How to Send an RCS Message with Twilio and C# .NET
Time to read: 5 minutes
With the advent of Rich Communication Services (RCS), businesses are leveling up how they engage their customers. RCS brings a modern messaging experience that mirrors the interactive features of messaging apps that today’s consumers are accustomed to, while still delivering the reliability of SMS.
Apple devices also now support RCS as of the release of iOS 18. This has opened the door to new opportunities for integrating RCS.
In this tutorial, we’ll show you how to get started with RCS using Twilio and C# with .NET.
Comparing RCS to SMS and WhatsApp
SMS is simple and sticky, meeting end users where they’re at. RCS enhances SMS by tacking on advanced features similar to those of modern messaging apps like WhatsApp. This brings a rich and interactive messaging experience to the consumer, and they don’t need to download a new app to get it. With RCS, businesses can leverage multimedia, verified sender profiles, and comprehensive engagement tracking support.
To see RCS in comparison with other messaging channels, see our Guide to Rich Communication Services: What Developers Need to Know.
Implementing RCS with Twilio and C# with .NET
If you’re ready to start sending messages via RCS, then Twilio is the place to get started. We’ll walk you through how to create an RCS sender and a messaging service. Then, we’ll show you how to send your first RCS message using C#.
Prerequisites
To get started, you will need the following:
- A Twilio account (sign up here).
- A Twilio phone number for sending SMS, as a fallback to your RCS service.
- A device capable of receiving RCS messages.
- The .NET framework installed on your development machine.
Step 1: Create an RCS Sender
1. Log in to the Twilio Console.
2. Navigate to the Explore Products section and click RCS.
• If RCS isn’t visible, you may need to request access here.
