Python Flask Quickstart for Twilio Two-factor Authentication
Warning
As of November 2022, Twilio no longer provides support for Authy SMS/Voice-only customers. Customers who were also using Authy TOTP or Push prior to March 1, 2023 are still supported. The Authy API is now closed to new customers and will be fully deprecated in the future.
For new development, we encourage you to use the Verify v2 API.
Existing customers will not be impacted at this time until Authy API has reached End of Life. For more information about migration, see Migrating from Authy to Verify for SMS.
Adding Two-factor Authentication to your application is the easiest way to increase security and trust in your product without unnecessarily burdening your users. This quickstart guides you through building a Python and Flask application that restricts access to a URL. Four Two-factor Authentication channels are demoed: SMS, Voice, Soft Tokens and Push Notifications.
Ready to protect your toy app's users from nefarious balaclava wearing hackers? Dive in!
Create a new Twilio account (you can sign up for a free Twilio trial), or sign into an existing Twilio account.
Once logged in, visit the Authy Console. Click on the red 'Create New Application' (or big red plus ('+') if you already created one) to create a new Authy application then name it something memorable.
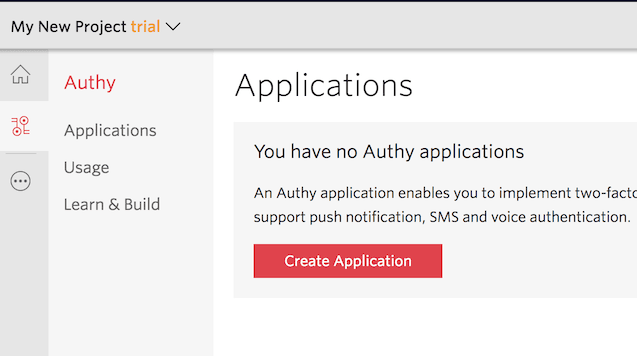
You'll automatically be transported to the Settings page next. Click the eyeball icon to reveal your Production API Key.
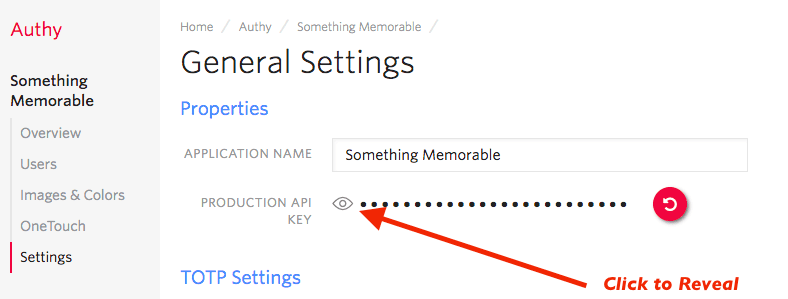
Copy your Production API Key to a safe place, you will use it during application setup.
This Two-factor Authentication demos two channels which require an installed Authy Client to test: Soft Tokens and Push Notifications. While SMS and Voice channels will work without the client, to try out all four authentication channels download and install Authy Client for Desktop or Mobile:
Clone our repository locally, then enter the directory. Install all of the necessary python modules:
pipenv install
or
pip -r requirements.txt
Next, open the file .env.example
. There, edit the ACCOUNT_SECURITY_API_KEY
, pasting in the API Key from the above step (in the console), and save the file as .env
.
Enter the API Key from the Account Security console and optionally change the port.
1SECRET_KEY=[create_a_key]23DATABASE_URI=45# You can get/create one here :6# https://www.twilio.com/console/authy/applications7ACCOUNT_SECURITY_API_KEY='ENTER_SECRET_HERE'8
Once you have added your API Key, you are ready to run! Launch Flask with:
./manage.py runserver
If your API Key is correct, you should get a message your new app is running!
With your phone (optionally with the Authy client installed) nearby, open a new browser tab and navigate to http://localhost:5000/register/
Enter your information and invent a password, then hit 'Register'. Your information is passed to Twilio (you will be able to see your user immediately in the console), and the application is returned a user_id
.
Now visit http://localhost:5000/login/ and login. You'll be presented with a happy screen:
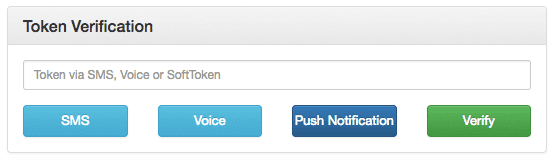
If your phone has the Authy Client installed, you can immediately enter a Soft Token from the client to Verify. Additionally, you can try a Push Notification by pushing the labeled button.
If you do not have the Authy Client installed, the SMS and Voice channels will also work in providing a token. To try different channels, you can logout to start the process again.
