How to Build an SMS Stock Notification App with Go and Twilio
Time to read:
This post is part of Twilio’s archive and may contain outdated information. We’re always building something new, so be sure to check out our latest posts for the most up-to-date insights.
How to Build an SMS Stock Notification App with Go and Twilio
Staying informed about the latest stock market movements is crucial for investors and traders alike. With the rise of mobile technology, receiving real-time stock price updates via SMS can be a game-changer.
This tutorial will guide you through creating a powerful SMS-based stock price update system using Go and Twilio. By the end of this tutorial, you'll have a working application that sends real-time stock price notifications directly to your mobile device, allowing you to make informed investment decisions on the go.
Prerequisites
Before we begin, ensure you have the following:
- Go version 1.22 or above
- ngrok and a free ngrok account; this is needed to tunnel the application to expose the locally running Go application to the internet, allowing Twilio's SMS webhook to send real-time stock price updates
- A Twilio account (either free or paid). If you are new to Twilio, click here to create a free account
- An Alpha Vantage account; I recommend requiring the Alpha Vantage service, as you use it throughout the tutorial
- Basic knowledge of Go
- A mobile phone that can send SMS
Set up the Project
To continue with this tutorial, you have to start by:
- Creating a new project directory and Go module
- Retrieving the Alpha Vantage API key and Twilio credentials and storing them in .env at the root of the project
- Installing the needed Go packages such as gorilla/mux, GoDotEnv, and twilio-go
- Creating an endpoint in the app to take incoming SMS, check the given stock prices, and respond by SMS to the given phone with the Twilio API
- Finally, you will open ngrok to open your local server to the world for testing, set up the webhook on Twilio, and live-notify prices of stocks through SMS
Create the Project directory
First, we'll create a new directory for our project, navigate into it, and initialize a new Go module.
Create the required environment variables
To keep your API keys and other sensitive information secure, you should use environment variables stored in a .env file. Now, create a .env file in the root of your project and add the following keys:
The ALPHA_VANTAGE_API_KEY
is required to access financial data via Alpha Vantage. The TWILIO_ACCOUNT_SID
, TWILIO_AUTH_TOKEN
, and TWILIO_PHONE_NUMBER
are credentials needed to interact with the Twilio API.
By replacing the placeholders (<your_alpha_vantage_api_key>
, <your_twilio_account_sid>
, <your_twilio_auth_token>
, and <your_twilio_phone_number>
) with actual values which you'll retrieve shortly, the application can authenticate and utilize the functionalities of both services.
Retrieve your Alpha Vantage API key
Alpha Vantage provides a comprehensive API for fetching stock data. You'll need to sign up for an API key here by entering your email address and pressing the GET FREE API KEY button.