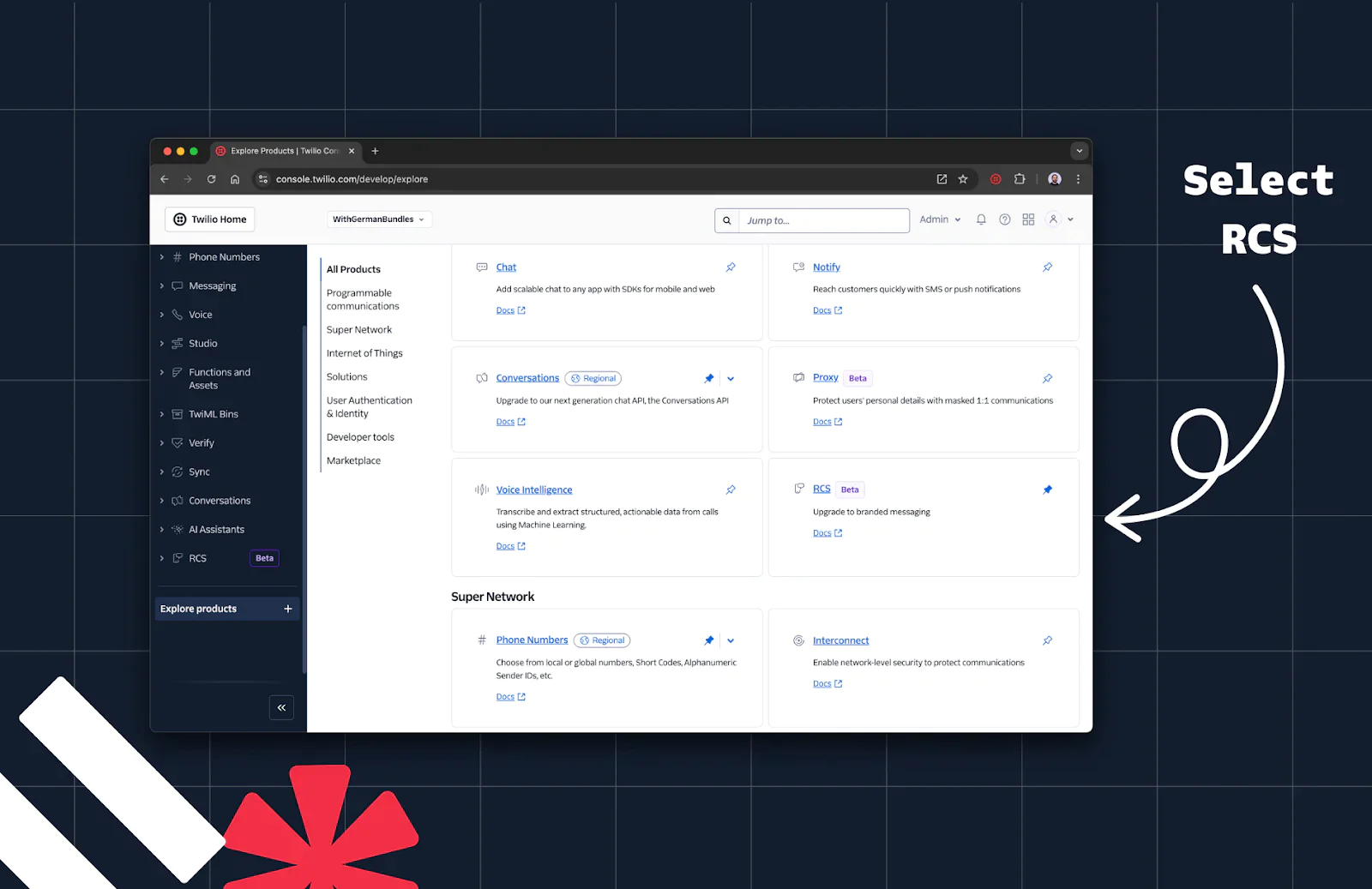
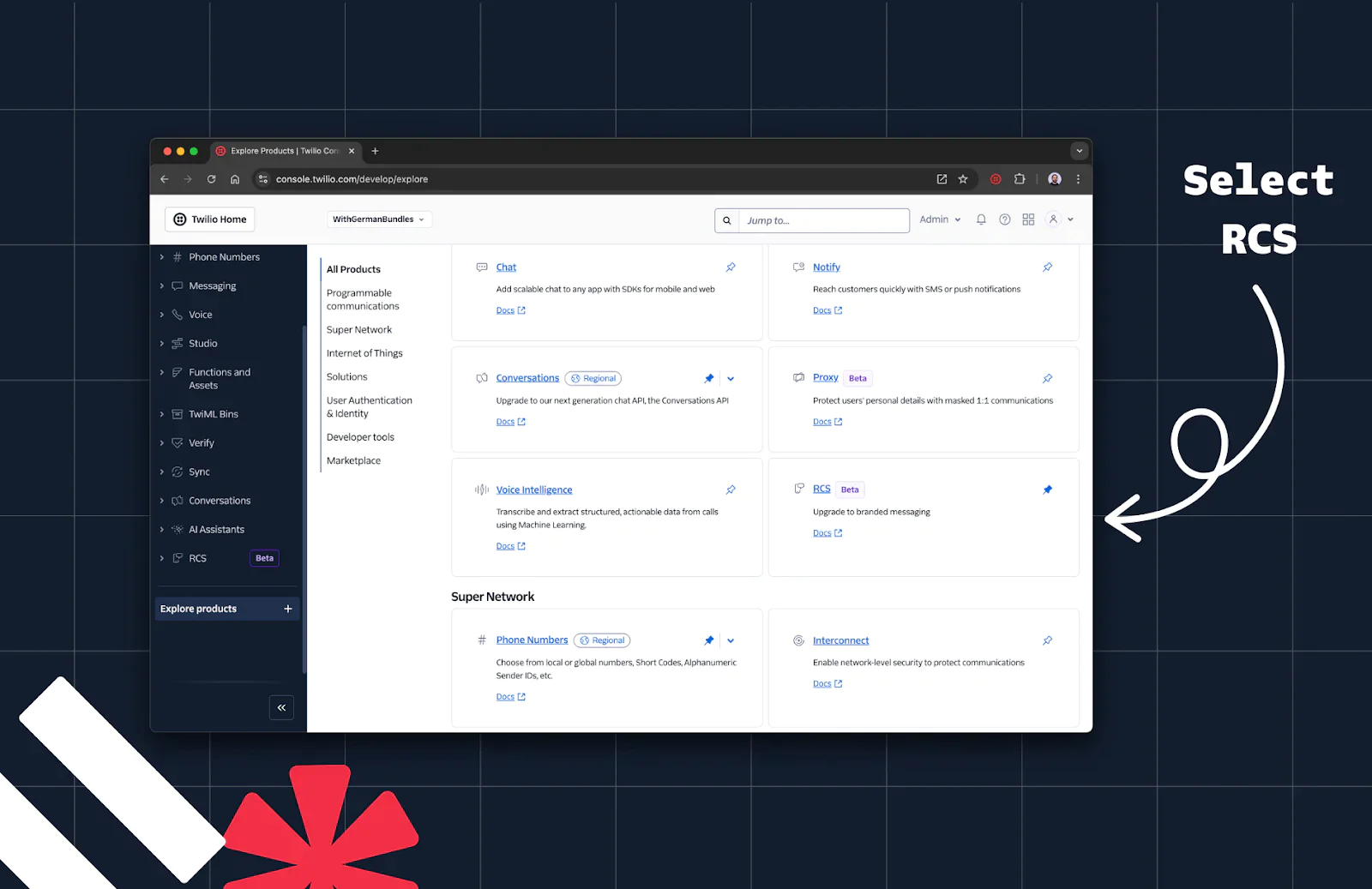
3. In the side panel, navigate to the Senders section.
4. Click Create new sender.
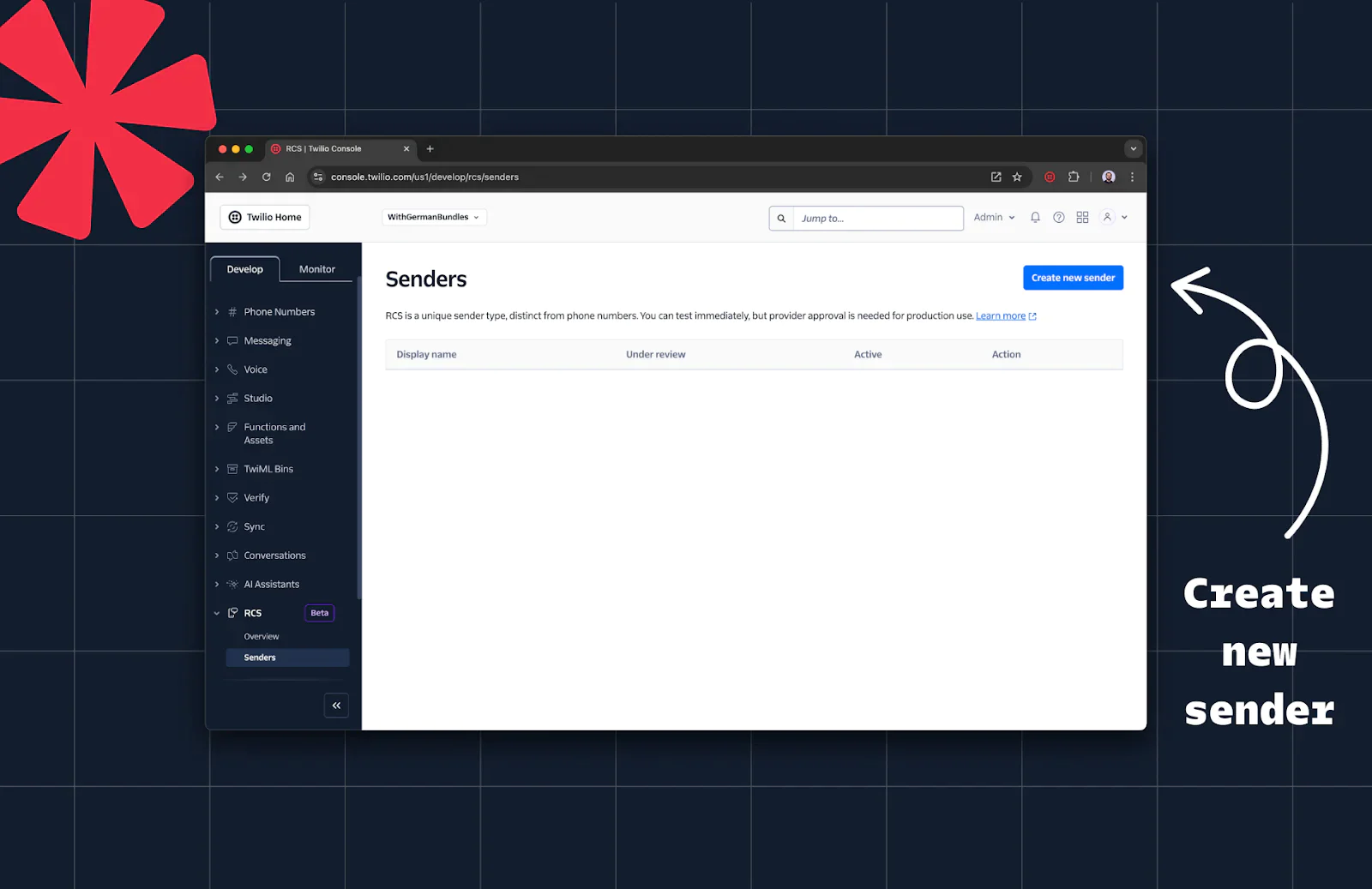
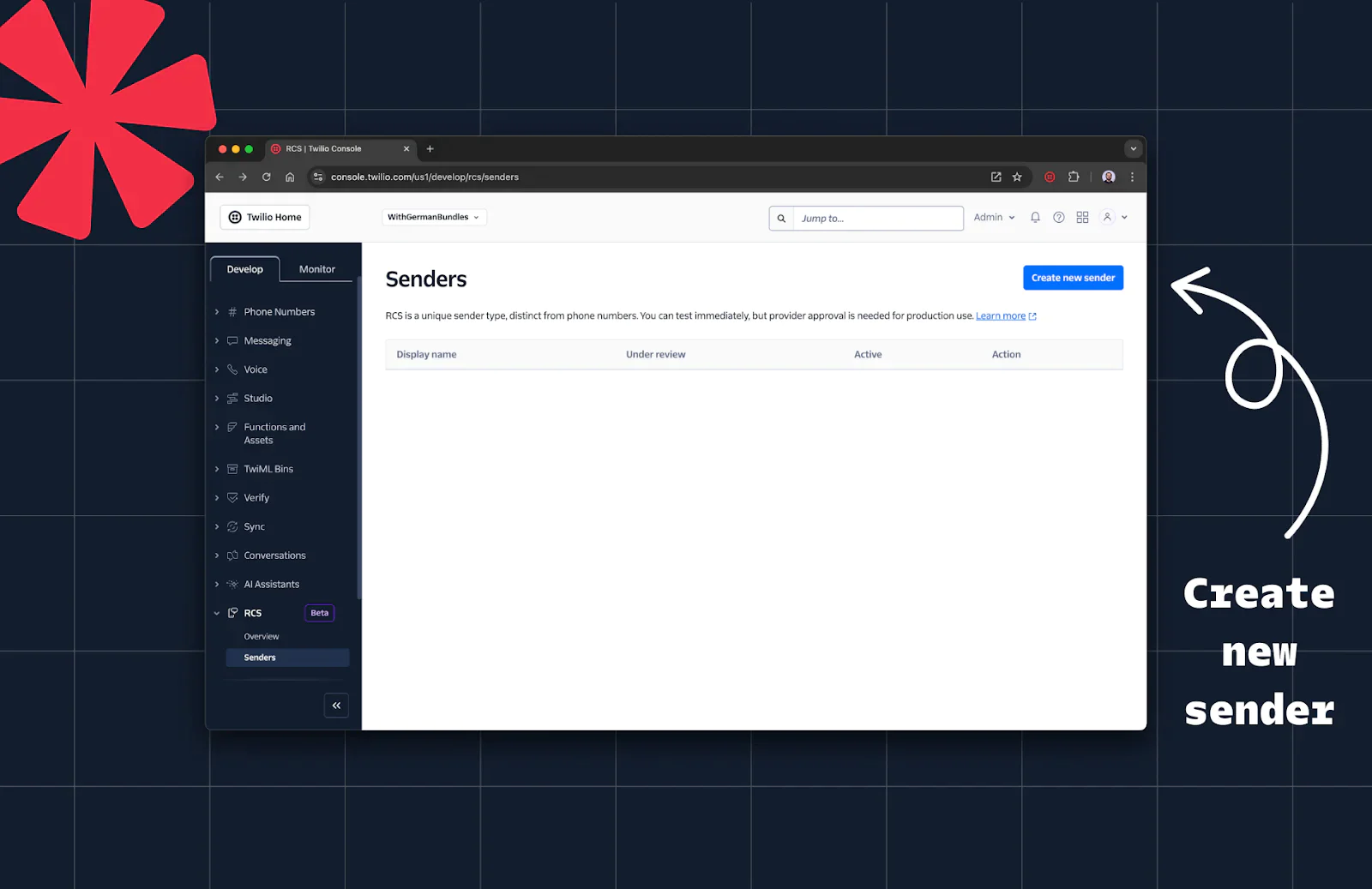
5. Provide a name (for example: “My First RCS Sender”). Click the continue button.
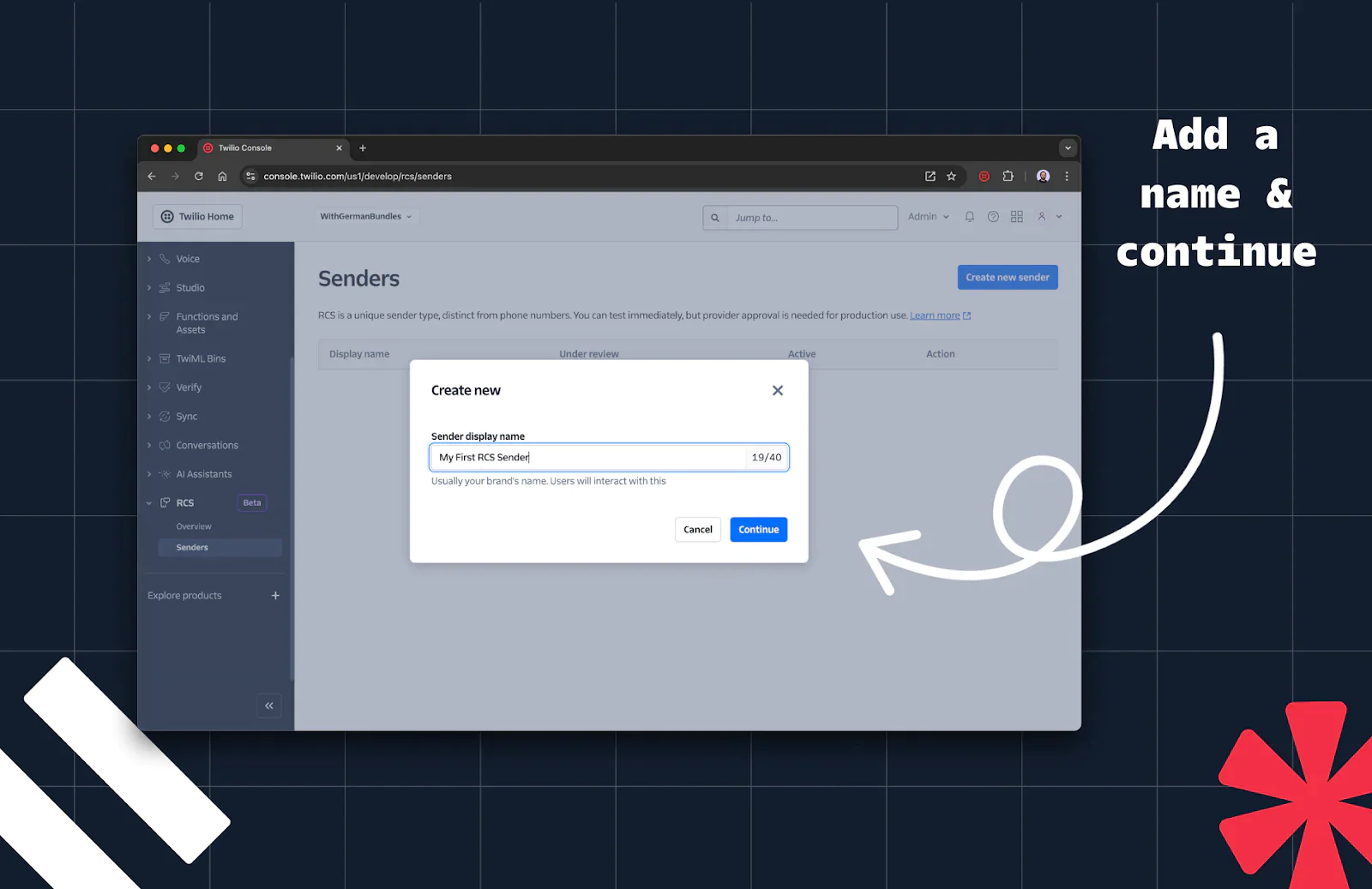
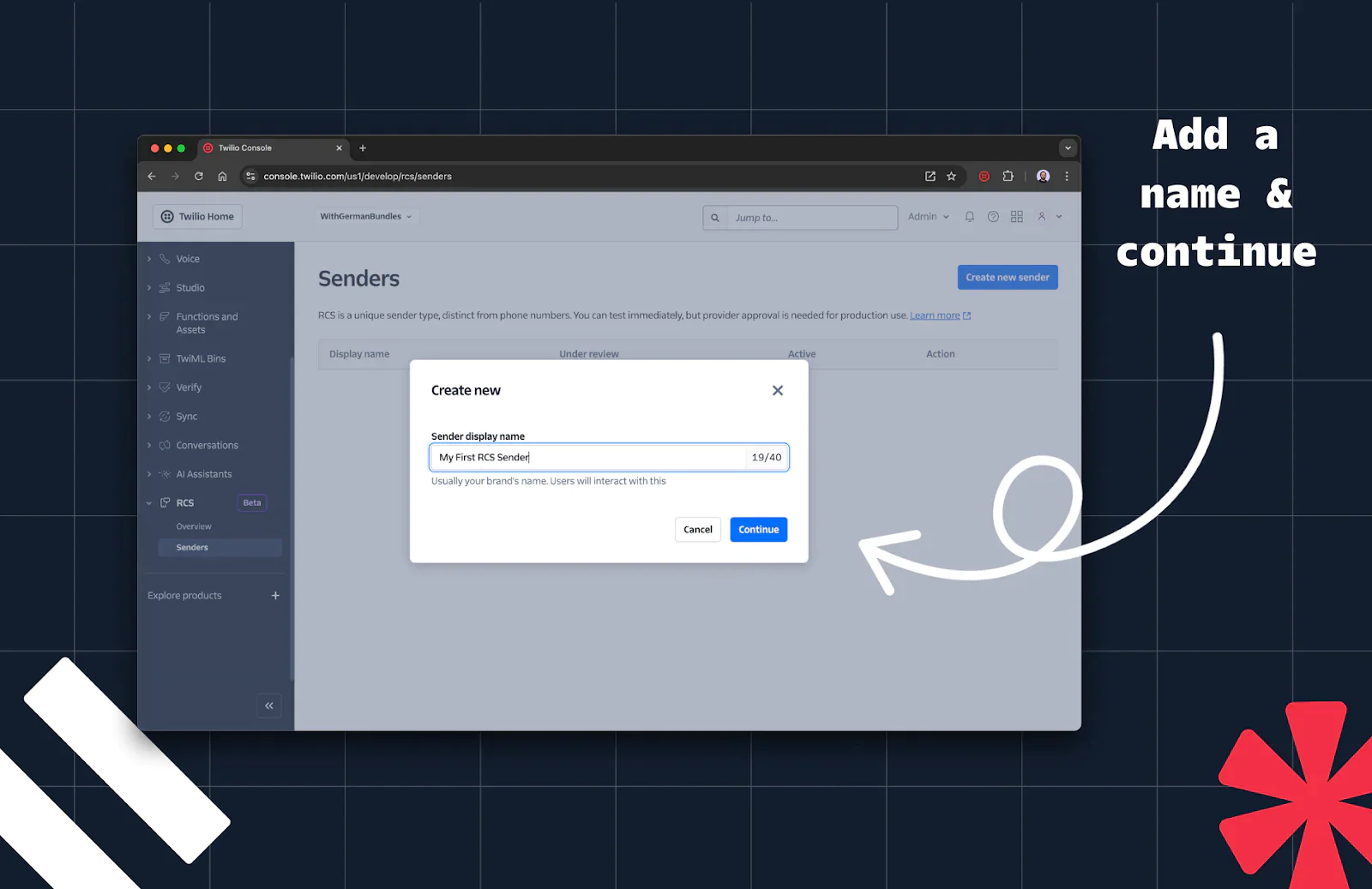
Step 2: Add Profile Information
Next, you’ll add profile information for your RCS sender. Use the following:
- Sender display name: Choose a recognizable name for your sender. Usually, this is your brand name. For our example, we will use “My First RCS Sender”.
- Description: Describe your brand. This description will be displayed just under your display name at the top of your user’s RCS interactions.
- Border color: Keep in mind the minimum contrast ratio. For testing purposes, start with
#000000
to keep it simple. - Logo: Your brand logo should meet the following requirements:
• Dimensions: 224x224 pixels
• File size: Max 50 KB
• Image type: JPEG/JPG/PNG
• For testing, you can use this example image:https://corn-lobster-7338.twil.io/assets/twiliodevs-logo-rcs.png
- Banner: Your brand banner should meet the following requirements:
• Dimensions: 1440x448 pixels
• File size: Max 200KB
• Image type: JPEG/JPG/PNG
• For testing, you can use this example image:https://corn-lobster-7338.twil.io/assets/twiliodevs-banner-rcs.png
- Contact Details - Phone number: A phone number associated with your RCS sender is required. Optionally, you can also add an email address.
- Link to privacy policy and Link to terms of service: For testing purposes, you can enter links to arbitrary information here. When you begin building for production usage, make sure to create an RCS sender with real information about your business.
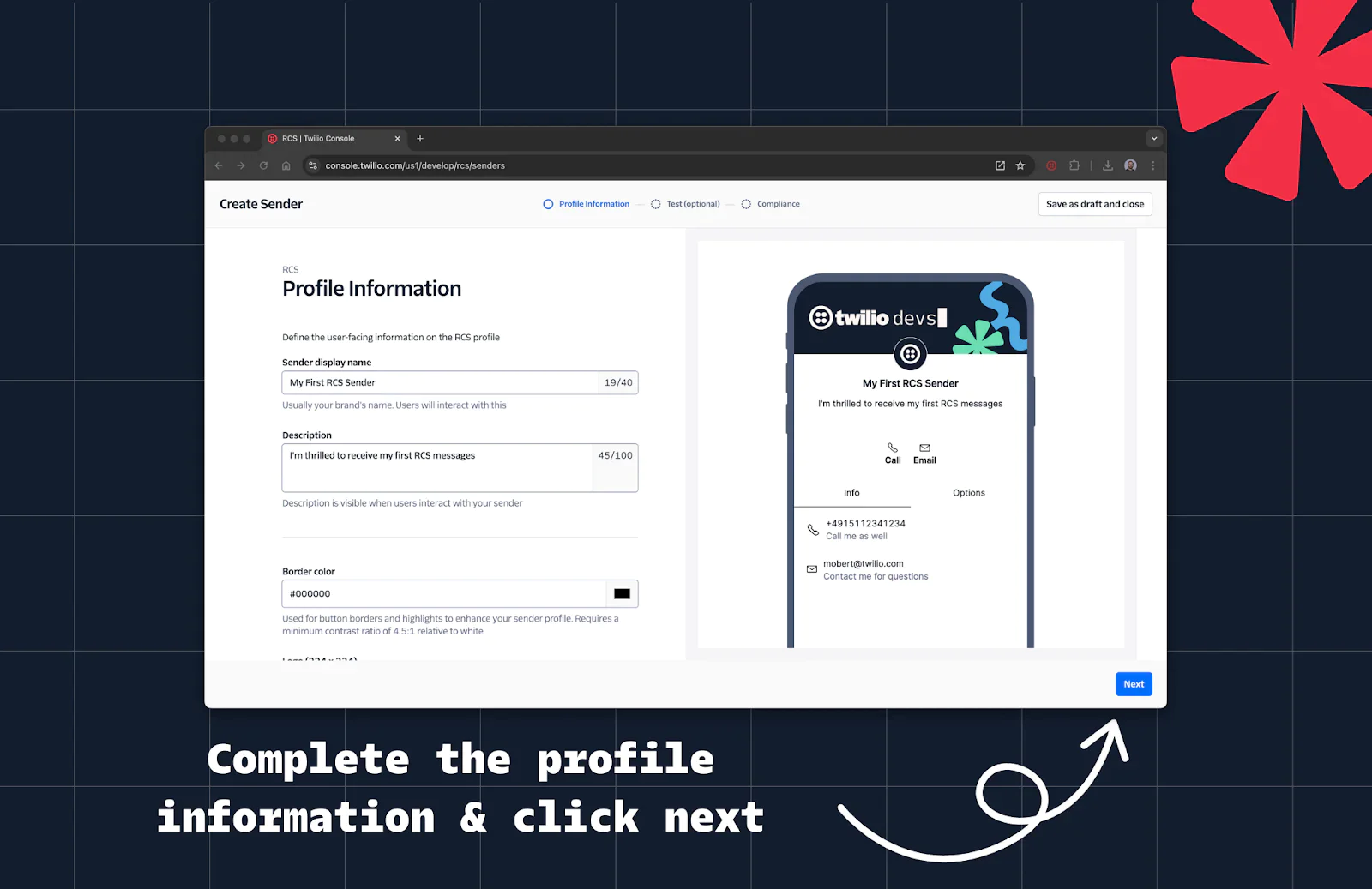
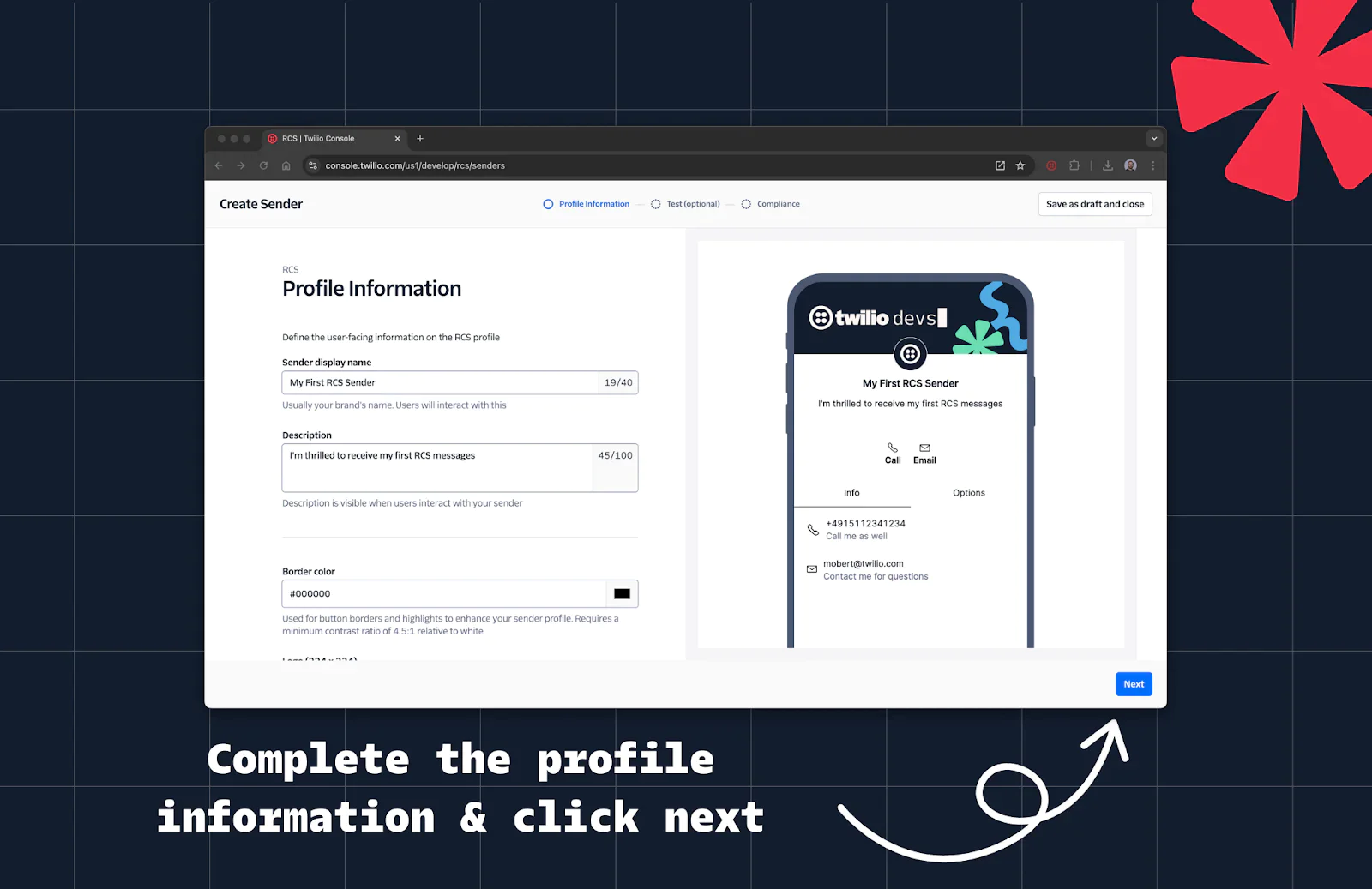
Step 3: Test Your RCS Sender
- On the optional Test tab, click Add device to test this sender.
