Automated Survey with Java and Servlets
Time to read: 5 minutes
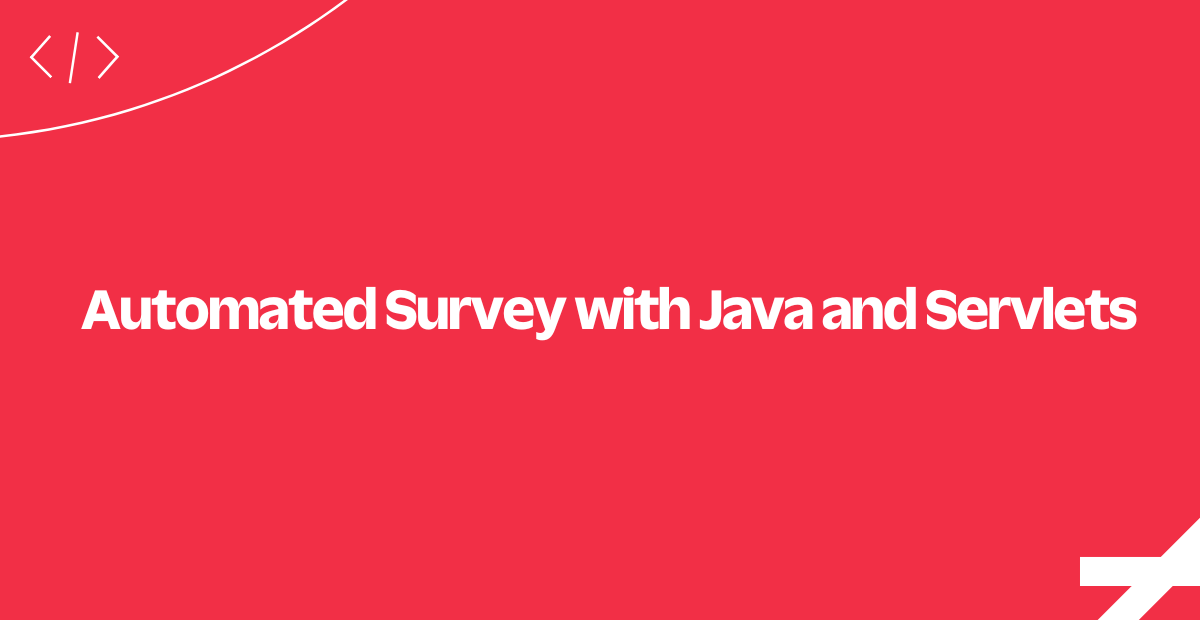
Have you ever wondered how to create an automated survey that can be answered over phone or SMS?
This tutorial will show how to do it using the Twilio API.
Here's how it works at a high level
- The end user calls or sends an SMS to the survey phone number.
- Twilio gets the call or text and makes an HTTP request to your application asking for instructions on how to respond.
- Your web application instructs Twilio (using TwiML) to
Gather
orRecord
user input over the phone, or prompt for text input withMessage
if the survey is taken via SMS. - After each question, Twilio makes another request to your server with the user's input, which your application stores in its database.
- After storing the answer, our server will instruct Twilio to
Redirect
the user to the next question or finish the survey.
Creating a Survey
In order to perform an automated survey, we first need to set up the survey questions. For your convenience, this application's repository already includes one survey. If the database is configured correctly every time that the app receives a request for a survey, it will create a new survey registration.
You can modify the survey questions by editing the survey.json file located in the root of the repository and re-running the app.
We want users to take our survey, so we still need to implement the handler for SMS and calls. First, let's take a moment to understand the flow of a Twilio-powered survey as an interview loop.
The Interview Loop
The user can answer your survey questions over the phone using either their phone's keypad or by voice input. After each interaction Twilio will make an HTTP request to your web application with either the string of keys the user pressed or a URL to a recording of their voice input.
For SMS surveys the user will answer questions by replying with another SMS to the Twilio number that sent the question.
It's up to the application to process, store, and respond to the user's input.
Let's dive into this flow to see how it actually works.
Configuring a Twilio Number
To initiate the interview process, we need to configure one of our Twilio numbers to send our web application an HTTP request when we get an incoming call or text.
Click on one of your numbers to configure your Voice and Message URLs to point to the /survey
route using HTTP GET.
