Understanding Function Calling with Claude 3 and Twilio
Time to read: 9 minutes
Understanding Function Calling with Claude 3 and Twilio
As the use of generative artificial intelligence (AI) models becomes wide and popular, users now require more from these models than just input and generative output based on information they are trained on. There is now a need for these models to be able to interact with external services like APIs to be able to perform tasks or enhance their capabilities to meet the user's needs.
Function calling in an AI model refers to the ability of the AI to not only respond to input with text but also to trigger specific, predefined functions that execute certain tasks or interact with external systems. This concept enhances the AI’s capability by allowing it to interact with external services, databases, APIs, or any other programmable feature, making the AI more dynamic and practical for real-world applications.
In this article you will learn how the function calling feature works in an AI model and build a demonstrable real-world solution using Twilio WhatsApp API.
Prerequisite
Here’s what you need to follow along in this tutorial:
- Node.js installed
- A free Twilio account ( sign up with Twilio for free).
- An Anthropic AI API key - After signing up for an account, navigate to the API Keys section to generate a key..
- Amadeus flights API key and secret
- Install Ngrok and make sure it’s authenticated.
Claude’s function calling explained
Claude's function calling capability allows it to interact with external tools and APIs in a structured way, enhancing its ability to perform specific tasks or retrieve information beyond its training data. This feature enables Claude to seamlessly integrate with various systems and services, expanding its functionality and usefulness.
The architecture
Claude's function calling system is built on a multi-layered architecture:
- Intent Recognition Layer: Utilizes advanced natural language understanding (NLU) algorithms to identify when a user's request requires external function execution.
- Function Matching Engine: Employs a combination of semantic similarity scoring and contextual relevance analysis to select the most appropriate function from the available set.
- Parameter Extraction Module: Leverages named entity recognition (NER) and contextual inference to populate function parameters accurately.
- Execution Interface: A robust API gateway that manages the communication between Claude and external systems, handling authentication, rate limiting, and error management.
- Response Integration Layer: Processes function outputs and seamlessly incorporates them into Claude's language generation pipeline.
Some of the key components
- Function Definitions: These are JSON-formatted descriptions of available functions, including their names, parameters, and expected outputs. They serve as a blueprint for Claude to understand how to use each function.
