How to Send Daily SMS Reminders in PHP 7 Laravel Web Apps with cron
Time to read: 4 minutes
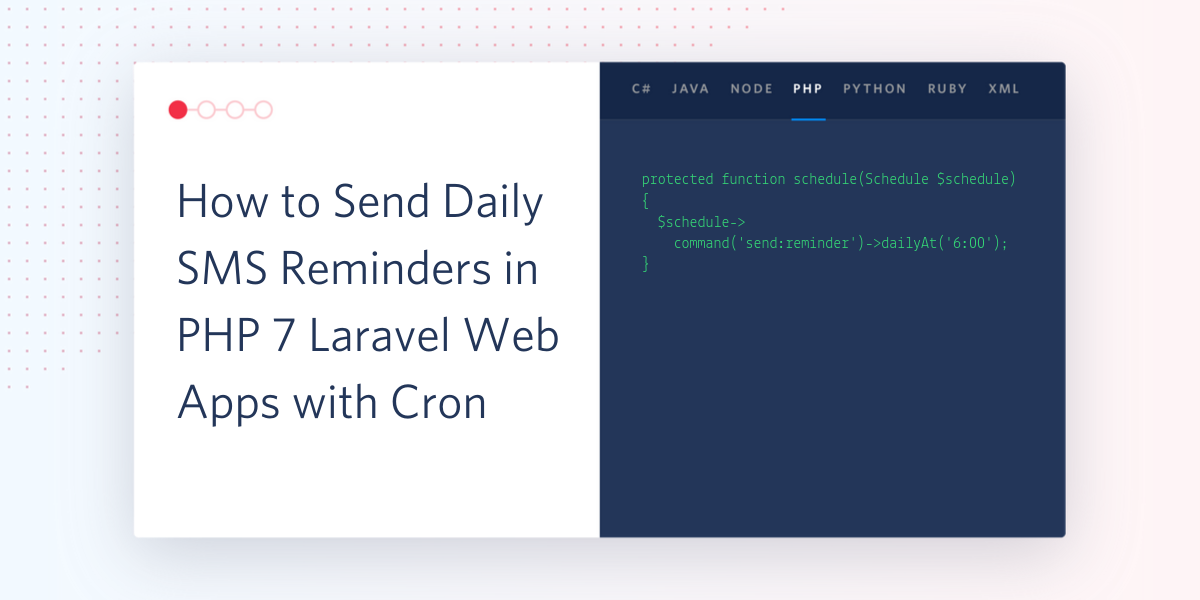
Sending and receiving SMS is easy with the Twilio API, but what if you want to send regularly-scheduled reminders from your Laravel web applications? In this tutorial, we'll combine Laravel, Twilio Programmable SMS and cron to send daily reminders that are stored in a Laravel data model.
Project Dependencies
We'll need the following dependencies to complete this tutorial:
- PHP version 7+
- Composer installed globally
- Your Twilio credentials, which you can sign up for an account for free
We can start building our project once you have PHP 7 and Composer installed. We'll sign up for a Twilio account at the appropriate step in the tutorial.
All of the code for the project can be found within this Git repository on GitHub.
Create a Laravel Project with Composer and the Twilio Helper Library
In your terminal, start a new Laravel project named reminder-app
using the following command:
You should see a bunch of standard Composer output that ends with the following three lines:
Change into the reminder-app
directory:
Install the Twilio PHP helper library. This library will help us to connect to the Twilio API directly from our application.
When Composer is finished installing the Twilio PHP helper library package you should see output similar to this:
Next, we will sign up for Twilio and populate a few environment variables.
Obtaining Our Twilio Credentials
Head over to twilio.com and sign up for an account if you do not already have one. After the registration process, take a look at your account credentials on the Twilio Console.