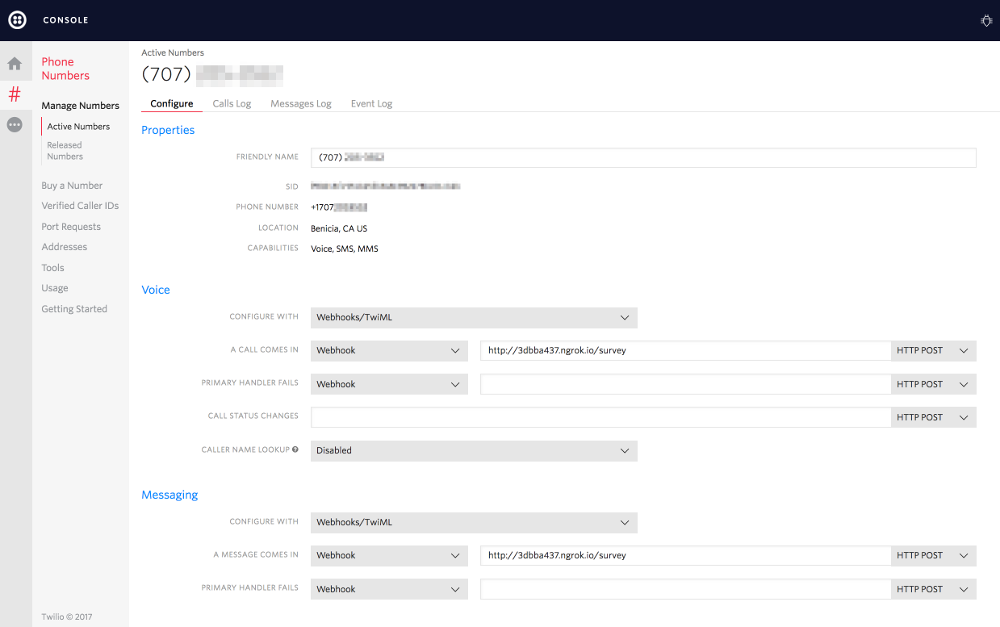
If you don't already have a server configured to use as your webhook, ngrok is a great tool for testing webhooks locally.
We that we have configured our webhooks in the Twilio Console, we are ready to create our webhook endpoints.
Responding to a Twilio Request
Right after receiving a call or SMS, Twilio sends a request to the URL specified in our phone number configuration.
The endpoint will then process the request. If the incoming request is not a response to a previous question, the application will build and return a welcome message for the user. This welcome message is constructed by using a Say
verb for a voice response or a Message
verb if the user is taking the survey via SMS.
Our response also includes a Redirect
for redirecting to the question's endpoint in order to continue with the survey flow.
Thats how you generate TwiML responses in your webhooks. Now lets see how to store the state of the survey using a controller.
Question Controller
This endpoint returns the Question
specified in the request. If no specific question is specified in the request, it will return the first question in the survey.
Each type of question and interaction (Call or SMS) will produce different instructions on how to proceed. For instance, we can record voice or gather a key press during a call, but we can't do the same for text messages.
AbstractTwiMLQuestionFactory.getInstance
solves that problem. It will return a different type of factory that knows how to build responses depending on wether the request originated via SMS or a voice call.
When the user is interacting with our survey over SMS we don't have something like an ongoing call session with a well defined state. It becomes harder to know if an SMS is answering question 2 or 20, since all requests are sent to our /survey
main endpoint.
To solve that, we'll store the id
of the survey and question at hand in the HTTP session. We can reuse these for subsequent SMS requests.
Let's see how the response is built.
Building Our TwiML Verbs
If the survey question is numeric or boolean ("yes/no") in nature we will use the <Gather>
verb to build our TwiML. However, if we expect the user to record a voice answer we want to use the <Record>
verb. Both verbs take an action
attribute and a method
attribute.
Twilio uses both attributes to define our response's endpoint to use as a callback. This endpoint is responsible for receiving and storing the caller's answer.
During the Record
verb creation, we also ask Twilio for a Transcription. Twilio will process the voice recording and extract all useful text, making a request to our response endpoint when the transcription is complete.
Now lets see what to do with the response.
Handling Responses
After the user has finished speaking and pressing keys, Twilio sends us a request explaining what happened and asking for further instructions.
In Survey.answerCall
and Survey.answerSMS
we extract request parameters from a Map
containing the information needed to answer the question.
Recovered parameters vary according to what we asked in our survey questions:
Body
contains the text message from an answer sent via SMS.Digits
contains the keys pressed for a numeric question.RecordingUrl
contains the URL for listening to a recorded message.TranscriptionText
contains the text of a voice recording transcription.
After answering a question, the SurveyController
redirects to our QuestionServlet
, which will ask the next question in the loop.
Now, let's see how to visualize the results.
Displaying the Survey's Results
For this route we simply query the database using a JPA query and then display the information within a JSP page. We display a list of surveys with their respective questions and answers.
You can access this page in the application's root route.
That's it!
If you have configured one of your Twilio numbers to work with the application built in this tutorial you should be able to take the survey and see the results under the root route of the application. We hope you found this sample application useful.
Where to next?
If you're a Java developer working with Twilio, you might enjoy these other tutorials:
SMS and MMS Notifications
Never miss another server outage. Learn how to build a server notification system that will alert all administrators via SMS when a server outage occurs.
Click to Call
Click-to-call enables your company to convert web traffic into phone calls with the click of a button.
Did this help?
Thanks for checking this tutorial out! If you have any feedback to share with us, we'd love to hear it. Reach out to us on Twitter and let us know what you build!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.