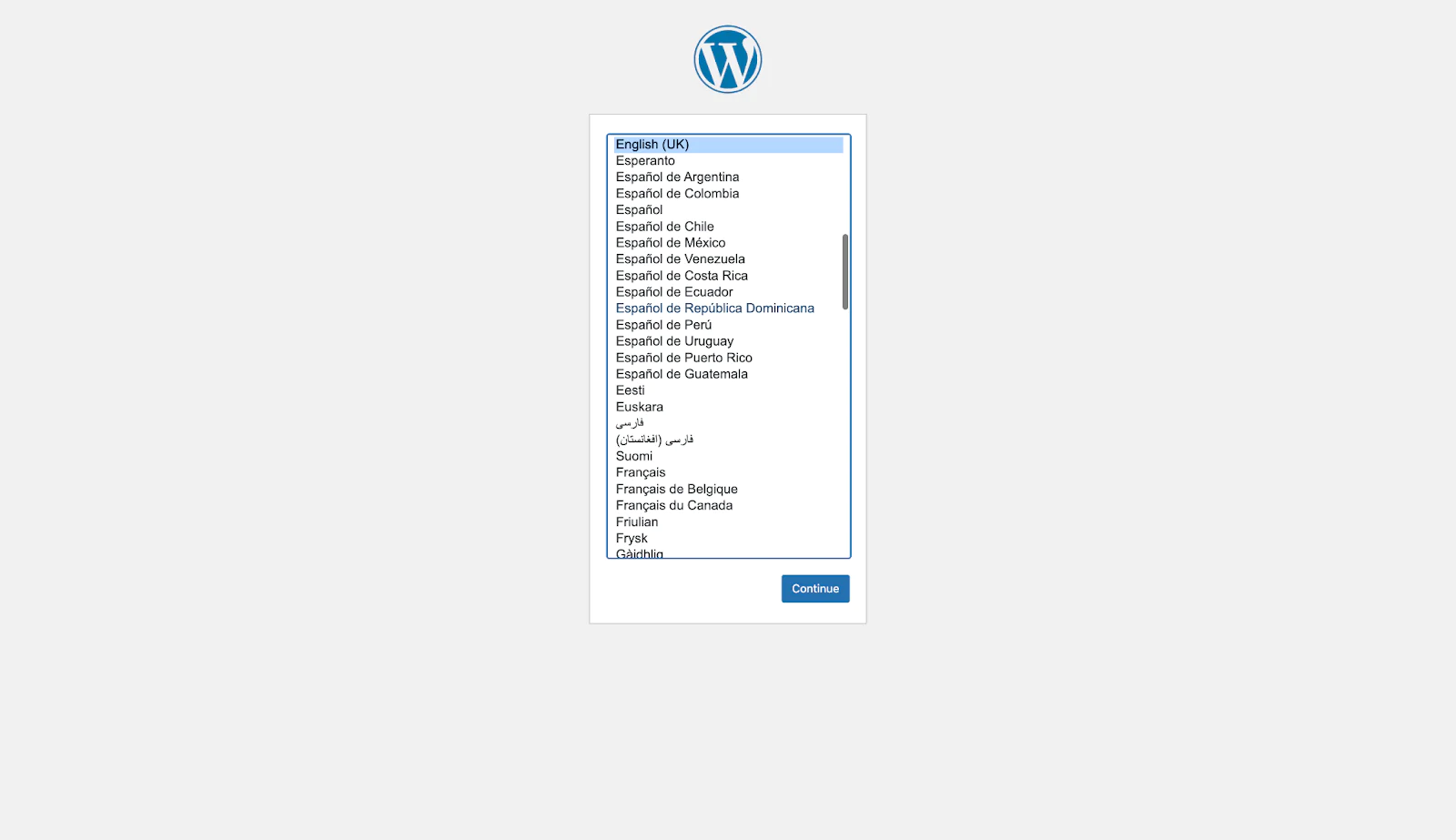
Step 1: Pick your language
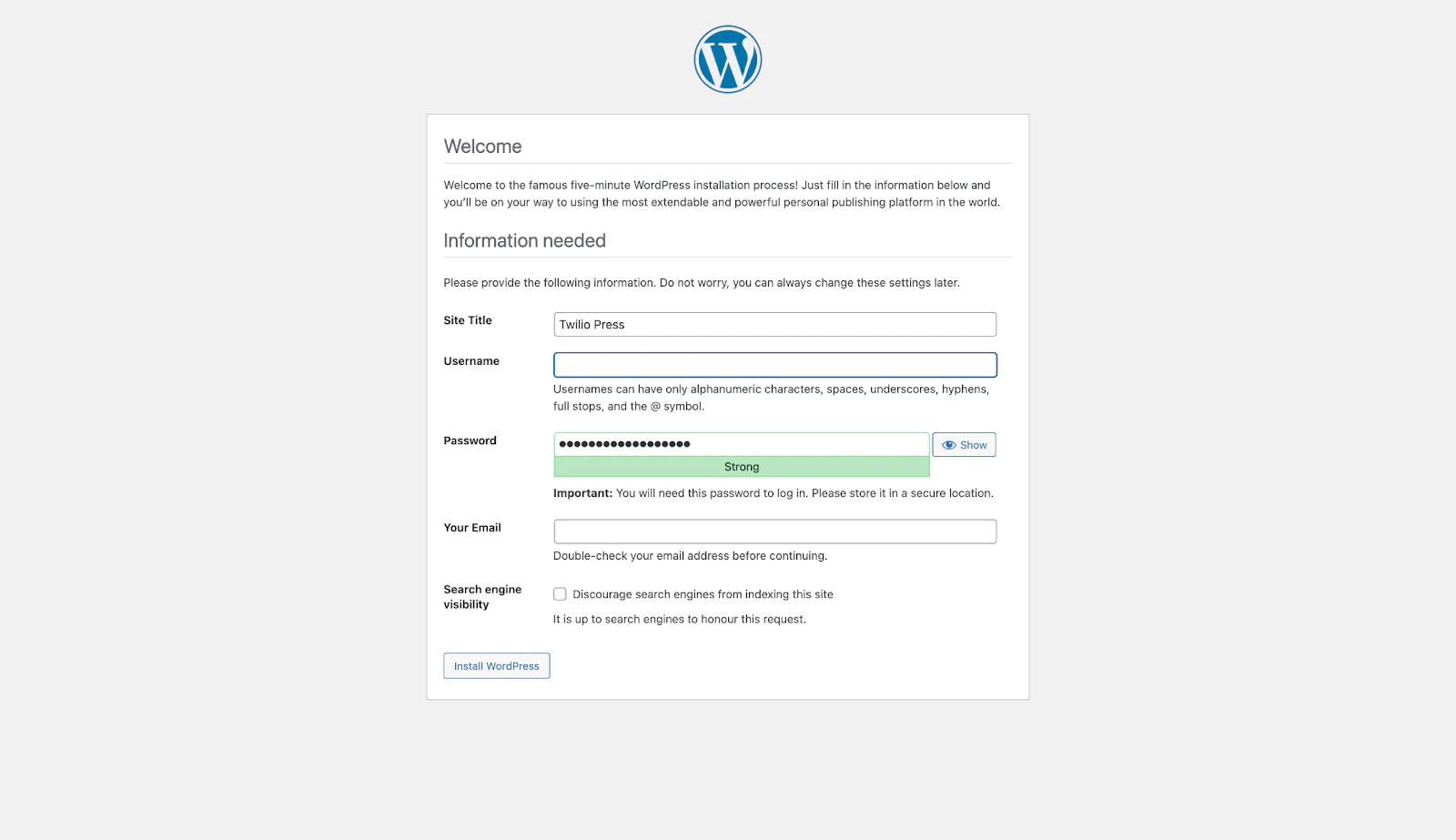
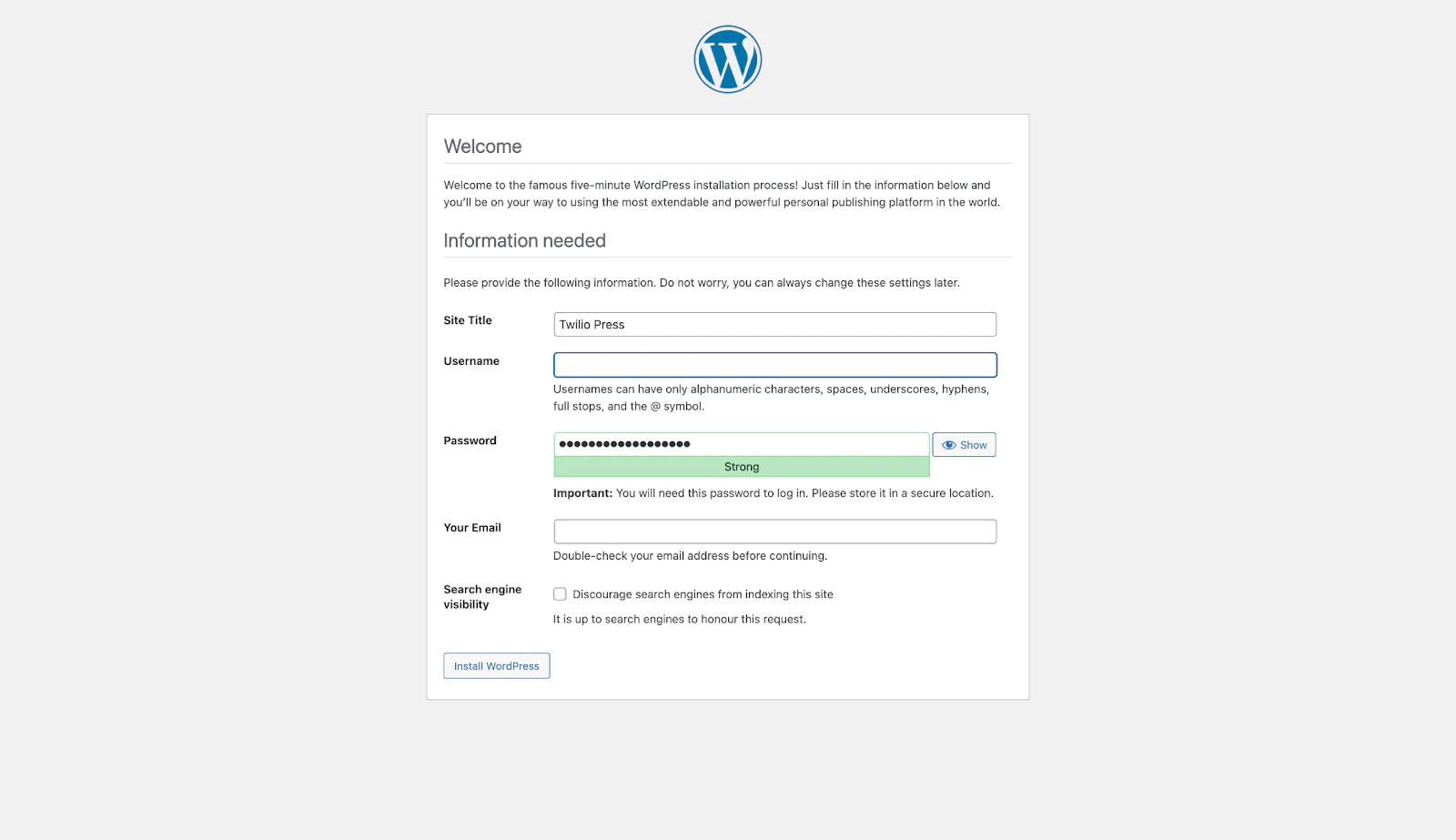
Step 2: Enter the name of your site. For this tutorial, I’ve named my website "Twilio Press", but you may choose any name you like. Then, enter your username, password and email address. To finish, click on Install WordPress.
Now, log into your new WordPress installation using the credentials you just used.
Install the GeneratePress theme
As of this writing, the default theme is the official WordPress Twenty Twenty-Four theme. However, this theme has not enabled widgets, so it’s not suitable for our plugin.
