Build an Automated Appointment Reminder Service with Go and Twilio
Time to read: 3 minutes
Build an Automated Appointment Reminder Service with Go and Twilio
Building an automated appointment reminder system using Go and Twilio can streamline communication by sending reminders to clients via SMS. This helps reduce no-shows and improves the overall efficiency of appointment-based businesses.
In this tutorial, you will learn how to build an automated appointment reminder system in Go using the Twilio Programmable Messaging API.
Prerequisites
To follow along with this tutorial, ensure you have the following requirements.
- Go 1.22 or above installed on your computer
- A Twilio account (free or paid) with an active phone number. If you don't have an account, sign up today.
- Access to a MySQL database
Initialize a new Go project
To get started, open your terminal and navigate to the directory where you want to create the new Go project. Then, run the command below to initialize the project.
After running the commands above, open the project in your preferred IDE or code editor, such as VS Code.
Install the required dependencies
Let’s first install the Twilio Go package to interact with the Twilio Programmable Messaging API. Run the command below to install the package.
In our project, we will also need to install gorilla/mux to handle the application's user interface, the Go MySQL driver to store patient appointment records, cron to send reminder SMS to patients with appointments scheduled for the next day, and GoDotEnv to manage environment variables.
To install these packages, run the command below.
Create the dotenv file
In the project’s root folder, create a file named .env and add the following environment variables to it.
From the code above, replace the <account_SID>
, <auth_token>
and <phone_number>
placeholders with your corresponding Twilio values.