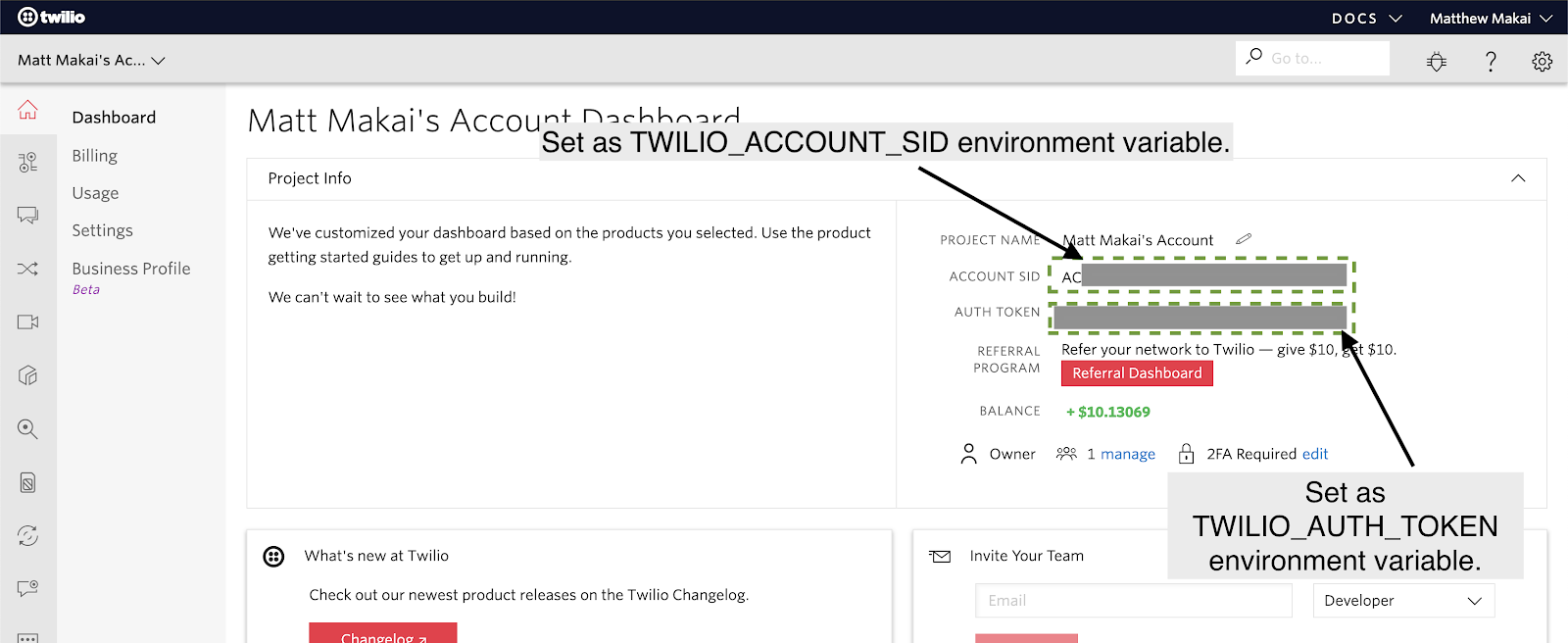
Use the phone number that comes with your trial account or purchase a new phone number to use for this project. Set the Twilio phone number as the TWILIO_PHONE_NUMBER
environment variable and the phone number you want to send the reminder to as TO_NUMBER
.
It's time to write some PHP code for our reminders application.
Creating the Laravel Model with Artisan
Run the following command to create the model to store our reminders:
You should see Model created successfully.
as the output. The command created a new file named Reminder.php
within the app
directory.
Open the app/Reminder.php
file and add the following new method to the class.
The above sendSMS
method imports your Twilio credentials from the environment variables TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
. It then uses them to instantiate the Twilio PHP helper library client and send an SMS to the phone number specified in the TWILIO_PHONE_NUMBER
environment variable.
Next we need a method that calls sendSMS
and specifies the phone number to send the reminder to, as well as what message to send. Add the following highlighted lines to app/Reminder.php
.
The above code only has a single number listed under the recipients
variable, but you can add as many as you would like to the array. The code loops through each element in the array and invokes the sendSMS
method with the number that needs the reminder and the message to send.
We need a way to test our code and easily call it with a command on the command line. Make sure app/Reminder.php
is saved, then go back to the base directory of your project. Use the following artisan
command to generate the boilerplate code to create a new Laravel command for this project:
The above code created a new file under the app/Console/Commands/
directory named sendReminders.php
. Open that file and add the following highlighted lines.
The new lines of code call the app/Reminder.php
code that we previously wrote. Let's give our application a try.
Testing Our Reminders Laravel App
Make sure the app/Console/Commands/sendReminders.php
file is saved, then execute this artisan
command.
