Build a Patient Reminder System with Laravel and Twilio
Time to read: 5 minutes
Build a Patient Reminder System with Laravel and Twilio
Failure to take drugs on time can lead to deterioration in patient health, higher hospitalization rates, and numerous other complications such as the treatment failing.
So, sending confirmation voice call messages to patients, which remind them to take their prescriptions, and explain how to do so to prevent drug abuse, can lead to better health results. This can also increase the likelihood that patients are going to stick to their prescribed treatment plans accurately.
This tutorial will teach you how to add patient reminder call functionality into a Laravel application, using Twilio Programmable Voice.
Prerequisites
- Composer installed globally
- PHP 8.2 or higher
- A free or paid Twilio account. Create a free account if you are new
- MySQL database
- Docker installed
- For Windows users, you must install and enable the Windows Subsystem for Linux 2 (WSL2). It allows you to run Linux binary executables natively on your Windows operating system. Also, ensure Docker Desktop is configured to use the WSL2 backend.
- ngrok
- A cron-job account
Create a new Laravel project
Laravel is a sophisticated and attractive PHP web application framework that simplifies web application development with an MVC (Model-View-Controller) design.
To create a new Laravel project, run the command below:
Next, change into the new project directory, and start your Laravel application by running the following commands:
Set up the database
With your new Laravel project setup, you can now use Laravel Sail to spin up the development environment including setting up a MYSQL database. To do this, run the following command in your terminal:
Next, publish the Sail Docker configuration by running the command below:
Running this command will prompt you to select which services you want to include in your Docker setup with: "Which services would you like to install?". Select the MYSQL service and continue. Depending on your internet connection speed, the remainder of the process may take a few minutes to complete, so please be patient.
Next, run this command to start your Laravel Sail configuration including all of its services:
Create the database migrations
Then, execute the following command in your terminal to generate a new migration file:
Once the migration file is created, navigate to the database/migrations directory and open the most recent migration file (ending with create_patiends_table.php). Inside the file, replace the existing code with the following and save the changes.
The code above defines the table's columns such as the patient's name, phone number, and email address, as well as the drug dosage, name, and reminder time.
Now, apply the changes to your database schema by running the migration:
This command initiates the database migration process, ensuring that your database schema is synchronized with the most recent modifications defined in your application.
Generate the patient model
Generating the patient model is an important phase in managing database interaction. The patient model serves as an interface to the database's patients
table, which the migration created, allowing you to organize and efficiently perform multiple tasks with that information.
To generate the patient model, run the command below:
Next, open app/Models/Patient.php and update the file to match the following code:
The code above provides a way to interact with the patients
table in your database, and also allows you to run any query while ensuring that only the specified fields can be mass-assigned.
Install the Twilio PHP Helper Library
The Twilio PHP Helper Library acts as a bridge to Twilio's APIs, enabling you to integrate and utilize them in your PHP application.
Install the helper library by running the command below in your terminal:
Retrieve your Twilio details
The next thing you need to do is to retrieve your Twilio access credentials (your Account SID and Auth Token) and your Twilio phone number. The credentials are necessary for authenticating the application's communication with Twilio's Programmable Voice API.
First, however, add the following environmental variables to the end of .env, so that you have a place to store the details.
Then, log in to your Twilio dashboard, where you'll find your Account SID and Auth Token in the Account Info section, as shown in the screenshot below.