1import flask23from authy.api import AuthyApiClient4from flask_login import login_required, login_user, logout_user, current_user56from . import app, login_manager, db7# from .database import db_session8from .decorators import twofa_required9from .forms import (10LoginForm,11RegistrationForm,12TokenVerificationForm,13PhoneVerificationForm,14TokenPhoneValidationForm,15)16from .models import User171819authy_api = AuthyApiClient(app.config.get('ACCOUNT_SECURITY_API_KEY'))202122@app.route('/protected')23@login_required24@twofa_required25def protected():26return flask.render_template('protected.html')272829@app.route('/login', methods=['GET', 'POST'])30def login():31form = LoginForm()32if form.validate_on_submit():33login_user(form.user, remember=True)34form.user.is_authenticated = True35db.session.add(form.user)36db.session.commit()37next = flask.request.args.get('next')38return flask.redirect(next or flask.url_for('protected'))39return flask.render_template('login.html', form=form)404142@app.route("/logout", methods=["GET"])43@login_required44def logout():45current_user.is_authenticated = False46db.session.add(current_user)47db.session.commit()48flask.session['authy'] = False49flask.session['is_verified'] = False50logout_user()51return flask.redirect('/login')525354@app.route('/', methods=['GET', 'POST'])55def index():56return flask.redirect('/login')575859login_manager.unauthorized_handler(index)606162@app.route('/register', methods=['GET', 'POST'])63def register():64form = RegistrationForm()65if form.validate_on_submit():66authy_user = authy_api.users.create(67form.email.data,68form.phone_number.data,69form.country_code.data,70)71if authy_user.ok():72user = User(73form.username.data,74form.email.data,75form.password.data,76authy_user.id,77is_authenticated=True78)79user.authy_id = authy_user.id80db.session.add(user)81db.session.commit()82login_user(user, remember=True)83return flask.redirect('/protected')84else:85form.errors['non_field'] = []86for key, value in authy_user.errors().items():87form.errors['non_field'].append(88'{key}: {value}'.format(key=key, value=value)89)90return flask.render_template('register.html', form=form)919293@app.route('/2fa', methods=['GET', 'POST'])94@login_required95def twofa():96form = TokenVerificationForm(current_user.authy_id)97if form.validate_on_submit():98flask.session['authy'] = True99return flask.redirect('/protected')100return flask.render_template('2fa.html', form=form)101102103@app.route('/token/sms', methods=['POST'])104@login_required105def token_sms():106sms = authy_api.users.request_sms(current_user.authy_id, {'force': True})107if sms.ok():108return flask.Response('SMS request successful', status=200)109else:110return flask.Response('SMS request failed', status=503)111112113@app.route('/token/voice', methods=['POST'])114@login_required115def token_voice():116call = authy_api.users.request_call(current_user.authy_id, {'force': True})117if call.ok():118return flask.Response('Call request successful', status=200)119else:120return flask.Response('Call request failed', status=503)121122123@app.route('/token/onetouch', methods=['POST'])124@login_required125def token_onetouch():126details = {127'Authy ID': current_user.authy_id,128'Username': current_user.username,129'Reason': 'Demo by Account Security'130}131132hidden_details = {133'test': 'This is a'134}135136response = authy_api.one_touch.send_request(137int(current_user.authy_id),138message='Login requested for Account Security account.',139seconds_to_expire=120,140details=details,141hidden_details=hidden_details142)143if response.ok():144flask.session['onetouch_uuid'] = response.get_uuid()145return flask.Response('OneTouch request successfull', status=200)146else:147return flask.Response('OneTouch request failed', status=503)148149150@app.route('/onetouch-status', methods=['POST'])151@login_required152def onetouch_status():153uuid = flask.session['onetouch_uuid']154approval_status = authy_api.one_touch.get_approval_status(uuid)155if approval_status.ok():156if approval_status['approval_request']['status'] == 'approved':157flask.session['authy'] = True158return flask.Response(159approval_status['approval_request']['status'],160status=200161)162else:163return flask.Response(approval_status.errros(), status=503)164165166######################167# Phone Verification #168######################169170@app.route('/verification', methods=['GET', 'POST'])171def phone_verification():172form = PhoneVerificationForm()173if form.validate_on_submit():174flask.session['phone_number'] = form.phone_number.data175flask.session['country_code'] = form.country_code.data176authy_api.phones.verification_start(177form.phone_number.data,178form.country_code.data,179via=form.via.data180)181return flask.redirect('/verification/token')182return flask.render_template('phone_verification.html', form=form)183184185@app.route('/verification/token', methods=['GET', 'POST'])186def token_validation():187form = TokenPhoneValidationForm()188if form.validate_on_submit():189verification = authy_api.phones.verification_check(190flask.session['phone_number'],191flask.session['country_code'],192form.token.data193)194if verification.ok():195flask.session['is_verified'] = True196return flask.redirect('/verified')197else:198form.errors['non_field'] = []199for error_msg in verification.errors().values():200form.errors['non_field'].append(error_msg)201return flask.render_template('token_validation.html', form=form)202203204@app.route('/verified')205def verified():206if not flask.session.get('is_verified'):207return flask.redirect('/verification')208return flask.render_template('verified.html')209
And there you go, Two-factor Authentication is on and your Flask app is protected!
Now that you are keeping the hackers out of this demo app using Two-factor Authentication, you can find all of the detailed descriptions for options and API calls in our Two-factor Authentication API Reference. If you're also building a registration flow, also check out our Phone Verification product and the Verification Quickstart which uses this codebase.
For additional guides and tutorials on account security and other products, in Python and in our other languages, take a look at the Docs.