Adding an MP3 to an Automated Voice Call using Twilio
Time to read: 4 minutes
Adding an MP3 to an Automated Voice Call using Twilio
Are you using Twilio to send automated voice messages? Text to speech can get the job done, but a recorded voice message using your own voice sounds a bit more personal. With Twilio you can attach a sound file such as an MP3 to an automated phone call and get your voice heard. You can also use an MP3 to add music and sound effects alongside text to speech for a customized flair to a programmable message.
Prerequisites
You’ll need a few things to follow along:
- A free or paid Twilio account. If you are new to Twilio, create a new one .
- A Twilio Phone Number .
- An OS that supports .NET (Windows/macOS/Linux).
- .NET 8 SDK .
- A code editor or IDE (I recommend VS Code with the C# plugin , or Visual Studio ).
- An MP3 that you want to use. Record your own or download one for testing.
- A smartphone with active service, to test the project.
Getting started with the MP3 automated voice call application
Make sure that your Twilio phone number is registered for automated calling. You can choose a toll free number, or a local number in your area code.
Before you get started programming your application, log in to your Twilio console . Take note of the Account SID and the Authorization Token which are in the lower left corner of your Dashboard page. You will need these to create the application. Also take note of the phone number that you purchased. That will be the number set for your outgoing calls. For incoming calls, choose a phone that you have access to for quick testing.
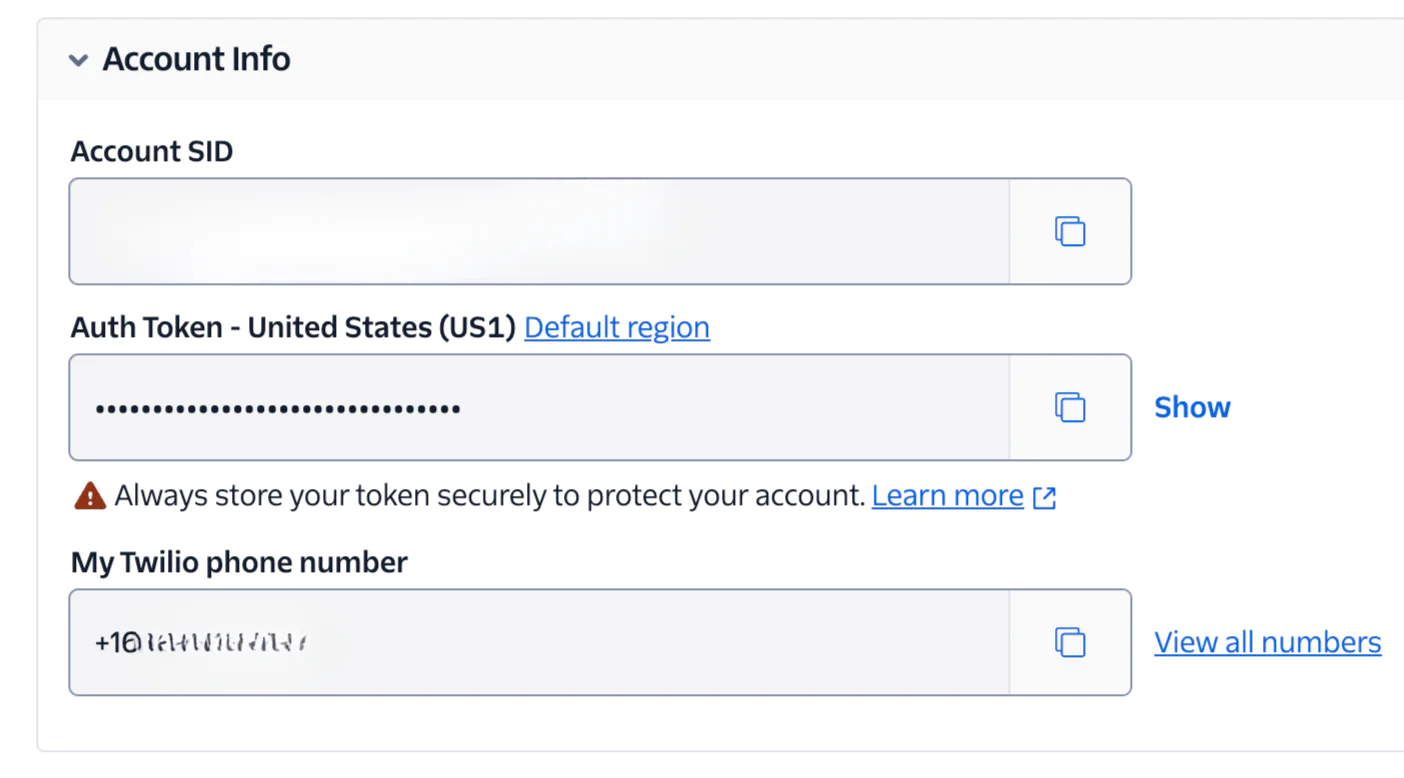
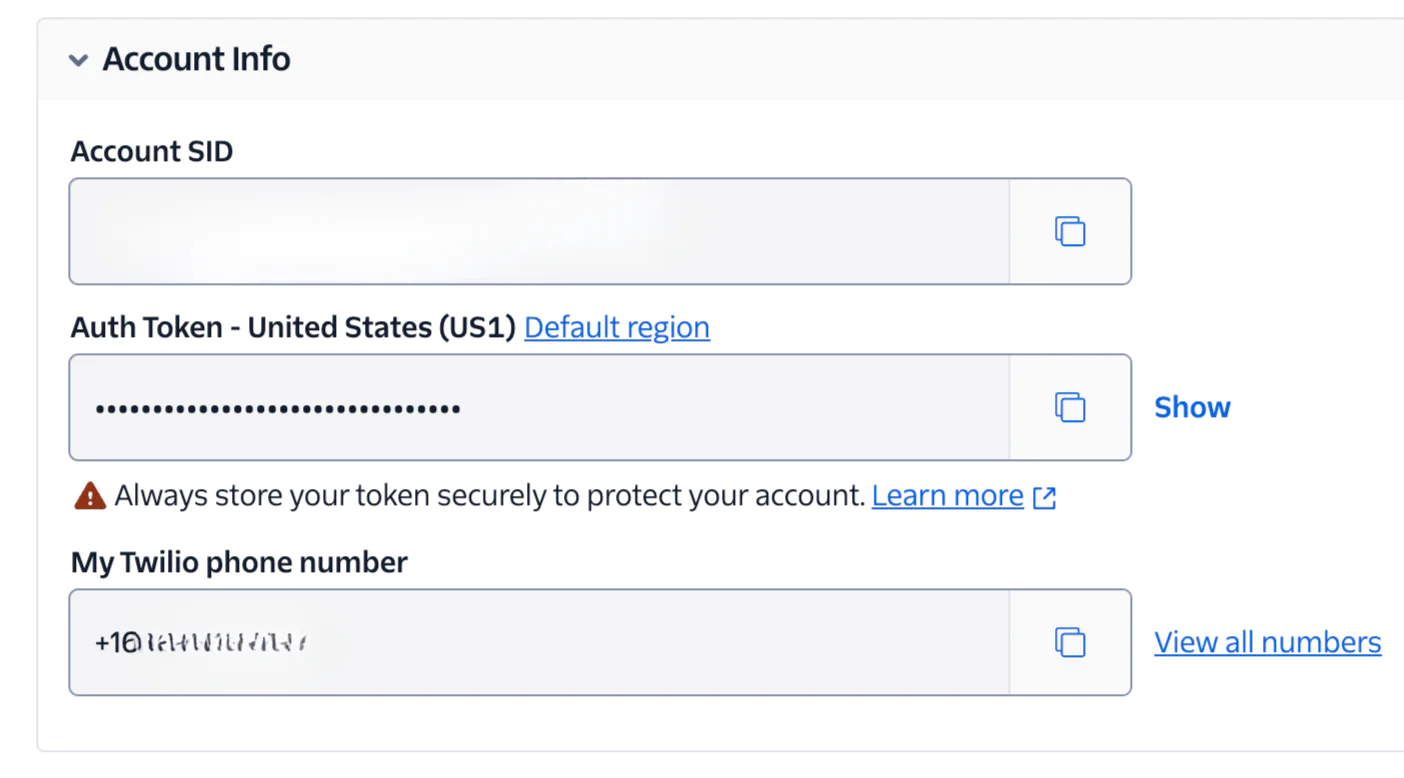
Upload an MP3 Asset
Once you have your Twilio account registered and have a phone number to use, you will need to get your MP3 asset uploaded to a public location on the web. You can use any server space or file that you can freely access. If you don't have access to your own server or files, this tutorial will go over how to upload an MP3 to Twilio Assets .
Twilio Assets is a space where you can upload files for free to use in your Twilio applications. This is an optional step. If you already have an MP3 that is publicly accessible to you, you can skip down the next segment of the tutorial to start coding your application.