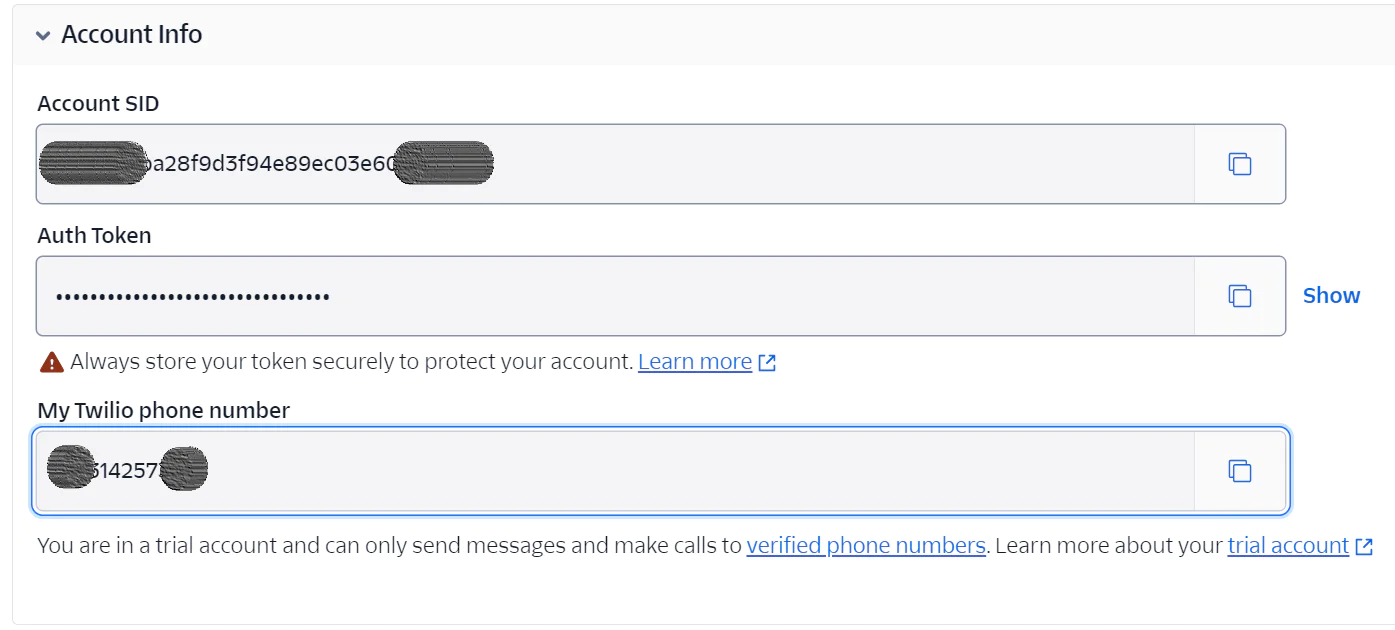
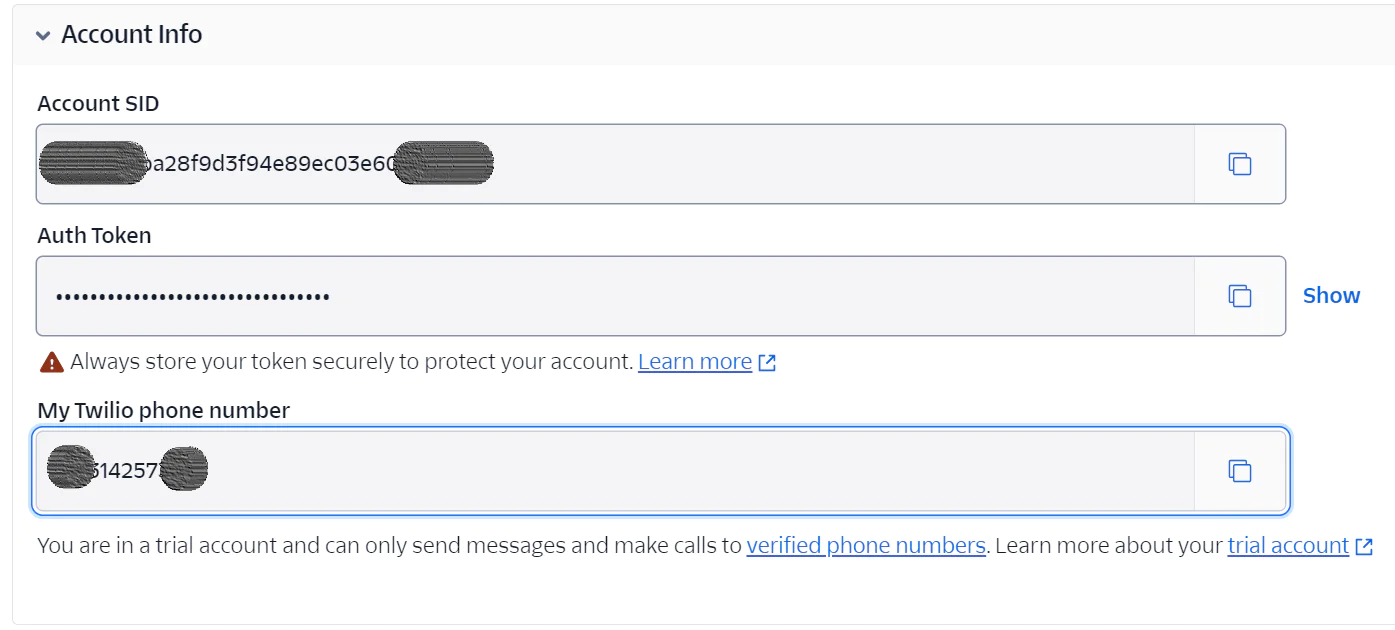
Finally, replace the three TWILIO_
placeholders in .env with the respective Twilio credentials that you just retrieved from the Twilio dashboard.
With that done, let's proceed to the next step of creating the controller.
Create the patient reminder controller
The patient reminder controller will handle the logic for interacting with Twilio's Programmable Voice API to send the patient reminders in your application. To create it, run the command below:
Next, open the new file (app/Http/Controllers/PatientController.php) and update it to match the following code:
The code defines four methods:
addPatientForm: This method displays the form for adding patient information.
addPatient: This method adds a new patient, checks the incoming request information provided with the form, ensures that all required fields are completed, and then redirects back to the form if the patient was successfully added.
sendMedicationReminders: This method is in charge of delivering medication reminders to patients and retrieving patients whose medication reminder time has either expired or is equal to the present time.
placeCallReminder: This method accepts your Twilio account credentials (Account SID and authentication token) along with your Twilio phone number, and sends a reminder call to the patient.
Create the required Blade template
Next, create a new file, add-patient.blade.php, in the resources/views directory and add the code below to the file:
The code above creates a patient registration page, capturing details such as the patient's name, email, gender, phone number, drug name, dosage, and reminder time.
Update the routing table
Now, create the route for this page by navigating to the routes/web.php and updating it to match the following code:
The code above defines the endpoints and the HTTP methods that are associated with various actions in your application; two for displaying the add patient form and processing it, and two for sending medication reminders to the patient.
Test the patient reminder application
Next, run the command below to expose the application to the public internet over secure tunnels:
You should see terminal output like the screenshot below:


Then, copy the Forwarding URL and paste it into your web browser's address bar. Once the page loads, you'll see a form displayed on your screen. Fill out the form with the required information, as shown in the image below, and submit it.