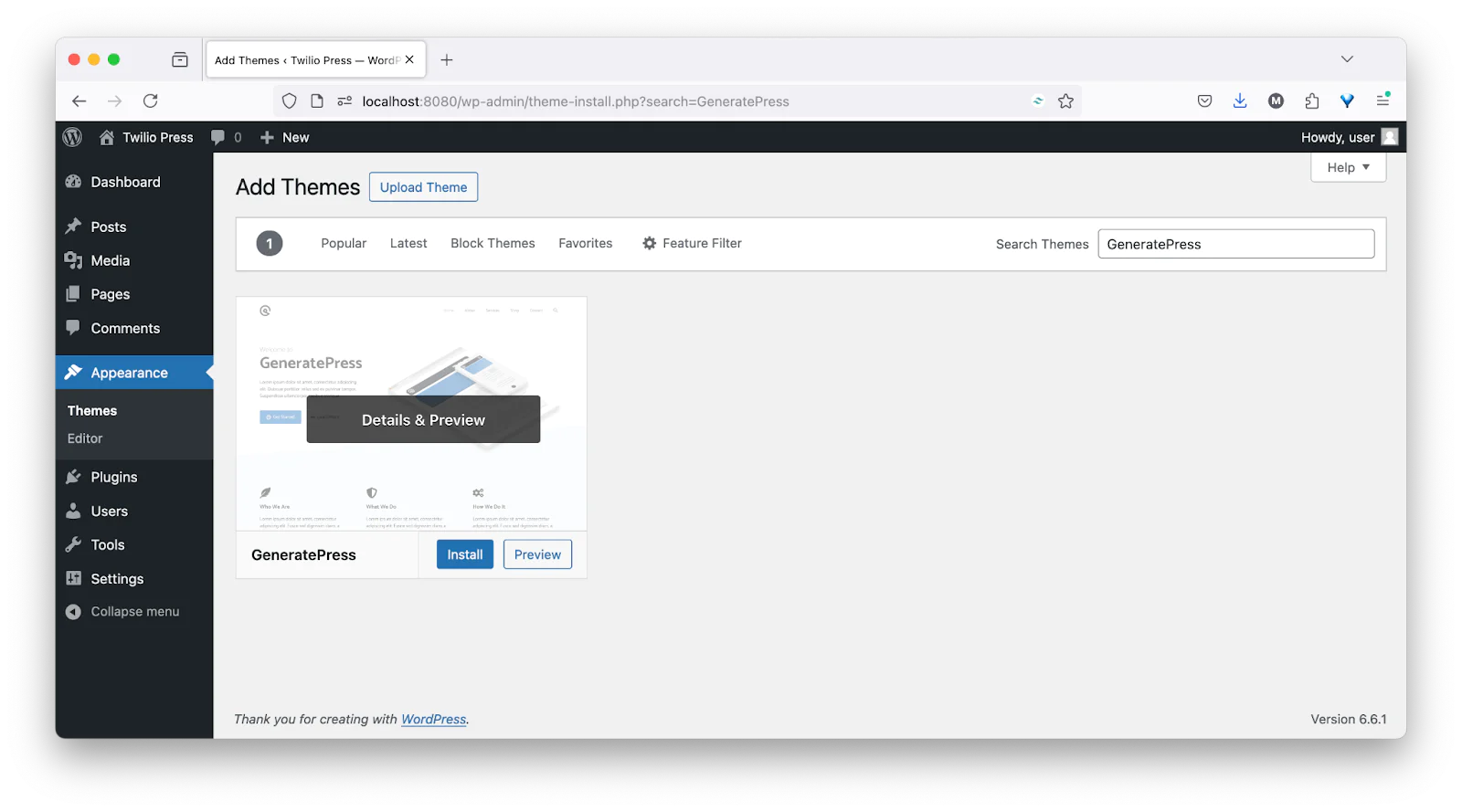
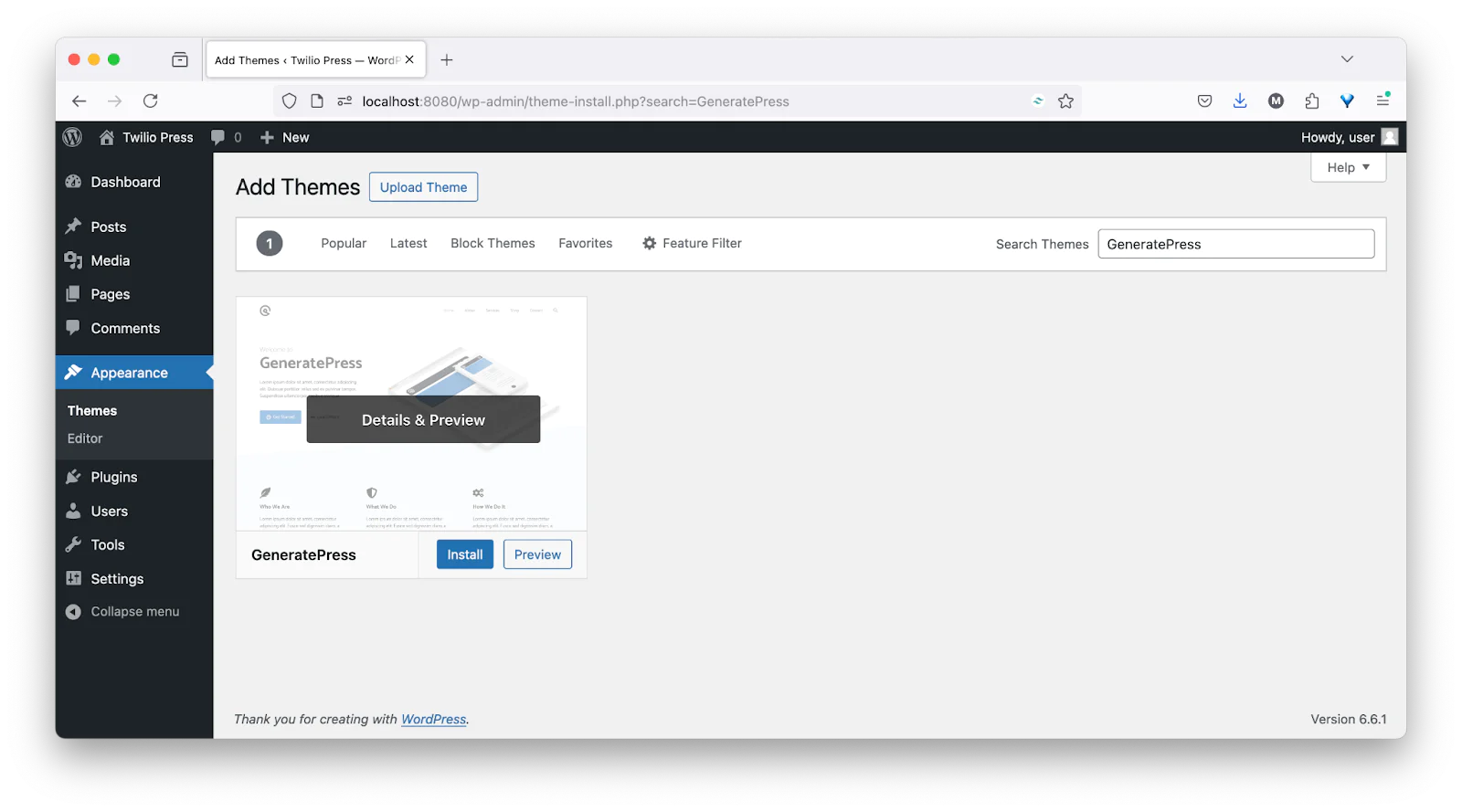
You need to install the free GeneratePress theme. To do this, navigate to Appearance > Themes and click on Add New Theme. Search for "GeneratePress". Then install and activate the theme. Once activated, you’ll notice the Appearance section now has a Widget option.
