Retrieve and Send Live Bitcoin Rates Every Day Using CEX.io, Twilio SMS, and PHP
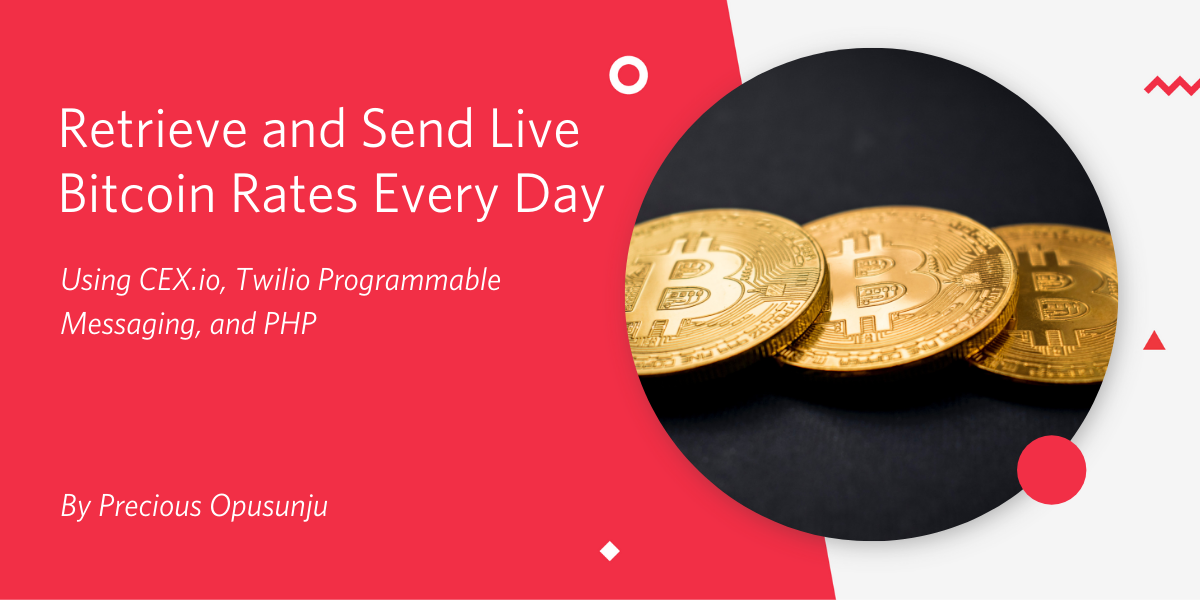
Ever wondered how platforms like Binance set price watchlists for different Bitcoin price alerts? If so, imagine getting a daily SMS alert whenever the price of Bitcoin changes.
This tutorial will teach you how to use Twilio Programmable SMS to send out live Bitcoin rates every day using your Laravel app. After we’re finished, you will be able to automatically send an SMS containing the conversion rate of Bitcoin to USD to your phone number, daily.
Installation Requirements
We will begin with a new Laravel project. Additionally, the following modules are required for proper functionality. These modules are:
- PHP 7.2+
- Composer
- Laravel
- Guzzle (Comes pre-installed with Laravel)
- A Twilio account
- CEX.io API
Having installed Laravel on your machine, proceed to create a new project using the Laravel CLI:
Or using the Composer create-project
command:
Next, install Twilio’s PHP SDK to interact with Twilio’s APIs as needed. To make this happen, use Composer to install the dependency with the following commands:
After the installation is done, add your Twilio API credentials in the .env file, as that will be used to authenticate the API requests.
Once the configuration above is completed, create a controller which will contain the functions to retrieve the current price of bitcoin and send them at different intervals.
Next, import Guzzle and the Twilio PHP SDK into the controller (found in ./app/Http/Controllers) by declaring the namespace at the top of the controller file:
Initialize the Constructor
Next, create a constructor to initialize the Twilio client, so that the appropriate APIs can be used. This is done to avoid repeating a block of code across all controller methods following the KISS principle.
