Build a Morse Code Application Using Go, Ngrok And Twilio
Time to read: 5 minutes
Build a Morse Code Application With Golang, Ngrok and Twilio
Morse Code is a way of encoding written letters as sequences of dots and dashes. It has been a dependable communication mechanism for over a century. While Morse Code is no longer commonly used in ordinary communication, developing a Morse Code application is an excellent method to improve your programming skills.
In this article, you’ll learn how to create a simple Morse Code application that converts text to Morse Code and sends it as an audio message using Twilio. Ngrok will be utilized to expose your locally running application to the internet, allowing it to receive Twilio webhooks.
Prerequisites
Before diving into the code, ensure you have the following installed:
- Go 1.22 or above
- A Twilio Account, either free or paid; create a new account if you don’t already have one
- A Twilio phone number
- Ngrok to expose the application publiclyon the internet
Project overview
Below is brief step by step explanation of what will be covered in the tutorial:
- Create a web app that can receive HTTP requests: Go will be used to build a web app that not only listens for HTTP requests, but also decodes the Morse code messages which they contain and triggers a phone call where the recipient can hear the Morse code as an audio message. To achieve this, you'll create a specific endpoint within the Go app to handle the voice requests forwarded by Twilio, allowing you to process and respond to it.
- Configure your Twilio phone number to respond to incoming voice calls: You'll configure your Twilio phone number to respond to incoming voice calls with a webhook that directs Twilio to send requests to our Go application.
- Create a function to convert text to Morse Code: You’ll write a Go function that takes text input and converts it into Morse Code, translating each character into its corresponding sequence of dots and dashes. Once you have the morse code, you’ll utilize TwiML to generate audio tones for the Morse Code, enabling the application to play back the Morse Code as sound during voice interactions.
Set up the project