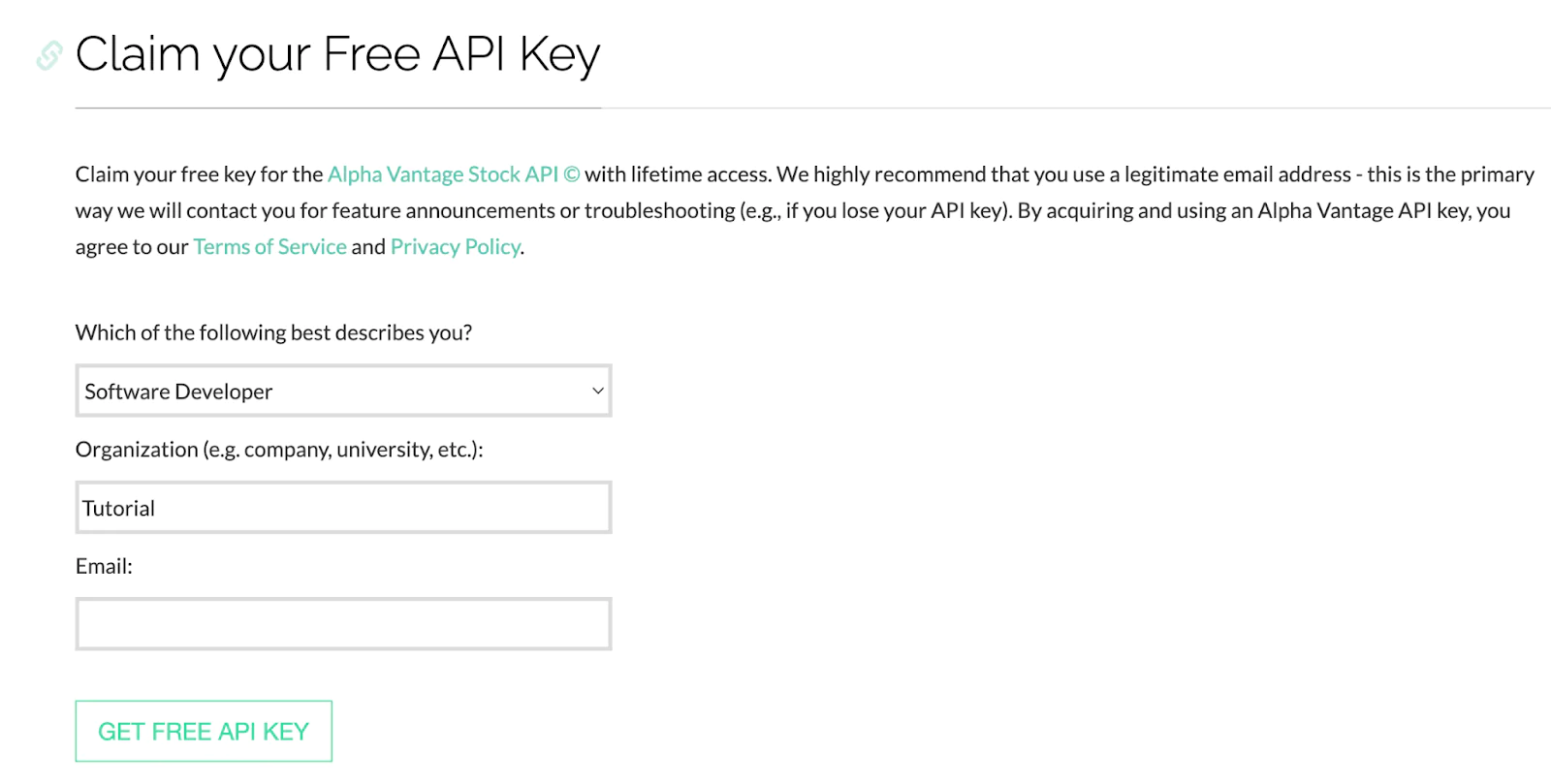
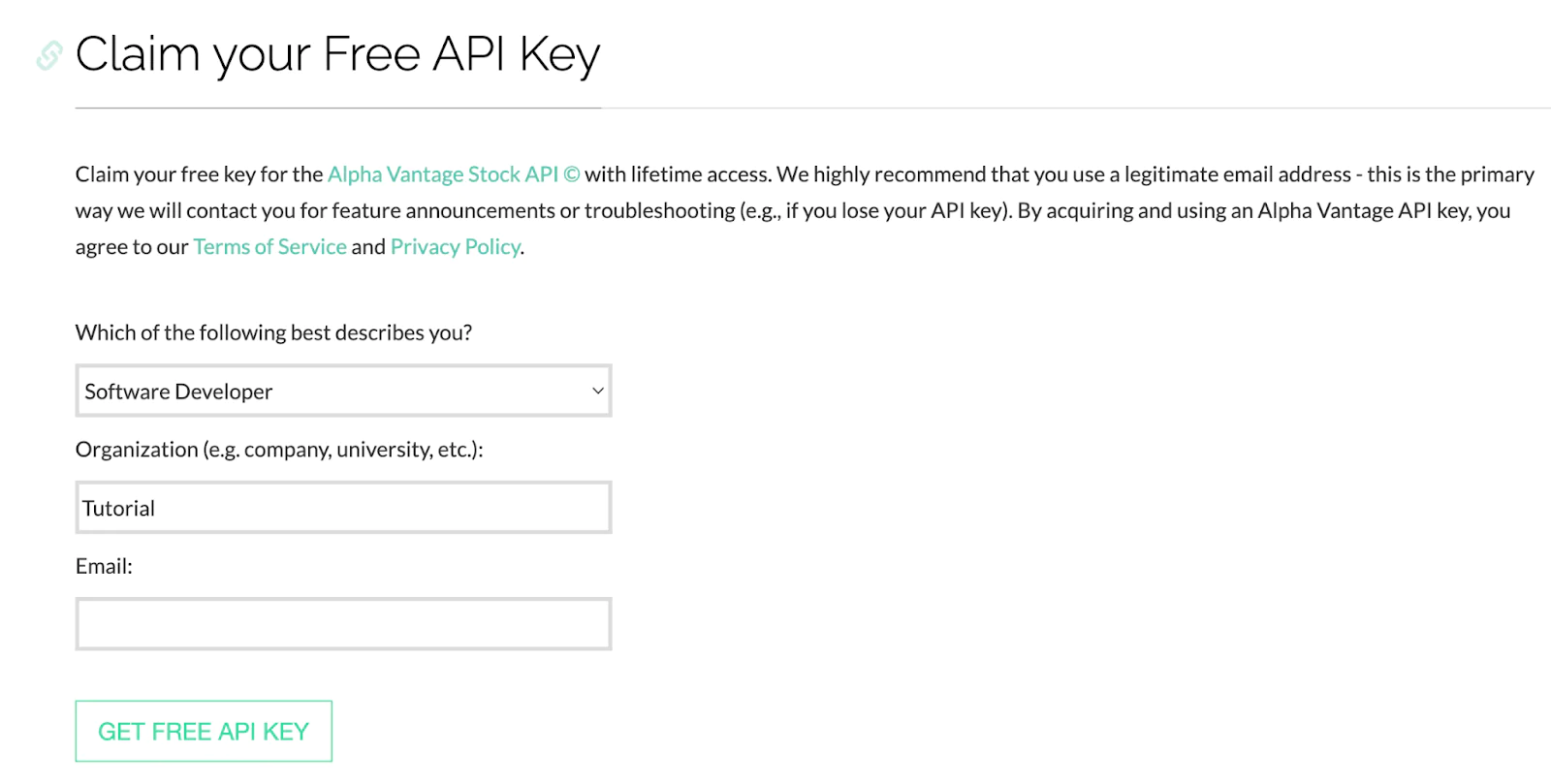
Then, copy the API key and place it in place of <your_alpha_vantage_api_key>
in .env.
Retrieve your Twilio Access Token
Next, log in to your Twilio Console to retrieve your Account SID, Auth Token, and Twilio phone number, as you can see in the screenshot below
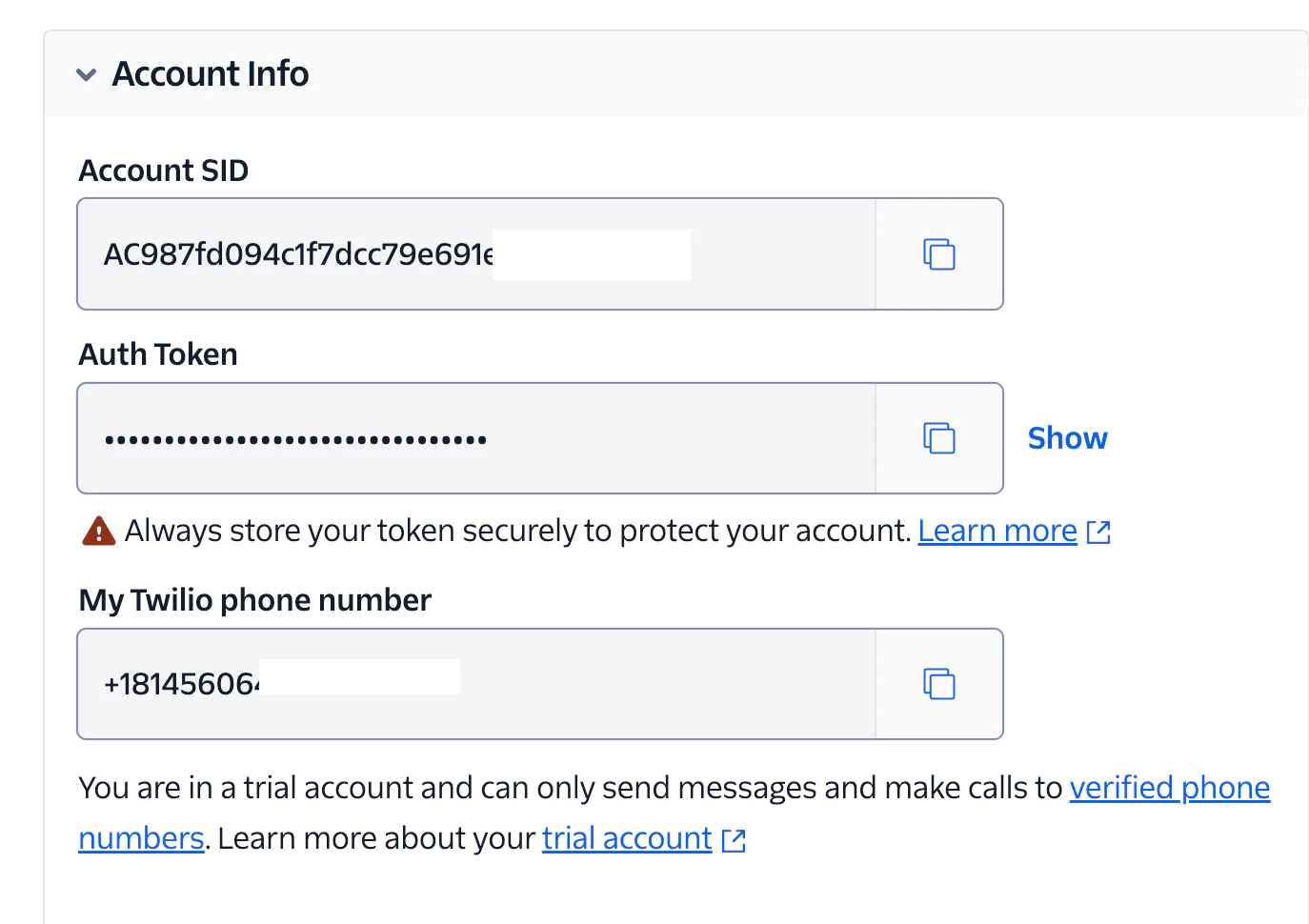
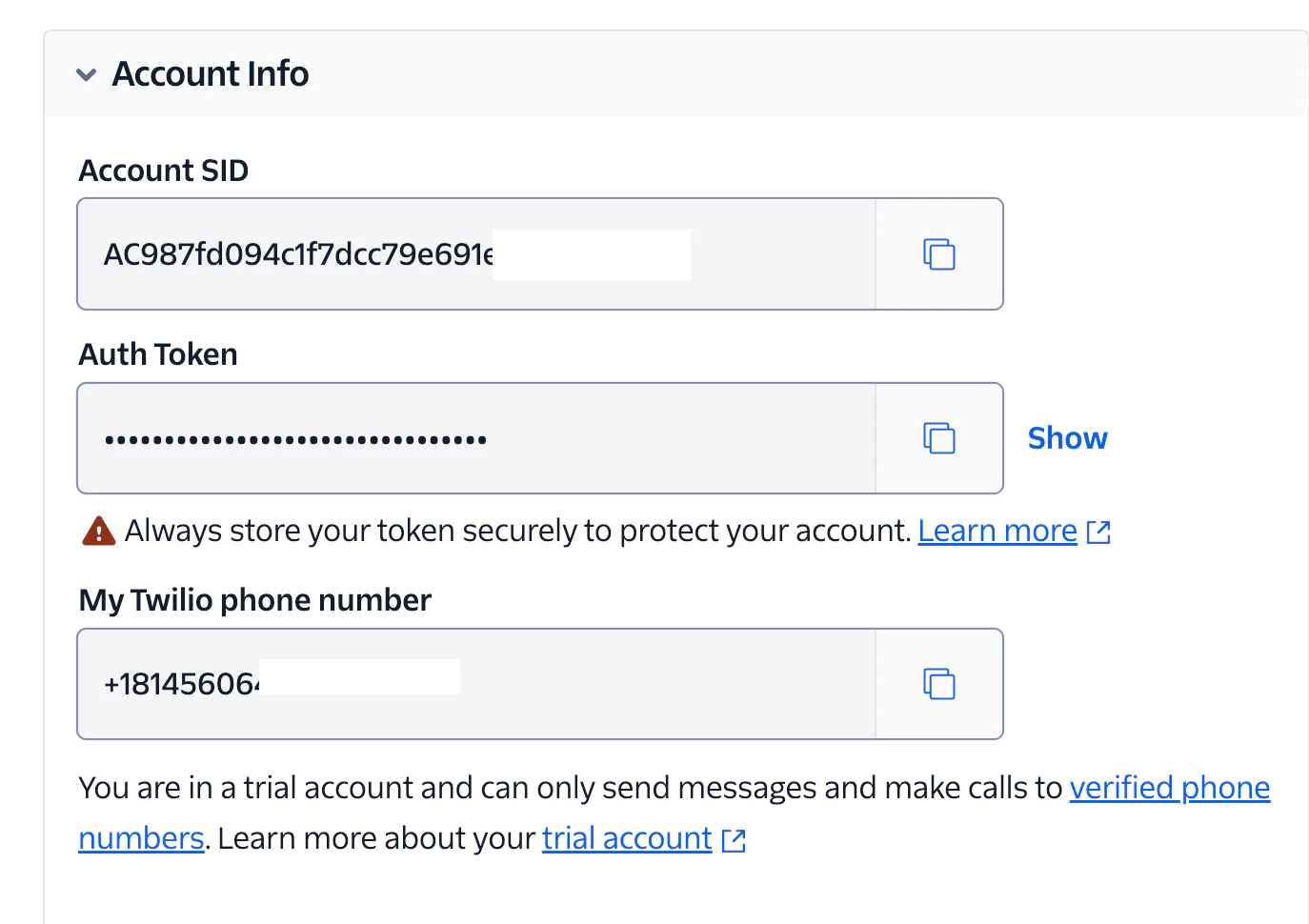
Then, use them to replace <your_twilio_account_sid>
, <your_twilio_auth_token>
, and <your_twilio_phone_number>
, respectively, in .env.
Install the necessary packages
We'll next need several packages to build our application. These are:
- Gorilla Mux: This package is a request router and dispatcher that matches incoming HTTP requests to their respective handlers. "Mux" is short for "HTTP request multiplexer," It supports matching based on URL host, path, schemes, headers, query values, and HTTP methods, and allows variables with optional regular expressions.
- GoDotEnv: This package simplifies managing environment variables. The package loads the required environment variables from .env into the Go application, aiding in configuration management.
- twilio-go: The package is a client library for interfacing with the Twilio API, enabling functionality such as sending messages and making calls.
To install the above packages, run the following command.
Create the application's logic
Next, let’s create an endpoint to handle incoming SMS messages. This endpoint will receive the stock symbol, fetch the stock price, and send a response back to the user.