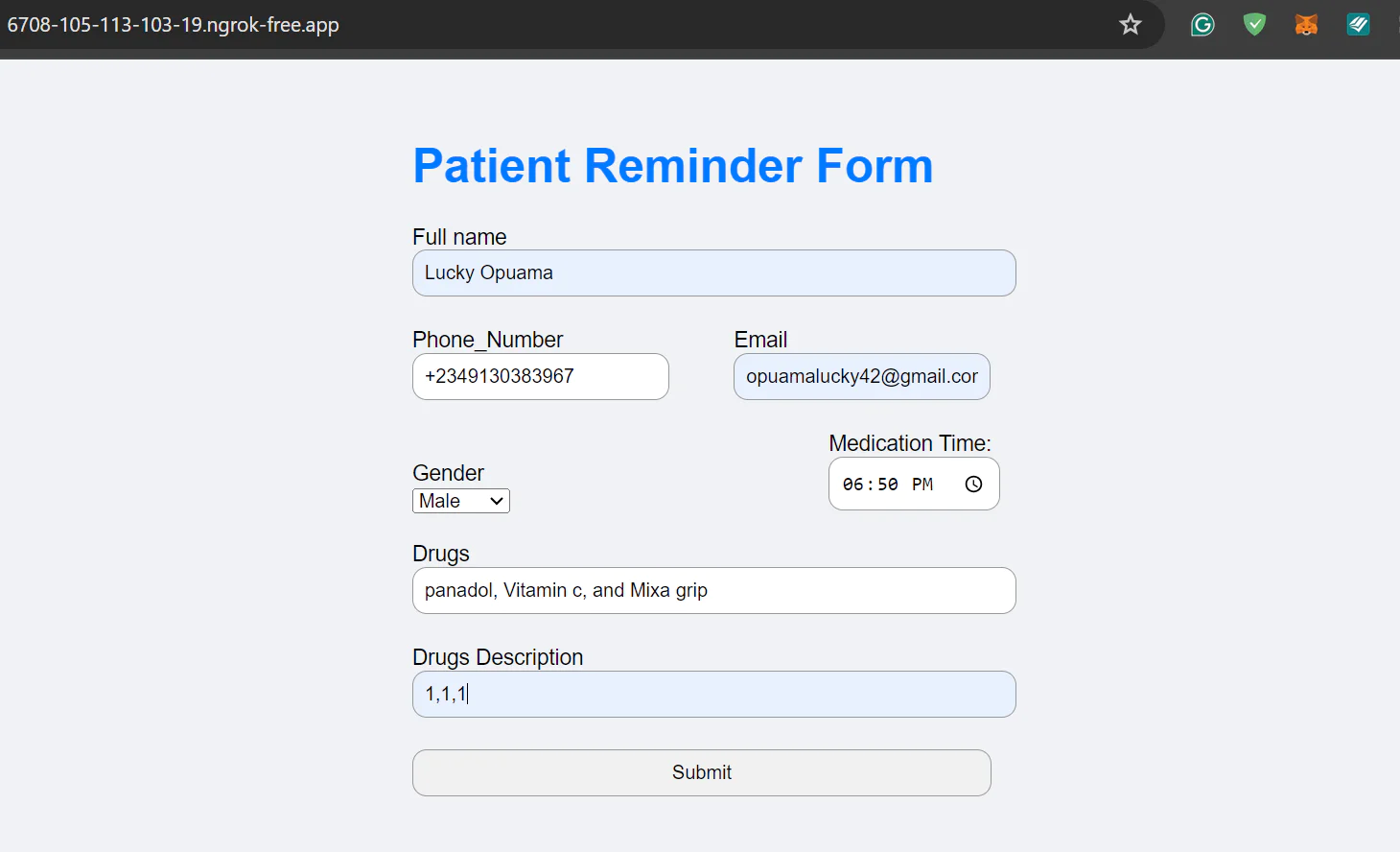
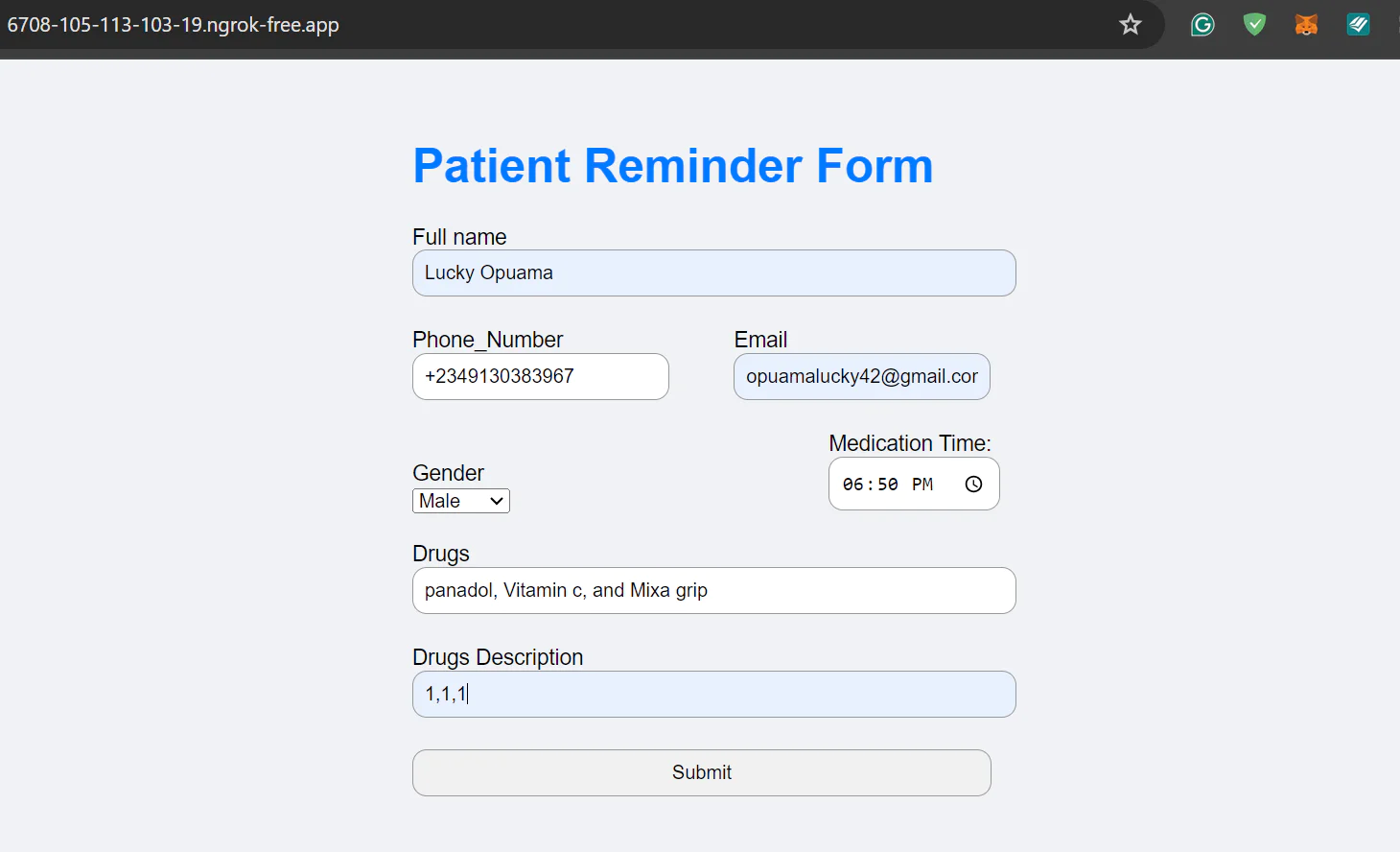
Congratulations! You've successfully added a new patient to your database.
