Send Branded SMS Messages Using Twilio Alphanumeric Sender ID
Time to read: 3 minutes
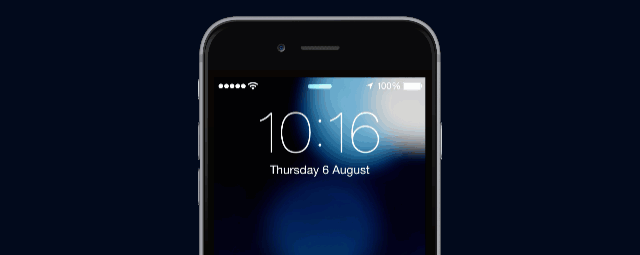
It’s been a few months since we hosted Signal and in this post I wanted to spend some time exploring one of the announcements that I found pretty exciting: alphanumeric sender ID’s. Being based in the UK and traveling often in Europe I regularly receive SMS messages with a business name as the sender ID instead of a phone number. This is great as I instantly recognize the name of the business sending me the message instead of seeing an incoming message from an unknown number.
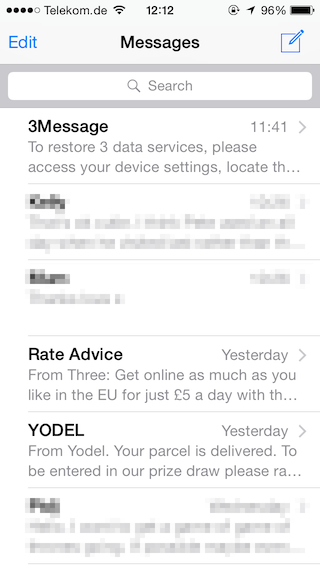
A word of warning
Before we dive into the code, there are a few things you should know about alphanumeric sender IDs. This style is great for alert or broadcast type messages, but no use for interactive applications as users cannot respond to an alphanumeric sender. As such, if you choose to use an alphanumeric sender ID you will need to provide alternative ways for users to opt out. You should also think carefully about the sender ID that you choose. Your business name or brand is fine, but if you try to get too creative things can really backfire.
Ready to start sending branded SMS messages? Let’s get on with it then.
Today’s toolbox
I’m going to demonstrate sending an SMS message from an alphanumeric ID using Ruby, so if you want to follow along, you’ll need the following:
- A Twilio account (to use alphanumeric sender IDs, you will need to upgrade your account and contact Twilio support to enable your account)
- A Twilio phone number (you don’t need this to send from an alphanumeric sender ID, but you’ll see why you need it soon)
- Ruby and the Twilio Ruby gem ( gem install twilio-ruby)
and that’s it, so let’s get on with sending some SMS messages!
Sending SMS messages
You may have seen how to send an SMS message from a phone number before, but here’s a quick recap:
You can save that to a file and run it or just type it straight into irb, either way it will just work. Pretty straightforward, right? How about sending an SMS message from an alphanumeric sender ID then?
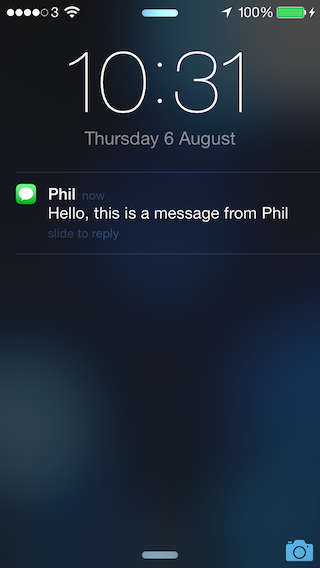
145 countries is not all all the countries
Well, ok, it’s that simple only if you are sending SMS messages to one of the 145 countries that support this feature. If you are sending to multiple countries and one of them, like the US, doesn’t support alphanumeric sender IDs then there is a bit more work to do.
Send a message using an alphanumeric sender ID to a number in a country that doesn’t support it and the Twilio API will respond with an HTTP 400 response. The body of the response will contain the Twilio specific error details, including a Twilio error code and error message. The error code that represents our problem, sending an SMS message from an alphanumeric sender ID to a country that doesn’t support it, is Twilio error code 21612.
The HTTP response will manifest itself in the Ruby library as a Twilio::REST::RequestError. We can rescue the exception and query the error for the code to check it is the error we expect by making sure it has code 21612. If it is we can retry sending the message using a Twilio number as the sender. Here’s how that would work:
You can now try this script against a number from a country that doesn’t support these messages, like the US, Japan or Belgium. Once the API responds with the error the script will fallback to using the supplied phone number as the sender.
If other errors are raised they will cause this script to crash and you should handle those errors in an appropriate manner for your application.
Brand those SMS messages
There we go, you can now:
- Improve the user experience for SMS alerts and broadcasts by branding your SMS message sender
- Fallback successfully in cases where the receiver is in a country that won’t accept an alphanumeric sender ID
Remember that using an alphanumeric sender ID is not appropriate in all possible uses of SMS as recipients cannot respond and in cases where you can use this feature it is important to provide alternative ways for users to opt out of these messages.
As always, if you have any further questions about this drop a comment below, give me a shout on Twitter or shoot me an email.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.