How to send an SMS magic link for one-click phone verification
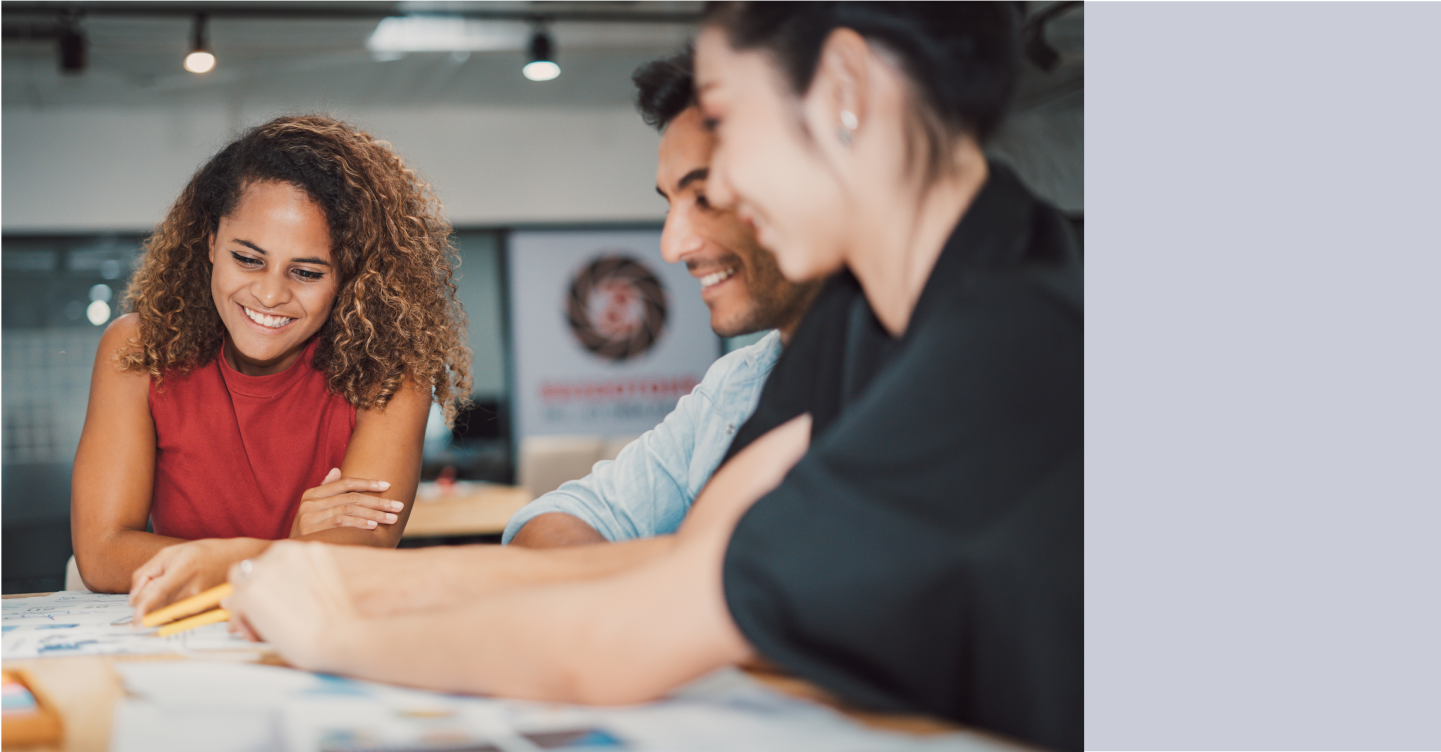
Time to read: 5 minutes
How to send an SMS magic link for one-click phone verification
Introduction
SMS verification is incredibly popular for a reason: it's widely accessible, user-friendly and intuitive. However, traditional SMS one-time passcodes (OTPs) require the additional step of typing in the code to a web form. Magic links, or clickable verification links, improve the user experience by creating a one-click verification experience that can be used for phone number verification, login, or authorization.
This tutorial will show you how to implement SMS magic links with the Twilio Verify API. The Verify API is a simple multichannel API for user verification and authentication that will abstract the more annoying parts of sending an SMS (or Voice call, WhatsApp message, email, and more) and let you focus on the business logic.
What sending an SMS magic link will look like
Step 1: The user inputs their phone number on your application OR user logs in and already has a phone number associated with their account.