Create a file named main.go and add the following code:
The Go code above uses gorilla/mux for routing, GoDotEnv to load environment variables, and the twilio-go client for sending SMS messages.
The getStockPrice() function makes an API call to Alpha Vantage to fetch the latest stock price for the given stock symbol. The "/sms" endpoint is a Twilio webhook that handles incoming SMS messages, extracting the stock symbol from the message body and using the getStockPrice() function to fetch the stock price. The stock price is then sent back to the user via Twilio's messaging API. The server listens on port 3000 and logs relevant information and errors for debugging purposes.
Start the application
Now, start the application by running the command below in your terminal:
Your application should now be running on port 3000.
Tunnel the application
To configure the Twilio SMS webhook, you need to make the application accessible online. Do this using ngrok by running the command below in a new terminal tab or session.
Next, copy the generated forwarding address as shown in the screenshot below.
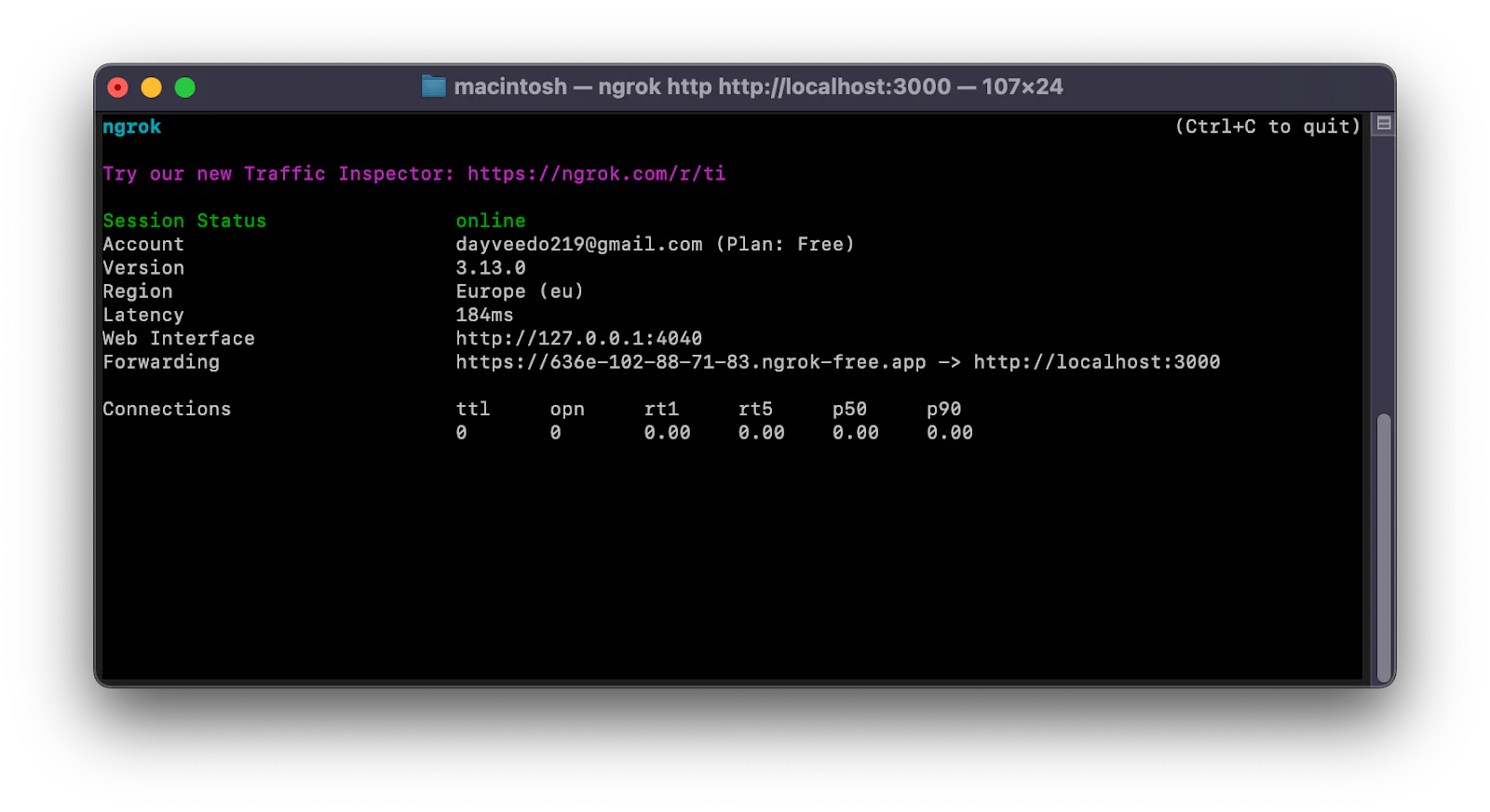
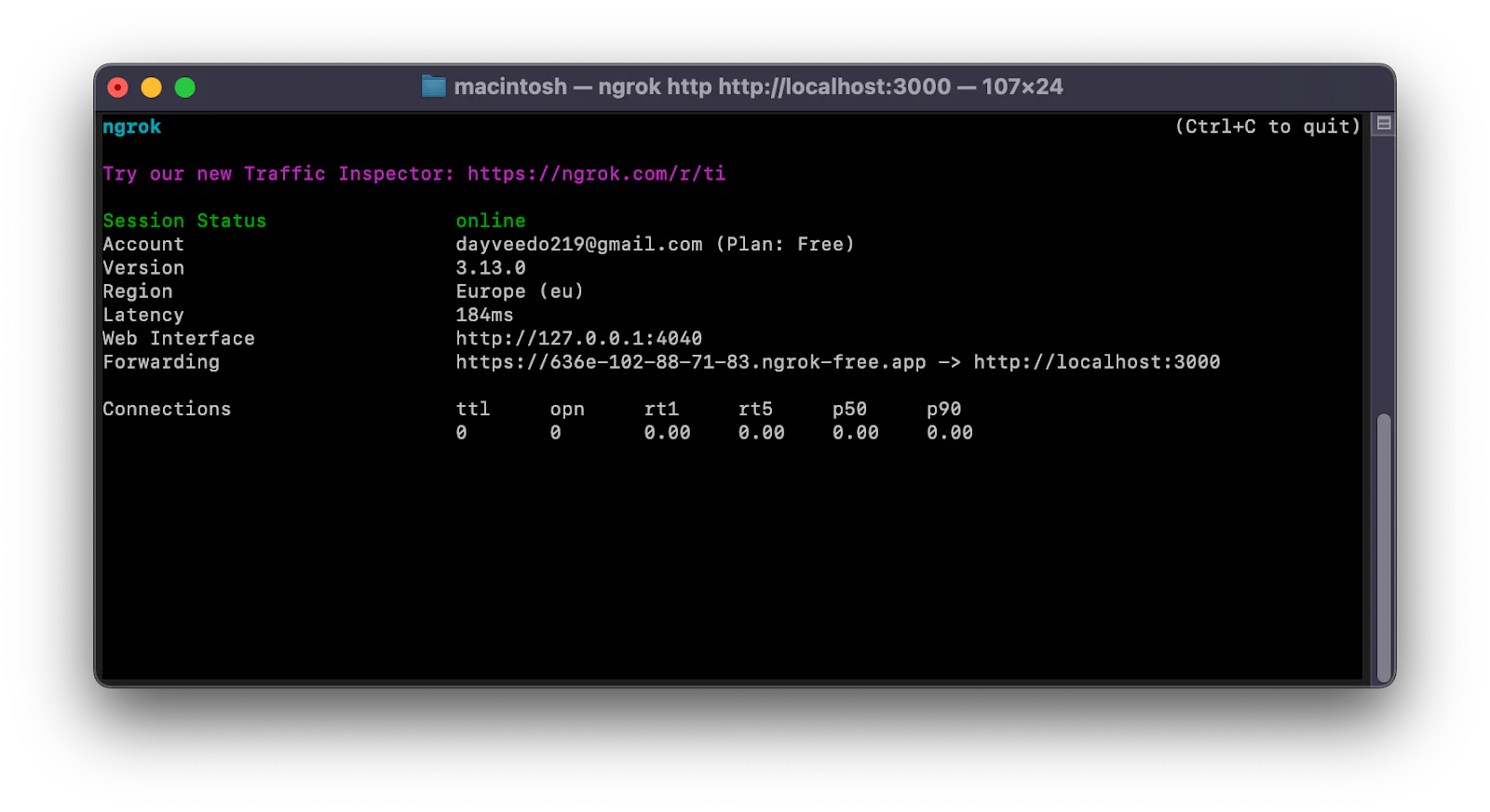
