Java Servlets Quickstart for Twilio Authy Two-factor Authentication
Warning
As of November 2022, Twilio no longer provides support for Authy SMS/Voice-only customers. Customers who were also using Authy TOTP or Push prior to March 1, 2023 are still supported. The Authy API is now closed to new customers and will be fully deprecated in the future.
For new development, we encourage you to use the Verify v2 API.
Existing customers will not be impacted at this time until Authy API has reached End of Life. For more information about migration, see Migrating from Authy to Verify for SMS.
Adding two-factor authentication is an excellent way to reduce fraud and increase trust from your users. This quickstart guides you through building a Java Servlets and AngularJS application that restricts access to a URL. Four Authy API channels are demoed: SMS, Voice, Soft Tokens and Push Notifications.
Ready to protect a toy app from malicious hackers? Dive in!
Create a new Twilio account (you can sign up for a free Twilio trial), or sign into an existing Twilio account.
Once logged in, visit the Authy Console. Click on the red 'Create New Application' (or big red plus ('+') if you already created one) to create a new Authy application then name it something memorable.
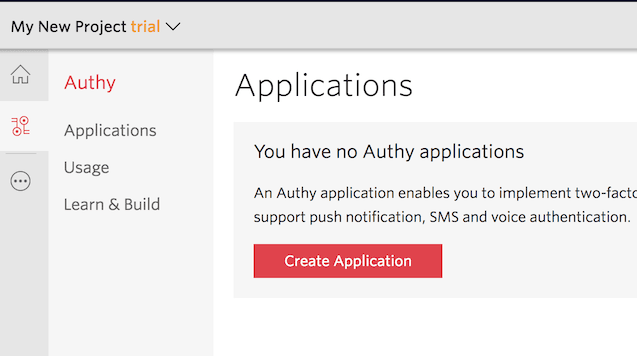
You'll automatically be transported to the Settings page next. Click the eyeball icon to reveal your Production API Key.
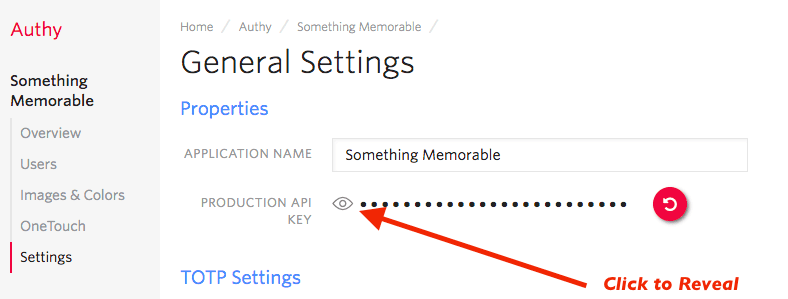
Copy your Production API Key to a safe place, you will use it during application setup.
This Two-factor Authentication demos two channels which require an installed Authy Client to test: Soft Tokens and Push Authentication. While SMS and Voice channels will work without the client, to try out all four authentication channels download and install Authy Client for Desktop or Mobile:
Clone our Java repository locally, then enter the directory. Install all of the necessary node modules:
gradle build
Next, open the file .env.example
. There, edit the ACCOUNT_SECURITY_API_KEY
, pasting in the API Key from the above step (in the console), and save the file as .env
before sourcing it.
In Windows, set the ACCOUNT_SECURITY_API_KEY
variable manually.
Enter the API Key from the Account Security console and optionally change the port.
1# You can get/create one here :2# https://www.twilio.com/console/authy/applications3ACCOUNT_SECURITY_API_KEY=ENTER_SECRET_HERE
Once you have added your API Key, you are ready to run! Launch the app with:
gradle appRun
You should get a message your new app is running!
With your phone (optionally with the Authy client installed) nearby, open a new browser tab and go to http://localhost:8080/register
Enter your information and invent a password, then hit 'Register'. Your information is passed to Twilio (you will be able to see your user immediately in the console), and the application is returned a user_id
.