- Natural Language Processing: Claude interprets user requests and determines when a function call is necessary to fulfill the task.
- Parameter Extraction: The AI identifies and extracts relevant information from the user's input to populate function parameters.
- Function Execution: Claude calls the appropriate function with the extracted parameters, which is then executed by the external system.
- Result Interpretation and Error handling: Once the function returns a result, Claude processes and interprets the output, incorporating it into its response to the user whether it is successful or an error response.
Use case with Twilio WhatsApp API
In the demo, we will be building a bot that takes prompts from WhatsApp and gets available flights between two cities at a given date. We will be using the Twilio WhatsApp API and make Claude call a function in the app to get the flight data and use whatsApp to send the response back to the user in a formatted way. Let us get into it!
Setting up Twilio account
To set up our Twilio account, sign up for an account and login into your Twilio Console using your account details. From the toolbar's Account menu, select API Keys and Tokens. Take note of the test credentials as shown in the photo below, which include the Account SID and Auth Token, we will use them later in your application.
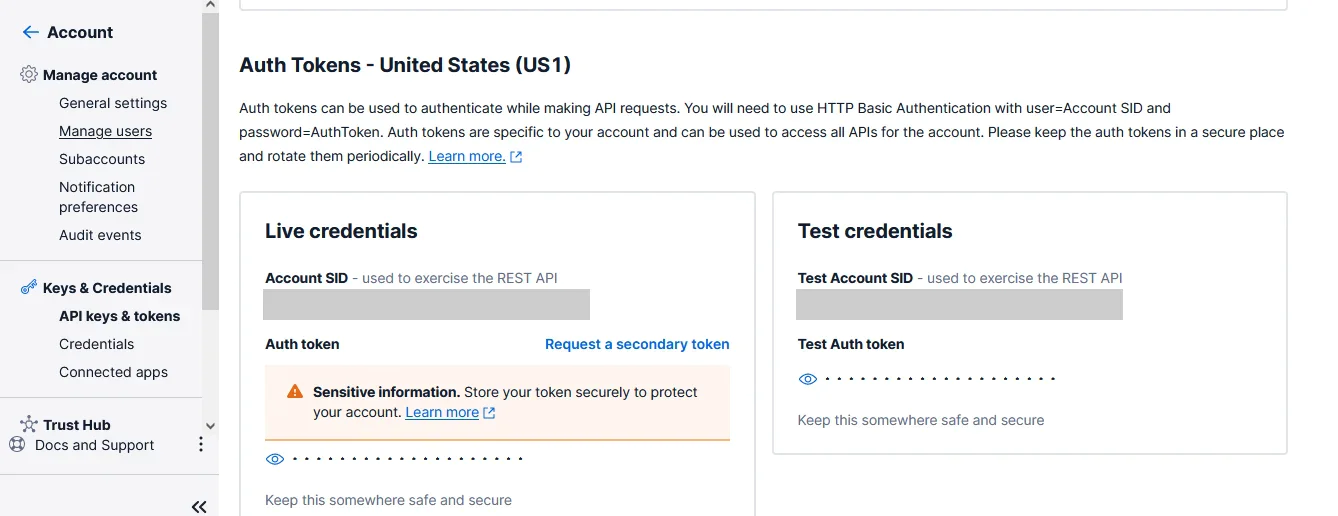
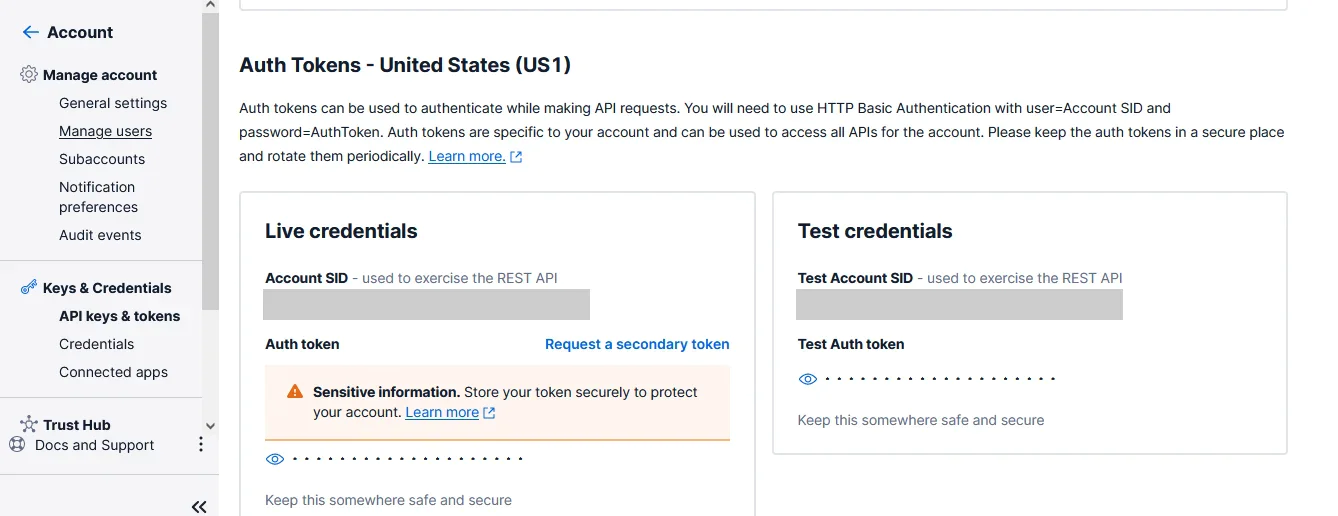
Setting up Twilio WhatsApp Sandbox
Access your Twilio Console dashboard and navigate to the WhatsApp sandbox in your account. This sandbox is designed to enable us to test our application in a development environment without requiring approval from WhatsApp. To access the sandbox, select Messaging from the left-hand menu, followed by Try It Out, and then Send A WhatsApp Message. From the sandbox tab, take note of the Twilio phone number and the join code. We will add the Twilio WhatsApp phone number to our .env file for later use.
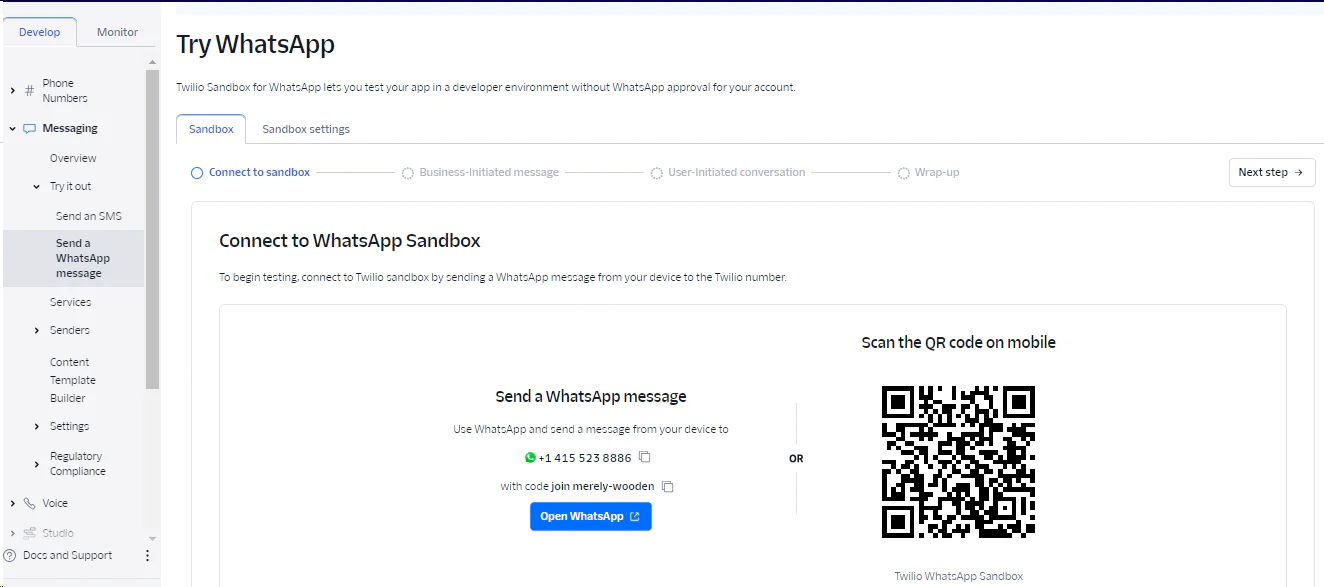
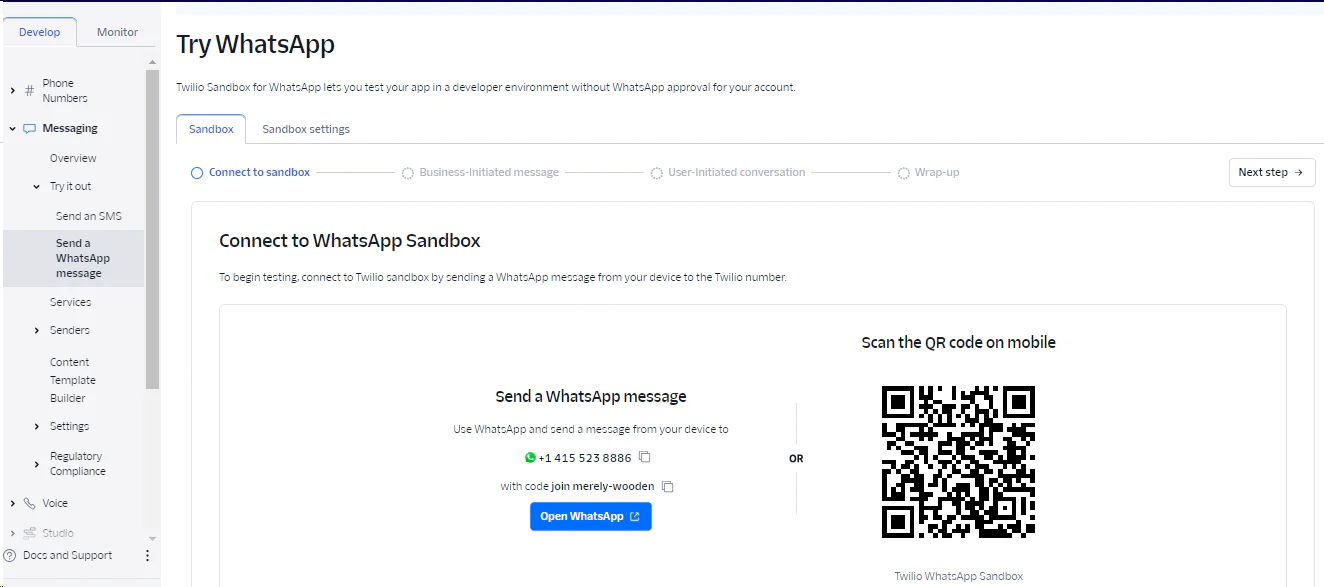
Building the app
Our Twilio account and sandbox is now set up. Ensure you also have a Claude API key from Anthropic and an Amadeus API key and secret from the prerequisites. Let's start building by creating our project directory.
Create a new directory for the project and navigate into it:
Then, create a new Node.js project using the npm command:
Next, install the following dependencies:
- dotenv: Zero-dependency module that loads environment variables from an env file into process.env.
- express: Minimal Node.js web application framework.
- body-parser: Node.js body parsing middleware.
- nodemon: Auto restart tool for node application in development mode.
- twilio: Twilio Node.js client SDK for utilizing Twilio in Node.js applications.
- anthropic-ai/sdk: Anthropic’s SDK for Javascript and Typescript.
- amadeus: Amadeus Node.js SDK for flight search functionalities.