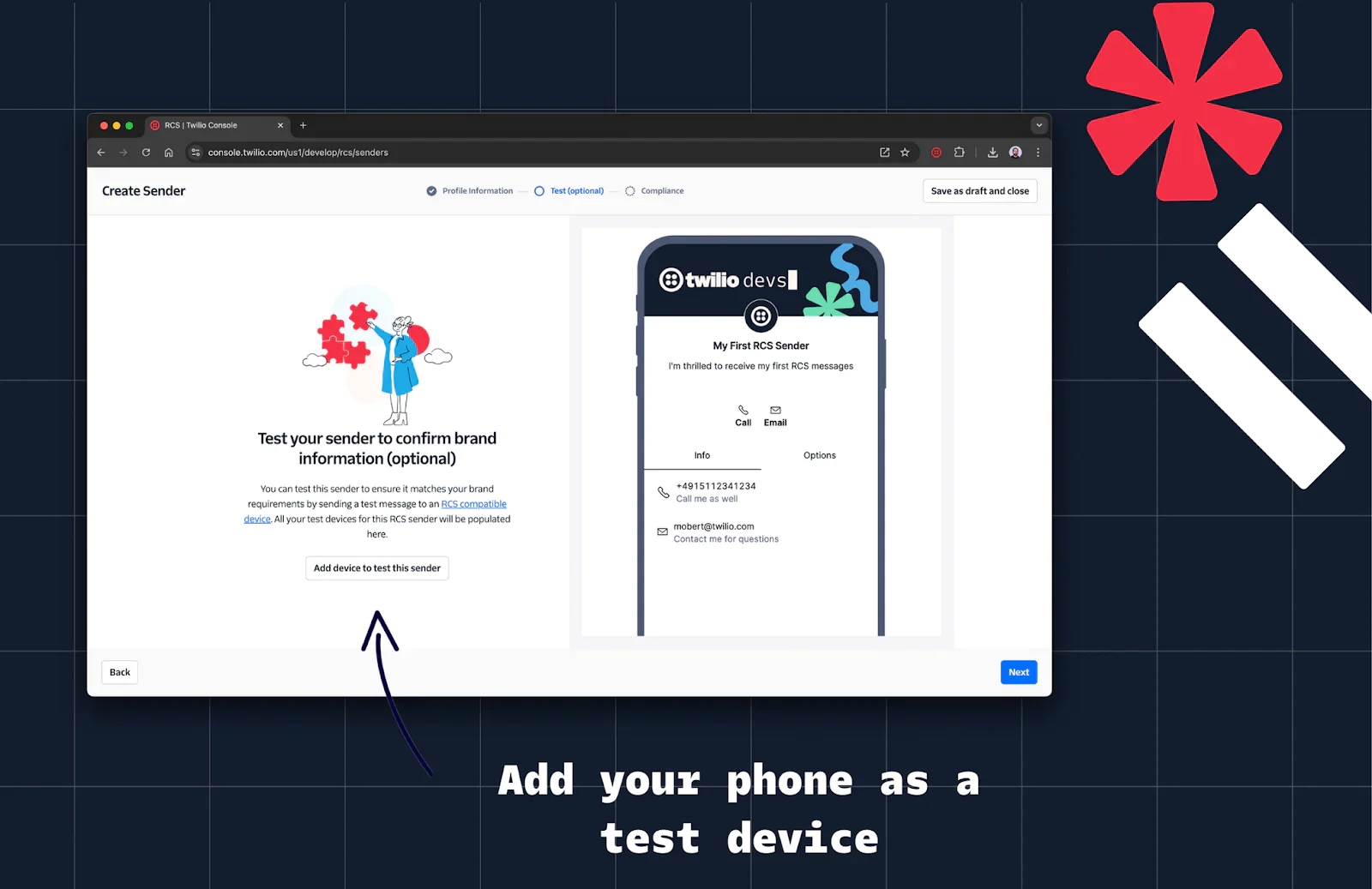
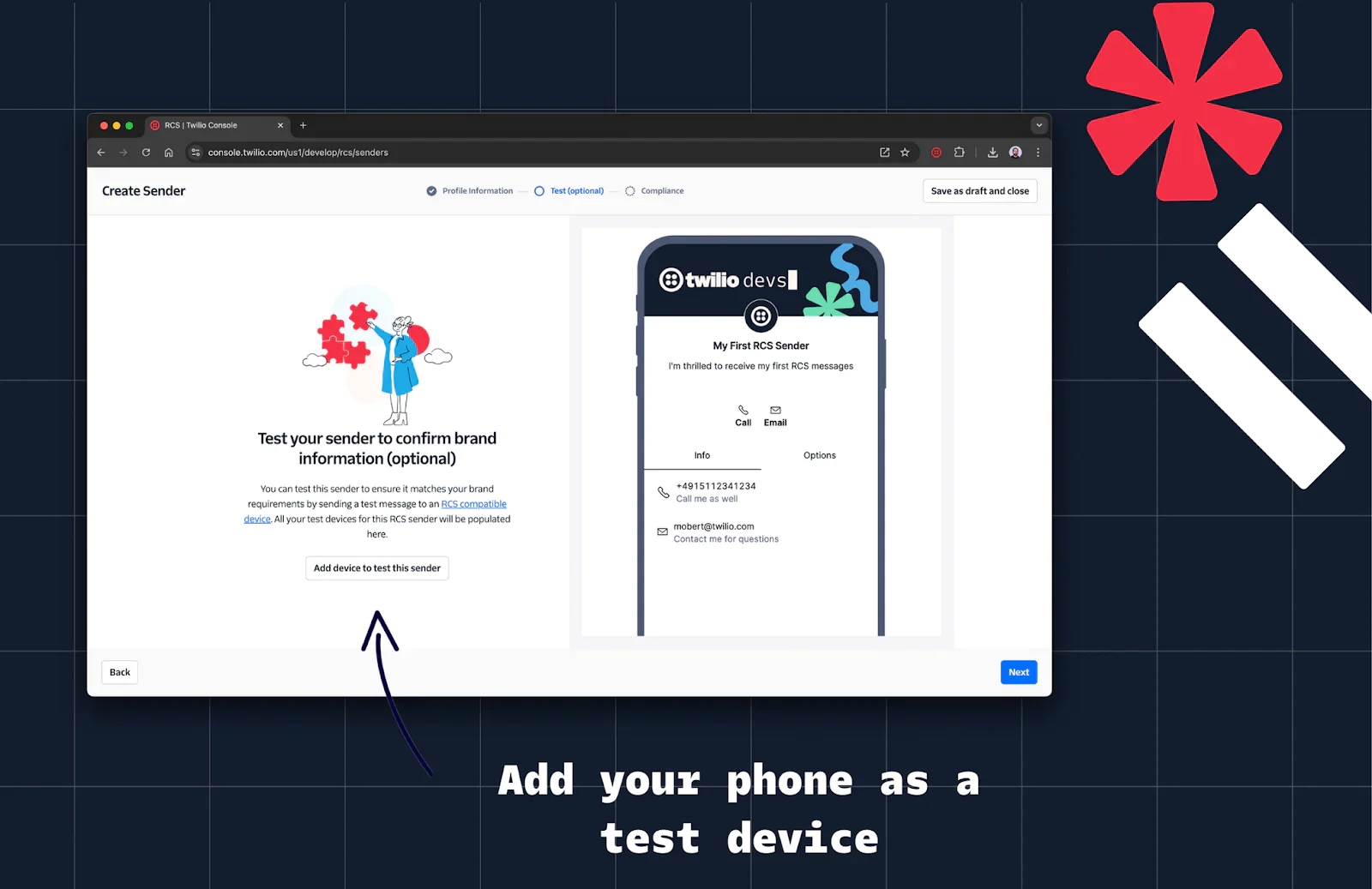
- Enter the phone number of your RCS-capable device and click Invite.
- You should receive an opt-in message from Twilio’s RCS agent on your device.
- Confirm the opt-in on your device by selecting Make me a tester.
- Check the dialog box in the Twilio Console, and send a test message.
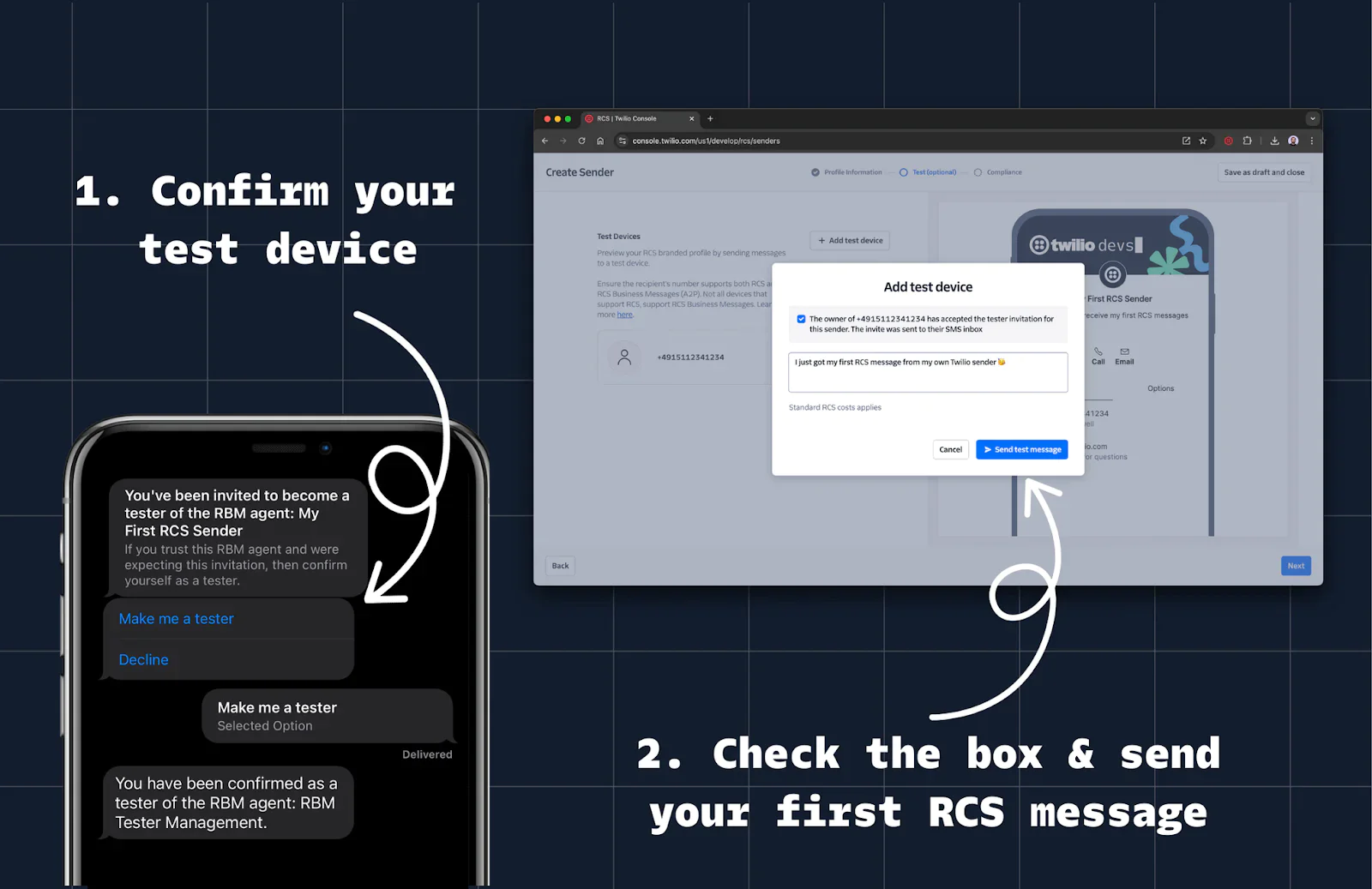
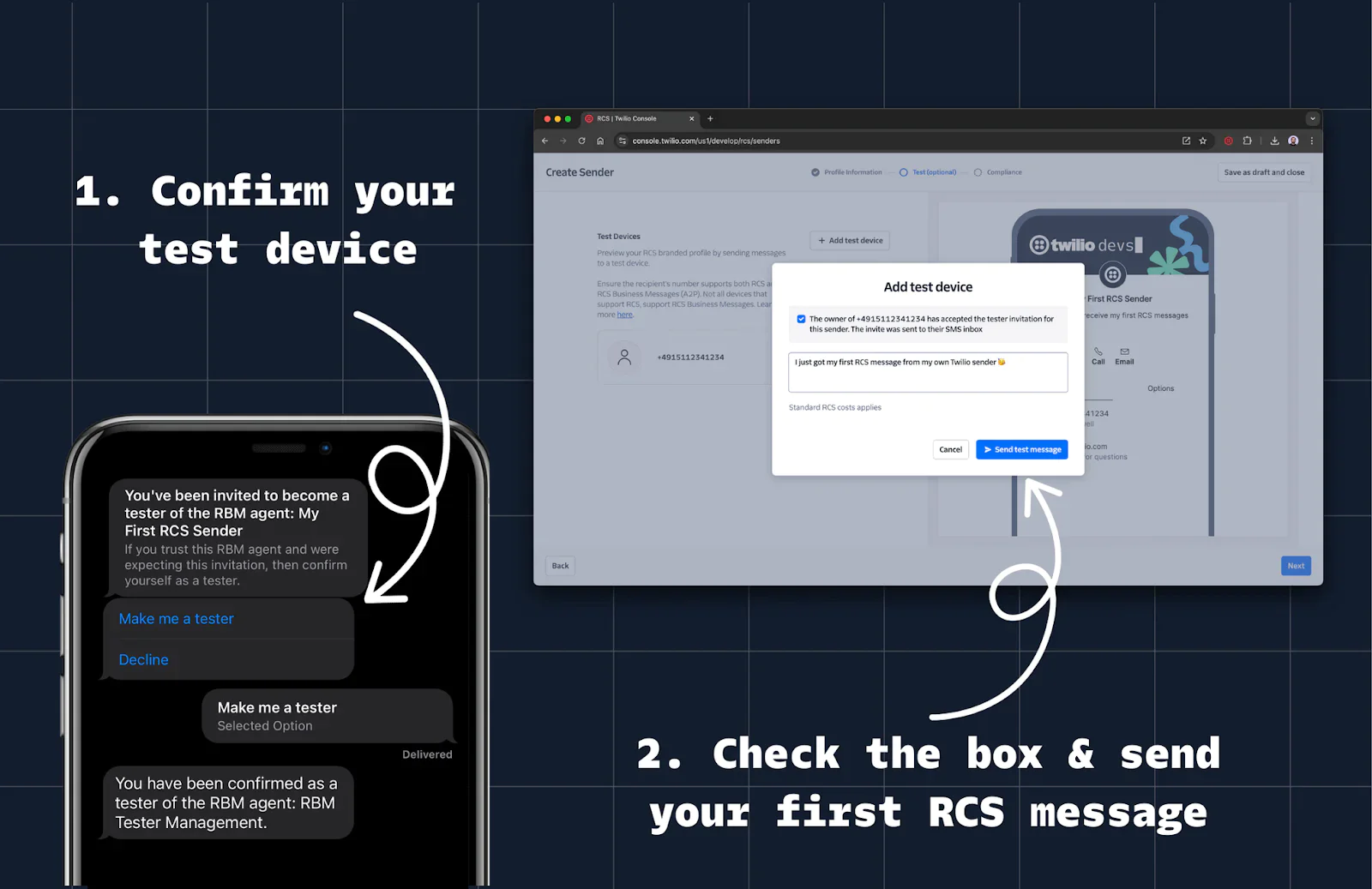
- Celebrate—you’ve just sent your first RCS message!