Provision the database
Now, login to your MySQL server and create a new database named reminder
. After creating the database, create a table named "appointments" with the following fields:
- id:int(11) Primary Key Auto Increment
- patient_name:varchar(99)
- doctor_name:varchar(99)
- patient_phone varchar(99)
- appointment_date:date
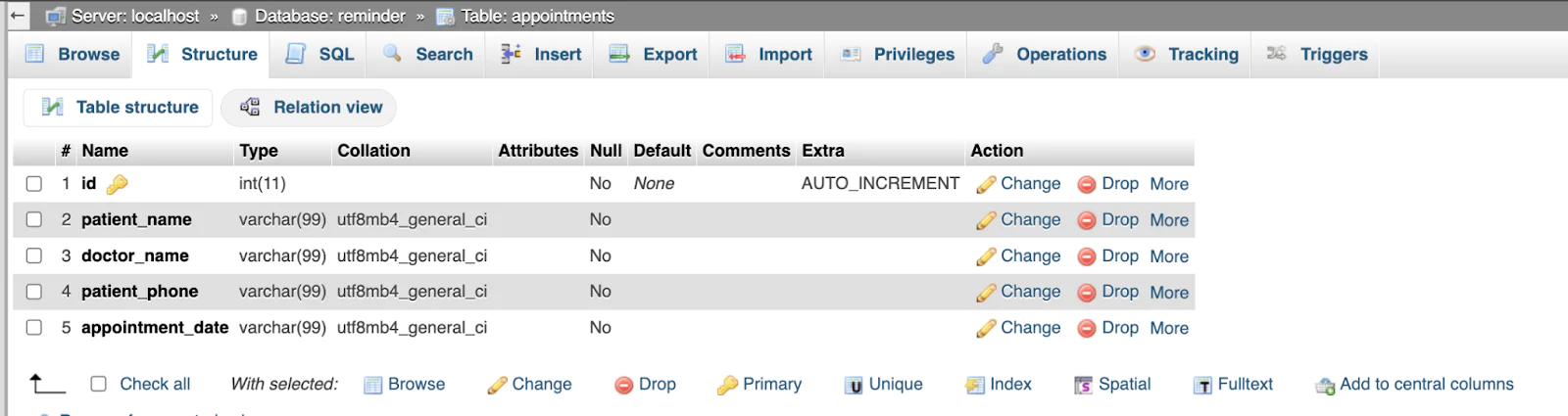
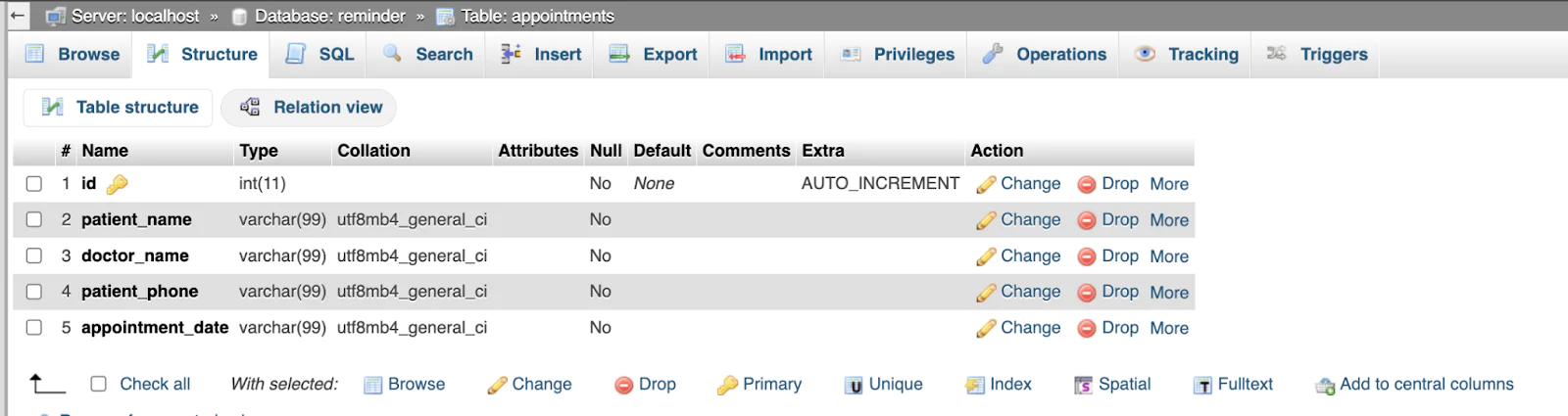
Retrieve your Twilio credentials
To connect your Go application to your Twilio account, log in to your Twilio dashboard to retrieve your credentials which will be used to authenticate the Twilio API calls. You'll find your Account SID and Auth Token under the Account Info section, as shown in the screenshot below.
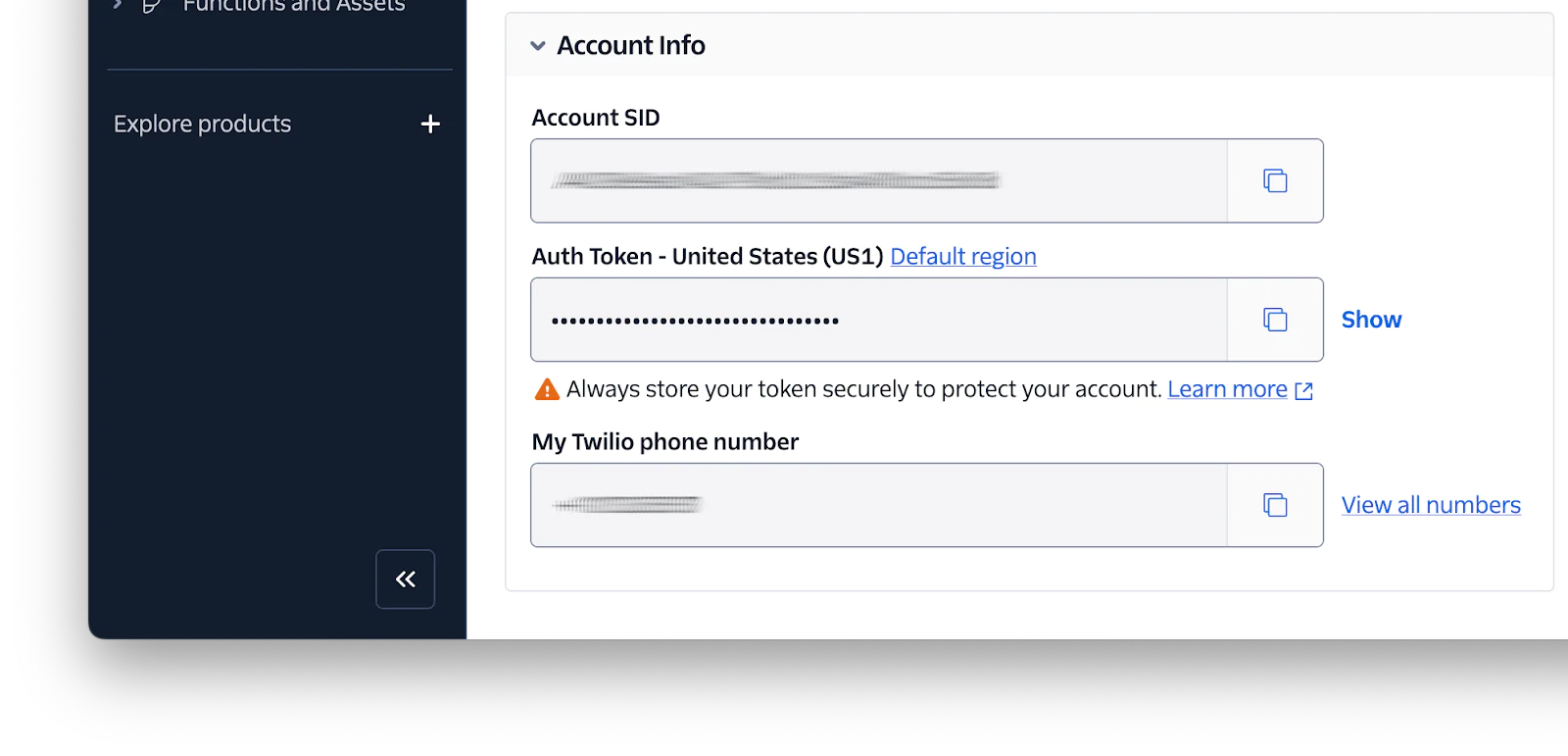
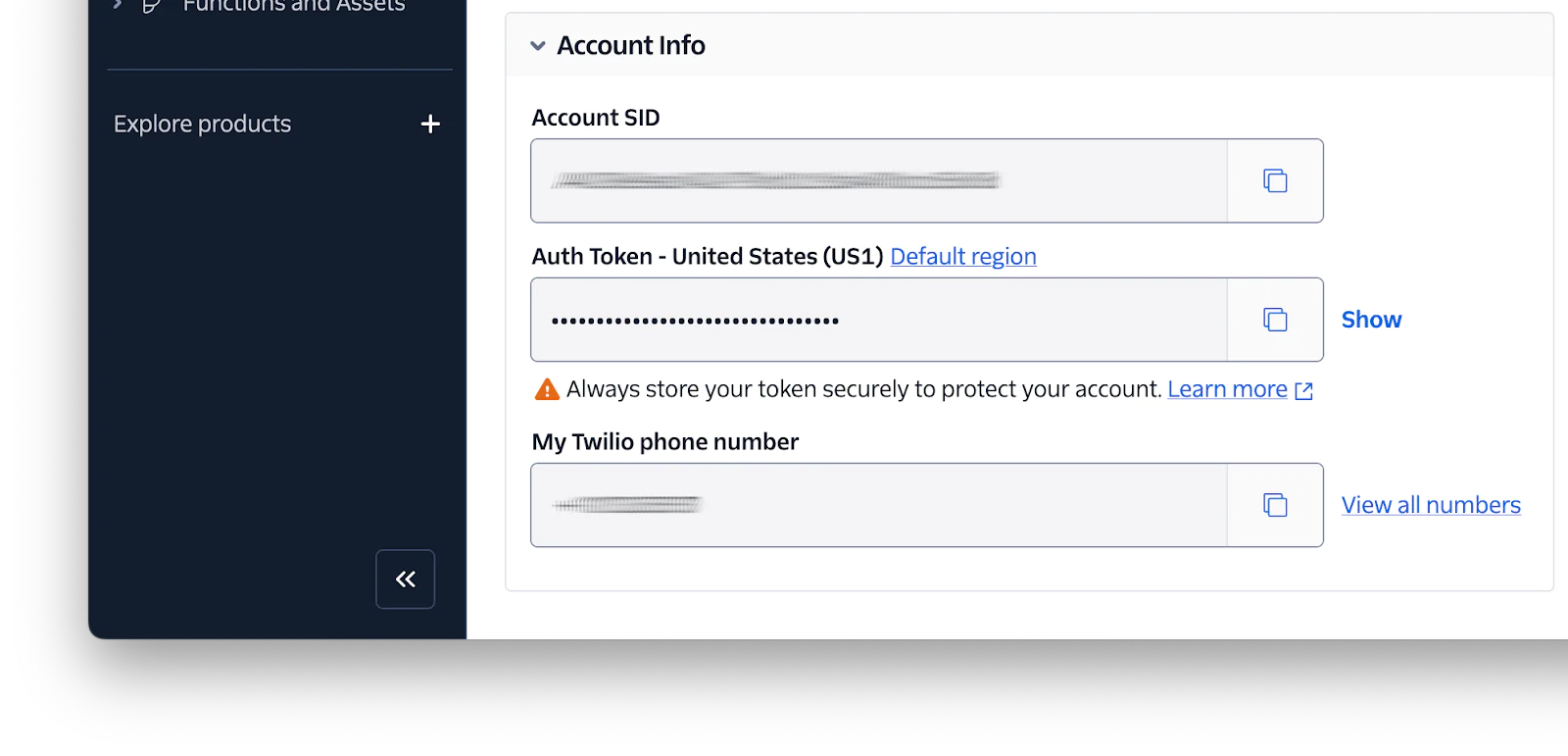
Create the application logic
Now, let’s create the application's core logic. To do this, create a file named main.go in your project folder.
