Automated Appointment Reminders with Twilio SendGrid and Java
Time to read: 7 minutes
Automated Appointment Reminders with Twilio SendGrid and Java
In this tutorial, you'll develop an appointment scheduling application in Java. What's special about that is that you'll leverage Twilio SendGrid's email sending capabilities to deliver customizable and dynamic appointment reminder emails to users, ensuring they don't miss their appointments.
When you are done with this tutorial, you will be able to create a Java application that can interact with the SendGrid API, regardless of the build tool you use to create it.
Prerequisites
To follow along with this tutorial, you will need the following:
- A SendGrid account (free or paid). If you do not have one already, Click here to create
- An IPinfo.io account. If you do not have one already, click here to create one
- A Java Development Kit (JDK), version 17 or higher. If you do not have one on your machine, download the Standard Edition (SE) and install before continuing.
- Your favorite IDE or text editor. In this tutorial we will use IntelliJ IDEA Community Edition. You can download the community edition if you don't have it already.
Create the Java application
Using IntelliJ IDEA, create a new Java project. Give it a name; you can call it whatever you want, but I recommend calling it "Scheduler". Then, choose "IntelliJ" for the build system. I will be using JDK 17, and advise you to do so as well. That way, you'll get the exact same results as me. But, I am sure newer versions will work just fine too.
Then, leave all the other settings as shown in the screenshot below, and click Create.
Create an environment file
The next thing you need to do is to initialize a dotenv file to store the credentials the application needs, keeping them outside of the code.
To do that, create a file named . env in the root of your project. Then, inside .env paste the code below.
The first one, API_KEY
, will store your SendGrid API key, allowing the application to make authenticated requests against SendGrid's API. The second is your IPinfo API token, allowing the application to do the same with IPinfo's API.
Add the required dependencies
Next you need to add three external packages which the code will make use of. The first is dotenv-java, which loads the variables defined in the .env file as environment variables within the application. The second is the official SendGrid Java API library, so that you can easily interact with SendGrid's API. The third is google/gson, a serialization/deserialization library for converting Java Objects to and from JSON.
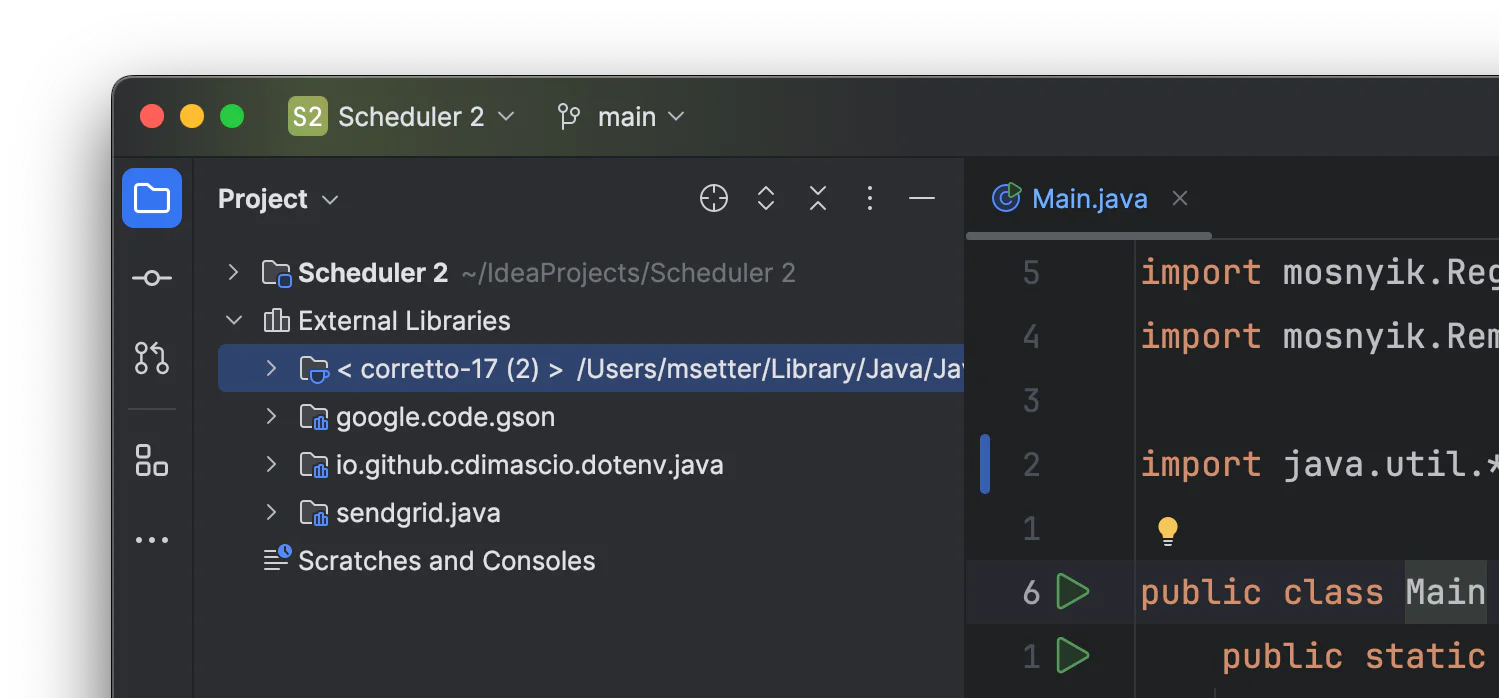
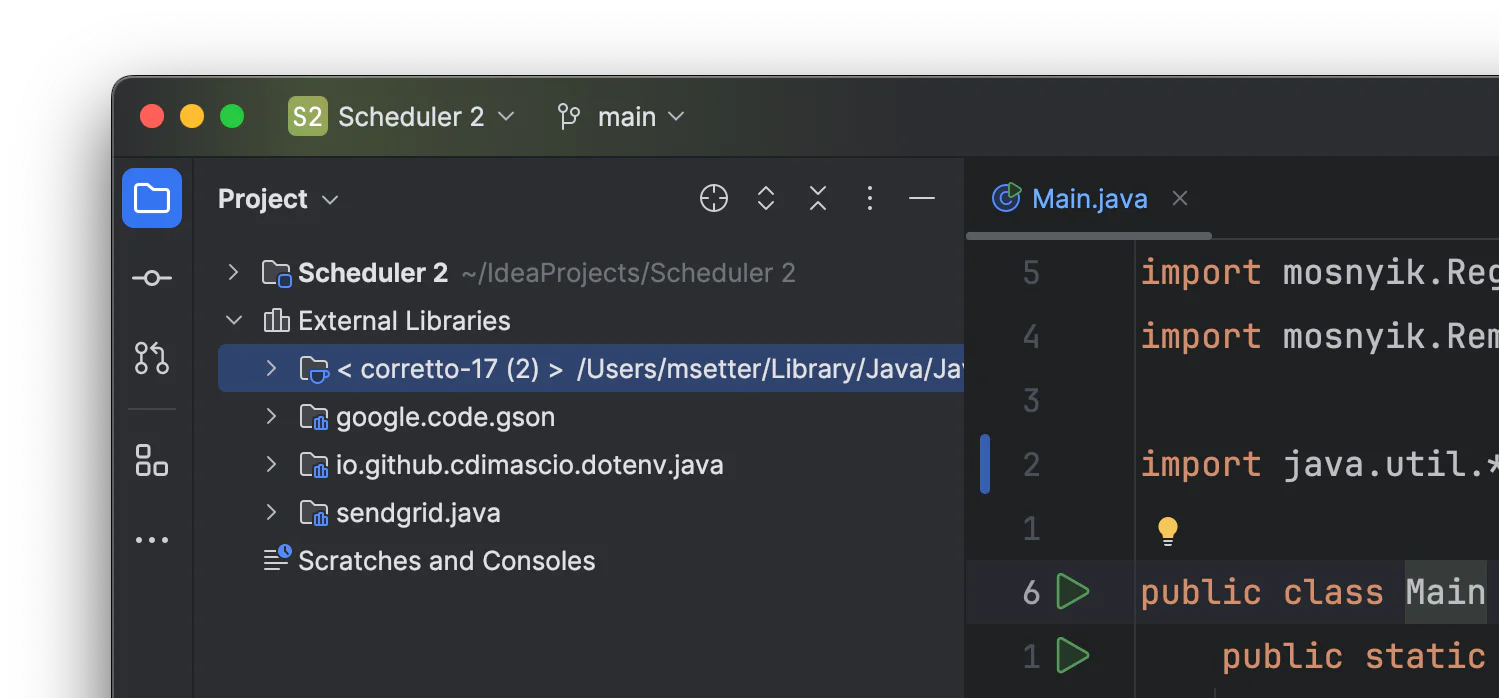
To add them, in Intellij IDEA, open the Project tool window ( CMD + 1 on macOS or Ctrl + 1 on Linux or Microsoft Windows). Then, under External Libraries right-click on the JDK and click Open Library Settings, which opens the Project Structure dialog.