To upload an MP3 to Twilio Assets, go to your console and check on Functions and Assets in the left side column. Expand the menu and click on Assets (Classic) at the bottom, as shown:
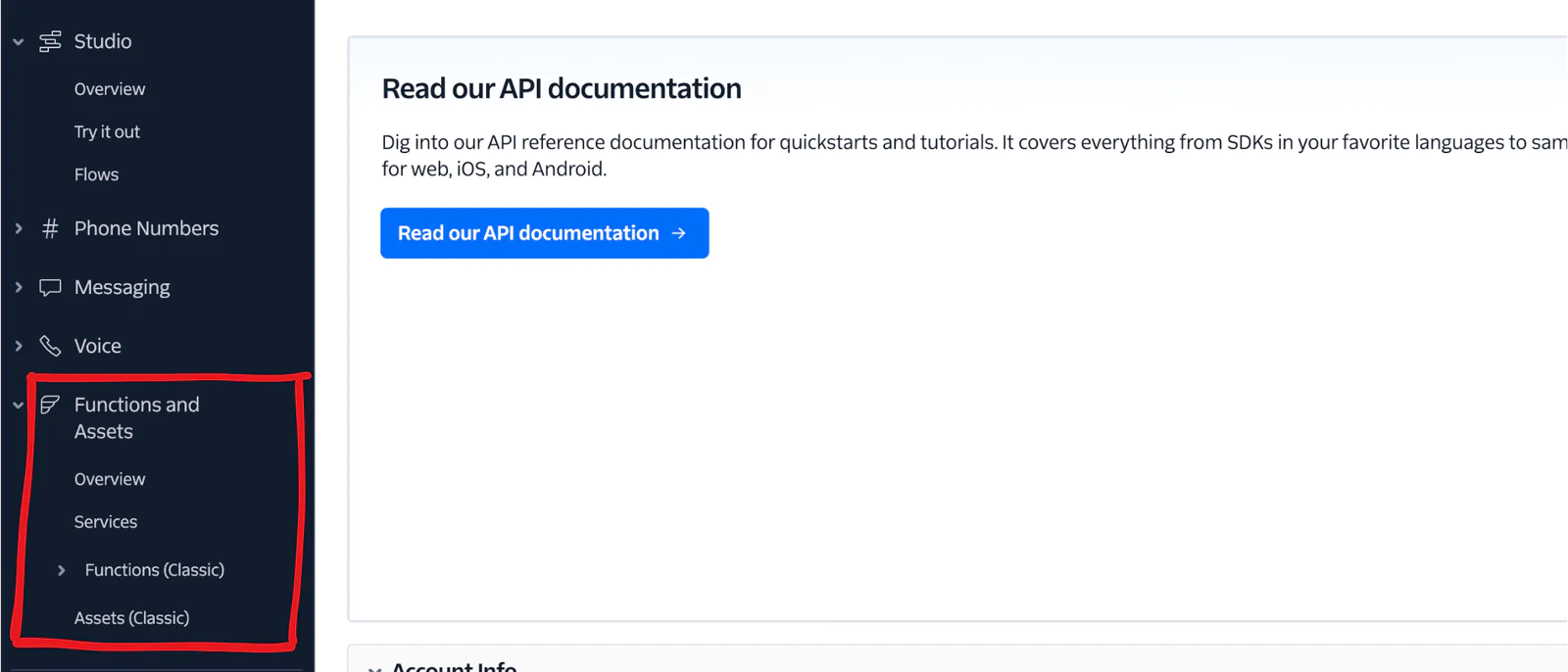
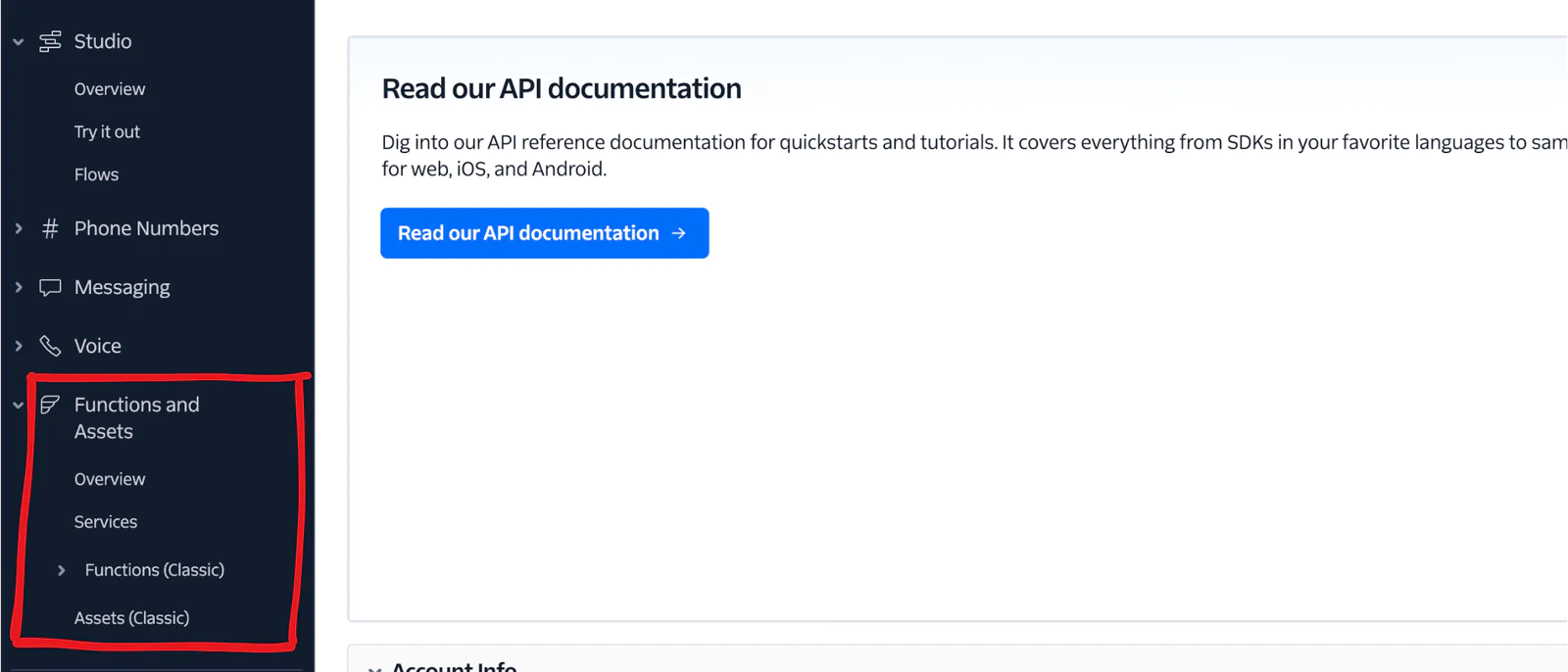
Click on the plus sign on the upper left hand corner of the window, as shown in the screenshot below:


This will access your computer to select an asset to upload. Navigate to your MP3 that you've chosen and upload it. Be sure that you upload the asset as public.
Once your file is uploaded to Assets, you can find the URL of the file on the Assets page. Click the Copy to Clipboard option on the right side if you want to copy the URL to use later.
Write the code for the automated voice call application
Now you are ready to write the code for your automated calling application. Start by creating a new .NET project in VS code. Name the project "MyPhoneCall", or any friendly name you want to use. If you are in VS Code, you can go into the Command Palette by typing Command + Shift + P or Control + Shift + P. Search for a .NET New Project, then choose Console App to tell VS Code to create a base project.
Open up a terminal. Get into the project you created, then add Twilio’s CLI to the project by typing the following into the terminal:
To ensure the security of your application, you will also need to install some environment variables to store your Twilio Account ID and Auth Token values you took note of earlier. Type the following into your terminal.
Go back to your Twilio dashboard and retrieve the Account SID and Auth Token values as seen earlier in this article. Copy paste those values and enter the following lines of code into your console, replacing the placeholders in brackets with the appropriate security codes.
Replace the code in your Program.cs file with this code:
This code above has several placeholders which you will now need to replace.