Create the project's directory structure
Now, in wp-content/plugins create a directory named twilio-newsletter-plugin and change into it,using the following commands.
The next step is to create the following files and folders inside the newly created project root directory.
Note that if you’re using Microsoft Windows, use the following commands instead, as it doesn't support the touch command nor the logical AND double ampersand.
Install the required packages
Now, run the following command to install the required packages.
The first package installed is the SendGrid helper library for PHP. The second is PHP dotenv for keeping sensitive credentials and other sensitive information out of code.
Build the widget class
A WordPress widget is a small block that performs a specific function. It can be added to various sections of a WordPress website, such as sidebars, footers, and other widget-ready areas. Widgets make it easy for users to customize the layout and functionality of their site without having to write any code.
Time to write the widget code. Open the twilio-newsletter-plugin-class.php file inside the wp-content/plugins/twilio-newsletter-plugin/includes folder and paste the following code into it.
To create a custom widget, you need to extend the WP_Widget class so that you can inherit its methods and properties. The constructor sets up the widget with two, specific options:
classname
: This defines the class name for the widgetdescription
: This describes the function of the widget
The next step is to output the contents of the widget with the widget($args, $instance)
method. The $args
parameter is used to configure the display of the widget:
$args["before_widget"]
: This specifies the HTML that wraps the widget instance$args["before_title"]
: This is the HTML that will be used to display the widget’s title
Then, the if statement checks if the title is set in the $instance
array and, if so, outputs it with echo $args["after_title”]
;
The form is created with fields for the user's name and email address, as well as a submit
button. The form's action
attribute points to a PHP script (newsletter-mailer.php) responsible for handling the form submission using SendGrid's API.
The form($instance)
function call is used to show the form in the WordPress admin area. It retrieves the current title and subject from the $instance
array if available. Otherwise, default values are used. Then, the input fields for the title and subject are created in the widget's settings.
The last function update($new_instance, $old_instance)
processes and saves the widget options. strip_tags()
is used to sanitize the title and subject inputs by removing any HTML tags. The sanitized values are stored in the $instance
array and then returned.
Register the widget
To register the widget, navigate the twilio-newsletter-plugin.php and paste the code below.
(!defined("ABSPATH")
ensures that the plugin file is being accessed through WordPress. ABSPATH is a constant defined by WordPress, and if it’s not defined, it means the file is being accessed directly. The exit()
statement prevents the script from running outside of the WordPress environment.
The plugin_dir_path(__FILE__)
function call returns the directory path of the current plugin file, ensuring the correct file path is used regardless of where the plugin is installed. The included file twilio-newsletter-plugin-class.php, defines the functionality of the plugin.
This part of the code handles the registration of the widget:
register_subscriber()
