How to Build an Email Monitoring Application with SMS and Semantic Analysis
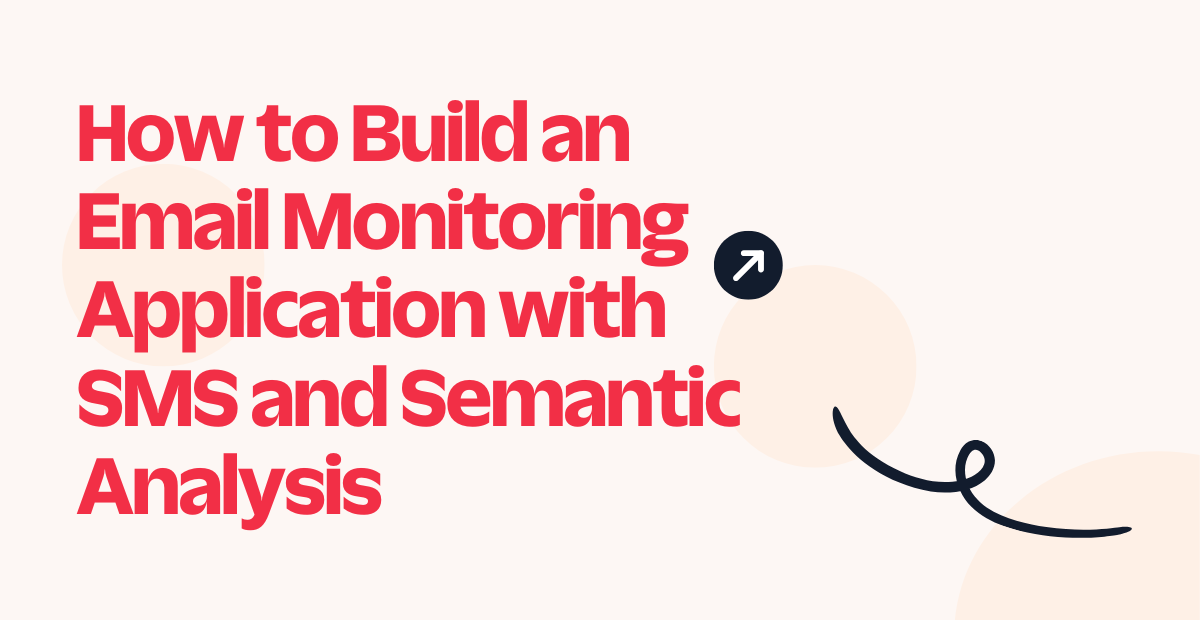
Time to read: 6 minutes
Managing your inbox can be overwhelming. What if you could have an intelligent assistant that reads your emails, determines their importance based on the ones that really matter to you, and texts you about them?
In this tutorial, you'll learn how to build a Python application that does just that, using Twilio SMS and semantic analysis with sentence transformers. Haven’t heard of semantic analysis? Semantic analysis is the process of understanding the meaning and context of words and sentences in natural language.
This smart email monitoring will:
- Retrieve unread emails from your inbox
- Use semantic similarity to analyze and classify each email's importance according to your use case
- Send SMS notifications about important emails that match specific keywords
- Provide detailed console output for monitoring
By the end of this tutorial, you won’t have to stress to stay on top of your most crucial communications without being tied to your inbox.
Prerequisites
Before proceeding, make sure you have the following:
- A free Twilio account. If you don't have one, sign up here.
- Python 3.7 or later installed on your computer.
- Basic knowledge of Python programming.
- An email account that supports IMAP access. Most major email providers (Gmail, Outlook, Yahoo) support this.
Set Up Your Development Environment
You can start by setting up a clean development environment. Open your terminal and run the following commands:
This creates a new directory for your project and sets up a virtual environment to manage your dependencies.
Install Required Packages
Now, install the necessary Python packages:
Here's what each package does:
twilio
: Twilio's Python SDK for making voice callssentence-transformers
: For semantic text similarity analysispython-dotenv
: For loading environment variables from a .env filecolorama
: For adding color to console outputchardet
: For detecting character encoding in emailsscikit-learn
: or computing cosine similarity
Configure Your Environment Variables
To keep your sensitive information secure, you'll use environment variables. Create a file named .env in your project directory with the following content:
Replace the placeholder values with your actual credentials. Here's how to get each:
EMAIL
: Your email addressPASSWORD
: Your email app password (see section below on how to get this for Gmail)IMAP_SERVER
: The IMAP server for your email provider (e.g.,imap.gmail.com
for Gmail)account_sid
andauth_token
: Find these in your Twilio Consoletwilio_phone_number
: Your Twilio phone number with SMS enabledto_phone_number
: The phone number associated with the Twilio account you created, also in the E.164 format e.g+2348160435459
Get an App Password for Gmail
If you're using Gmail, you'll need to generate an app password instead of using your regular account password. This is necessary for securely logging into your Gmail account via IMAP and authenticating the connection. Gmail requires an app password when two-factor authentication (2FA) is enabled. Here's how:
- Go to your Google Account.