The next step is to create a new file named .env and add the following environment variables:
Adding helper functions
Now let us work on the core logic and functions of the app. Create a /helpers folder in the root directory of your project. It will contain the helper functions that will help us extract the core information from the prompts coming from the user and also the function for getting the flights. In the /helpers folder add two files; flights.js and request.js. Add the following code to them:
flights.js
In the flight.js file, we initialize the Amadeus client with the credentials. We then use the client to search for flights with the destination, origin, number of passengers and departure date as parameters. The resultant flights are then returned for use by Claude.
request.js
This helper function above uses the generative power of Claude to extract important information like origin, destination, departure date and return date from the user prompt. It then returns them in a structured manner for the getItinerary()
function to use. If any of these key data is missing, it will return the error and the send message function will send the response back to the user via WhatsApp.
Implementing function calling
Now that we have the helper functions we need, let us work on the messaging service and the itinerary generator itself. Create a new folder in the root directory of your project called lib. In the lib folder, create these new files; itinerary.js and messaging.js. Add the following codes to them.
messaging.js
In the messaging.js file we are initializing the Twilio client and using it to send WhatsApp messages. It accepts the destination number and the message to be sent as parameters. If the message fails to send, the catch block will handle the error and return it.
itinerary.js
The itinerary.js file contains the core logic of this app. First, we initialize the Claude client via the Anthropic SDK, then define the flight search schema for Claude to use. Function calling is all about chaining conversations together as if you are chatting with the Claude model. We gave it the initial prompt to compile a list of flights with the given parameters. We also let it know that there is a tool available to use for that action by passing the tool parameter to it which is the schema we defined earlier.
With that schema it will do validation and error handling when the data available is not matching the types defined in the schema. The first response is the AI letting us know that it has recognized the tool and is ready to use it. Then we pass the parameters to the function we want to call to get the real life results. After a successful function call, we chain the response with the first two prompts(one from the user at the start and the other from Claude response) our last request for Claude to use the response from the function call to prepare a formatted response for the user.