Step 2: Your application calls the Twilio Verify API /verifications
endpoint to send an SMS to the end user. The Verify API populates a template containing your verification URL with a user identifier and the OTP e.g. yourco.biz/verify?id=+14151234567&code=123456.
Step 3: The user receives the SMS and clicks on the link.
Step 4: Your application calls the Twilio Verify API /verification-check
endpoint to validate the provided OTP.
Step 5: Your application checks that the verification is approved
and sets a session or sends a callback to the initiating page indicating verification success.
Prerequisites for sending an SMS magic link verification
To code along with this tutorial you will need:
- A Twilio Account: sign up or sign in.
- A Twilio Verify Service. Create a Verify Service in the console and make note of the Service SID.
- Basic understanding of JavaScript
Create a Twilio Function service for testing your magic link
In your favorite text editor, create a new file called verify.html
and add the following code. Save the file somewhere you can easily find it to upload in the next step.
Head over to the Twilio Code Exchange and deploy this sample project as a starting point. This project will provide a few things:
- A phone number input field and general boilerplate
- A live URL you can use for your SMS magic link
After you deploy your project, click the option to Edit your customizations directly in code. This will take you to the functions editor in the Twilio Console.
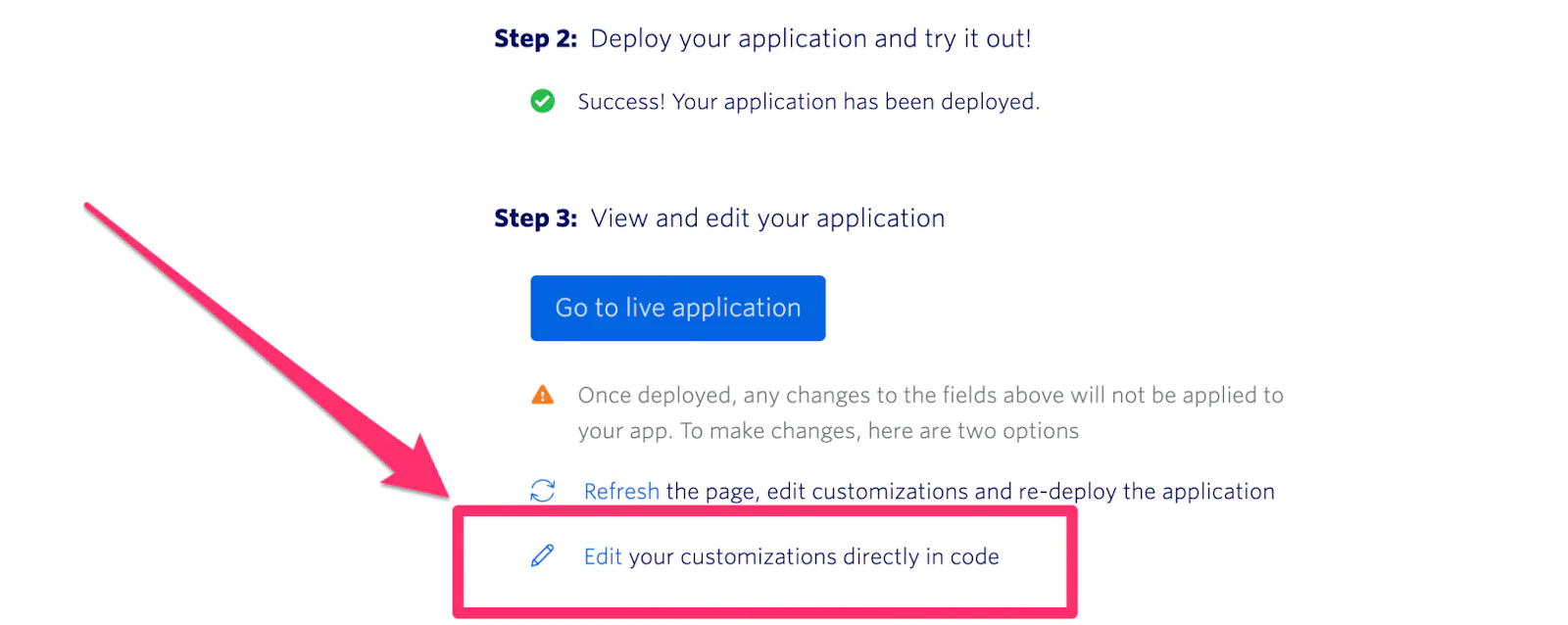
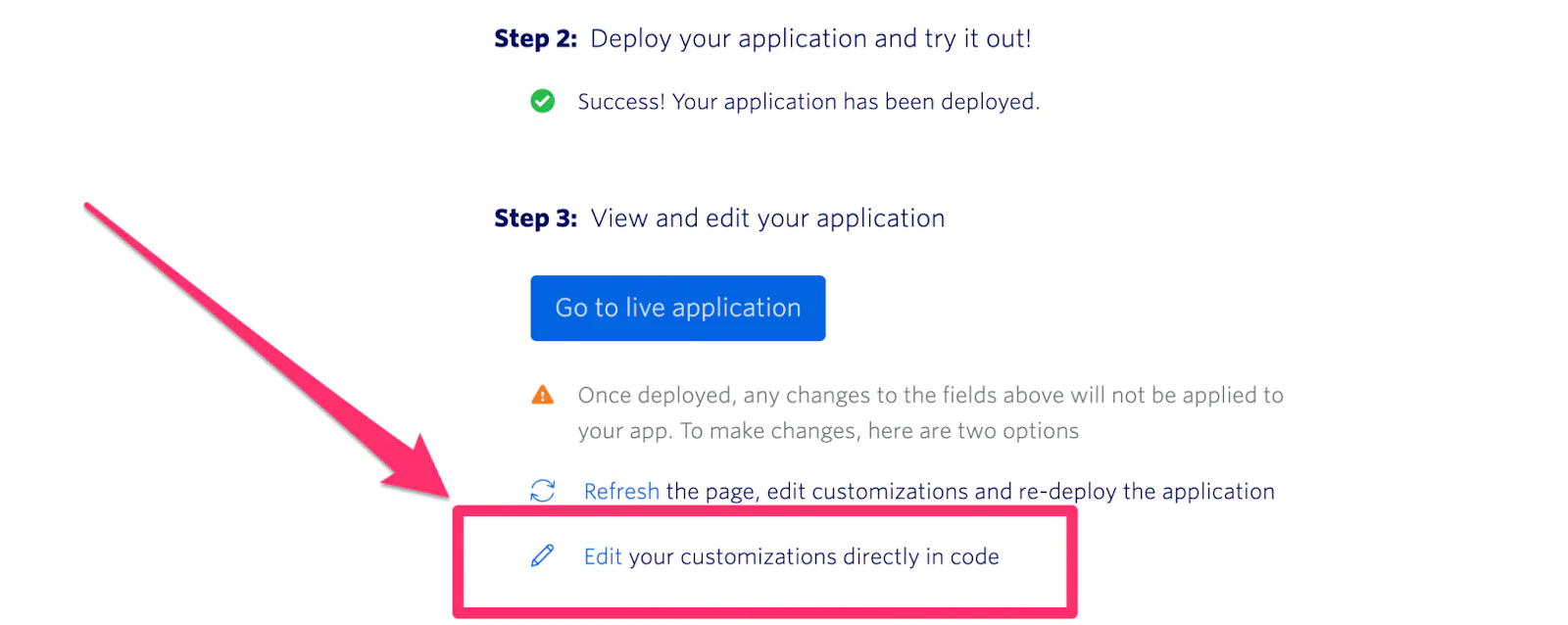
Click the Add + button to upload the verify.html
file you created. Select "Public" visibility and upload your file. Learn more about assets.