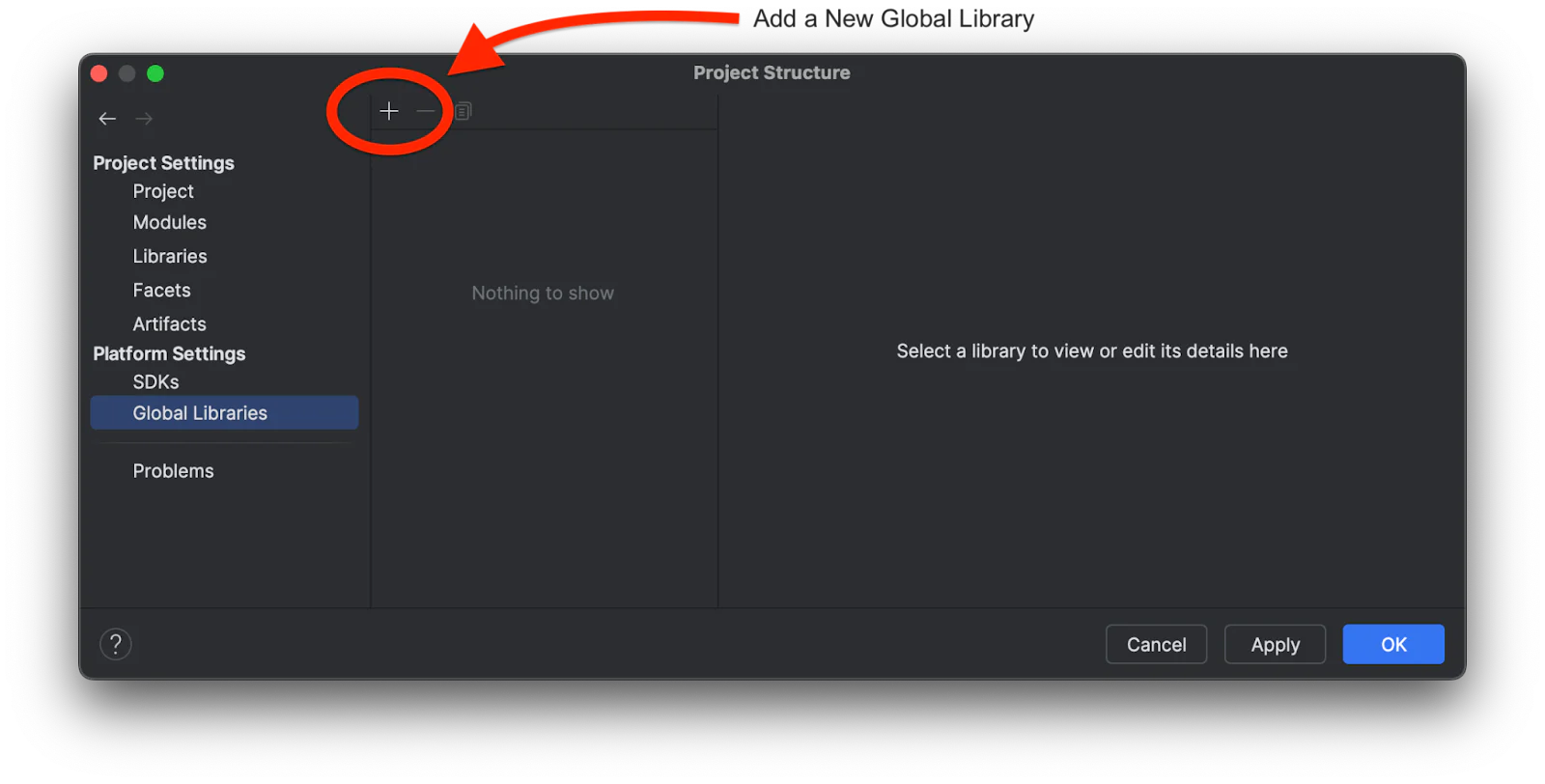
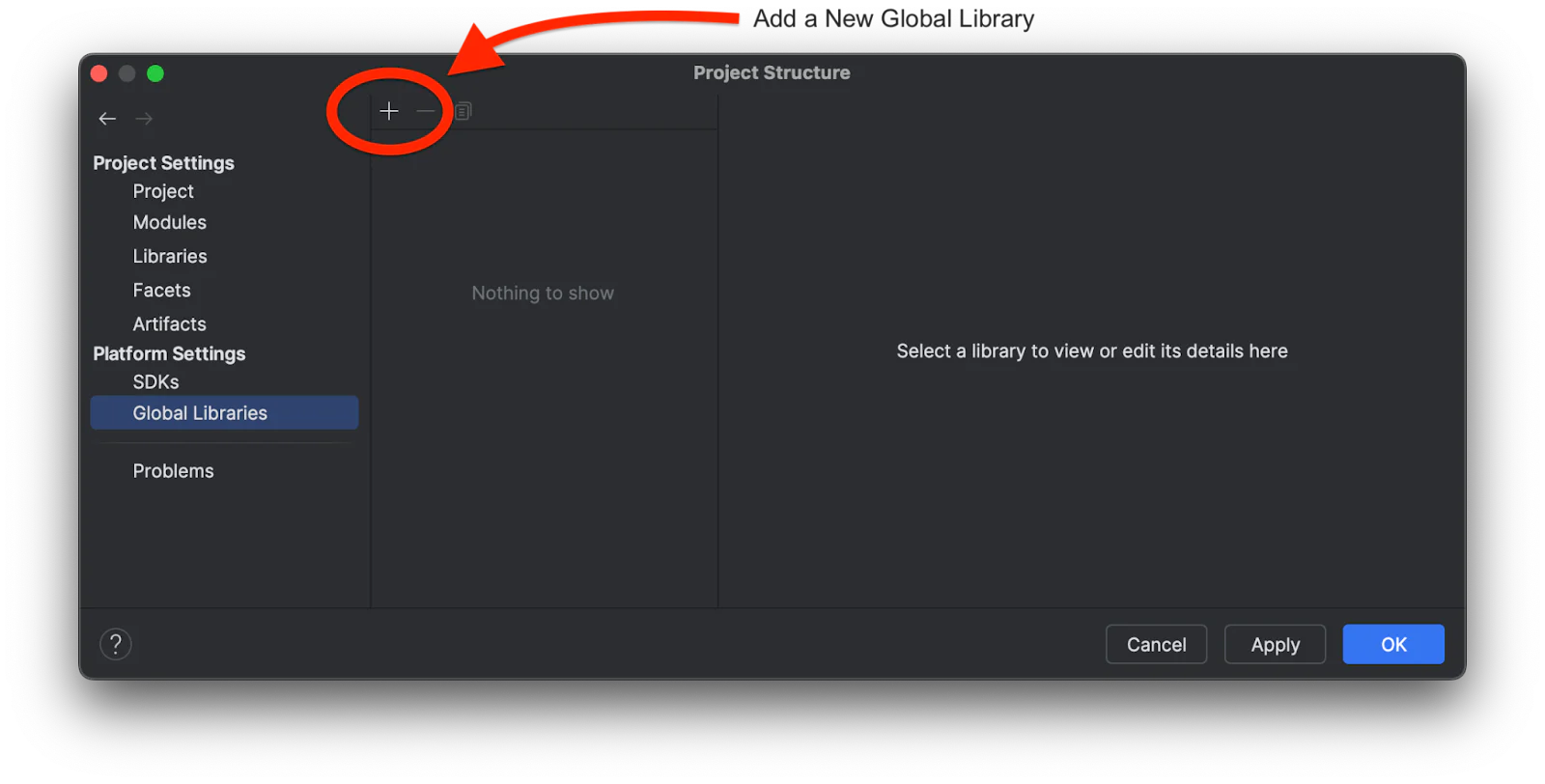
From there, in the left-hand navigation column, under Platform Settings, click Global Libraries. Then, click on the + icon at the top of the middle column (alternatively, click CMD + N on macOS, or Ctrl + N on Linux or Microsoft Windows) to add a new global library. In the popup that appears, click From Maven….