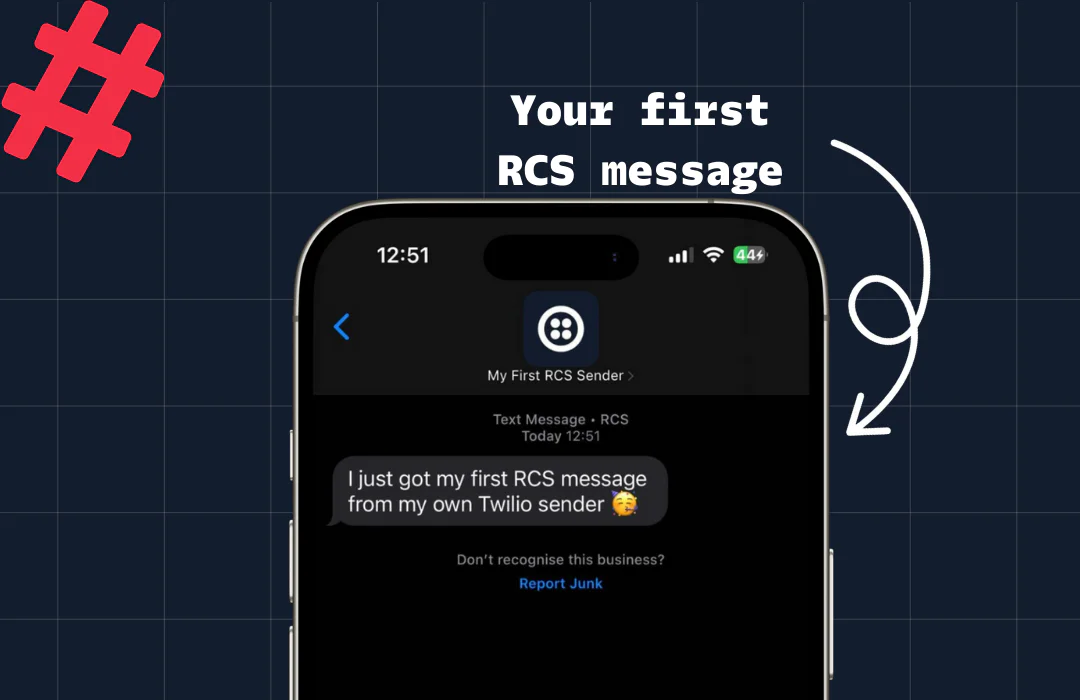
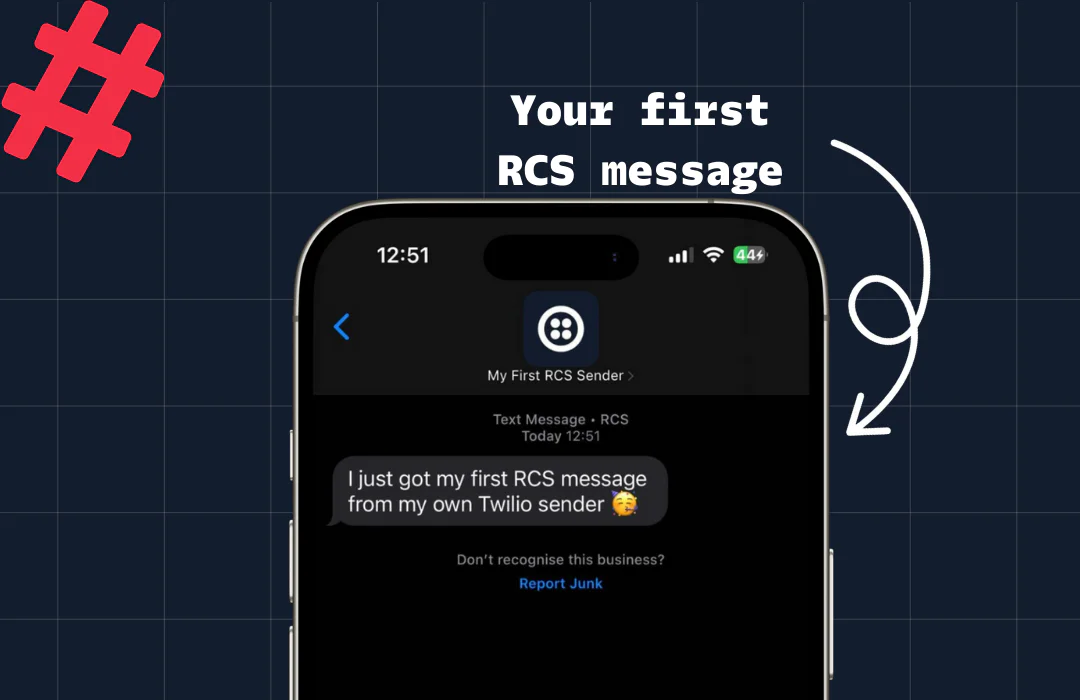
- Since this is just a test sender, click Save as draft and close.
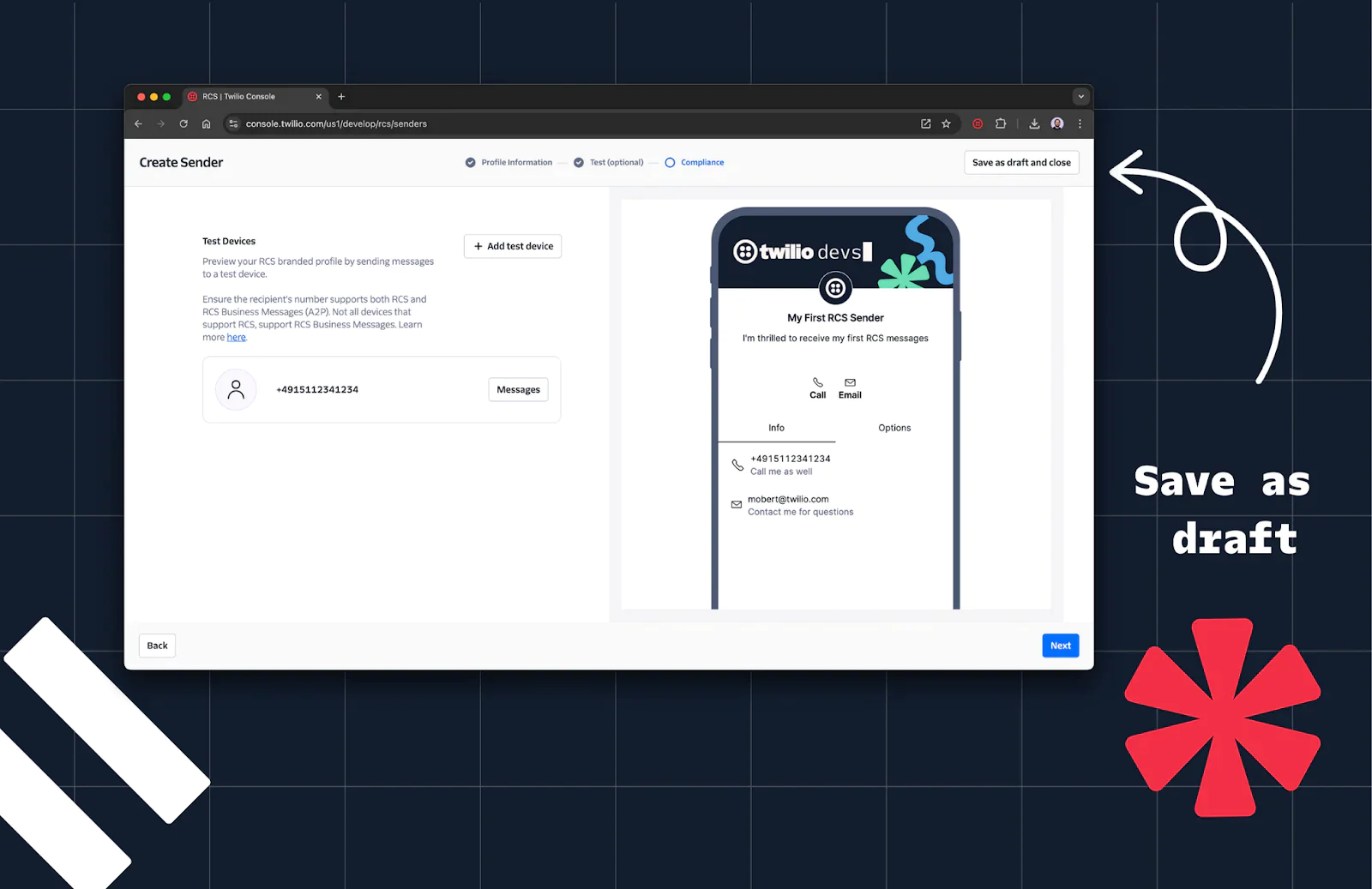
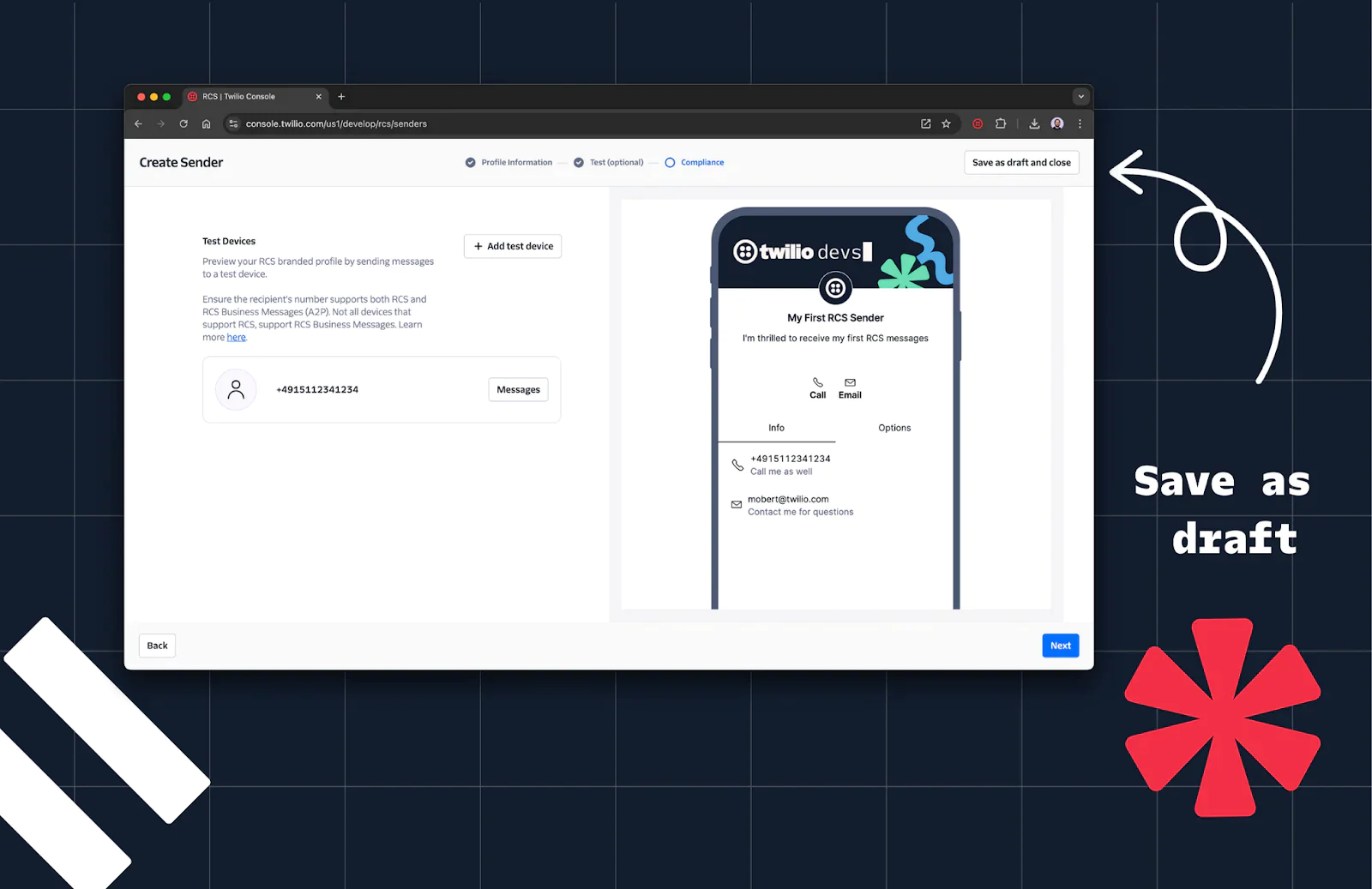
Step 4: Create a Messaging Service
- In the Console, navigate to Messaging > Services.
- Click Create Messaging Service.