The code for the constructor is as follows:
Call the CEX.io API
Next, create a function that calls the CEX.io API using Guzzle.
Next, create a function that sends out the current rate when needed.
NOTE: Don’t forget to add your phone number in the $to
variable.
Create the Command
Next, we create a command that will eventually run every 24 hours. The following command will generate a file within the app/Console/Commands folder.
Next, we call the BitcoinPriceController
in the command’s __construct()
and initialize it.
Next, overwrite the commands signature
and description
.
Next, we initialize the BitcoinPriceController
in the command’s construct:
Now we can call the sendRate()
function in the controller.
Now we are done with the commands. Next we’ll work on scheduling the function.
Schedule the BitcoinPriceWatcher Command
Finally, let’s set up the scheduler in the Kernel.php file inside the App/Console folder.
First step, add the newly created command to the list of commands.
Second step, define the schedule for our command.
Test the Bitcoin Price Watcher
At this point, you have all of the code you need to get daily price alerts for Bitcoin’s market rate. All that’s left is to test your code. Run the command by calling:
Then an SMS will be sent out with the current rate of Bitcoin.
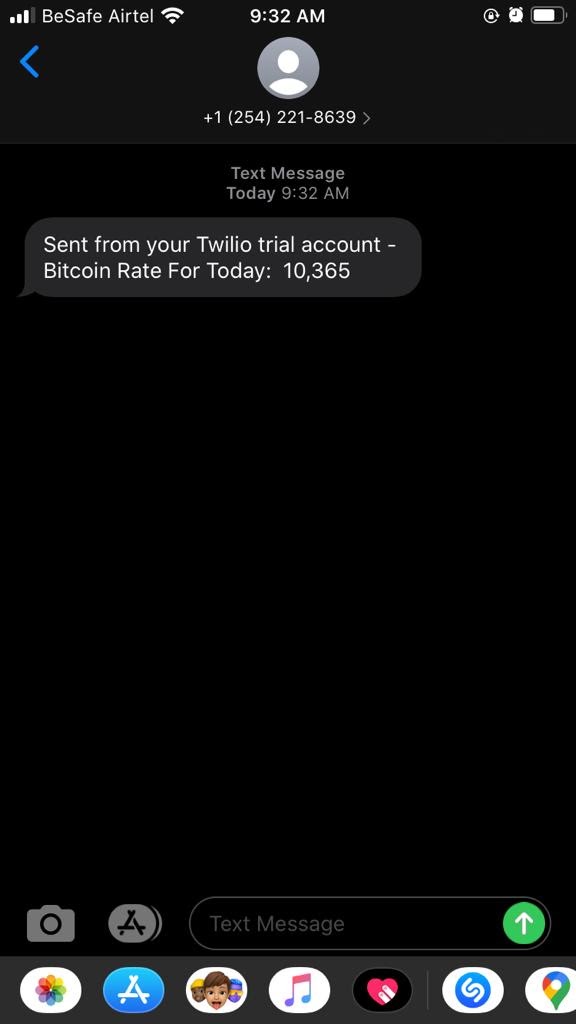
Set up a cron job
Now, you should create a cron job to run the command automatically, every 24 hours. You can use the crontab command below to set this up.
To edit or create your crontab file, type the following command at the UNIX / Linux shell prompt:
For the cron job to run at 3 am every day, add the following line:
Checking Bitcoin’s Daily Price with a Bitcoin API and Twilio
Now that you have set up a Bitcoin Rate monitoring app you can see how straightforward it was to automate this process. Using CEX.io and Twilio’s Programmable Messaging, you’ll always know the price of Bitcoin, whether you have internet access or not!
If you would like to extend this tutorial, you should check out CEX.io's API for more tools and API endpoints to personalize your Bitcoin SMS delivery.
You can find the code repository for this tutorial here.
Precious Opusunju is a backend software engineer and technical content creator. He can be reached via:
Email: masterpreshy1@gmail.com
Github: https://github.com/Preshy
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.