Configure the Twilio SMS webhook
To configure Twilio to send incoming SMS messages to your application, follow these steps:
- Log in to your Twilio Console
- Navigate to Phone Numbers > Manage > Active numbers and select the phone number you want to use
- In the Messaging section, set A message comes in to "Webhook", the URL field next to it to the ngrok Forwarding URL, and append "/sms" to the end of the URL, e.g., https://d2c6-102-88-71-831.ngrok-free.app/sms
- Click Save configuration to save the changes
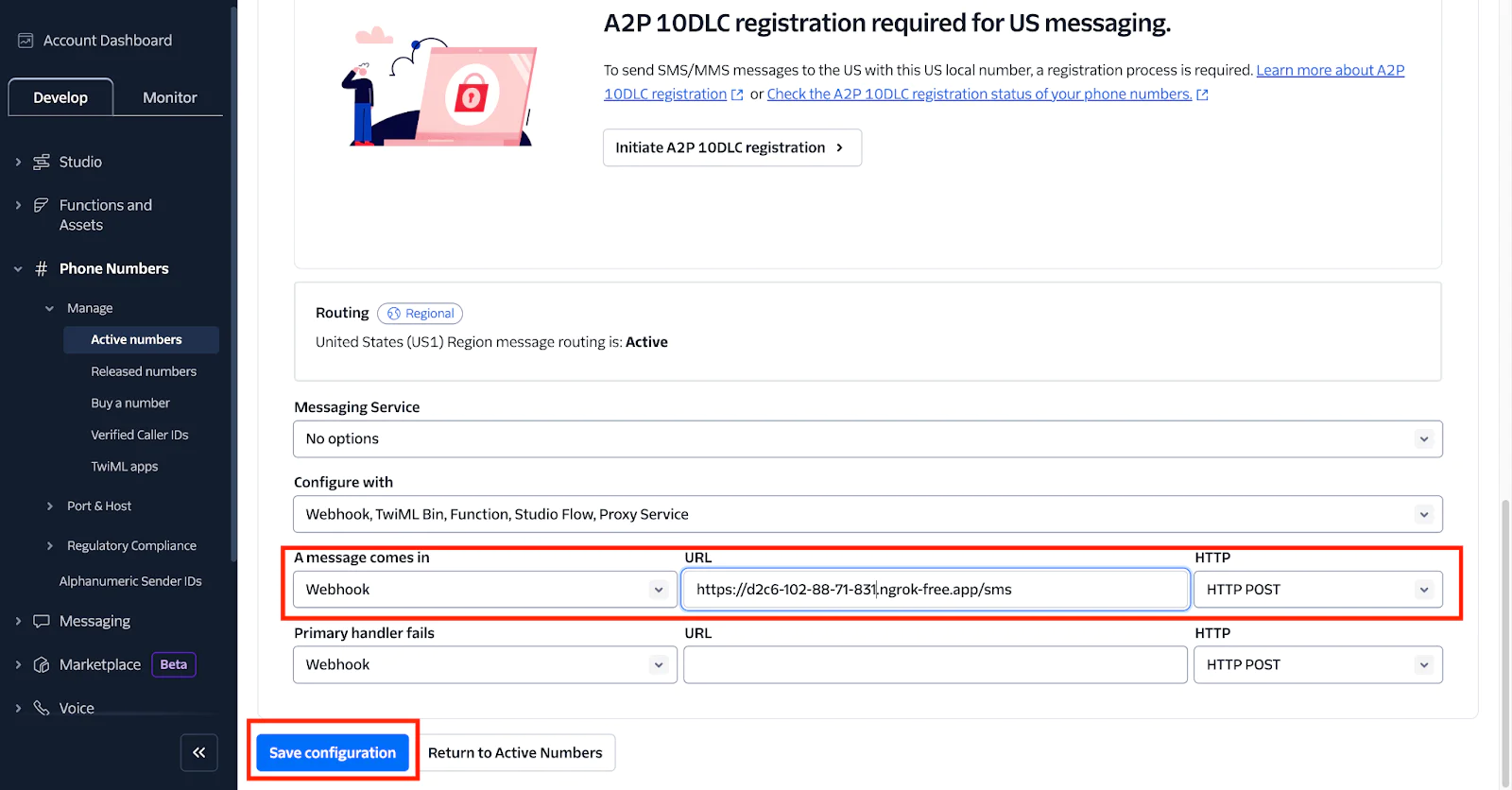