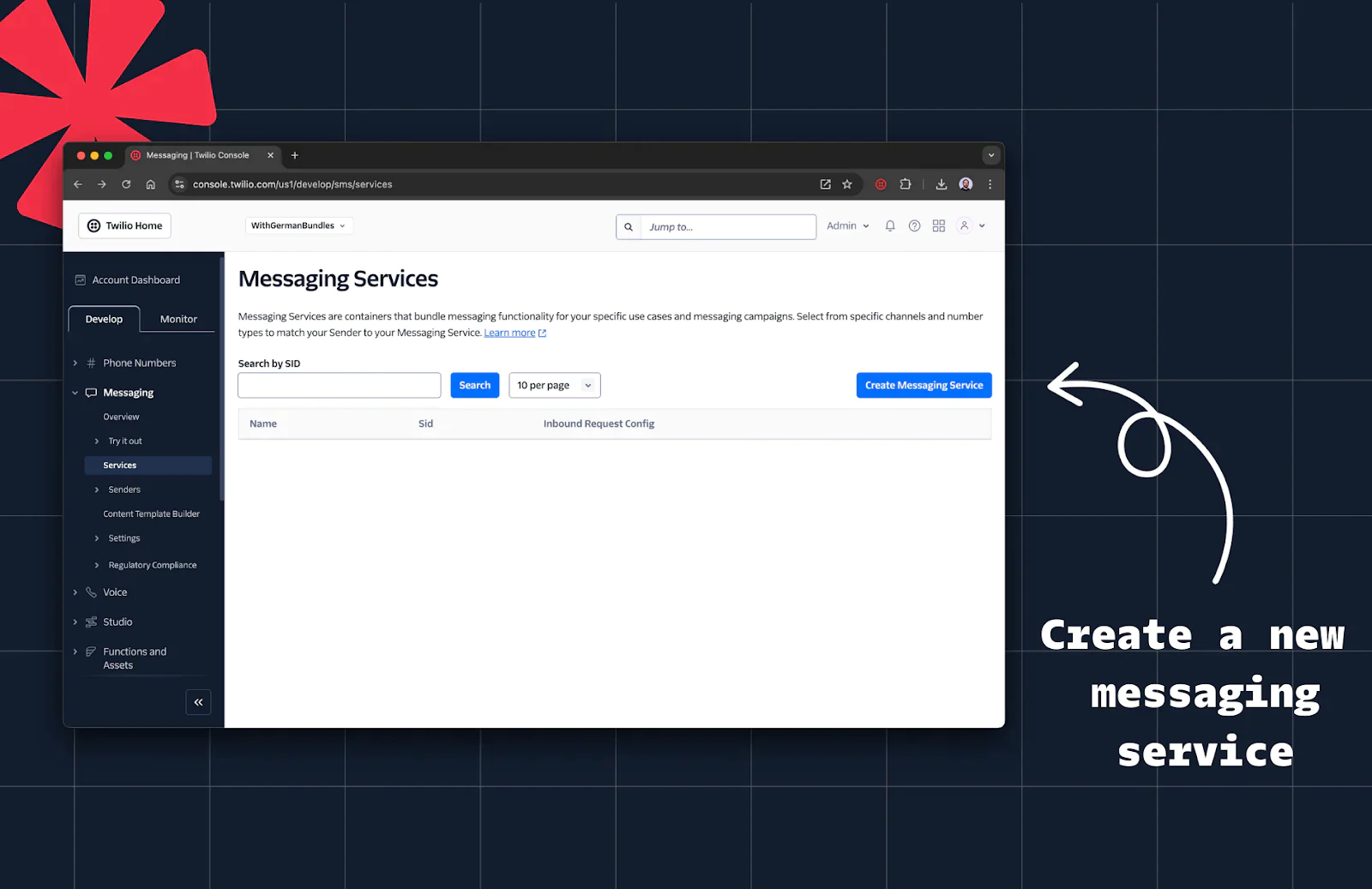
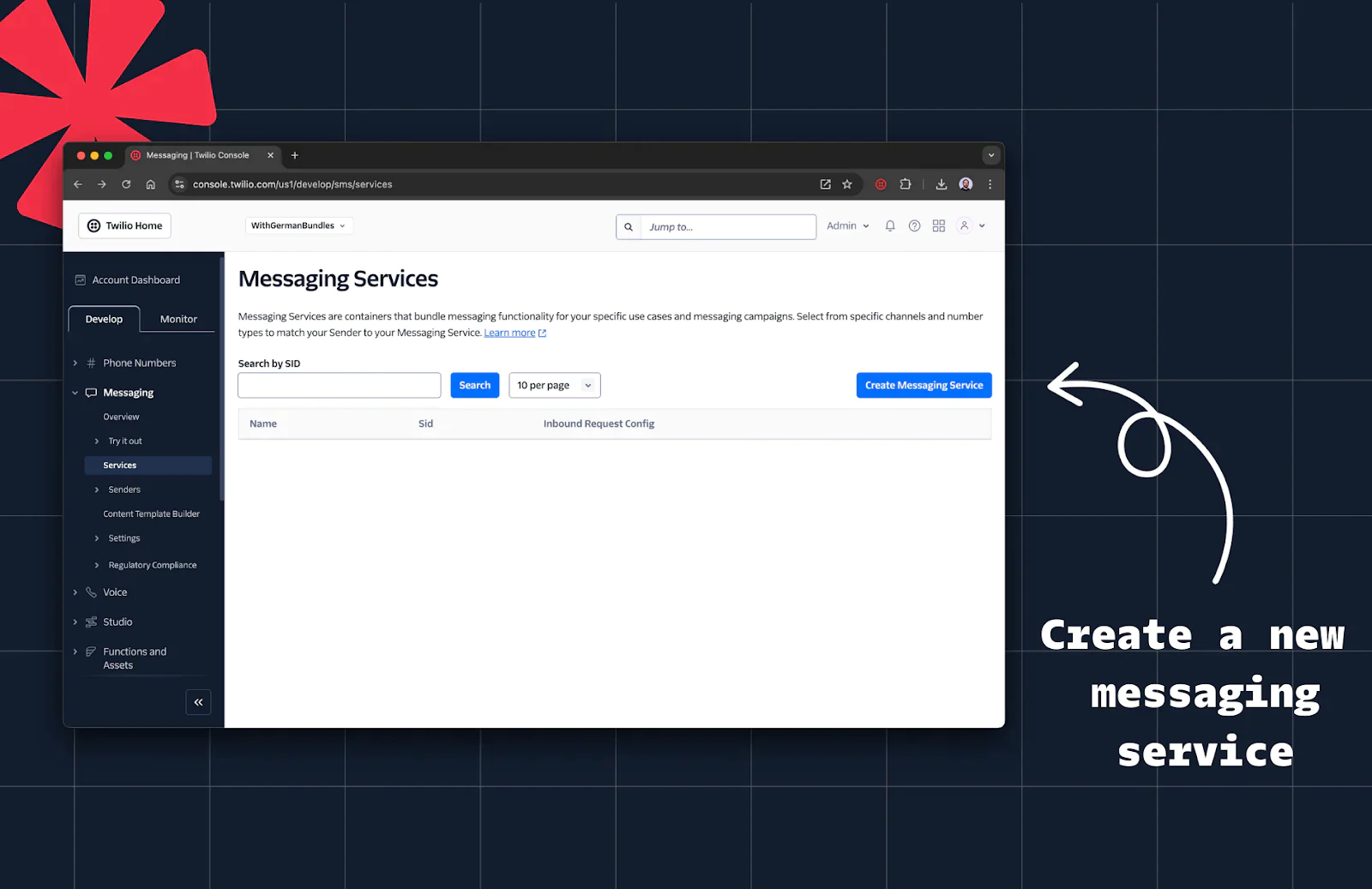
- Give the new service a name (for example: “My RCS Service”) and click Create Messaging Service.
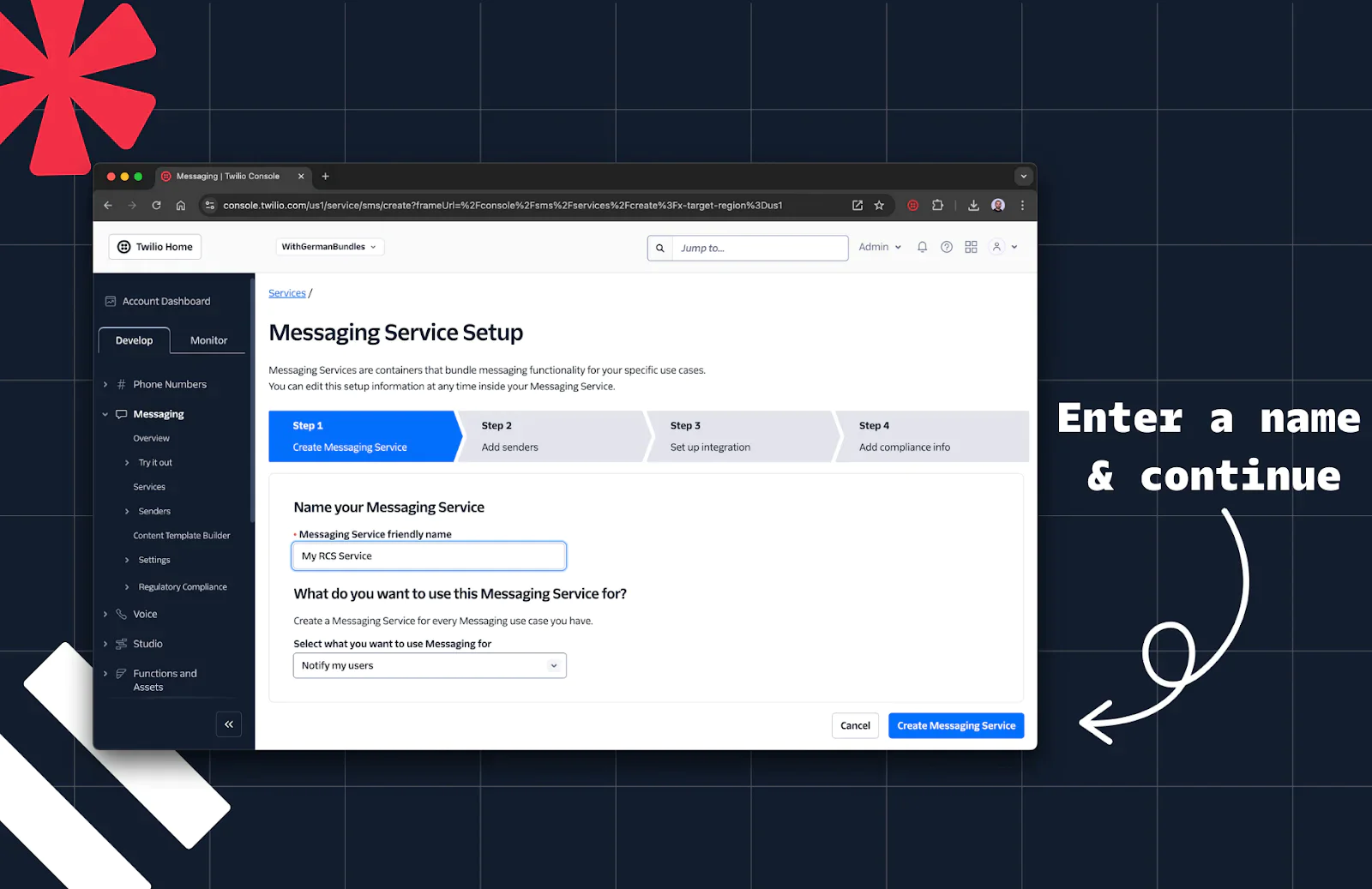
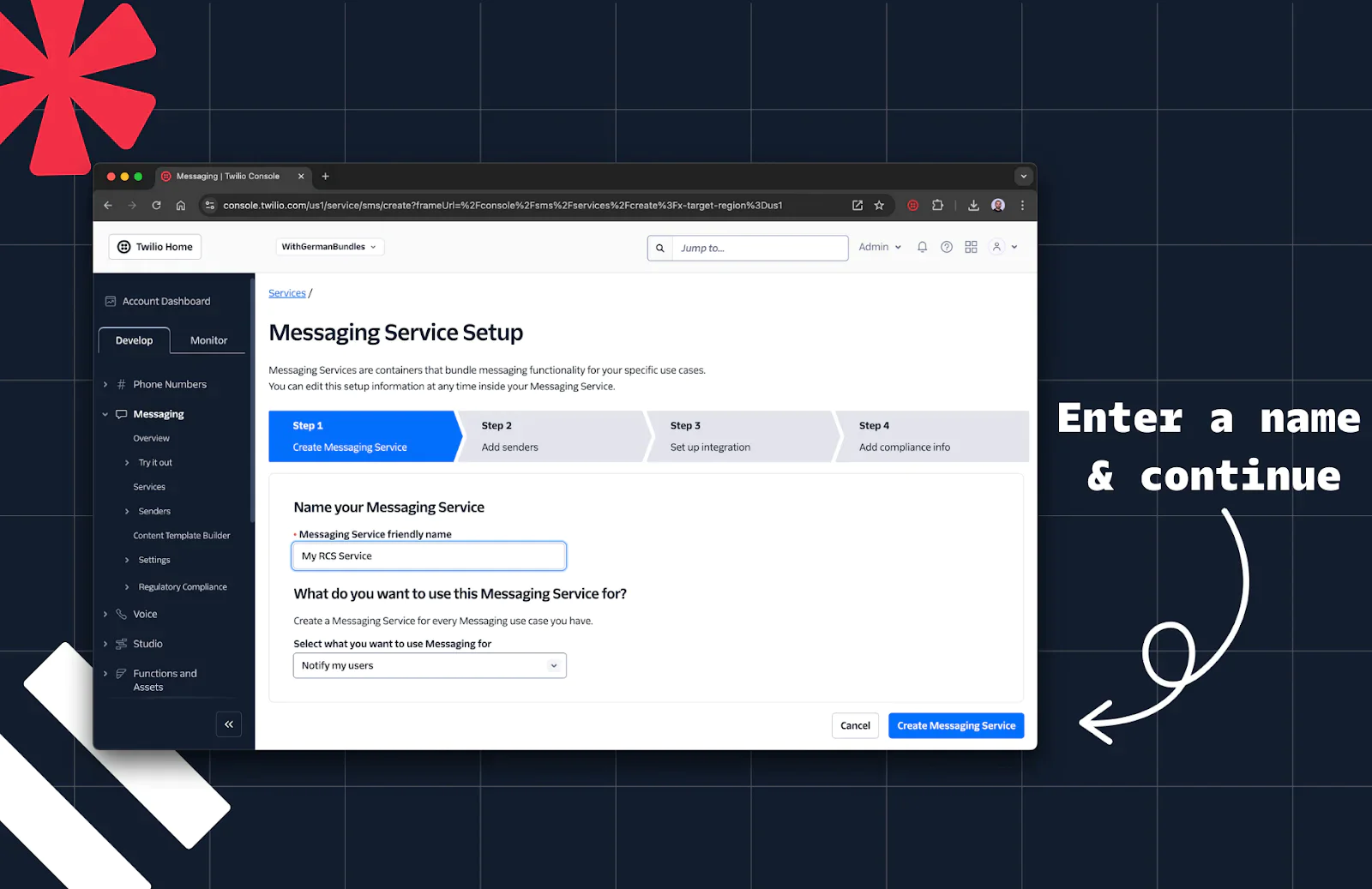
- Add your RCS sender.
- Click Add Sender, select RCS Sender.
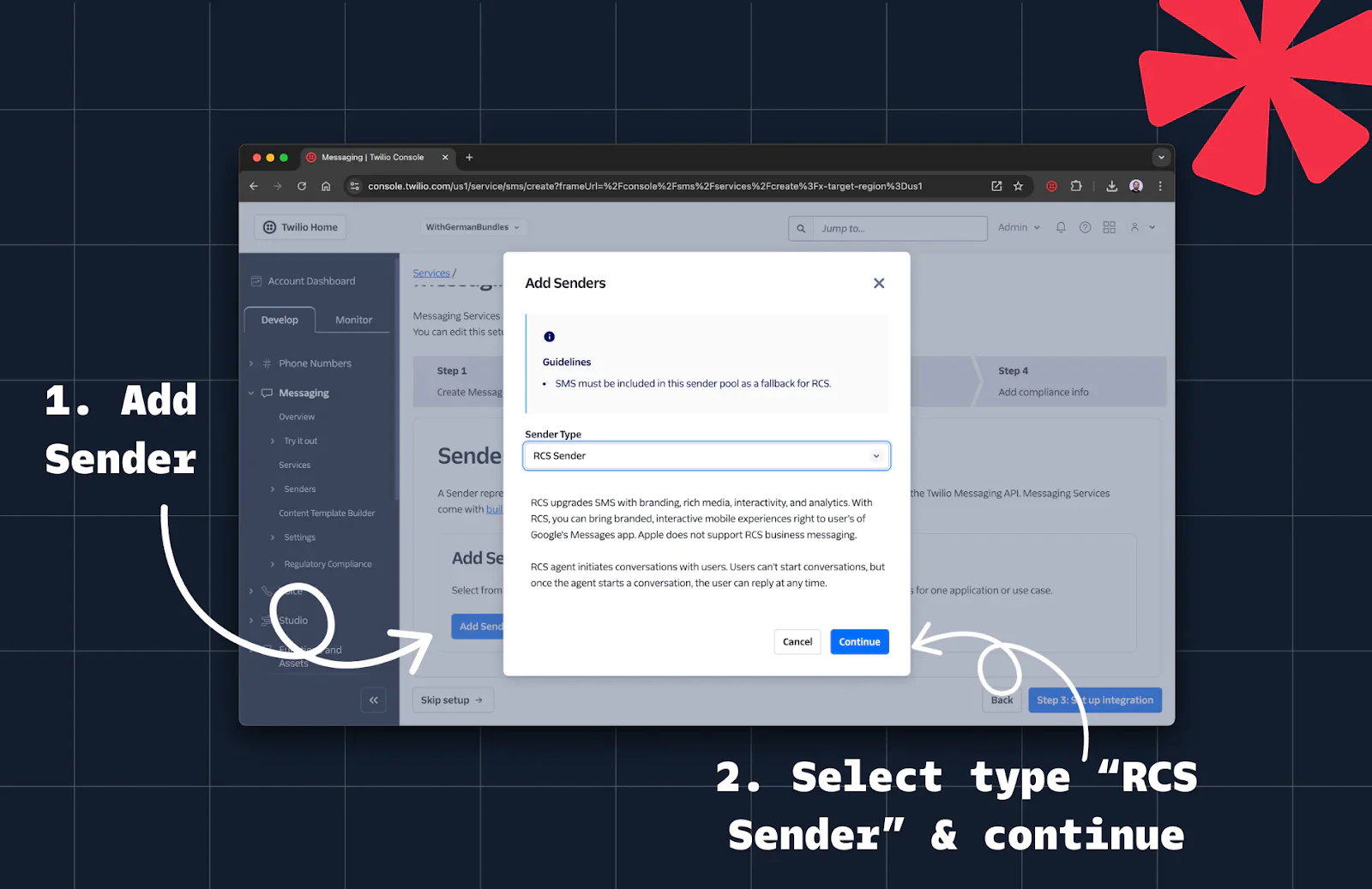
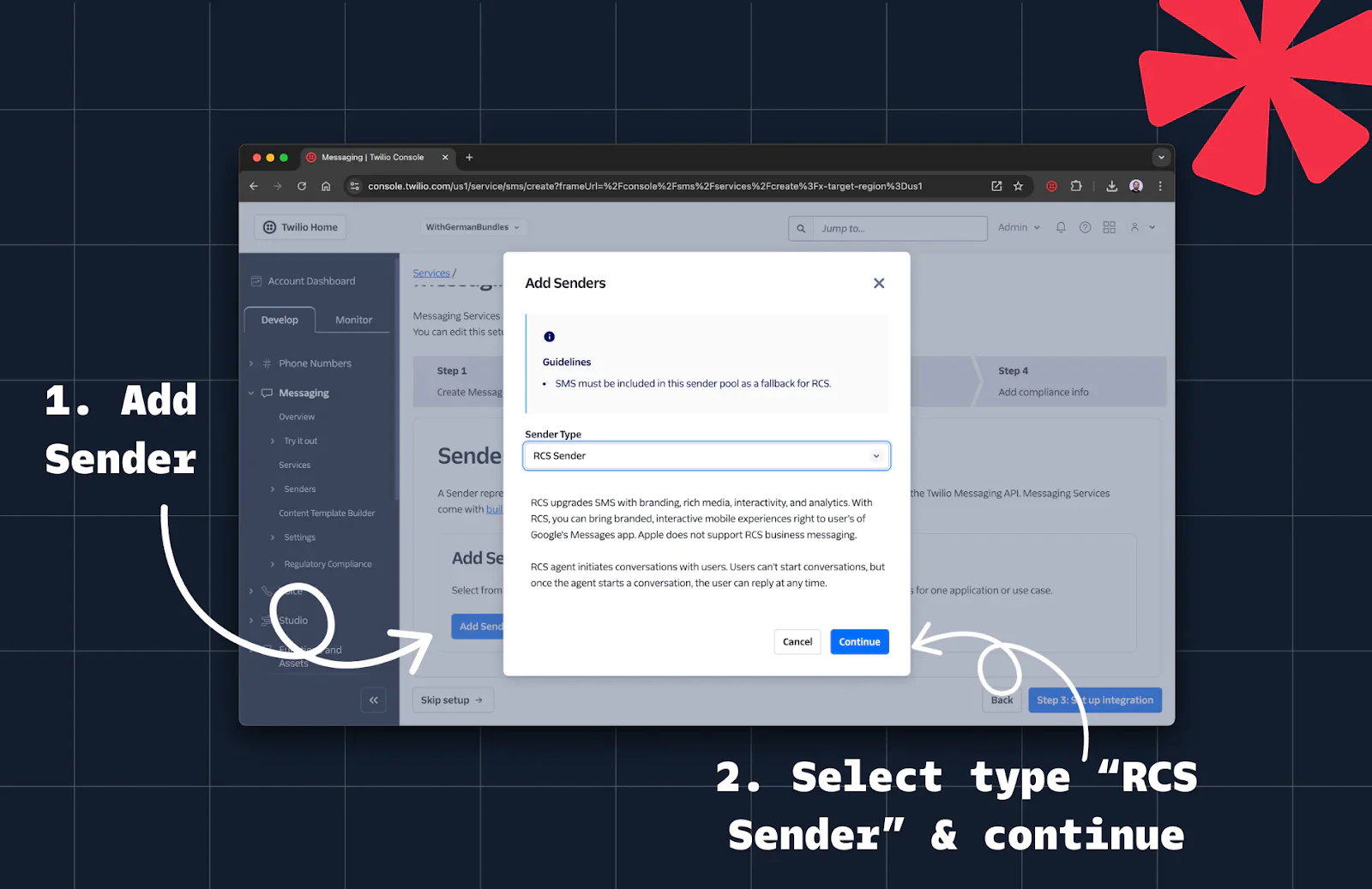
- Add the RCS sender you just created.