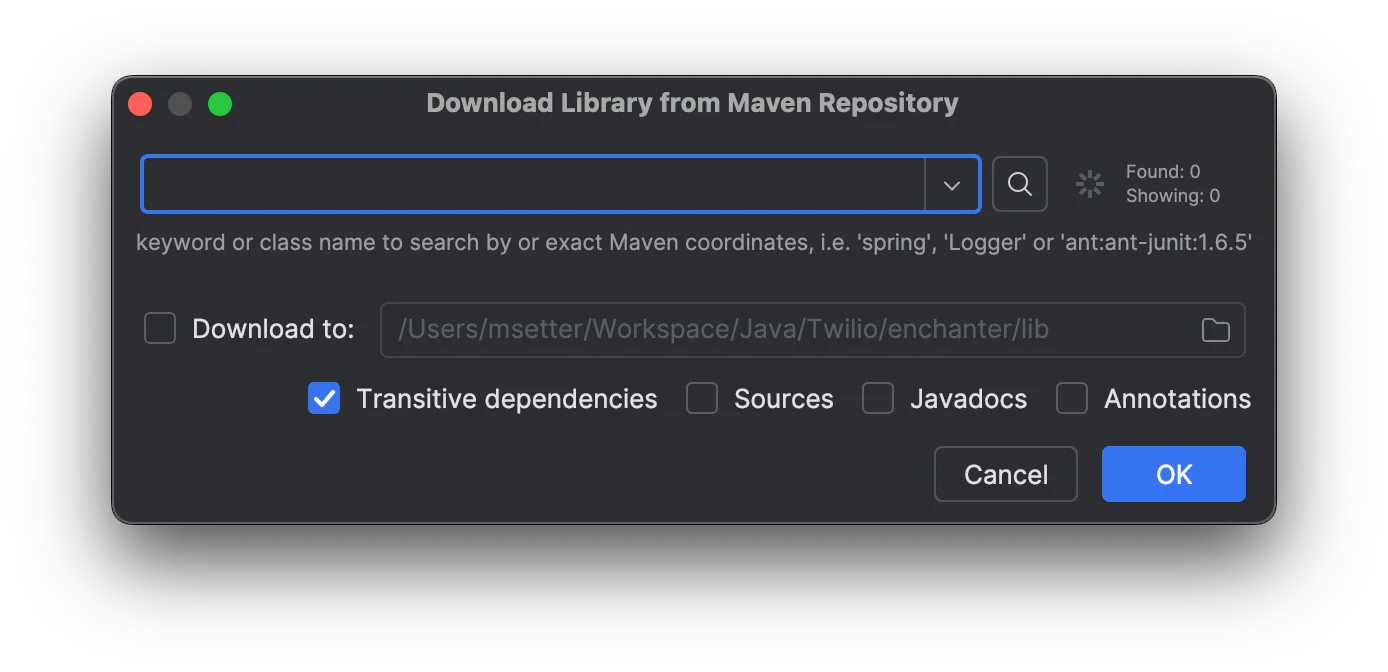
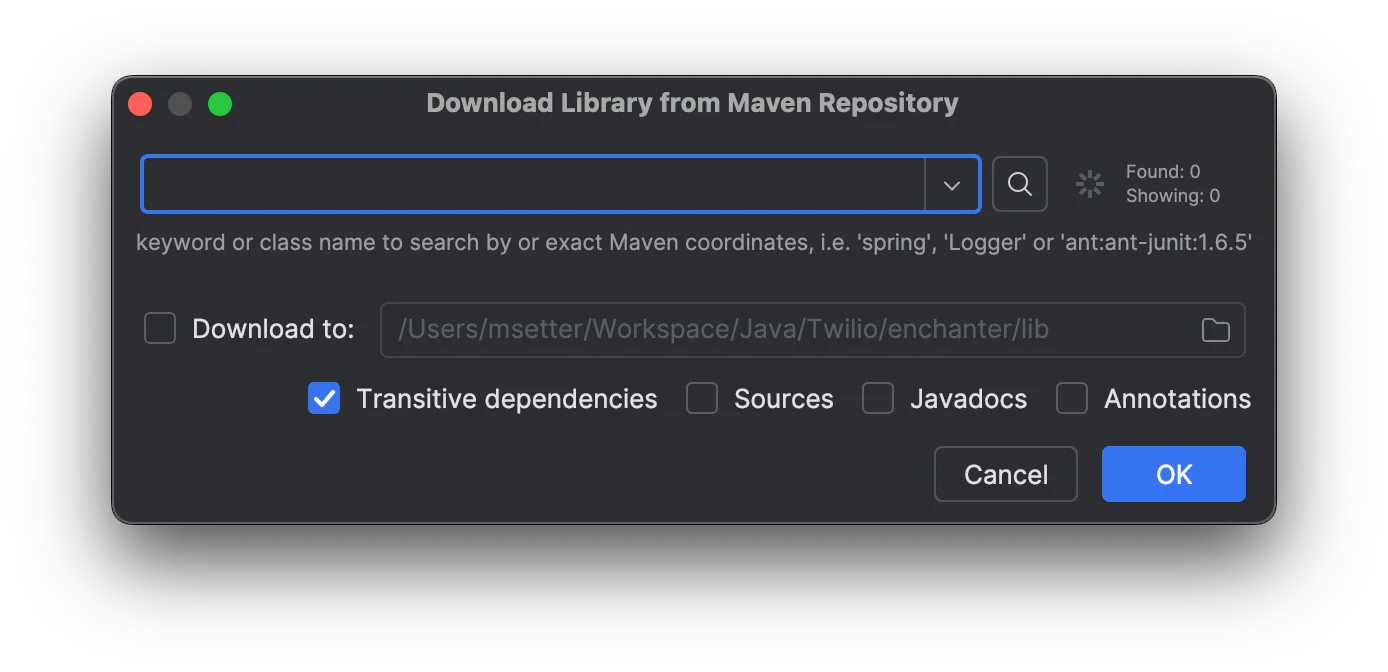
Now, in the search bar of the "Download Library from Maven Repository" window, first, search for "dotenv". You will see quite a long list of search results. The one you need is io.github.cdimascio.dotenv-java:3.0.0
. Click it and click OK in the Choose Modules window.
Next, search for "SendGrid". From the search results, choose com.sendgrid:sendgrid-java:4.10.1.
After that, click OK in the Choose Modules window.
Then, search for "google.code.gson". From the search results, choose com.google.code.gson:gson:2.11.0. After that, click OK in the Choose Modules window.
With the dependencies installed, click OK in the Project Structure window and you're done.
Create a custom Java package
In the src directory, create a package and call it mosnyik. This is where you will add all your classes.
The first class you'll create is the SendMail
class. This is the class that will do the work of sending out dynamic emails depending on trigger events like user registration, logins, or when appointments are due.
Create a new Java class in src/mosnyik called SendMail
. Then, update the file to match the following code.
With that done, replace <ENTER-YOUR-SENDER-EMAIL-HERE>
with the authenticated email from the Single Sender Verification email addressyou set up earlier.
Now, you have created a class to send mail to users when they register, when they log in, when they book an appointment, and when they need a reminder.
Next, you need to create the RegisterUser
class. To do that, in src/mosnyik create a Java class and name it RegisterUser
. Then, copy and paste the code below into the class.