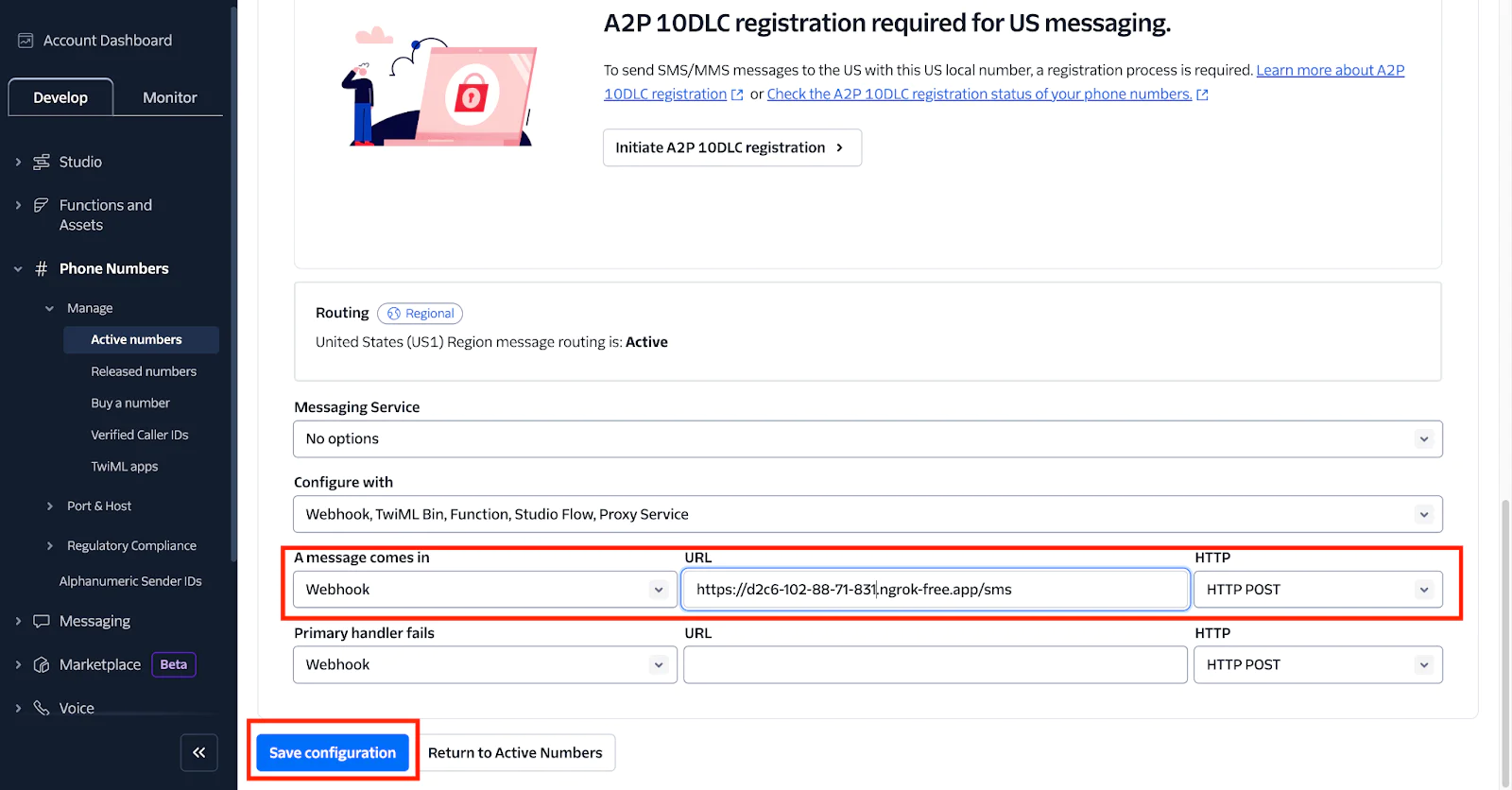
Test the application
To test the application, open the SMS app on your phone and send your stock quote from the phone number you used to register for Twilio to your Twilio-generated phone number. After sending the message, you will receive the current stock price for that quote as shown below.
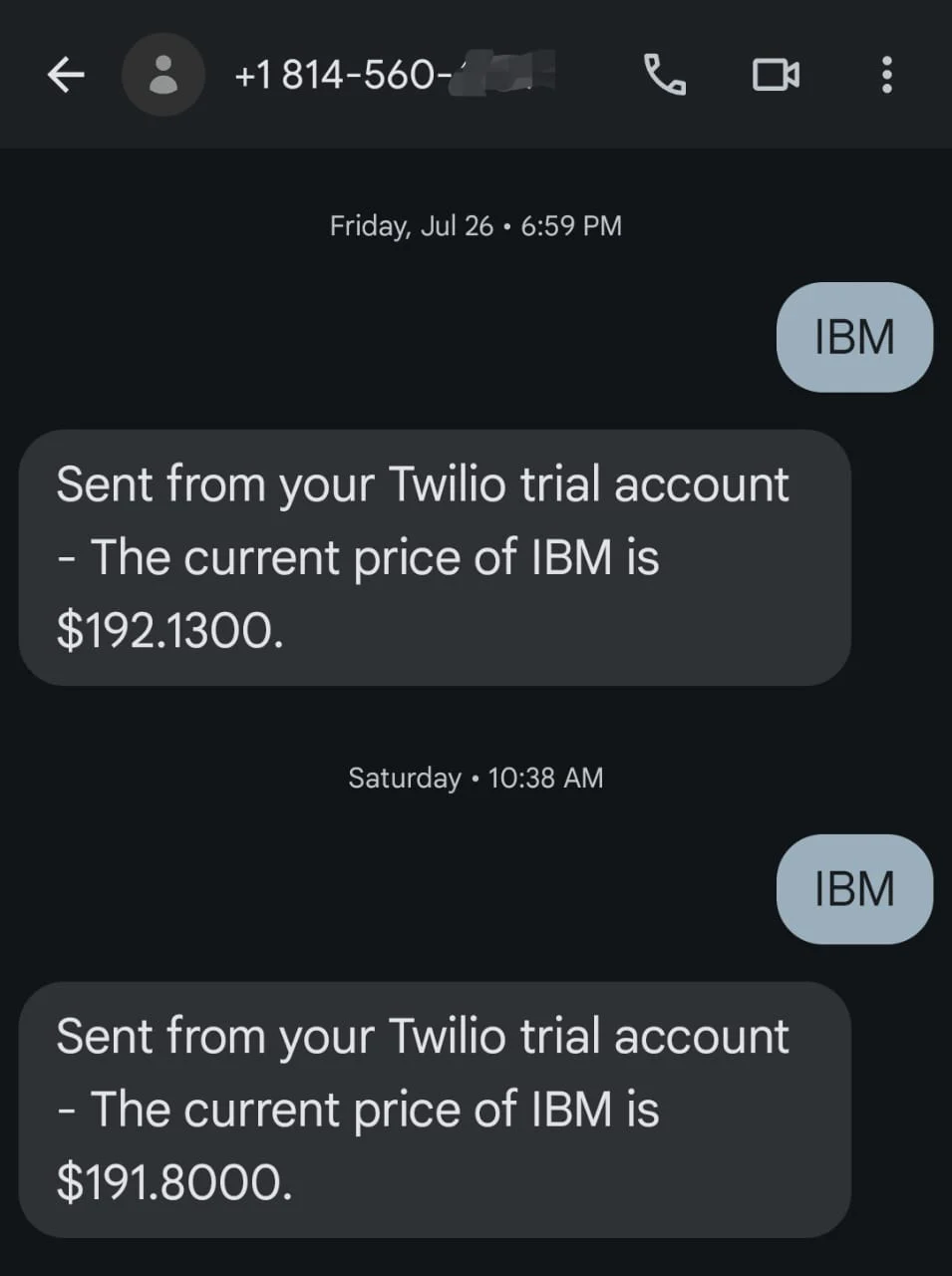
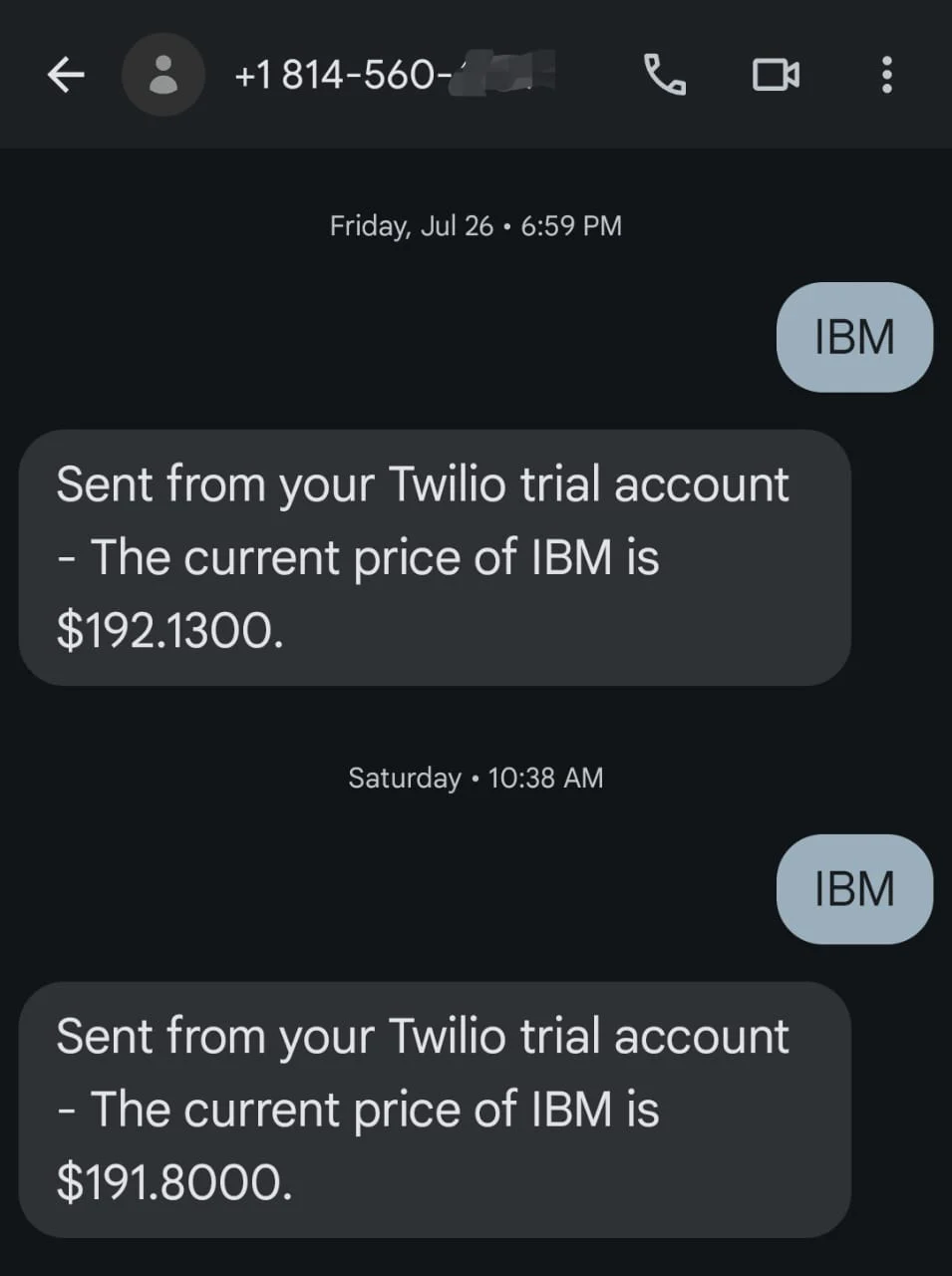
The image output displays IBM's current price at $192.130.
Retrieve the stock price
Below is an image showing the current stock price of different stocks using the newly generated API.
