How to Send an SMS With TypeScript Using Twilio
Time to read: 4 minutes
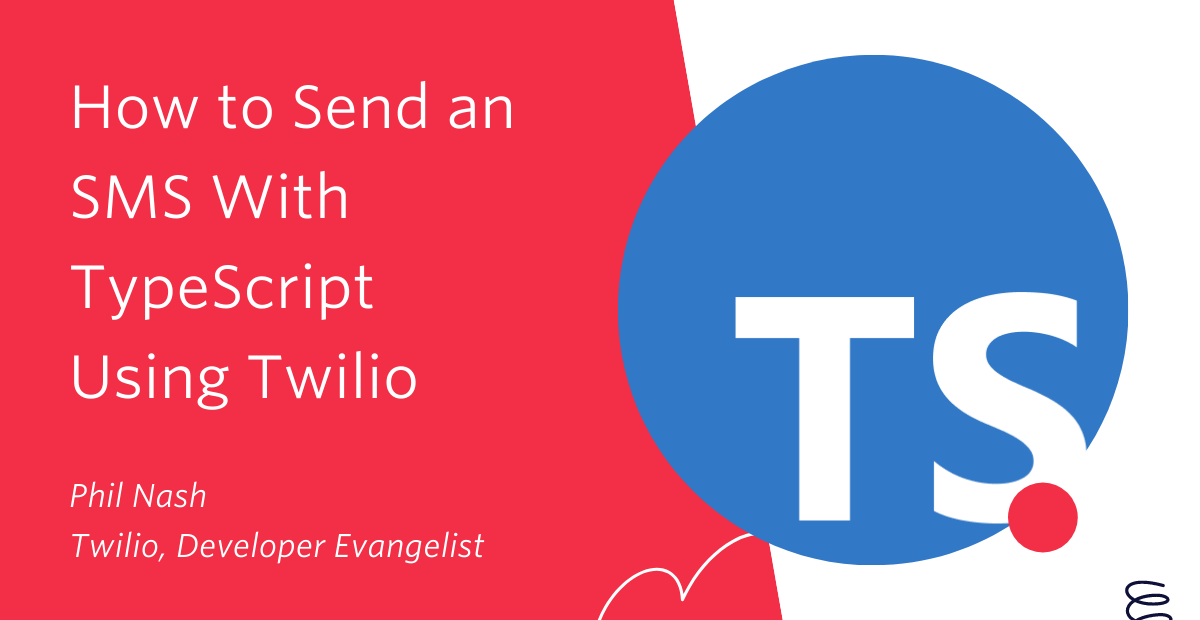
Writing Node.js applications with TypeScript means you can take advantage of the TypeScript type system and tooling. We've seen on this blog how to send an SMS message with Node.js, but let's have a look at how to do this with TypeScript.
What you need
To follow this tutorial you will need:
- A Twilio account (if you don't have one yet, sign up for a free Twilio account here and receive $10 credit when you upgrade)
- A Twilio phone number that can send SMS messages
- Node.js installed
Once you've got all that, let's dive into the code.
Getting started with TypeScript
Let's start a new TypeScript project for this example. In a terminal, run the following commands to create a new project directory and create a package.json file.
Now we need to install some dependencies. We'll need TypeScript in our development dependencies so we can compile our code to JavaScript before we run it and we'll need the Twilio Node module which makes it easy to use the API to send SMS messages.
Initialise the project with a tsconfig.json
file by running:
We'll leave the tsconfig.json
with the default settings. If you are interested in changing them the settings are well commented. You can also read more about the different options in the TSConfig Reference.
We need a file into which we will write our program. Create a file called index.ts
:
Next we'll set up a script to compile our TypeScript to JavaScript and a script to run the JavaScript. Open package.json
and to the "scripts"
property add the following:
tsc
is the TypeScript compiler command that will take our index.ts
file and compile it to index.js
. The "send"
script will then call the compiled index.js
file. Now we're ready to write some TypeScript!
Let's write some TypeScript
Open index.ts
and start by importing the Twilio library:
For this script we will send the parameters in via environment variables. Environment variables are a great place to store information that you need in an app but shouldn't check in to source control. You can read more about setting environment variables in this post.
We will need our Account SID and Auth Token, available in your Twilio console, a Twilio phone number that can send SMS messages and a phone number to send the message to. We'll get those variables out of the environment like so:
Using the accountSid
and authToken
initialise a Twilio API client:
Using the client, we can make an API request to create a message. To the create
method we need to pass:
- the number we're sending the message from, which will be our Twilio number
- the number we are sending it to, which is your phone number
- a message body
The create
method returns a Promise when the request is successful and we'll log the SID of the message resource we created to show it worked.