From the code above, we:
- Import all the necessary packages and define the path to the application's booking HTML template
- Initialize the Twilio client, which connects to the Twilio endpoint using
accountSID
andauthToken
- Use the
initializeDatabase()
function to establish a connection to the database server
Next, let’s add the application functions to the main.go file. To do this, add the following code at the bottom of the main.go file.
From the code above:
- The
ServeAppointmentForm()
function is responsible for displaying the HTML form used to book an appointment - The
HandleAppointmentSubmission()
function processes the form submission and stores the appointment in the database - The
getNextDayAppointments()
function retrieves appointments scheduled for the following day - Finally, the
SendReminders()
function sends reminder SMS messages to patients with appointments scheduled for the next day
Create the application routes
Now, let's define the application routes and set up the cron job that will send reminder SMS to users one day before their appointment. To do that, in the main.go file, add the following code.
Create the application user interface
Let’s create a user interface where patients can fill in their appointment details. To do this, create an HTML file named appointment.html and add the following code to it.
Test the application
To test the application, start the application development server by running the command below.
After starting the application, open http://localhost:8080 in your browser of choice and book an appointment with a doctor.
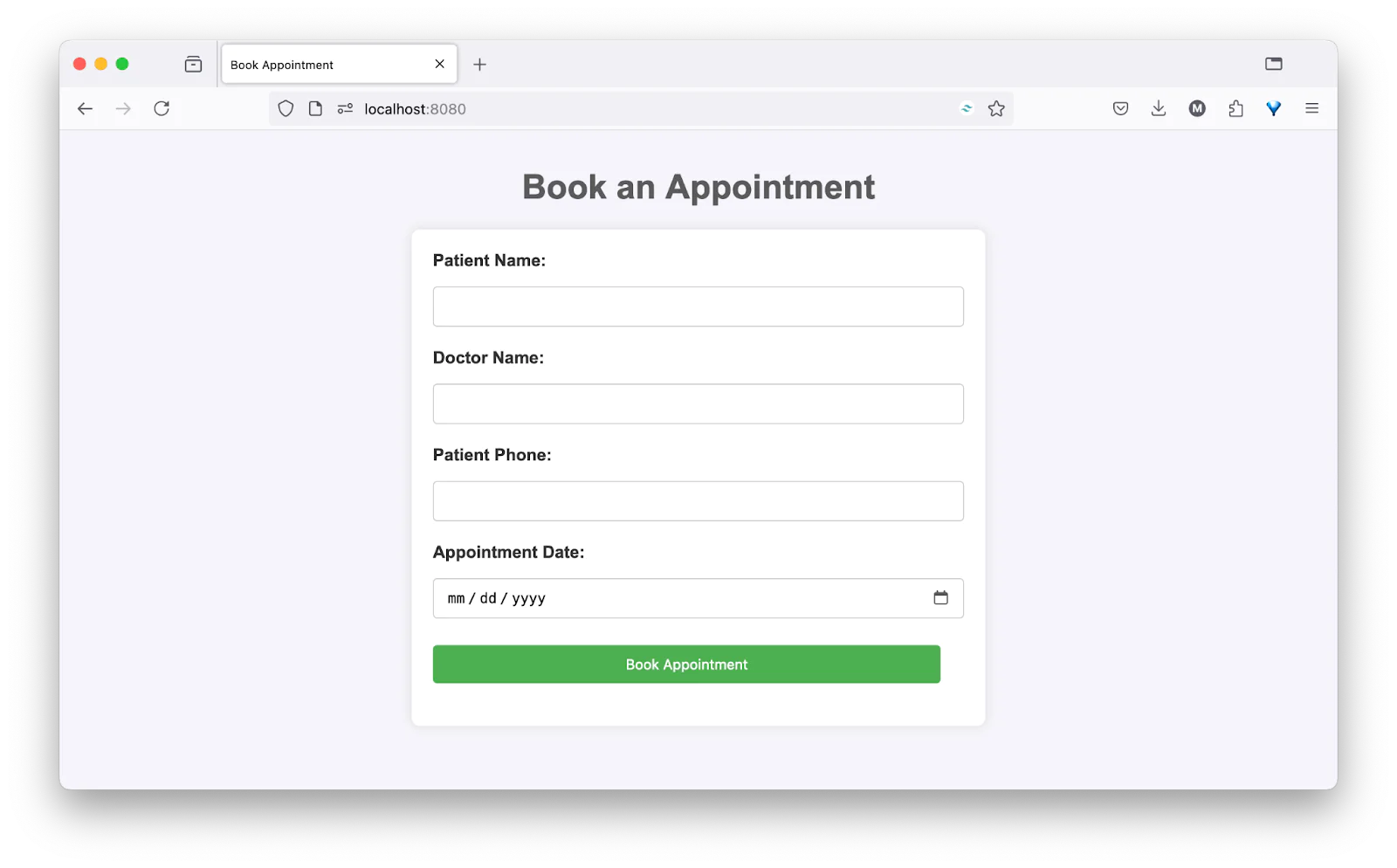
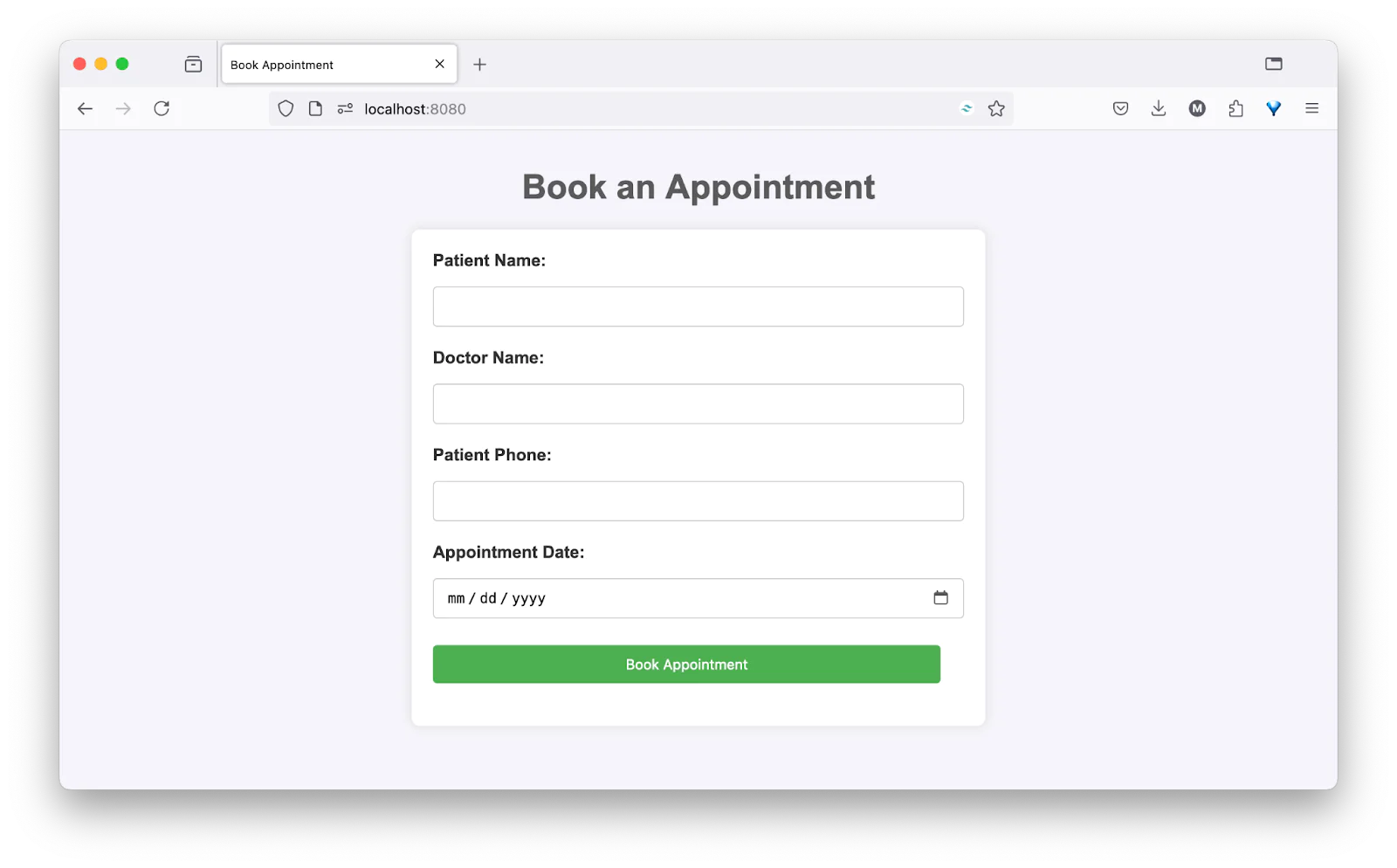
Once your appointment is successfully booked, you will receive a confirmation SMS, as shown in the image below.