Great, now you can now register a user and send them a welcome mail. So, up next, we'll work on the login()
method. Add the code in the code block below after the addUser()
user method.
The login()
method requests the user to provide their registered email address and password. Once provided, we enter into a while loop to check the stored registered users against the email and password pair. If there is a match, the user gets redirected to the "Schedule Appointment" page, otherwise they will see the message “Invalid email or password. Please try again”.
With that done, your user can now login and be redirected to the "Schedule Appointment" page. That is the next method you will create in src/mosnyik/RegisterUser.java, by pasting the function below at the end of the class.
With the above code, you can now add a new appointment, and receive a confirmation email sent to the user by email.
Next, you will be building the listAppointments()
method. This code block should be added after the scheduleAppointment()
methodinside the RegisterUser
class.
Next, you need to create a ReminderService
class that checks for reminders on a periodic basis. So, in src/mosnyik, create a new class named ReminderService
. Then, update the class' code to match the following.
At this point, you have built almost the entire app, but in bits and pieces. So let's now tie those pieces together. In your Main
class, add the following code.
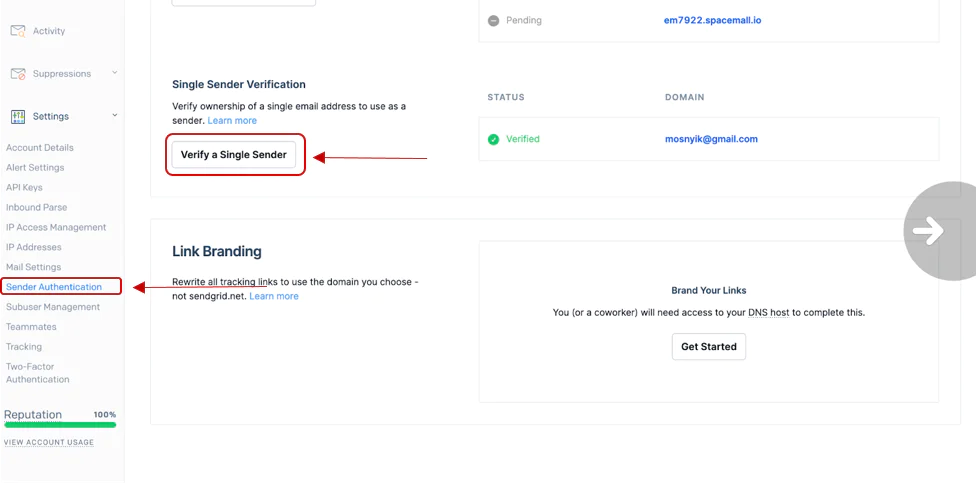
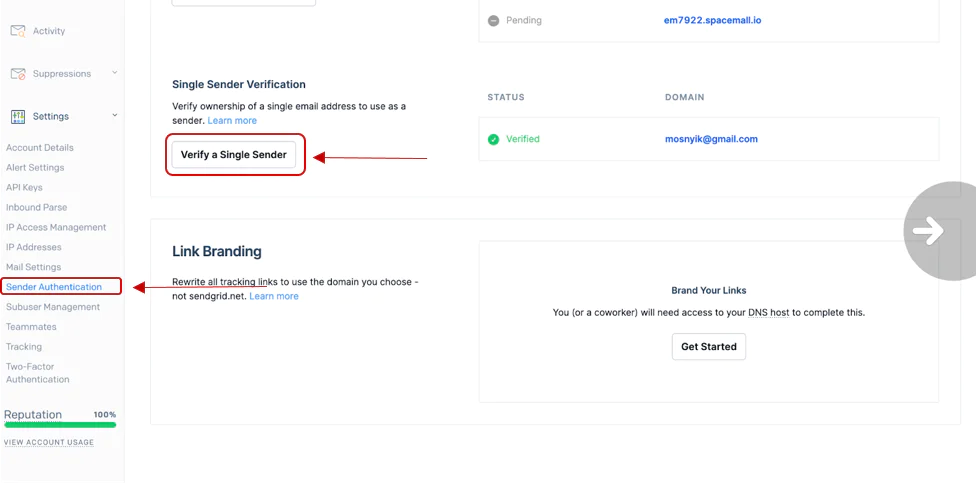
