How to Validate an E.164 Phone Number in Go
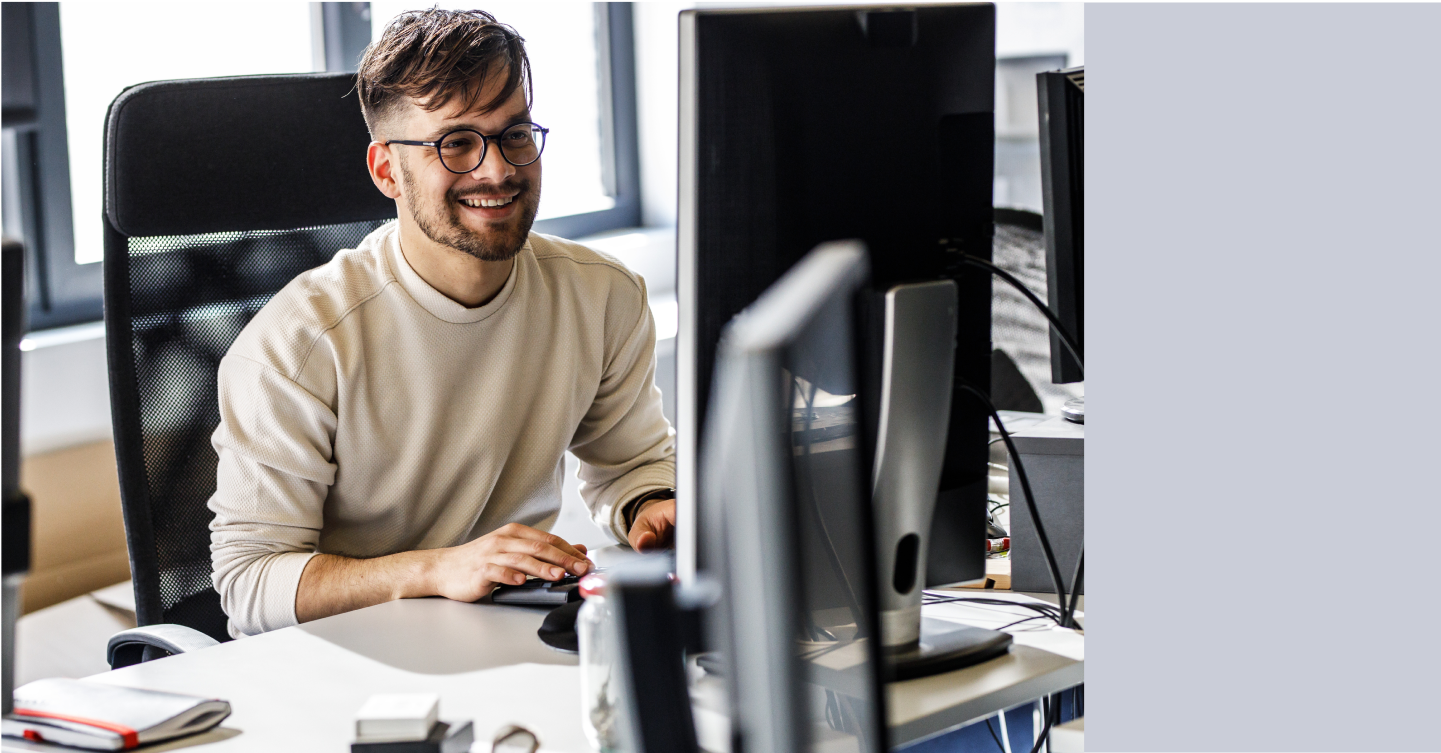
Time to read: 2 minutes
If you're sending SMS, MMS — or performing any other form of communication using Twilio's Programmable Messaging API — you'll know that phone numbers must be in E.164 format. The question is, how can you validate them in Go?
Well, in this short tutorial, you're going to learn how, using Twilio's E.164 regular expression (tl;dr, it's ^\+[1-9]\d{1,14}$
).
Actually, you're going to learn a bit more than that. Just showing the core code to validate a phone number would only take about 30 seconds. So, instead, I'll make the tutorial a bit more interesting and fun.
You're going to learn how to create a small Go-powered API that can accept requests with a phone number, and return a small JSON response confirming whether the number is correctly formatted or not.
Prerequisites
To follow along with this tutorial, you're going to need the following:
- Go
- curl
- Your favourite text editor or IDE. I recommend Visual Studio Code.
- Some prior experience with Go would be helpful, though not required
Create the project directory structure
Where you keep your Go projects: create a new project directory, change into it, and track modules, by running the following commands.
Build the Go API
Now, let's write the Go code. Create a new file named main.go, and in that file paste the code below.
The code defines a small function, IsValidPhoneNumber()
. It uses Twilio's E.164 regex and Go's regexp package to determine if the phone number, phone_number
, is formatted correctly. It returns true
if it is or false
otherwise.
Then, it defines another function, validate()
. The function starts by attempting to retrieve the phone_number
parameter from the request. If it was not set or was empty, a response is returned with a message stating this. Otherwise, the retrieved phone number is validated with IsValidPhoneNumber()
. The response's body is then set to a message confirming whether the number was valid or not.
Finally the main()
function is defined. It sets up the application to handle HTTP requests, with the validate()
function handling requests to the application's default route.
Test the code
With the code written, it's now time to test that it works. Start by launching the application with the following command.
Then, run the following command to make a request to the app with curl. Make sure to replace the phone number placeholder with the phone number that you want to test.
If the phone number is valid, you should see the following output in the terminal (with the phone number you entered instead of the placeholder).
That's how to validate that an E.164 phone number in Go
There's not a lot to do. But it's still helpful to know how. I hope that you found the approach that I took to be fun and interesting.
How would you check whether a phone number is in E.164 format? Let me know on LinkedIn?
Matthew Setter is a PHP and Go Editor in the Twilio Voices team and a PHP and Go developer. He’s also the author of Mezzio Essentials and Deploy With Docker Compose. When he's not writing PHP code, he's editing great PHP articles here at Twilio. You can find him at msetter[at]twilio.com, on LinkedIn and GitHub.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.