You should see:
And on the phone with the number you set as your TO_NUMBER
:
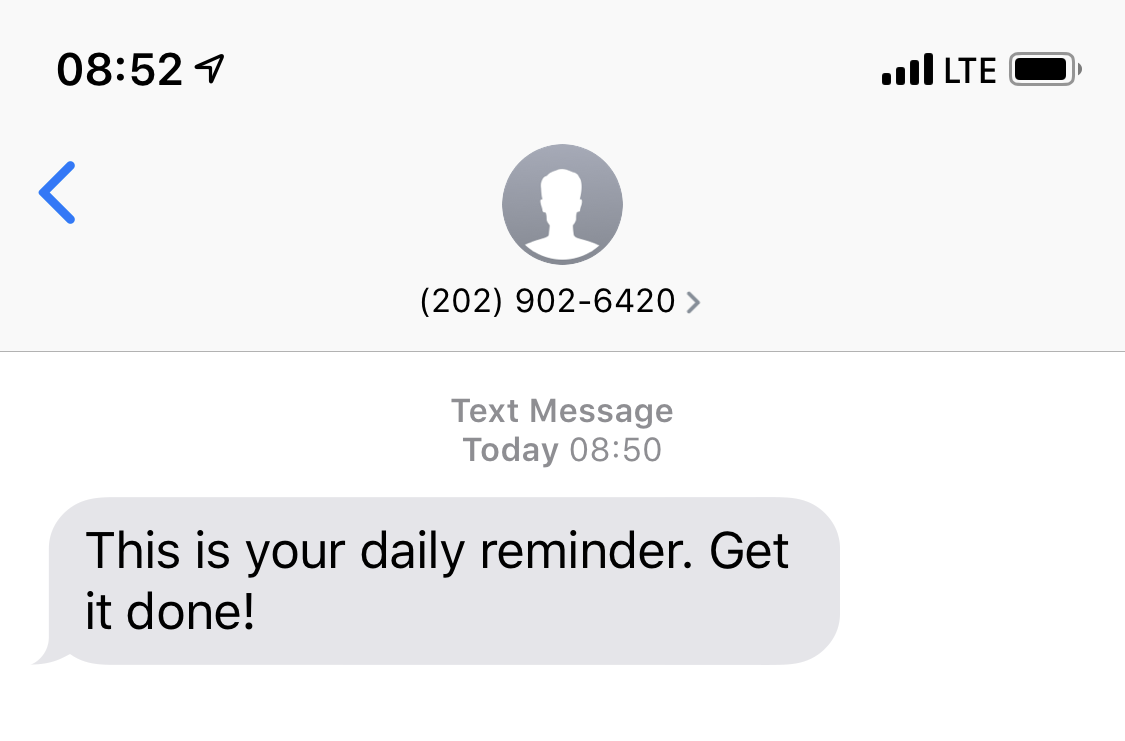
That'll do it. The code works! We just need a cron job to kick off the reminder each day.
Sending Daily SMS Reminders with PHP and Chronos (cron)
We’ll need a bit more PHP, as well as a cron job to execute our code on a regular basis so the messages are sent daily. If you've never used cron before, this tutorial is great for understanding the basics.
Open the app/Console/Kernel.php
file and update it with the following highlighted lines of code:
The above line of code schedules the send:reminder
command to run each day at 6am, but we still need a cron job to initiate the command schedule. Add a new command to cron with this command:
The cron configuration file will open in your default text editor. Now add this line to the configuration:
This cron job will call the Laravel scheduler and run the tasks that are due for execution.
Wrapping up Our PHP Reminders Application
We just created an application to send daily text messages with one or more reminders of our choice. The web app is built with Laravel which minimized the amount of custom code we needed to get the job done.
That is it for this tutorial, but there are many ways to expand your application. Next, you should view Twilio's PHP Voices page, which contains the latest and greatest PHP tutorials we’ve published.
Here are a few great tutorials on there that you can build next or add to this reminders app:
- How to Initiate a Voice Call from Laravel PHP with Twilio Programmable Voice by Ugendu Ositadinma
- Send Inspirational Quotes Every Morning in PHP Using Cron, SendGrid, Twilio SMS and Quote APIs by Michael Jaroya
- What's New in Laravel 6: Ignition and Flare by Gary Hockin
That's all for now, happy coding and we can't wait to see what you build next!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.