Send an SMS Message with C# in 30 Seconds
Time to read: 1 minute
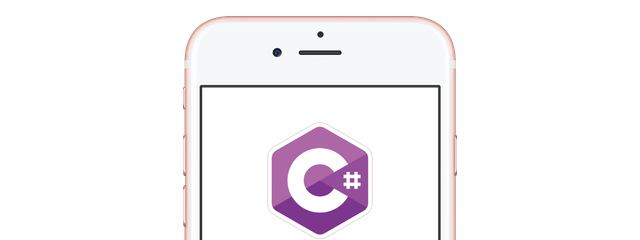
You’re building a .NET app and you need to send SMS messages. What if I told you you can get it done in 30 seconds with the Twilio API? Here’s a video showing you how quick it is to send an SMS message with C# and the Twilio API.
Video: How To Send SMS with C# in 30 Seconds
But you can’t copy and paste from a video, so here’s all the code you will need.
Install the Twilio helper library for .NET to your project using the package manager console.
Import the Twilio namespace into your class and initialize the Twilio REST Client passing your Account SID and Auth Token, which are available on the Twilio account portal:
You will need three things now:
- The Twilio number you are sending the message from
- The number you are sending the message to
- The body of the message
Add those into the MessageResource.Create method which will send a text message:
Now run it and wait for the magic to happen.
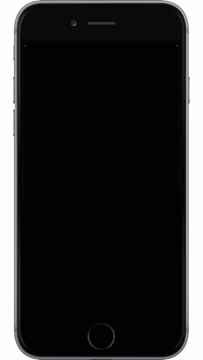
We can’t wait to see what you build
You’ve sent an SMS message and now you’re ready to take on the world of communications. Take a look at the Twilio REST API documentation to see what else you can do and the documentation for working with the .NET helper library. Then check out our tutorials to see some more examples, like: sending SMS notifications, masking phone numbers for user privacy or two factor authentication for user security.
Excited about the possibilities? Then let me know! Hit me up on Twitter or just give me a shout in the comments below.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.