To send emails using the SendGrid API, you need to authenticate your sender email address. There are two ways to authenticate your mail: Domain Authentication and Single Sender Verification. We'll be using the latter in this tutorial.
To set it up for your account, login to your SendGrid dashboard, then open Sender Authentication. There, under Settings, click on the Single Sender Verification button. It will open a form for you to fill in the appropriate data for verifying your email address. When you are done, click on the create button.
You will get an email from SendGrid to verify the email address. Once you verify your email address, you are done. You can now send mails from that email address. You can read more in the Sender Authentication documentation.
Retrieve the required API keys
Generated a SendGrid API key
The first thing you need to do is create a SendGrid API key, so that the application can securely interact with SendGrid's API. To do that, in your SendGrid dashboard, click on Settings, then click on API keys.
There, click on Create API Key. Then, enter the project name at the top where you see the label name, e.g., "Tutorial Key", as seen in the image below. After that, click on Restricted Access, and then go down to Send Mail.
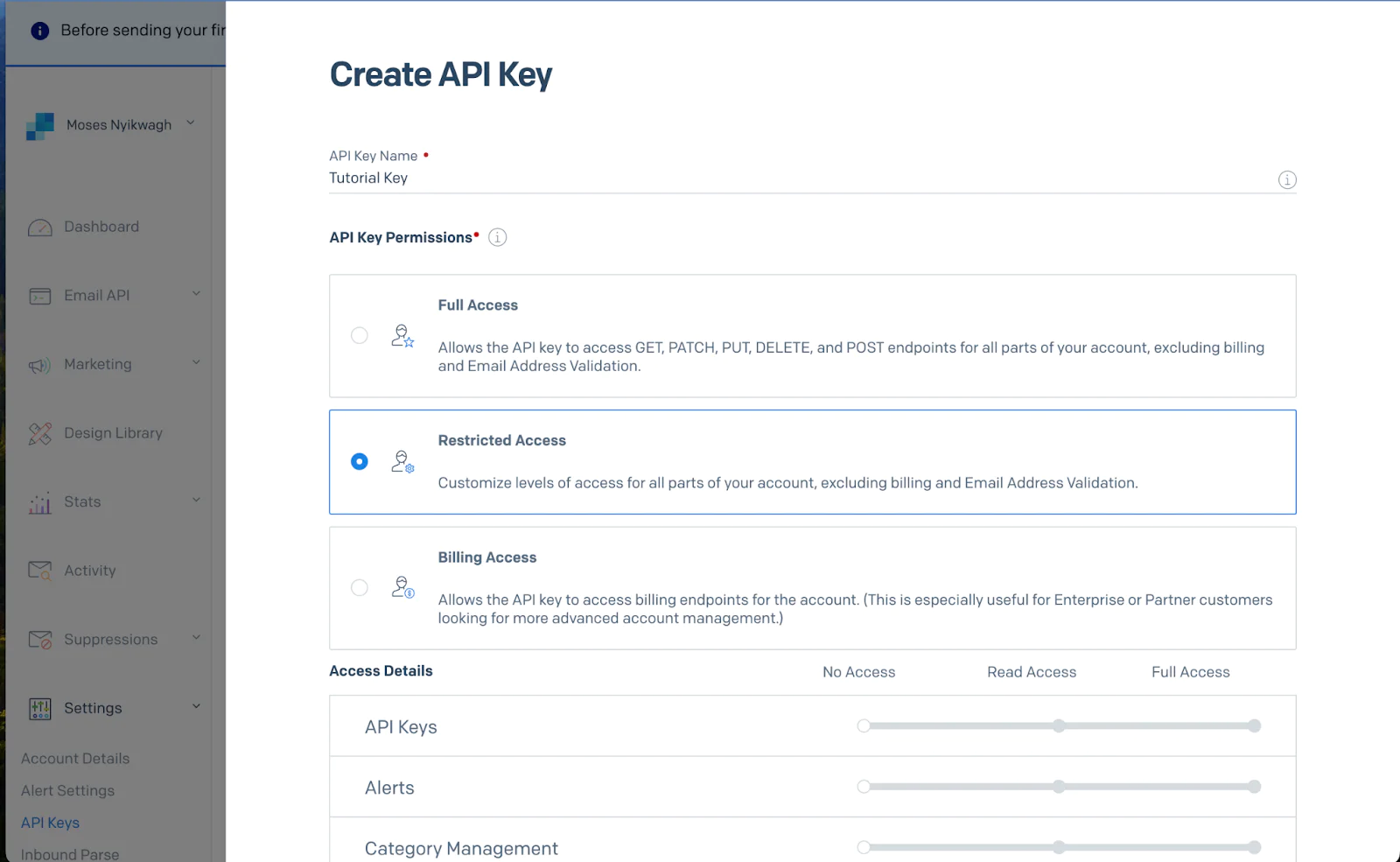
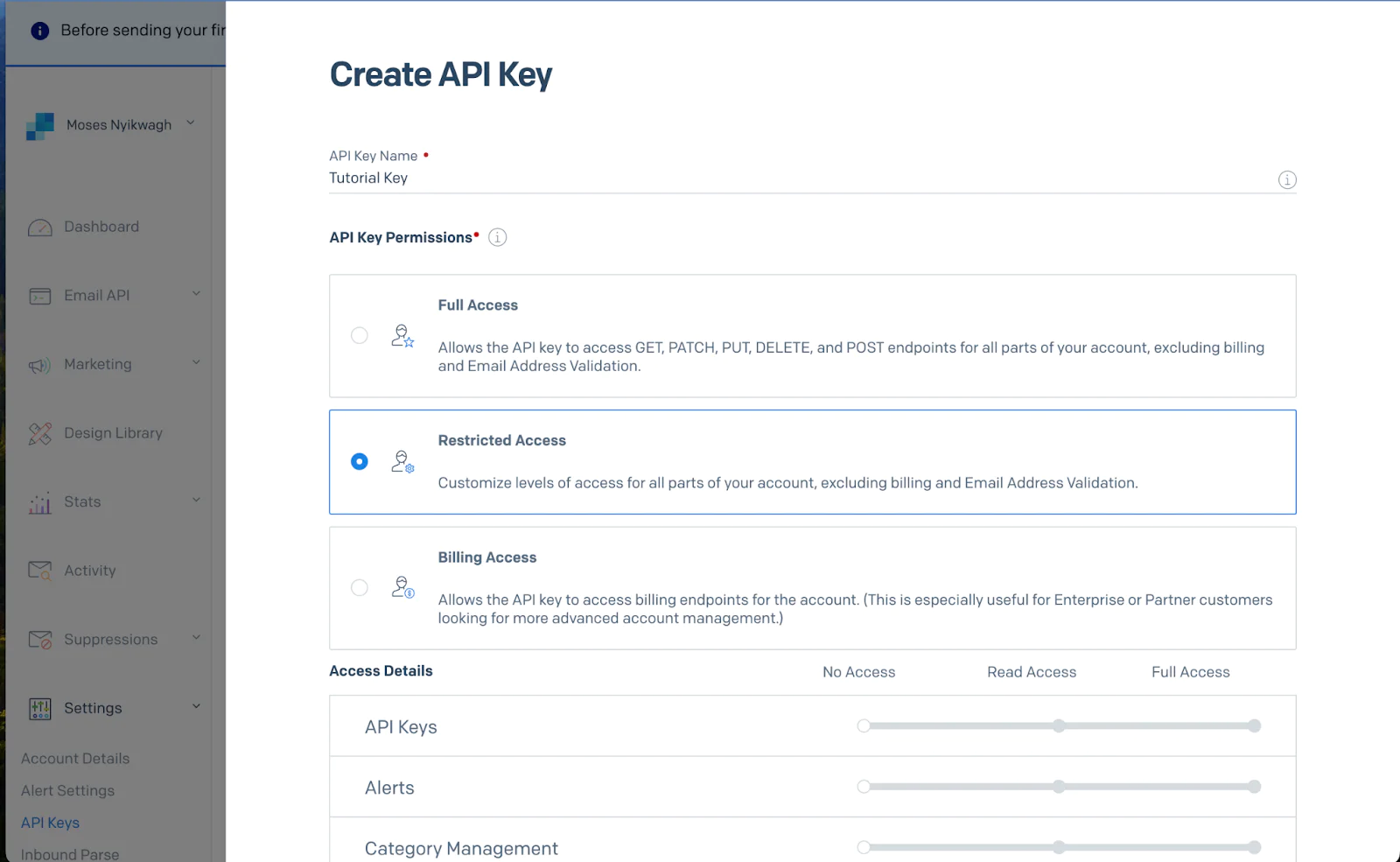
Scroll down to Mail Send and click it, then check the Mail Send option.
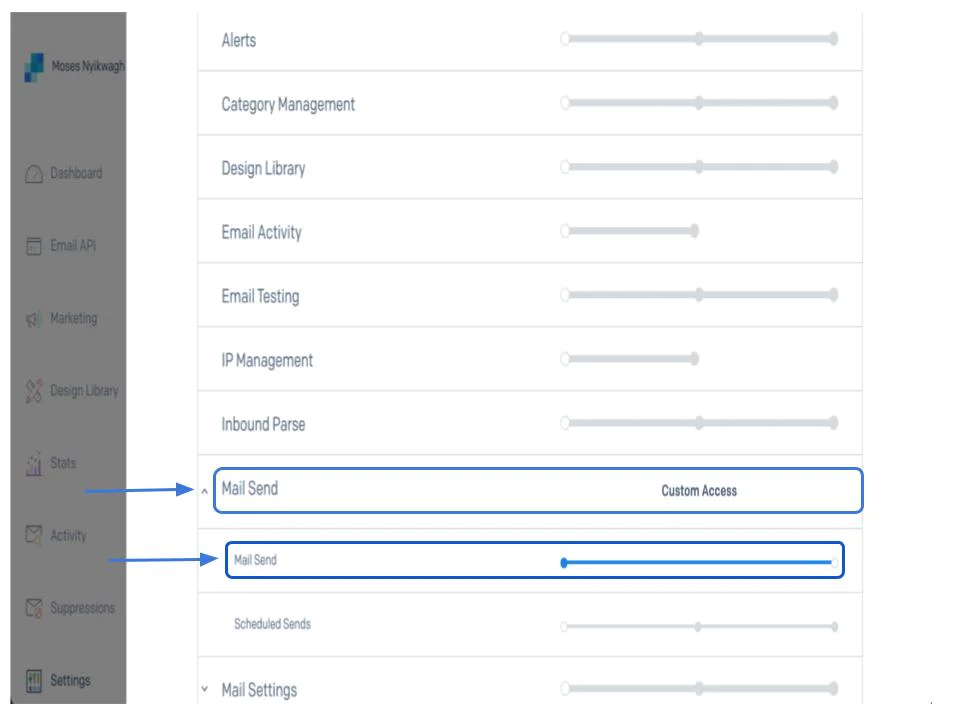
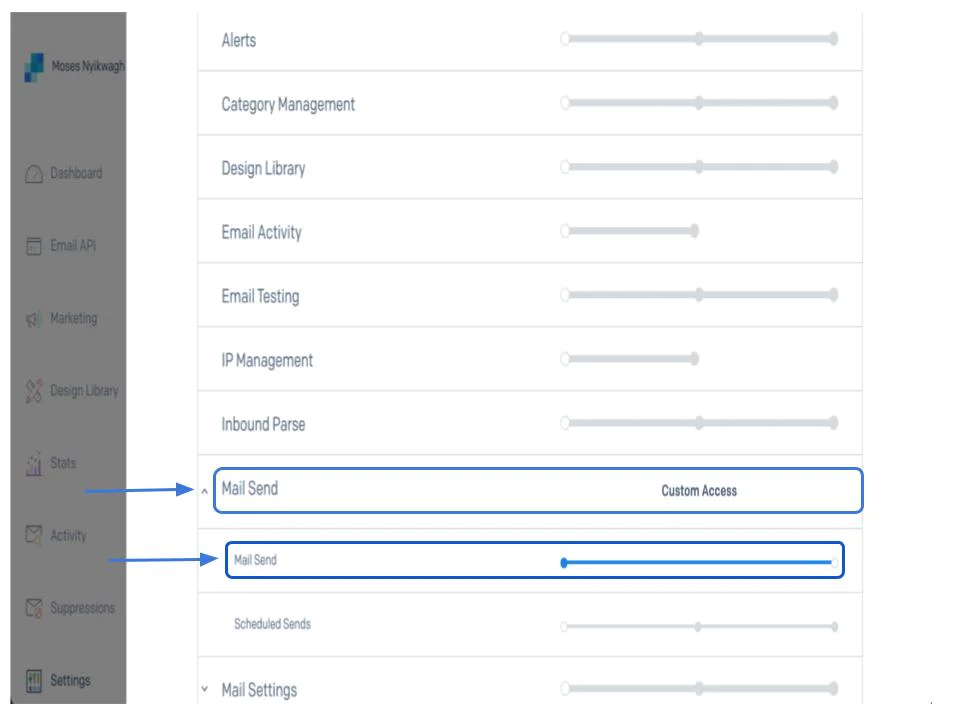
Now, go to the bottom of the screen and click on Create & View to generate the API key. Now, copy and paste it into .env in place of <<YOUR_SENDGRID_API_KEY>>
.