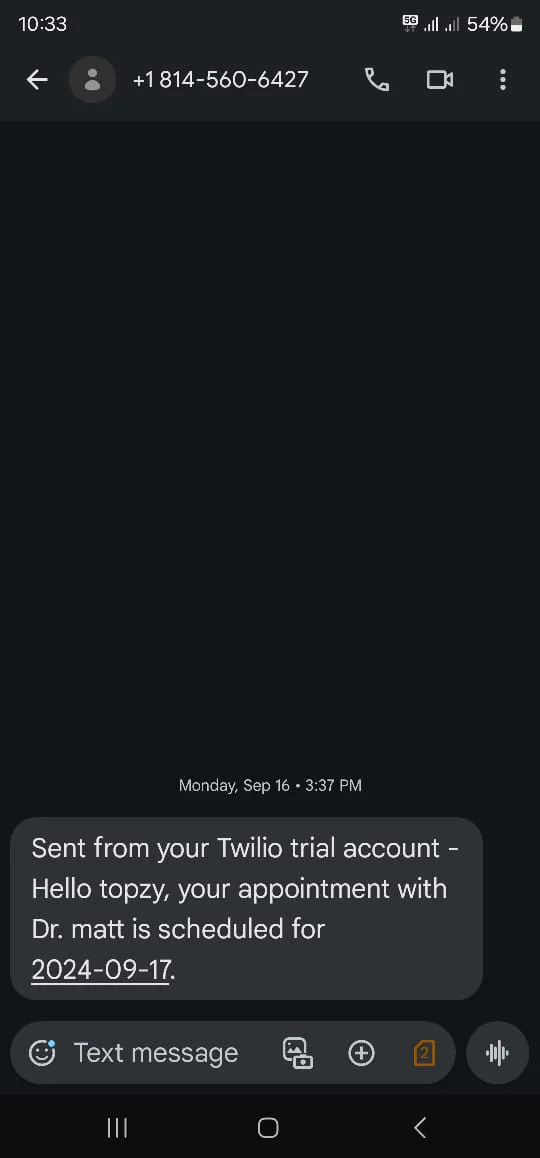
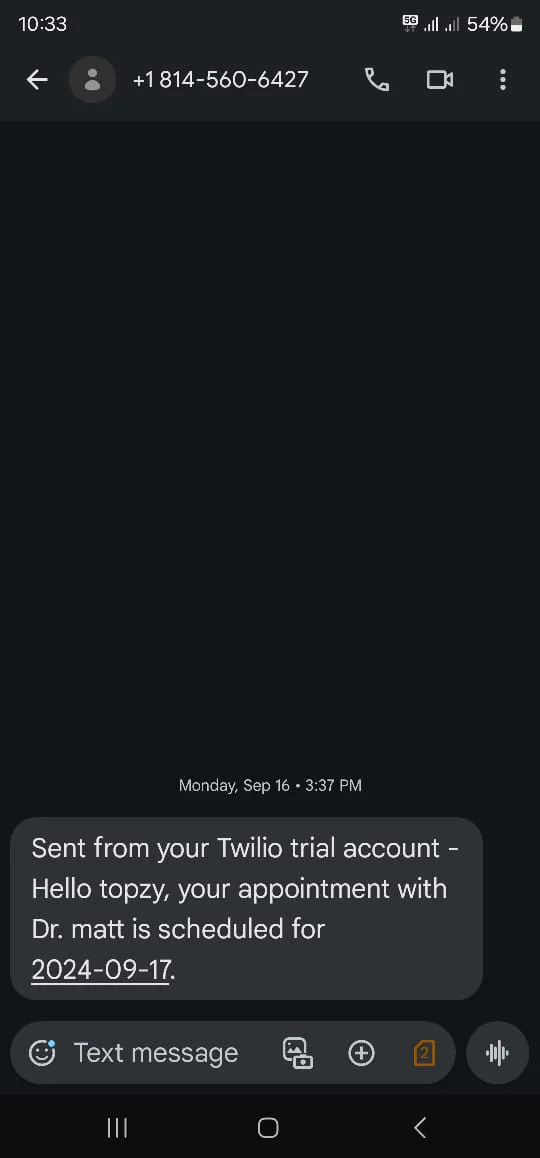
One day before your appointment with the doctor, you will receive a reminder SMS message, as shown in the screenshot below.