: This is a function that registers the widget with WordPress. It calls theregister_widget()
function, passing the name of the widget class (Twilio_Newsletter_Widget
) as a parameteradd_action("widgets_init", "register_subscriber")
: This line hooks the register_subscriber function into thewidgets_init
action. This action is triggered by WordPress during its initialization process, making it the appropriate time to register widgets
Now, let’s check out the registered widget. Open your WordPress dashboard and navigate to Plugins > Installed Plugins. You should see a plugin named Twilio Newsletter Plugin as in the image below.
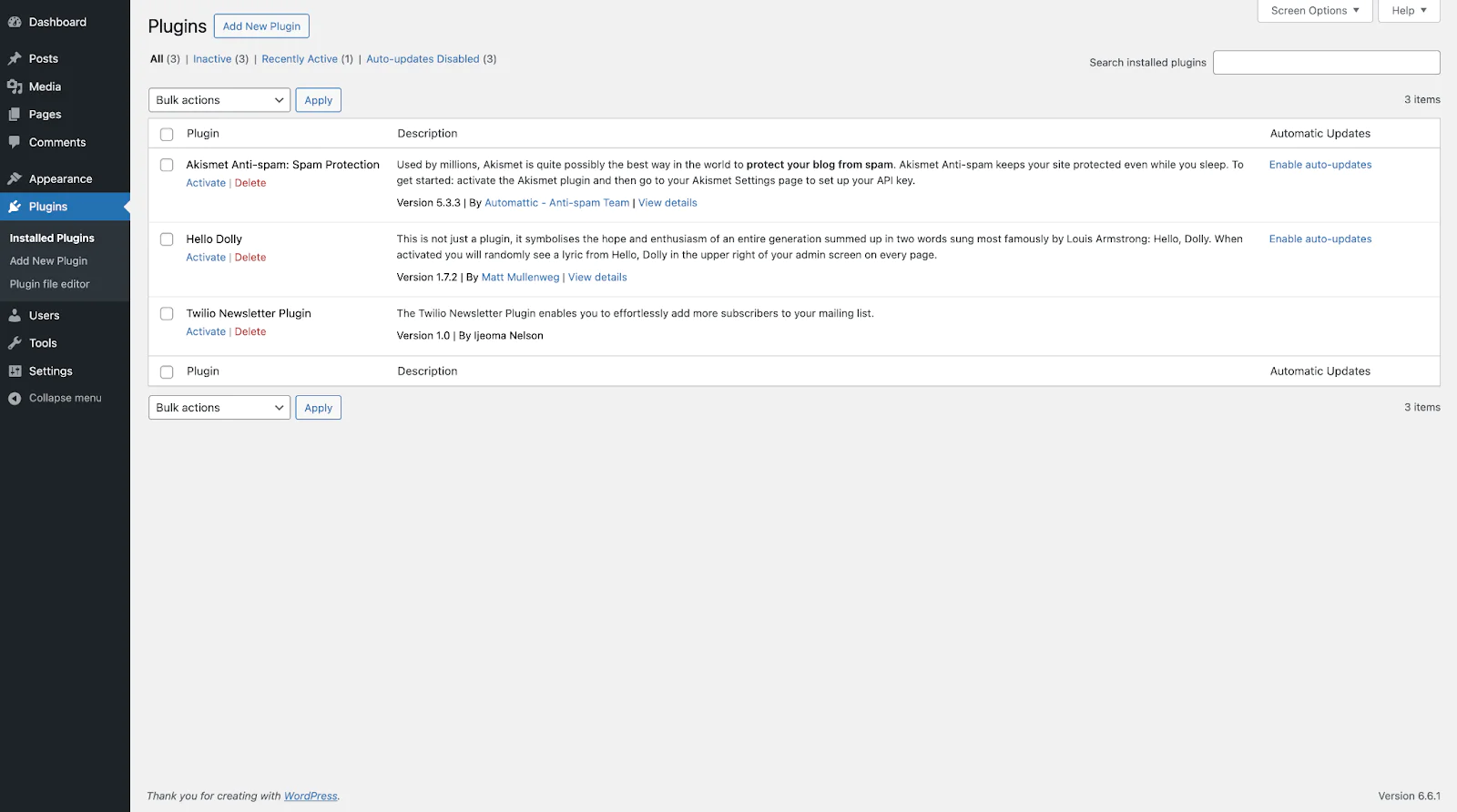
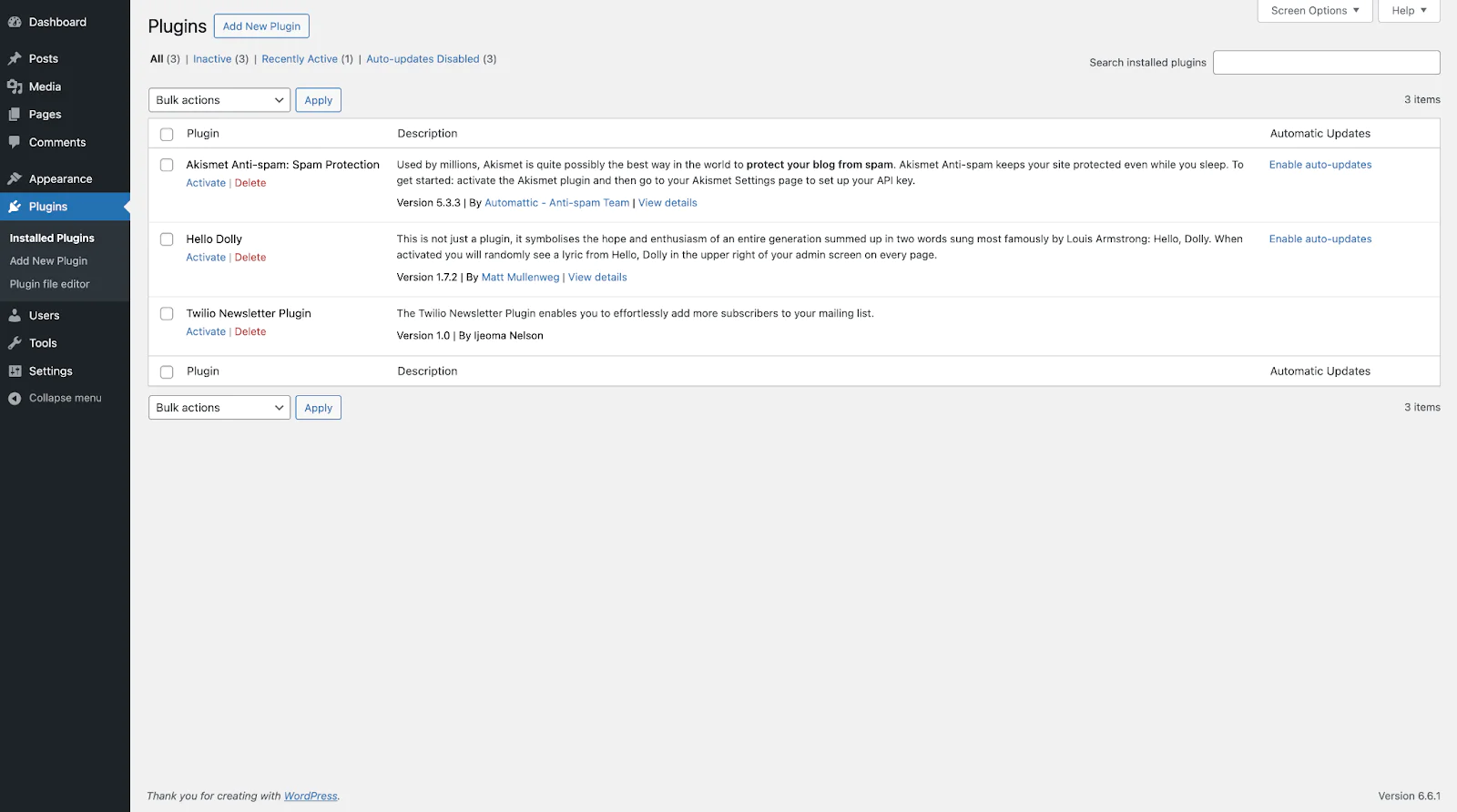
Because I know you can’t resist the temptation to click that Activate button underneath the plugin's name, click it! Once activated, you can display it on the front end. To do this, go to Appearance, click on Widgets and add the Twilio Newsletter Plugin as a widget
Now, let’s finish by configuring the SendGrid integration.
SendGrid API integration
First, open the wp-content/plugins/twilio-newsletter-plugin/.env file. Copy the configuration below into the file, then replace <ADD YOUR SENDGRID API KEY HERE>
with your SendGrid API key. To find or create your SendGrid API key, login to your account and from the dashboard click on settings and then API Keys.
To configure the SendGrid integration, paste the code below inside the file named newsletter-mailer.php.
The code begins by importing the Mail
class from SendGrid's PHP Helper Library and installing the dependencies. if (isset($_POST["submit”]))
is used to check if the form has been submitted and if so, the following happens:
strip_tags()
: removes HTML tags and extra spaces from the name inputfilter_var()
: trims spaces and sanitizes the email input to remove illegal charactersnew Mail()
: initializes a new SendGrid Mail objectsetFrom()
: sets the sender's email and name. Note that the email must be your verified SendGrid sender’s email. You can choose any name you like for the name fieldsetSubject()
: sets the email subjectaddTo()
: adds the recipient's email and name. This is the email address and name of the person you’re sending toaddContent()
: sets the email content type to HTML and constructs the email body with the subscriber's name and emailnew \SendGrid(getenv("API_KEY”))
: creates a new SendGrid client instance using the API key from the environment variables
Finally, a try/catch block is created to send the email using the SendGrid email client. If successful, the user is redirected to index.php which is a confirmation page. Otherwise, the error is caught and echoed.
Create the success page
When the subscriber submits the form, it would be a better user experience if they’re redirected to a welcome page. To do this, navigate to wp-content/plugins/twilio-newsletter-plugin/index.php and paste the following code inside the file.
This HTML document defines a simple webpage with the following features:
- Specifies English as the language and includes metadata for character encoding and viewport settings
- Provides a brief description of the page content
- Imports the Poppins font from Google Fonts
- Sets the title of the webpage
- Links to an external CSS stylesheet for additional styling
- Contains a main container with a heading and a subscription confirmation message
Next, we’ll pretty it all up with a little CSS styling.
Add some CSS
Navigate to the style.css file inside the plugin's css folder and paste the code below inside it.