- Select "Security" from the left navigation panel.
- Under "How you sign in to Google," select "2-Step Verification" and verify your identity. The process won’t work without 2 step verification activated.
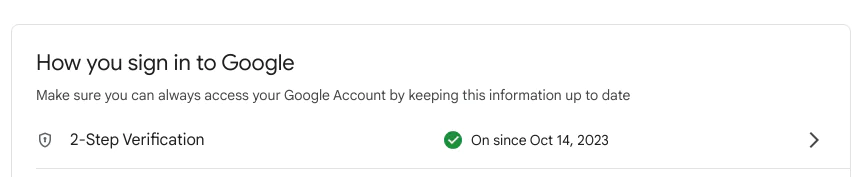
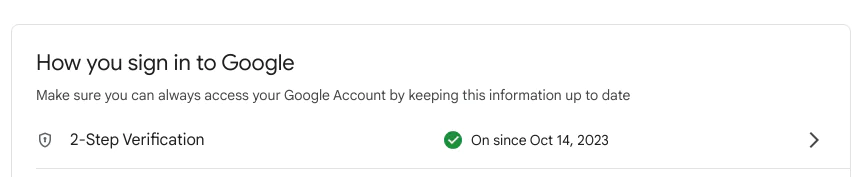
- Go to App passwords, you may need to sign in.
- Enter a name for the app (e.g., "Email-analyzer") and click "Create".
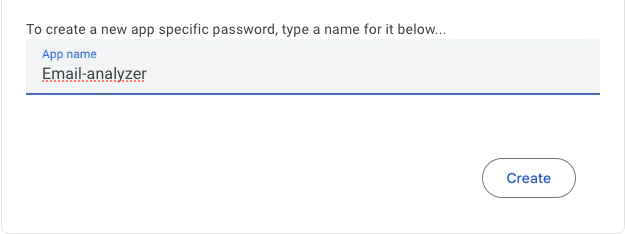
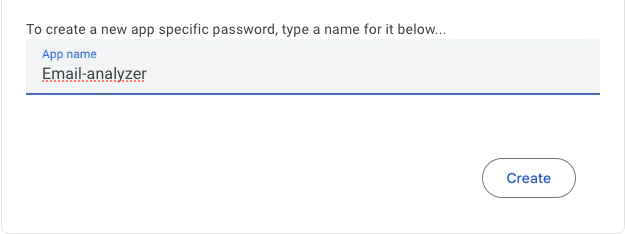
- Google will display a 16-character app password. Copy this password.
- Use this app password in your
.env
file for thePASSWORD
field.
Build the Email Analyzer
The main components of the email monitoring application will be implemented in a file named email_monitor.py. This is where you will add the core functionality to retrieve emails, analyze their importance according to your set keywords, and send an SMS.
To start, create a new file in your project directory with the name email_monitor.py. This is where you will write the code for the email monitoring application. To begin, copy the code snippet below and paste it in your email_monitor.py.
The above code does the following:
- Imports our libraries for email fetching, SMS sending, text analysis, and environment variable management.
- Loads sensitive information like email credentials and Twilio API keys from the .env file.
- Loads a pre-trained NLP model (
'all-MiniLM-L6-v2'
) for generating sentence embeddings to compare and analyze text semantically.
Decode the emails
Implement the functions below for the email decoding functionality. Copy and paste the following code below:
The safe_decode
function is crucial for handling email content because:
- Emails can come from various sources with different character encodings
- Some emails might use non-standard or legacy encodings
- The function tries multiple common encodings to ensure readability
- It uses
chardet
to automatically detect the most likely encoding - If all decoding attempts fail, it returns a string representation as a fallback
Retrieve Unread Emails
You will now implement the email retrieval function. Copy and paste the following code below:
This get_emails
function performs several important tasks:
- Connects securely to your email server using SSL
- The
SENTSINCE 18-Nov-2024
filter ensures we only process unseen emails from 18th of August, 2024 (adjust this date as needed according to your use case) - Handles both single-part and multi-part email messages
- Extracts the sender, subject, and body text
- Use the
safe_decode
function to handle various character encodings
Analyze emails with semantic similarity analysis
Next, you will implement the core functionality, where the magic happens—analyzing emails using semantic analysis. Copy and paste the code below:
Semantic similarity analysis uses the sentence transformer model to convert text into numerical vectors.