The final response from Claude is now sent to the user via Twilio WhatsApp API. To couple the whole app together, create a new file in the root directory of your project called index.js. This is our entry file and where the webhook from Twilio will be hitting whenever a user types any prompt in the WhatsApp bot. In the index.js file add the following code:
This file is a simple Express app setup that uses the body parser middleware to parse request body. It exposes a POST request route which will serve as an incoming webhook route and will handle the users request and send response when request is being fulfilled or fulfilled.
The webhook has the user’s prompt as Body
and the user's number as From
in the request body. The user is messaged that their request is being processed and the Body is passed to the function that extracts the core details, calls the itinerary tool powered by Claude and returns the formatted response of available flights for the destinations and durations. The response is now sent to the user and that ends the cycle.
Testing the app
Our application is ready for testing now. Before we go into testing the application functionality, make sure you create a .gitignore file and add the node_modules folder and the .env file so that you will not commit and push your secret keys to version control systems or wherever you host your code.
First update your package.json file to include the run scripts for our project to use. We are using nodemon to run the project locally so that it can also watch for changes and restart the application. In the script section, update with the command below:
We want to mock a production environment so we will use ngrok to proxy our localhost to a live host URL. First, run the start command:
When the app has started successfully we will open a new terminal and run this ngrok command to proxy the localhost server. Make sure you have already installed and authenticated ngrok in your dev environment.
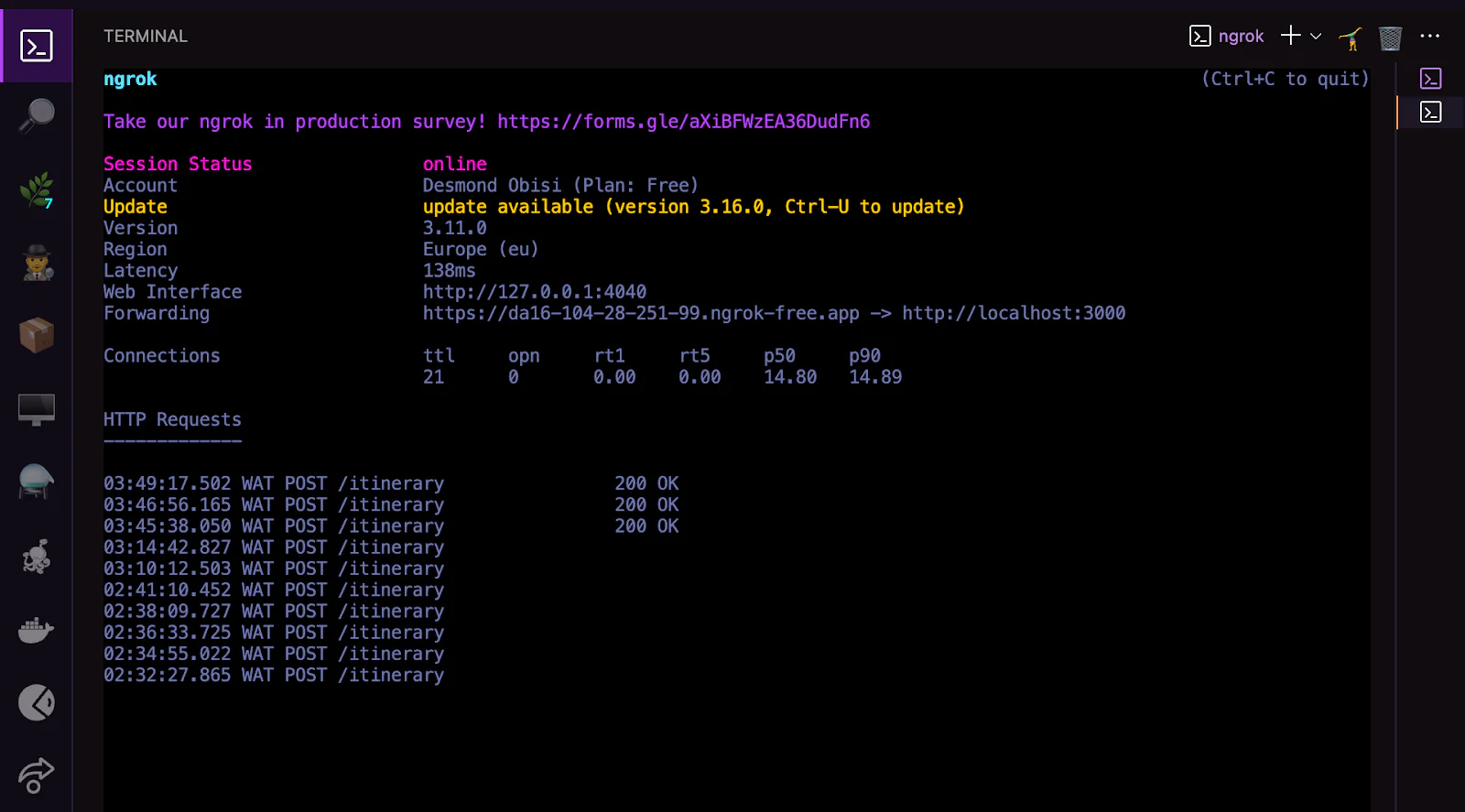
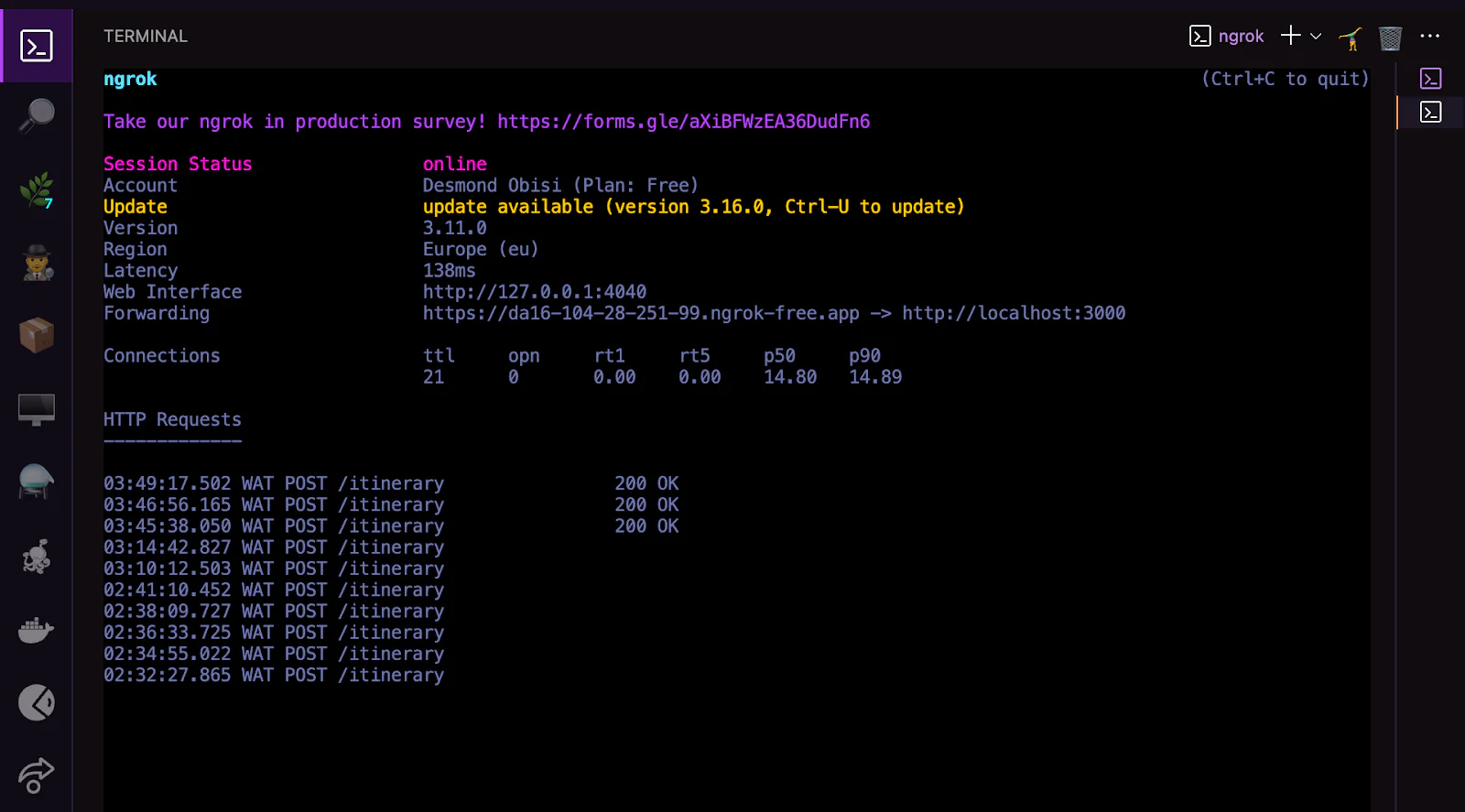
After you have started the ngrok server, you will head to your Twilio console where you have your WhatsApp sandbox configuration and add ngrok_url/itinerary as the webhook URL when Twilio will send the webhook to. That way, our application will get the message or prompt from the Twilio WhatsApp bot because our Express app is exposing the /itinerary route for that purpose.
The ngrok URL is the public address ngrok port forwarded your local application to, from the image above, you can see it under Forwarding. Note that this sandbox settings page can be seen when you run the WhatsApp demo on the sandbox to the end.