Retrieve your IPinfo API key
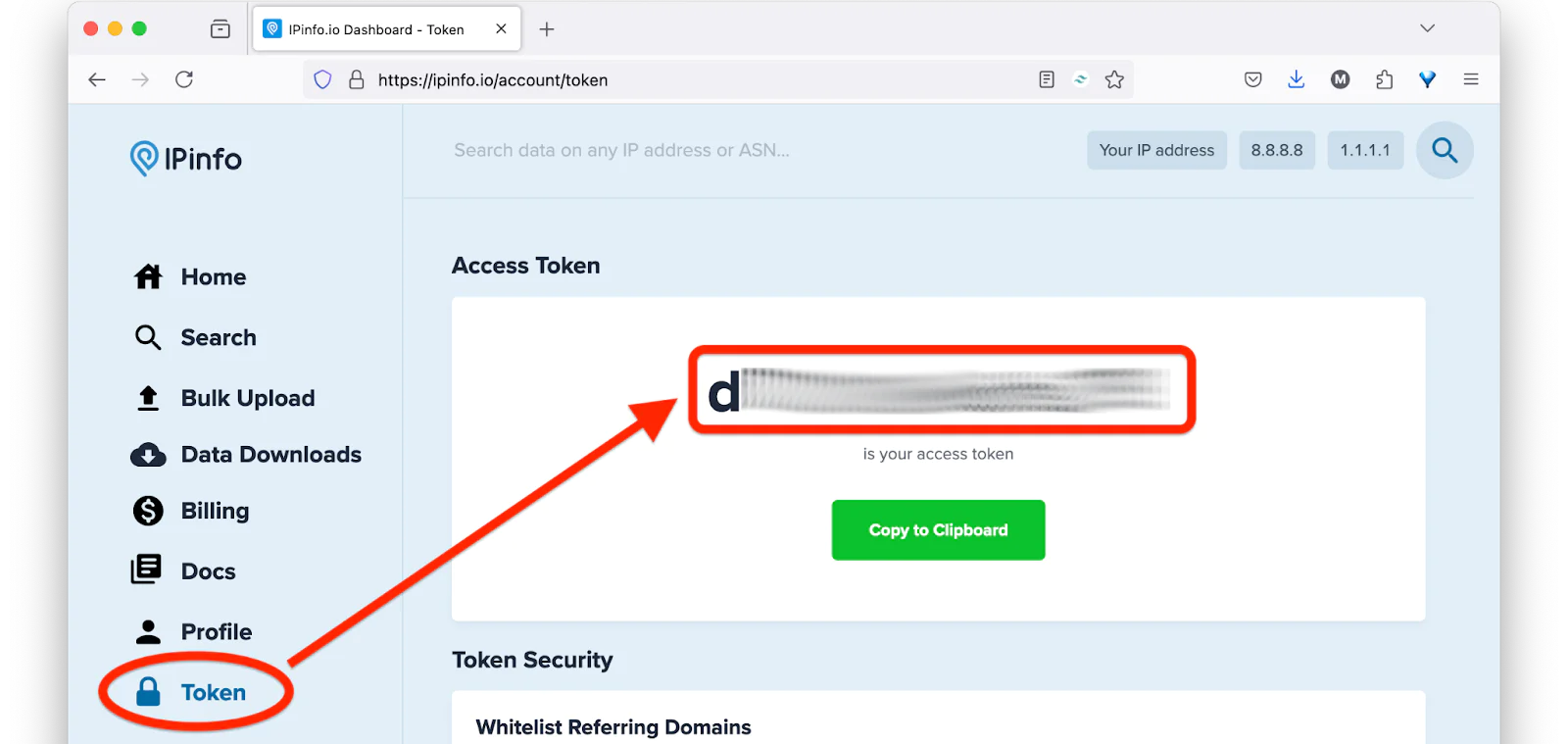
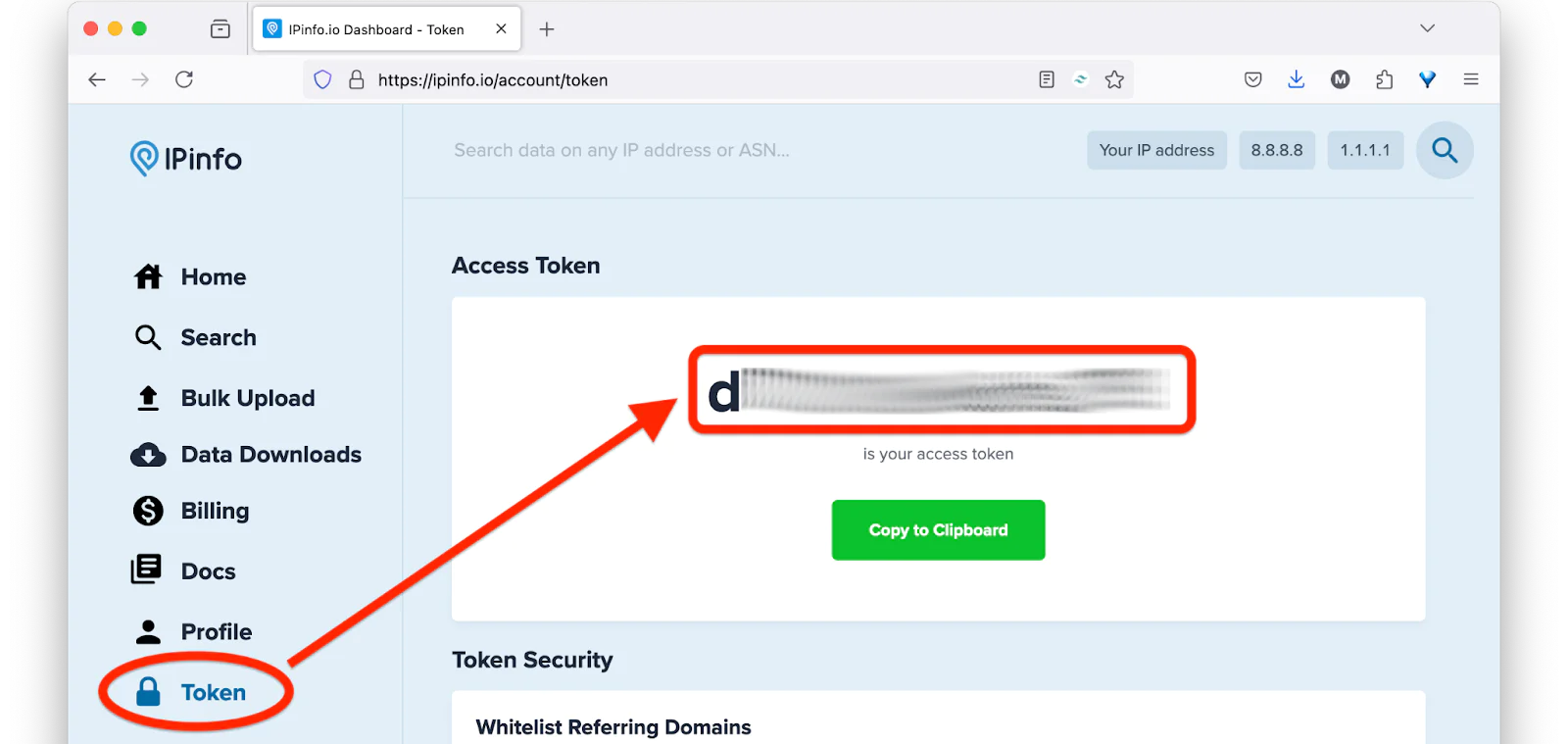
The app needs access to a geolocation API, so head over to ipinfo.io and login to your account. On the dashboard, click the Token tab, then copy your Access Token and paste it into .env in place of <YOUR-IPINFO-API-TOKEN>
.