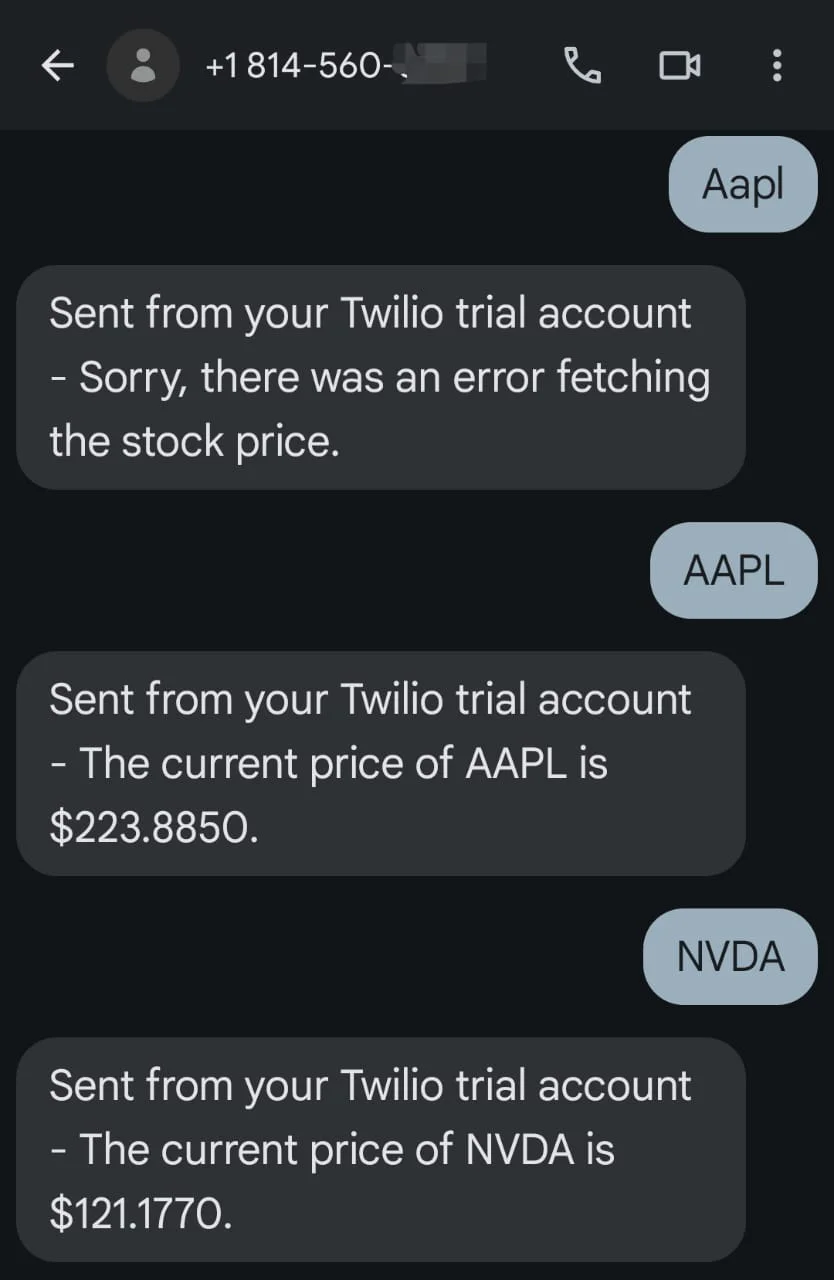
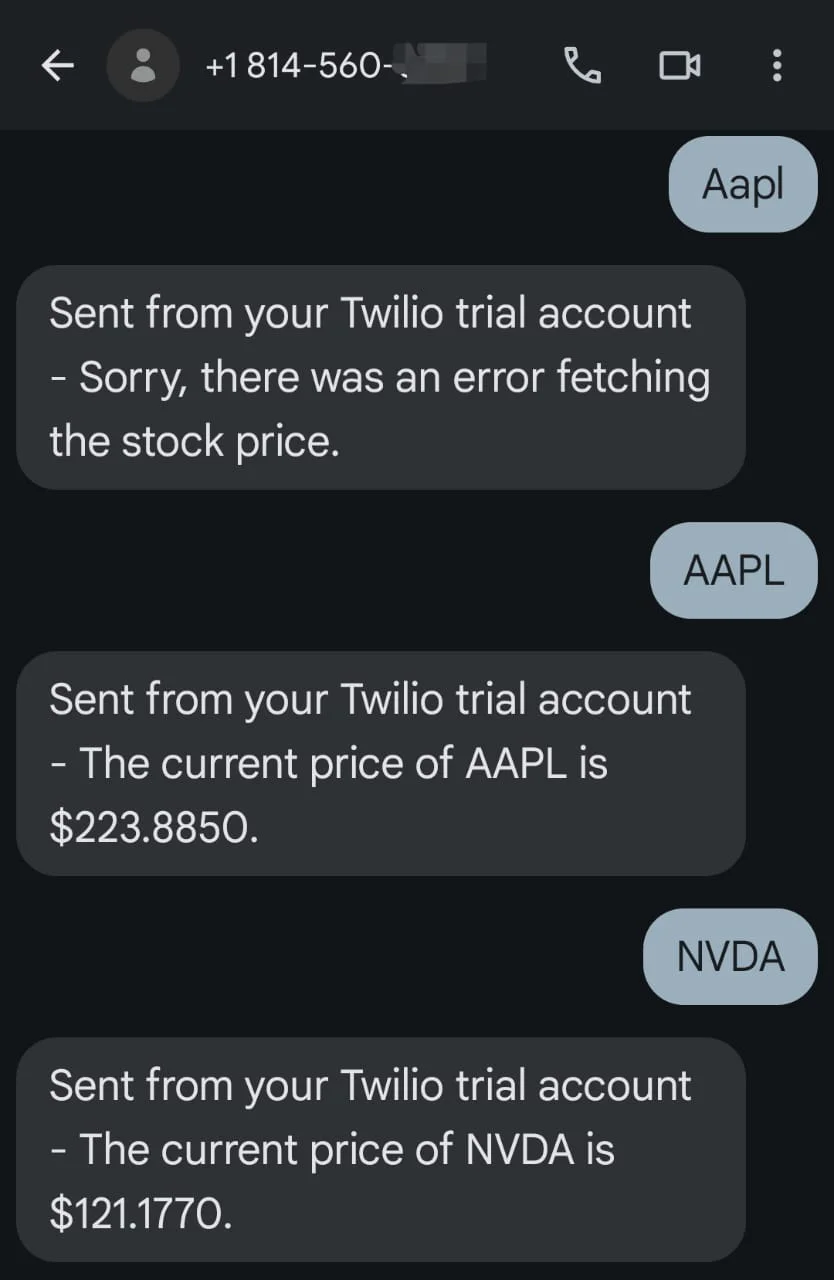
That's how to build an SMS stock price update app with Go and Twilio
In this tutorial, we developed a Go application to retrieve stock prices and send updates via SMS using Twilio. We covered setting up the environment, fetching stock prices, sending SMS, and enhancing the script with additional features. By following these steps, you can automate stock price notifications and tailor the program to suit your needs.
David Fagbuyiro is a software engineer, technical writer, and experienced technical content editor who is passionate about extending his knowledge to developers through technical writing. You can find him on LinkedIn.
Stock icon in the post's main image was created by ultimatearm on Flaticon.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.