This CSS style gives the page a clean and modern look:
- The
.main-container
class centers text, uses the Poppins font, and converts text to uppercase - The
h1
element has a dark blue colour, large font size, extra letter spacing, and top padding to position text in the mid-section of the page - The
.subscribed
class adds a dark gray color, moderate font size, and increased letter spacing for clarity and emphasis
Test that the plugin works
It's time to test the plugin. Navigate to the frontend of your WordPress site and submit a name and email in the input fields like so.
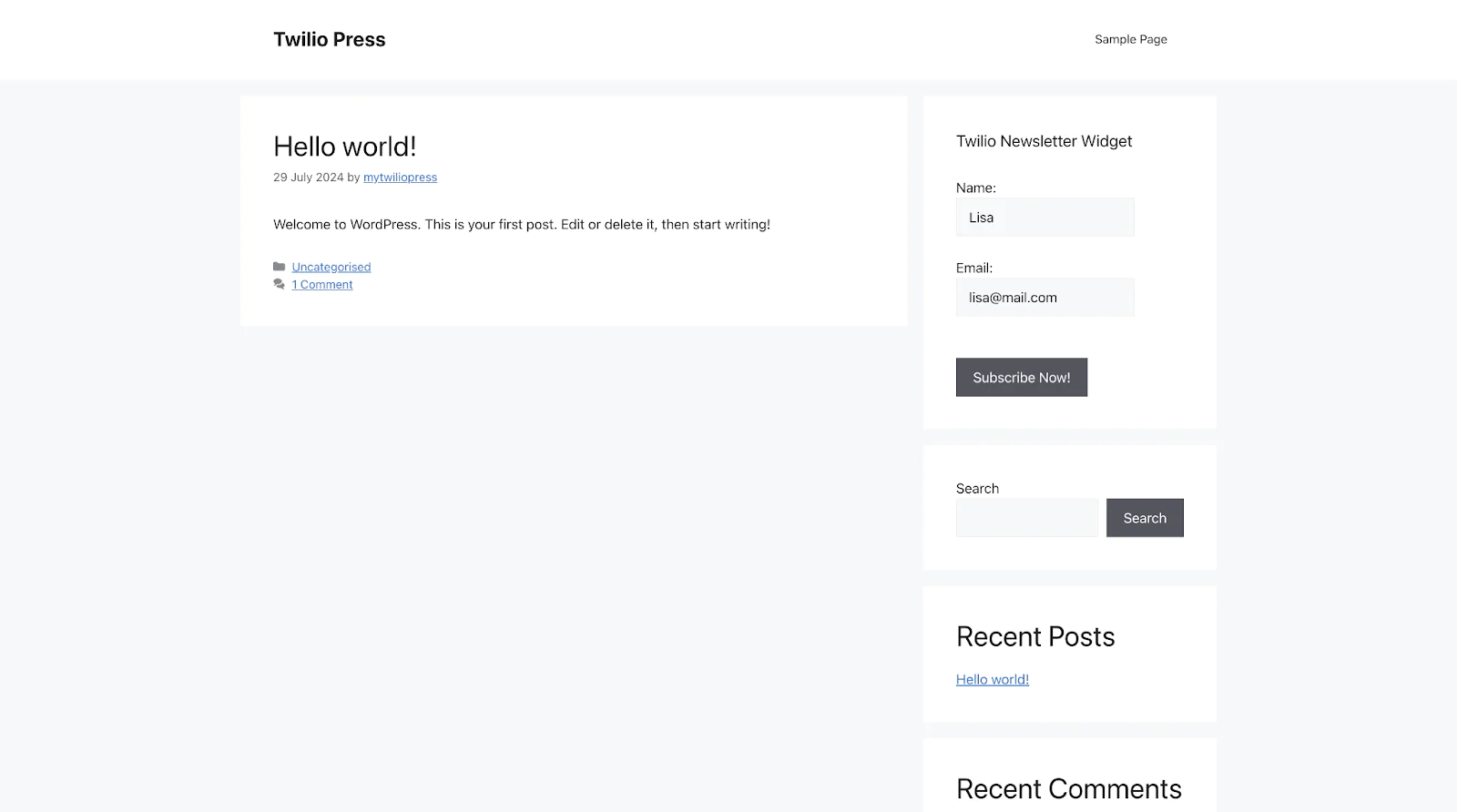
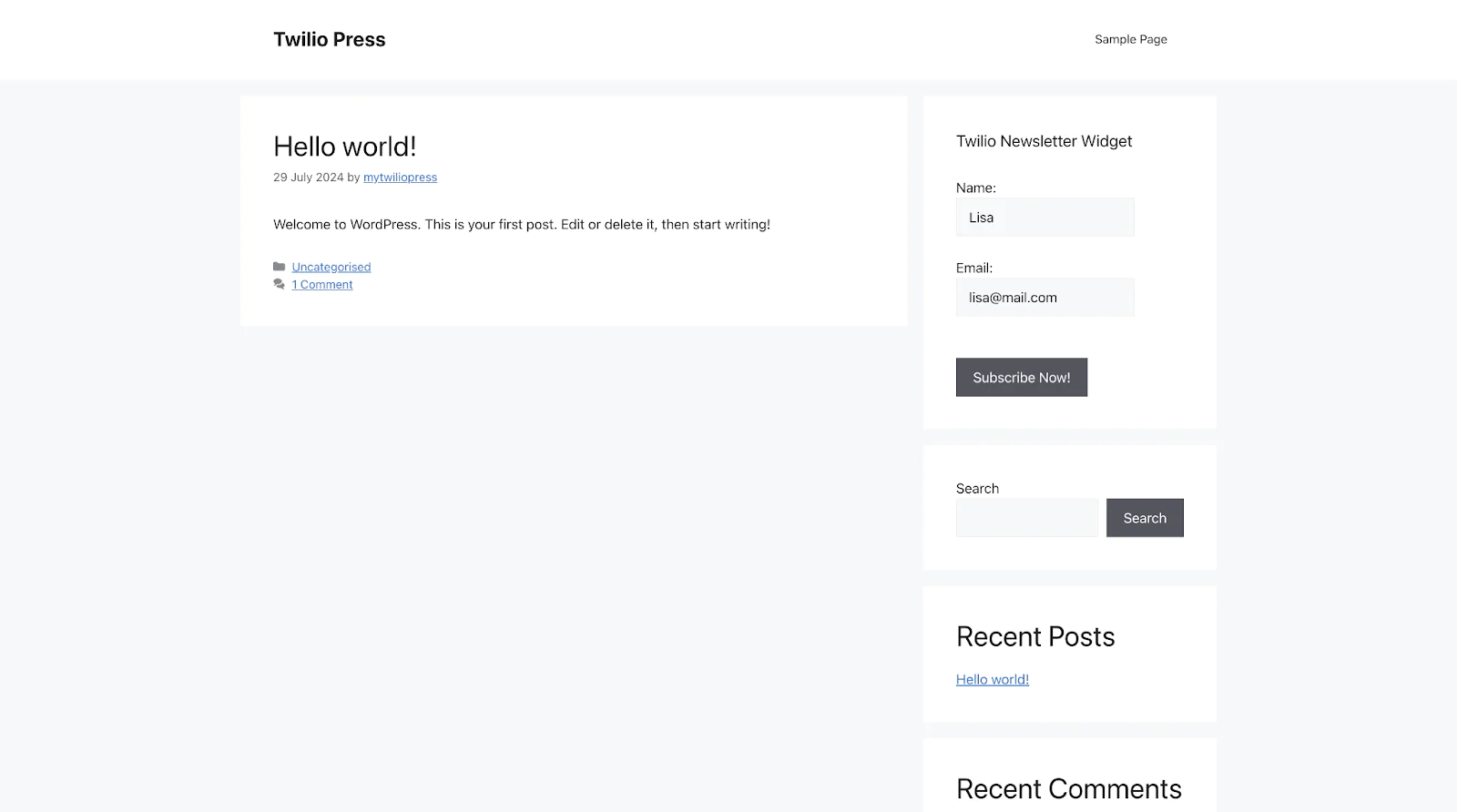
You should be redirected to a page that looks like the one below.