Replace `YOUR_ASSET_URL` with the URL of your chosen MP3 file. Replace the `INCOMING_PHONE_NUMBER` placeholder with the phone number you wish to call, including country code. For the `TWILIO_OUTGOING_PHONE_NUMBER` placeholder, add your Twilio phone number in that spot.
Once all the variables are properly set, you are done. Test your application and you will receive an automated phone call at the number you entered in the `to` line, containing your MP3.
Test the application with TwiML
The `twiml` line in the code above is flexible enough that you can add additional information to your phone call if you desire.
For example, try replacing the line:
With this line:
Now your application will use the text to speech line first, followed by your MP3 file. This small change will allow you to create customized variables that can personalize the call for every user with a combination of text to speech and MP3 content.
What's next for MP3 automated voice call applications?
If you're concerned about the security of your application, this article will explain some additional ways to secure environment variables in C# .
You could also add additional TwiML to your application and create programmable messages that are customized for your user. Check out this post on how to get the most out of your automated phone calls for some more helpful info.
This tutorial taught you how to send an MP3 with automated voice using the C# programming language. But if another language is your favorite, Twilio's Programmable Voice documentation has code examples for many different languages. Try this tutorial for making a mysterious phone call using Java . Or you can use Java to handle incoming calls with this tutorial .
Amanda Lange is a .NET Engineer of Technical Content. She is here to teach how to create great things using C# and .NET programming. She can be reached at amlange [ at] twilio.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.