If this was regular JavaScript we'd be done with this example script. If you are using an editor with tooling for TypeScript, like VS Code, you will have already seen there are some issues with this script. If you don't have TypeScript support in your editor, you can see the errors by running the build script we defined earlier; npm run build
.
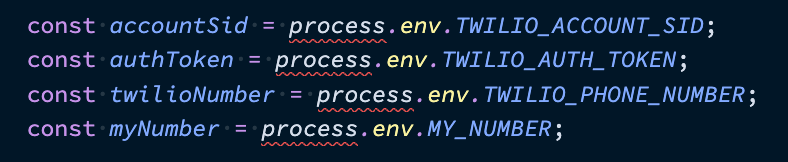
Squiggly red underlines mean we have failed to satisfy TypeScript with this code. In this case, TypeScript is not sure what the process
object is. We may know that it is a Node.js global that provides information about and control over the running Node.js process, but TypeScript does not. To tell TypeScript about process
we need to install the types for Node.js. We can do that with:
With that complete, the errors move further down the script.
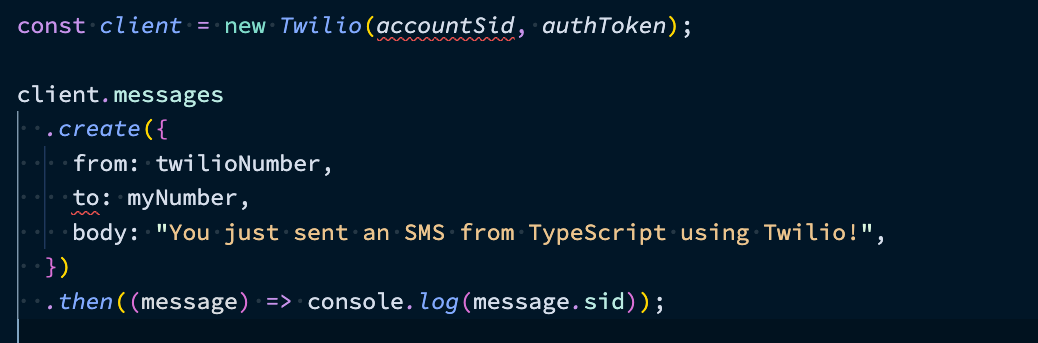
The problem now is that fetching values from the environment could result in either a string
or undefined
. So each of accountSid
, authToken
, twilioNumber
, and myNumber
are of type string | undefined
. The constructor for the API client requires the first two arguments to be of type string
and string | undefined
doesn't satisfy that. Similarly, when sending a message there should always be a to
number.
So, before constructing an API client we need to ensure that accountSid
and authToken
are both string
s. Before sending a message we also need to ensure that myNumber
is a number. from
is actually defined as a type of string | undefined
, but that is because we can also pass a messagingServiceSid
if we want to use a pool of sender numbers from a messaging service. So while we are checking the presence of these other variables, we should also ensure there is a twilioNumber
too.
For this example let's wrap the client initialization and message creation in a conditional based on the presence of the required variables. We can then show an error message if one is missing.
You should find that there are no more red underlines, which means we can compile the TypeScript into JavaScript. In the project directory, run the script we defined earlier:
When the script completes, you will find an index.js
file in your project. You can now run the other script we defined earlier to send an SMS message.
Of course, you should set all the relevant environment variables. When running a single script like this, we can do it all on one line:
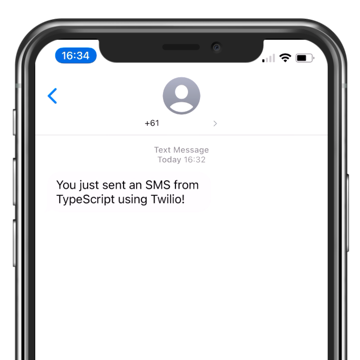
That's it, you've sent an SMS message using TypeScript and Twilio.
What's next?
Now you've seen how to get started with sending SMS messages with Twilio and TypeScript there's plenty more you can do. You can find the full code for this blog post, plus other example TypeScript projects, on GitHub. The Twilio Node.js package ships with TypeScript types, so you can use it to gain the benefits from TypeScript without having to write your own types. You can also use TypeScript to write functions you deploy to Twilio Runtime.
If you're looking for other things to build with TypeScript and Twilio check out how to receive and reply to SMS messages in TypeScript, how to build an SMS weather bot with Twilio and TypeScript or how to get started with Twilio Video and TypeScript. If you like the look of TypeScript, but you're currently working with JavaScript, take a look at this guide for moving your project to TypeScript at your own pace. Or hit up the TypeScript tag on this blog for all the latest posts on Twilio and TypeScript.
Are you using TypeScript to build amazing things with Twilio? I would love to hear about it. Drop me an email at philnash@twilio.com or send me a message on Twitter at @philnash.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.