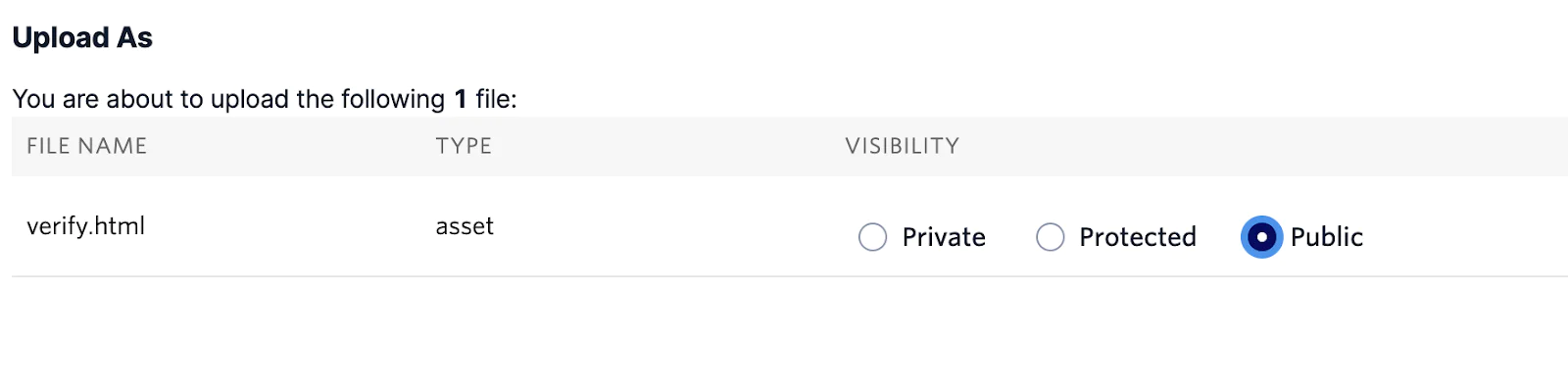
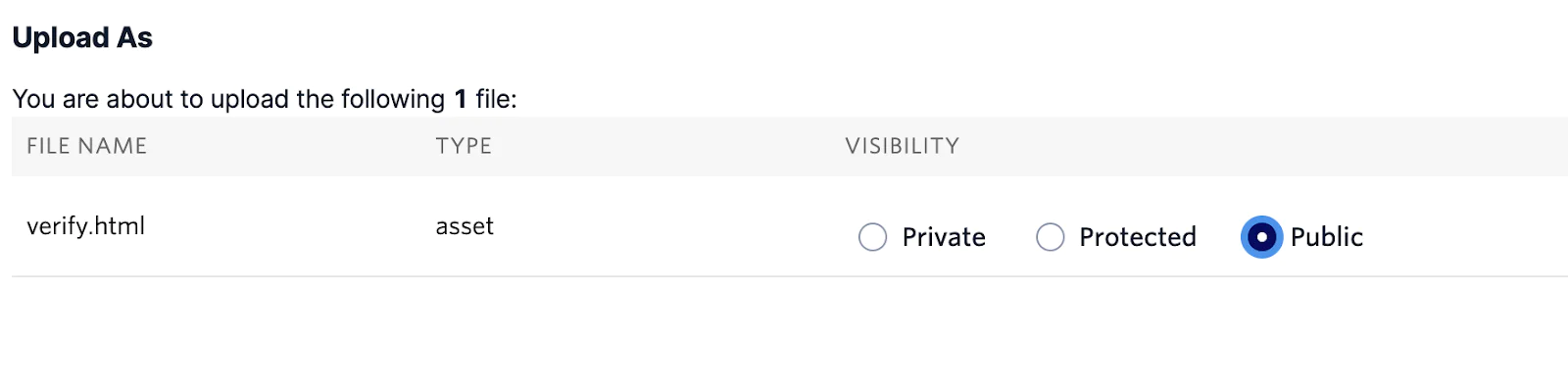
Click Deploy All then copy the URL of your new file. This is what you will use in the next step to create your SMS magic link template.
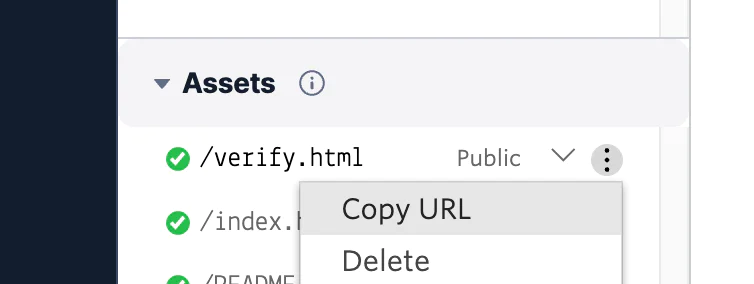
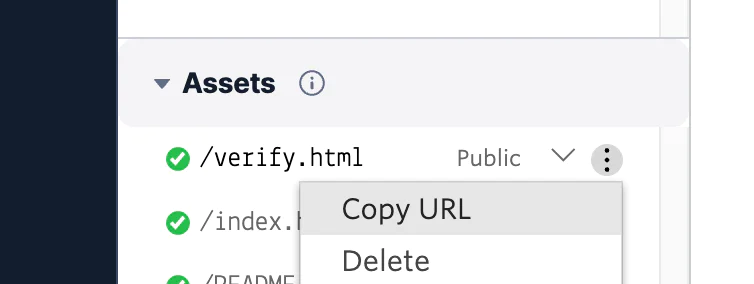
Note: as an alternative to Twilio Functions, you can host this code on any publicly accessible hosting provider (so that it can be accessed after clicking a link in an SMS), e.g. Vercel or GitHub pages. Ensure that the URL will not change since it will be hardcoded into a template in the next step.