First, create a new directory for your project, change into it, and initialize a new Go module inside it, by running the following commands:
After initializing the project, you then need to install the required dependencies required for this project:
- github.com/joho/godotenv: This dependency is used to load variables from a .env file as environment variables, accessible in your Go application. Environment variables are typically used to store information such as API keys, authentication tokens, and configuration settings, keeping them out of your code.
- github.com/twilio/twilio-go: This is the official Twilio Go Helper Library, which provides a set of tools and functions to simplify interacting with Twilio's APIs. It simplifies the process of sending and receiving SMS messages, making voice calls, and handling various communication tasks. For this project, it will be used to simplify sending SMS messages containing Morse Code, as well as for handling incoming messages or voice calls directed to your Twilio phone number.
Run the following command to install them:
Download the beep sounds
You need to download the beep sound audio files for this project. They can be found via this link. Once you do that, create a folder named static and inside it create another folder called audio. This is where the downloaded sounds need to be stored.
Set up ngrok
Next, expose port 8080 on your local machine to the public internet, by running the command below.
You should see your ngrok running like this:
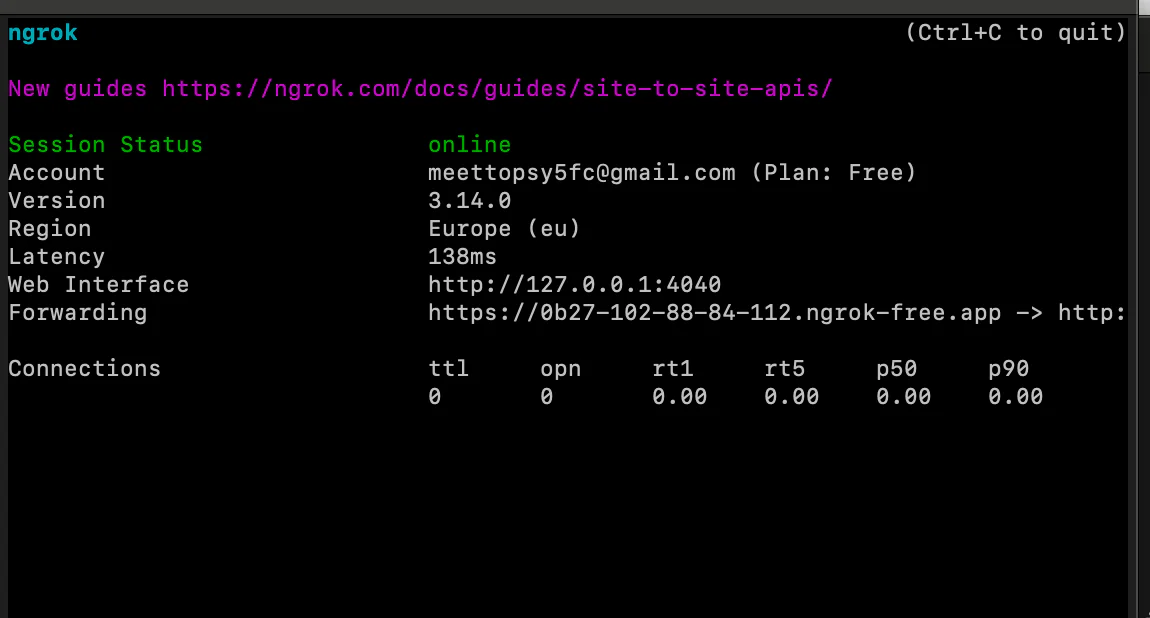
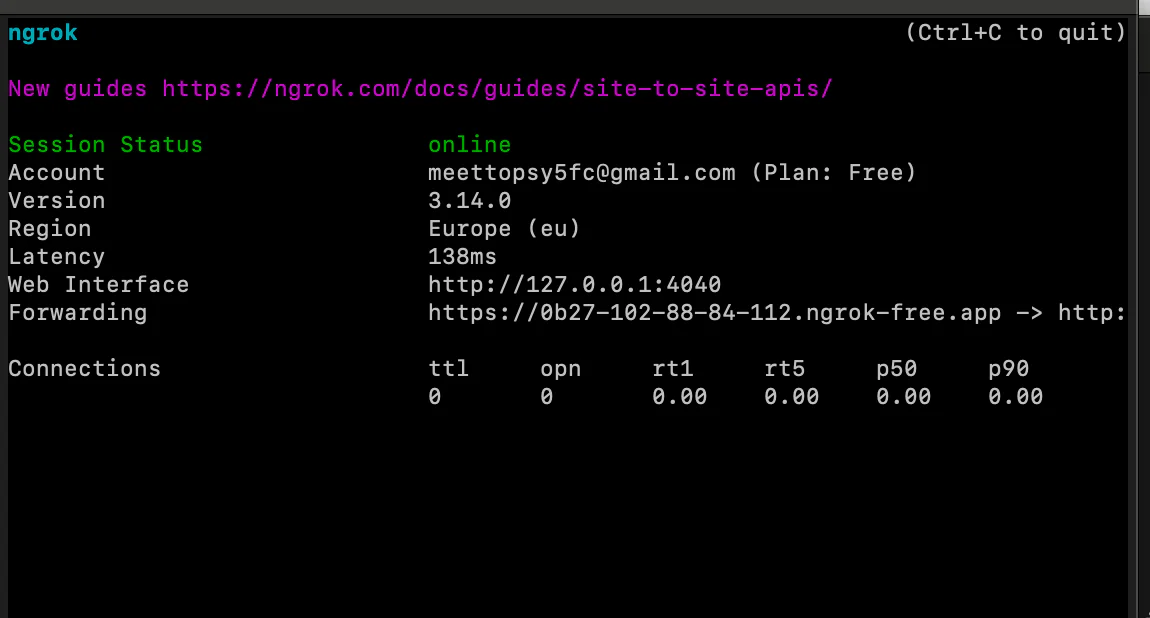
ngrok will provide you with a public (Forwarding) URL in its terminal output. You’ll need it for one of your functions that will be created when building to help expose your local web server to the internet, so copy it and keep it handy.
Set up the environment variables
Create a .env file in the top-level directory of your project, and add the configuration below to the file:
Then, to get your Twilio credentials, open the Twilio Console and, from the Account Info panel on the main dashboard, copy your Account SID, Auth Token, and phone number. Then, replace the <<your_account_sid>>
, <<your_auth_token>>
, and <<your_twilio_phone_number>>
placeholders, respectively, with the details that you just copied.