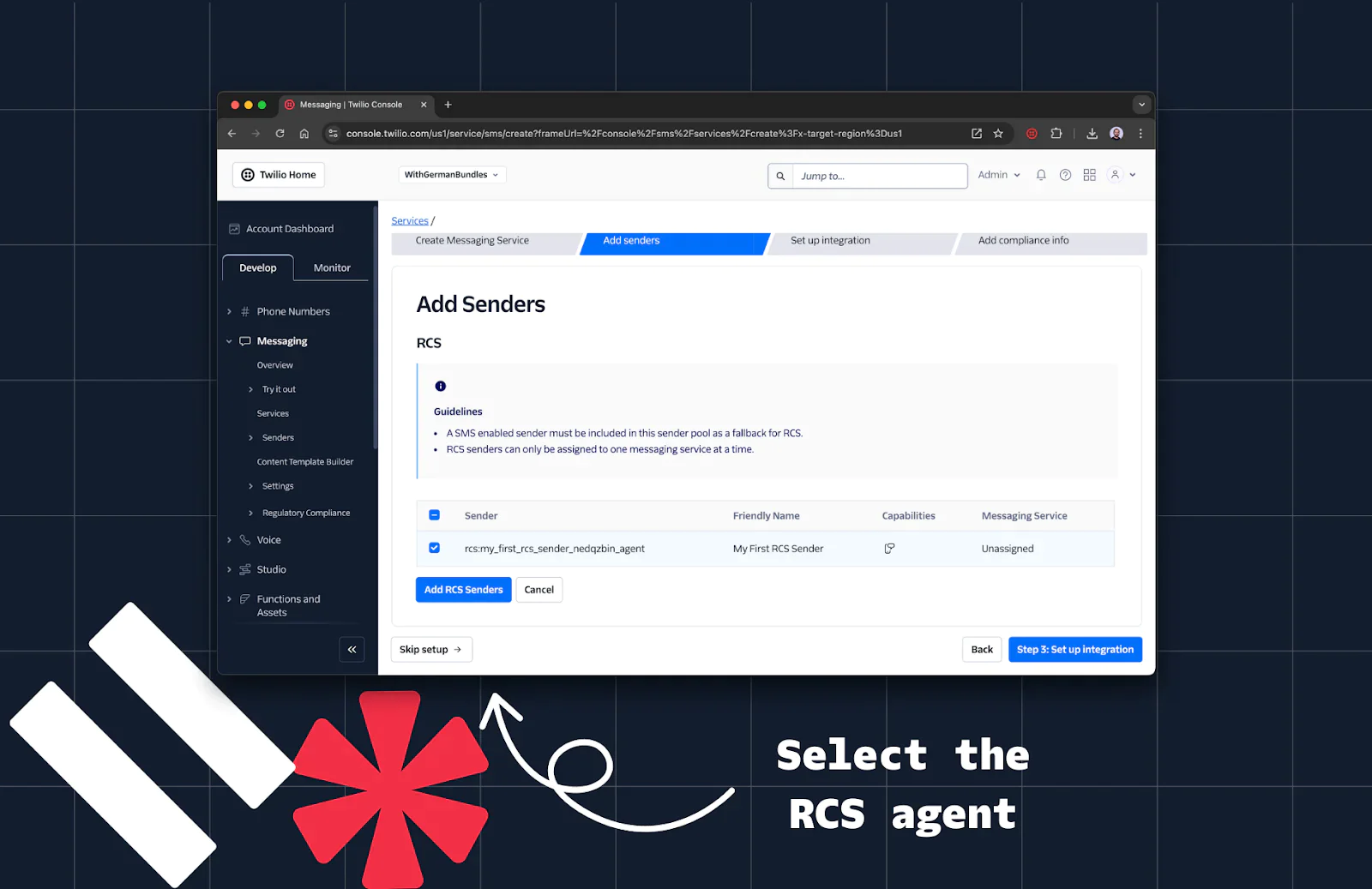
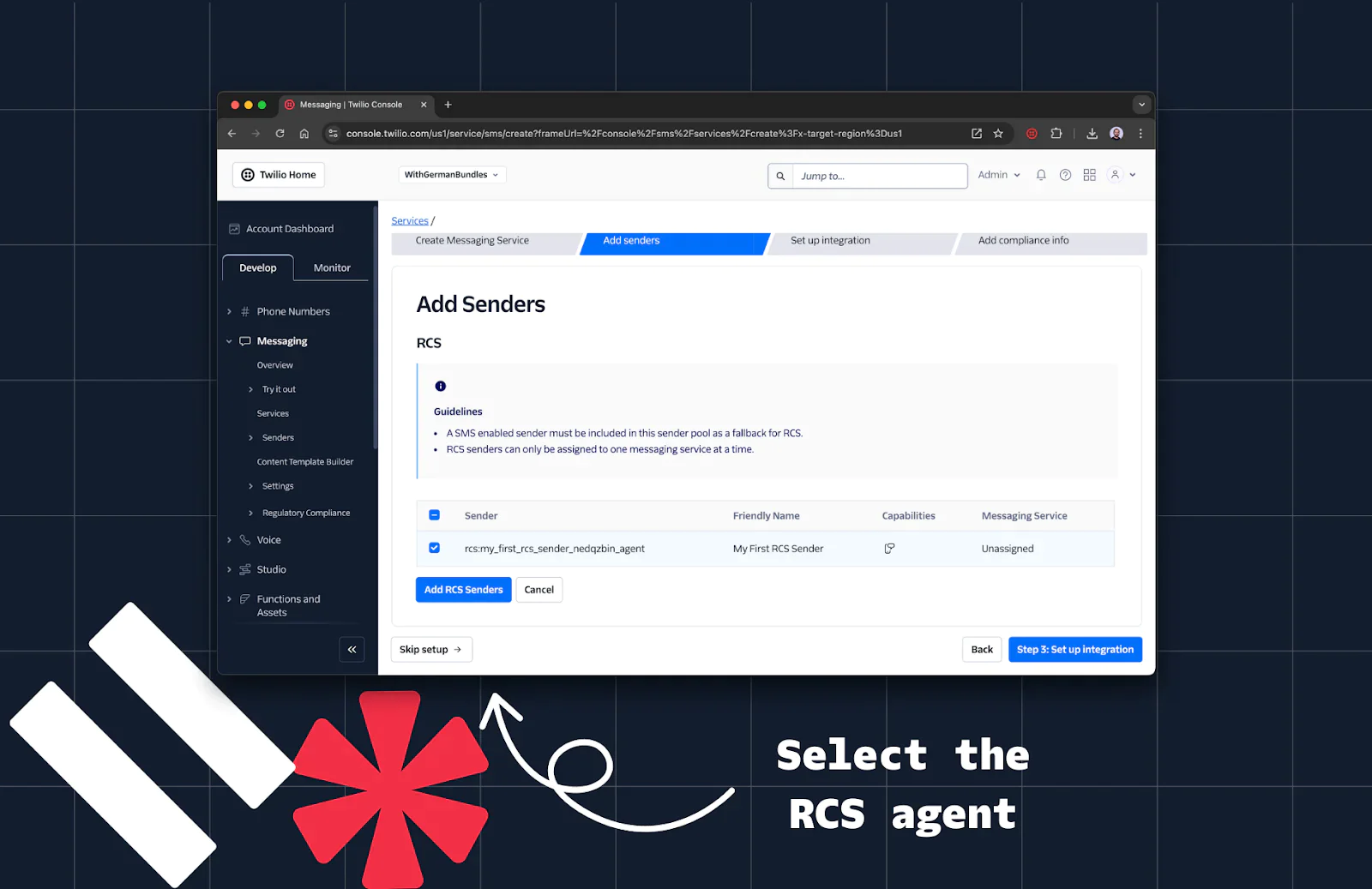
- Add an SMS fallback sender by repeating the Add Sender process and selecting a phone number (or other SMS-capable sender). Then, click Step 3: Set up integration.
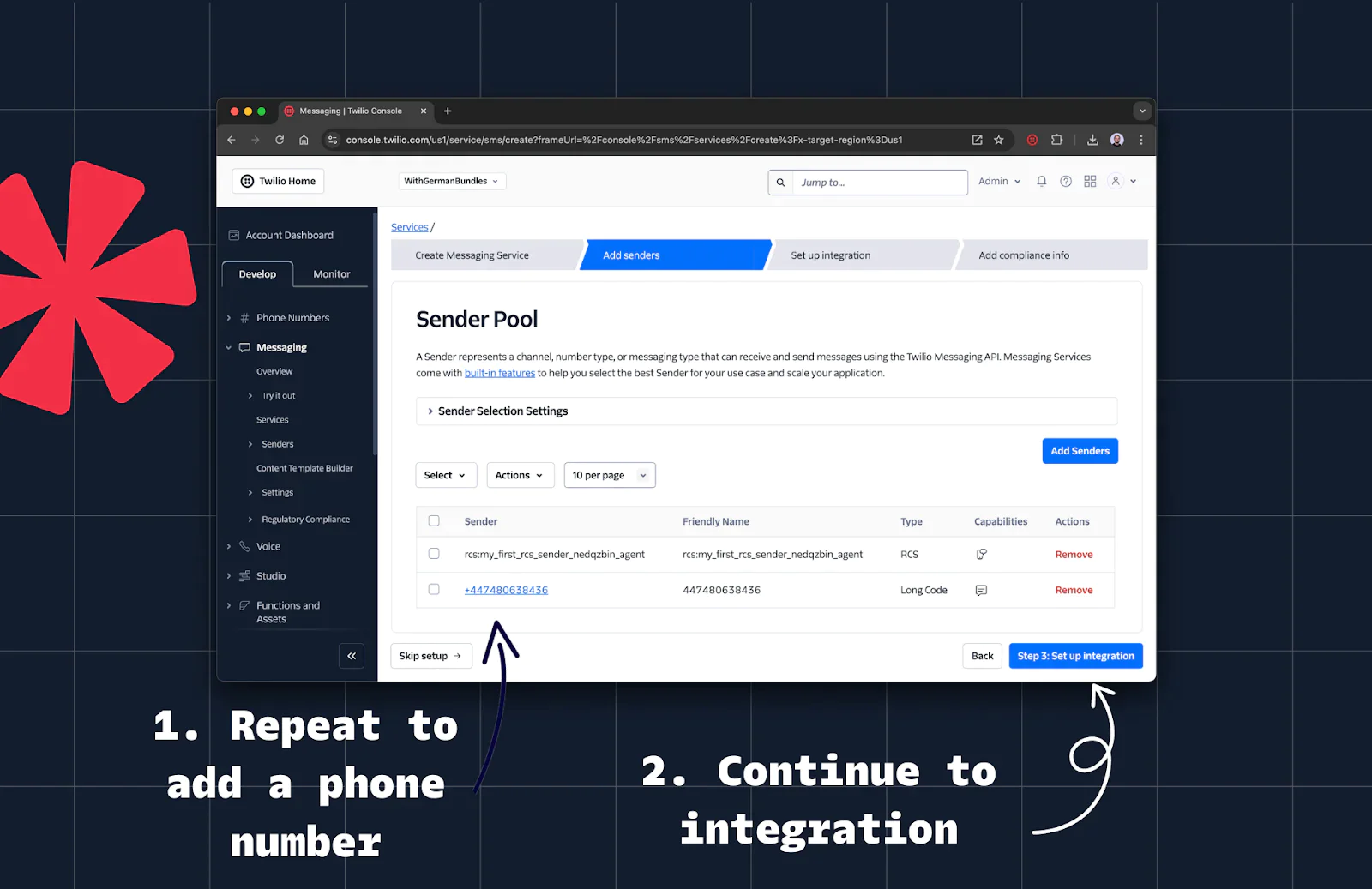
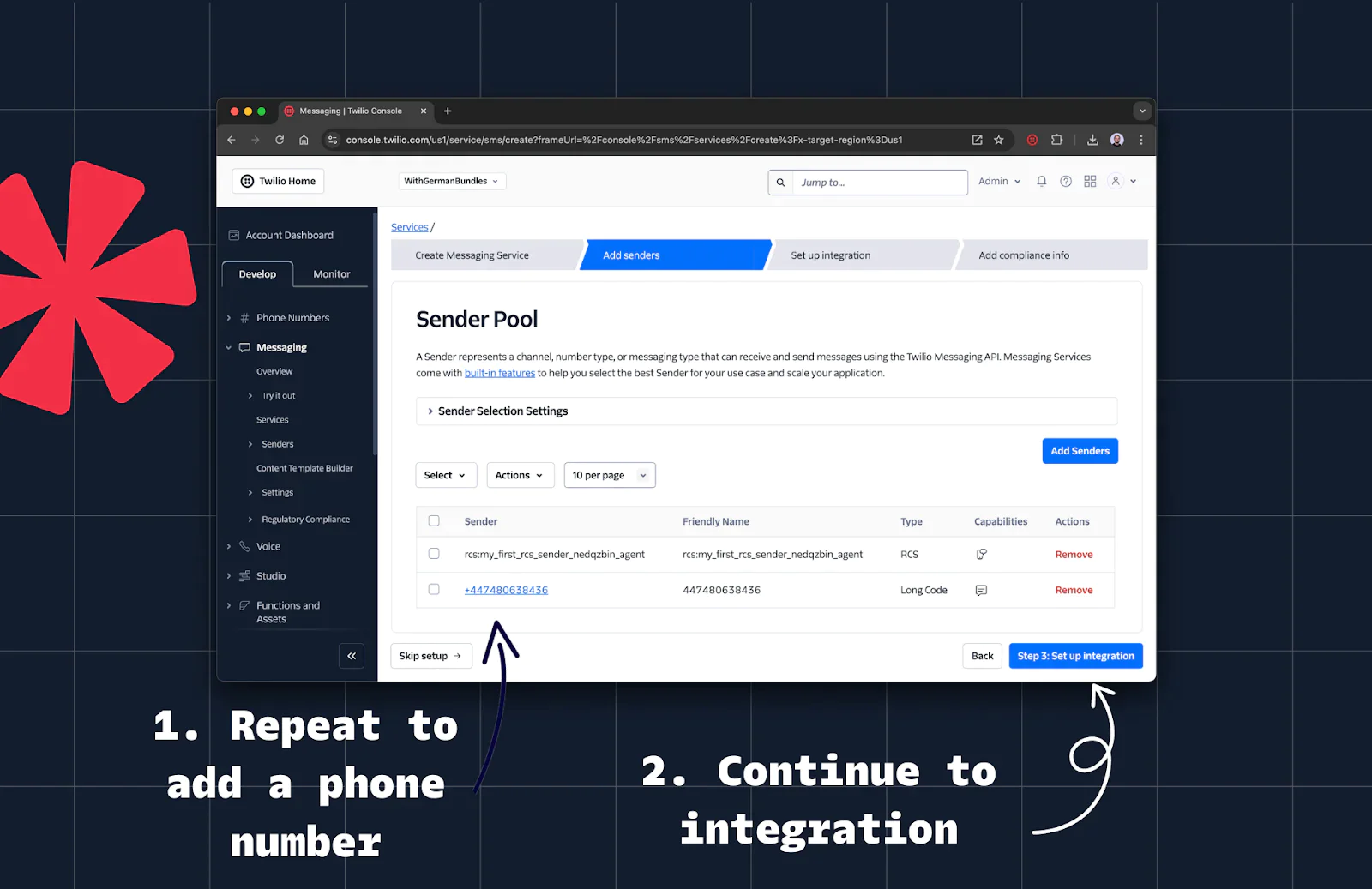
Step 5: (Optional) Set Up Webhook Integration
- Webhooks allow you to handle incoming messages in the same way as SMS or WhatsApp. Learn more in Twilio's docs about Incoming Message Webhooks.