The default similarity threshold of 0.7 was chosen for more concise and targeted emails. It can catch semantic variations (e.g., "Position available" might match "Job opportunity"). It catches words that are similar in meaning to your set keywords, which will be later explained in the article.
The function returns both matched keywords and the relevant sentences for context.
Implement the Twilio SMS notifications
Let's add the SMS notification functionality. Copy and paste the code below:
The code initializes a Twilio client with your account credentials and sends an SMS using Twilio's messages.create()
method.
Let's implement the main function that ties everything together. Copy and paste the code below:
The main()
function:
- Defines a list of keywords that indicate important emails you need to be notified about in your inbox, in this case it is targeted at job opportunities
- These keywords were chosen to cover situations for seeking new job opportunities (Adjust the keywords as needed)
- Provides color-coded console output for better visibility
- Sends concise SMS notifications for important emails
- Includes error handling for termination
Run and test the application
You can get the code from this GitHub repository.
The script will start running and check for new emails from your set date. To test it:
Send yourself these sample emails:
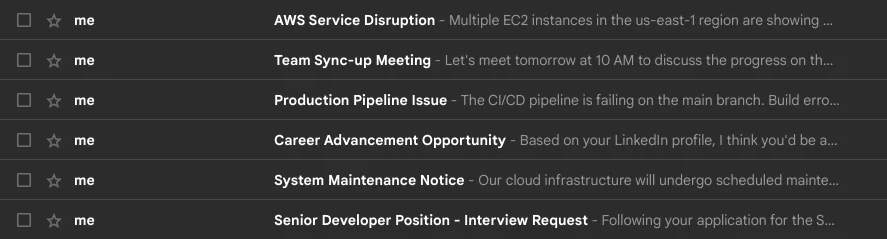
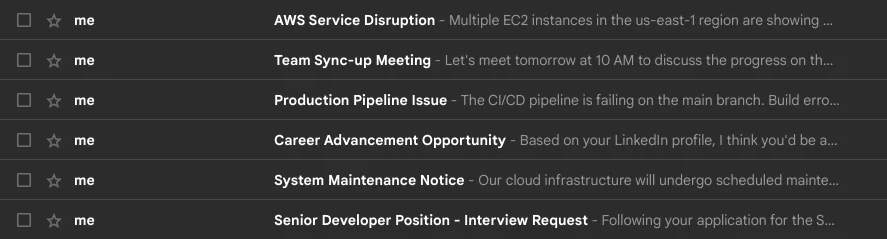
To run your email monitor, make sure all your credentials are correctly set in the .env file, then execute:


Your application will take time to download the 'all-MiniLM-L6-v2'
model.