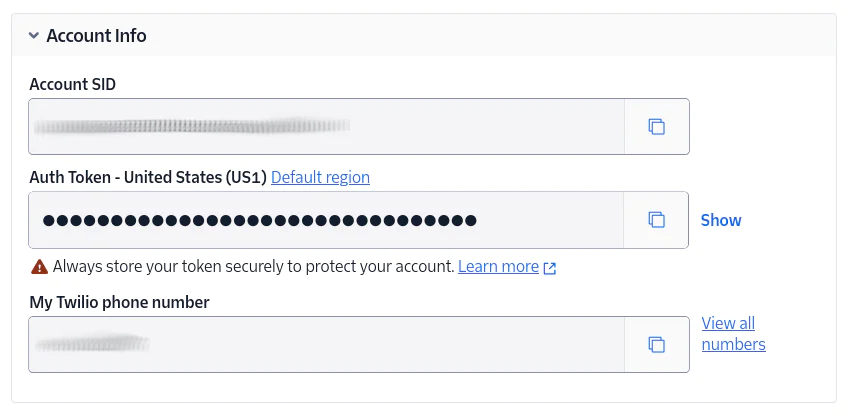
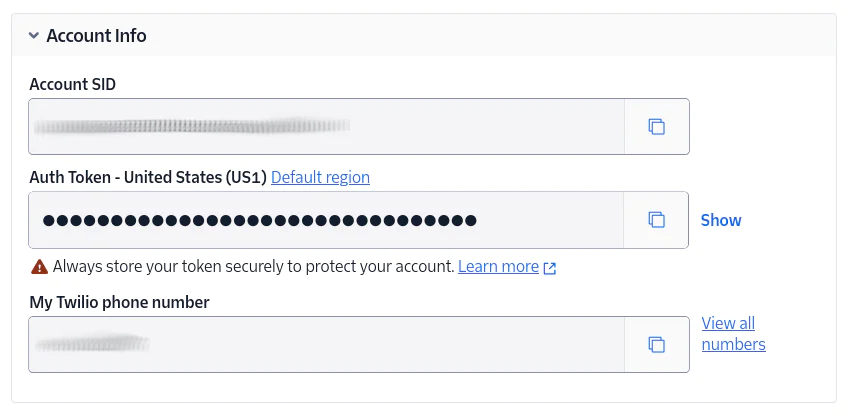
Build the project
Now that you have everything set up, let’s start writing Go code.
Import dependencies and define Morse Code mapping
First, create a new Go file, named main.go. Then, paste the code below into the file to add the necessary imports and define the Morse Code mapping:
This code imports the necessary packages, and defines a map for Morse Code whereeach letter and number is associated with its Morse Code representation.
Define the TwiML structures
You next need to define two structsthat define the XML format for Twilio's TwiML, which you'll use to generate the audio for the Morse Code. Paste the following after the initialisation of MorseCode in main.go.
Implement Morse Code conversion
Now, let's add two functions to convert text to Morse Code, and Morse Code to TwiML by pasting the following at the end of main.go:
The textToMorse()
function converts a string to its Morse Code representation. morseToTwiML()
converts Morse Code to TwiML, using short tones for dots, long tones for dashes, and pauses for spaces.
Implement the HTTP handlers and the main function
Now, you need to create three handler functions: one for the index page, one to handle form submission, and one for receiving and processing the Twilio webhook. To do that, add the following three functions at the end of main.go.
The handleIndex()
function handles requests to serve the HTML form, handleSubmit()
handles requests to process form submissions, and handleVoiceRequest()
initiates Twilio voice calls.
Finally, let's implement the main()
function to tie everything together, by pasting the following at the end of main.go:
Create the HTML form
Create a file named index.html in the top-level directory of your project with the following content:
This is just a basic HTML form that allows the users to input a text message and a phone number. When the form is submitted to the "/submit" POST route, the provided message and phone number will be processed by the server to initiate a phone call that transmits the message in Morse code.