Request a custom template for your verification message
Verify has a variety of predefined templates, but in order to ensure compliance, Twilio requires approval of a custom template with the verification URL users will click on.
To request a custom template, head over to our support site and tell the bot:
> I need to submit a ticket to request a custom Twilio Verify template
Follow the prompts to submit a ticket. In the description of the support ticket make sure you include the following information:
- Twilio Account SID ( find here)
- Twilio Verify SID ( find or create here)
- Your template, including:
{{code}}
variable - required{{verification_id}}
variable or something that can be used as a user identifier in order to look up the user's phone number for theto
parameter in the /verification-check endpoint- The URL you created in the last step, e.g.
https://international-telephone-input-1234-abcxyz.twil.io/verify.html
. For compliance reasons, the link must be embedded into the template, it cannot be a variable.
Here's an example ticket description:
You can submit requests for multiple custom templates, we encourage at least two templates so you can have:
- One template for testing.
- One template for production with your branded URL.
Sending an SMS verification magic link
Once your template is approved, you can start sending magic links. You can complete the next few steps in preparation for your approved template, but won't be able to test end to end yet.
Head to the Twilio Console functions editor, select your Function Service, and add two environment variables (found under "Settings & More").
VERIFY_SERVICE_SID
. Starts with VA, find or create hereVERIFY_TEMPLATE_SID
. Starts withHJ
, will be in your completed Support ticket, or find here)
Add a new function to send verifications. Click Add + and select Add Function. Name your function verify/start
, set the visibility to Public, and add the following code:
This code uses the /verifications
endpoint to send an SMS with a few additional parameters:
-
templateSid
: your custom template SID, this can also be set as a default at the Verify Service level. -
templateCustomSubstitutions
: note that thecode
will be automatically populated by Twilio, so only provide other variables you want to include in the SMS. You will need some kind of identifier for the user, this example uses the phone number.