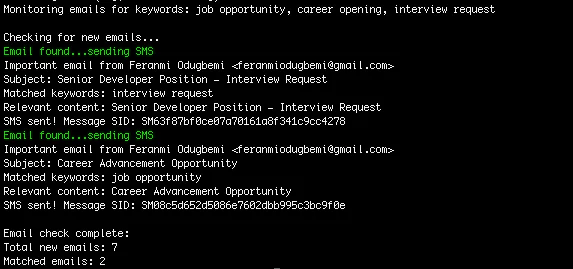
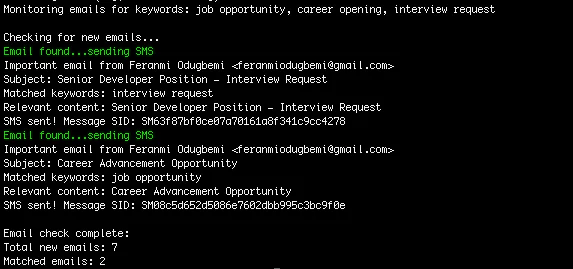
Your application has successfully gone through the emails and sent an SMS as seen below:
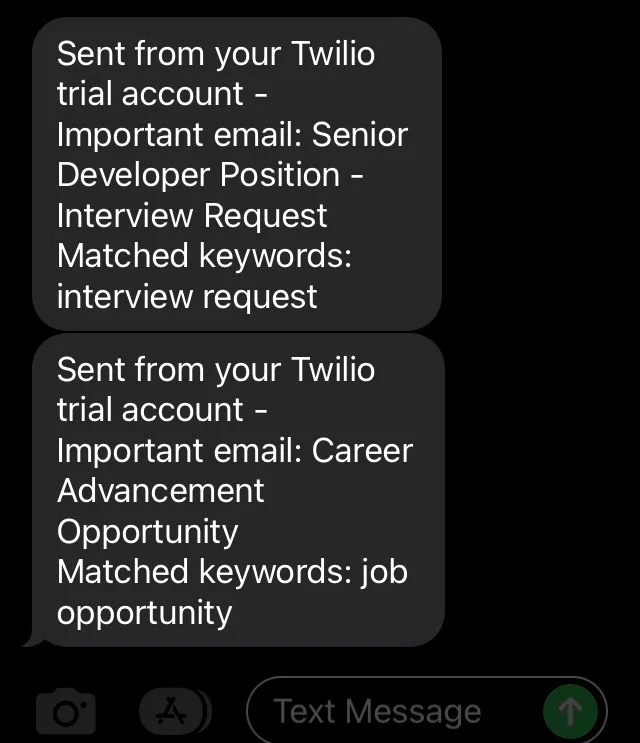
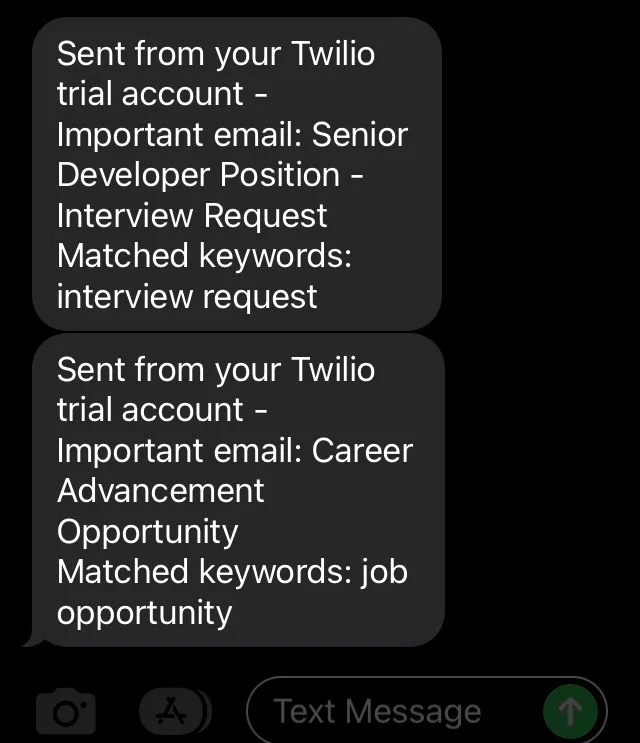
What's next for building email monitoring systems and Twilio SMS?
Congratulations! You've built a sophisticated email monitoring system that demonstrates how modern NLP techniques can be used to create powerful, cost-effective email monitoring solutions. By leveraging sentence transformers and semantic similarity, you built a system that identifies important emails without relying on expensive API calls or complex setups, showcasing how AI and cloud communications can create powerful productivity tools.
This email monitoring application can be extended in various ways:
- Keyword Categories: Group keywords by importance level.
- Web Interface: monitoring and configuration.
- Scheduled Runs: You can schedule the script to run at specific times using cron jobs.
- Email Actions: Implement functionality to reply to or forward important emails automatically.
As you continue to develop this application, consider exploring more of Twilio's communication APIs. The possibilities for enhancing and customizing this tool are endless!
For more tutorials and ideas, check out the Twilio Blog, where you can explore articles like Building a Multilingual Email App with SendGrid and Amazon Translate, Send Personalized Emails Using Gemini, SendGrid, and Node.js, and Send SMS with Node.js and AWS Lambda.
This tutorial was written by Feranmi Odugbemi, a software developer specializing in AI and cloud communications. With extensive experience in Python and a passion for creating innovative solutions, Feranmi Odugbemi enjoys exploring the intersection of artificial intelligence and practical applications. For more information or to get in touch, visit Feranmi Odugbemi or email feranmiodugbemi@gmail.com .
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.