- Use the Set Up Integration option to register a webhook. Feel free to reuse an existing one if available.
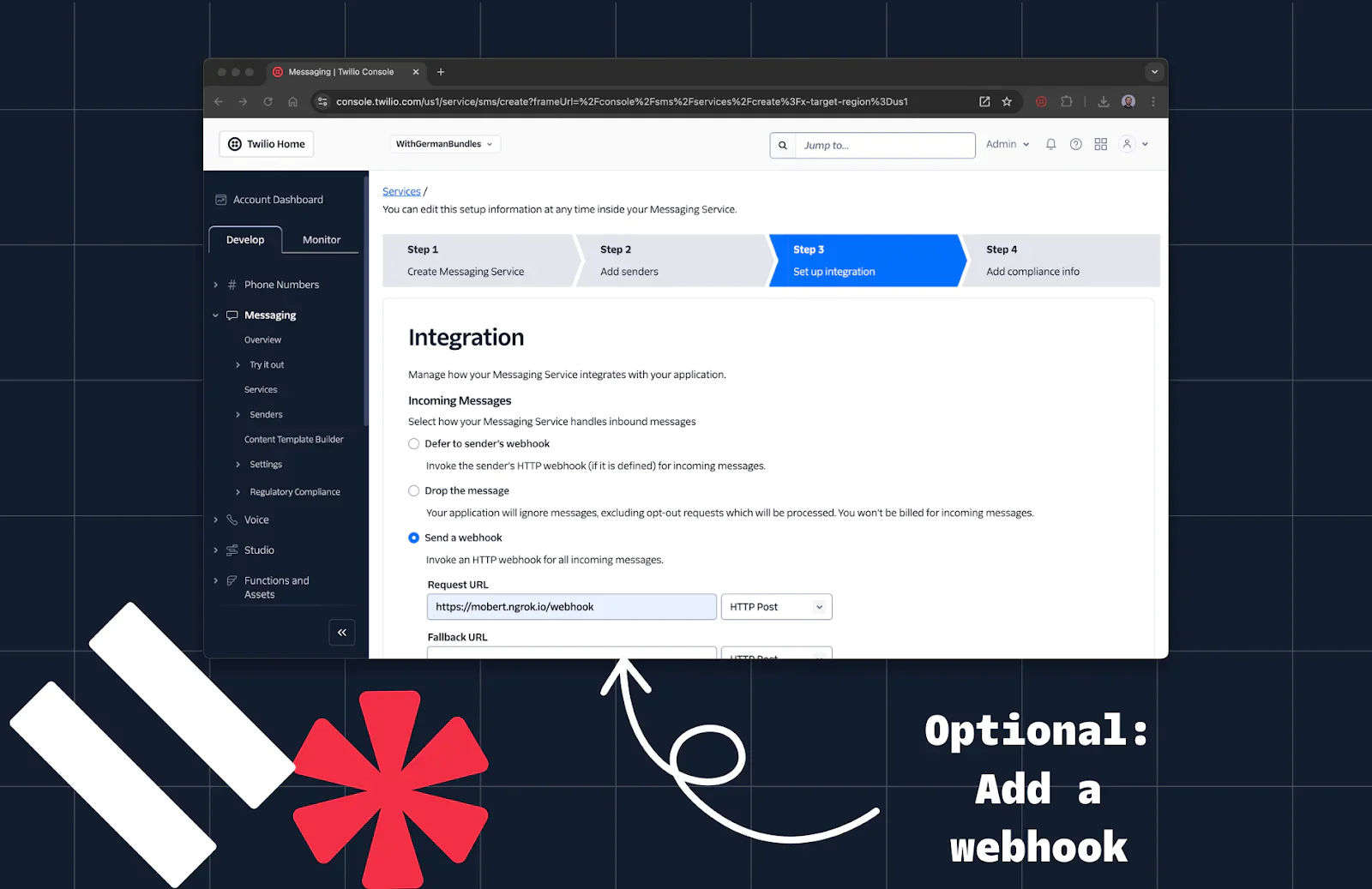
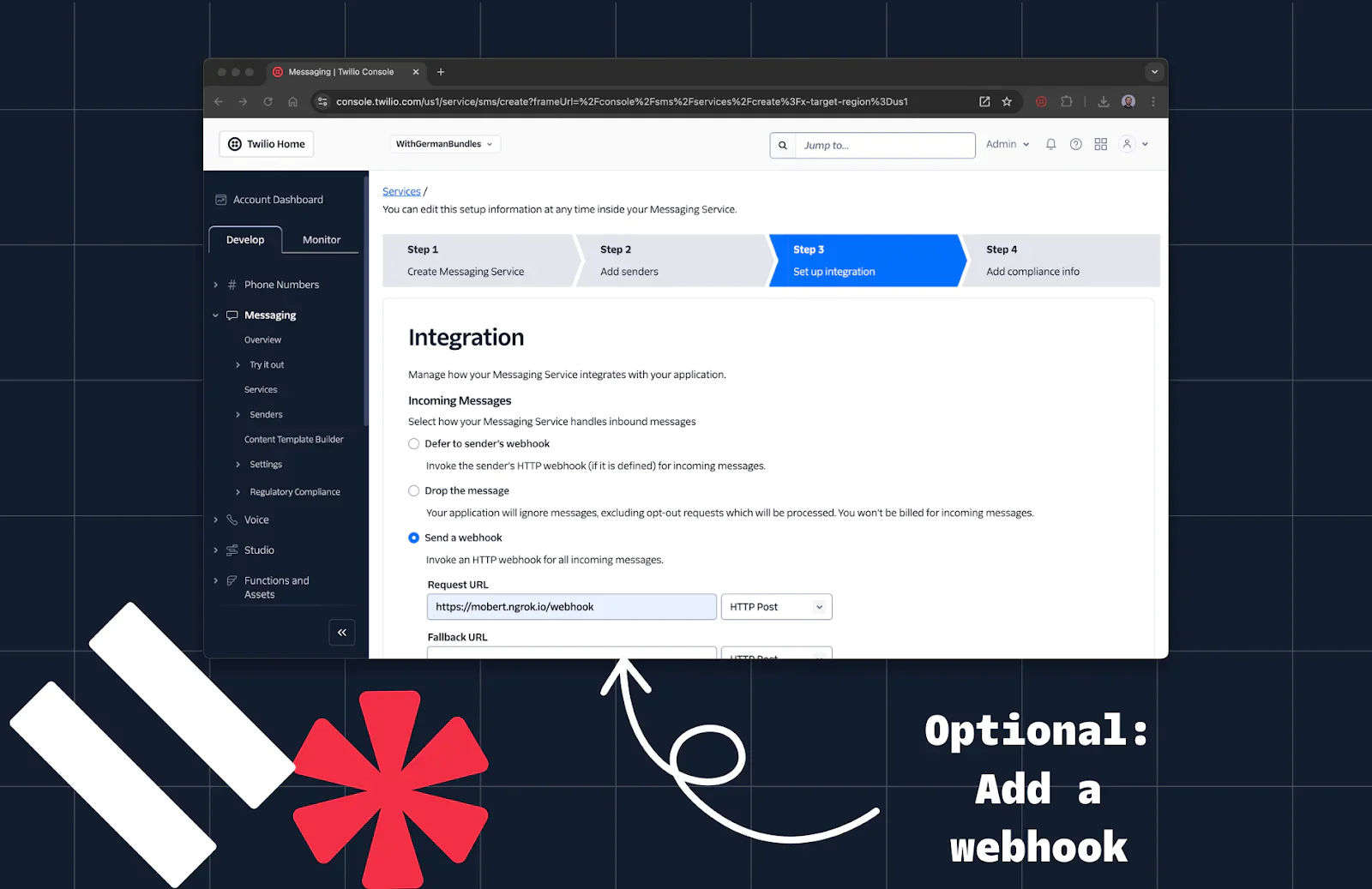
Step 6: Send Your First RCS Message with C#
1. Finish the wizard flow by clicking the white Skip setup button.
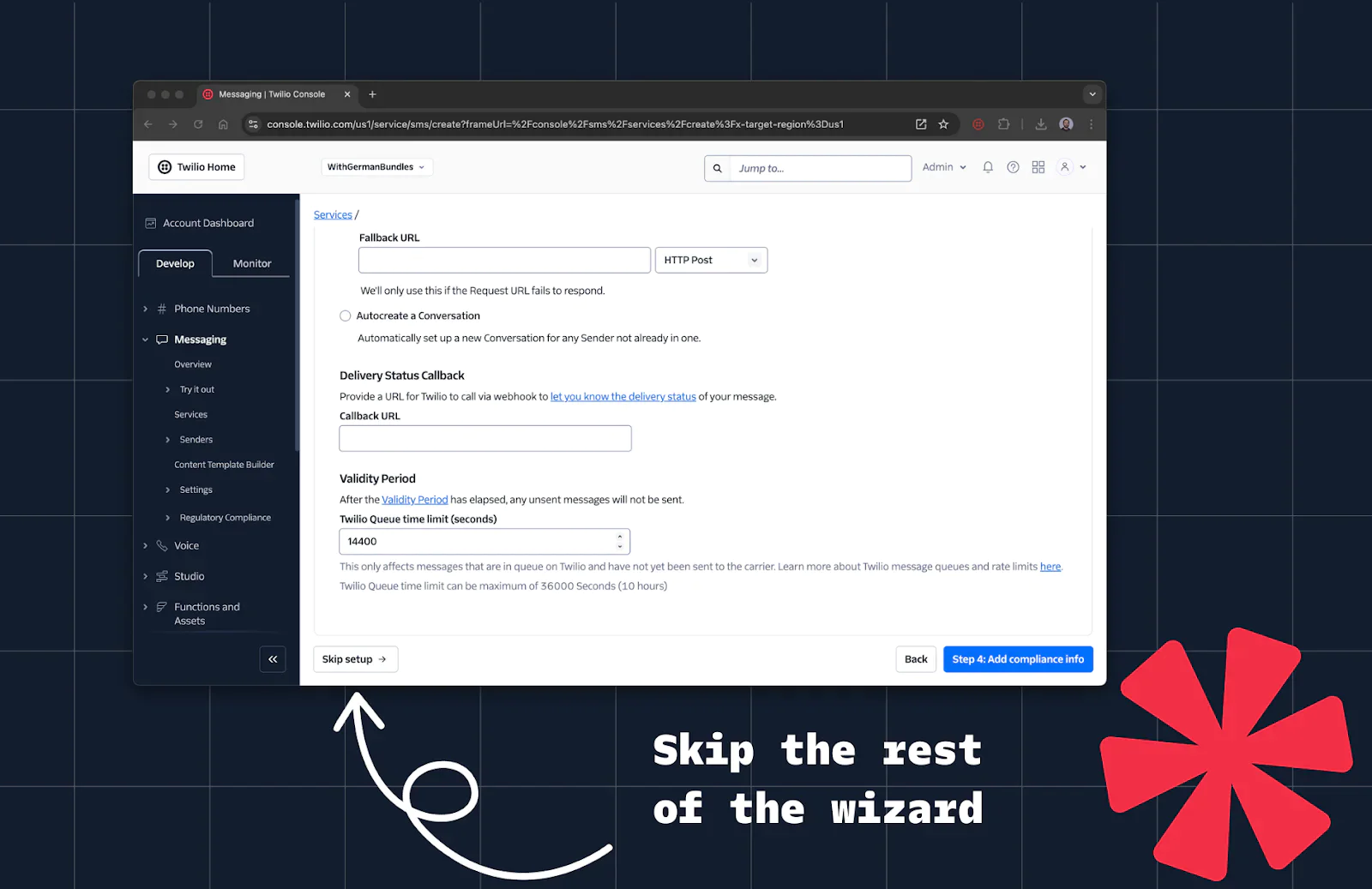
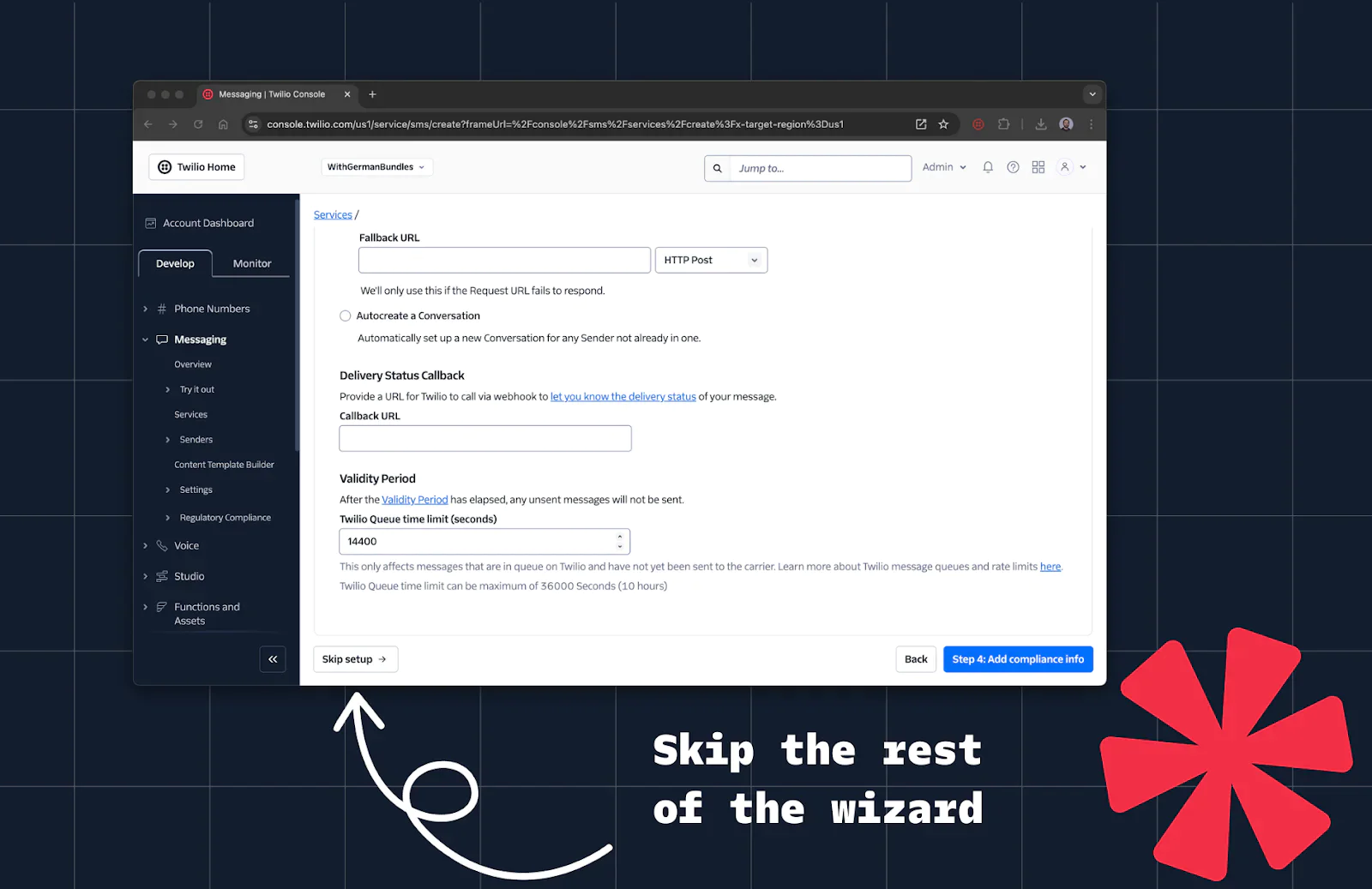
2. Copy the Messaging Service SID (like MGe1fdfb207e9aa5b44f398b1094d88a8b
) from the Console.