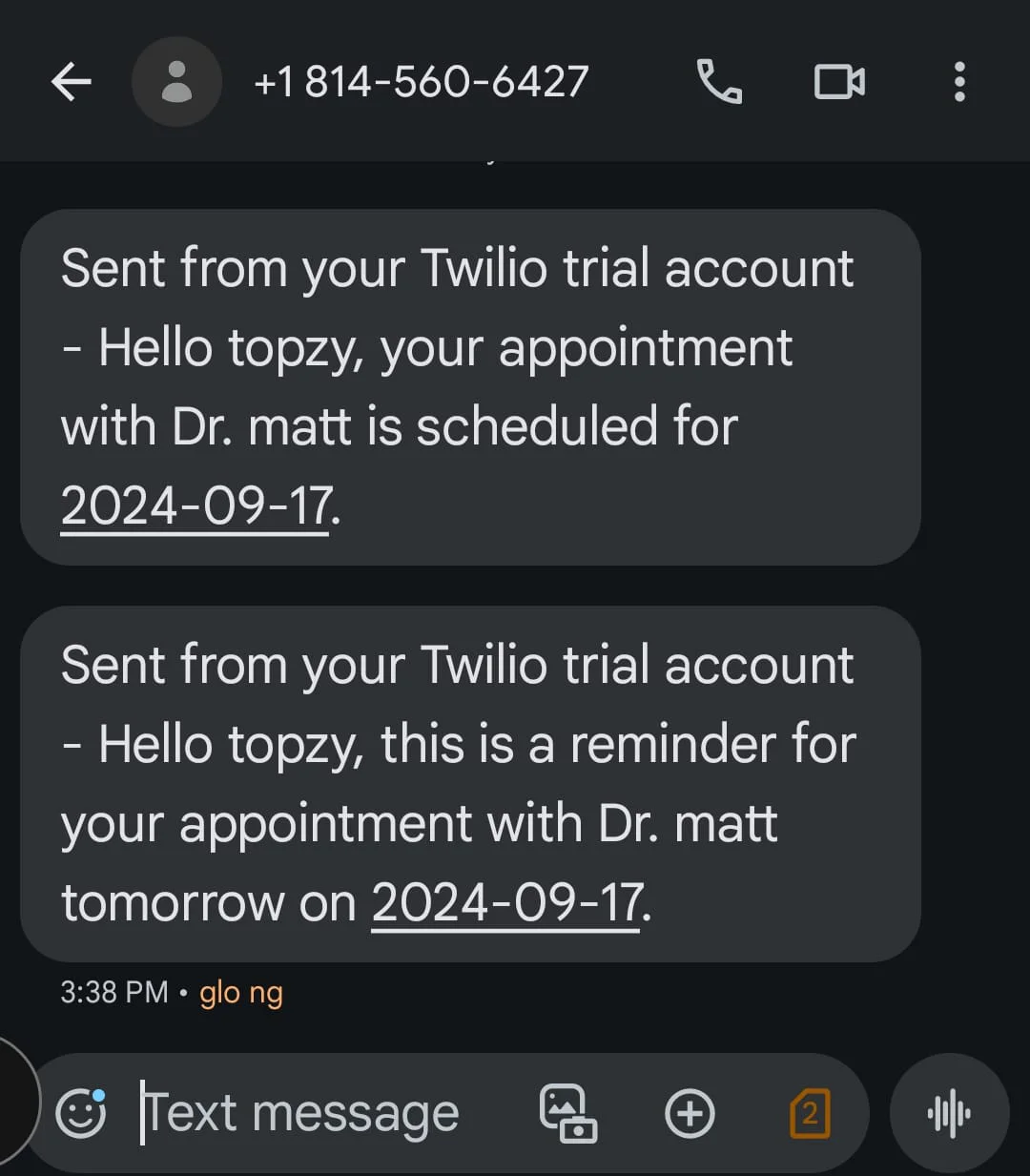
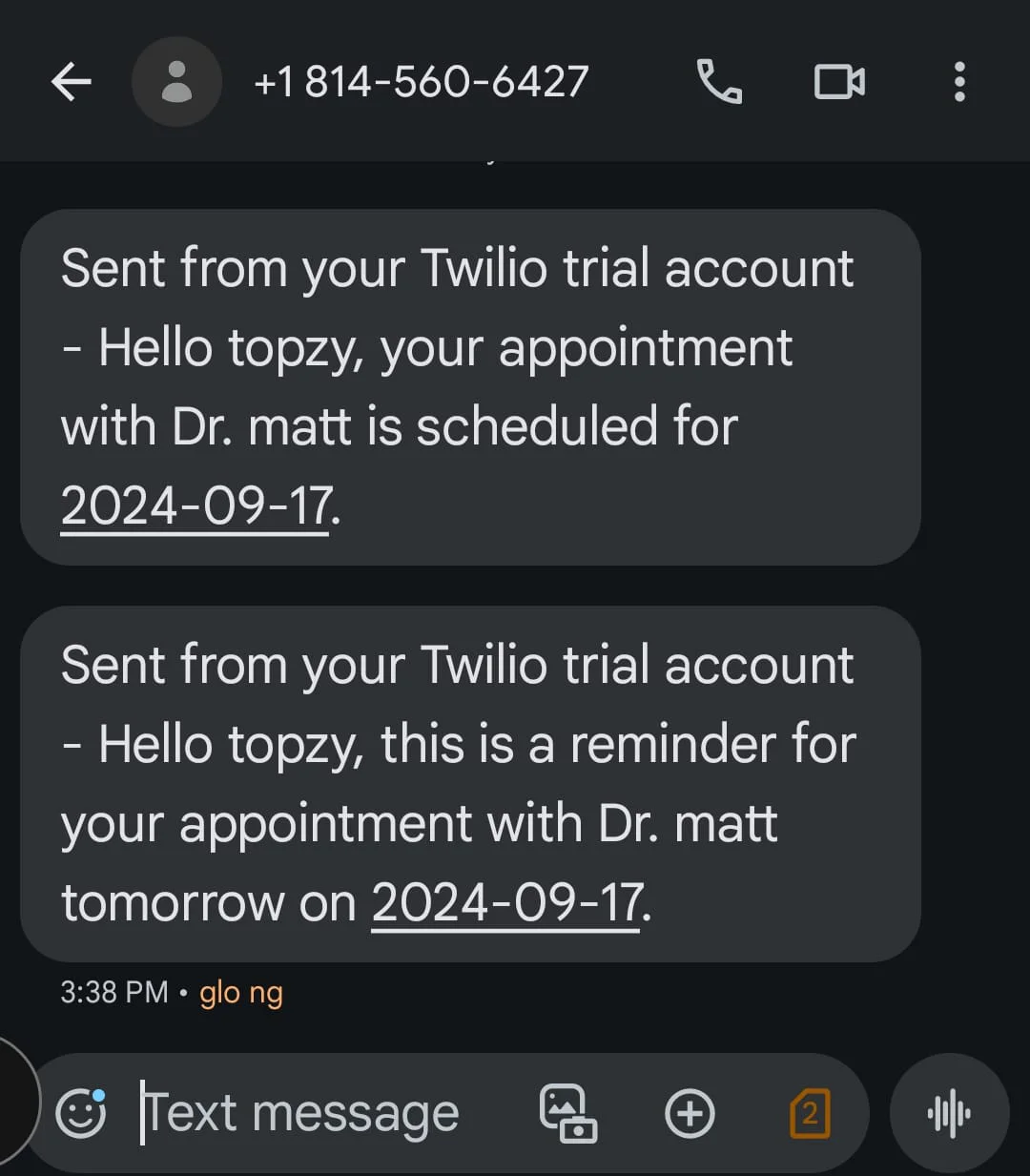
That is how to build automated appointment reminders with Go and Twilio
In this article, we've built a Go application that automatically sends appointment reminder SMS messages using Twilio. We used cron jobs to schedule reminders and connected to a MySQL database to store and retrieve appointment information.
Popoola Temitope is a mobile developer and a technical writer who loves writing about frontend technologies. He can be reached on LinkedIn.
Schedule icon in the post's main image was created by Freepik on Flaticon.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.