Schedule medication reminders
To schedule medication reminders, follow these steps:
Log in to your cron-job.org dashboard
Click on CREATE CRONJOB to create a new cron job
Enter the details for the new cronjob, using the ngrok Forwarding URL plus
/send-medication-reminders
for the URL field, along with specifying the timing for the reminders, similar to the screenshot below.- Click the CREATE button to finalize the setup
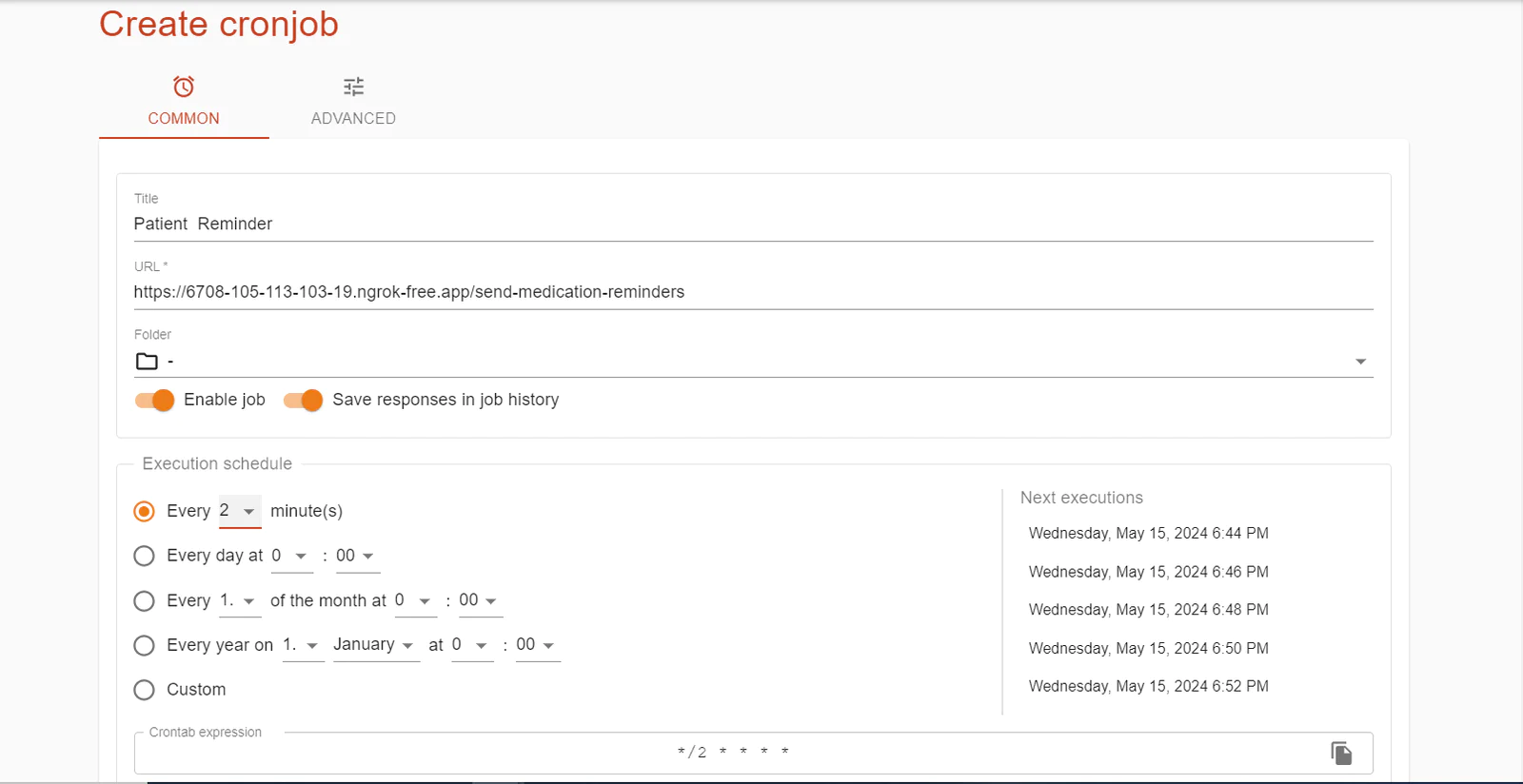
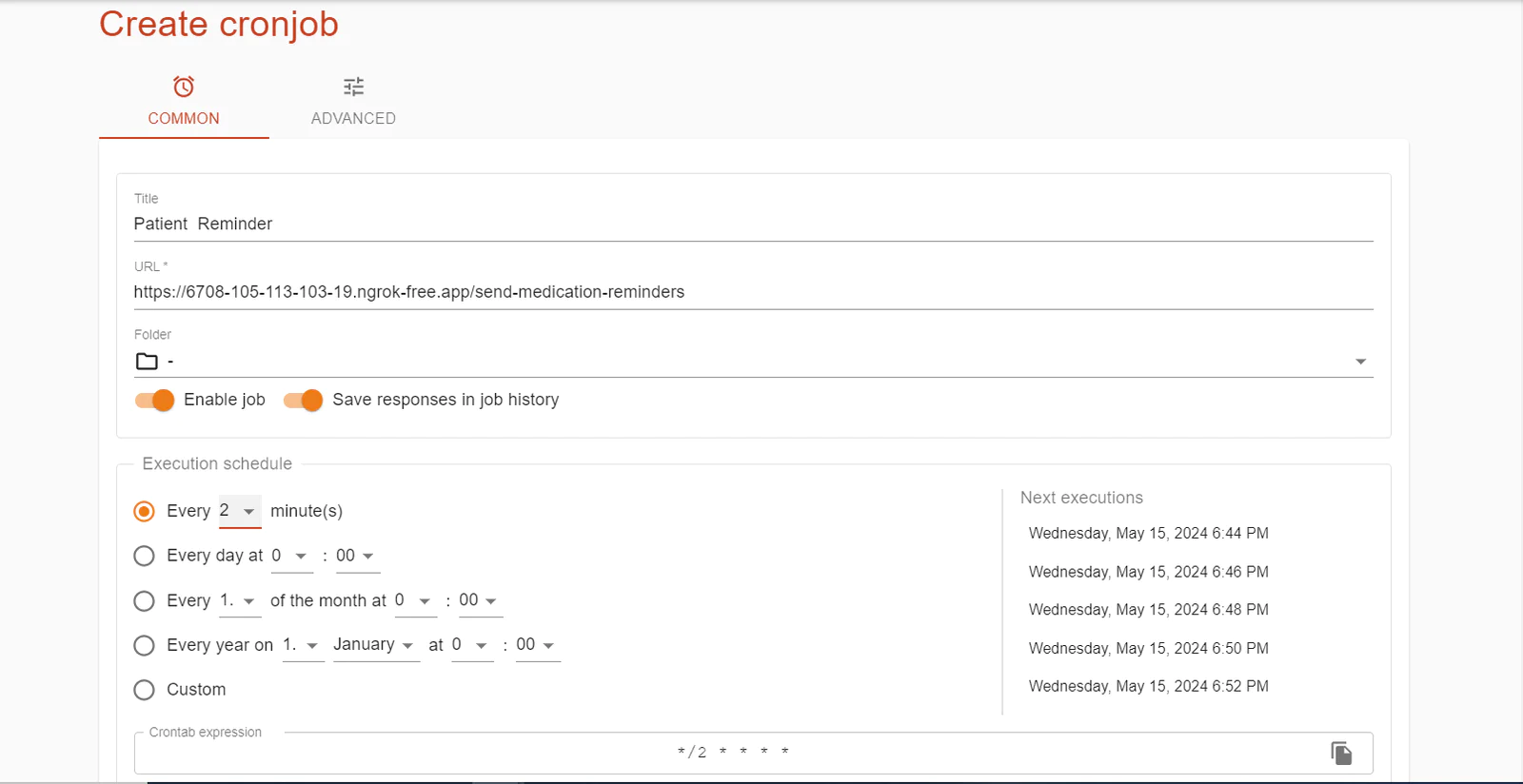
When the scheduled time stored in the database elapses, you will receive a call, as configured in your cron job, reminding you to take your medication. During the call, you will not only be reminded verbally, but you will also hear the names of the drugs and their dosages.
That's how to integrate patient reminder calls with Laravel, Cron Jobs, and Twilio
Patient reminder calls are an effective solution for healthcare applications that want to enhance drug adherence and patient outcomes. In this article, we integrated a patient reminder voice call system in Laravel using Twilio Programmable Voice and automated scheduling to trigger the calls at the relevant times.
Lucky Opuama is a software engineer and technical writer with a passion for exploring new tech stacks and writing about them. You can find them on LinkedIn.
Patient icon in main image was created by RaftelDesign - Flaticon.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.