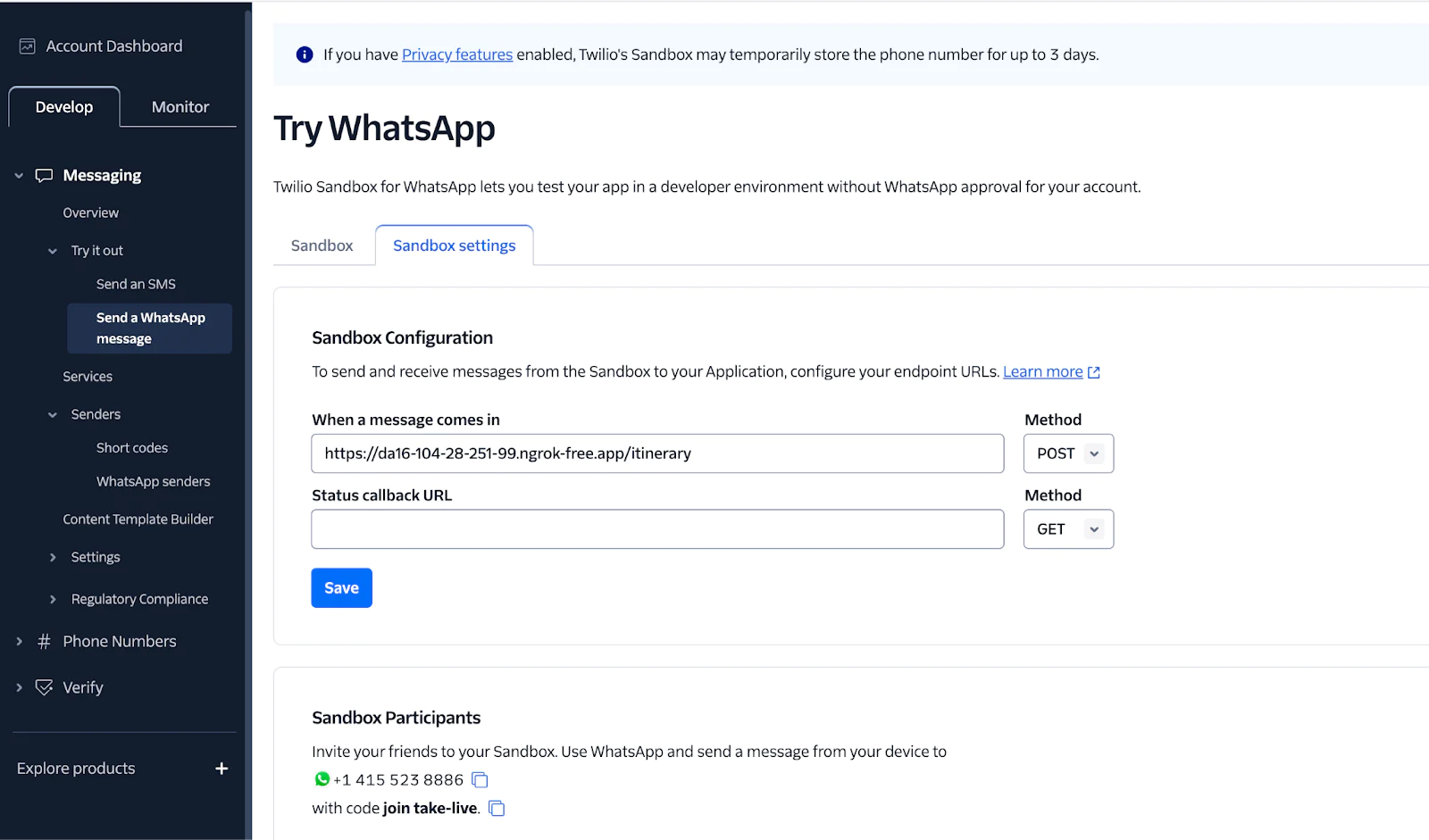
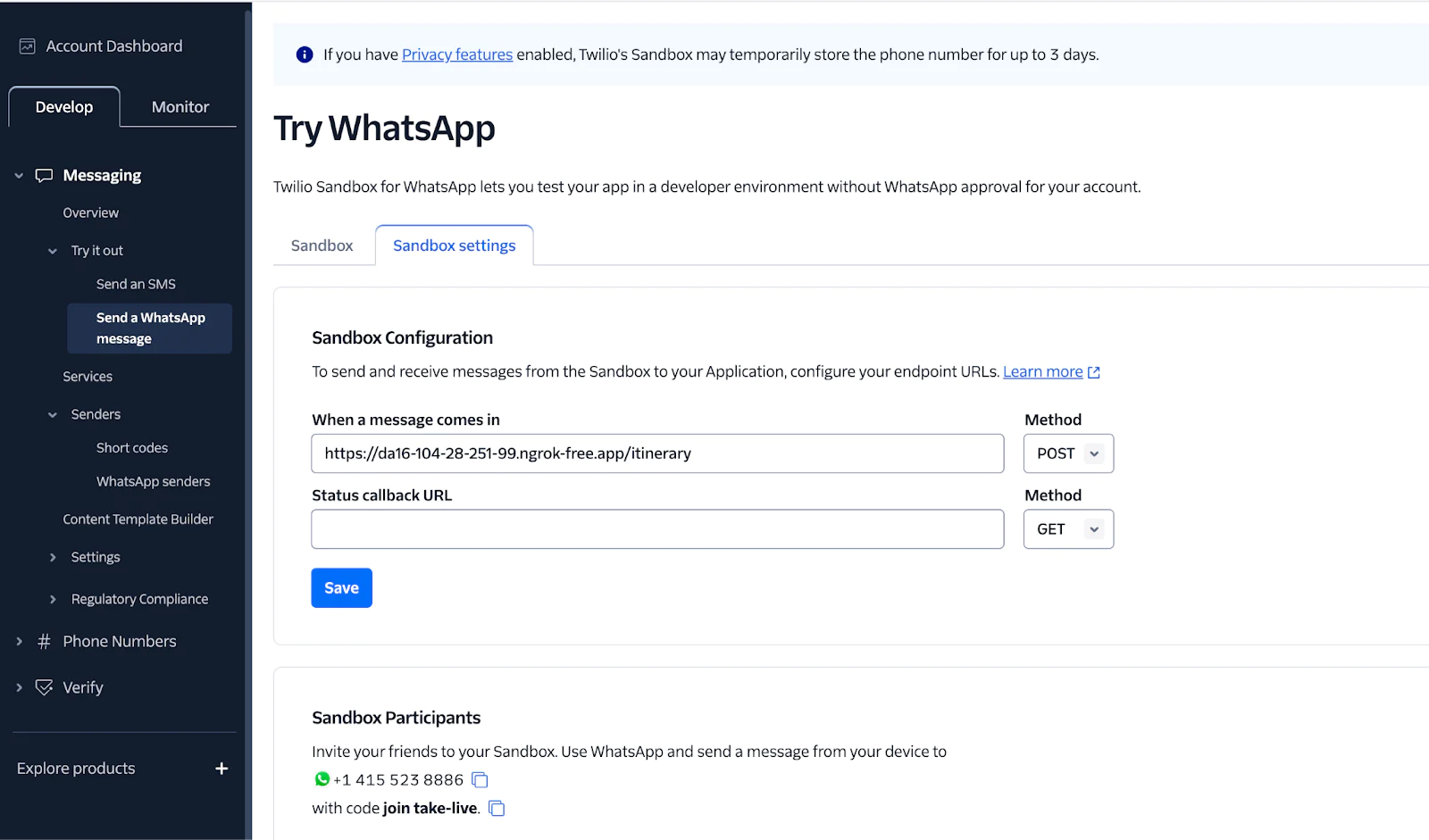
Finally, open WhatsApp on your device, add the Twilio WhatsApp number and join with the code that is displayed in your sandbox. When the sandbox connection is established, you can now prompt the AI to get flights for your holiday or vacation. A typical prompt will be this: “I want to go on vacation in Mexico from Lagos from 2024/12/13 to 2024/12/30. Get me the available flights in a list.” See below a typical response from Claude:
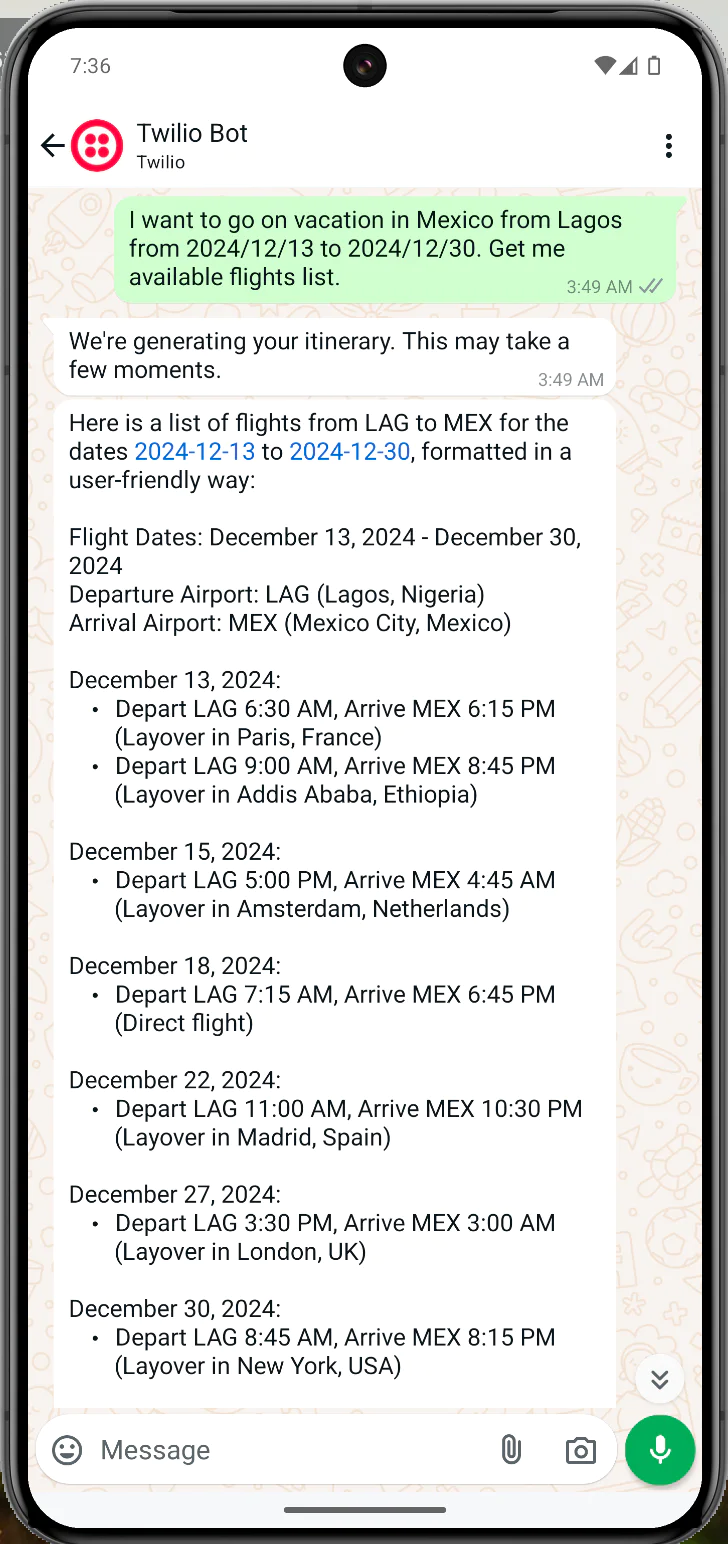
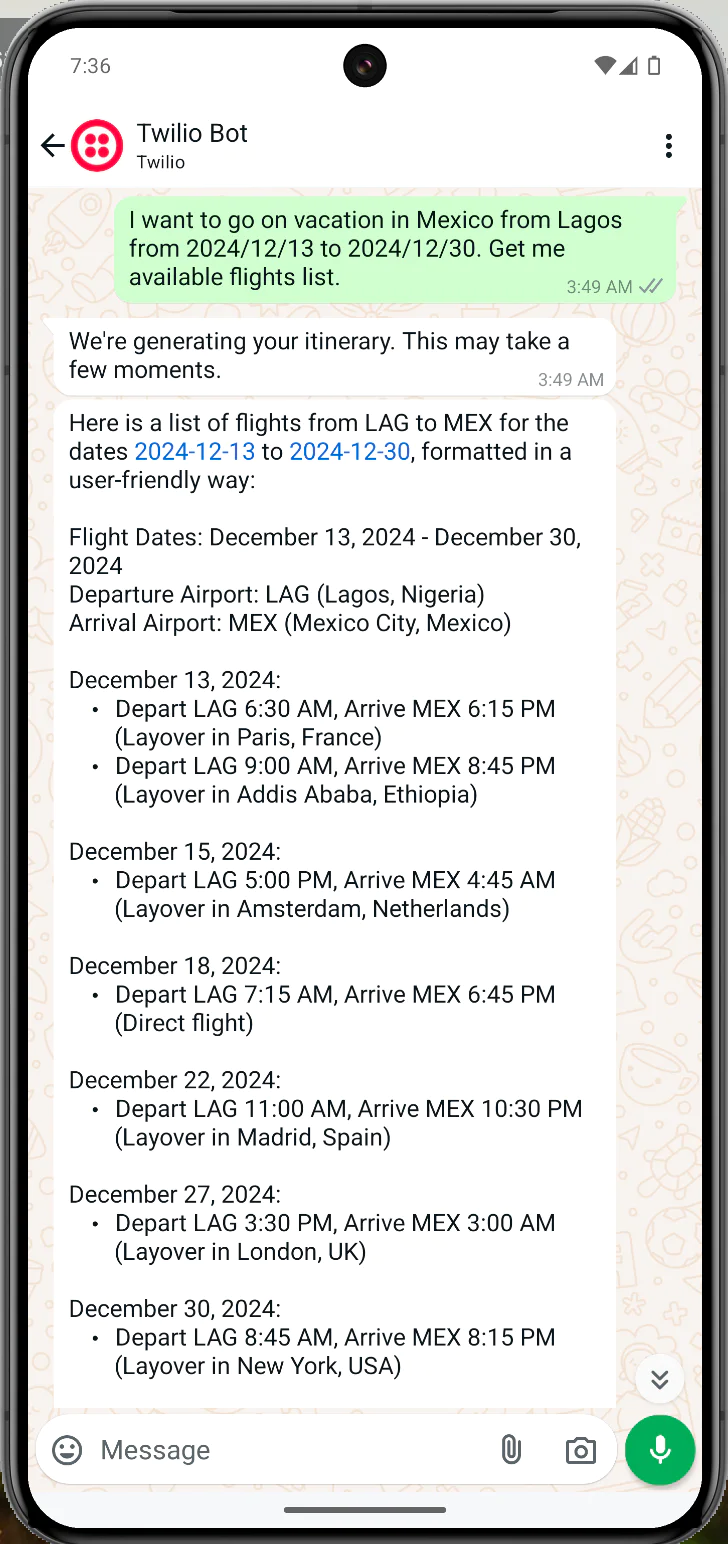
Benefits and limitations of function calling in AI workflows
Function calling in AI systems like Claude offers significant advantages but also comes with certain constraints. Understanding these can help developers and users optimize their AI-powered workflows.
Benefits of function calling
- Enhanced Capabilities: Function calling allows Claude to perform tasks beyond its core language model, such as real-time data retrieval or complex calculations.