Go to http://localhost:8080/login
and login. You'll be presented with a happy screen:
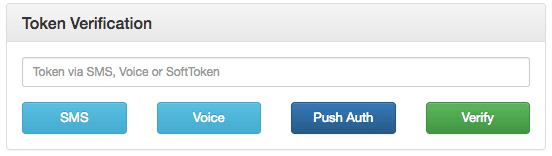
If your phone has the Authy Client installed, you can immediately enter a Soft Token from the client to Verify. Additionally, you can try a Push Notification by pushing the labeled button.
If you do not have the Authy Client installed, the SMS and Voice channels will also work in providing a token. To try different channels, you can logout to start the process again.
1package com.twilio.accountsecurity.services;23import com.authy.AuthyApiClient;4import com.authy.OneTouchException;5import com.authy.api.ApprovalRequestParams;6import com.authy.api.Hash;7import com.authy.api.OneTouchResponse;8import com.authy.api.Token;9import com.twilio.accountsecurity.exceptions.TokenVerificationException;10import com.twilio.accountsecurity.models.UserModel;11import com.twilio.accountsecurity.repository.UserRepository;12import org.slf4j.Logger;13import org.slf4j.LoggerFactory;1415import static com.twilio.accountsecurity.config.Settings.authyId;1617public class TokenService {1819private static final Logger LOGGER = LoggerFactory.getLogger(TokenService.class);2021private AuthyApiClient authyClient;22private UserRepository userRepository;2324public TokenService(AuthyApiClient authyClient, UserRepository userRepository) {25this.authyClient = authyClient;26this.userRepository = userRepository;27}2829public TokenService() {30this.authyClient = new AuthyApiClient(authyId());31this.userRepository = new UserRepository();32}3334public void sendSmsToken(String username) {35Hash hash = authyClient36.getUsers()37.requestSms(getUserAuthyId(username));3839if(!hash.isOk()) {40logAndThrow("Problem sending token over SMS");41}42}4344public void sendVoiceToken(String username) {45UserModel user = userRepository.findByUsername(username);4647Hash hash = authyClient.getUsers().requestCall(user.getAuthyId());48if(!hash.isOk()) {49logAndThrow("Problem sending the token on a call");50}51}5253public String sendOneTouchToken(String username) {54UserModel user = userRepository.findByUsername(username);5556try {57ApprovalRequestParams params = new ApprovalRequestParams58.Builder(user.getAuthyId(), "Login requested for Account Security account.")59.setSecondsToExpire(120L)60.addDetail("Authy ID", user.getAuthyId().toString())61.addDetail("Username", user.getUsername())62.addDetail("Location", "San Francisco, CA")63.addDetail("Reason", "Demo by Account Security")64.build();65OneTouchResponse response = authyClient66.getOneTouch()67.sendApprovalRequest(params);6869if(!response.isSuccess()) {70logAndThrow("Problem sending the token with OneTouch");71}72return response.getApprovalRequest().getUUID();73} catch (OneTouchException e) {74logAndThrow("Problem sending the token with OneTouch: " + e.getMessage());75}76return null;77}7879public void verify(String username, String token) {80Token verificationResult = authyClient81.getTokens()82.verify(getUserAuthyId(username), token);8384if(!verificationResult.isOk()) {85logAndThrow("Token verification failed");86}87}8889public String retrieveOneTouchStatus(String uuid) {90try {91return authyClient92.getOneTouch()93.getApprovalRequestStatus(uuid)94.getApprovalRequest()95.getStatus();96} catch (OneTouchException e) {97logAndThrow(e.getMessage());98return "";99}100}101102private void logAndThrow(String message) {103LOGGER.warn(message);104throw new TokenVerificationException(message);105}106107private Integer getUserAuthyId(String username) {108UserModel user = userRepository.findByUsername(username);109return user.getAuthyId();110}111}112
And there you go, Authy Two-factor Authentication is on and your Java app is protected!
Now that you are keeping the hackers out of this demo app using Twilio Authy Two-factor Authentication, you can find all of the detailed descriptions for options and API calls in our Two-factor Authentication API Reference. If you're also building a registration flow, also check out our Phone Verification product and the Verification Quickstart which uses this codebase.
For additional guides and tutorials on account security and other products, in Node.js and in our other languages, take a look at the Docs.