In assets/index.html
, update the script to call the /verify/start
endpoint instead of the existing phone number lookup endpoint. Replace everything from the start of function process…
until the closing </script>
tag with the following code:
Save and Deploy All and test sending a magic link. Click the three dots next to index.html
and select Copy URL to navigate to your application. Enter your phone number to test the application, you should receive an SMS with a link!
Verification Logic
Clicking the link won't validate anything yet, so add the verification logic next.
Add a new function to check verifications. Click Add + and select Add Function. Name your function verify/check
, set the visibility to Public, and add the following code:
Test the flow again, but this time when you click on the SMS link, you will be redirected to an approval screen:
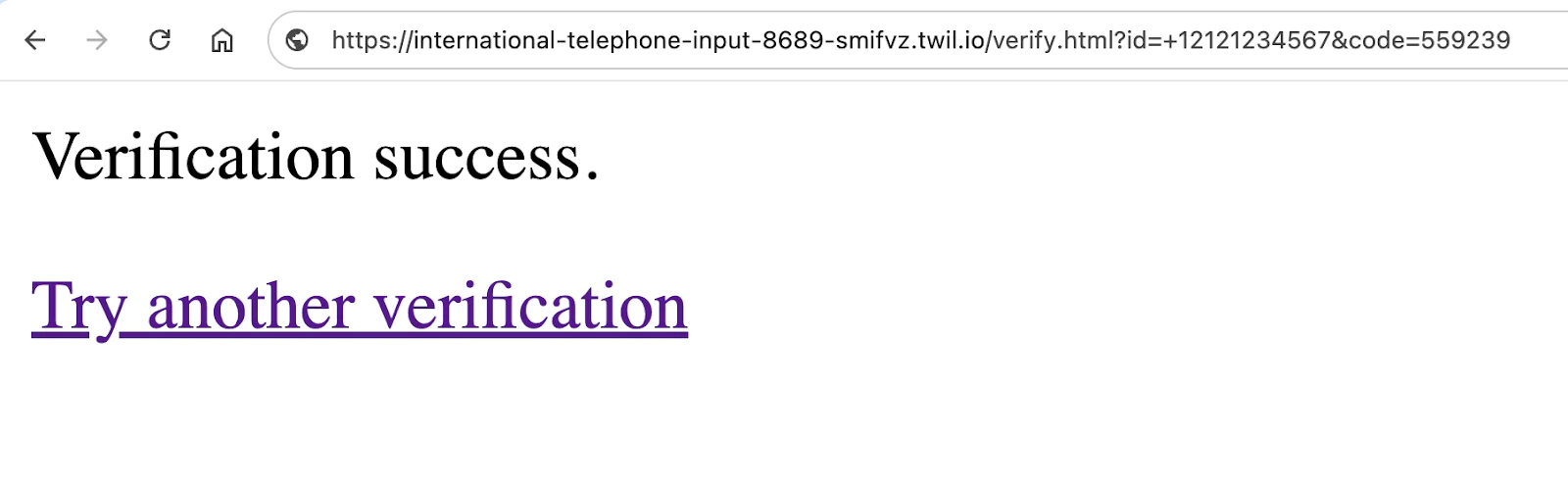
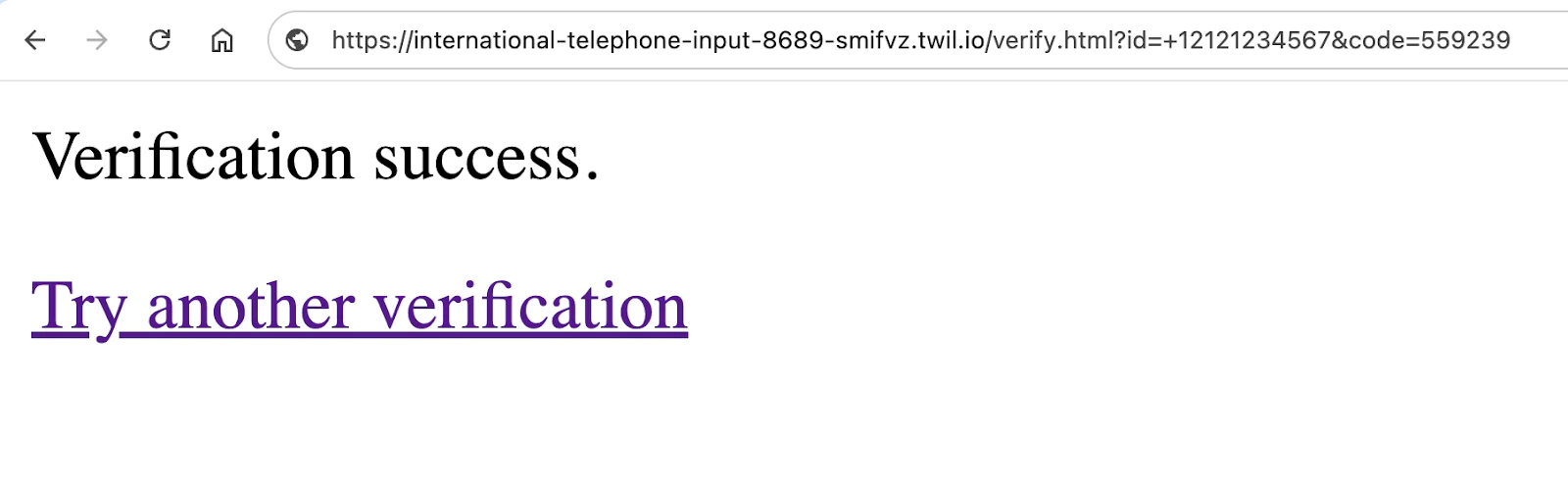
Built-in fraud prevention with Verify
This code uses the Verify API's stateless verification tracking to validate the OTP that was included in the request's query parameter. The Verify API includes several features for both better developer experience and stronger security and fraud prevention, including:
- OTP management, with single-use OTPs that will be invalid after an approved check.
- Automatic OTP timeout after 10 minutes (timeout is configurable).
Next steps for user verification with magic links
At this point you would mark the user as verified for whatever purpose you're checking. How you manage that in your application will vary, for example:
- For phone number verification, mark the user's number as verified in your database
- For login, set a session cookie or use a callback to indicate verification success.
Note that the user may start and check the verification on two different devices. Keep that in mind as you determine how to move forward.
Magic links provide a user-friendly way to quickly validate phone numbers for authentication or authorization. For more verification resources, best practices, and examples, check out the following:
- Verify API documentation
- Email magic link verification
- How to test Verify without getting rate limited
I can't wait to see what you build and secure!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.