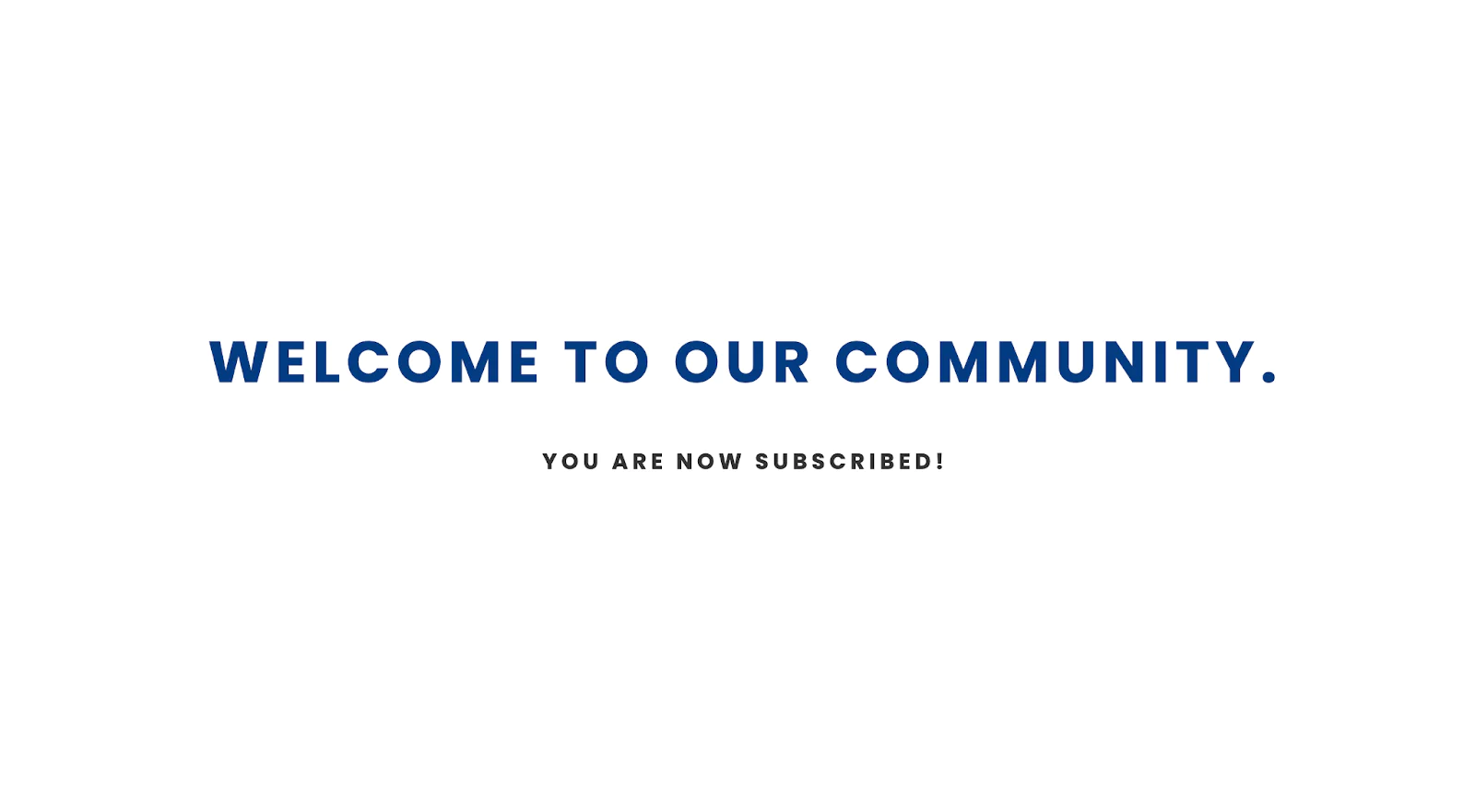
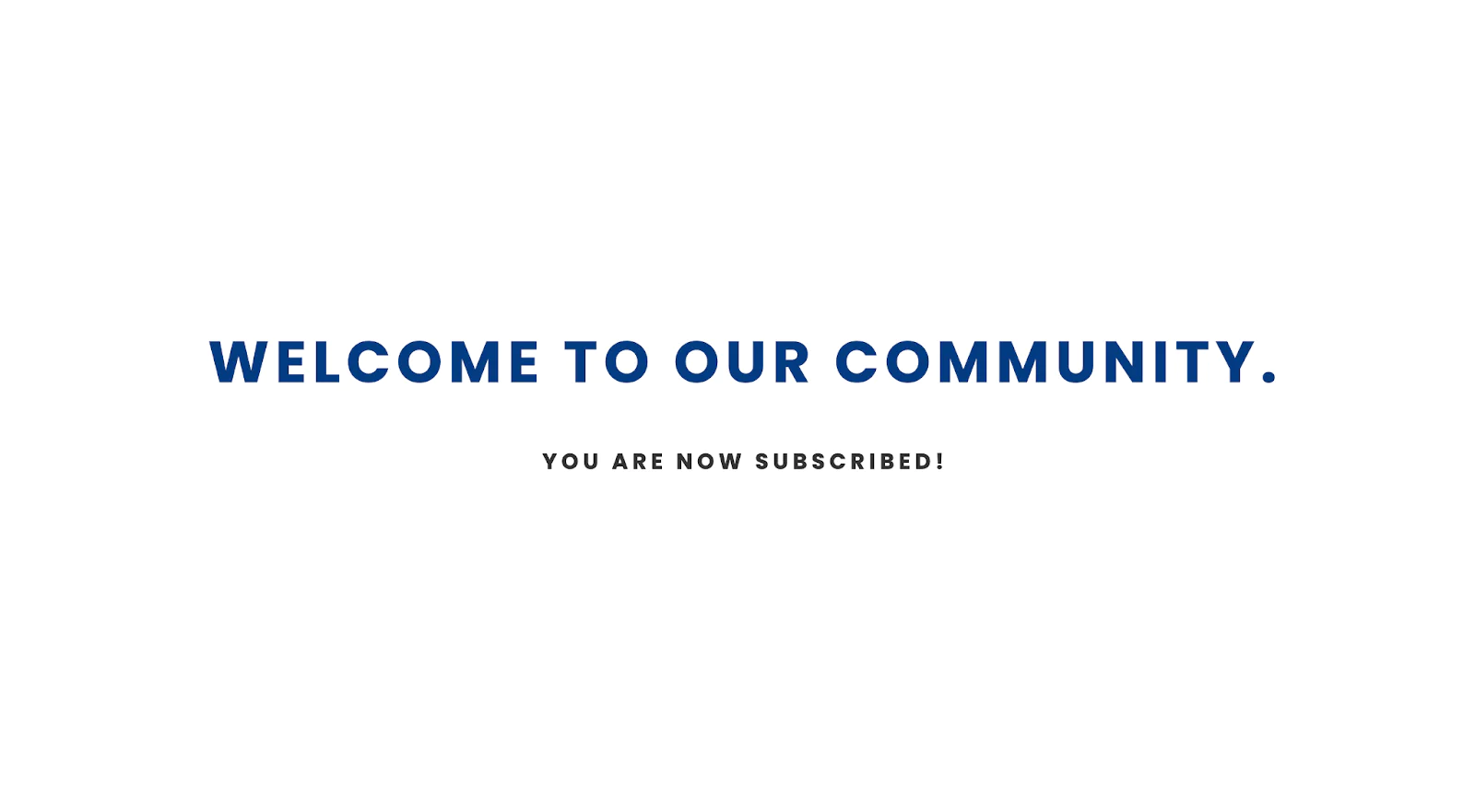
And if you check your email, you should have an email sent from your newsletter plugin.
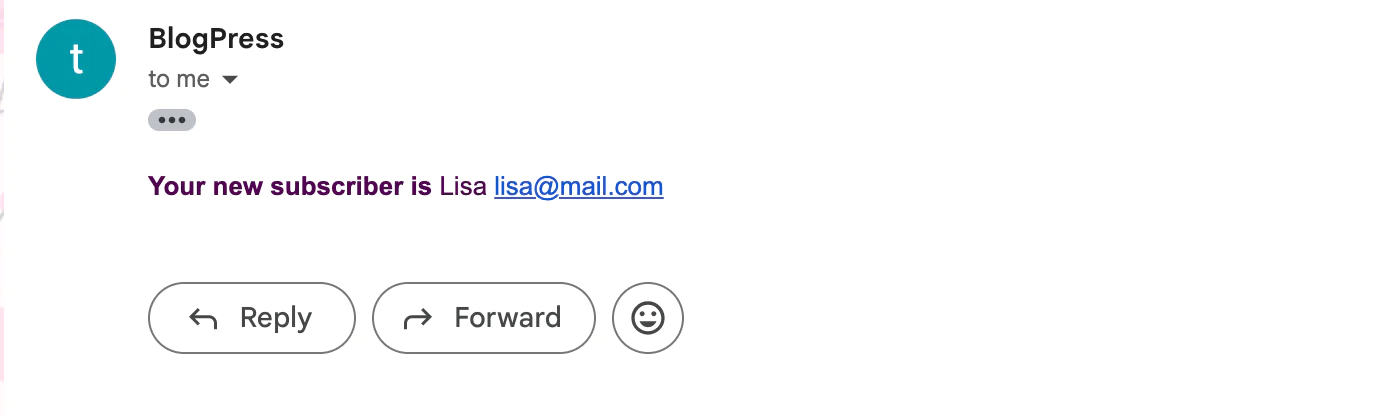
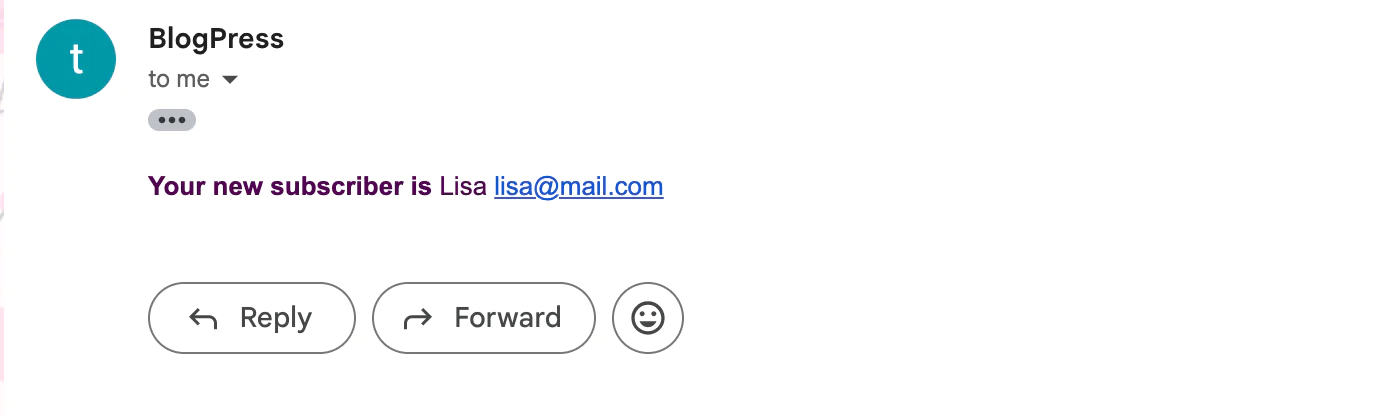
Congratulations on completing this tutorial! Whilst a very simple email marketing tool, you’ve added another potent technical skill to your toolkit. If you’re the tinkering kind, there are so many ways you can extend this widget. You could add a dashboard that monitors all new subscribers, or you could even add user authentication and turn it into a micro-sass company. Whatever you decide, I wish you every success in using your new skill.
Ijeoma Nelson is a London-based software developer and technical writer with a passion for teaching how to build awesome things.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.