- Flexibility: New functions can be added or modified without retraining the entire model, allowing for easy expansion of capabilities.
- Accuracy: By using structured function calls, Claude can interact with external systems more precisely than through natural language alone.
- Integration: This feature enables seamless integration with various APIs and services, making Claude a versatile tool for diverse applications.
Limitations and Considerations
- Function Availability: Claude can only use functions that have been defined and made available to it.
- Security: Proper safeguards must be in place to ensure secure handling of sensitive information and prevent unauthorized function calls.
- Dependency on External Systems: The reliability and performance of function calling depend on the availability and responsiveness of the external systems being called.
Rounding off
Function calling in artificial intelligent models like Claude represents a powerful shift from static text generation to dynamic, actionable interactions. By integrating function calling, developers can extend the AI’s capabilities beyond conversation, enabling it to perform complex tasks in response to user inputs. This makes AI applications more practical, customizable, and efficient for real-world use cases like customer support, notifications, and data retrieval.
With tools like Claude and function calling, AI is not just a chatbot but a versatile assistant capable of interacting with the digital world in a meaningful and impactful way.
You can learn more about transactions by referring to:
Desmond Obisi is a software engineer and a technical writer who loves doing developer experience engineering. He’s very invested in building products and providing the best experience to users through documentation, guides, building relations, and strategies around products. He can be reached on Twitter, LinkedIn, or through email at desmond.obisi.g20@gmail.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.