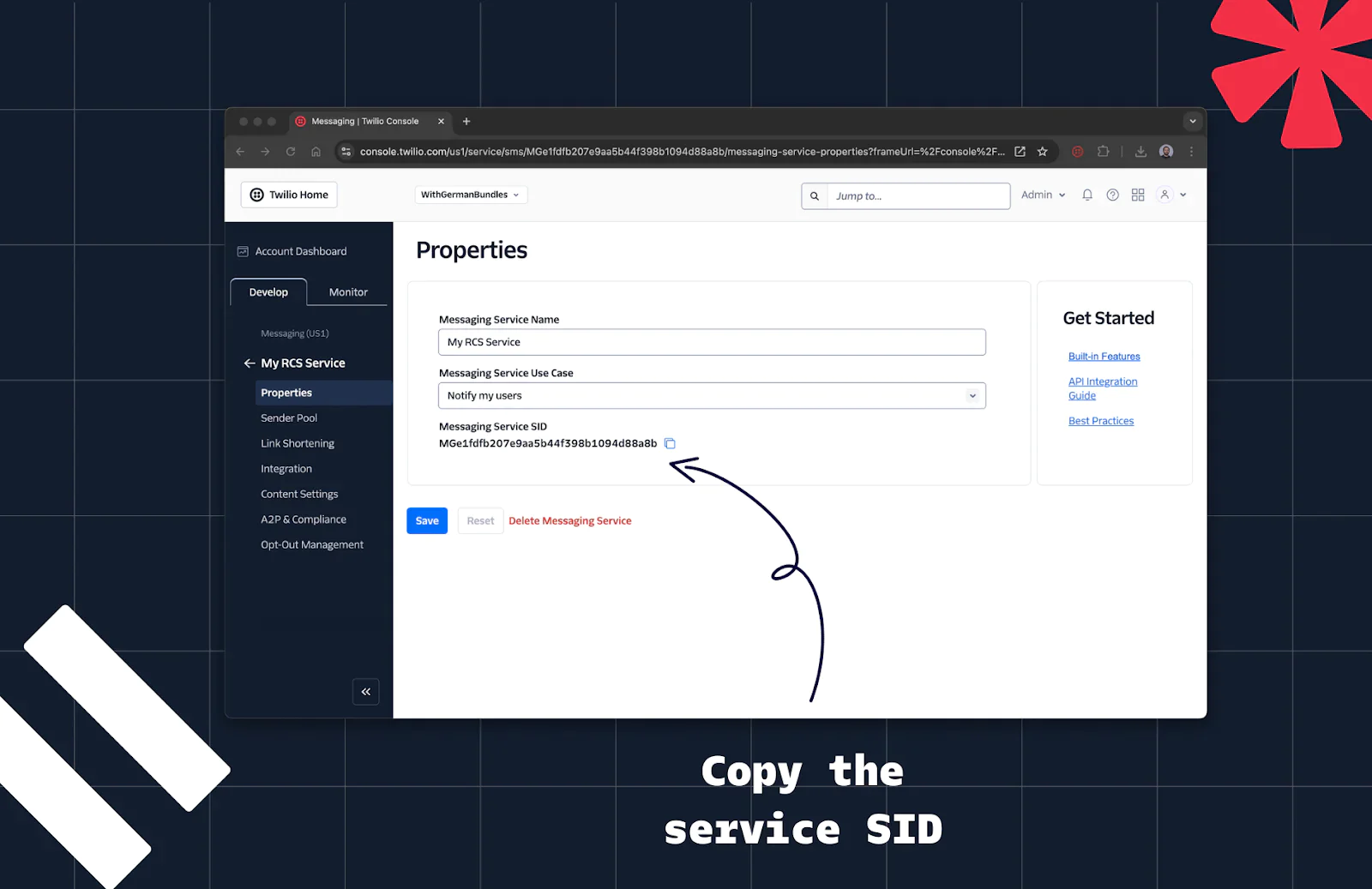
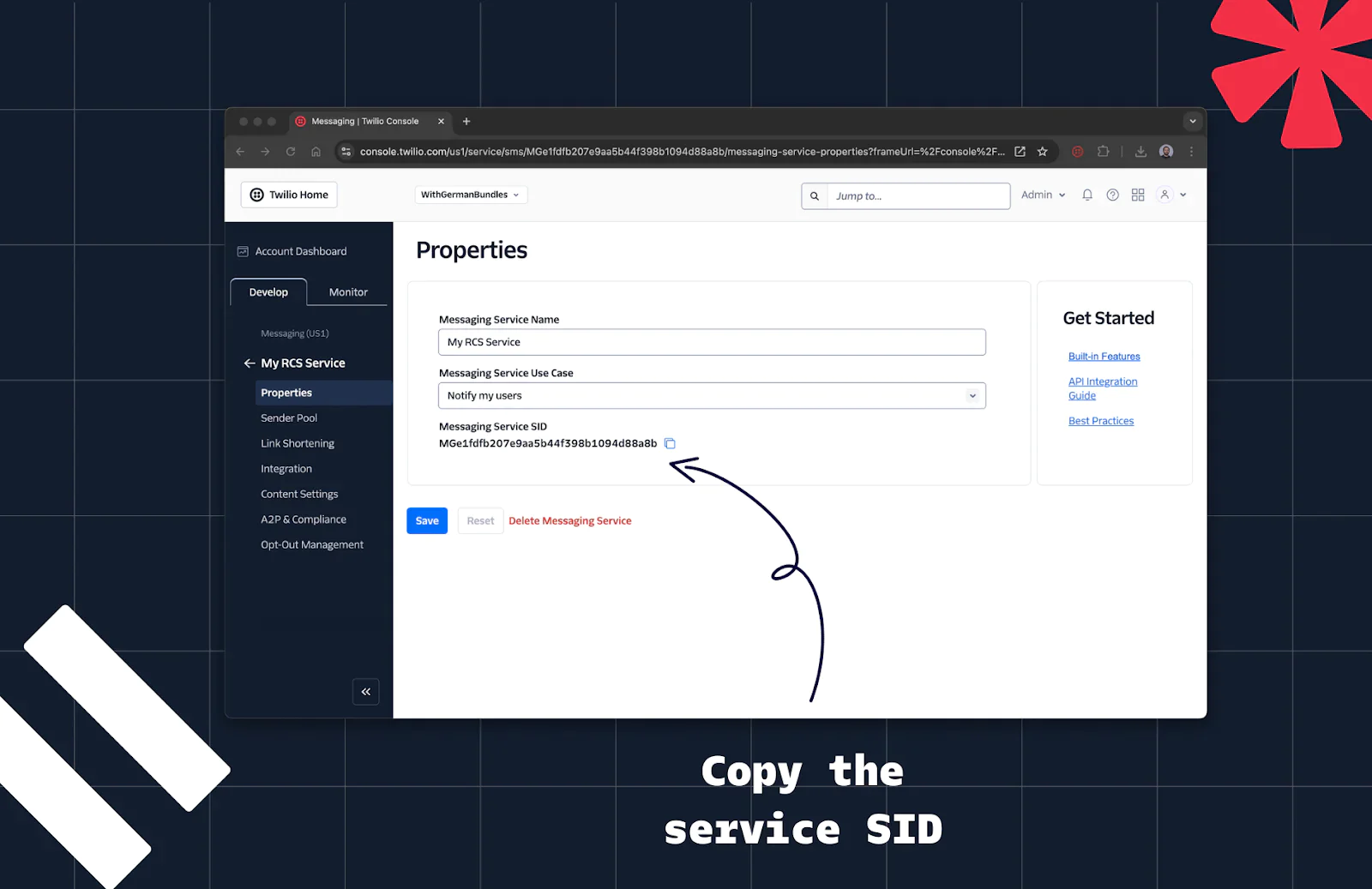
3. Retrieve your Account SID and Auth Token from the Twilio Console.
4. Create a new project with the .NET CLI.
5. Create a file in your project folder called .env
. Add the credentials from the previous steps, so that your file looks like this:
6. Add the dependency packages you will use in your application code.
7. Edit the auto-generated Program.cs
file to look like this:
8. Build and run the application.
9. See the result on your RCS-enabled device.
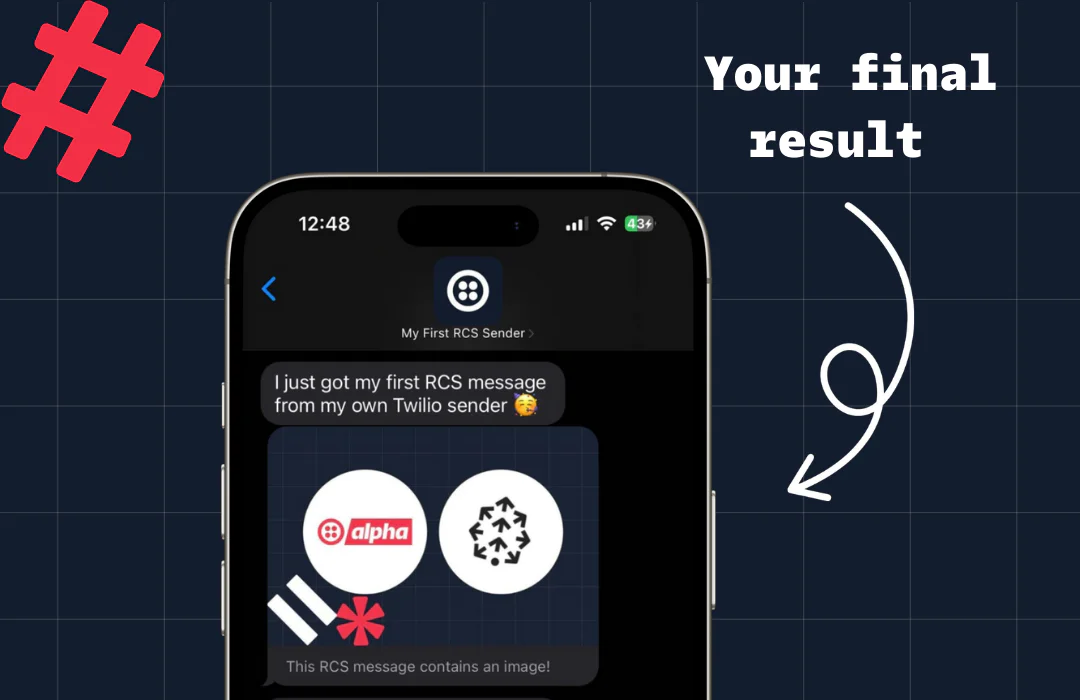
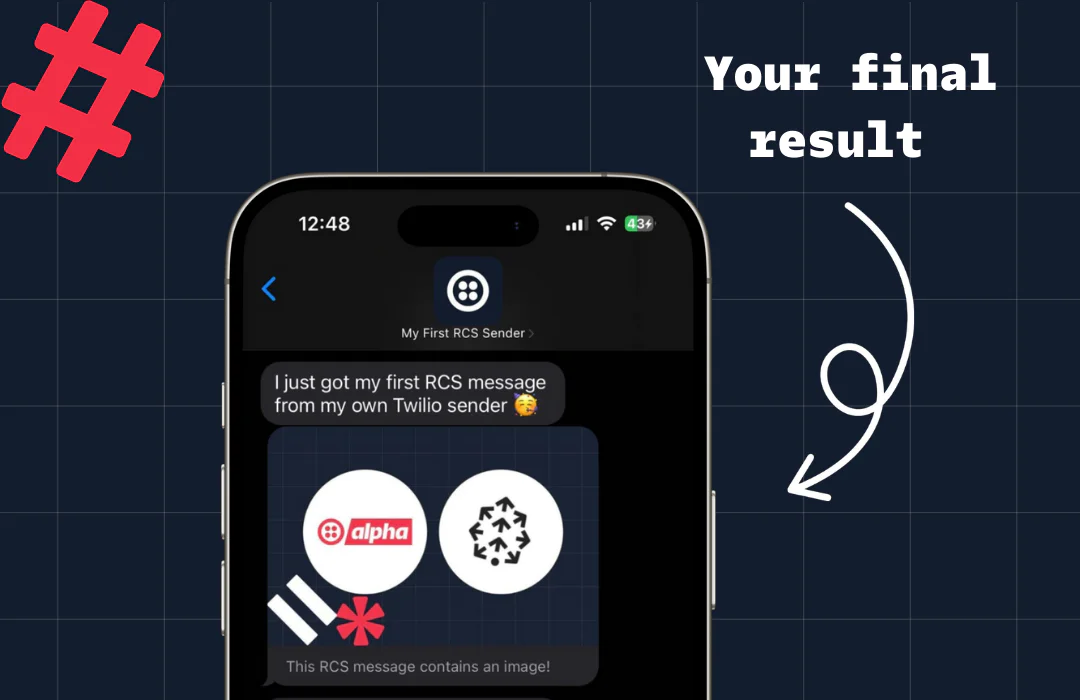
Explore Advanced RCS Features
You have successfully sent an RCS message using C# with .NET. Nice work!
When businesses use RCS to engage their customers, they get access to new avenues for engagement and interactivity. To continue leveling up your skills, check out some of the other things you can do in conjunction with RCS:
- Use the Content Template Builder to create rich message content which you can use across various channels, including SMS, WhatsApp, and RCS.
- Explore the Programmable Messaging Logs to see message statuses and troubleshoot any errors.
- Work with Twilio Verify to send OTP codes with RCS. Twilio Verify lets you deliver secure and engaging one-time passwords to your users.
Twilio is continuously enhancing its RCS capabilities. This includes new features like failover for undelivered messages and quick reply buttons. There’s more coming, so stay tuned for updates!
Modern businesses are using RCS to transform their customer engagement approach. With RCS, you get the reliability of SMS with the interactive features that end users expect from the messaging apps they know and love.
We can’t wait to see what you build!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.