Test that the app works
Now, you can test out your application and register a user. Run the application and select "1" to register your user.
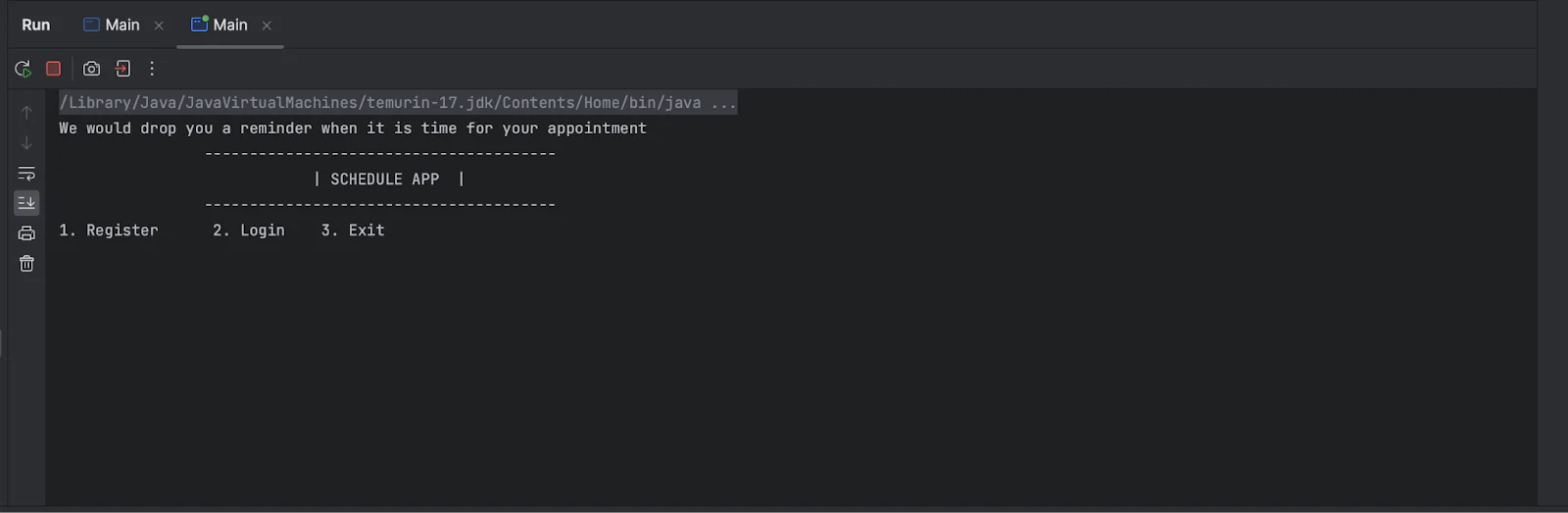
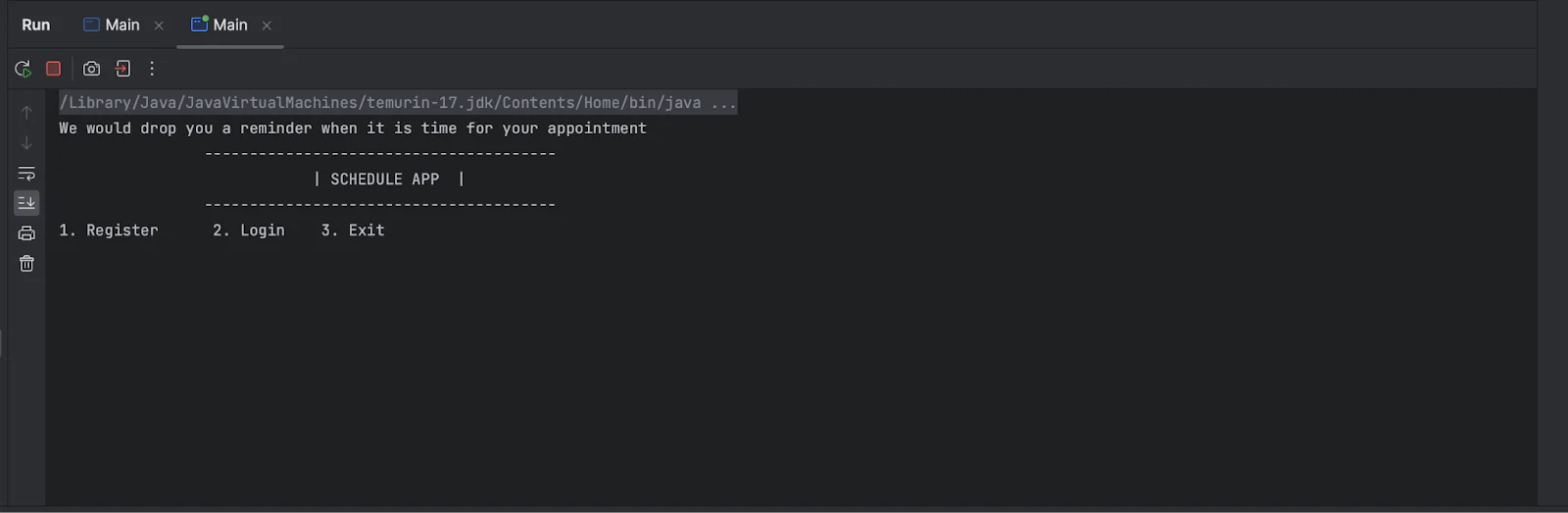
Enter the required details to create a user. When you have created a user successfully, you will be redirected to login with the details you just used to create the user in the screen like you see below.


When you enter the login details, you will be redirected to schedule an appointment as seen below.