Run the application
You already started ngrok earlier in the tutorial, so you just need to run the Go code now. To do so, run the following command:
Now, open http://localhost:8080/, where you'll see something it render like the screenshot below:
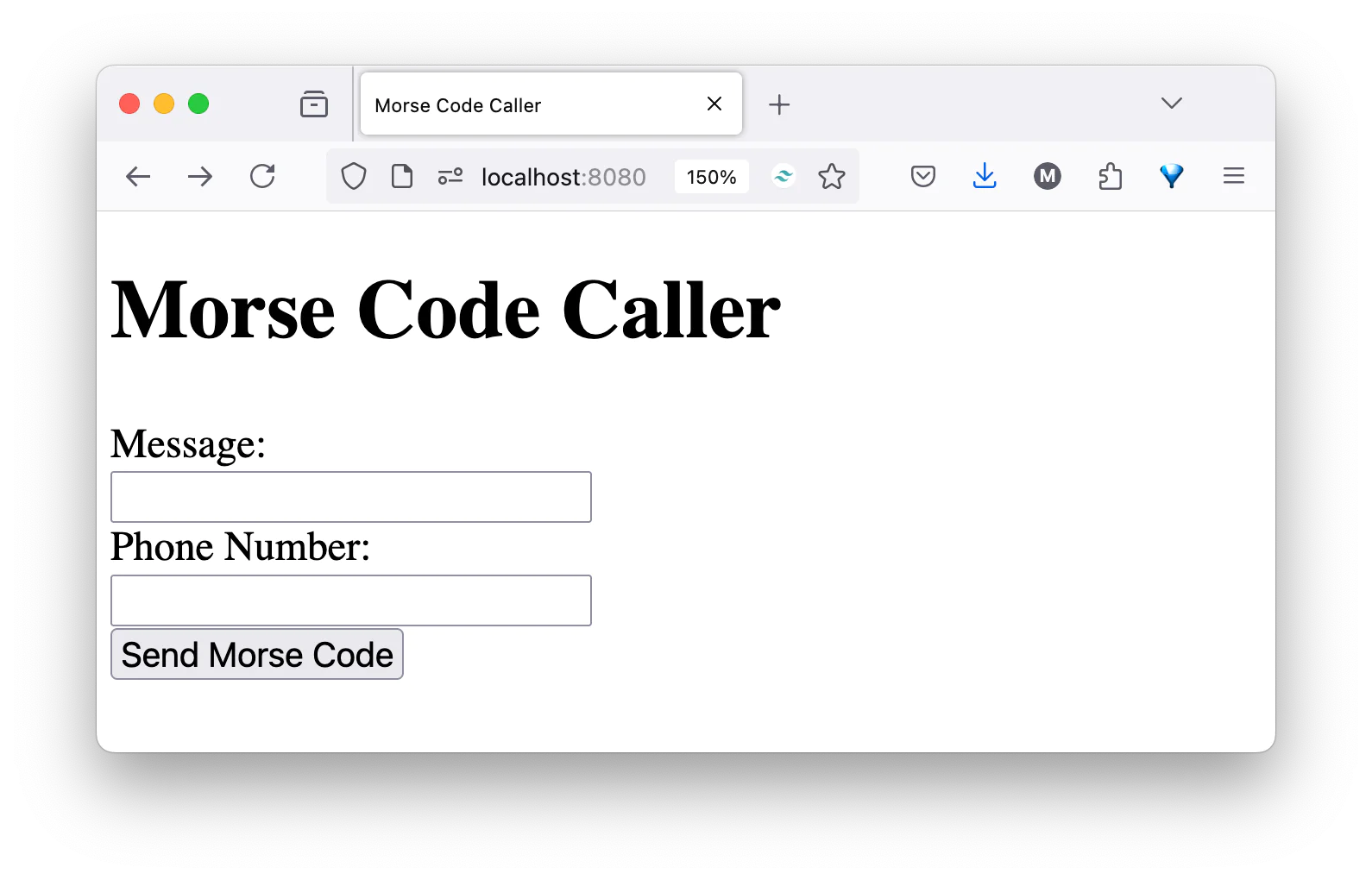
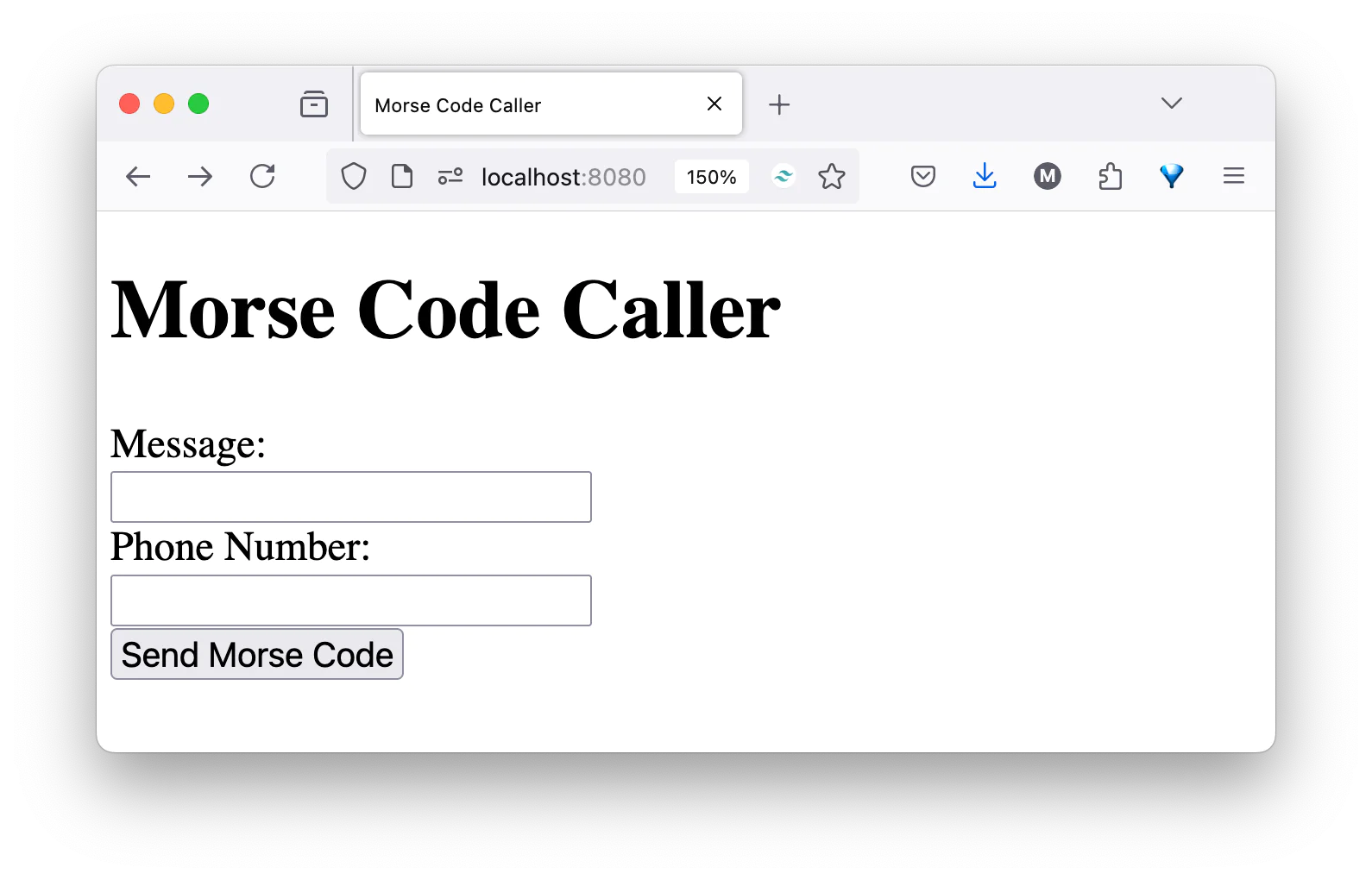
Fill out the form and submit it. You'll see "Call initiated to <the phone supplied number> with message: Here is a message" output to the browser, and then the app will generate a Morse Code audio file for the message that you wrote and send it to the specified phone number.
That’s how to build a Morse Code application in Go
In this article, you built a web application that converts text to Morse Code and initiates a phone call to play it. Used Go for the backend, Twilio for making calls, and created a simple HTML form for the user interface. Ngrok was also used to make your local server accessible to Twilio.
This project shows how flexible twilio can be for creating anything! It also demonstrates how to work with web servers in Go, interact with external APIs, generate audio output through phone calls, and use tunneling services for local development.
Tolulope Babatunde is a software developer with a passion for translating complex concepts in clear content through tech writing.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.