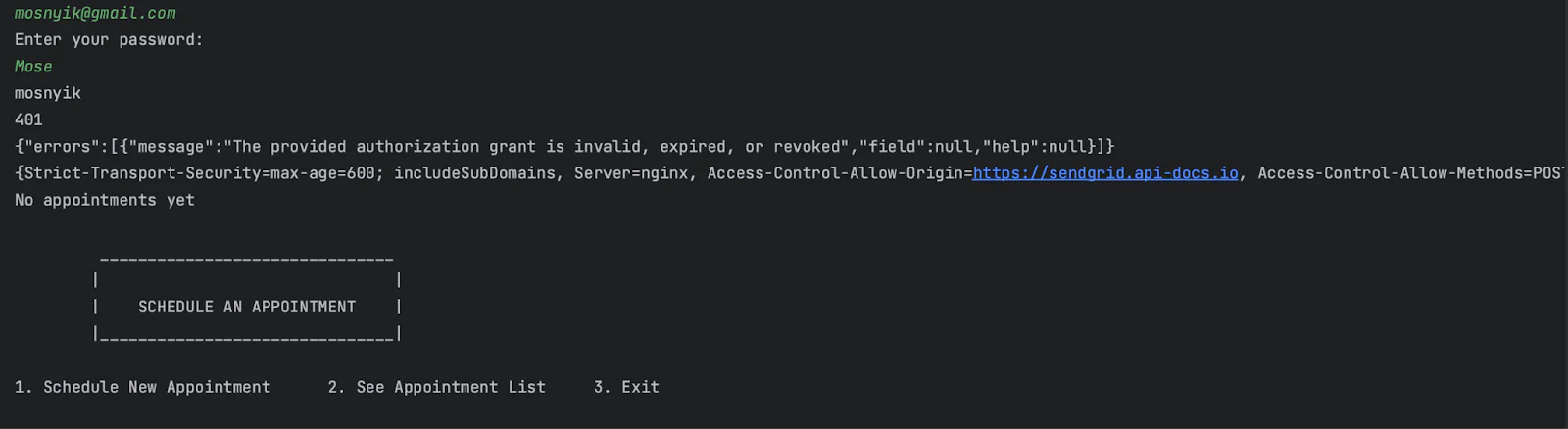
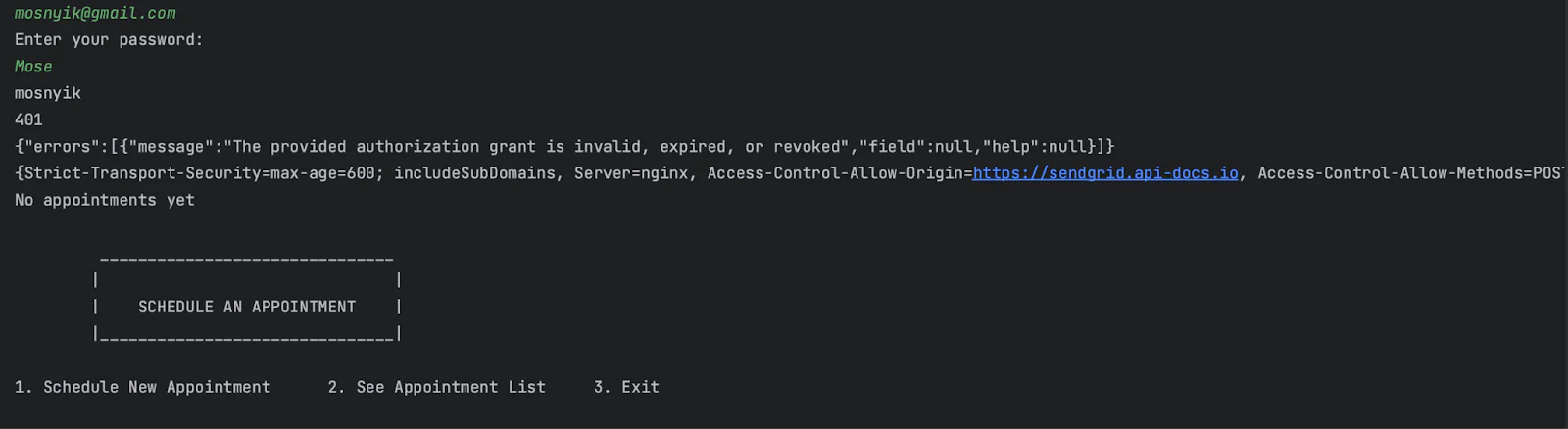
From here you can schedule appointments. When you select "1" to schedule an appointment, you get the prompt to enter the appointment category and then the appointment name, once you are done, it returns you to this screen, and you can now check the list of appointments you have by selecting "2", check image below.
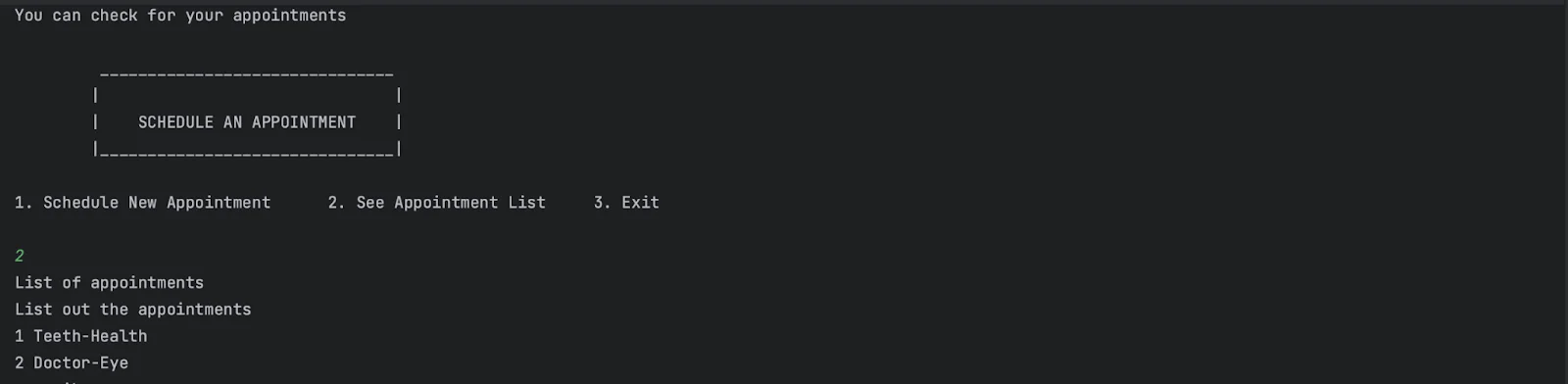
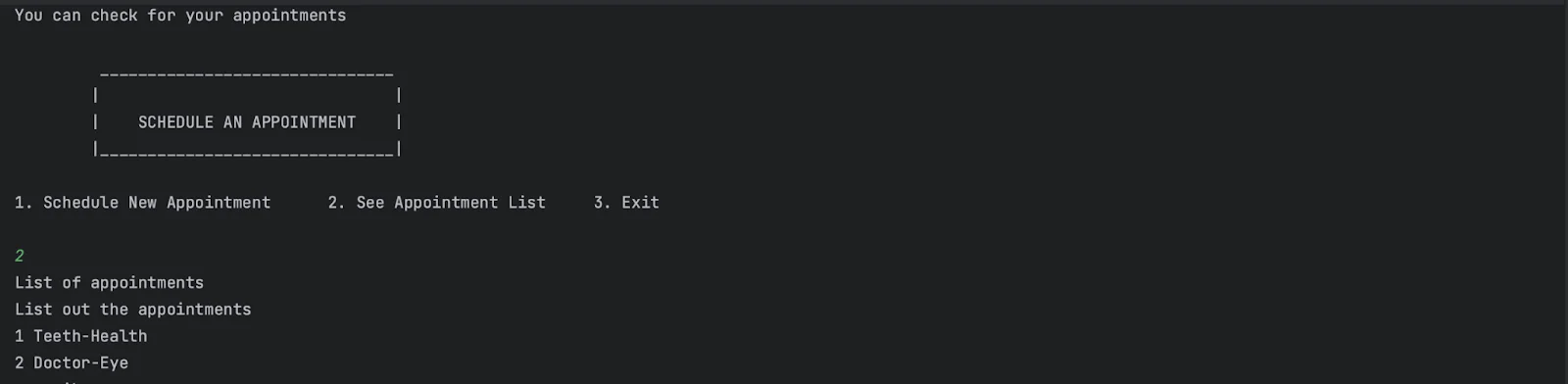
This is the GitHub link of the complete app, should you want to compare your code and straighten any errors you may have along the way.
That's how to automate appointment reminders with Twilio SendGrid and Java
Twilio gives an easy to use way to interage mailing to your application ranging up to dynamic mailing for your marketing. It is even better now with the verification. The steps and very easy and the integration, very light weight.
Nyikwagh Moses is a Blockchain Full Stack Developer in Abuja, Nigeria. He is very enthusiastic about anything technology, especially Blockchain Technology. He loves to make like minded friends and would love to connect, you can find him on LinkedIn and X (formerly known as Twitter), if you have a question or you also want to connect.
Java logo in the tutorial's main image was created by Mark Anderson (work for hire for Sun Microsystems) - http://www.logoeps.com/java-eps-vector-logo/40925/, Fair use, https://en.wikipedia.org/w/index.php?curid=51298819 and the appointment icon was created by Vectors Tank on Flaticon.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.